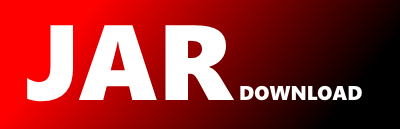
net.gdface.sdk.thrift.client.FaceApi Maven / Gradle / Ivy
The newest version!
package net.gdface.sdk.thrift.client;
import com.facebook.swift.codec.*;
import com.facebook.swift.codec.ThriftField.Requiredness;
import com.facebook.swift.service.*;
import com.google.common.util.concurrent.ListenableFuture;
import java.io.*;
import java.util.*;
@ThriftService("FaceApi")
public interface FaceApi
{
@ThriftService("FaceApi")
public interface Async
{
@ThriftMethod(value = "compare2Face",
exception = {
@ThriftException(type=ImageErrorException.class, id=1),
@ThriftException(type=NotFaceDetectedException.class, id=2),
@ThriftException(type=ServiceRuntimeException.class, id=3)
})
ListenableFuture compare2Face(
@ThriftField(value=1, name="imgData1", requiredness=Requiredness.OPTIONAL) final byte [] imgData1,
@ThriftField(value=2, name="facePos1", requiredness=Requiredness.OPTIONAL) final CodeInfo facePos1,
@ThriftField(value=3, name="imgData2", requiredness=Requiredness.OPTIONAL) final byte [] imgData2,
@ThriftField(value=4, name="facePos2", requiredness=Requiredness.OPTIONAL) final CodeInfo facePos2
);
@ThriftMethod(value = "compareCode",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
ListenableFuture compareCode(
@ThriftField(value=1, name="code1", requiredness=Requiredness.OPTIONAL) final byte [] code1,
@ThriftField(value=2, name="code2", requiredness=Requiredness.OPTIONAL) final byte [] code2
);
@ThriftMethod(value = "compareCodes",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
ListenableFuture> compareCodes(
@ThriftField(value=1, name="code1", requiredness=Requiredness.OPTIONAL) final byte [] code1,
@ThriftField(value=2, name="codes", requiredness=Requiredness.OPTIONAL) final List codes
);
@ThriftMethod(value = "compareFaces",
exception = {
@ThriftException(type=ImageErrorException.class, id=1),
@ThriftException(type=NotFaceDetectedException.class, id=2),
@ThriftException(type=ServiceRuntimeException.class, id=3)
})
ListenableFuture compareFaces(
@ThriftField(value=1, name="code", requiredness=Requiredness.OPTIONAL) final byte [] code,
@ThriftField(value=2, name="imgData", requiredness=Requiredness.OPTIONAL) final byte [] imgData,
@ThriftField(value=3, name="faceNum", requiredness=Requiredness.REQUIRED) final int faceNum
);
@ThriftMethod(value = "compareFeatures",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
ListenableFuture> compareFeatures(
@ThriftField(value=1, name="code1", requiredness=Requiredness.OPTIONAL) final byte [] code1,
@ThriftField(value=2, name="codes", requiredness=Requiredness.OPTIONAL) final List codes
);
@ThriftMethod(value = "detectAndCompare2Face",
exception = {
@ThriftException(type=ImageErrorException.class, id=1),
@ThriftException(type=NotFaceDetectedException.class, id=2),
@ThriftException(type=ServiceRuntimeException.class, id=3)
})
ListenableFuture detectAndCompare2Face(
@ThriftField(value=1, name="imgData1", requiredness=Requiredness.OPTIONAL) final byte [] imgData1,
@ThriftField(value=2, name="detectRect1", requiredness=Requiredness.OPTIONAL) final FRect detectRect1,
@ThriftField(value=3, name="imgData2", requiredness=Requiredness.OPTIONAL) final byte [] imgData2,
@ThriftField(value=4, name="detectRect2", requiredness=Requiredness.OPTIONAL) final FRect detectRect2
);
@ThriftMethod(value = "detectAndGetCodeInfo",
exception = {
@ThriftException(type=ImageErrorException.class, id=1),
@ThriftException(type=NotFaceDetectedException.class, id=2),
@ThriftException(type=ServiceRuntimeException.class, id=3)
})
ListenableFuture> detectAndGetCodeInfo(
@ThriftField(value=1, name="imgData", requiredness=Requiredness.OPTIONAL) final byte [] imgData,
@ThriftField(value=2, name="faceNum", requiredness=Requiredness.REQUIRED) final int faceNum
);
@ThriftMethod(value = "detectCenterFace",
exception = {
@ThriftException(type=ImageErrorException.class, id=1),
@ThriftException(type=NotFaceDetectedException.class, id=2),
@ThriftException(type=ServiceRuntimeException.class, id=3)
})
ListenableFuture detectCenterFace(
@ThriftField(value=1, name="imgData", requiredness=Requiredness.OPTIONAL) final byte [] imgData
);
@ThriftMethod(value = "detectFace",
exception = {
@ThriftException(type=ImageErrorException.class, id=1),
@ThriftException(type=ServiceRuntimeException.class, id=2)
})
ListenableFuture> detectFace(
@ThriftField(value=1, name="imgData", requiredness=Requiredness.OPTIONAL) final byte [] imgData
);
@ThriftMethod(value = "detectFaceWithOptions",
exception = {
@ThriftException(type=ImageErrorException.class, id=1),
@ThriftException(type=ServiceRuntimeException.class, id=2)
})
ListenableFuture> detectFaceWithOptions(
@ThriftField(value=1, name="imgData", requiredness=Requiredness.OPTIONAL) final byte [] imgData,
@ThriftField(value=2, name="jsonOptions", requiredness=Requiredness.OPTIONAL) final String jsonOptions
);
@ThriftMethod(value = "detectMaxFace",
exception = {
@ThriftException(type=ImageErrorException.class, id=1),
@ThriftException(type=NotFaceDetectedException.class, id=2),
@ThriftException(type=ServiceRuntimeException.class, id=3)
})
ListenableFuture detectMaxFace(
@ThriftField(value=1, name="imgData", requiredness=Requiredness.OPTIONAL) final byte [] imgData
);
@ThriftMethod(value = "detectMaxFaceAndGetCodeInfo",
exception = {
@ThriftException(type=ImageErrorException.class, id=1),
@ThriftException(type=NotFaceDetectedException.class, id=2),
@ThriftException(type=ServiceRuntimeException.class, id=3)
})
ListenableFuture detectMaxFaceAndGetCodeInfo(
@ThriftField(value=1, name="imgData", requiredness=Requiredness.OPTIONAL) final byte [] imgData
);
@ThriftMethod(value = "getCodeInfo",
exception = {
@ThriftException(type=NotFaceDetectedException.class, id=1),
@ThriftException(type=ServiceRuntimeException.class, id=2)
})
ListenableFuture> getCodeInfo(
@ThriftField(value=1, name="imgData", requiredness=Requiredness.OPTIONAL) final byte [] imgData,
@ThriftField(value=2, name="faceNum", requiredness=Requiredness.REQUIRED) final int faceNum,
@ThriftField(value=3, name="facePos", requiredness=Requiredness.OPTIONAL) final List facePos
);
@ThriftMethod(value = "getCodeInfoSingle",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
ListenableFuture getCodeInfoSingle(
@ThriftField(value=1, name="imgData", requiredness=Requiredness.OPTIONAL) final byte [] imgData,
@ThriftField(value=2, name="facePos", requiredness=Requiredness.OPTIONAL) final CodeInfo facePos
);
@ThriftMethod(value = "getFeature",
exception = {
@ThriftException(type=NotFaceDetectedException.class, id=1),
@ThriftException(type=ServiceRuntimeException.class, id=2)
})
ListenableFuture getFeature(
@ThriftField(value=1, name="faces", requiredness=Requiredness.OPTIONAL) final Map faces
);
@ThriftMethod(value = "hasFace",
exception = {
@ThriftException(type=ImageErrorException.class, id=1),
@ThriftException(type=ServiceRuntimeException.class, id=2)
})
ListenableFuture hasFace(
@ThriftField(value=1, name="imgData", requiredness=Requiredness.OPTIONAL) final byte [] imgData
);
@ThriftMethod(value = "isLocal",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
ListenableFuture isLocal();
@ThriftMethod(value = "matDetectAndGetCodeInfo",
exception = {
@ThriftException(type=NotFaceDetectedException.class, id=1),
@ThriftException(type=ServiceRuntimeException.class, id=2)
})
ListenableFuture> matDetectAndGetCodeInfo(
@ThriftField(value=1, name="matType", requiredness=Requiredness.OPTIONAL) final MatType matType,
@ThriftField(value=2, name="matData", requiredness=Requiredness.OPTIONAL) final byte [] matData,
@ThriftField(value=3, name="width", requiredness=Requiredness.REQUIRED) final int width,
@ThriftField(value=4, name="height", requiredness=Requiredness.REQUIRED) final int height,
@ThriftField(value=5, name="faceNum", requiredness=Requiredness.REQUIRED) final int faceNum
);
@ThriftMethod(value = "matDetectAndGetCodeInfoUnchecked",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
ListenableFuture> matDetectAndGetCodeInfoUnchecked(
@ThriftField(value=1, name="matType", requiredness=Requiredness.OPTIONAL) final MatType matType,
@ThriftField(value=2, name="matData", requiredness=Requiredness.OPTIONAL) final byte [] matData,
@ThriftField(value=3, name="width", requiredness=Requiredness.REQUIRED) final int width,
@ThriftField(value=4, name="height", requiredness=Requiredness.REQUIRED) final int height
);
@ThriftMethod(value = "matDetectFace",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
ListenableFuture> matDetectFace(
@ThriftField(value=1, name="matType", requiredness=Requiredness.OPTIONAL) final MatType matType,
@ThriftField(value=2, name="matData", requiredness=Requiredness.OPTIONAL) final byte [] matData,
@ThriftField(value=3, name="width", requiredness=Requiredness.REQUIRED) final int width,
@ThriftField(value=4, name="height", requiredness=Requiredness.REQUIRED) final int height
);
@ThriftMethod(value = "matDetectFaceFacenum",
exception = {
@ThriftException(type=NotFaceDetectedException.class, id=1),
@ThriftException(type=ServiceRuntimeException.class, id=2)
})
ListenableFuture> matDetectFaceFacenum(
@ThriftField(value=1, name="matType", requiredness=Requiredness.OPTIONAL) final MatType matType,
@ThriftField(value=2, name="matData", requiredness=Requiredness.OPTIONAL) final byte [] matData,
@ThriftField(value=3, name="width", requiredness=Requiredness.REQUIRED) final int width,
@ThriftField(value=4, name="height", requiredness=Requiredness.REQUIRED) final int height,
@ThriftField(value=5, name="facenum", requiredness=Requiredness.REQUIRED) final int facenum
);
@ThriftMethod(value = "matDetectMaxFace",
exception = {
@ThriftException(type=NotFaceDetectedException.class, id=1),
@ThriftException(type=ServiceRuntimeException.class, id=2)
})
ListenableFuture matDetectMaxFace(
@ThriftField(value=1, name="matType", requiredness=Requiredness.OPTIONAL) final MatType matType,
@ThriftField(value=2, name="matData", requiredness=Requiredness.OPTIONAL) final byte [] matData,
@ThriftField(value=3, name="width", requiredness=Requiredness.REQUIRED) final int width,
@ThriftField(value=4, name="height", requiredness=Requiredness.REQUIRED) final int height
);
@ThriftMethod(value = "matDetectMaxFaceAndGetCodeInfo",
exception = {
@ThriftException(type=NotFaceDetectedException.class, id=1),
@ThriftException(type=ServiceRuntimeException.class, id=2)
})
ListenableFuture matDetectMaxFaceAndGetCodeInfo(
@ThriftField(value=1, name="matType", requiredness=Requiredness.OPTIONAL) final MatType matType,
@ThriftField(value=2, name="matData", requiredness=Requiredness.OPTIONAL) final byte [] matData,
@ThriftField(value=3, name="width", requiredness=Requiredness.REQUIRED) final int width,
@ThriftField(value=4, name="height", requiredness=Requiredness.REQUIRED) final int height
);
@ThriftMethod(value = "matGetCodeInfo",
exception = {
@ThriftException(type=NotFaceDetectedException.class, id=1),
@ThriftException(type=ServiceRuntimeException.class, id=2)
})
ListenableFuture> matGetCodeInfo(
@ThriftField(value=1, name="matType", requiredness=Requiredness.OPTIONAL) final MatType matType,
@ThriftField(value=2, name="matData", requiredness=Requiredness.OPTIONAL) final byte [] matData,
@ThriftField(value=3, name="width", requiredness=Requiredness.REQUIRED) final int width,
@ThriftField(value=4, name="height", requiredness=Requiredness.REQUIRED) final int height,
@ThriftField(value=5, name="facenum", requiredness=Requiredness.REQUIRED) final int facenum,
@ThriftField(value=6, name="facePos", requiredness=Requiredness.OPTIONAL) final List facePos
);
@ThriftMethod(value = "matGetCodeInfoSingle",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
ListenableFuture matGetCodeInfoSingle(
@ThriftField(value=1, name="matType", requiredness=Requiredness.OPTIONAL) final MatType matType,
@ThriftField(value=2, name="matData", requiredness=Requiredness.OPTIONAL) final byte [] matData,
@ThriftField(value=3, name="width", requiredness=Requiredness.REQUIRED) final int width,
@ThriftField(value=4, name="height", requiredness=Requiredness.REQUIRED) final int height,
@ThriftField(value=5, name="facePos", requiredness=Requiredness.OPTIONAL) final CodeInfo facePos
);
@ThriftMethod(value = "matHasFace",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
ListenableFuture matHasFace(
@ThriftField(value=1, name="matType", requiredness=Requiredness.OPTIONAL) final MatType matType,
@ThriftField(value=2, name="matData", requiredness=Requiredness.OPTIONAL) final byte [] matData,
@ThriftField(value=3, name="width", requiredness=Requiredness.REQUIRED) final int width,
@ThriftField(value=4, name="height", requiredness=Requiredness.REQUIRED) final int height
);
@ThriftMethod(value = "matSearchFaces",
exception = {
@ThriftException(type=ImageErrorException.class, id=1),
@ThriftException(type=NotFaceDetectedException.class, id=2),
@ThriftException(type=ServiceRuntimeException.class, id=3)
})
ListenableFuture> matSearchFaces(
@ThriftField(value=1, name="matType", requiredness=Requiredness.OPTIONAL) final MatType matType,
@ThriftField(value=2, name="matData", requiredness=Requiredness.OPTIONAL) final byte [] matData,
@ThriftField(value=3, name="width", requiredness=Requiredness.REQUIRED) final int width,
@ThriftField(value=4, name="height", requiredness=Requiredness.REQUIRED) final int height,
@ThriftField(value=5, name="facePos", requiredness=Requiredness.OPTIONAL) final CodeInfo facePos,
@ThriftField(value=6, name="similarty", requiredness=Requiredness.REQUIRED) final double similarty,
@ThriftField(value=7, name="rows", requiredness=Requiredness.REQUIRED) final int rows,
@ThriftField(value=8, name="imgMD5Set", requiredness=Requiredness.OPTIONAL) final List imgMD5Set,
@ThriftField(value=9, name="group", requiredness=Requiredness.REQUIRED) final int group
);
@ThriftMethod(value = "matWearMask",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
ListenableFuture matWearMask(
@ThriftField(value=1, name="matType", requiredness=Requiredness.OPTIONAL) final MatType matType,
@ThriftField(value=2, name="matData", requiredness=Requiredness.OPTIONAL) final byte [] matData,
@ThriftField(value=3, name="width", requiredness=Requiredness.REQUIRED) final int width,
@ThriftField(value=4, name="height", requiredness=Requiredness.REQUIRED) final int height,
@ThriftField(value=5, name="faceInfo", requiredness=Requiredness.OPTIONAL) final CodeInfo faceInfo
);
@ThriftMethod(value = "sdkCapacity",
exception = {
@ThriftException(type=ServiceRuntimeException.class, id=1)
})
ListenableFuture
© 2015 - 2025 Weber Informatics LLC | Privacy Policy