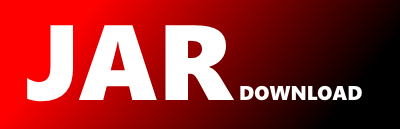
net.gdface.facedb.BaseDao Maven / Gradle / Ivy
The newest version!
// ______________________________________________________
// Generated by sql2java - https://github.com/10km/sql2java
// JDBC driver used at code generation time: com.mysql.jdbc.Driver
// template: base.dao.java.vm
// ______________________________________________________
package net.gdface.facedb;
import static com.google.common.base.Preconditions.*;
import static gu.sql2java.manager.Managers.*;
import com.google.common.base.Throwables;
import java.text.SimpleDateFormat;
import java.util.Collection;
import java.util.Date;
import java.util.List;
import java.util.concurrent.Callable;
import com.google.common.base.Function;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.Lists;
import gu.sql2java.TableManager;
import gu.sql2java.BaseBean;
import gu.sql2java.manager.Managers;
import gu.sql2java.store.URLStore;
import net.gdface.facedb.db.Constant;
import net.gdface.facedb.db.*;
import gu.sql2java.exception.ObjectRetrievalException;
import gu.sql2java.exception.RuntimeDaoException;
/**
* 数据库访问基础方法
* @author guyadong
*
*/
class BaseDao implements CommonConstant,Constant {
static{
TableManagerInitializer.INSTANCE.init();
}
protected URLStore getImageURLStore() {
return TableManagerInitializer.INSTANCE.storeInstance;
}
/** 生成 SQL where 语句,example: {@code WHERE create_time >'2017-09-02 12:12:12'} */
static private String makeWhere(Date timestamp,String field){
SimpleDateFormat formatter = new SimpleDateFormat(TIMESTAMP_FORMATTER_STR);
return String.format(
"WHERE %s > '%s'",
field,
formatter.format(checkNotNull(timestamp)));
}
/**
* 事务执行
* @throws RuntimeDaoException
*/
protected static T daoRunAsTransaction(Callable fun)
throws RuntimeDaoException{
return Managers.getSqlRunner(ALIAS_NAME).runAsTransaction(checkNotNull(fun));
}
/**
* 事务执行
* @throws RuntimeDaoException
*/
protected static void daoRunAsTransaction(Runnable fun)
throws RuntimeDaoException{
Managers.getSqlRunner(ALIAS_NAME).runAsTransaction(checkNotNull(fun));
}
/**
* 查询 {@code table}表的{@code column}字段的数据
* @param table 数据库表名
* @param column 有效的table表字段名或table对应java类的字段名
* @param distinct 为{@code true}只返回不重复记录
* @param where 'where'起始的SQL 查询条件语句,可为{@code null}
* @param startRow 返回记录的起始行(首行=1,尾行=-1)
* @param numRows 返回记录条数(小于0时返回所有记录)
* @return {@code column}字段记录
* @see TableManager#loadColumnAsList(String,boolean,String,int,int)
* @throws RuntimeDaoException
*/
protectedList daoLoadColumnAsList(String table,String column,boolean distinct,String where,int startRow, int numRows)
throws RuntimeDaoException{
return managerOf(table).loadColumnAsList(column, distinct, where, startRow, numRows);
}
/**
* 查询 {@code table}表的{@code column}字段的数据
* @param table 数据库表名
* @param column 有效的table表字段名或table对应java类的字段名
* @param distinct 为{@code true}只返回不重复记录
* @param where 'where'起始的SQL 查询条件语句,可为{@code null}
* @return {@code column}字段记录
* @see TableManager#loadColumnAsList(String,boolean,String,int,int)
* @throws RuntimeDaoException
*/
protectedList daoLoadColumnAsList(String table,String column,boolean distinct,String where)
throws RuntimeDaoException{
return managerOf(table).loadColumnAsList(column, distinct, where, 1, -1);
}
protected static final void throwCauseIfInstanceOf(Exception error,Class expType) throws T {
if(null != error.getCause()){
Throwables.throwIfInstanceOf(error.getCause(),expType);
}
}
//////////// FD_FACE /////////
//1
/**
* 根据主键从数据库读取记录,没有找到记录返回{@code null}
*
* @param id 主键
* @return FaceBean 对象
* @see IFaceManager#loadByPrimaryKey(Integer)
* @throws RuntimeDaoException
*/
protected FaceBean daoGetFace(Integer id)throws RuntimeDaoException{
return instanceOf(IFaceManager.class).loadByPrimaryKey(id);
}
//1-2
/**
* 根据主键从数据库读取记录,没有找到记录抛出异常
*
* @param id 主键
* @return FaceBean 对象
* @see IFaceManager#loadByPrimaryKeyChecked(Integer)
* @throws RuntimeDaoException
* @throws ObjectRetrievalException 没有找到记录
*/
protected FaceBean daoGetFaceChecked(Integer id)throws RuntimeDaoException,ObjectRetrievalException{
return instanceOf(IFaceManager.class).loadByPrimaryKeyChecked(id);
}
//2
/**
* 根据主键从数据库读取记录
* @return 返回与输入参数下标对应的 FaceBean 列表,没有查到记录的返回{@code null}
* @see IFaceManager#loadByPrimaryKey(Collection)
* @throws RuntimeDaoException
*/
protected List daoGetFaces(Collection idCollection)
throws RuntimeDaoException{
return instanceOf(IFaceManager.class).loadByPrimaryKey(idCollection);
}
//3
/**
* 删除主键列表({@code idCollection})指定的记录
* @return 返回删除的记录条数
* @see IFaceManager#deleteByPrimaryKey(Collection)
* @throws RuntimeDaoException
*/
protected int daoDeleteFacesByPrimaryKey(Collection idCollection)
throws RuntimeDaoException{
int count =0;
if(null != idCollection){
for(Integer id:idCollection){
count += daoDeleteFace(id);
}
}
return count;
}
//3-5
/** transformer : FaceBean to fd_face.id */
protected final Function daoCastFaceToPk = new Function(){
@Override
public Integer apply(FaceBean input) {
return null == input ? null : input.getId();
}};
//3-6
/** transformer : fd_face.id to FaceBean */
protected final Function daoCastFaceFromPk = new Function(){
@Override
public FaceBean apply(Integer input) {
return daoGetFace(input);
}};
//3-8
/**
* unwrap primary key from {@link FaceBean}
* if {@code beans} is {@code null},return a empty list(immutable)
*
* @param beans {@link FaceBean} collection
* @return primary key list
* @see IFaceManager#toPrimaryKeyList(Collection)
*/
protected List daoToPrimaryKeyListFromFaces(Collection beans){
if (null == beans){
return ImmutableList.of();
}else{
return instanceOf(IFaceManager.class).toPrimaryKeyList(beans);
}
}
//3-9
/**
* unwrap primary key from {@link FaceBean}
*
* the returned list is a transformed view of {@code beans};
* changes to {@code beans} will be reflected in the returned list and vice versa.
*
* if {@code beans} is {@code null},return a empty list(immutable)
* @param beans {@link FaceBean} list
* @return primary key list
* @see Lists#transform(List, Function)
*/
protected List daoToPrimaryKeyListFromFaces(List beans){
if(null == beans){
return ImmutableList.of();
}else{
return Lists.transform(beans,daoCastFaceToPk);
}
}
//4
/**
* 判断主键指定的记录是否存在
*
* @param id 主键
* @see IFaceManager#existsPrimaryKey(Integer)
* @throws RuntimeDaoException
*/
protected boolean daoExistsFace(Integer id)
throws RuntimeDaoException{
return instanceOf(IFaceManager.class).existsPrimaryKey(id);
}
//4-2
/**
* 判断指定的记录是否存在
* @see TableManager#existsByPrimaryKey(BaseBean)
* @throws RuntimeDaoException
*/
protected boolean daoExistsFace(FaceBean bean)
throws RuntimeDaoException{
return instanceOf(IFaceManager.class).existsByPrimaryKey(bean);
}
//5
/**
* 删除主键指定的记录
*
* @param id 主键
* @return 返回删除的记录条数(1),如果记录不存在返回0
* @see IFaceManager#deleteByPrimaryKey(Integer)
* @throws RuntimeDaoException
*/
protected int daoDeleteFace(Integer id)
throws RuntimeDaoException{
return instanceOf(IFaceManager.class).deleteByPrimaryKey(id);
}
//5-2
/**
* 删除指定的记录
* @param bean 要删除的记录
* @return 返回删除的记录条数(1),如果{@code bean}为{@code null}或记录不存在返回0
* @see #daoDeleteFace(Integer)
* @throws RuntimeDaoException
*/
protected int daoDeleteFace(FaceBean bean)
throws RuntimeDaoException{
return null == bean ? 0 : daoDeleteFace(bean.getId());
}
//6
/**
* 删除{@code faceBeanCollection}列表指定的记录
* @return 返回删除的记录条数
* @see #daoDeleteFace(Integer)
* @throws RuntimeDaoException
*/
protected int daoDeleteFaces(Collection beans)
throws RuntimeDaoException{
int count =0;
if(null != beans){
for(FaceBean bean:beans){
count += daoDeleteFace(bean);
}
}
return count;
}
//7
/**
* 检查数据库中是否有(主键)相同的记录,如果有则抛出异常
* @see TableManager#checkDuplicate(BaseBean)
* @throws RuntimeDaoException
* @throws DuplicateRecordException if exists duplicated row
*/
protected FaceBean daoCheckDuplicate(FaceBean faceBean)
throws RuntimeDaoException,DuplicateRecordException{
try{
return instanceOf(IFaceManager.class).checkDuplicate(faceBean);
}catch(ObjectRetrievalException e){
throw new DuplicateRecordException();
}
}
//7-3
/**
* 检查数据库中是否有(主键)相同的记录,如果有则抛出异常
*
* @param id 主键
* @see TableManager#checkDuplicate(BaseBean)
* @throws DuplicateRecordException if exists duplicated row
* @return always {@code idOfFace}
* @throws RuntimeDaoException
* @throws DuplicateRecordException
*/
protected Integer daoCheckDuplicateFace(Integer id)
throws RuntimeDaoException,DuplicateRecordException{
if(instanceOf(IFaceManager.class).existsPrimaryKey(id)){
throw new DuplicateRecordException();
}
return id;
}
//8-3
/**
* 返回外键(fd_face.feature_md5)引用的 fd_feature 记录
* @param bean
* @see IFaceManager#getReferencedByFeatureMd5(FaceBean)
* @throws RuntimeDaoException
*/
protected FeatureBean daoGetReferencedByFeatureMd5OnFace(FaceBean bean)
throws RuntimeDaoException{
return instanceOf(IFaceManager.class).getReferencedByFeatureMd5(bean);
}
//8-4
/**
* 设置外键fd_face(feature_md5)引用为{@code beanToSet}指定的记录,
* 如果{@code beanToSet}没有保存则先save
* @param bean
* @param beanToSet 被引用的记录
* @see IFaceManager#setReferencedByFeatureMd5(FaceBean,FeatureBean)
* @throws RuntimeDaoException
*/
protected FeatureBean daoSetReferencedByFeatureMd5OnFace(FaceBean bean,FeatureBean beanToSet)
throws RuntimeDaoException{
return instanceOf(IFaceManager.class).setReferencedByFeatureMd5(bean,beanToSet);
}
//8-6
/** transformer : FaceBean to fd_face.feature_md5 */
protected final Function daoCastFaceToFeatureMd5 = new Function(){
@Override
public String apply(FaceBean input) {
return null == input ? null : input.getFeatureMd5();
}};
//8-8
/** transformer : fd_face.id to fd_face.feature_md5 */
protected final Function daoCastFacePkToFeatureMd5 = new Function(){
@Override
public String apply(Integer input) {
return null == input
? null
: daoCastFaceToFeatureMd5.apply(daoGetFace(input));
}};
//8-10
/** transformer : FaceBean to FeatureBean */
protected final Function daoCastFaceToReferencedFeatureBean = new Function(){
@Override
public FeatureBean apply(FaceBean input) {
return instanceOf(IFaceManager.class).getReferencedByFeatureMd5(input);
}};
//8-3
/**
* 返回外键(fd_face.image_md5)引用的 fd_image 记录
* @param bean
* @see IFaceManager#getReferencedByImageMd5(FaceBean)
* @throws RuntimeDaoException
*/
protected ImageBean daoGetReferencedByImageMd5OnFace(FaceBean bean)
throws RuntimeDaoException{
return instanceOf(IFaceManager.class).getReferencedByImageMd5(bean);
}
//8-4
/**
* 设置外键fd_face(image_md5)引用为{@code beanToSet}指定的记录,
* 如果{@code beanToSet}没有保存则先save
* @param bean
* @param beanToSet 被引用的记录
* @see IFaceManager#setReferencedByImageMd5(FaceBean,ImageBean)
* @throws RuntimeDaoException
*/
protected ImageBean daoSetReferencedByImageMd5OnFace(FaceBean bean,ImageBean beanToSet)
throws RuntimeDaoException{
return instanceOf(IFaceManager.class).setReferencedByImageMd5(bean,beanToSet);
}
//8-6
/** transformer : FaceBean to fd_face.image_md5 */
protected final Function daoCastFaceToImageMd5 = new Function(){
@Override
public String apply(FaceBean input) {
return null == input ? null : input.getImageMd5();
}};
//8-8
/** transformer : fd_face.id to fd_face.image_md5 */
protected final Function daoCastFacePkToImageMd5 = new Function(){
@Override
public String apply(Integer input) {
return null == input
? null
: daoCastFaceToImageMd5.apply(daoGetFace(input));
}};
//8-10
/** transformer : FaceBean to ImageBean */
protected final Function daoCastFaceToReferencedImageBean = new Function(){
@Override
public ImageBean apply(FaceBean input) {
return instanceOf(IFaceManager.class).getReferencedByImageMd5(input);
}};
//14
/**
* 参见 {@link TableManager#save(BaseBean)}
* @throws RuntimeDaoException
*/
protected FaceBean daoSaveFace(FaceBean faceBean)
throws RuntimeDaoException{
return instanceOf(IFaceManager.class).save(faceBean);
}
//15
/** 同步保存
* see also {@link IFaceManager#save(FaceBean , FeatureBean, ImageBean )}
* @throws RuntimeDaoException
*/
protected FaceBean daoSaveFace(FaceBean faceBean
, FeatureBean refFeatureByFeatureMd5
, ImageBean refImageByImageMd5
)throws RuntimeDaoException{
return instanceOf(IFaceManager.class).save(faceBean
, refFeatureByFeatureMd5
, refImageByImageMd5
);
}
//12-3-3
/**
* 添加新记录
* @param beans 要添加的新记录集合
* @return always {@code beans}
* @see #daoSaveFace(FaceBean)
* @throws RuntimeDaoException
*/
protected Collection daoSaveFaces(Collection beans)
throws RuntimeDaoException {
if(null != beans){
for(FaceBean bean : beans){
daoSaveFace(bean);
}
}
return beans;
}
//12-3-5
/**
* {@link #daoSaveFaces(Collection)}的事务化版本
* @throws RuntimeDaoException
*/
protected Collection daoSaveFacesAsTransaction(final Collection beans)
throws RuntimeDaoException {
try{
return daoRunAsTransaction(new Callable>(){
@Override
public Collection call() throws Exception {
return daoSaveFaces(beans);
}});
}catch(RuntimeException e){
throw e;
}
}
//16
/**
* 查询{@code where} SQL条件语句指定的 fd_face 记录
* @param where SQL 条件语句,为{@code null}或空时加载所有记录
* @param startRow 返回记录的起始行(首行=1,尾行=-1)
* @param numRows 返回记录条数(小于0时返回所有记录)
* @see IFaceManager#loadByWhereAsList(String,int[],int,int)
* @throws RuntimeDaoException
*/
protected List daoLoadFaceByWhere(String where,int startRow, int numRows)
throws RuntimeDaoException{
return instanceOf(IFaceManager.class).loadByWhereAsList(where,null,startRow,numRows);
}
//16-2
/**
* 以{@code bean} 为模板查询 fd_face 记录
* @param bean 模板对象
* @param startRow 返回记录的起始行(首行=1,尾行=-1)
* @param numRows 返回记录条数(小于0时返回所有记录)
* @see TableManager#loadUsingTemplate(BaseBean,int,int)
* @throws RuntimeDaoException
*/
protected List daoLoadFaceUsingTemplate(FaceBean bean,int startRow, int numRows)
throws RuntimeDaoException{
return instanceOf(IFaceManager.class).loadUsingTemplateAsList(bean,startRow,numRows);
}
//16-3
/**
* 查询 fd_face 的{@code column}字段的数据
* @param column 有效的fd_face表字段名或{@link FaceBean} 字段名
* @param distinct 只返回不重复记录
* @param where 'where'起始的SQL 查询条件语句,可为{@code null}
* @return {@code column}字段记录
* @param startRow 返回记录的起始行(首行=1,尾行=-1)
* @param numRows 返回记录条数(小于0时返回所有记录)
* @see IFaceManager#loadColumnAsList(String,boolean,String,int,int)
* @throws RuntimeDaoException
*/
protected List daoLoadColumnOfFaceAsList(String column,boolean distinct,String where,int startRow, int numRows)
throws RuntimeDaoException{
return instanceOf(IFaceManager.class).loadColumnAsList(column, distinct, where, startRow, numRows);
}
//17
/**
* 返回 fd_face 表的所有记录
* @see IFaceManager#loadAllAsList()
* @throws RuntimeDaoException
*/
protected List daoLoadFaceAll()
throws RuntimeDaoException{
return instanceOf(IFaceManager.class).loadAllAsList();
}
//17-2
/**
* 返回满足{@code where} SQL条件语句的 fd_face 记录总数
* @see TableManager#countWhere(String)
* @throws RuntimeDaoException
*/
protected int daoCountFaceByWhere(String where)
throws RuntimeDaoException{
return instanceOf(IFaceManager.class).countWhere(where);
}
//17-3
/**
* 对满足{@code where} SQL条件语句的 fd_face 记录执行{@code action}
* @param where the sql 'where' clause
* @param action Action object for do something(not null)
* @see {@link IFaceManager#loadByWhereForAction(String,int[],int,int,Action)}
* @throws RuntimeDaoException
*/
protected int daoLoadFaceByWhereForAction(String where,TableManager.Action action)
throws RuntimeDaoException{
return instanceOf(IFaceManager.class).loadByWhereForAction(where,null,null,1,-1,action);
}
//18
/**
* 查询{@code where}条件指定的记录
* @return 返回查询结果记录的主键
* @see #daoLoadFaceByWhere(String,int,int)
* @throws RuntimeDaoException
*/
protected List daoLoadFaceIdByWhere(String where)
throws RuntimeDaoException{
return daoToPrimaryKeyListFromFaces(daoLoadFaceByWhere(where,1,-1));
}
//19
/**
* (主动更新机制实现)
* 返回 fd_face.create_time 字段大于指定时间戳({@code timestamp})的所有记录
* @see #daoLoadFaceByWhere(String,int,int)
* @throws RuntimeDaoException
* @throws IllegalArgumentException {@code timestamp}为{@code null}时
*/
protected List daoLoadFaceByCreateTime(Date timestamp,int startRow, int numRows)
throws RuntimeDaoException{
return daoLoadFaceByWhere(makeWhere(timestamp,"create_time"),startRow,numRows);
}
//20
/**
* 参见 {@link #daoLoadFaceByCreateTime(Date,int,int)}
* @throws RuntimeDaoException
*/
protected List daoLoadFaceByCreateTime(Date timestamp)
throws RuntimeDaoException{
return daoLoadFaceByCreateTime(timestamp,1,-1);
}
//20-5
/**
* 返回fd_face.create_time 字段大于指定时间戳({@code timestamp})的记录总数
* @see #daoCountFaceByWhere(String)
* @throws RuntimeDaoException
*/
protected int daoCountFaceByCreateTime(Date timestamp)
throws RuntimeDaoException{
return daoCountFaceByWhere(makeWhere(timestamp,"create_time"));
}
//21
/**
* (主动更新机制实现)
* 返回 fd_face.create_time 字段大于指定时间戳({@code timestamp})的所有记录
* @return 返回查询结果记录的主键
* @see #daoLoadFaceIdByWhere(String)
* @throws RuntimeDaoException
*/
protected List daoLoadFaceIdByCreateTime(Date timestamp)
throws RuntimeDaoException{
return daoLoadFaceIdByWhere(makeWhere(timestamp,"create_time"));
}
//////////// FD_FEATURE /////////
//1
/**
* 根据主键从数据库读取记录,没有找到记录返回{@code null}
*
* @param md5 主键,特征码md5校验码
* @return FeatureBean 对象
* @see IFeatureManager#loadByPrimaryKey(String)
* @throws RuntimeDaoException
*/
protected FeatureBean daoGetFeature(String md5)throws RuntimeDaoException{
return instanceOf(IFeatureManager.class).loadByPrimaryKey(md5);
}
//1-2
/**
* 根据主键从数据库读取记录,没有找到记录抛出异常
*
* @param md5 主键,特征码md5校验码
* @return FeatureBean 对象
* @see IFeatureManager#loadByPrimaryKeyChecked(String)
* @throws RuntimeDaoException
* @throws ObjectRetrievalException 没有找到记录
*/
protected FeatureBean daoGetFeatureChecked(String md5)throws RuntimeDaoException,ObjectRetrievalException{
return instanceOf(IFeatureManager.class).loadByPrimaryKeyChecked(md5);
}
//2
/**
* 根据主键从数据库读取记录
* @return 返回与输入参数下标对应的 FeatureBean 列表,没有查到记录的返回{@code null}
* @see IFeatureManager#loadByPrimaryKey(Collection)
* @throws RuntimeDaoException
*/
protected List daoGetFeatures(Collection md5Collection)
throws RuntimeDaoException{
return instanceOf(IFeatureManager.class).loadByPrimaryKey(md5Collection);
}
//3
/**
* 删除主键列表({@code md5Collection})指定的记录
* @return 返回删除的记录条数
* @see IFeatureManager#deleteByPrimaryKey(Collection)
* @throws RuntimeDaoException
*/
protected int daoDeleteFeaturesByPrimaryKey(Collection md5Collection)
throws RuntimeDaoException{
int count =0;
if(null != md5Collection){
for(String md5:md5Collection){
count += daoDeleteFeature(md5);
}
}
return count;
}
//3-5
/** transformer : FeatureBean to fd_feature.md5 */
protected final Function daoCastFeatureToPk = new Function(){
@Override
public String apply(FeatureBean input) {
return null == input ? null : input.getMd5();
}};
//3-6
/** transformer : fd_feature.md5 to FeatureBean */
protected final Function daoCastFeatureFromPk = new Function(){
@Override
public FeatureBean apply(String input) {
return daoGetFeature(input);
}};
//3-8
/**
* unwrap primary key from {@link FeatureBean}
* if {@code beans} is {@code null},return a empty list(immutable)
*
* @param beans {@link FeatureBean} collection
* @return primary key list
* @see IFeatureManager#toPrimaryKeyList(Collection)
*/
protected List daoToPrimaryKeyListFromFeatures(Collection beans){
if (null == beans){
return ImmutableList.of();
}else{
return instanceOf(IFeatureManager.class).toPrimaryKeyList(beans);
}
}
//3-9
/**
* unwrap primary key from {@link FeatureBean}
*
* the returned list is a transformed view of {@code beans};
* changes to {@code beans} will be reflected in the returned list and vice versa.
*
* if {@code beans} is {@code null},return a empty list(immutable)
* @param beans {@link FeatureBean} list
* @return primary key list
* @see Lists#transform(List, Function)
*/
protected List daoToPrimaryKeyListFromFeatures(List beans){
if(null == beans){
return ImmutableList.of();
}else{
return Lists.transform(beans,daoCastFeatureToPk);
}
}
//4
/**
* 判断主键指定的记录是否存在
*
* @param md5 主键,特征码md5校验码
* @see IFeatureManager#existsPrimaryKey(String)
* @throws RuntimeDaoException
*/
protected boolean daoExistsFeature(String md5)
throws RuntimeDaoException{
return instanceOf(IFeatureManager.class).existsPrimaryKey(md5);
}
//4-2
/**
* 判断指定的记录是否存在
* @see TableManager#existsByPrimaryKey(BaseBean)
* @throws RuntimeDaoException
*/
protected boolean daoExistsFeature(FeatureBean bean)
throws RuntimeDaoException{
return instanceOf(IFeatureManager.class).existsByPrimaryKey(bean);
}
//5
/**
* 删除主键指定的记录
*
* @param md5 主键,特征码md5校验码
* @return 返回删除的记录条数(1),如果记录不存在返回0
* @see IFeatureManager#deleteByPrimaryKey(String)
* @throws RuntimeDaoException
*/
protected int daoDeleteFeature(String md5)
throws RuntimeDaoException{
return instanceOf(IFeatureManager.class).deleteByPrimaryKey(md5);
}
//5-2
/**
* 删除指定的记录
* @param bean 要删除的记录
* @return 返回删除的记录条数(1),如果{@code bean}为{@code null}或记录不存在返回0
* @see #daoDeleteFeature(String)
* @throws RuntimeDaoException
*/
protected int daoDeleteFeature(FeatureBean bean)
throws RuntimeDaoException{
return null == bean ? 0 : daoDeleteFeature(bean.getMd5());
}
//6
/**
* 删除{@code featureBeanCollection}列表指定的记录
* @return 返回删除的记录条数
* @see #daoDeleteFeature(String)
* @throws RuntimeDaoException
*/
protected int daoDeleteFeatures(Collection beans)
throws RuntimeDaoException{
int count =0;
if(null != beans){
for(FeatureBean bean:beans){
count += daoDeleteFeature(bean);
}
}
return count;
}
//7
/**
* 检查数据库中是否有(主键)相同的记录,如果有则抛出异常
* @see TableManager#checkDuplicate(BaseBean)
* @throws RuntimeDaoException
* @throws DuplicateRecordException if exists duplicated row
*/
protected FeatureBean daoCheckDuplicate(FeatureBean featureBean)
throws RuntimeDaoException,DuplicateRecordException{
try{
return instanceOf(IFeatureManager.class).checkDuplicate(featureBean);
}catch(ObjectRetrievalException e){
throw new DuplicateRecordException();
}
}
//7-3
/**
* 检查数据库中是否有(主键)相同的记录,如果有则抛出异常
*
* @param md5 主键,特征码md5校验码
* @see TableManager#checkDuplicate(BaseBean)
* @throws DuplicateRecordException if exists duplicated row
* @return always {@code md5OfFeature}
* @throws RuntimeDaoException
* @throws DuplicateRecordException
*/
protected String daoCheckDuplicateFeature(String md5)
throws RuntimeDaoException,DuplicateRecordException{
if(instanceOf(IFeatureManager.class).existsPrimaryKey(md5)){
throw new DuplicateRecordException();
}
return md5;
}
//8
/**
* 返回外键(fd_face.feature_md5)引用指定记录(fd_feature.md5)的所有{@code fd_face}记录
*
* @param md5OfFeature 主键,特征码md5校验码
* @see IFeatureManager#getFaceBeansByFeatureMd5AsList(String)
* @throws RuntimeDaoException
*/
protected List daoGetFaceBeansByFeatureMd5OnFeature(String md5OfFeature)
throws RuntimeDaoException{
return instanceOf(IFeatureManager.class).getFaceBeansByFeatureMd5AsList(md5OfFeature);
}
//8-2
/**
* 删除外键(md5OfFeature))引用指定记录(fd_feature.md5)的所有{@code fd_face}记录
*
* @param md5OfFeature 主键,特征码md5校验码
* @see IFeatureManager#deleteFaceBeansByFeatureMd5(String)
* @throws RuntimeDaoException
*/
protected int daoDeleteFaceBeansByFeatureMd5OnFeature(String md5OfFeature)
throws RuntimeDaoException{
return instanceOf(IFeatureManager.class).deleteFaceBeansByFeatureMd5(md5OfFeature);
}
//12
/**
* 添加新记录
* fd_feature 表只支持添加删除,不支持修改,所以如果数据库中已经存在相同记录或{@link FeatureBean#isNew()}返回{@code false},则抛出异常
* @param featureBean 要添加的新记录
* @see TableManager#save(BaseBean)
* @see TableManager#checkDuplicate(BaseBean)
* @throws RuntimeDaoException
* @throws DuplicateRecordException if exists duplicated row
* @throws IllegalArgumentException if {@code featureBean.isNew()} is {@code false}
*/
protected FeatureBean daoAddFeature(FeatureBean featureBean)
throws RuntimeDaoException,DuplicateRecordException{
checkArgument(null == featureBean || featureBean.isNew(),"can be add,delete,but modify record for fd_feature,so the _isNew field must be true");
return instanceOf(IFeatureManager.class).save(daoCheckDuplicate(featureBean));
}
//13
/**
* 添加新记录(同步保存)
* fd_feature 表只允许添加删除,不允许修改,所以如果数据库中已经存在相同记录或{@link FeatureBean#isNew()}返回{@code false},则抛出异常
* see also {@link IFeatureManager#save(FeatureBean , Collection )}
* @see TableManager#checkDuplicate(BaseBean)
* @throws RuntimeDaoException
* @throws DuplicateRecordException if exists duplicated row
* @throws IllegalArgumentException if {@code featureBean.isNew()} is {@code false}
*/
protected FeatureBean daoAddFeature(FeatureBean featureBean
, Collection impFaceByFeatureMd5 )throws RuntimeDaoException,DuplicateRecordException{
checkArgument(null == featureBean || featureBean.isNew(),"can be add,delete,but modify record for fd_feature,so the _isNew field must be true");
daoCheckDuplicate(featureBean);
return instanceOf(IFeatureManager.class).save(featureBean
, impFaceByFeatureMd5 );
}
//12-3-3
/**
* 添加新记录
* @param beans 要添加的新记录集合
* @return always {@code beans}
* @see #daoAddFeature(FeatureBean)
* @throws RuntimeDaoException
*/
protected Collection daoAddFeatures(Collection beans)
throws RuntimeDaoException ,DuplicateRecordException{
if(null != beans){
for(FeatureBean bean : beans){
daoAddFeature(bean);
}
}
return beans;
}
//12-3-5
/**
* {@link #daoAddFeatures(Collection)}的事务化版本
* @throws RuntimeDaoException
*/
protected Collection daoAddFeaturesAsTransaction(final Collection beans)
throws RuntimeDaoException ,DuplicateRecordException{
try{
return daoRunAsTransaction(new Callable>(){
@Override
public Collection call() throws Exception {
return daoAddFeatures(beans);
}});
}catch(RuntimeException e){
throwCauseIfInstanceOf(e,DuplicateRecordException.class);
throw e;
}
}
//16
/**
* 查询{@code where} SQL条件语句指定的 fd_feature 记录
* @param where SQL 条件语句,为{@code null}或空时加载所有记录
* @param startRow 返回记录的起始行(首行=1,尾行=-1)
* @param numRows 返回记录条数(小于0时返回所有记录)
* @see IFeatureManager#loadByWhereAsList(String,int[],int,int)
* @throws RuntimeDaoException
*/
protected List daoLoadFeatureByWhere(String where,int startRow, int numRows)
throws RuntimeDaoException{
return instanceOf(IFeatureManager.class).loadByWhereAsList(where,null,startRow,numRows);
}
//16-2
/**
* 以{@code bean} 为模板查询 fd_feature 记录
* @param bean 模板对象
* @param startRow 返回记录的起始行(首行=1,尾行=-1)
* @param numRows 返回记录条数(小于0时返回所有记录)
* @see TableManager#loadUsingTemplate(BaseBean,int,int)
* @throws RuntimeDaoException
*/
protected List daoLoadFeatureUsingTemplate(FeatureBean bean,int startRow, int numRows)
throws RuntimeDaoException{
return instanceOf(IFeatureManager.class).loadUsingTemplateAsList(bean,startRow,numRows);
}
//16-3
/**
* 查询 fd_feature 的{@code column}字段的数据
* @param column 有效的fd_feature表字段名或{@link FeatureBean} 字段名
* @param distinct 只返回不重复记录
* @param where 'where'起始的SQL 查询条件语句,可为{@code null}
* @return {@code column}字段记录
* @param startRow 返回记录的起始行(首行=1,尾行=-1)
* @param numRows 返回记录条数(小于0时返回所有记录)
* @see IFeatureManager#loadColumnAsList(String,boolean,String,int,int)
* @throws RuntimeDaoException
*/
protected List daoLoadColumnOfFeatureAsList(String column,boolean distinct,String where,int startRow, int numRows)
throws RuntimeDaoException{
return instanceOf(IFeatureManager.class).loadColumnAsList(column, distinct, where, startRow, numRows);
}
//17
/**
* 返回 fd_feature 表的所有记录
* @see IFeatureManager#loadAllAsList()
* @throws RuntimeDaoException
*/
protected List daoLoadFeatureAll()
throws RuntimeDaoException{
return instanceOf(IFeatureManager.class).loadAllAsList();
}
//17-2
/**
* 返回满足{@code where} SQL条件语句的 fd_feature 记录总数
* @see TableManager#countWhere(String)
* @throws RuntimeDaoException
*/
protected int daoCountFeatureByWhere(String where)
throws RuntimeDaoException{
return instanceOf(IFeatureManager.class).countWhere(where);
}
//17-3
/**
* 对满足{@code where} SQL条件语句的 fd_feature 记录执行{@code action}
* @param where the sql 'where' clause
* @param action Action object for do something(not null)
* @see {@link IFeatureManager#loadByWhereForAction(String,int[],int,int,Action)}
* @throws RuntimeDaoException
*/
protected int daoLoadFeatureByWhereForAction(String where,TableManager.Action action)
throws RuntimeDaoException{
return instanceOf(IFeatureManager.class).loadByWhereForAction(where,null,null,1,-1,action);
}
//18
/**
* 查询{@code where}条件指定的记录
* @return 返回查询结果记录的主键
* @see #daoLoadFeatureByWhere(String,int,int)
* @throws RuntimeDaoException
*/
protected List daoLoadFeatureMd5ByWhere(String where)
throws RuntimeDaoException{
return daoToPrimaryKeyListFromFeatures(daoLoadFeatureByWhere(where,1,-1));
}
//19
/**
* (主动更新机制实现)
* 返回 fd_feature.create_time 字段大于指定时间戳({@code timestamp})的所有记录
* @see #daoLoadFeatureByWhere(String,int,int)
* @throws RuntimeDaoException
* @throws IllegalArgumentException {@code timestamp}为{@code null}时
*/
protected List daoLoadFeatureByCreateTime(Date timestamp,int startRow, int numRows)
throws RuntimeDaoException{
return daoLoadFeatureByWhere(makeWhere(timestamp,"create_time"),startRow,numRows);
}
//20
/**
* 参见 {@link #daoLoadFeatureByCreateTime(Date,int,int)}
* @throws RuntimeDaoException
*/
protected List daoLoadFeatureByCreateTime(Date timestamp)
throws RuntimeDaoException{
return daoLoadFeatureByCreateTime(timestamp,1,-1);
}
//20-5
/**
* 返回fd_feature.create_time 字段大于指定时间戳({@code timestamp})的记录总数
* @see #daoCountFeatureByWhere(String)
* @throws RuntimeDaoException
*/
protected int daoCountFeatureByCreateTime(Date timestamp)
throws RuntimeDaoException{
return daoCountFeatureByWhere(makeWhere(timestamp,"create_time"));
}
//21
/**
* (主动更新机制实现)
* 返回 fd_feature.create_time 字段大于指定时间戳({@code timestamp})的所有记录
* @return 返回查询结果记录的主键
* @see #daoLoadFeatureMd5ByWhere(String)
* @throws RuntimeDaoException
*/
protected List daoLoadFeatureMd5ByCreateTime(Date timestamp)
throws RuntimeDaoException{
return daoLoadFeatureMd5ByWhere(makeWhere(timestamp,"create_time"));
}
//////////// FD_IMAGE /////////
//1
/**
* 根据主键从数据库读取记录,没有找到记录返回{@code null}
*
* @param md5 主键,图像md5检验码,同时也是从 fd_store 获取图像数据的key
* @return ImageBean 对象
* @see IImageManager#loadByPrimaryKey(String)
* @throws RuntimeDaoException
*/
protected ImageBean daoGetImage(String md5)throws RuntimeDaoException{
return instanceOf(IImageManager.class).loadByPrimaryKey(md5);
}
//1-2
/**
* 根据主键从数据库读取记录,没有找到记录抛出异常
*
* @param md5 主键,图像md5检验码,同时也是从 fd_store 获取图像数据的key
* @return ImageBean 对象
* @see IImageManager#loadByPrimaryKeyChecked(String)
* @throws RuntimeDaoException
* @throws ObjectRetrievalException 没有找到记录
*/
protected ImageBean daoGetImageChecked(String md5)throws RuntimeDaoException,ObjectRetrievalException{
return instanceOf(IImageManager.class).loadByPrimaryKeyChecked(md5);
}
//2
/**
* 根据主键从数据库读取记录
* @return 返回与输入参数下标对应的 ImageBean 列表,没有查到记录的返回{@code null}
* @see IImageManager#loadByPrimaryKey(Collection)
* @throws RuntimeDaoException
*/
protected List daoGetImages(Collection md5Collection)
throws RuntimeDaoException{
return instanceOf(IImageManager.class).loadByPrimaryKey(md5Collection);
}
//3
/**
* 删除主键列表({@code md5Collection})指定的记录
* @return 返回删除的记录条数
* @see IImageManager#deleteByPrimaryKey(Collection)
* @throws RuntimeDaoException
*/
protected int daoDeleteImagesByPrimaryKey(Collection md5Collection)
throws RuntimeDaoException{
int count =0;
if(null != md5Collection){
for(String md5:md5Collection){
count += daoDeleteImage(md5);
}
}
return count;
}
//3-5
/** transformer : ImageBean to fd_image.md5 */
protected final Function daoCastImageToPk = new Function(){
@Override
public String apply(ImageBean input) {
return null == input ? null : input.getMd5();
}};
//3-6
/** transformer : fd_image.md5 to ImageBean */
protected final Function daoCastImageFromPk = new Function(){
@Override
public ImageBean apply(String input) {
return daoGetImage(input);
}};
//3-8
/**
* unwrap primary key from {@link ImageBean}
* if {@code beans} is {@code null},return a empty list(immutable)
*
* @param beans {@link ImageBean} collection
* @return primary key list
* @see IImageManager#toPrimaryKeyList(Collection)
*/
protected List daoToPrimaryKeyListFromImages(Collection beans){
if (null == beans){
return ImmutableList.of();
}else{
return instanceOf(IImageManager.class).toPrimaryKeyList(beans);
}
}
//3-9
/**
* unwrap primary key from {@link ImageBean}
*
* the returned list is a transformed view of {@code beans};
* changes to {@code beans} will be reflected in the returned list and vice versa.
*
* if {@code beans} is {@code null},return a empty list(immutable)
* @param beans {@link ImageBean} list
* @return primary key list
* @see Lists#transform(List, Function)
*/
protected List daoToPrimaryKeyListFromImages(List beans){
if(null == beans){
return ImmutableList.of();
}else{
return Lists.transform(beans,daoCastImageToPk);
}
}
//4
/**
* 判断主键指定的记录是否存在
*
* @param md5 主键,图像md5检验码,同时也是从 fd_store 获取图像数据的key
* @see IImageManager#existsPrimaryKey(String)
* @throws RuntimeDaoException
*/
protected boolean daoExistsImage(String md5)
throws RuntimeDaoException{
return instanceOf(IImageManager.class).existsPrimaryKey(md5);
}
//4-2
/**
* 判断指定的记录是否存在
* @see TableManager#existsByPrimaryKey(BaseBean)
* @throws RuntimeDaoException
*/
protected boolean daoExistsImage(ImageBean bean)
throws RuntimeDaoException{
return instanceOf(IImageManager.class).existsByPrimaryKey(bean);
}
//5
/**
* 删除主键指定的记录
*
* @param md5 主键,图像md5检验码,同时也是从 fd_store 获取图像数据的key
* @return 返回删除的记录条数(1),如果记录不存在返回0
* @see IImageManager#deleteByPrimaryKey(String)
* @throws RuntimeDaoException
*/
protected int daoDeleteImage(String md5)
throws RuntimeDaoException{
return instanceOf(IImageManager.class).deleteByPrimaryKey(md5);
}
//5-2
/**
* 删除指定的记录
* @param bean 要删除的记录
* @return 返回删除的记录条数(1),如果{@code bean}为{@code null}或记录不存在返回0
* @see #daoDeleteImage(String)
* @throws RuntimeDaoException
*/
protected int daoDeleteImage(ImageBean bean)
throws RuntimeDaoException{
return null == bean ? 0 : daoDeleteImage(bean.getMd5());
}
//6
/**
* 删除{@code imageBeanCollection}列表指定的记录
* @return 返回删除的记录条数
* @see #daoDeleteImage(String)
* @throws RuntimeDaoException
*/
protected int daoDeleteImages(Collection beans)
throws RuntimeDaoException{
int count =0;
if(null != beans){
for(ImageBean bean:beans){
count += daoDeleteImage(bean);
}
}
return count;
}
//7
/**
* 检查数据库中是否有(主键)相同的记录,如果有则抛出异常
* @see TableManager#checkDuplicate(BaseBean)
* @throws RuntimeDaoException
* @throws DuplicateRecordException if exists duplicated row
*/
protected ImageBean daoCheckDuplicate(ImageBean imageBean)
throws RuntimeDaoException,DuplicateRecordException{
try{
return instanceOf(IImageManager.class).checkDuplicate(imageBean);
}catch(ObjectRetrievalException e){
throw new DuplicateRecordException();
}
}
//7-3
/**
* 检查数据库中是否有(主键)相同的记录,如果有则抛出异常
*
* @param md5 主键,图像md5检验码,同时也是从 fd_store 获取图像数据的key
* @see TableManager#checkDuplicate(BaseBean)
* @throws DuplicateRecordException if exists duplicated row
* @return always {@code md5OfImage}
* @throws RuntimeDaoException
* @throws DuplicateRecordException
*/
protected String daoCheckDuplicateImage(String md5)
throws RuntimeDaoException,DuplicateRecordException{
if(instanceOf(IImageManager.class).existsPrimaryKey(md5)){
throw new DuplicateRecordException();
}
return md5;
}
//8
/**
* 返回外键(fd_face.image_md5)引用指定记录(fd_image.md5)的所有{@code fd_face}记录
*
* @param md5OfImage 主键,图像md5检验码,同时也是从 fd_store 获取图像数据的key
* @see IImageManager#getFaceBeansByImageMd5AsList(String)
* @throws RuntimeDaoException
*/
protected List daoGetFaceBeansByImageMd5OnImage(String md5OfImage)
throws RuntimeDaoException{
return instanceOf(IImageManager.class).getFaceBeansByImageMd5AsList(md5OfImage);
}
//8-2
/**
* 删除外键(md5OfImage))引用指定记录(fd_image.md5)的所有{@code fd_face}记录
*
* @param md5OfImage 主键,图像md5检验码,同时也是从 fd_store 获取图像数据的key
* @see IImageManager#deleteFaceBeansByImageMd5(String)
* @throws RuntimeDaoException
*/
protected int daoDeleteFaceBeansByImageMd5OnImage(String md5OfImage)
throws RuntimeDaoException{
return instanceOf(IImageManager.class).deleteFaceBeansByImageMd5(md5OfImage);
}
//12
/**
* 添加新记录
* fd_image 表只支持添加删除,不支持修改,所以如果数据库中已经存在相同记录或{@link ImageBean#isNew()}返回{@code false},则抛出异常
* @param imageBean 要添加的新记录
* @see TableManager#save(BaseBean)
* @see TableManager#checkDuplicate(BaseBean)
* @throws RuntimeDaoException
* @throws DuplicateRecordException if exists duplicated row
* @throws IllegalArgumentException if {@code imageBean.isNew()} is {@code false}
*/
protected ImageBean daoAddImage(ImageBean imageBean)
throws RuntimeDaoException,DuplicateRecordException{
checkArgument(null == imageBean || imageBean.isNew(),"can be add,delete,but modify record for fd_image,so the _isNew field must be true");
return instanceOf(IImageManager.class).save(daoCheckDuplicate(imageBean));
}
//13
/**
* 添加新记录(同步保存)
* fd_image 表只允许添加删除,不允许修改,所以如果数据库中已经存在相同记录或{@link ImageBean#isNew()}返回{@code false},则抛出异常
* see also {@link IImageManager#save(ImageBean , Collection )}
* @see TableManager#checkDuplicate(BaseBean)
* @throws RuntimeDaoException
* @throws DuplicateRecordException if exists duplicated row
* @throws IllegalArgumentException if {@code imageBean.isNew()} is {@code false}
*/
protected ImageBean daoAddImage(ImageBean imageBean
, Collection impFaceByImageMd5 )throws RuntimeDaoException,DuplicateRecordException{
checkArgument(null == imageBean || imageBean.isNew(),"can be add,delete,but modify record for fd_image,so the _isNew field must be true");
daoCheckDuplicate(imageBean);
return instanceOf(IImageManager.class).save(imageBean
, impFaceByImageMd5 );
}
//12-3-3
/**
* 添加新记录
* @param beans 要添加的新记录集合
* @return always {@code beans}
* @see #daoAddImage(ImageBean)
* @throws RuntimeDaoException
*/
protected Collection daoAddImages(Collection beans)
throws RuntimeDaoException ,DuplicateRecordException{
if(null != beans){
for(ImageBean bean : beans){
daoAddImage(bean);
}
}
return beans;
}
//12-3-5
/**
* {@link #daoAddImages(Collection)}的事务化版本
* @throws RuntimeDaoException
*/
protected Collection daoAddImagesAsTransaction(final Collection beans)
throws RuntimeDaoException ,DuplicateRecordException{
try{
return daoRunAsTransaction(new Callable>(){
@Override
public Collection call() throws Exception {
return daoAddImages(beans);
}});
}catch(RuntimeException e){
throwCauseIfInstanceOf(e,DuplicateRecordException.class);
throw e;
}
}
//16
/**
* 查询{@code where} SQL条件语句指定的 fd_image 记录
* @param where SQL 条件语句,为{@code null}或空时加载所有记录
* @param startRow 返回记录的起始行(首行=1,尾行=-1)
* @param numRows 返回记录条数(小于0时返回所有记录)
* @see IImageManager#loadByWhereAsList(String,int[],int,int)
* @throws RuntimeDaoException
*/
protected List daoLoadImageByWhere(String where,int startRow, int numRows)
throws RuntimeDaoException{
return instanceOf(IImageManager.class).loadByWhereAsList(where,null,startRow,numRows);
}
//16-2
/**
* 以{@code bean} 为模板查询 fd_image 记录
* @param bean 模板对象
* @param startRow 返回记录的起始行(首行=1,尾行=-1)
* @param numRows 返回记录条数(小于0时返回所有记录)
* @see TableManager#loadUsingTemplate(BaseBean,int,int)
* @throws RuntimeDaoException
*/
protected List daoLoadImageUsingTemplate(ImageBean bean,int startRow, int numRows)
throws RuntimeDaoException{
return instanceOf(IImageManager.class).loadUsingTemplateAsList(bean,startRow,numRows);
}
//16-3
/**
* 查询 fd_image 的{@code column}字段的数据
* @param column 有效的fd_image表字段名或{@link ImageBean} 字段名
* @param distinct 只返回不重复记录
* @param where 'where'起始的SQL 查询条件语句,可为{@code null}
* @return {@code column}字段记录
* @param startRow 返回记录的起始行(首行=1,尾行=-1)
* @param numRows 返回记录条数(小于0时返回所有记录)
* @see IImageManager#loadColumnAsList(String,boolean,String,int,int)
* @throws RuntimeDaoException
*/
protected List daoLoadColumnOfImageAsList(String column,boolean distinct,String where,int startRow, int numRows)
throws RuntimeDaoException{
return instanceOf(IImageManager.class).loadColumnAsList(column, distinct, where, startRow, numRows);
}
//17
/**
* 返回 fd_image 表的所有记录
* @see IImageManager#loadAllAsList()
* @throws RuntimeDaoException
*/
protected List daoLoadImageAll()
throws RuntimeDaoException{
return instanceOf(IImageManager.class).loadAllAsList();
}
//17-2
/**
* 返回满足{@code where} SQL条件语句的 fd_image 记录总数
* @see TableManager#countWhere(String)
* @throws RuntimeDaoException
*/
protected int daoCountImageByWhere(String where)
throws RuntimeDaoException{
return instanceOf(IImageManager.class).countWhere(where);
}
//17-3
/**
* 对满足{@code where} SQL条件语句的 fd_image 记录执行{@code action}
* @param where the sql 'where' clause
* @param action Action object for do something(not null)
* @see {@link IImageManager#loadByWhereForAction(String,int[],int,int,Action)}
* @throws RuntimeDaoException
*/
protected int daoLoadImageByWhereForAction(String where,TableManager.Action action)
throws RuntimeDaoException{
return instanceOf(IImageManager.class).loadByWhereForAction(where,null,null,1,-1,action);
}
//18
/**
* 查询{@code where}条件指定的记录
* @return 返回查询结果记录的主键
* @see #daoLoadImageByWhere(String,int,int)
* @throws RuntimeDaoException
*/
protected List daoLoadImageMd5ByWhere(String where)
throws RuntimeDaoException{
return daoToPrimaryKeyListFromImages(daoLoadImageByWhere(where,1,-1));
}
//19
/**
* (主动更新机制实现)
* 返回 fd_image.create_time 字段大于指定时间戳({@code timestamp})的所有记录
* @see #daoLoadImageByWhere(String,int,int)
* @throws RuntimeDaoException
* @throws IllegalArgumentException {@code timestamp}为{@code null}时
*/
protected List daoLoadImageByCreateTime(Date timestamp,int startRow, int numRows)
throws RuntimeDaoException{
return daoLoadImageByWhere(makeWhere(timestamp,"create_time"),startRow,numRows);
}
//20
/**
* 参见 {@link #daoLoadImageByCreateTime(Date,int,int)}
* @throws RuntimeDaoException
*/
protected List daoLoadImageByCreateTime(Date timestamp)
throws RuntimeDaoException{
return daoLoadImageByCreateTime(timestamp,1,-1);
}
//20-5
/**
* 返回fd_image.create_time 字段大于指定时间戳({@code timestamp})的记录总数
* @see #daoCountImageByWhere(String)
* @throws RuntimeDaoException
*/
protected int daoCountImageByCreateTime(Date timestamp)
throws RuntimeDaoException{
return daoCountImageByWhere(makeWhere(timestamp,"create_time"));
}
//21
/**
* (主动更新机制实现)
* 返回 fd_image.create_time 字段大于指定时间戳({@code timestamp})的所有记录
* @return 返回查询结果记录的主键
* @see #daoLoadImageMd5ByWhere(String)
* @throws RuntimeDaoException
*/
protected List daoLoadImageMd5ByCreateTime(Date timestamp)
throws RuntimeDaoException{
return daoLoadImageMd5ByWhere(makeWhere(timestamp,"create_time"));
}
//19
/**
* (主动更新机制实现)
* 返回 fd_image.update_time 字段大于指定时间戳({@code timestamp})的所有记录
* @see #daoLoadImageByWhere(String,int,int)
* @throws RuntimeDaoException
* @throws IllegalArgumentException {@code timestamp}为{@code null}时
*/
protected List daoLoadImageByUpdateTime(Date timestamp,int startRow, int numRows)
throws RuntimeDaoException{
return daoLoadImageByWhere(makeWhere(timestamp,"update_time"),startRow,numRows);
}
//20
/**
* 参见 {@link #daoLoadImageByUpdateTime(Date,int,int)}
* @throws RuntimeDaoException
*/
protected List daoLoadImageByUpdateTime(Date timestamp)
throws RuntimeDaoException{
return daoLoadImageByUpdateTime(timestamp,1,-1);
}
//20-5
/**
* 返回fd_image.update_time 字段大于指定时间戳({@code timestamp})的记录总数
* @see #daoCountImageByWhere(String)
* @throws RuntimeDaoException
*/
protected int daoCountImageByUpdateTime(Date timestamp)
throws RuntimeDaoException{
return daoCountImageByWhere(makeWhere(timestamp,"update_time"));
}
//21
/**
* (主动更新机制实现)
* 返回 fd_image.update_time 字段大于指定时间戳({@code timestamp})的所有记录
* @return 返回查询结果记录的主键
* @see #daoLoadImageMd5ByWhere(String)
* @throws RuntimeDaoException
*/
protected List daoLoadImageMd5ByUpdateTime(Date timestamp)
throws RuntimeDaoException{
return daoLoadImageMd5ByWhere(makeWhere(timestamp,"update_time"));
}
//////////// FD_STORE /////////
//1
/**
* 根据主键从数据库读取记录,没有找到记录返回{@code null}
*
* @param md5 主键,md5检验码
* @return StoreBean 对象
* @see IStoreManager#loadByPrimaryKey(String)
* @throws RuntimeDaoException
*/
protected StoreBean daoGetStore(String md5)throws RuntimeDaoException{
return instanceOf(IStoreManager.class).loadByPrimaryKey(md5);
}
//1-2
/**
* 根据主键从数据库读取记录,没有找到记录抛出异常
*
* @param md5 主键,md5检验码
* @return StoreBean 对象
* @see IStoreManager#loadByPrimaryKeyChecked(String)
* @throws RuntimeDaoException
* @throws ObjectRetrievalException 没有找到记录
*/
protected StoreBean daoGetStoreChecked(String md5)throws RuntimeDaoException,ObjectRetrievalException{
return instanceOf(IStoreManager.class).loadByPrimaryKeyChecked(md5);
}
//2
/**
* 根据主键从数据库读取记录
* @return 返回与输入参数下标对应的 StoreBean 列表,没有查到记录的返回{@code null}
* @see IStoreManager#loadByPrimaryKey(Collection)
* @throws RuntimeDaoException
*/
protected List daoGetStores(Collection md5Collection)
throws RuntimeDaoException{
return instanceOf(IStoreManager.class).loadByPrimaryKey(md5Collection);
}
//3
/**
* 删除主键列表({@code md5Collection})指定的记录
* @return 返回删除的记录条数
* @see IStoreManager#deleteByPrimaryKey(Collection)
* @throws RuntimeDaoException
*/
protected int daoDeleteStoresByPrimaryKey(Collection md5Collection)
throws RuntimeDaoException{
int count =0;
if(null != md5Collection){
for(String md5:md5Collection){
count += daoDeleteStore(md5);
}
}
return count;
}
//3-5
/** transformer : StoreBean to fd_store.md5 */
protected final Function daoCastStoreToPk = new Function(){
@Override
public String apply(StoreBean input) {
return null == input ? null : input.getMd5();
}};
//3-6
/** transformer : fd_store.md5 to StoreBean */
protected final Function daoCastStoreFromPk = new Function(){
@Override
public StoreBean apply(String input) {
return daoGetStore(input);
}};
//3-8
/**
* unwrap primary key from {@link StoreBean}
* if {@code beans} is {@code null},return a empty list(immutable)
*
* @param beans {@link StoreBean} collection
* @return primary key list
* @see IStoreManager#toPrimaryKeyList(Collection)
*/
protected List daoToPrimaryKeyListFromStores(Collection beans){
if (null == beans){
return ImmutableList.of();
}else{
return instanceOf(IStoreManager.class).toPrimaryKeyList(beans);
}
}
//3-9
/**
* unwrap primary key from {@link StoreBean}
*
* the returned list is a transformed view of {@code beans};
* changes to {@code beans} will be reflected in the returned list and vice versa.
*
* if {@code beans} is {@code null},return a empty list(immutable)
* @param beans {@link StoreBean} list
* @return primary key list
* @see Lists#transform(List, Function)
*/
protected List daoToPrimaryKeyListFromStores(List beans){
if(null == beans){
return ImmutableList.of();
}else{
return Lists.transform(beans,daoCastStoreToPk);
}
}
//4
/**
* 判断主键指定的记录是否存在
*
* @param md5 主键,md5检验码
* @see IStoreManager#existsPrimaryKey(String)
* @throws RuntimeDaoException
*/
protected boolean daoExistsStore(String md5)
throws RuntimeDaoException{
return instanceOf(IStoreManager.class).existsPrimaryKey(md5);
}
//4-2
/**
* 判断指定的记录是否存在
* @see TableManager#existsByPrimaryKey(BaseBean)
* @throws RuntimeDaoException
*/
protected boolean daoExistsStore(StoreBean bean)
throws RuntimeDaoException{
return instanceOf(IStoreManager.class).existsByPrimaryKey(bean);
}
//5
/**
* 删除主键指定的记录
*
* @param md5 主键,md5检验码
* @return 返回删除的记录条数(1),如果记录不存在返回0
* @see IStoreManager#deleteByPrimaryKey(String)
* @throws RuntimeDaoException
*/
protected int daoDeleteStore(String md5)
throws RuntimeDaoException{
return instanceOf(IStoreManager.class).deleteByPrimaryKey(md5);
}
//5-2
/**
* 删除指定的记录
* @param bean 要删除的记录
* @return 返回删除的记录条数(1),如果{@code bean}为{@code null}或记录不存在返回0
* @see #daoDeleteStore(String)
* @throws RuntimeDaoException
*/
protected int daoDeleteStore(StoreBean bean)
throws RuntimeDaoException{
return null == bean ? 0 : daoDeleteStore(bean.getMd5());
}
//6
/**
* 删除{@code storeBeanCollection}列表指定的记录
* @return 返回删除的记录条数
* @see #daoDeleteStore(String)
* @throws RuntimeDaoException
*/
protected int daoDeleteStores(Collection beans)
throws RuntimeDaoException{
int count =0;
if(null != beans){
for(StoreBean bean:beans){
count += daoDeleteStore(bean);
}
}
return count;
}
//7
/**
* 检查数据库中是否有(主键)相同的记录,如果有则抛出异常
* @see TableManager#checkDuplicate(BaseBean)
* @throws RuntimeDaoException
* @throws DuplicateRecordException if exists duplicated row
*/
protected StoreBean daoCheckDuplicate(StoreBean storeBean)
throws RuntimeDaoException,DuplicateRecordException{
try{
return instanceOf(IStoreManager.class).checkDuplicate(storeBean);
}catch(ObjectRetrievalException e){
throw new DuplicateRecordException();
}
}
//7-3
/**
* 检查数据库中是否有(主键)相同的记录,如果有则抛出异常
*
* @param md5 主键,md5检验码
* @see TableManager#checkDuplicate(BaseBean)
* @throws DuplicateRecordException if exists duplicated row
* @return always {@code md5OfStore}
* @throws RuntimeDaoException
* @throws DuplicateRecordException
*/
protected String daoCheckDuplicateStore(String md5)
throws RuntimeDaoException,DuplicateRecordException{
if(instanceOf(IStoreManager.class).existsPrimaryKey(md5)){
throw new DuplicateRecordException();
}
return md5;
}
//12
/**
* 添加新记录
* fd_store 表只支持添加删除,不支持修改,所以如果数据库中已经存在相同记录或{@link StoreBean#isNew()}返回{@code false},则抛出异常
* @param storeBean 要添加的新记录
* @see TableManager#save(BaseBean)
* @see TableManager#checkDuplicate(BaseBean)
* @throws RuntimeDaoException
* @throws DuplicateRecordException if exists duplicated row
* @throws IllegalArgumentException if {@code storeBean.isNew()} is {@code false}
*/
protected StoreBean daoAddStore(StoreBean storeBean)
throws RuntimeDaoException,DuplicateRecordException{
checkArgument(null == storeBean || storeBean.isNew(),"can be add,delete,but modify record for fd_store,so the _isNew field must be true");
return instanceOf(IStoreManager.class).save(daoCheckDuplicate(storeBean));
}
//12-3-3
/**
* 添加新记录
* @param beans 要添加的新记录集合
* @return always {@code beans}
* @see #daoAddStore(StoreBean)
* @throws RuntimeDaoException
*/
protected Collection daoAddStores(Collection beans)
throws RuntimeDaoException ,DuplicateRecordException{
if(null != beans){
for(StoreBean bean : beans){
daoAddStore(bean);
}
}
return beans;
}
//12-3-5
/**
* {@link #daoAddStores(Collection)}的事务化版本
* @throws RuntimeDaoException
*/
protected Collection daoAddStoresAsTransaction(final Collection beans)
throws RuntimeDaoException ,DuplicateRecordException{
try{
return daoRunAsTransaction(new Callable>(){
@Override
public Collection call() throws Exception {
return daoAddStores(beans);
}});
}catch(RuntimeException e){
throwCauseIfInstanceOf(e,DuplicateRecordException.class);
throw e;
}
}
//16
/**
* 查询{@code where} SQL条件语句指定的 fd_store 记录
* @param where SQL 条件语句,为{@code null}或空时加载所有记录
* @param startRow 返回记录的起始行(首行=1,尾行=-1)
* @param numRows 返回记录条数(小于0时返回所有记录)
* @see IStoreManager#loadByWhereAsList(String,int[],int,int)
* @throws RuntimeDaoException
*/
protected List daoLoadStoreByWhere(String where,int startRow, int numRows)
throws RuntimeDaoException{
return instanceOf(IStoreManager.class).loadByWhereAsList(where,null,startRow,numRows);
}
//16-2
/**
* 以{@code bean} 为模板查询 fd_store 记录
* @param bean 模板对象
* @param startRow 返回记录的起始行(首行=1,尾行=-1)
* @param numRows 返回记录条数(小于0时返回所有记录)
* @see TableManager#loadUsingTemplate(BaseBean,int,int)
* @throws RuntimeDaoException
*/
protected List daoLoadStoreUsingTemplate(StoreBean bean,int startRow, int numRows)
throws RuntimeDaoException{
return instanceOf(IStoreManager.class).loadUsingTemplateAsList(bean,startRow,numRows);
}
//16-3
/**
* 查询 fd_store 的{@code column}字段的数据
* @param column 有效的fd_store表字段名或{@link StoreBean} 字段名
* @param distinct 只返回不重复记录
* @param where 'where'起始的SQL 查询条件语句,可为{@code null}
* @return {@code column}字段记录
* @param startRow 返回记录的起始行(首行=1,尾行=-1)
* @param numRows 返回记录条数(小于0时返回所有记录)
* @see IStoreManager#loadColumnAsList(String,boolean,String,int,int)
* @throws RuntimeDaoException
*/
protected List daoLoadColumnOfStoreAsList(String column,boolean distinct,String where,int startRow, int numRows)
throws RuntimeDaoException{
return instanceOf(IStoreManager.class).loadColumnAsList(column, distinct, where, startRow, numRows);
}
//17
/**
* 返回 fd_store 表的所有记录
* @see IStoreManager#loadAllAsList()
* @throws RuntimeDaoException
*/
protected List daoLoadStoreAll()
throws RuntimeDaoException{
return instanceOf(IStoreManager.class).loadAllAsList();
}
//17-2
/**
* 返回满足{@code where} SQL条件语句的 fd_store 记录总数
* @see TableManager#countWhere(String)
* @throws RuntimeDaoException
*/
protected int daoCountStoreByWhere(String where)
throws RuntimeDaoException{
return instanceOf(IStoreManager.class).countWhere(where);
}
//17-3
/**
* 对满足{@code where} SQL条件语句的 fd_store 记录执行{@code action}
* @param where the sql 'where' clause
* @param action Action object for do something(not null)
* @see {@link IStoreManager#loadByWhereForAction(String,int[],int,int,Action)}
* @throws RuntimeDaoException
*/
protected int daoLoadStoreByWhereForAction(String where,TableManager.Action action)
throws RuntimeDaoException{
return instanceOf(IStoreManager.class).loadByWhereForAction(where,null,null,1,-1,action);
}
//18
/**
* 查询{@code where}条件指定的记录
* @return 返回查询结果记录的主键
* @see #daoLoadStoreByWhere(String,int,int)
* @throws RuntimeDaoException
*/
protected List daoLoadStoreMd5ByWhere(String where)
throws RuntimeDaoException{
return daoToPrimaryKeyListFromStores(daoLoadStoreByWhere(where,1,-1));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy