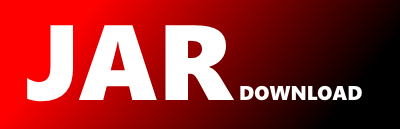
net.facelib.live.FaceLiveBridge Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of facelive Show documentation
Show all versions of facelive Show documentation
detect live face for video stream
package net.facelib.live;
import static net.facelib.jni.BridgeLog.BRIDEG_LOGGER;
import java.util.Arrays;
import java.util.concurrent.atomic.AtomicReference;
import net.facelib.akcore.LicenseManager;
import net.facelib.jni.SdkStatus;
import net.facelib.sdk.ImageSupport;
import net.gdface.image.MatType;
import net.facelib.jni.BaseJniBridge;
import net.facelib.jni.SdkInitException;
import net.facelib.jni.SdkRuntimeException;
/**
* 活体检测 for android/arm JNI 接口
* 此类为非线程安全的,不可多线程共享调用,需要在外部进一步封装实现线程安全
* @author guyadong
*
*/
public class FaceLiveBridge extends BaseJniBridge{
private final long[] liveHandle = new long[]{-1};
static final String FACE_LIVE_PRODUCT_ID = "ZYFLIVE_android";
/** 活体检测的授权管理器实例,NOTE:与父类不同 */
public static final LicenseManager LICENSE_MANAGER = new LiveLicenseManager();
/**
* 初始化状态
*/
private volatile SdkStatus status =SdkStatus.SDK_UNINITIALIZED;
/**
* 内部同步对象
*/
// private static final Object internalLock = new Object();
static {
try {
BRIDEG_LOGGER.log("loadLibrary FS_AndroidLiveSDK");
// 加载算法动态库
System.loadLibrary("FS_AndroidLiveSDK");
} catch (Exception e) {
BRIDEG_LOGGER.log(e.getMessage());
throw new ExceptionInInitializerError(e);
}
}
public FaceLiveBridge() {
}
/**
* 返回当前SDK初始化状态
* @return SDK初始化状态
*/
@Override
public SdkStatus getStatus() {
return status;
}
@Override
protected LicenseManager licenseManager(){
return LICENSE_MANAGER;
}
@Override
protected void sdkInit(String licenseFile, String licenseKey, String licenseCode) throws SdkInitException {
if(SdkStatus.SDK_INIT_OK == status){
return;
}
try {
// synchronized (internalLock) {
int ffFlag = FLInit(
licenseCode.getBytes(),
licenseKey.getBytes(),
licenseFile.getBytes(),
liveHandle);
BRIDEG_LOGGER.log("FLInit liveHandle: [{}]",liveHandle[0]);
status = SdkStatus.jniCode(ffFlag);
BRIDEG_LOGGER.log("live module: {}", this);
if(status !=SdkStatus.SDK_INIT_OK){
throw new SdkInitException(status);
}
// }
} finally {
// 如果没有初始化成功,则destroy已经初始化模块
if(SdkStatus.SDK_INIT_OK != status){
BRIDEG_LOGGER.log("FLDestroy caused by init fail {}",this);
// synchronized (internalLock) {
FLDestroy(liveHandle[0]);
// }
}
}
}
@Override
protected void sdkUninit() {
if(liveHandle[0] >= 0 ){
BRIDEG_LOGGER.log("FLDestroy {}",this);
status = SdkStatus.SDK_UNINITIALIZED;
// synchronized (internalLock) {
FLDestroy(liveHandle[0]);
// }
liveHandle[0] = -1;
}
}
/**
* 对图像进行活体检测
* @param matType 矩阵类型
* @param matData 矩阵图像数据
* @param width
* @param height
* @param rect
* @return 0--非活体,1--活体,2--缓存标志,<0 错误代码
*/
int liveProcess(MatType matType, byte[] matData, int width, int height, double[] rect){
return liveProcess(matType,matData,width,height,rect, null);
}
/**
* 对图像进行活体检测
* @param matType 矩阵类型
* @param matData 矩阵图像数据
* @param width
* @param height
* @param rect
* @param score [out]活体检测得分
* @return 0--非活体,1--活体,2--缓存标志,<0 错误代码
*/
int liveProcess(MatType matType, byte[] matData, int width, int height,double[] rect, AtomicReferencescore){
double[] scoreOut = new double[1];
byte[] bgr = ImageSupport.toBGR(matType, matData, width, height);
// BRIDEG_LOGGER.log("before FLProcess liveHandle: [{}] width={},height={}",liveHandle[0],width,height);
int ret = FLProcess(liveHandle[0], bgr, width, height, rect, scoreOut);
if(ret<0){
SdkStatus code = SdkStatus.jniCode(ret);
BRIDEG_LOGGER.log("FLProcess liveHandle: [{}],code={}",liveHandle[0],code);
throw new SdkRuntimeException(code);
}
if(score != null){
score.set(scoreOut[0]);
}
return ret;
}
////////////////////////////////////////活体检测系列///////////////////////////////////////////
/**
* 活体检测模块初始化函数
* @param licenseCode 授权码
* @param licenseKey 授权关键字
* @param path 授权码文件本地路径
* @param handle [out]结构体
* @return 初化结果状态码
*/
static native int FLInit(byte[] licenseCode, byte[] licenseKey, byte[] path, long[] handle);
static native void FLDestroy(long handle);
/**
* @param handle
* @param BGR
* @param width
* @param height
* @param rect
* @param socre
* @return 0--非活体,1--活体,2--缓存标志,<0 错误代码
*/
static native int FLProcess(long handle, byte[] BGR, int width, int height, double[] rect, double[] socre);
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
builder.append("FaceLiveBridge [liveHandle=");
builder.append(Arrays.toString(liveHandle));
builder.append(", status=");
builder.append(status);
builder.append("]");
return builder.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy