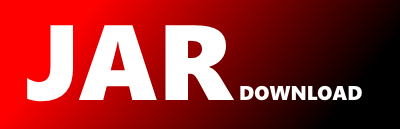
net.gdface.facedb.thrift.FaceDbThriftClient Maven / Gradle / Ivy
The newest version!
// ______________________________________________________
// Generated by codegen - https://gitee.com/l0km/codegen
// template: thrift/client/perservice/client.service.decorator.class.vm
// ______________________________________________________
package net.gdface.facedb.thrift;
import com.gitee.l0km.com4j.base.exception.NotFoundBeanException;
import com.gitee.l0km.ximage.ImageErrorException;
import com.gitee.l0km.ximage.MatType;
import java.nio.ByteBuffer;
import java.util.Date;
import java.util.List;
import java.util.Map;
import net.gdface.facedb.DuplicateRecordException;
import net.gdface.facedb.FaceDb;
import net.gdface.facedb.SearchResult;
import net.gdface.facedb.db.FaceBean;
import net.gdface.facedb.db.FeatureBean;
import net.gdface.facedb.db.ImageBean;
import net.gdface.sdk.CodeInfo;
import net.gdface.sdk.CompareResult;
import net.gdface.sdk.NotFaceDetectedException;
import com.gitee.l0km.xthrift.thrift.ClientFactory;
import com.gitee.l0km.xthrift.thrift.TypeTransformer;
import com.facebook.swift.service.RuntimeTApplicationException;
import com.google.common.net.HostAndPort;
import static com.google.common.base.Preconditions.*;
/**
* 基于thrift/swift框架生成的client端代码提供{@link FaceDb}接口的RPC实现(线程安全)
* 转发所有{@link FaceDb}接口方法到{@link #delegate()}指定的实例
* 所有服务端抛出的{@link RuntimeException}异常被封装到{@link ServiceRuntimeException}中抛出
* Example:
*
* FaceDbThriftClient thriftInstance = ClientFactory
* .builder()
* .setHostAndPort("127.0.0.1",26413)
* .build(FaceDb.class, FaceDbThriftClient.class);
*
* 计算机生成代码(generated by automated tools ThriftServiceDecoratorGenerator @author guyadong)
* @author guyadong
*
*/
public class FaceDbThriftClient implements FaceDb {
private final ClientFactory factory;
public ClientFactory getFactory() {
return factory;
}
public FaceDbThriftClient(ClientFactory factory) {
super();
this.factory = checkNotNull(factory,"factory is null");
}
/**
* @param host RPC service host
* @param port RPC service port
*/
public FaceDbThriftClient(String host,int port) {
this(ClientFactory.builder().setHostAndPort(host,port));
}
/**
* @param hostAndPort RPC service host and port
*/
public FaceDbThriftClient(HostAndPort hostAndPort) {
this(ClientFactory.builder().setHostAndPort(hostAndPort));
}
/**
* test if connectable for RPC service
* @return return {@code true} if connectable ,otherwise {@code false}
*/
public boolean testConnect(){
return factory.testConnect();
}
/**
* @return 返回{@link net.gdface.facedb.thrift.client.FaceDb}实例
*/
protected net.gdface.facedb.thrift.client.FaceDb delegate() {
return factory.applyInstance(net.gdface.facedb.thrift.client.FaceDb.class);
}
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
builder.append("FaceDbThriftClient [factory=");
builder.append(factory);
builder.append(",interface=");
builder.append(FaceDb.class.getName());
builder.append("]");
return builder.toString();
}
@Override
public FeatureBean addFeature(byte[] feature,
Map faces)
throws DuplicateRecordException{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.addFeature(feature,
TypeTransformer.getInstance().to(
faces,
ByteBuffer.class,
CodeInfo.class,
byte[].class,
net.gdface.facedb.thrift.client.CodeInfo.class)),
net.gdface.facedb.thrift.client.FeatureBean.class,
FeatureBean.class);
}
catch(net.gdface.facedb.thrift.client.DuplicateRecordException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.DuplicateRecordException.class,
DuplicateRecordException.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public ImageBean addImage(byte[] imgData,
List features)
throws DuplicateRecordException{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.addImage(imgData,
TypeTransformer.getInstance().to(
features,
CodeInfo.class,
net.gdface.facedb.thrift.client.CodeInfo.class)),
net.gdface.facedb.thrift.client.ImageBean.class,
ImageBean.class);
}
catch(net.gdface.facedb.thrift.client.DuplicateRecordException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.DuplicateRecordException.class,
DuplicateRecordException.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public ImageBean addImageIfAbsent(byte[] imgData,
CodeInfo code,
double similarty)
throws ImageErrorException,NotFaceDetectedException{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.addImageIfAbsent(imgData,
TypeTransformer.getInstance().to(
code,
CodeInfo.class,
net.gdface.facedb.thrift.client.CodeInfo.class),
similarty),
net.gdface.facedb.thrift.client.ImageBean.class,
ImageBean.class);
}
catch(net.gdface.facedb.thrift.client.ImageErrorException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.ImageErrorException.class,
ImageErrorException.class);
}
catch(net.gdface.facedb.thrift.client.NotFaceDetectedException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.NotFaceDetectedException.class,
NotFaceDetectedException.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public double[] compareFaces(String featureId,
byte[] imgData,
CodeInfo[] facePos)
throws NotFoundBeanException,NotFaceDetectedException{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().todoubleArray(
instance.compareFaces(featureId,
imgData,
TypeTransformer.getInstance().to(
facePos,
CodeInfo.class,
net.gdface.facedb.thrift.client.CodeInfo.class)),
double.class,
double.class);
}
catch(net.gdface.facedb.thrift.client.NotFoundBeanException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.NotFoundBeanException.class,
NotFoundBeanException.class);
}
catch(net.gdface.facedb.thrift.client.NotFaceDetectedException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.NotFaceDetectedException.class,
NotFaceDetectedException.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public double compareFeature(String featureId,
byte[] feature)
throws NotFoundBeanException{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return instance.compareFeature(featureId,
feature);
}
catch(net.gdface.facedb.thrift.client.NotFoundBeanException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.NotFoundBeanException.class,
NotFoundBeanException.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public double compareFeatureId(String featureId1,
String featureId2)
throws NotFoundBeanException{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return instance.compareFeatureId(featureId1,
featureId2);
}
catch(net.gdface.facedb.thrift.client.NotFoundBeanException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.NotFoundBeanException.class,
NotFoundBeanException.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public double[] compareFeatures(String featureId,
CodeInfo[] features)
throws NotFoundBeanException{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().todoubleArray(
instance.compareFeatures(featureId,
TypeTransformer.getInstance().to(
features,
CodeInfo.class,
net.gdface.facedb.thrift.client.CodeInfo.class)),
double.class,
double.class);
}
catch(net.gdface.facedb.thrift.client.NotFoundBeanException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.NotFoundBeanException.class,
NotFoundBeanException.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public Map dbCapacity()
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.dbCapacity(),
String.class,
String.class,
String.class,
String.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public boolean deleteFeature(String featureId,
boolean cascade)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return instance.deleteFeature(featureId,
cascade);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int deleteFeatures(List featureIdList,
boolean cascade)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return instance.deleteFeatures(featureIdList,
cascade);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public boolean deleteImage(String imgMd5,
boolean cascade)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return instance.deleteImage(imgMd5,
cascade);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int deleteImages(List imgMd5List,
boolean cascade)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return instance.deleteImages(imgMd5List,
cascade);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public ImageBean detectAndAddFeatures(byte[] imgData,
int faceNum)
throws ImageErrorException,DuplicateRecordException,NotFaceDetectedException{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.detectAndAddFeatures(imgData,
faceNum),
net.gdface.facedb.thrift.client.ImageBean.class,
ImageBean.class);
}
catch(net.gdface.facedb.thrift.client.ImageErrorException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.ImageErrorException.class,
ImageErrorException.class);
}
catch(net.gdface.facedb.thrift.client.DuplicateRecordException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.DuplicateRecordException.class,
DuplicateRecordException.class);
}
catch(net.gdface.facedb.thrift.client.NotFaceDetectedException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.NotFaceDetectedException.class,
NotFaceDetectedException.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public CompareResult detectAndCompareFaces(String featureId,
byte[] imgData,
int faceNum)
throws NotFoundBeanException,ImageErrorException,NotFaceDetectedException{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.detectAndCompareFaces(featureId,
imgData,
faceNum),
net.gdface.facedb.thrift.client.CompareResult.class,
CompareResult.class);
}
catch(net.gdface.facedb.thrift.client.NotFoundBeanException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.NotFoundBeanException.class,
NotFoundBeanException.class);
}
catch(net.gdface.facedb.thrift.client.ImageErrorException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.ImageErrorException.class,
ImageErrorException.class);
}
catch(net.gdface.facedb.thrift.client.NotFaceDetectedException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.NotFaceDetectedException.class,
NotFaceDetectedException.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public CodeInfo[] detectAndGetCodeInfo(byte[] imgData)
throws ImageErrorException{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().toArray(
instance.detectAndGetCodeInfo(imgData),
net.gdface.facedb.thrift.client.CodeInfo.class,
CodeInfo.class);
}
catch(net.gdface.facedb.thrift.client.ImageErrorException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.ImageErrorException.class,
ImageErrorException.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public CodeInfo[] detectAndGetCodeInfo(MatType matType,
byte[] matData,
int width,
int height)
throws ImageErrorException{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().toArray(
instance.detectAndGetCodeInfoMat(TypeTransformer.getInstance().to(
matType,
MatType.class,
net.gdface.facedb.thrift.client.MatType.class),
matData,
width,
height),
net.gdface.facedb.thrift.client.CodeInfo.class,
CodeInfo.class);
}
catch(net.gdface.facedb.thrift.client.ImageErrorException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.ImageErrorException.class,
ImageErrorException.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public SearchResult[] detectAndSearchFaces(byte[] imgData,
double similarty,
int rows,
String where)
throws ImageErrorException,NotFaceDetectedException{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().toArray(
instance.detectAndSearchFaces(imgData,
similarty,
rows,
where),
net.gdface.facedb.thrift.client.SearchResult.class,
SearchResult.class);
}
catch(net.gdface.facedb.thrift.client.ImageErrorException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.ImageErrorException.class,
ImageErrorException.class);
}
catch(net.gdface.facedb.thrift.client.NotFaceDetectedException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.NotFaceDetectedException.class,
NotFaceDetectedException.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public CodeInfo getCodeInfo(int faceId)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getCodeInfo(faceId),
net.gdface.facedb.thrift.client.CodeInfo.class,
CodeInfo.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public CodeInfo getCodeInfoByFeatureId(String featureId)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getCodeInfoByFeatureId(featureId),
net.gdface.facedb.thrift.client.CodeInfo.class,
CodeInfo.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public CodeInfo getCodeInfoByImageMd5(String imageMd5)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getCodeInfoByImageMd5(imageMd5),
net.gdface.facedb.thrift.client.CodeInfo.class,
CodeInfo.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getCodeInfosByFeatureId(String featureId)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getCodeInfosByFeatureId(featureId),
net.gdface.facedb.thrift.client.CodeInfo.class,
CodeInfo.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getCodeInfosByImageMd5(String imageMd5)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getCodeInfosByImageMd5(imageMd5),
net.gdface.facedb.thrift.client.CodeInfo.class,
CodeInfo.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public FaceBean getFace(int faceId)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getFace(faceId),
net.gdface.facedb.thrift.client.FaceBean.class,
FaceBean.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public FaceBean getFaceByFeatureId(String featureId)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getFaceByFeatureId(featureId),
net.gdface.facedb.thrift.client.FaceBean.class,
FaceBean.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public FaceBean getFaceByImageMd5(String imageMd5)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getFaceByImageMd5(imageMd5),
net.gdface.facedb.thrift.client.FaceBean.class,
FaceBean.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int getFaceCount(String where)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return instance.getFaceCount(where);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getFacesByFeatureId(String featureId)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getFacesByFeatureId(featureId),
net.gdface.facedb.thrift.client.FaceBean.class,
FaceBean.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getFacesByImageMd5(String imageMd5)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getFacesByImageMd5(imageMd5),
net.gdface.facedb.thrift.client.FaceBean.class,
FaceBean.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public FeatureBean getFeature(String featureId)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getFeature(featureId),
net.gdface.facedb.thrift.client.FeatureBean.class,
FeatureBean.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public FeatureBean getFeatureByFaceId(int faceId)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getFeatureByFaceId(faceId),
net.gdface.facedb.thrift.client.FeatureBean.class,
FeatureBean.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public FeatureBean getFeatureByImageMd5(String imageMd5)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getFeatureByImageMd5(imageMd5),
net.gdface.facedb.thrift.client.FeatureBean.class,
FeatureBean.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int getFeatureCount()
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return instance.getFeatureCount();
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getFeaturesByImageMd5(String imageMd5)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getFeaturesByImageMd5(imageMd5),
net.gdface.facedb.thrift.client.FeatureBean.class,
FeatureBean.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public ImageBean getImage(String imageMd5)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getImage(imageMd5),
net.gdface.facedb.thrift.client.ImageBean.class,
ImageBean.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public ImageBean getImage(String primaryKey,
String refType)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getImageRef(primaryKey,
refType),
net.gdface.facedb.thrift.client.ImageBean.class,
ImageBean.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public ImageBean getImageByFaceId(int faceId)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getImageByFaceId(faceId),
net.gdface.facedb.thrift.client.ImageBean.class,
ImageBean.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public ImageBean getImageByFeatureId(String featureId)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getImageByFeatureId(featureId),
net.gdface.facedb.thrift.client.ImageBean.class,
ImageBean.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public byte[] getImageBytes(String imageMd5)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return instance.getImageBytes(imageMd5);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public byte[] getImageBytes(String primaryKey,
String refType)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return instance.getImageBytesRef(primaryKey,
refType);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int getImageCount(String where)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return instance.getImageCount(where);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getImagesByFeatureId(String featureId)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getImagesByFeatureId(featureId),
net.gdface.facedb.thrift.client.ImageBean.class,
ImageBean.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public boolean hasFeature(byte[] feature)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return instance.hasFeature(feature);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public boolean hasFeatureByMD5(String featureId)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return instance.hasFeatureByMD5(featureId);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public boolean hasImage(String imageMd5)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return instance.hasImage(imageMd5);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public boolean isLocal(){
return false;
}
@Override
public List loadFeaturesMd5ByCreateTime(String timestamp)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return instance.loadFeaturesMd5ByCreateTimeTimeStr(timestamp);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadFeaturesMd5ByCreateTime(Date timestamp)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return instance.loadFeaturesMd5ByCreateTime(TypeTransformer.getInstance().to(
timestamp,
Date.class,
Long.class));
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadFeaturesMd5ByWhere(String where)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return instance.loadFeaturesMd5ByWhere(where);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadImagesByWhere(String where,
int startRow,
int numRows)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.loadImagesByWhere(where,
startRow,
numRows),
net.gdface.facedb.thrift.client.ImageBean.class,
ImageBean.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadImagesMd5ByCreateTime(String timestamp)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return instance.loadImagesMd5ByCreateTimeTimeStr(timestamp);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadImagesMd5ByCreateTime(Date timestamp)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return instance.loadImagesMd5ByCreateTime(TypeTransformer.getInstance().to(
timestamp,
Date.class,
Long.class));
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadImagesMd5ByWhere(String where)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return instance.loadImagesMd5ByWhere(where);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public SearchResult[] searchFaces(byte[] imgData,
CodeInfo facePos,
double similarty,
int rows,
String where)
throws ImageErrorException,NotFaceDetectedException{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().toArray(
instance.searchFaces(imgData,
TypeTransformer.getInstance().to(
facePos,
CodeInfo.class,
net.gdface.facedb.thrift.client.CodeInfo.class),
similarty,
rows,
where),
net.gdface.facedb.thrift.client.SearchResult.class,
SearchResult.class);
}
catch(net.gdface.facedb.thrift.client.ImageErrorException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.ImageErrorException.class,
ImageErrorException.class);
}
catch(net.gdface.facedb.thrift.client.NotFaceDetectedException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.NotFaceDetectedException.class,
NotFaceDetectedException.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public SearchResult[] searchFaces(MatType matType,
byte[] matData,
int width,
int height,
CodeInfo facePos,
double similarty,
int rows,
String where)
throws NotFaceDetectedException{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().toArray(
instance.searchFacesMat(TypeTransformer.getInstance().to(
matType,
MatType.class,
net.gdface.facedb.thrift.client.MatType.class),
matData,
width,
height,
TypeTransformer.getInstance().to(
facePos,
CodeInfo.class,
net.gdface.facedb.thrift.client.CodeInfo.class),
similarty,
rows,
where),
net.gdface.facedb.thrift.client.SearchResult.class,
SearchResult.class);
}
catch(net.gdface.facedb.thrift.client.NotFaceDetectedException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.NotFaceDetectedException.class,
NotFaceDetectedException.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public SearchResult[] searchFeatures(byte[] feature,
double similarty,
int rows,
String where)
{
net.gdface.facedb.thrift.client.FaceDb instance = delegate();
try{
return TypeTransformer.getInstance().toArray(
instance.searchFeatures(feature,
similarty,
rows,
where),
net.gdface.facedb.thrift.client.SearchResult.class,
SearchResult.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy