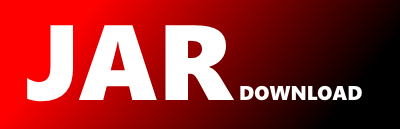
net.gdface.facedb.thrift.client.FeatureBean Maven / Gradle / Ivy
// Automatically generated by the Thrifty compiler; do not edit!
// Generated on: 2019-09-24T07:53:45.885Z
// Source: J:\facedb\FaceDb.thrift at 49:1
package net.gdface.facedb.thrift.client;
import com.microsoft.thrifty.Adapter;
import com.microsoft.thrifty.Struct;
import com.microsoft.thrifty.StructBuilder;
import com.microsoft.thrifty.TType;
import com.microsoft.thrifty.ThriftField;
import com.microsoft.thrifty.protocol.FieldMetadata;
import com.microsoft.thrifty.protocol.Protocol;
import com.microsoft.thrifty.util.ProtocolUtil;
import java.io.IOException;
import javax.annotation.Generated;
import okio.ByteString;
@Generated(
value = "com.microsoft.thrifty.gen.ThriftyCodeGenerator",
comments = "https://github.com/microsoft/thrifty"
)
public final class FeatureBean implements Struct {
public static final Adapter ADAPTER = new FeatureBeanAdapter();
@ThriftField(
fieldId = 1,
isRequired = true
)
public final Boolean _new;
@ThriftField(
fieldId = 2,
isRequired = true
)
public final Integer modified;
@ThriftField(
fieldId = 3,
isRequired = true
)
public final Integer initialized;
@ThriftField(
fieldId = 4,
isOptional = true
)
public final String md5;
@ThriftField(
fieldId = 5,
isOptional = true
)
public final ByteString feature;
@ThriftField(
fieldId = 6,
isOptional = true
)
public final Long createTime;
private FeatureBean(Builder builder) {
this._new = builder._new;
this.modified = builder.modified;
this.initialized = builder.initialized;
this.md5 = builder.md5;
this.feature = builder.feature;
this.createTime = builder.createTime;
}
@Override
@SuppressWarnings({"NumberEquality", "StringEquality"})
public boolean equals(Object other) {
if (this == other) return true;
if (other == null) return false;
if (!(other instanceof FeatureBean)) return false;
FeatureBean that = (FeatureBean) other;
return (this._new == that._new || this._new.equals(that._new))
&& (this.modified == that.modified || this.modified.equals(that.modified))
&& (this.initialized == that.initialized || this.initialized.equals(that.initialized))
&& (this.md5 == that.md5 || (this.md5 != null && this.md5.equals(that.md5)))
&& (this.feature == that.feature || (this.feature != null && this.feature.equals(that.feature)))
&& (this.createTime == that.createTime || (this.createTime != null && this.createTime.equals(that.createTime)));
}
@Override
public int hashCode() {
int code = 16777619;
code ^= this._new.hashCode();
code *= 0x811c9dc5;
code ^= this.modified.hashCode();
code *= 0x811c9dc5;
code ^= this.initialized.hashCode();
code *= 0x811c9dc5;
code ^= (this.md5 == null) ? 0 : this.md5.hashCode();
code *= 0x811c9dc5;
code ^= (this.feature == null) ? 0 : this.feature.hashCode();
code *= 0x811c9dc5;
code ^= (this.createTime == null) ? 0 : this.createTime.hashCode();
code *= 0x811c9dc5;
return code;
}
@Override
public String toString() {
return "FeatureBean{_new=" + this._new + ", modified=" + this.modified + ", initialized=" + this.initialized + ", md5=" + this.md5 + ", feature=" + this.feature + ", createTime=" + this.createTime + "}";
}
@Override
public void write(Protocol protocol) throws IOException {
ADAPTER.write(protocol, this);
}
public static final class Builder implements StructBuilder {
private Boolean _new;
private Integer modified;
private Integer initialized;
private String md5;
private ByteString feature;
private Long createTime;
public Builder() {
}
public Builder(FeatureBean struct) {
this._new = struct._new;
this.modified = struct.modified;
this.initialized = struct.initialized;
this.md5 = struct.md5;
this.feature = struct.feature;
this.createTime = struct.createTime;
}
public Builder _new(Boolean _new) {
if (_new == null) {
throw new NullPointerException("Required field '_new' cannot be null");
}
this._new = _new;
return this;
}
public Builder modified(Integer modified) {
if (modified == null) {
throw new NullPointerException("Required field 'modified' cannot be null");
}
this.modified = modified;
return this;
}
public Builder initialized(Integer initialized) {
if (initialized == null) {
throw new NullPointerException("Required field 'initialized' cannot be null");
}
this.initialized = initialized;
return this;
}
public Builder md5(String md5) {
this.md5 = md5;
return this;
}
public Builder feature(ByteString feature) {
this.feature = feature;
return this;
}
public Builder createTime(Long createTime) {
this.createTime = createTime;
return this;
}
@Override
public FeatureBean build() {
if (this._new == null) {
throw new IllegalStateException("Required field '_new' is missing");
}
if (this.modified == null) {
throw new IllegalStateException("Required field 'modified' is missing");
}
if (this.initialized == null) {
throw new IllegalStateException("Required field 'initialized' is missing");
}
return new FeatureBean(this);
}
@Override
public void reset() {
this._new = null;
this.modified = null;
this.initialized = null;
this.md5 = null;
this.feature = null;
this.createTime = null;
}
}
private static final class FeatureBeanAdapter implements Adapter {
@Override
public void write(Protocol protocol, FeatureBean struct) throws IOException {
protocol.writeStructBegin("FeatureBean");
protocol.writeFieldBegin("_new", 1, TType.BOOL);
protocol.writeBool(struct._new);
protocol.writeFieldEnd();
protocol.writeFieldBegin("modified", 2, TType.I32);
protocol.writeI32(struct.modified);
protocol.writeFieldEnd();
protocol.writeFieldBegin("initialized", 3, TType.I32);
protocol.writeI32(struct.initialized);
protocol.writeFieldEnd();
if (struct.md5 != null) {
protocol.writeFieldBegin("md5", 4, TType.STRING);
protocol.writeString(struct.md5);
protocol.writeFieldEnd();
}
if (struct.feature != null) {
protocol.writeFieldBegin("feature", 5, TType.STRING);
protocol.writeBinary(struct.feature);
protocol.writeFieldEnd();
}
if (struct.createTime != null) {
protocol.writeFieldBegin("createTime", 6, TType.I64);
protocol.writeI64(struct.createTime);
protocol.writeFieldEnd();
}
protocol.writeFieldStop();
protocol.writeStructEnd();
}
@Override
public FeatureBean read(Protocol protocol, Builder builder) throws IOException {
protocol.readStructBegin();
while (true) {
FieldMetadata field = protocol.readFieldBegin();
if (field.typeId == TType.STOP) {
break;
}
switch (field.fieldId) {
case 1: {
if (field.typeId == TType.BOOL) {
boolean value = protocol.readBool();
builder._new(value);
} else {
ProtocolUtil.skip(protocol, field.typeId);
}
}
break;
case 2: {
if (field.typeId == TType.I32) {
int value = protocol.readI32();
builder.modified(value);
} else {
ProtocolUtil.skip(protocol, field.typeId);
}
}
break;
case 3: {
if (field.typeId == TType.I32) {
int value = protocol.readI32();
builder.initialized(value);
} else {
ProtocolUtil.skip(protocol, field.typeId);
}
}
break;
case 4: {
if (field.typeId == TType.STRING) {
String value = protocol.readString();
builder.md5(value);
} else {
ProtocolUtil.skip(protocol, field.typeId);
}
}
break;
case 5: {
if (field.typeId == TType.STRING) {
ByteString value = protocol.readBinary();
builder.feature(value);
} else {
ProtocolUtil.skip(protocol, field.typeId);
}
}
break;
case 6: {
if (field.typeId == TType.I64) {
long value = protocol.readI64();
builder.createTime(value);
} else {
ProtocolUtil.skip(protocol, field.typeId);
}
}
break;
default: {
ProtocolUtil.skip(protocol, field.typeId);
}
break;
}
protocol.readFieldEnd();
}
protocol.readStructEnd();
return builder.build();
}
@Override
public FeatureBean read(Protocol protocol) throws IOException {
return read(protocol, new Builder());
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy