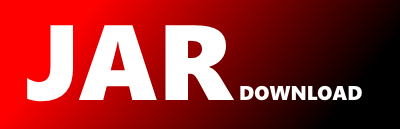
net.gdface.facedb.dborm.DaoConstant Maven / Gradle / Ivy
The newest version!
// ______________________________________________________
// Generated by sql2java - https://github.com/10km/sql2java-2-6-7 (custom branch)
// modified by guyadong from
// sql2java original version https://sourceforge.net/projects/sql2java/
// JDBC driver used at code generation time: com.mysql.jdbc.Driver
// template: dao.constant.java.vm
// ______________________________________________________
package net.gdface.facedb.dborm;
/**
* constant declare
* @author guyadong
*/
public interface DaoConstant {
public static final int LONG_BIT_NUM = 64;
public static final String SQL_LIKE_WILDCARD = "%";
/** set =QUERY for loadUsingTemplate */
public static final int SEARCH_EXACT = 0;
/** set %QUERY% for loadLikeTemplate */
public static final int SEARCH_LIKE = 1;
/** set %QUERY for loadLikeTemplate */
public static final int SEARCH_STARTING_LIKE = 2;
/** set QUERY% for loadLikeTemplate */
public static final int SEARCH_ENDING_LIKE = 3;
/** meta data of table's column */
public static class ColumnMeta{
final String table;
final String field;
final String fullName;
final int index;
final int ordinal;
final long mask;
final String getter;
final String setter;
private ColumnMeta(String table,String field,int ordinal,String getter,String setter){
this.table = table;
this.field = field;
this.fullName = this.table + field;
this.ordinal = ordinal;
this.index = ordinal -1;
this.mask = (1L << index);
this.getter = setter;
this.setter = setter;
}
}
/** Constant definition for fd_face */
public static interface FaceConst{
public static final String TABLENAME = "fd_face";
/** column count for fd_face table */
public static final int COLUMN_COUNT = 21;
/** primary keys count for fd_face table */
public static final int PRIMARY_KEY_COUNT = 1;
/** foreign keys count for fd_face table */
public static final int FOREIGN_KEY_COUNT = 2;
/** imported keys count for fd_face table */
public static final int IMPORTED_KEY_COUNT = 0;
/** Contains all the primary key fields of the fd_face table. */
public static final String[] PRIMARYKEY_NAMES = {"id"};
/** Contains all the full fields of the fd_face table.*/
public static final String FULL_FIELDS ="fd_face.id"
+ ",fd_face.image_md5"
+ ",fd_face.feature_md5"
+ ",fd_face.face_left"
+ ",fd_face.face_top"
+ ",fd_face.face_width"
+ ",fd_face.face_height"
+ ",fd_face.eye_leftx"
+ ",fd_face.eye_lefty"
+ ",fd_face.eye_rightx"
+ ",fd_face.eye_righty"
+ ",fd_face.mouth_x"
+ ",fd_face.mouth_y"
+ ",fd_face.nose_x"
+ ",fd_face.nose_y"
+ ",fd_face.angle_yaw"
+ ",fd_face.angle_pitch"
+ ",fd_face.angle_roll"
+ ",fd_face.angle_confidence"
+ ",fd_face.ext_info"
+ ",fd_face.create_time";
/** Field that contains the comma separated fields of the fd_face table. */
public static final String FIELDS = "id"
+ ",image_md5"
+ ",feature_md5"
+ ",face_left"
+ ",face_top"
+ ",face_width"
+ ",face_height"
+ ",eye_leftx"
+ ",eye_lefty"
+ ",eye_rightx"
+ ",eye_righty"
+ ",mouth_x"
+ ",mouth_y"
+ ",nose_x"
+ ",nose_y"
+ ",angle_yaw"
+ ",angle_pitch"
+ ",angle_roll"
+ ",angle_confidence"
+ ",ext_info"
+ ",create_time";
/** immutable list of {@link #FIELDS} */
public static final java.util.List FIELDS_LIST = java.util.Collections.unmodifiableList(java.util.Arrays.asList(FIELDS.split(",")));
/** Field that contains the comma separated java fields of the fd_face table. */
public static final String JAVA_FIELDS = "id"
+ ",imageMd5"
+ ",featureMd5"
+ ",faceLeft"
+ ",faceTop"
+ ",faceWidth"
+ ",faceHeight"
+ ",eyeLeftx"
+ ",eyeLefty"
+ ",eyeRightx"
+ ",eyeRighty"
+ ",mouthX"
+ ",mouthY"
+ ",noseX"
+ ",noseY"
+ ",angleYaw"
+ ",anglePitch"
+ ",angleRoll"
+ ",angleConfidence"
+ ",extInfo"
+ ",createTime";
/** immutable list of {@link #JAVA_FIELDS} */
public static final java.util.List JAVA_FIELDS_LIST = java.util.Collections.unmodifiableList(java.util.Arrays.asList(JAVA_FIELDS.split(",")));
public static enum Fk{
/** foreign key fd_face(feature_md5) -> fd_feature */featureMd5,
/** foreign key fd_face(image_md5) -> fd_image */imageMd5
}
public static enum Ik{
}
public static enum Index{
/** fd_face index (feature_md5) */indexFeatureMd5,
/** fd_face index (image_md5) */indexImageMd5
}
/** Column Constant definition for fd_face */
public static enum Column{
/** constant for fd_face.id */
id("fd_face","id",1,"getId","setId"),
/** constant for fd_face.image_md5 */
imageMd5("fd_face","image_md5",2,"getImageMd5","setImageMd5"),
/** constant for fd_face.feature_md5 */
featureMd5("fd_face","feature_md5",3,"getFeatureMd5","setFeatureMd5"),
/** constant for fd_face.face_left */
faceLeft("fd_face","face_left",4,"getFaceLeft","setFaceLeft"),
/** constant for fd_face.face_top */
faceTop("fd_face","face_top",5,"getFaceTop","setFaceTop"),
/** constant for fd_face.face_width */
faceWidth("fd_face","face_width",6,"getFaceWidth","setFaceWidth"),
/** constant for fd_face.face_height */
faceHeight("fd_face","face_height",7,"getFaceHeight","setFaceHeight"),
/** constant for fd_face.eye_leftx */
eyeLeftx("fd_face","eye_leftx",8,"getEyeLeftx","setEyeLeftx"),
/** constant for fd_face.eye_lefty */
eyeLefty("fd_face","eye_lefty",9,"getEyeLefty","setEyeLefty"),
/** constant for fd_face.eye_rightx */
eyeRightx("fd_face","eye_rightx",10,"getEyeRightx","setEyeRightx"),
/** constant for fd_face.eye_righty */
eyeRighty("fd_face","eye_righty",11,"getEyeRighty","setEyeRighty"),
/** constant for fd_face.mouth_x */
mouthX("fd_face","mouth_x",12,"getMouthX","setMouthX"),
/** constant for fd_face.mouth_y */
mouthY("fd_face","mouth_y",13,"getMouthY","setMouthY"),
/** constant for fd_face.nose_x */
noseX("fd_face","nose_x",14,"getNoseX","setNoseX"),
/** constant for fd_face.nose_y */
noseY("fd_face","nose_y",15,"getNoseY","setNoseY"),
/** constant for fd_face.angle_yaw */
angleYaw("fd_face","angle_yaw",16,"getAngleYaw","setAngleYaw"),
/** constant for fd_face.angle_pitch */
anglePitch("fd_face","angle_pitch",17,"getAnglePitch","setAnglePitch"),
/** constant for fd_face.angle_roll */
angleRoll("fd_face","angle_roll",18,"getAngleRoll","setAngleRoll"),
/** constant for fd_face.angle_confidence */
angleConfidence("fd_face","angle_confidence",19,"getAngleConfidence","setAngleConfidence"),
/** constant for fd_face.ext_info */
extInfo("fd_face","ext_info",20,"getExtInfo","setExtInfo"),
/** constant for fd_face.create_time */
createTime("fd_face","create_time",21,"getCreateTime","setCreateTime");
final ColumnMeta meta;
private Column(String table,String field,int ordinal,String getter,String setter){
meta = new ColumnMeta(table,field,ordinal,getter,setter);
}
}
}
/** Constant definition for fd_feature */
public static interface FeatureConst{
public static final String TABLENAME = "fd_feature";
/** column count for fd_feature table */
public static final int COLUMN_COUNT = 3;
/** primary keys count for fd_feature table */
public static final int PRIMARY_KEY_COUNT = 1;
/** foreign keys count for fd_feature table */
public static final int FOREIGN_KEY_COUNT = 0;
/** imported keys count for fd_feature table */
public static final int IMPORTED_KEY_COUNT = 1;
/** Contains all the primary key fields of the fd_feature table. */
public static final String[] PRIMARYKEY_NAMES = {"md5"};
/** Contains all the full fields of the fd_feature table.*/
public static final String FULL_FIELDS ="fd_feature.md5"
+ ",fd_feature.feature"
+ ",fd_feature.create_time";
/** Field that contains the comma separated fields of the fd_feature table. */
public static final String FIELDS = "md5"
+ ",feature"
+ ",create_time";
/** immutable list of {@link #FIELDS} */
public static final java.util.List FIELDS_LIST = java.util.Collections.unmodifiableList(java.util.Arrays.asList(FIELDS.split(",")));
/** Field that contains the comma separated java fields of the fd_feature table. */
public static final String JAVA_FIELDS = "md5"
+ ",feature"
+ ",createTime";
/** immutable list of {@link #JAVA_FIELDS} */
public static final java.util.List JAVA_FIELDS_LIST = java.util.Collections.unmodifiableList(java.util.Arrays.asList(JAVA_FIELDS.split(",")));
public static enum Fk{
}
public static enum Ik{
/** imported key fd_face(feature_md5) -> fd_feature */featureMd5OfFace
}
public static enum Index{
}
/** Column Constant definition for fd_feature */
public static enum Column{
/** constant for fd_feature.md5 */
md5("fd_feature","md5",1,"getMd5","setMd5"),
/** constant for fd_feature.feature */
feature("fd_feature","feature",2,"getFeature","setFeature"),
/** constant for fd_feature.create_time */
createTime("fd_feature","create_time",3,"getCreateTime","setCreateTime");
final ColumnMeta meta;
private Column(String table,String field,int ordinal,String getter,String setter){
meta = new ColumnMeta(table,field,ordinal,getter,setter);
}
}
}
/** Constant definition for fd_image */
public static interface ImageConst{
public static final String TABLENAME = "fd_image";
/** column count for fd_image table */
public static final int COLUMN_COUNT = 8;
/** primary keys count for fd_image table */
public static final int PRIMARY_KEY_COUNT = 1;
/** foreign keys count for fd_image table */
public static final int FOREIGN_KEY_COUNT = 0;
/** imported keys count for fd_image table */
public static final int IMPORTED_KEY_COUNT = 1;
/** Contains all the primary key fields of the fd_image table. */
public static final String[] PRIMARYKEY_NAMES = {"md5"};
/** Contains all the full fields of the fd_image table.*/
public static final String FULL_FIELDS ="fd_image.md5"
+ ",fd_image.format"
+ ",fd_image.width"
+ ",fd_image.height"
+ ",fd_image.depth"
+ ",fd_image.face_num"
+ ",fd_image.update_time"
+ ",fd_image.create_time";
/** Field that contains the comma separated fields of the fd_image table. */
public static final String FIELDS = "md5"
+ ",format"
+ ",width"
+ ",height"
+ ",depth"
+ ",face_num"
+ ",update_time"
+ ",create_time";
/** immutable list of {@link #FIELDS} */
public static final java.util.List FIELDS_LIST = java.util.Collections.unmodifiableList(java.util.Arrays.asList(FIELDS.split(",")));
/** Field that contains the comma separated java fields of the fd_image table. */
public static final String JAVA_FIELDS = "md5"
+ ",format"
+ ",width"
+ ",height"
+ ",depth"
+ ",faceNum"
+ ",updateTime"
+ ",createTime";
/** immutable list of {@link #JAVA_FIELDS} */
public static final java.util.List JAVA_FIELDS_LIST = java.util.Collections.unmodifiableList(java.util.Arrays.asList(JAVA_FIELDS.split(",")));
public static enum Fk{
}
public static enum Ik{
/** imported key fd_face(image_md5) -> fd_image */imageMd5OfFace
}
public static enum Index{
}
/** Column Constant definition for fd_image */
public static enum Column{
/** constant for fd_image.md5 */
md5("fd_image","md5",1,"getMd5","setMd5"),
/** constant for fd_image.format */
format("fd_image","format",2,"getFormat","setFormat"),
/** constant for fd_image.width */
width("fd_image","width",3,"getWidth","setWidth"),
/** constant for fd_image.height */
height("fd_image","height",4,"getHeight","setHeight"),
/** constant for fd_image.depth */
depth("fd_image","depth",5,"getDepth","setDepth"),
/** constant for fd_image.face_num */
faceNum("fd_image","face_num",6,"getFaceNum","setFaceNum"),
/** constant for fd_image.update_time */
updateTime("fd_image","update_time",7,"getUpdateTime","setUpdateTime"),
/** constant for fd_image.create_time */
createTime("fd_image","create_time",8,"getCreateTime","setCreateTime");
final ColumnMeta meta;
private Column(String table,String field,int ordinal,String getter,String setter){
meta = new ColumnMeta(table,field,ordinal,getter,setter);
}
}
}
/** Constant definition for fd_store */
public static interface StoreConst{
public static final String TABLENAME = "fd_store";
/** column count for fd_store table */
public static final int COLUMN_COUNT = 3;
/** primary keys count for fd_store table */
public static final int PRIMARY_KEY_COUNT = 1;
/** foreign keys count for fd_store table */
public static final int FOREIGN_KEY_COUNT = 0;
/** imported keys count for fd_store table */
public static final int IMPORTED_KEY_COUNT = 0;
/** Contains all the primary key fields of the fd_store table. */
public static final String[] PRIMARYKEY_NAMES = {"md5"};
/** Contains all the full fields of the fd_store table.*/
public static final String FULL_FIELDS ="fd_store.md5"
+ ",fd_store.encoding"
+ ",fd_store.data";
/** Field that contains the comma separated fields of the fd_store table. */
public static final String FIELDS = "md5"
+ ",encoding"
+ ",data";
/** immutable list of {@link #FIELDS} */
public static final java.util.List FIELDS_LIST = java.util.Collections.unmodifiableList(java.util.Arrays.asList(FIELDS.split(",")));
/** Field that contains the comma separated java fields of the fd_store table. */
public static final String JAVA_FIELDS = "md5"
+ ",encoding"
+ ",data";
/** immutable list of {@link #JAVA_FIELDS} */
public static final java.util.List JAVA_FIELDS_LIST = java.util.Collections.unmodifiableList(java.util.Arrays.asList(JAVA_FIELDS.split(",")));
public static enum Fk{
}
public static enum Ik{
}
public static enum Index{
}
/** Column Constant definition for fd_store */
public static enum Column{
/** constant for fd_store.md5 */
md5("fd_store","md5",1,"getMd5","setMd5"),
/** constant for fd_store.encoding */
encoding("fd_store","encoding",2,"getEncoding","setEncoding"),
/** constant for fd_store.data */
data("fd_store","data",3,"getData","setData");
final ColumnMeta meta;
private Column(String table,String field,int ordinal,String getter,String setter){
meta = new ColumnMeta(table,field,ordinal,getter,setter);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy