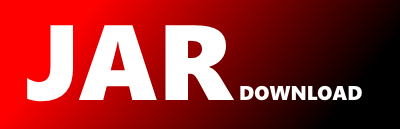
net.gdface.sdk.thrift.FaceApiThriftClientAsync Maven / Gradle / Ivy
package net.gdface.sdk.thrift;
import java.nio.ByteBuffer;
import java.util.List;
import java.util.Map;
import net.gdface.sdk.CodeInfo;
import net.gdface.sdk.FRect;
import net.gdface.sdk.FaceApi;
import net.gdface.thrift.TypeTransformer;
import com.google.common.base.Function;
import com.google.common.util.concurrent.FutureCallback;
import com.google.common.util.concurrent.Futures;
import com.google.common.util.concurrent.ListenableFuture;
import net.gdface.thrift.ClientFactory;
import static com.google.common.base.Preconditions.*;
/**
* 基于thrift/swift框架生成的client端代码提供{@link FaceApi}接口的异步RPC实现(线程安全)
* 转发所有{@link FaceApi}接口方法到{@link #delegate()}指定的实例
* 所有服务端抛出的{@link RuntimeException}异常被封装到{@link ServiceRuntimeException}中抛出
* Example:
*
* FaceApiThriftClientAsync thriftInstance = ClientFactory
* .builder()
* .setHostAndPort("127.0.0.1",26413)
* .build(FaceApi.class, FaceApiThriftClientAsync.class);
*
* 计算机生成代码(generated by automated tools ThriftServiceDecoratorGenerator @author guyadong)
* @author guyadong
*
*/
public class FaceApiThriftClientAsync {
private final ClientFactory factory;
public FaceApiThriftClientAsync(ClientFactory factory) {
super();
this.factory = checkNotNull(factory,"factory is null");
}
/**
* 返回{@link net.gdface.sdk.thrift.client.FaceApi.Async}实例
* @return
*/
protected net.gdface.sdk.thrift.client.FaceApi.Async delegate() {
return factory.applyInstance(net.gdface.sdk.thrift.client.FaceApi.Async.class);
}
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
builder.append("FaceApiThriftClientAsync [factory=");
builder.append(factory);
builder.append(",interface=");
builder.append(FaceApi.class.getName());
builder.append("]");
return builder.toString();
}
/**
* 默认的{@link FutureCallback}实现
* @author guyadong
*
* @param
*/
public static class DefaultCallback implements FutureCallback{
@Override
public void onSuccess(V result) {
// DO NOTHING
}
@Override
public void onFailure(Throwable t) {
try{
throw t;
}
catch(net.gdface.sdk.thrift.client.ImageErrorException e){
onImageErrorException(e);
}
catch(net.gdface.sdk.thrift.client.NotFaceDetectedException e){
onNotFaceDetectedException(e);
}
catch(net.gdface.sdk.thrift.client.ServiceRuntimeException e){
onServiceRuntimeException(e);
}
catch(Throwable e){
onThrowable(e);
}
}
protected void onImageErrorException(net.gdface.sdk.thrift.client.ImageErrorException e){
System.out.println(e.getServiceStackTraceMessage());
}
protected void onNotFaceDetectedException(net.gdface.sdk.thrift.client.NotFaceDetectedException e){
System.out.println(e.getServiceStackTraceMessage());
}
protected void onServiceRuntimeException(net.gdface.sdk.thrift.client.ServiceRuntimeException e){
System.out.println(e.getServiceStackTraceMessage());
}
protected void onThrowable(Throwable e){
e.printStackTrace();
}
}
/**
* see also {@link net.gdface.sdk.FaceApi#compare2Face(byte[],net.gdface.sdk.CodeInfo,byte[],net.gdface.sdk.CodeInfo)}
*/
public ListenableFuture compare2Face(byte[] imgData1,
CodeInfo facePos1,
byte[] imgData2,
CodeInfo facePos2){
net.gdface.sdk.thrift.client.FaceApi.Async async = delegate();
ListenableFuture future = async.compare2Face(imgData1,
TypeTransformer.getInstance().to(
facePos1,
CodeInfo.class,
net.gdface.sdk.thrift.client.CodeInfo.class),
imgData2,
TypeTransformer.getInstance().to(
facePos2,
CodeInfo.class,
net.gdface.sdk.thrift.client.CodeInfo.class));
return factory.wrap(async,future);
}
public void compare2Face(byte[] imgData1,
CodeInfo facePos1,
byte[] imgData2,
CodeInfo facePos2,
FutureCallbackcallback){
factory.addCallback(compare2Face(imgData1,facePos1,imgData2,facePos2), callback);
}
/**
* see also {@link net.gdface.sdk.FaceApi#compareCode(byte[],byte[])}
*/
public ListenableFuture compareCode(byte[] code1,
byte[] code2){
net.gdface.sdk.thrift.client.FaceApi.Async async = delegate();
ListenableFuture future = async.compareCode(code1,
code2);
return factory.wrap(async,future);
}
public void compareCode(byte[] code1,
byte[] code2,
FutureCallbackcallback){
factory.addCallback(compareCode(code1,code2), callback);
}
/**
* see also {@link net.gdface.sdk.FaceApi#compareCodes(byte[],net.gdface.sdk.CodeInfo[])}
*/
public ListenableFuture compareCodes(byte[] code1,
CodeInfo[] codes){
net.gdface.sdk.thrift.client.FaceApi.Async async = delegate();
ListenableFuture future = Futures.transform(
async.compareCodes(code1,
TypeTransformer.getInstance().to(
codes,
CodeInfo.class,
net.gdface.sdk.thrift.client.CodeInfo.class)),
new Function,double[]>(){
@Override
public double[] apply(java.util.List input) {
return TypeTransformer.getInstance().todoubleArray(
input,
double.class,
double.class);
}
});
return factory.wrap(async,future);
}
public void compareCodes(byte[] code1,
CodeInfo[] codes,
FutureCallbackcallback){
factory.addCallback(compareCodes(code1,codes), callback);
}
/**
* see also {@link net.gdface.sdk.FaceApi#compareFaces(byte[],byte[],int,net.gdface.sdk.FRect)}
*/
public ListenableFuture
© 2015 - 2024 Weber Informatics LLC | Privacy Policy