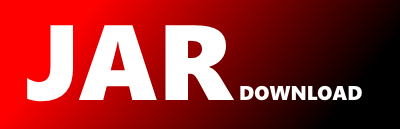
net.gdface.facedb.thrift.FaceDbThriftClient Maven / Gradle / Ivy
// ______________________________________________________
// Generated by codegen - https://gitee.com/l0km/codegen
// template: thrift/client_thrifty/perservice/client.service.decorator.class.vm
// ______________________________________________________
package net.gdface.facedb.thrift;
import java.nio.ByteBuffer;
import java.util.Date;
import java.util.List;
import java.util.Map;
import net.gdface.exception.NotFoundBeanException;
import net.gdface.facedb.DuplicateRecordException;
import net.gdface.facedb.FaceDb;
import net.gdface.facedb.SearchResult;
import net.gdface.facedb.db.FaceBean;
import net.gdface.facedb.db.FeatureBean;
import net.gdface.facedb.db.ImageBean;
import net.gdface.image.ImageErrorException;
import net.gdface.sdk.CodeInfo;
import net.gdface.sdk.FRect;
import net.gdface.sdk.NotFaceDetectedException;
import net.gdface.thrift.TypeTransformer;
import java.io.IOException;
import java.util.concurrent.atomic.AtomicReference;
import net.gdface.thrift.ClientFactory;
import com.google.common.base.Function;
import com.google.common.base.Throwables;
import com.microsoft.thrifty.service.AsyncClientBase;
import com.microsoft.thrifty.service.ServiceMethodCallback;
import static com.google.common.base.Preconditions.*;
import static net.gdface.thrift.ThriftUtils.returnNull;
/**
* 基于thrift/swift框架生成的client端代码提供{@link FaceDb}接口的RPC实现(线程安全)
* 转发所有{@link FaceDb}接口方法到{@link net.gdface.facedb.thrift.client.FaceDbClient}实例
* 所有服务端抛出的{@link RuntimeException}异常被封装到{@link ServiceRuntimeException}中抛出
* Example:
*
* FaceDbThriftClient thriftInstance = ClientFactory
* .builder()
* .setHostAndPort("127.0.0.1",26413)
* .build(FaceDbThriftClient.class);
*
* 计算机生成代码(generated by automated tools ThriftServiceDecoratorGenerator @author guyadong)
* @author guyadong
*
*/
public class FaceDbThriftClient implements FaceDb {
private final ClientFactory factory;
public ClientFactory getFactory() {
return factory;
}
public FaceDbThriftClient(ClientFactory factory) {
super();
this.factory = checkNotNull(factory,"factory is null");
}
/**
* 服务异步调用接口
*
* @param
*/
private interface ServiceAsyncCall {
public void call(net.gdface.facedb.thrift.client.FaceDbClient service,ServiceMethodCallback callback);
}
/**
* 异常调用转同步调用实现
* @param transformer
* @param serviceCall
* @return
* @throws Throwable
*/
private R syncCall(final Function transformer,final ServiceAsyncCall serviceCall) throws Throwable{
final AtomicReference res = new AtomicReference(null);
final AtomicReference err = new AtomicReference(null);
/* 异步调用结束标志信号量 */
final Object lock = new Object();
final ServiceMethodCallback callback = new ServiceMethodCallback() {
@Override
public void onSuccess(L result) {
res.set(transformer.apply(result));
/* 异步调用结束,唤醒等待线程 */
synchronized(lock){
lock.notifyAll();
}
}
@Override
public void onError(Throwable error) {
err.set(error);
/* 异步调用结束,唤醒等待线程 */
synchronized(lock){
lock.notifyAll();
}
}
};
/* 连接关闭侦听器当检测到异常时调用callback来处理 */
AsyncClientBase.Listener closeListener = new AsyncClientBase.Listener(){
@Override
public void onTransportClosed() {
}
@Override
public void onError(Throwable error) {
callback.onError(error);
}};
net.gdface.facedb.thrift.client.FaceDbClient service = factory.applyInstance(net.gdface.facedb.thrift.client.FaceDbClient.class,closeListener);
synchronized(lock){
try {
serviceCall.call(service,callback);
/* 等待异步调用结束 */
lock.wait();
} catch (InterruptedException e) {
err.set(e);
} finally{
try {
/* 调用结束关闭连接 */
service.close();
} catch (IOException e) {}
}
}
Throwable e = err.get();
if(null != e){
/** 判断异常类型返回null */
returnNull(e);
}
return res.get();
}
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
builder.append("FaceDbThriftClient [factory=");
builder.append(factory);
builder.append(",interface=");
builder.append(FaceDb.class.getName());
builder.append("]");
return builder.toString();
}
@Override
public FeatureBean addFeature(final byte[] feature,
final Map faces)
throws DuplicateRecordException{
try{
return syncCall(new Function() {
@Override
public FeatureBean apply(net.gdface.facedb.thrift.client.FeatureBean input) {
return TypeTransformer.getInstance().to(
input,
net.gdface.facedb.thrift.client.FeatureBean.class,
FeatureBean.class);
}},
new ServiceAsyncCall(){
@Override
public void call(net.gdface.facedb.thrift.client.FaceDbClient service,ServiceMethodCallback nativeCallback){
service.addFeature(TypeTransformer.getInstance().to(
feature,
byte[].class,
okio.ByteString.class),TypeTransformer.getInstance().to(
faces,
ByteBuffer.class,
CodeInfo.class,
okio.ByteString.class,
net.gdface.facedb.thrift.client.CodeInfo.class),nativeCallback);
}});
}
catch(net.gdface.facedb.thrift.client.DuplicateRecordException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.DuplicateRecordException.class,
DuplicateRecordException.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch (Throwable e) {
Throwables.throwIfUnchecked(e);
throw new RuntimeException(e);
}
}
@Override
public ImageBean addImage(final byte[] imgData,
final List features)
throws DuplicateRecordException{
try{
return syncCall(new Function() {
@Override
public ImageBean apply(net.gdface.facedb.thrift.client.ImageBean input) {
return TypeTransformer.getInstance().to(
input,
net.gdface.facedb.thrift.client.ImageBean.class,
ImageBean.class);
}},
new ServiceAsyncCall(){
@Override
public void call(net.gdface.facedb.thrift.client.FaceDbClient service,ServiceMethodCallback nativeCallback){
service.addImage(TypeTransformer.getInstance().to(
imgData,
byte[].class,
okio.ByteString.class),TypeTransformer.getInstance().to(
features,
CodeInfo.class,
net.gdface.facedb.thrift.client.CodeInfo.class),nativeCallback);
}});
}
catch(net.gdface.facedb.thrift.client.DuplicateRecordException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.DuplicateRecordException.class,
DuplicateRecordException.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch (Throwable e) {
Throwables.throwIfUnchecked(e);
throw new RuntimeException(e);
}
}
@Override
public ImageBean addImageIfAbsent(final byte[] imgData,
final CodeInfo code,
final double similarty)
throws ImageErrorException,NotFaceDetectedException{
try{
return syncCall(new Function() {
@Override
public ImageBean apply(net.gdface.facedb.thrift.client.ImageBean input) {
return TypeTransformer.getInstance().to(
input,
net.gdface.facedb.thrift.client.ImageBean.class,
ImageBean.class);
}},
new ServiceAsyncCall(){
@Override
public void call(net.gdface.facedb.thrift.client.FaceDbClient service,ServiceMethodCallback nativeCallback){
service.addImageIfAbsent(TypeTransformer.getInstance().to(
imgData,
byte[].class,
okio.ByteString.class),TypeTransformer.getInstance().to(
code,
CodeInfo.class,
net.gdface.facedb.thrift.client.CodeInfo.class),similarty,nativeCallback);
}});
}
catch(net.gdface.facedb.thrift.client.ImageErrorException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.ImageErrorException.class,
ImageErrorException.class);
}
catch(net.gdface.facedb.thrift.client.NotFaceDetectedException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.NotFaceDetectedException.class,
NotFaceDetectedException.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch (Throwable e) {
Throwables.throwIfUnchecked(e);
throw new RuntimeException(e);
}
}
@Override
public double[] compareFaces(final String featureId,
final byte[] imgData,
final CodeInfo[] facePos)
throws NotFoundBeanException,NotFaceDetectedException{
try{
return syncCall(new Function,double[]>() {
@Override
public double[] apply(java.util.List input) {
return TypeTransformer.getInstance().todoubleArray(
input,
double.class,
double.class);
}},
new ServiceAsyncCall>(){
@Override
public void call(net.gdface.facedb.thrift.client.FaceDbClient service,ServiceMethodCallback> nativeCallback){
service.compareFaces(featureId,TypeTransformer.getInstance().to(
imgData,
byte[].class,
okio.ByteString.class),TypeTransformer.getInstance().to(
facePos,
CodeInfo.class,
net.gdface.facedb.thrift.client.CodeInfo.class),nativeCallback);
}});
}
catch(net.gdface.facedb.thrift.client.NotFoundBeanException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.NotFoundBeanException.class,
NotFoundBeanException.class);
}
catch(net.gdface.facedb.thrift.client.NotFaceDetectedException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.NotFaceDetectedException.class,
NotFaceDetectedException.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch (Throwable e) {
Throwables.throwIfUnchecked(e);
throw new RuntimeException(e);
}
}
@Override
public double compareFeature(final String featureId,
final byte[] feature)
throws NotFoundBeanException{
try{
return syncCall(new Function() {
@Override
public Double apply(Double input) {
return input;
}},
new ServiceAsyncCall(){
@Override
public void call(net.gdface.facedb.thrift.client.FaceDbClient service,ServiceMethodCallback nativeCallback){
service.compareFeature(featureId,TypeTransformer.getInstance().to(
feature,
byte[].class,
okio.ByteString.class),nativeCallback);
}});
}
catch(net.gdface.facedb.thrift.client.NotFoundBeanException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.NotFoundBeanException.class,
NotFoundBeanException.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch (Throwable e) {
Throwables.throwIfUnchecked(e);
throw new RuntimeException(e);
}
}
@Override
public double compareFeature(final String featureId1,
final String featureId2)
throws NotFoundBeanException{
try{
return syncCall(new Function() {
@Override
public Double apply(Double input) {
return input;
}},
new ServiceAsyncCall(){
@Override
public void call(net.gdface.facedb.thrift.client.FaceDbClient service,ServiceMethodCallback nativeCallback){
service.compareFeatureByFeatureId(featureId1,featureId2,nativeCallback);
}});
}
catch(net.gdface.facedb.thrift.client.NotFoundBeanException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.NotFoundBeanException.class,
NotFoundBeanException.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch (Throwable e) {
Throwables.throwIfUnchecked(e);
throw new RuntimeException(e);
}
}
@Override
public double[] compareFeatures(final String featureId,
final CodeInfo[] features)
throws NotFoundBeanException{
try{
return syncCall(new Function,double[]>() {
@Override
public double[] apply(java.util.List input) {
return TypeTransformer.getInstance().todoubleArray(
input,
double.class,
double.class);
}},
new ServiceAsyncCall>(){
@Override
public void call(net.gdface.facedb.thrift.client.FaceDbClient service,ServiceMethodCallback> nativeCallback){
service.compareFeatures(featureId,TypeTransformer.getInstance().to(
features,
CodeInfo.class,
net.gdface.facedb.thrift.client.CodeInfo.class),nativeCallback);
}});
}
catch(net.gdface.facedb.thrift.client.NotFoundBeanException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.NotFoundBeanException.class,
NotFoundBeanException.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch (Throwable e) {
Throwables.throwIfUnchecked(e);
throw new RuntimeException(e);
}
}
@Override
public boolean deleteFeature(final String featureId,
final boolean cascade)
{
try{
return syncCall(new Function() {
@Override
public Boolean apply(Boolean input) {
return input;
}},
new ServiceAsyncCall(){
@Override
public void call(net.gdface.facedb.thrift.client.FaceDbClient service,ServiceMethodCallback nativeCallback){
service.deleteFeature(featureId,cascade,nativeCallback);
}});
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch (Throwable e) {
Throwables.throwIfUnchecked(e);
throw new RuntimeException(e);
}
}
@Override
public int deleteFeatures(final List featureIdList,
final boolean cascade)
{
try{
return syncCall(new Function() {
@Override
public Integer apply(Integer input) {
return input;
}},
new ServiceAsyncCall(){
@Override
public void call(net.gdface.facedb.thrift.client.FaceDbClient service,ServiceMethodCallback nativeCallback){
service.deleteFeatures(TypeTransformer.getInstance().to(
featureIdList,
String.class,
String.class),cascade,nativeCallback);
}});
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch (Throwable e) {
Throwables.throwIfUnchecked(e);
throw new RuntimeException(e);
}
}
@Override
public boolean deleteImage(final String imgMd5,
final boolean cascade)
{
try{
return syncCall(new Function() {
@Override
public Boolean apply(Boolean input) {
return input;
}},
new ServiceAsyncCall(){
@Override
public void call(net.gdface.facedb.thrift.client.FaceDbClient service,ServiceMethodCallback nativeCallback){
service.deleteImage(imgMd5,cascade,nativeCallback);
}});
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch (Throwable e) {
Throwables.throwIfUnchecked(e);
throw new RuntimeException(e);
}
}
@Override
public int deleteImages(final List imgMd5List,
final boolean cascade)
{
try{
return syncCall(new Function() {
@Override
public Integer apply(Integer input) {
return input;
}},
new ServiceAsyncCall(){
@Override
public void call(net.gdface.facedb.thrift.client.FaceDbClient service,ServiceMethodCallback nativeCallback){
service.deleteImages(TypeTransformer.getInstance().to(
imgMd5List,
String.class,
String.class),cascade,nativeCallback);
}});
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch (Throwable e) {
Throwables.throwIfUnchecked(e);
throw new RuntimeException(e);
}
}
@Override
public ImageBean detectAndAddFeatures(final byte[] imgData,
final int faceNum,
final FRect detectRectangle)
throws DuplicateRecordException,ImageErrorException,NotFaceDetectedException{
try{
return syncCall(new Function() {
@Override
public ImageBean apply(net.gdface.facedb.thrift.client.ImageBean input) {
return TypeTransformer.getInstance().to(
input,
net.gdface.facedb.thrift.client.ImageBean.class,
ImageBean.class);
}},
new ServiceAsyncCall(){
@Override
public void call(net.gdface.facedb.thrift.client.FaceDbClient service,ServiceMethodCallback nativeCallback){
service.detectAndAddFeatures(TypeTransformer.getInstance().to(
imgData,
byte[].class,
okio.ByteString.class),faceNum,TypeTransformer.getInstance().to(
detectRectangle,
FRect.class,
net.gdface.facedb.thrift.client.FRect.class),nativeCallback);
}});
}
catch(net.gdface.facedb.thrift.client.DuplicateRecordException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.DuplicateRecordException.class,
DuplicateRecordException.class);
}
catch(net.gdface.facedb.thrift.client.ImageErrorException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.ImageErrorException.class,
ImageErrorException.class);
}
catch(net.gdface.facedb.thrift.client.NotFaceDetectedException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facedb.thrift.client.NotFaceDetectedException.class,
NotFaceDetectedException.class);
}
catch(net.gdface.facedb.thrift.client.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch (Throwable e) {
Throwables.throwIfUnchecked(e);
throw new RuntimeException(e);
}
}
@Override
public Map detectAndCompareFaces(final String featureId,
final byte[] imgData,
final int faceNum,
final FRect detectRectangle)
throws NotFoundBeanException,ImageErrorException,NotFaceDetectedException{
try{
return syncCall(new Function
© 2015 - 2024 Weber Informatics LLC | Privacy Policy