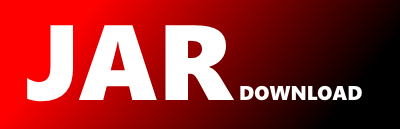
net.gdface.facedb.thrift.client.FInt2 Maven / Gradle / Ivy
// Automatically generated by the Thrifty compiler; do not edit!
// Generated on: 2019-09-24T07:53:45.881Z
// Source: J:\facedb\FaceDb.thrift at 22:1
package net.gdface.facedb.thrift.client;
import com.microsoft.thrifty.Adapter;
import com.microsoft.thrifty.Struct;
import com.microsoft.thrifty.StructBuilder;
import com.microsoft.thrifty.TType;
import com.microsoft.thrifty.ThriftField;
import com.microsoft.thrifty.protocol.FieldMetadata;
import com.microsoft.thrifty.protocol.Protocol;
import com.microsoft.thrifty.util.ProtocolUtil;
import java.io.IOException;
import javax.annotation.Generated;
@Generated(
value = "com.microsoft.thrifty.gen.ThriftyCodeGenerator",
comments = "https://github.com/microsoft/thrifty"
)
public final class FInt2 implements Struct {
public static final Adapter ADAPTER = new FInt2Adapter();
@ThriftField(
fieldId = 1,
isRequired = true
)
public final Integer x;
@ThriftField(
fieldId = 2,
isRequired = true
)
public final Integer y;
private FInt2(Builder builder) {
this.x = builder.x;
this.y = builder.y;
}
@Override
@SuppressWarnings("NumberEquality")
public boolean equals(Object other) {
if (this == other) return true;
if (other == null) return false;
if (!(other instanceof FInt2)) return false;
FInt2 that = (FInt2) other;
return (this.x == that.x || this.x.equals(that.x))
&& (this.y == that.y || this.y.equals(that.y));
}
@Override
public int hashCode() {
int code = 16777619;
code ^= this.x.hashCode();
code *= 0x811c9dc5;
code ^= this.y.hashCode();
code *= 0x811c9dc5;
return code;
}
@Override
public String toString() {
return "FInt2{x=" + this.x + ", y=" + this.y + "}";
}
@Override
public void write(Protocol protocol) throws IOException {
ADAPTER.write(protocol, this);
}
public static final class Builder implements StructBuilder {
private Integer x;
private Integer y;
public Builder() {
}
public Builder(FInt2 struct) {
this.x = struct.x;
this.y = struct.y;
}
public Builder x(Integer x) {
if (x == null) {
throw new NullPointerException("Required field 'x' cannot be null");
}
this.x = x;
return this;
}
public Builder y(Integer y) {
if (y == null) {
throw new NullPointerException("Required field 'y' cannot be null");
}
this.y = y;
return this;
}
@Override
public FInt2 build() {
if (this.x == null) {
throw new IllegalStateException("Required field 'x' is missing");
}
if (this.y == null) {
throw new IllegalStateException("Required field 'y' is missing");
}
return new FInt2(this);
}
@Override
public void reset() {
this.x = null;
this.y = null;
}
}
private static final class FInt2Adapter implements Adapter {
@Override
public void write(Protocol protocol, FInt2 struct) throws IOException {
protocol.writeStructBegin("FInt2");
protocol.writeFieldBegin("x", 1, TType.I32);
protocol.writeI32(struct.x);
protocol.writeFieldEnd();
protocol.writeFieldBegin("y", 2, TType.I32);
protocol.writeI32(struct.y);
protocol.writeFieldEnd();
protocol.writeFieldStop();
protocol.writeStructEnd();
}
@Override
public FInt2 read(Protocol protocol, Builder builder) throws IOException {
protocol.readStructBegin();
while (true) {
FieldMetadata field = protocol.readFieldBegin();
if (field.typeId == TType.STOP) {
break;
}
switch (field.fieldId) {
case 1: {
if (field.typeId == TType.I32) {
int value = protocol.readI32();
builder.x(value);
} else {
ProtocolUtil.skip(protocol, field.typeId);
}
}
break;
case 2: {
if (field.typeId == TType.I32) {
int value = protocol.readI32();
builder.y(value);
} else {
ProtocolUtil.skip(protocol, field.typeId);
}
}
break;
default: {
ProtocolUtil.skip(protocol, field.typeId);
}
break;
}
protocol.readFieldEnd();
}
protocol.readStructEnd();
return builder.build();
}
@Override
public FInt2 read(Protocol protocol) throws IOException {
return read(protocol, new Builder());
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy