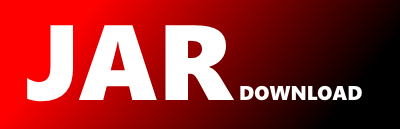
net.gdface.facedb.dborm.Constant Maven / Gradle / Ivy
// ______________________________________________________
// Generated by sql2java - https://github.com/10km/sql2java-2-6-7 (custom branch)
// modified by guyadong from
// sql2java original version https://sourceforge.net/projects/sql2java/
// JDBC driver used at code generation time: com.mysql.jdbc.Driver
// template: constant.java.vm
// ______________________________________________________
package net.gdface.facedb.dborm;
/**
* constant declare
* @author guyadong
*/
public interface Constant {
public static final int LONG_BIT_NUM = 64;
public static final String SQL_LIKE_WILDCARD = "%";
/** set =QUERY for loadUsingTemplate */
public static final int SEARCH_EXACT = 0;
/** set %QUERY% for loadLikeTemplate */
public static final int SEARCH_LIKE = 1;
/** set %QUERY for loadLikeTemplate */
public static final int SEARCH_STARTING_LIKE = 2;
/** set QUERY% for loadLikeTemplate */
public static final int SEARCH_ENDING_LIKE = 3;
/** JDBC property name definition */
public static enum JdbcProperty{
/** debug status */DEBUG("isDebug"),
/** JDBC driver class name */JDBC_DRIVER("jdbc.driver"),
/** JDBC connection url */JDBC_URL("jdbc.url"),
/** JDBC user name */JDBC_USERNAME("jdbc.username"),
/** JDBC password */JDBC_PASSWORD("jdbc.password"),
/** data source type, c3p0 supported only now */DATASOURCE("datasource"),
/** c3p0 property */C3P0_MINPOOLSIZE("c3p0.minPoolSize"),
/** c3p0 property */C3P0_MAXPOOLSIZE("c3p0.maxPoolSize"),
/** c3p0 property */C3P0_MAXIDLETIME("c3p0.maxIdleTime"),
/** c3p0 property */C3P0_IDLECONNECTIONTESTPERIOD("c3p0.idleConnectionTestPeriod");
/** JDBC property name */
public final String key;
JdbcProperty(String key){
this.key = key;
}
/** return {@link #key} with {@code prefix} */
public String withPrefix(String prefix){
return new StringBuffer().append(prefix).append(key).toString();
}
/**
* cast key to {@link JdbcProperty} instance if {@link #key} field equal the argument {@code key},
* otherwise return {@code null}
*/
public static final JdbcProperty fromKey(String key){
for(JdbcProperty p: values()){
if(p.key.equals(key)){
return p;
}
}
return null;
}
}
//////////////////////////////////////
// COLUMN COLUMN CONSTANT
//////////////////////////////////////
public static final int FD_FACE_COLUMN_COUNT = 21;
public static final int FD_FACE_PK_COUNT = 1;
public static final int FD_FEATURE_COLUMN_COUNT = 3;
public static final int FD_FEATURE_PK_COUNT = 1;
public static final int FD_IMAGE_COLUMN_COUNT = 8;
public static final int FD_IMAGE_PK_COUNT = 1;
public static final int FD_STORE_COLUMN_COUNT = 3;
public static final int FD_STORE_PK_COUNT = 1;
//////////////////////////////////////
// FOREIGN KEY INDEX DECLARE
//////////////////////////////////////
/** foreign key fd_face(feature_md5) -> fd_feature */
public static final int FD_FACE_FK_FEATURE_MD5 = 0;
/** foreign key fd_face(image_md5) -> fd_image */
public static final int FD_FACE_FK_IMAGE_MD5 = 1;
//////////////////////////////////////
// IMPORTED KEY INDEX DECLARE
//////////////////////////////////////
/** imported key fd_face(feature_md5) -> fd_feature */
public static final int FD_FEATURE_IK_FD_FACE_FEATURE_MD5 = 0;
/** imported key fd_face(image_md5) -> fd_image */
public static final int FD_IMAGE_IK_FD_FACE_IMAGE_MD5 = 0;
//////////////////////////////////////
// INDEX INDEX DECLARE
//////////////////////////////////////
/** fd_face index (feature_md5) */
public static final int FD_FACE_INDEX_FEATURE_MD5 = 0;
/** fd_face index (image_md5) */
public static final int FD_FACE_INDEX_IMAGE_MD5 = 1;
//////////////////////////////////////
// COLUMN ID DECLARE
//////////////////////////////////////
/** Identify the fd_face.id field (ordinal:1). */
public static final int FD_FACE_ID_ID = 0;
public static final long FD_FACE_ID_ID_MASK = 1L << 0;
/** Identify the fd_face.image_md5 field (ordinal:2). */
public static final int FD_FACE_ID_IMAGE_MD5 = 1;
public static final long FD_FACE_ID_IMAGE_MD5_MASK = 1L << 1;
/** Identify the fd_face.feature_md5 field (ordinal:3). */
public static final int FD_FACE_ID_FEATURE_MD5 = 2;
public static final long FD_FACE_ID_FEATURE_MD5_MASK = 1L << 2;
/** Identify the fd_face.face_left field (ordinal:4). */
public static final int FD_FACE_ID_FACE_LEFT = 3;
public static final long FD_FACE_ID_FACE_LEFT_MASK = 1L << 3;
/** Identify the fd_face.face_top field (ordinal:5). */
public static final int FD_FACE_ID_FACE_TOP = 4;
public static final long FD_FACE_ID_FACE_TOP_MASK = 1L << 4;
/** Identify the fd_face.face_width field (ordinal:6). */
public static final int FD_FACE_ID_FACE_WIDTH = 5;
public static final long FD_FACE_ID_FACE_WIDTH_MASK = 1L << 5;
/** Identify the fd_face.face_height field (ordinal:7). */
public static final int FD_FACE_ID_FACE_HEIGHT = 6;
public static final long FD_FACE_ID_FACE_HEIGHT_MASK = 1L << 6;
/** Identify the fd_face.eye_leftx field (ordinal:8). */
public static final int FD_FACE_ID_EYE_LEFTX = 7;
public static final long FD_FACE_ID_EYE_LEFTX_MASK = 1L << 7;
/** Identify the fd_face.eye_lefty field (ordinal:9). */
public static final int FD_FACE_ID_EYE_LEFTY = 8;
public static final long FD_FACE_ID_EYE_LEFTY_MASK = 1L << 8;
/** Identify the fd_face.eye_rightx field (ordinal:10). */
public static final int FD_FACE_ID_EYE_RIGHTX = 9;
public static final long FD_FACE_ID_EYE_RIGHTX_MASK = 1L << 9;
/** Identify the fd_face.eye_righty field (ordinal:11). */
public static final int FD_FACE_ID_EYE_RIGHTY = 10;
public static final long FD_FACE_ID_EYE_RIGHTY_MASK = 1L << 10;
/** Identify the fd_face.mouth_x field (ordinal:12). */
public static final int FD_FACE_ID_MOUTH_X = 11;
public static final long FD_FACE_ID_MOUTH_X_MASK = 1L << 11;
/** Identify the fd_face.mouth_y field (ordinal:13). */
public static final int FD_FACE_ID_MOUTH_Y = 12;
public static final long FD_FACE_ID_MOUTH_Y_MASK = 1L << 12;
/** Identify the fd_face.nose_x field (ordinal:14). */
public static final int FD_FACE_ID_NOSE_X = 13;
public static final long FD_FACE_ID_NOSE_X_MASK = 1L << 13;
/** Identify the fd_face.nose_y field (ordinal:15). */
public static final int FD_FACE_ID_NOSE_Y = 14;
public static final long FD_FACE_ID_NOSE_Y_MASK = 1L << 14;
/** Identify the fd_face.angle_yaw field (ordinal:16). */
public static final int FD_FACE_ID_ANGLE_YAW = 15;
public static final long FD_FACE_ID_ANGLE_YAW_MASK = 1L << 15;
/** Identify the fd_face.angle_pitch field (ordinal:17). */
public static final int FD_FACE_ID_ANGLE_PITCH = 16;
public static final long FD_FACE_ID_ANGLE_PITCH_MASK = 1L << 16;
/** Identify the fd_face.angle_roll field (ordinal:18). */
public static final int FD_FACE_ID_ANGLE_ROLL = 17;
public static final long FD_FACE_ID_ANGLE_ROLL_MASK = 1L << 17;
/** Identify the fd_face.angle_confidence field (ordinal:19). */
public static final int FD_FACE_ID_ANGLE_CONFIDENCE = 18;
public static final long FD_FACE_ID_ANGLE_CONFIDENCE_MASK = 1L << 18;
/** Identify the fd_face.ext_info field (ordinal:20). */
public static final int FD_FACE_ID_EXT_INFO = 19;
public static final long FD_FACE_ID_EXT_INFO_MASK = 1L << 19;
/** Identify the fd_face.create_time field (ordinal:21). */
public static final int FD_FACE_ID_CREATE_TIME = 20;
public static final long FD_FACE_ID_CREATE_TIME_MASK = 1L << 20;
/** Identify the fd_feature.md5 field (ordinal:1). */
public static final int FD_FEATURE_ID_MD5 = 0;
public static final long FD_FEATURE_ID_MD5_MASK = 1L << 0;
/** Identify the fd_feature.feature field (ordinal:2). */
public static final int FD_FEATURE_ID_FEATURE = 1;
public static final long FD_FEATURE_ID_FEATURE_MASK = 1L << 1;
/** Identify the fd_feature.create_time field (ordinal:3). */
public static final int FD_FEATURE_ID_CREATE_TIME = 2;
public static final long FD_FEATURE_ID_CREATE_TIME_MASK = 1L << 2;
/** Identify the fd_image.md5 field (ordinal:1). */
public static final int FD_IMAGE_ID_MD5 = 0;
public static final long FD_IMAGE_ID_MD5_MASK = 1L << 0;
/** Identify the fd_image.format field (ordinal:2). */
public static final int FD_IMAGE_ID_FORMAT = 1;
public static final long FD_IMAGE_ID_FORMAT_MASK = 1L << 1;
/** Identify the fd_image.width field (ordinal:3). */
public static final int FD_IMAGE_ID_WIDTH = 2;
public static final long FD_IMAGE_ID_WIDTH_MASK = 1L << 2;
/** Identify the fd_image.height field (ordinal:4). */
public static final int FD_IMAGE_ID_HEIGHT = 3;
public static final long FD_IMAGE_ID_HEIGHT_MASK = 1L << 3;
/** Identify the fd_image.depth field (ordinal:5). */
public static final int FD_IMAGE_ID_DEPTH = 4;
public static final long FD_IMAGE_ID_DEPTH_MASK = 1L << 4;
/** Identify the fd_image.face_num field (ordinal:6). */
public static final int FD_IMAGE_ID_FACE_NUM = 5;
public static final long FD_IMAGE_ID_FACE_NUM_MASK = 1L << 5;
/** Identify the fd_image.update_time field (ordinal:7). */
public static final int FD_IMAGE_ID_UPDATE_TIME = 6;
public static final long FD_IMAGE_ID_UPDATE_TIME_MASK = 1L << 6;
/** Identify the fd_image.create_time field (ordinal:8). */
public static final int FD_IMAGE_ID_CREATE_TIME = 7;
public static final long FD_IMAGE_ID_CREATE_TIME_MASK = 1L << 7;
/** Identify the fd_store.md5 field (ordinal:1). */
public static final int FD_STORE_ID_MD5 = 0;
public static final long FD_STORE_ID_MD5_MASK = 1L << 0;
/** Identify the fd_store.encoding field (ordinal:2). */
public static final int FD_STORE_ID_ENCODING = 1;
public static final long FD_STORE_ID_ENCODING_MASK = 1L << 1;
/** Identify the fd_store.data field (ordinal:3). */
public static final int FD_STORE_ID_DATA = 2;
public static final long FD_STORE_ID_DATA_MASK = 1L << 2;
//////////////////////////////////////
// COLUMN NAME DECLARE
//////////////////////////////////////
/////////////////// fd_face ////////////
/** Contains all the full fields of the fd_face table.*/
public static final String FD_FACE_FULL_FIELDS ="fd_face.id"
+ ",fd_face.image_md5"
+ ",fd_face.feature_md5"
+ ",fd_face.face_left"
+ ",fd_face.face_top"
+ ",fd_face.face_width"
+ ",fd_face.face_height"
+ ",fd_face.eye_leftx"
+ ",fd_face.eye_lefty"
+ ",fd_face.eye_rightx"
+ ",fd_face.eye_righty"
+ ",fd_face.mouth_x"
+ ",fd_face.mouth_y"
+ ",fd_face.nose_x"
+ ",fd_face.nose_y"
+ ",fd_face.angle_yaw"
+ ",fd_face.angle_pitch"
+ ",fd_face.angle_roll"
+ ",fd_face.angle_confidence"
+ ",fd_face.ext_info"
+ ",fd_face.create_time";
/** Field that contains the comma separated fields of the fd_face table. */
public static final String FD_FACE_FIELDS = "id"
+ ",image_md5"
+ ",feature_md5"
+ ",face_left"
+ ",face_top"
+ ",face_width"
+ ",face_height"
+ ",eye_leftx"
+ ",eye_lefty"
+ ",eye_rightx"
+ ",eye_righty"
+ ",mouth_x"
+ ",mouth_y"
+ ",nose_x"
+ ",nose_y"
+ ",angle_yaw"
+ ",angle_pitch"
+ ",angle_roll"
+ ",angle_confidence"
+ ",ext_info"
+ ",create_time";
public static final java.util.List FD_FACE_FIELDS_LIST = java.util.Arrays.asList(FD_FACE_FIELDS.split(","));
/** Field that contains the comma separated java fields of the fd_face table. */
public static final String FD_FACE_JAVA_FIELDS = "id"
+ ",imageMd5"
+ ",featureMd5"
+ ",faceLeft"
+ ",faceTop"
+ ",faceWidth"
+ ",faceHeight"
+ ",eyeLeftx"
+ ",eyeLefty"
+ ",eyeRightx"
+ ",eyeRighty"
+ ",mouthX"
+ ",mouthY"
+ ",noseX"
+ ",noseY"
+ ",angleYaw"
+ ",anglePitch"
+ ",angleRoll"
+ ",angleConfidence"
+ ",extInfo"
+ ",createTime";
public static final java.util.List FD_FACE_JAVA_FIELDS_LIST = java.util.Arrays.asList(FD_FACE_JAVA_FIELDS.split(","));
/////////////////// fd_feature ////////////
/** Contains all the full fields of the fd_feature table.*/
public static final String FD_FEATURE_FULL_FIELDS ="fd_feature.md5"
+ ",fd_feature.feature"
+ ",fd_feature.create_time";
/** Field that contains the comma separated fields of the fd_feature table. */
public static final String FD_FEATURE_FIELDS = "md5"
+ ",feature"
+ ",create_time";
public static final java.util.List FD_FEATURE_FIELDS_LIST = java.util.Arrays.asList(FD_FEATURE_FIELDS.split(","));
/** Field that contains the comma separated java fields of the fd_feature table. */
public static final String FD_FEATURE_JAVA_FIELDS = "md5"
+ ",feature"
+ ",createTime";
public static final java.util.List FD_FEATURE_JAVA_FIELDS_LIST = java.util.Arrays.asList(FD_FEATURE_JAVA_FIELDS.split(","));
/////////////////// fd_image ////////////
/** Contains all the full fields of the fd_image table.*/
public static final String FD_IMAGE_FULL_FIELDS ="fd_image.md5"
+ ",fd_image.format"
+ ",fd_image.width"
+ ",fd_image.height"
+ ",fd_image.depth"
+ ",fd_image.face_num"
+ ",fd_image.update_time"
+ ",fd_image.create_time";
/** Field that contains the comma separated fields of the fd_image table. */
public static final String FD_IMAGE_FIELDS = "md5"
+ ",format"
+ ",width"
+ ",height"
+ ",depth"
+ ",face_num"
+ ",update_time"
+ ",create_time";
public static final java.util.List FD_IMAGE_FIELDS_LIST = java.util.Arrays.asList(FD_IMAGE_FIELDS.split(","));
/** Field that contains the comma separated java fields of the fd_image table. */
public static final String FD_IMAGE_JAVA_FIELDS = "md5"
+ ",format"
+ ",width"
+ ",height"
+ ",depth"
+ ",faceNum"
+ ",updateTime"
+ ",createTime";
public static final java.util.List FD_IMAGE_JAVA_FIELDS_LIST = java.util.Arrays.asList(FD_IMAGE_JAVA_FIELDS.split(","));
/////////////////// fd_store ////////////
/** Contains all the full fields of the fd_store table.*/
public static final String FD_STORE_FULL_FIELDS ="fd_store.md5"
+ ",fd_store.encoding"
+ ",fd_store.data";
/** Field that contains the comma separated fields of the fd_store table. */
public static final String FD_STORE_FIELDS = "md5"
+ ",encoding"
+ ",data";
public static final java.util.List FD_STORE_FIELDS_LIST = java.util.Arrays.asList(FD_STORE_FIELDS.split(","));
/** Field that contains the comma separated java fields of the fd_store table. */
public static final String FD_STORE_JAVA_FIELDS = "md5"
+ ",encoding"
+ ",data";
public static final java.util.List FD_STORE_JAVA_FIELDS_LIST = java.util.Arrays.asList(FD_STORE_JAVA_FIELDS.split(","));
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy