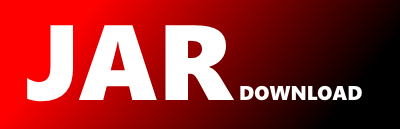
net.gdface.facelog.thrift.IFaceLogThriftClient Maven / Gradle / Ivy
The newest version!
// ______________________________________________________
// Generated by codegen - https://gitee.com/l0km/codegen
// template: thrift/client/perservice/client.service.decorator.class.vm
// ______________________________________________________
package net.gdface.facelog.thrift;
import gu.sql2java.StringMatchType;
import java.util.List;
import java.util.Map;
import net.gdface.facelog.DuplicateRecordException;
import net.gdface.facelog.FuzzyMatchCountExceedLimitException;
import net.gdface.facelog.IFaceLog;
import net.gdface.facelog.LockWakeupResponse;
import net.gdface.facelog.MQParam;
import net.gdface.facelog.MatchEntry;
import net.gdface.facelog.PersonDataPackage;
import net.gdface.facelog.ServiceSecurityException;
import net.gdface.facelog.TmpPwdTargetType;
import net.gdface.facelog.TmpwdTargetInfo;
import net.gdface.facelog.Token;
import net.gdface.facelog.TopGroupInfo;
import net.gdface.facelog.db.DeviceBean;
import net.gdface.facelog.db.DeviceGroupBean;
import net.gdface.facelog.db.ErrorLogBean;
import net.gdface.facelog.db.FaceBean;
import net.gdface.facelog.db.FeatureBean;
import net.gdface.facelog.db.ImageBean;
import net.gdface.facelog.db.LogBean;
import net.gdface.facelog.db.LogLightBean;
import net.gdface.facelog.db.PermitBean;
import net.gdface.facelog.db.PersonBean;
import net.gdface.facelog.db.PersonGroupBean;
import com.gitee.l0km.xthrift.thrift.ClientFactory;
import com.gitee.l0km.xthrift.thrift.TypeTransformer;
import com.facebook.swift.service.RuntimeTApplicationException;
import com.google.common.net.HostAndPort;
import static com.google.common.base.Preconditions.*;
/**
* 基于thrift/swift框架生成的client端代码提供{@link IFaceLog}接口的RPC实现(线程安全)
* 转发所有{@link IFaceLog}接口方法到{@link #delegate()}指定的实例
* 所有服务端抛出的{@link RuntimeException}异常被封装到{@link ServiceRuntimeException}中抛出
* Example:
*
* IFaceLogThriftClient thriftInstance = ClientFactory
* .builder()
* .setHostAndPort("127.0.0.1",26413)
* .build(IFaceLog.class, IFaceLogThriftClient.class);
*
* 计算机生成代码(generated by automated tools ThriftServiceDecoratorGenerator @author guyadong)
* @author guyadong
*
*/
public class IFaceLogThriftClient implements IFaceLog {
private final ClientFactory factory;
public ClientFactory getFactory() {
return factory;
}
public IFaceLogThriftClient(ClientFactory factory) {
super();
this.factory = checkNotNull(factory,"factory is null");
}
/**
* @param host RPC service host
* @param port RPC service port
*/
public IFaceLogThriftClient(String host,int port) {
this(ClientFactory.builder().setHostAndPort(host,port));
}
/**
* @param hostAndPort RPC service host and port
*/
public IFaceLogThriftClient(HostAndPort hostAndPort) {
this(ClientFactory.builder().setHostAndPort(hostAndPort));
}
/**
* test if connectable for RPC service
* @return return {@code true} if connectable ,otherwise {@code false}
*/
public boolean testConnect(){
return factory.testConnect();
}
/**
* @return 返回{@link net.gdface.facelog.client.thrift.IFaceLog}实例
*/
protected net.gdface.facelog.client.thrift.IFaceLog delegate() {
return factory.applyInstance(net.gdface.facelog.client.thrift.IFaceLog.class);
}
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
builder.append("IFaceLogThriftClient [factory=");
builder.append(factory);
builder.append(",interface=");
builder.append(IFaceLog.class.getName());
builder.append("]");
return builder.toString();
}
@Override
public void addErrorLog(ErrorLogBean errorLogBean,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
instance.addErrorLog(TypeTransformer.getInstance().to(
errorLogBean,
ErrorLogBean.class,
net.gdface.facelog.client.thrift.ErrorLogBean.class),
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public FeatureBean addFeature(byte[] feature,
String featureVersion,
Integer personId,
boolean asIdPhotoIfAbsent,
byte[] featurePhoto,
FaceBean faceBean,
String removed,
Token token)
throws DuplicateRecordException{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.addFeatureWithImage(feature,
featureVersion,
personId,
asIdPhotoIfAbsent,
featurePhoto,
TypeTransformer.getInstance().to(
faceBean,
FaceBean.class,
net.gdface.facelog.client.thrift.FaceBean.class),
removed,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.FeatureBean.class,
FeatureBean.class);
}
catch(net.gdface.facelog.client.thrift.DuplicateRecordException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facelog.client.thrift.DuplicateRecordException.class,
DuplicateRecordException.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public FeatureBean addFeature(byte[] feature,
String featureVersion,
Integer personId,
List faecBeans,
String removed,
Token token)
throws DuplicateRecordException{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.addFeature(feature,
featureVersion,
personId,
TypeTransformer.getInstance().to(
faecBeans,
FaceBean.class,
net.gdface.facelog.client.thrift.FaceBean.class),
removed,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.FeatureBean.class,
FeatureBean.class);
}
catch(net.gdface.facelog.client.thrift.DuplicateRecordException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facelog.client.thrift.DuplicateRecordException.class,
DuplicateRecordException.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public FeatureBean addFeature(byte[] feature,
String featureVersion,
Integer personId,
List photos,
List faces,
String removed,
Token token)
throws DuplicateRecordException{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.addFeatureMulti(feature,
featureVersion,
personId,
photos,
TypeTransformer.getInstance().to(
faces,
FaceBean.class,
net.gdface.facelog.client.thrift.FaceBean.class),
removed,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.FeatureBean.class,
FeatureBean.class);
}
catch(net.gdface.facelog.client.thrift.DuplicateRecordException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facelog.client.thrift.DuplicateRecordException.class,
DuplicateRecordException.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public ImageBean addImage(byte[] imageData,
Integer deviceId,
FaceBean faceBean,
Integer personId,
Token token)
throws DuplicateRecordException{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.addImage(imageData,
deviceId,
TypeTransformer.getInstance().to(
faceBean,
FaceBean.class,
net.gdface.facelog.client.thrift.FaceBean.class),
personId,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.ImageBean.class,
ImageBean.class);
}
catch(net.gdface.facelog.client.thrift.DuplicateRecordException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facelog.client.thrift.DuplicateRecordException.class,
DuplicateRecordException.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public void addLog(LogBean logBean,
byte[] faceImage,
Token token)
throws DuplicateRecordException{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
instance.addLogWithFaceImage(TypeTransformer.getInstance().to(
logBean,
LogBean.class,
net.gdface.facelog.client.thrift.LogBean.class),
faceImage,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.DuplicateRecordException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facelog.client.thrift.DuplicateRecordException.class,
DuplicateRecordException.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public void addLog(LogBean logBean,
Token token)
throws DuplicateRecordException{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
instance.addLog(TypeTransformer.getInstance().to(
logBean,
LogBean.class,
net.gdface.facelog.client.thrift.LogBean.class),
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.DuplicateRecordException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facelog.client.thrift.DuplicateRecordException.class,
DuplicateRecordException.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public void addLog(LogBean logBean,
FaceBean faceBean,
byte[] featureImage,
Token token)
throws DuplicateRecordException{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
instance.addLogFull(TypeTransformer.getInstance().to(
logBean,
LogBean.class,
net.gdface.facelog.client.thrift.LogBean.class),
TypeTransformer.getInstance().to(
faceBean,
FaceBean.class,
net.gdface.facelog.client.thrift.FaceBean.class),
featureImage,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.DuplicateRecordException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facelog.client.thrift.DuplicateRecordException.class,
DuplicateRecordException.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public void addLogs(List logBeans,
List faceBeans,
List featureImages,
Token token)
throws DuplicateRecordException{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
instance.addLogsFull(TypeTransformer.getInstance().to(
logBeans,
LogBean.class,
net.gdface.facelog.client.thrift.LogBean.class),
TypeTransformer.getInstance().to(
faceBeans,
FaceBean.class,
net.gdface.facelog.client.thrift.FaceBean.class),
featureImages,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.DuplicateRecordException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facelog.client.thrift.DuplicateRecordException.class,
DuplicateRecordException.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public void addLogs(List logBeans,
List faceImages,
Token token)
throws DuplicateRecordException{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
instance.addLogsWithFaceImage(TypeTransformer.getInstance().to(
logBeans,
LogBean.class,
net.gdface.facelog.client.thrift.LogBean.class),
faceImages,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.DuplicateRecordException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facelog.client.thrift.DuplicateRecordException.class,
DuplicateRecordException.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public void addLogs(List logBeans,
Token token)
throws DuplicateRecordException{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
instance.addLogs(TypeTransformer.getInstance().to(
logBeans,
LogBean.class,
net.gdface.facelog.client.thrift.LogBean.class),
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.DuplicateRecordException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facelog.client.thrift.DuplicateRecordException.class,
DuplicateRecordException.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public String applyAckChannel(int duration,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.applyAckChannelWithDuration(duration,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public String applyAckChannel(Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.applyAckChannel(TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int applyCmdSn(Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.applyCmdSn(TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public Token applyPersonToken(int personId,
String password,
boolean isMd5)
throws ServiceSecurityException{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.applyPersonToken(personId,
password,
isMd5),
net.gdface.facelog.client.thrift.Token.class,
Token.class);
}
catch(net.gdface.facelog.client.thrift.ServiceSecurityException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facelog.client.thrift.ServiceSecurityException.class,
ServiceSecurityException.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public Token applyRootToken(String password,
boolean isMd5)
throws ServiceSecurityException{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.applyRootToken(password,
isMd5),
net.gdface.facelog.client.thrift.Token.class,
Token.class);
}
catch(net.gdface.facelog.client.thrift.ServiceSecurityException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facelog.client.thrift.ServiceSecurityException.class,
ServiceSecurityException.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public Token applyUserToken(int userid,
String password,
boolean isMd5)
throws ServiceSecurityException{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.applyUserToken(userid,
password,
isMd5),
net.gdface.facelog.client.thrift.Token.class,
Token.class);
}
catch(net.gdface.facelog.client.thrift.ServiceSecurityException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facelog.client.thrift.ServiceSecurityException.class,
ServiceSecurityException.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public void bindBorder(int personGroupId,
int deviceGroupId,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
instance.bindBorder(personGroupId,
deviceGroupId,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List childListForDeviceGroup(int deviceGroupId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.childListForDeviceGroup(deviceGroupId);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List childListForPersonGroup(int personGroupId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.childListForPersonGroup(personGroupId);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int countDeviceByWhere(String where)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.countDeviceByWhere(where);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int countDeviceByWhere(String where,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.countDeviceByWhereSafe(where,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int countDeviceGroupByWhere(String where)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.countDeviceGroupByWhere(where);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int countDeviceGroupByWhere(String where,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.countDeviceGroupByWhereSafe(where,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int countErrorLogByWhere(String where)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.countErrorLogByWhere(where);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int countLogByWhere(String where)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.countLogByWhere(where);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int countLogByWhere(String where,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.countLogByWhereSafe(where,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int countLogLightByVerifyTime(String timestamp)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.countLogLightByVerifyTimeTimestr(timestamp);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int countLogLightByVerifyTime(String timestamp,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.countLogLightByVerifyTimeTimestrSafe(timestamp,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int countLogLightByVerifyTime(long timestamp)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.countLogLightByVerifyTime(timestamp);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int countLogLightByVerifyTime(long timestamp,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.countLogLightByVerifyTimeSafe(timestamp,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int countLogLightByWhere(String where)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.countLogLightByWhere(where);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int countLogLightByWhere(String where,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.countLogLightByWhereSafe(where,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int countPersonByWhere(String where)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.countPersonByWhere(where);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int countPersonByWhere(String where,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.countPersonByWhereSafe(where,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int countPersonGroupByWhere(String where)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.countPersonGroupByWhere(where);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int countPersonGroupByWhere(String where,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.countPersonGroupByWhereSafe(where,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public Map countPersonLog(int personId,
Long startDate,
Long endDate)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.countPersonLog(personId,
startDate,
endDate),
String.class,
Integer.class,
String.class,
Integer.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public Map countPersonLog(int personId,
Long startDate,
Long endDate,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.countPersonLogSafe(personId,
startDate,
endDate,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
String.class,
Integer.class,
String.class,
Integer.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public Map countPersonLog(int personId,
String startDate,
String endDate)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.countPersonLogTimeStr(personId,
startDate,
endDate),
String.class,
Integer.class,
String.class,
Integer.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public Map countPersonLog(int personId,
String startDate,
String endDate,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.countPersonLogTimeStrSafe(personId,
startDate,
endDate,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
String.class,
Integer.class,
String.class,
Integer.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public String createTempPwd(int targetId,
TmpPwdTargetType targetType,
int duration,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.createTempPwdWithDuration(targetId,
TypeTransformer.getInstance().to(
targetType,
TmpPwdTargetType.class,
net.gdface.facelog.client.thrift.TmpPwdTargetType.class),
duration,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public String createTempPwd(int targetId,
TmpPwdTargetType targetType,
String expiryDate,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.createTempPwd(targetId,
TypeTransformer.getInstance().to(
targetType,
TmpPwdTargetType.class,
net.gdface.facelog.client.thrift.TmpPwdTargetType.class),
expiryDate,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int deleteAllFeaturesByPersonId(int personId,
boolean deleteImage,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.deleteAllFeaturesByPersonId(personId,
deleteImage,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public boolean deleteDevice(int id,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.deleteDevice(id,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public boolean deleteDeviceByMac(String mac,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.deleteDeviceByMac(mac,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int deleteDeviceGroup(int deviceGroupId,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.deleteDeviceGroup(deviceGroupId,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int deleteErrorLogByWhere(String where,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.deleteErrorLogByWhere(where,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List deleteFeature(String featureMd5,
boolean deleteImage,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.deleteFeature(featureMd5,
deleteImage,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int deleteGroupPermitOnDeviceGroup(int deviceGroupId,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.deleteGroupPermitOnDeviceGroup(deviceGroupId,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int deleteImage(String imageMd5,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.deleteImage(imageMd5,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int deleteLogByWhere(String where,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.deleteLogByWhere(where,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int deletePermit(int deviceGroupId,
int personGroupId,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.deletePermitById(deviceGroupId,
personGroupId,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int deletePerson(int personId,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.deletePerson(personId,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int deletePersonByMobilePhone(String mobilePhone,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.deletePersonByMobilePhone(mobilePhone,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int deletePersonByPapersNum(String papersNum,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.deletePersonByPapersNum(papersNum,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int deletePersonGroup(int personGroupId,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.deletePersonGroup(personGroupId,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int deletePersonGroupPermit(int personGroupId,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.deletePersonGroupPermit(personGroupId,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int deletePersons(List personIdList,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.deletePersons(personIdList,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int deletePersonsByMobilePhone(List mobilePhoneList,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.deletePersonsByMobilePhone(mobilePhoneList,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int deletePersonsByPapersNum(List papersNumList,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.deletePersonsByPapersNum(papersNumList,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public void disablePerson(int personId,
Integer moveToGroupId,
boolean deletePhoto,
boolean deleteFeature,
boolean deleteLog,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
instance.disablePerson(personId,
moveToGroupId,
deletePhoto,
deleteFeature,
deleteLog,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public void disablePerson(List personIdList,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
instance.disablePersonList(personIdList,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public boolean existsDevice(int id)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.existsDevice(id);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public boolean existsFeature(String md5)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.existsFeature(md5);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public boolean existsImage(String md5)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.existsImage(md5);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public boolean existsPerson(int persionId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.existsPerson(persionId);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int faceRecognizePersonPermitted(byte[] imageData,
Float threshold,
int group,
int deviceId,
boolean searchInPermited)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.faceRecognizePersonPermitted(imageData,
(threshold==null)?null:threshold.doubleValue(),
group,
deviceId,
searchInPermited);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List fuzzySearch(String tablename,
String column,
String pattern,
StringMatchType matchType,
int matchFlags,
int parentGroupId,
int maxMatchCount)
throws FuzzyMatchCountExceedLimitException{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.fuzzySearch(tablename,
column,
pattern,
TypeTransformer.getInstance().to(
matchType,
StringMatchType.class,
net.gdface.facelog.client.thrift.StringMatchType.class),
matchFlags,
parentGroupId,
maxMatchCount),
net.gdface.facelog.client.thrift.MatchEntry.class,
MatchEntry.class);
}
catch(net.gdface.facelog.client.thrift.FuzzyMatchCountExceedLimitException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facelog.client.thrift.FuzzyMatchCountExceedLimitException.class,
FuzzyMatchCountExceedLimitException.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List fuzzySearchPerson(String column,
String pattern,
StringMatchType matchType,
int matchFlags,
int parentGroupId,
int maxMatchCount,
Token token)
throws FuzzyMatchCountExceedLimitException{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.fuzzySearchPerson(column,
pattern,
TypeTransformer.getInstance().to(
matchType,
StringMatchType.class,
net.gdface.facelog.client.thrift.StringMatchType.class),
matchFlags,
parentGroupId,
maxMatchCount,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.MatchEntry.class,
MatchEntry.class);
}
catch(net.gdface.facelog.client.thrift.FuzzyMatchCountExceedLimitException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facelog.client.thrift.FuzzyMatchCountExceedLimitException.class,
FuzzyMatchCountExceedLimitException.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public DeviceBean getDevice(int deviceId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getDevice(deviceId),
net.gdface.facelog.client.thrift.DeviceBean.class,
DeviceBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public DeviceBean getDeviceByMac(String mac)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getDeviceByMac(mac),
net.gdface.facelog.client.thrift.DeviceBean.class,
DeviceBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public DeviceGroupBean getDeviceGroup(int deviceGroupId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getDeviceGroup(deviceGroupId),
net.gdface.facelog.client.thrift.DeviceGroupBean.class,
DeviceGroupBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getDeviceGroups(List groupIdList)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getDeviceGroups(groupIdList),
net.gdface.facelog.client.thrift.DeviceGroupBean.class,
DeviceGroupBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getDeviceGroupsBelongs(int deviceId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.getDeviceGroupsBelongs(deviceId);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getDeviceGroupsPermit(int personGroupId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.getDeviceGroupsPermit(personGroupId);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getDeviceGroupsPermittedBy(int personGroupId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.getDeviceGroupsPermittedBy(personGroupId);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public Integer getDeviceIdOfFeature(String featureMd5)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.getDeviceIdOfFeature(featureMd5);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getDevices(List idList)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getDevices(idList),
net.gdface.facelog.client.thrift.DeviceBean.class,
DeviceBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getDevicesOfGroup(int deviceGroupId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.getDevicesOfGroup(deviceGroupId);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public FaceBean getFace(int faceId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getFace(faceId),
net.gdface.facelog.client.thrift.FaceBean.class,
FaceBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public Map getFaceApiParameters(Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getFaceApiParameters(TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
String.class,
String.class,
String.class,
String.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getFacesOfFeature(String featureMd5)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getFacesOfFeature(featureMd5),
net.gdface.facelog.client.thrift.FaceBean.class,
FaceBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getFacesOfImage(String imageMd5)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getFacesOfImage(imageMd5),
net.gdface.facelog.client.thrift.FaceBean.class,
FaceBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public FeatureBean getFeature(String md5)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getFeature(md5),
net.gdface.facelog.client.thrift.FeatureBean.class,
FeatureBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public byte[] getFeatureBytes(String md5)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.getFeatureBytes(md5);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public byte[] getFeatureBytes(String md5,
boolean truncation)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.getFeatureBytesTruncation(md5,
truncation);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getFeatureBytesList(List md5List,
boolean truncation)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.getFeatureBytesList(md5List,
truncation);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getFeatures(List md5List)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getFeatures(md5List),
net.gdface.facelog.client.thrift.FeatureBean.class,
FeatureBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getFeaturesByPersonIdAndSdkVersion(int personId,
String sdkVersion)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.getFeaturesByPersonIdAndSdkVersion(personId,
sdkVersion);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getFeaturesOfImage(String imageMd5)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.getFeaturesOfImage(imageMd5);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getFeaturesOfPerson(int personId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.getFeaturesOfPerson(personId);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getFeaturesPermittedOnDevice(int deviceId,
boolean ignoreSchedule,
String sdkVersion,
List excludeFeatureIds,
Long timestamp)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.getFeaturesPermittedOnDevice(deviceId,
ignoreSchedule,
sdkVersion,
excludeFeatureIds,
timestamp);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getGroupIdsByPath(String tablename,
String path)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.getGroupIdsByPath(tablename,
path);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public PermitBean getGroupPermit(int deviceId,
int personGroupId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getGroupPermit(deviceId,
personGroupId),
net.gdface.facelog.client.thrift.PermitBean.class,
PermitBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public PermitBean getGroupPermitOnDeviceGroup(int deviceGroupId,
int personGroupId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getGroupPermitOnDeviceGroup(deviceGroupId,
personGroupId),
net.gdface.facelog.client.thrift.PermitBean.class,
PermitBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getGroupPermits(int deviceId,
List personGroupIdList)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getGroupPermits(deviceId,
personGroupIdList),
net.gdface.facelog.client.thrift.PermitBean.class,
PermitBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public ImageBean getImage(String imageMD5)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getImage(imageMD5),
net.gdface.facelog.client.thrift.ImageBean.class,
ImageBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public ImageBean getImage(String primaryKey,
String refType)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getImageRef(primaryKey,
refType),
net.gdface.facelog.client.thrift.ImageBean.class,
ImageBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public byte[] getImageBytes(String imageMD5)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.getImageBytes(imageMD5);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public byte[] getImageBytes(String primaryKey,
String refType)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.getImageBytesRef(primaryKey,
refType);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getImagesAssociatedByFeature(String featureMd5)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.getImagesAssociatedByFeature(featureMd5);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getLogBeansByPersonId(int personId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getLogBeansByPersonId(personId),
net.gdface.facelog.client.thrift.LogBean.class,
LogBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getLogBeansByPersonId(int personId,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getLogBeansByPersonIdSafe(personId,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.LogBean.class,
LogBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public Map getMessageQueueParameters(Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getMessageQueueParameters(TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.MQParam.class,
String.class,
MQParam.class,
String.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public PersonBean getPerson(int personId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getPerson(personId),
net.gdface.facelog.client.thrift.PersonBean.class,
PersonBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public PersonBean getPerson(int personId,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getPersonReal(personId,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.PersonBean.class,
PersonBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public PersonBean getPersonByMobilePhone(String mobilePhone)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getPersonByMobilePhone(mobilePhone),
net.gdface.facelog.client.thrift.PersonBean.class,
PersonBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public PersonBean getPersonByMobilePhone(String mobilePhone,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getPersonByMobilePhoneReal(mobilePhone,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.PersonBean.class,
PersonBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public PersonBean getPersonByPapersNum(String papersNum)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getPersonByPapersNum(papersNum),
net.gdface.facelog.client.thrift.PersonBean.class,
PersonBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public PersonBean getPersonByPapersNum(String papersNum,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getPersonByPapersNumSafe(papersNum,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.PersonBean.class,
PersonBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public PersonGroupBean getPersonGroup(int personGroupId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getPersonGroup(personGroupId),
net.gdface.facelog.client.thrift.PersonGroupBean.class,
PersonGroupBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getPersonGroups(List groupIdList)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getPersonGroups(groupIdList),
net.gdface.facelog.client.thrift.PersonGroupBean.class,
PersonGroupBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getPersonGroupsBelongs(int personId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.getPersonGroupsBelongs(personId);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getPersonGroupsPermittedBy(int deviceGroupId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.getPersonGroupsPermittedBy(deviceGroupId);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public PermitBean getPersonPermit(int deviceId,
int personId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getPersonPermit(deviceId,
personId),
net.gdface.facelog.client.thrift.PermitBean.class,
PermitBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getPersonPermits(int deviceId,
List personIdList)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getPersonPermits(deviceId,
personIdList),
net.gdface.facelog.client.thrift.PermitBean.class,
PermitBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getPersons(List idList)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getPersons(idList),
net.gdface.facelog.client.thrift.PersonBean.class,
PersonBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getPersons(List idList,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getPersonsReal(idList,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.PersonBean.class,
PersonBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getPersonsOfGroup(int personGroupId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.getPersonsOfGroup(personGroupId);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getPersonsPermittedOnDevice(int deviceId,
boolean ignoreSchedule,
List excludePersonIds,
Long timestamp)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.getPersonsPermittedOnDevice(deviceId,
ignoreSchedule,
excludePersonIds,
timestamp);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getPersonsPermittedOnDeviceByGroup(int deviceId,
boolean ignoreSchedule,
List excludePersonIds,
Long timestamp)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.getPersonsPermittedOnDeviceByGroup(deviceId,
ignoreSchedule,
excludePersonIds,
timestamp);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public Map getProperties(String prefix,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getProperties(prefix,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
String.class,
String.class,
String.class,
String.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public String getProperty(String key,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.getProperty(key,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public Map getRedisParameters(Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getRedisParameters(TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.MQParam.class,
String.class,
MQParam.class,
String.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public Map getServiceConfig(Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getServiceConfig(TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
String.class,
String.class,
String.class,
String.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getSubDeviceGroup(int deviceGroupId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.getSubDeviceGroup(deviceGroupId);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List getSubPersonGroup(int personGroupId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.getSubPersonGroup(personGroupId);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public TmpwdTargetInfo getTargetInfo4PwdOnDevice(String pwd,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.getTargetInfo4PwdOnDevice(pwd,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.TmpwdTargetInfo.class,
TmpwdTargetInfo.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int initTopGroup(TopGroupInfo groupInfo,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.initTopGroup(TypeTransformer.getInstance().to(
groupInfo,
TopGroupInfo.class,
net.gdface.facelog.client.thrift.TopGroupInfo.class),
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public boolean isDisable(int personId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.isDisable(personId);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public boolean isLocal(){
return false;
}
@Override
public boolean isValidAckChannel(String ackChannel)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.isValidAckChannel(ackChannel);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public boolean isValidCmdSn(int cmdSn)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.isValidCmdSn(cmdSn);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public boolean isValidDeviceToken(Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.isValidDeviceToken(TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public boolean isValidPassword(String userId,
String password,
boolean isMd5)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.isValidPassword(userId,
password,
isMd5);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public boolean isValidPersonToken(Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.isValidPersonToken(TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public boolean isValidRootToken(Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.isValidRootToken(TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public boolean isValidToken(Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.isValidToken(TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public boolean isValidUserToken(Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.isValidUserToken(TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public String iso8601Time()
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.iso8601Time();
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List listOfParentForDeviceGroup(int deviceGroupId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.listOfParentForDeviceGroup(deviceGroupId);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List listOfParentForPersonGroup(int personGroupId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.listOfParentForPersonGroup(personGroupId);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadAllPerson()
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.loadAllPerson();
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadAllPerson(Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.loadAllPersonSafe(TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadDeviceByWhere(String where,
int startRow,
int numRows)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.loadDeviceByWhere(where,
startRow,
numRows),
net.gdface.facelog.client.thrift.DeviceBean.class,
DeviceBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadDeviceByWhere(String where,
int startRow,
int numRows,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.loadDeviceByWhereSafe(where,
startRow,
numRows,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.DeviceBean.class,
DeviceBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadDeviceGroupByWhere(String where,
int startRow,
int numRows)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.loadDeviceGroupByWhere(where,
startRow,
numRows);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadDeviceGroupIdByWhere(String where)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.loadDeviceGroupIdByWhere(where);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadDeviceGroupIdByWhere(String where,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.loadDeviceGroupIdByWhereSafe(where,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadDeviceIdByWhere(String where)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.loadDeviceIdByWhere(where);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadDeviceIdByWhere(String where,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.loadDeviceIdByWhereSafe(where,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadDistinctIntegerColumn(String table,
String column,
String where)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.loadDistinctIntegerColumn(table,
column,
where);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadDistinctStringColumn(String table,
String column,
String where)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.loadDistinctStringColumn(table,
column,
where);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadErrorLogByWhere(String where,
int startRow,
int numRows)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.loadErrorLogByWhere(where,
startRow,
numRows),
net.gdface.facelog.client.thrift.ErrorLogBean.class,
ErrorLogBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadFeatureMd5ByUpdate(String timestamp)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.loadFeatureMd5ByUpdateTimeStr(timestamp);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadFeatureMd5ByUpdate(String timestamp,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.loadFeatureMd5ByUpdateTimeStrSafe(timestamp,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadFeatureMd5ByUpdate(long timestamp)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.loadFeatureMd5ByUpdate(timestamp);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadFeatureMd5ByUpdate(long timestamp,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.loadFeatureMd5ByUpdateSafe(timestamp,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadLogByWhere(String where,
int startRow,
int numRows)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.loadLogByWhere(where,
startRow,
numRows),
net.gdface.facelog.client.thrift.LogBean.class,
LogBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadLogByWhere(String where,
int startRow,
int numRows,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.loadLogByWhereSafe(where,
startRow,
numRows,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.LogBean.class,
LogBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadLogLightByVerifyTime(String timestamp,
int startRow,
int numRows)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.loadLogLightByVerifyTimeTimestr(timestamp,
startRow,
numRows),
net.gdface.facelog.client.thrift.LogLightBean.class,
LogLightBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadLogLightByVerifyTime(String timestamp,
int startRow,
int numRows,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.loadLogLightByVerifyTimeTimestrSafe(timestamp,
startRow,
numRows,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.LogLightBean.class,
LogLightBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadLogLightByVerifyTime(long timestamp,
int startRow,
int numRows)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.loadLogLightByVerifyTime(timestamp,
startRow,
numRows),
net.gdface.facelog.client.thrift.LogLightBean.class,
LogLightBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadLogLightByVerifyTime(long timestamp,
int startRow,
int numRows,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.loadLogLightByVerifyTimeSafe(timestamp,
startRow,
numRows,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.LogLightBean.class,
LogLightBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadLogLightByWhere(String where,
int startRow,
int numRows)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.loadLogLightByWhere(where,
startRow,
numRows),
net.gdface.facelog.client.thrift.LogLightBean.class,
LogLightBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadLogLightByWhere(String where,
int startRow,
int numRows,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.loadLogLightByWhereSafe(where,
startRow,
numRows,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.LogLightBean.class,
LogLightBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadPermitByUpdate(String timestamp)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.loadPermitByUpdateTimestr(timestamp),
net.gdface.facelog.client.thrift.PermitBean.class,
PermitBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadPermitByUpdate(long timestamp)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.loadPermitByUpdate(timestamp),
net.gdface.facelog.client.thrift.PermitBean.class,
PermitBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadPersonByWhere(String where,
int startRow,
int numRows)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.loadPersonByWhere(where,
startRow,
numRows),
net.gdface.facelog.client.thrift.PersonBean.class,
PersonBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadPersonByWhere(String where,
int startRow,
int numRows,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.loadPersonByWhereReal(where,
startRow,
numRows,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.PersonBean.class,
PersonBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadPersonDataPackages(List personIds,
String sdkVersion,
int deviceId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.loadPersonDataPackages(personIds,
sdkVersion,
deviceId),
net.gdface.facelog.client.thrift.PersonDataPackage.class,
PersonDataPackage.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadPersonDataPackagesInSameGroup(List personIds,
String sdkVersion,
int deviceId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.loadPersonDataPackagesInSameGroup(personIds,
sdkVersion,
deviceId),
net.gdface.facelog.client.thrift.PersonDataPackage.class,
PersonDataPackage.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadPersonDataPackagesPermittedOnDevice(int deviceId,
boolean ignoreSchedule,
List excludePersonIds,
Long timestamp,
String sdkVersion)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.loadPersonDataPackagesPermittedOnDevice(deviceId,
ignoreSchedule,
excludePersonIds,
timestamp,
sdkVersion),
net.gdface.facelog.client.thrift.PersonDataPackage.class,
PersonDataPackage.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadPersonGroupByWhere(String where,
int startRow,
int numRows)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.loadPersonGroupByWhere(where,
startRow,
numRows);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadPersonGroupIdByWhere(String where)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.loadPersonGroupIdByWhere(where);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadPersonGroupIdByWhere(String where,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.loadPersonGroupIdByWhereSafe(where,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadPersonIdByUpdateTime(String timestamp)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.loadPersonIdByUpdateTimeTimeStr(timestamp);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadPersonIdByUpdateTime(String timestamp,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.loadPersonIdByUpdateTimeTimeStrSafe(timestamp,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadPersonIdByUpdateTime(long timestamp)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.loadPersonIdByUpdateTime(timestamp);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadPersonIdByUpdateTime(long timestamp,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.loadPersonIdByUpdateTimeSafe(timestamp,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadPersonIdByWhere(String where)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.loadPersonIdByWhere(where);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadPersonIdByWhere(String where,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.loadPersonIdByWhereSafe(where,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadUpdatedPersons(String timestamp)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.loadUpdatedPersonsTimestr(timestamp);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadUpdatedPersons(String timestamp,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.loadUpdatedPersonsTimestrSafe(timestamp,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadUpdatedPersons(long timestamp)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.loadUpdatedPersons(timestamp);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public List loadUpdatedPersons(long timestamp,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.loadUpdatedPersonsSafe(timestamp,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public LockWakeupResponse lockWakeup(DeviceBean deviceBean,
boolean ignoreSchedule,
String sdkVersion)
throws ServiceSecurityException{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.lockWakeup(TypeTransformer.getInstance().to(
deviceBean,
DeviceBean.class,
net.gdface.facelog.client.thrift.DeviceBean.class),
ignoreSchedule,
sdkVersion),
net.gdface.facelog.client.thrift.LockWakeupResponse.class,
LockWakeupResponse.class);
}
catch(net.gdface.facelog.client.thrift.ServiceSecurityException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facelog.client.thrift.ServiceSecurityException.class,
ServiceSecurityException.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public void offline(Token token)
throws ServiceSecurityException{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
instance.offline(TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceSecurityException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facelog.client.thrift.ServiceSecurityException.class,
ServiceSecurityException.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public Token online(DeviceBean device)
throws ServiceSecurityException{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.online(TypeTransformer.getInstance().to(
device,
DeviceBean.class,
net.gdface.facelog.client.thrift.DeviceBean.class)),
net.gdface.facelog.client.thrift.Token.class,
Token.class);
}
catch(net.gdface.facelog.client.thrift.ServiceSecurityException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facelog.client.thrift.ServiceSecurityException.class,
ServiceSecurityException.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public String pathOf(String tablename,
int groupId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.pathOf(tablename,
groupId);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public DeviceBean registerDevice(DeviceBean newDevice)
throws ServiceSecurityException{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.registerDevice(TypeTransformer.getInstance().to(
newDevice,
DeviceBean.class,
net.gdface.facelog.client.thrift.DeviceBean.class)),
net.gdface.facelog.client.thrift.DeviceBean.class,
DeviceBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceSecurityException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facelog.client.thrift.ServiceSecurityException.class,
ServiceSecurityException.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public void releasePersonToken(Token token)
throws ServiceSecurityException{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
instance.releasePersonToken(TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceSecurityException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facelog.client.thrift.ServiceSecurityException.class,
ServiceSecurityException.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public void releaseRootToken(Token token)
throws ServiceSecurityException{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
instance.releaseRootToken(TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceSecurityException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facelog.client.thrift.ServiceSecurityException.class,
ServiceSecurityException.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public void releaseUserToken(Token token)
throws ServiceSecurityException{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
instance.releaseUserToken(TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceSecurityException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facelog.client.thrift.ServiceSecurityException.class,
ServiceSecurityException.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public void replaceFeature(int personId,
String featureMd5,
boolean deleteOldFeatureImage,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
instance.replaceFeature(personId,
featureMd5,
deleteOldFeatureImage,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public Integer rootGroupOfDevice(int deviceId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.rootGroupOfDevice(deviceId);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public Integer rootGroupOfDeviceGroup(int groupId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.rootGroupOfDeviceGroup(groupId);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public Integer rootGroupOfPerson(int personId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.rootGroupOfPerson(personId);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public Integer rootGroupOfPersonGroup(int groupId)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.rootGroupOfPersonGroup(groupId);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public String runCmd(List target,
boolean group,
String cmdpath,
String jsonArgs,
String ackChannel,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.runCmd(target,
group,
cmdpath,
jsonArgs,
ackChannel,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public Integer runTask(String taskQueue,
String cmdpath,
String jsonArgs,
String ackChannel,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.runTask(taskQueue,
cmdpath,
jsonArgs,
ackChannel,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public String runTaskSync(String taskQueue,
String cmdpath,
String jsonArgs,
int timeoutSecs,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.runTaskSync(taskQueue,
cmdpath,
jsonArgs,
timeoutSecs,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public DeviceBean saveDevice(DeviceBean deviceBean,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.saveDevice(TypeTransformer.getInstance().to(
deviceBean,
DeviceBean.class,
net.gdface.facelog.client.thrift.DeviceBean.class),
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.DeviceBean.class,
DeviceBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public DeviceGroupBean saveDeviceGroup(DeviceGroupBean deviceGroupBean,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.saveDeviceGroup(TypeTransformer.getInstance().to(
deviceGroupBean,
DeviceGroupBean.class,
net.gdface.facelog.client.thrift.DeviceGroupBean.class),
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.DeviceGroupBean.class,
DeviceGroupBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public PermitBean savePermit(int deviceGroupId,
int personGroupId,
String column,
String value,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.savePermitWithColumn(deviceGroupId,
personGroupId,
column,
value,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.PermitBean.class,
PermitBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public PermitBean savePermit(PermitBean permitBean,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.savePermit(TypeTransformer.getInstance().to(
permitBean,
PermitBean.class,
net.gdface.facelog.client.thrift.PermitBean.class),
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.PermitBean.class,
PermitBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public PersonBean savePerson(PersonBean personBean,
byte[] idPhoto,
boolean exractFeature,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.savePersonWithPhotoAndExtractFeature(TypeTransformer.getInstance().to(
personBean,
PersonBean.class,
net.gdface.facelog.client.thrift.PersonBean.class),
idPhoto,
exractFeature,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.PersonBean.class,
PersonBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public PersonBean savePerson(PersonBean personBean,
byte[] idPhoto,
byte[] feature,
String featureVersion,
byte[] featureImage,
FaceBean faceBean,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.savePersonFull(TypeTransformer.getInstance().to(
personBean,
PersonBean.class,
net.gdface.facelog.client.thrift.PersonBean.class),
idPhoto,
feature,
featureVersion,
featureImage,
TypeTransformer.getInstance().to(
faceBean,
FaceBean.class,
net.gdface.facelog.client.thrift.FaceBean.class),
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.PersonBean.class,
PersonBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public PersonBean savePerson(PersonBean personBean,
byte[] idPhoto,
byte[] feature,
String featureVersion,
List photos,
List faces,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.savePersonWithPhotoAndFeatureMultiImage(TypeTransformer.getInstance().to(
personBean,
PersonBean.class,
net.gdface.facelog.client.thrift.PersonBean.class),
idPhoto,
feature,
featureVersion,
photos,
TypeTransformer.getInstance().to(
faces,
FaceBean.class,
net.gdface.facelog.client.thrift.FaceBean.class),
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.PersonBean.class,
PersonBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public PersonBean savePerson(PersonBean personBean,
byte[] idPhoto,
byte[] feature,
String featureVersion,
List faceBeans,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.savePersonWithPhotoAndFeatureMultiFaces(TypeTransformer.getInstance().to(
personBean,
PersonBean.class,
net.gdface.facelog.client.thrift.PersonBean.class),
idPhoto,
feature,
featureVersion,
TypeTransformer.getInstance().to(
faceBeans,
FaceBean.class,
net.gdface.facelog.client.thrift.FaceBean.class),
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.PersonBean.class,
PersonBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public PersonBean savePerson(PersonBean personBean,
byte[] idPhoto,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.savePersonWithPhoto(TypeTransformer.getInstance().to(
personBean,
PersonBean.class,
net.gdface.facelog.client.thrift.PersonBean.class),
idPhoto,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.PersonBean.class,
PersonBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public PersonBean savePerson(PersonBean personBean,
byte[] idPhoto,
FeatureBean featureBean,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.savePersonWithPhotoAndFeature(TypeTransformer.getInstance().to(
personBean,
PersonBean.class,
net.gdface.facelog.client.thrift.PersonBean.class),
idPhoto,
TypeTransformer.getInstance().to(
featureBean,
FeatureBean.class,
net.gdface.facelog.client.thrift.FeatureBean.class),
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.PersonBean.class,
PersonBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public PersonBean savePerson(PersonBean personBean,
String idPhotoMd5,
String featureMd5,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.savePersonWithPhotoAndFeatureSaved(TypeTransformer.getInstance().to(
personBean,
PersonBean.class,
net.gdface.facelog.client.thrift.PersonBean.class),
idPhotoMd5,
featureMd5,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.PersonBean.class,
PersonBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public PersonBean savePerson(PersonBean personBean,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.savePerson(TypeTransformer.getInstance().to(
personBean,
PersonBean.class,
net.gdface.facelog.client.thrift.PersonBean.class),
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.PersonBean.class,
PersonBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public PersonGroupBean savePersonGroup(PersonGroupBean personGroupBean,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.savePersonGroup(TypeTransformer.getInstance().to(
personGroupBean,
PersonGroupBean.class,
net.gdface.facelog.client.thrift.PersonGroupBean.class),
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.PersonGroupBean.class,
PersonGroupBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public int savePersons(List photos,
List persons,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.savePersonsWithPhoto(photos,
TypeTransformer.getInstance().to(
persons,
PersonBean.class,
net.gdface.facelog.client.thrift.PersonBean.class),
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public void savePersons(List persons,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
instance.savePersons(TypeTransformer.getInstance().to(
persons,
PersonBean.class,
net.gdface.facelog.client.thrift.PersonBean.class),
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public void saveServiceConfig(Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
instance.saveServiceConfig(TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public String sdkTaskQueueOf(String task,
String sdkVersion,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.sdkTaskQueueOf(task,
sdkVersion,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public void setPersonExpiryDate(int personId,
String expiryDate,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
instance.setPersonExpiryDateTimeStr(personId,
expiryDate,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public void setPersonExpiryDate(int personId,
long expiryDate,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
instance.setPersonExpiryDate(personId,
expiryDate,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public void setPersonExpiryDate(List personIdList,
long expiryDate,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
instance.setPersonExpiryDateList(personIdList,
expiryDate,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public void setProperties(Map config,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
instance.setProperties(TypeTransformer.getInstance().to(
config,
String.class,
String.class,
String.class,
String.class),
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public void setProperty(String key,
String value,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
instance.setProperty(key,
value,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public String taskQueueOf(String task,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.taskQueueOf(task,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public void unbindBorder(int personGroupId,
int deviceGroupId,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
instance.unbindBorder(personGroupId,
deviceGroupId,
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public void unregisterDevice(Token token)
throws ServiceSecurityException{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
instance.unregisterDevice(TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class));
}
catch(net.gdface.facelog.client.thrift.ServiceSecurityException e){
throw TypeTransformer.getInstance().to(
e,
net.gdface.facelog.client.thrift.ServiceSecurityException.class,
ServiceSecurityException.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public DeviceBean updateDevice(DeviceBean deviceBean,
Token token)
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.updateDevice(TypeTransformer.getInstance().to(
deviceBean,
DeviceBean.class,
net.gdface.facelog.client.thrift.DeviceBean.class),
TypeTransformer.getInstance().to(
token,
Token.class,
net.gdface.facelog.client.thrift.Token.class)),
net.gdface.facelog.client.thrift.DeviceBean.class,
DeviceBean.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public String version()
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return instance.version();
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
@Override
public Map versionInfo()
{
net.gdface.facelog.client.thrift.IFaceLog instance = delegate();
try{
return TypeTransformer.getInstance().to(
instance.versionInfo(),
String.class,
String.class,
String.class,
String.class);
}
catch(net.gdface.facelog.client.thrift.ServiceRuntimeException e){
throw new ServiceRuntimeException(e);
}
catch(RuntimeTApplicationException e){
return com.gitee.l0km.xthrift.thrift.ThriftUtils.returnNull(e);
}
finally{
factory.releaseInstance(instance);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy