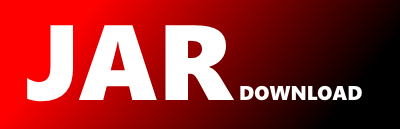
net.gdface.facedb.thrift.client.EyeInfo Maven / Gradle / Ivy
// Automatically generated by the Thrifty compiler; do not edit!
// Generated on: 2019-09-24T07:53:45.880Z
// Source: J:\facedb\FaceDb.thrift at 15:1
package net.gdface.facedb.thrift.client;
import com.microsoft.thrifty.Adapter;
import com.microsoft.thrifty.Struct;
import com.microsoft.thrifty.StructBuilder;
import com.microsoft.thrifty.TType;
import com.microsoft.thrifty.ThriftField;
import com.microsoft.thrifty.protocol.FieldMetadata;
import com.microsoft.thrifty.protocol.Protocol;
import com.microsoft.thrifty.util.ProtocolUtil;
import java.io.IOException;
import javax.annotation.Generated;
@Generated(
value = "com.microsoft.thrifty.gen.ThriftyCodeGenerator",
comments = "https://github.com/microsoft/thrifty"
)
public final class EyeInfo implements Struct {
public static final Adapter ADAPTER = new EyeInfoAdapter();
@ThriftField(
fieldId = 1,
isRequired = true
)
public final Integer leftx;
@ThriftField(
fieldId = 2,
isRequired = true
)
public final Integer lefty;
@ThriftField(
fieldId = 3,
isRequired = true
)
public final Integer rightx;
@ThriftField(
fieldId = 4,
isRequired = true
)
public final Integer righty;
private EyeInfo(Builder builder) {
this.leftx = builder.leftx;
this.lefty = builder.lefty;
this.rightx = builder.rightx;
this.righty = builder.righty;
}
@Override
@SuppressWarnings("NumberEquality")
public boolean equals(Object other) {
if (this == other) return true;
if (other == null) return false;
if (!(other instanceof EyeInfo)) return false;
EyeInfo that = (EyeInfo) other;
return (this.leftx == that.leftx || this.leftx.equals(that.leftx))
&& (this.lefty == that.lefty || this.lefty.equals(that.lefty))
&& (this.rightx == that.rightx || this.rightx.equals(that.rightx))
&& (this.righty == that.righty || this.righty.equals(that.righty));
}
@Override
public int hashCode() {
int code = 16777619;
code ^= this.leftx.hashCode();
code *= 0x811c9dc5;
code ^= this.lefty.hashCode();
code *= 0x811c9dc5;
code ^= this.rightx.hashCode();
code *= 0x811c9dc5;
code ^= this.righty.hashCode();
code *= 0x811c9dc5;
return code;
}
@Override
public String toString() {
return "EyeInfo{leftx=" + this.leftx + ", lefty=" + this.lefty + ", rightx=" + this.rightx + ", righty=" + this.righty + "}";
}
@Override
public void write(Protocol protocol) throws IOException {
ADAPTER.write(protocol, this);
}
public static final class Builder implements StructBuilder {
private Integer leftx;
private Integer lefty;
private Integer rightx;
private Integer righty;
public Builder() {
}
public Builder(EyeInfo struct) {
this.leftx = struct.leftx;
this.lefty = struct.lefty;
this.rightx = struct.rightx;
this.righty = struct.righty;
}
public Builder leftx(Integer leftx) {
if (leftx == null) {
throw new NullPointerException("Required field 'leftx' cannot be null");
}
this.leftx = leftx;
return this;
}
public Builder lefty(Integer lefty) {
if (lefty == null) {
throw new NullPointerException("Required field 'lefty' cannot be null");
}
this.lefty = lefty;
return this;
}
public Builder rightx(Integer rightx) {
if (rightx == null) {
throw new NullPointerException("Required field 'rightx' cannot be null");
}
this.rightx = rightx;
return this;
}
public Builder righty(Integer righty) {
if (righty == null) {
throw new NullPointerException("Required field 'righty' cannot be null");
}
this.righty = righty;
return this;
}
@Override
public EyeInfo build() {
if (this.leftx == null) {
throw new IllegalStateException("Required field 'leftx' is missing");
}
if (this.lefty == null) {
throw new IllegalStateException("Required field 'lefty' is missing");
}
if (this.rightx == null) {
throw new IllegalStateException("Required field 'rightx' is missing");
}
if (this.righty == null) {
throw new IllegalStateException("Required field 'righty' is missing");
}
return new EyeInfo(this);
}
@Override
public void reset() {
this.leftx = null;
this.lefty = null;
this.rightx = null;
this.righty = null;
}
}
private static final class EyeInfoAdapter implements Adapter {
@Override
public void write(Protocol protocol, EyeInfo struct) throws IOException {
protocol.writeStructBegin("EyeInfo");
protocol.writeFieldBegin("leftx", 1, TType.I32);
protocol.writeI32(struct.leftx);
protocol.writeFieldEnd();
protocol.writeFieldBegin("lefty", 2, TType.I32);
protocol.writeI32(struct.lefty);
protocol.writeFieldEnd();
protocol.writeFieldBegin("rightx", 3, TType.I32);
protocol.writeI32(struct.rightx);
protocol.writeFieldEnd();
protocol.writeFieldBegin("righty", 4, TType.I32);
protocol.writeI32(struct.righty);
protocol.writeFieldEnd();
protocol.writeFieldStop();
protocol.writeStructEnd();
}
@Override
public EyeInfo read(Protocol protocol, Builder builder) throws IOException {
protocol.readStructBegin();
while (true) {
FieldMetadata field = protocol.readFieldBegin();
if (field.typeId == TType.STOP) {
break;
}
switch (field.fieldId) {
case 1: {
if (field.typeId == TType.I32) {
int value = protocol.readI32();
builder.leftx(value);
} else {
ProtocolUtil.skip(protocol, field.typeId);
}
}
break;
case 2: {
if (field.typeId == TType.I32) {
int value = protocol.readI32();
builder.lefty(value);
} else {
ProtocolUtil.skip(protocol, field.typeId);
}
}
break;
case 3: {
if (field.typeId == TType.I32) {
int value = protocol.readI32();
builder.rightx(value);
} else {
ProtocolUtil.skip(protocol, field.typeId);
}
}
break;
case 4: {
if (field.typeId == TType.I32) {
int value = protocol.readI32();
builder.righty(value);
} else {
ProtocolUtil.skip(protocol, field.typeId);
}
}
break;
default: {
ProtocolUtil.skip(protocol, field.typeId);
}
break;
}
protocol.readFieldEnd();
}
protocol.readStructEnd();
return builder.build();
}
@Override
public EyeInfo read(Protocol protocol) throws IOException {
return read(protocol, new Builder());
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy