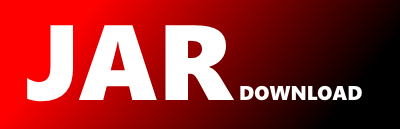
net.gdface.facelog.db.mysql.DeviceGroupManager Maven / Gradle / Ivy
// ______________________________________________________
// Generated by sql2java - https://github.com/10km/sql2java-2-6-7 (custom branch)
// modified by guyadong from
// sql2java original version https://sourceforge.net/projects/sql2java/
// JDBC driver used at code generation time: com.mysql.jdbc.Driver
// template: manager.java.vm
// ______________________________________________________
package net.gdface.facelog.db.mysql;
import java.util.concurrent.Callable;
import net.gdface.facelog.db.Constant;
import net.gdface.facelog.db.DeviceGroupBean;
import net.gdface.facelog.db.IBeanConverter;
import net.gdface.facelog.db.IDbConverter;
import net.gdface.facelog.db.TableManager;
import net.gdface.facelog.db.IDeviceGroupManager;
import net.gdface.facelog.db.DeviceBean;
import net.gdface.facelog.db.PermitBean;
import net.gdface.facelog.db.PersonGroupBean;
import net.gdface.facelog.db.TableListener;
import net.gdface.facelog.db.exception.RuntimeDaoException;
import net.gdface.facelog.db.exception.ObjectRetrievalException;
import net.gdface.facelog.dborm.exception.DaoException;
/**
* Handles database calls (save, load, count, etc...) for the fl_device_group table.
* all {@link DaoException} be wrapped as {@link RuntimeDaoException} to throw.
* Remarks: 设备组信息
* @author guyadong
*/
public class DeviceGroupManager extends TableManager.BaseAdapter implements IDeviceGroupManager
{
private net.gdface.facelog.dborm.device.FlDeviceGroupManager nativeManager = net.gdface.facelog.dborm.device.FlDeviceGroupManager.getInstance();
private IDbConverter<
net.gdface.facelog.dborm.device.FlDeviceBean,
net.gdface.facelog.dborm.device.FlDeviceGroupBean,
net.gdface.facelog.dborm.face.FlFaceBean,
net.gdface.facelog.dborm.face.FlFeatureBean,
net.gdface.facelog.dborm.image.FlImageBean,
net.gdface.facelog.dborm.log.FlLogBean,
net.gdface.facelog.dborm.permit.FlPermitBean,
net.gdface.facelog.dborm.person.FlPersonBean,
net.gdface.facelog.dborm.person.FlPersonGroupBean,
net.gdface.facelog.dborm.image.FlStoreBean,
net.gdface.facelog.dborm.log.FlLogLightBean> dbConverter = DbConverter.INSTANCE;
private IBeanConverter beanConverter = dbConverter.getDeviceGroupBeanConverter();
private static DeviceGroupManager singleton = new DeviceGroupManager();
protected DeviceGroupManager(){}
protected DeviceManager instanceOfDeviceManager(){
return DeviceManager.getInstance();
}
protected PermitManager instanceOfPermitManager(){
return PermitManager.getInstance();
}
protected PersonGroupManager instanceOfPersonGroupManager(){
return PersonGroupManager.getInstance();
}
protected DeviceGroupManager instanceOfDeviceGroupManager(){
return this;
}
@Override
public String getTableName() {
return this.nativeManager.getTableName();
}
@Override
public String getFields() {
return this.nativeManager.getFields();
}
@Override
public String getFullFields() {
return this.nativeManager.getFullFields();
}
@Override
public String[] getPrimarykeyNames() {
return this.nativeManager.getPrimarykeyNames();
}
/**
* Get the {@link DeviceGroupManager} singleton.
*
* @return {@link DeviceGroupManager}
*/
public static DeviceGroupManager getInstance()
{
return singleton;
}
@Override
protected Class beanType(){
return DeviceGroupBean.class;
}
//////////////////////////////////////
// PRIMARY KEY METHODS
//////////////////////////////////////
//1 override IDeviceGroupManager
@Override
public DeviceGroupBean loadByPrimaryKey(Integer id)
{
try{
return loadByPrimaryKeyChecked(id);
}catch(ObjectRetrievalException e){
// not found
return null;
}
}
//1.1 override IDeviceGroupManager
@Override
public DeviceGroupBean loadByPrimaryKeyChecked(Integer id) throws ObjectRetrievalException
{
try{
return this.beanConverter.fromRight(nativeManager.loadByPrimaryKeyChecked(id));
}catch(net.gdface.facelog.dborm.exception.ObjectRetrievalException e){
throw new ObjectRetrievalException();
}catch(DaoException e){
throw new RuntimeDaoException(e);
}
}
//1.2
@Override
public DeviceGroupBean loadByPrimaryKey(DeviceGroupBean bean)
{
return bean==null?null:loadByPrimaryKey(bean.getId());
}
//1.2.2
@Override
public DeviceGroupBean loadByPrimaryKeyChecked(DeviceGroupBean bean) throws ObjectRetrievalException
{
if(null == bean){
throw new NullPointerException();
}
return loadByPrimaryKeyChecked(bean.getId());
}
//1.3
@Override
public DeviceGroupBean loadByPrimaryKey(Object ...keys){
try{
return loadByPrimaryKeyChecked(keys);
}catch(ObjectRetrievalException e){
// not found
return null;
}
}
//1.3.2
@Override
public DeviceGroupBean loadByPrimaryKeyChecked(Object ...keys) throws ObjectRetrievalException{
if(null == keys){
throw new NullPointerException();
}
if(keys.length != FL_DEVICE_GROUP_PK_COUNT){
throw new IllegalArgumentException("argument number mismatch with primary key number");
}
if(! (keys[0] instanceof Integer)){
throw new IllegalArgumentException("invalid type for the No.1 argument,expected type:Integer");
}
return loadByPrimaryKeyChecked((Integer)keys[0]);
}
//1.4 override IDeviceGroupManager
@Override
public boolean existsPrimaryKey(Integer id)
{
try{
return nativeManager.existsPrimaryKey(id);
}
catch(DaoException e)
{
throw new RuntimeDaoException(e);
}
}
//1.6
@Override
public boolean existsByPrimaryKey(DeviceGroupBean bean)
{
return null == bean ? false : existsPrimaryKey(bean.getId());
}
//1.7
@Override
public DeviceGroupBean checkDuplicate(DeviceGroupBean bean)throws ObjectRetrievalException{
if(null != bean){
checkDuplicate(bean.getId());
}
return bean;
}
//1.4.1 override IDeviceGroupManager
@Override
public Integer checkDuplicate(Integer id)throws ObjectRetrievalException{
try{
return this.nativeManager.checkDuplicate(id);
}catch(net.gdface.facelog.dborm.exception.ObjectRetrievalException e){
throw new ObjectRetrievalException(e);
}catch(DaoException e){
throw new RuntimeDaoException(e);
}
}
//1.8 override IDeviceGroupManager
@Override
public java.util.List loadByPrimaryKey(int... keys){
if(null == keys){
return new java.util.ArrayList();
}
java.util.ArrayList list = new java.util.ArrayList(keys.length);
for(int i = 0 ;i< keys.length;++i){
list.add(loadByPrimaryKey(keys[i]));
}
return list;
}
//1.9 override IDeviceGroupManager
@Override
public java.util.List loadByPrimaryKey(java.util.Collection keys){
if(null == keys ){
return new java.util.ArrayList();
}
java.util.ArrayList list = new java.util.ArrayList(keys.size());
if(keys instanceof java.util.List){
for(Integer key: keys){
list.add(loadByPrimaryKey(key));
}
}else{
DeviceGroupBean bean;
for(Integer key: keys){
if(null != (bean = loadByPrimaryKey(key))){
list.add(bean);
}
}
}
return list;
}
//2 override IDeviceGroupManager
@Override
public int deleteByPrimaryKey(Integer id)
{
try
{
return nativeManager.deleteByPrimaryKey(id);
}
catch(DaoException e)
{
throw new RuntimeDaoException(e);
}
}
//2
@Override
public int delete(DeviceGroupBean bean){
try
{
return nativeManager.delete(this.beanConverter.toRight(bean));
}
catch(DaoException e)
{
throw new RuntimeDaoException(e);
}
}
//2.1
@Override
public int deleteByPrimaryKey(Object ...keys){
if(null == keys){
throw new NullPointerException();
}
if(keys.length != FL_DEVICE_GROUP_PK_COUNT){
throw new IllegalArgumentException("argument number mismatch with primary key number");
}
if(! (keys[0] instanceof Integer)){
throw new IllegalArgumentException("invalid type for the No.1 argument,expected type:Integer");
}
return deleteByPrimaryKey((Integer)keys[0]);
}
//2.2 override IDeviceGroupManager
@Override
public int deleteByPrimaryKey(int... keys){
int count = 0;
if(null != keys){
for(int key:keys){
count += deleteByPrimaryKey(key);
}
}
return count;
}
//2.3 override IDeviceGroupManager
@Override
public int deleteByPrimaryKey(java.util.Collection keys){
int count = 0;
if(null != keys){
for(Integer key :keys){
count += deleteByPrimaryKey(key);
}
}
return count;
}
//2.4 override IDeviceGroupManager
@Override
public int delete(DeviceGroupBean... beans){
int count = 0;
if(null != beans){
for(DeviceGroupBean bean :beans){
count += delete(bean);
}
}
return count;
}
//2.5 override IDeviceGroupManager
@Override
public int delete(java.util.Collection beans){
int count = 0;
if(null != beans){
for(DeviceGroupBean bean :beans){
count += delete(bean);
}
}
return count;
}
//////////////////////////////////////
// IMPORT KEY GENERIC METHOD
//////////////////////////////////////
private static final Class>[] IMPORTED_BEAN_TYPES = new Class>[]{DeviceBean.class,DeviceGroupBean.class,PermitBean.class};
/**
* @see #getImportedBeansAsList(DeviceGroupBean,int)
*/
@SuppressWarnings("unchecked")
@Override
public > T[] getImportedBeans(DeviceGroupBean bean, int ikIndex){
return getImportedBeansAsList(bean, ikIndex).toArray((T[])java.lang.reflect.Array.newInstance(IMPORTED_BEAN_TYPES[ikIndex],0));
}
/**
* Retrieves imported T objects by ikIndex.
* @param
*
* - {@link Constant#FL_DEVICE_GROUP_IK_FL_DEVICE_GROUP_ID} TO {@link DeviceBean}
* - {@link Constant#FL_DEVICE_GROUP_IK_FL_DEVICE_GROUP_PARENT} TO {@link DeviceGroupBean}
* - {@link Constant#FL_DEVICE_GROUP_IK_FL_PERMIT_DEVICE_GROUP_ID} TO {@link PermitBean}
*
* @param bean the {@link DeviceGroupBean} object to use
* @param ikIndex valid values: {@link Constant#FL_DEVICE_GROUP_IK_FL_DEVICE_GROUP_ID},{@link Constant#FL_DEVICE_GROUP_IK_FL_DEVICE_GROUP_PARENT},{@link Constant#FL_DEVICE_GROUP_IK_FL_PERMIT_DEVICE_GROUP_ID}
* @return the associated T beans or {@code null} if {@code bean} is {@code null}
*/
@SuppressWarnings("unchecked")
@Override
public > java.util.List getImportedBeansAsList(DeviceGroupBean bean,int ikIndex){
switch(ikIndex){
case FL_DEVICE_GROUP_IK_FL_DEVICE_GROUP_ID:
return (java.util.List)this.getDeviceBeansByGroupIdAsList(bean);
case FL_DEVICE_GROUP_IK_FL_DEVICE_GROUP_PARENT:
return (java.util.List)this.getDeviceGroupBeansByParentAsList(bean);
case FL_DEVICE_GROUP_IK_FL_PERMIT_DEVICE_GROUP_ID:
return (java.util.List)this.getPermitBeansByDeviceGroupIdAsList(bean);
default:
throw new IllegalArgumentException(String.format("invalid ikIndex %d", ikIndex));
}
}
/**
* Set the T objects as imported beans of bean object by ikIndex.
* @param
*
*
* - {@link Constant#FL_DEVICE_GROUP_IK_FL_DEVICE_GROUP_ID} TO {@link DeviceBean}
* - {@link Constant#FL_DEVICE_GROUP_IK_FL_DEVICE_GROUP_PARENT} TO {@link DeviceGroupBean}
* - {@link Constant#FL_DEVICE_GROUP_IK_FL_PERMIT_DEVICE_GROUP_ID} TO {@link PermitBean}
*
* @param bean the {@link DeviceGroupBean} object to use
* @param importedBeans the FlPermitBean array to associate to the {@link DeviceGroupBean}
* @param ikIndex valid values: {@link Constant#FL_DEVICE_GROUP_IK_FL_DEVICE_GROUP_ID},{@link Constant#FL_DEVICE_GROUP_IK_FL_DEVICE_GROUP_PARENT},{@link Constant#FL_DEVICE_GROUP_IK_FL_PERMIT_DEVICE_GROUP_ID}
* @return importedBeans always
*/
@SuppressWarnings("unchecked")
@Override
public > T[] setImportedBeans(DeviceGroupBean bean,T[] importedBeans,int ikIndex){
switch(ikIndex){
case FL_DEVICE_GROUP_IK_FL_DEVICE_GROUP_ID:
return (T[])setDeviceBeansByGroupId(bean,(DeviceBean[])importedBeans);
case FL_DEVICE_GROUP_IK_FL_DEVICE_GROUP_PARENT:
return (T[])setDeviceGroupBeansByParent(bean,(DeviceGroupBean[])importedBeans);
case FL_DEVICE_GROUP_IK_FL_PERMIT_DEVICE_GROUP_ID:
return (T[])setPermitBeansByDeviceGroupId(bean,(PermitBean[])importedBeans);
default:
throw new IllegalArgumentException(String.format("invalid ikIndex %d", ikIndex));
}
}
/**
* Set the importedBeans associates to the bean by ikIndex
* @param
*
* - {@link Constant#FL_DEVICE_GROUP_IK_FL_DEVICE_GROUP_ID} TO {@link DeviceBean}
* - {@link Constant#FL_DEVICE_GROUP_IK_FL_DEVICE_GROUP_PARENT} TO {@link DeviceGroupBean}
* - {@link Constant#FL_DEVICE_GROUP_IK_FL_PERMIT_DEVICE_GROUP_ID} TO {@link PermitBean}
*
* @param bean the {@link DeviceGroupBean} object to use
* @param importedBeans the T object to associate to the {@link DeviceGroupBean}
* @param ikIndex valid values: {@link Constant#FL_DEVICE_GROUP_IK_FL_DEVICE_GROUP_ID},{@link Constant#FL_DEVICE_GROUP_IK_FL_DEVICE_GROUP_PARENT},{@link Constant#FL_DEVICE_GROUP_IK_FL_PERMIT_DEVICE_GROUP_ID}
* @return importedBeans always
*/
@SuppressWarnings("unchecked")
@Override
public ,C extends java.util.Collection> C setImportedBeans(DeviceGroupBean bean,C importedBeans,int ikIndex){
switch(ikIndex){
case FL_DEVICE_GROUP_IK_FL_DEVICE_GROUP_ID:
return (C)setDeviceBeansByGroupId(bean,(java.util.Collection)importedBeans);
case FL_DEVICE_GROUP_IK_FL_DEVICE_GROUP_PARENT:
return (C)setDeviceGroupBeansByParent(bean,(java.util.Collection)importedBeans);
case FL_DEVICE_GROUP_IK_FL_PERMIT_DEVICE_GROUP_ID:
return (C)setPermitBeansByDeviceGroupId(bean,(java.util.Collection)importedBeans);
default:
throw new IllegalArgumentException(String.format("invalid ikIndex %d", ikIndex));
}
}
//////////////////////////////////////
// GET/SET IMPORTED KEY BEAN METHOD
//////////////////////////////////////
//3.1 GET IMPORTED override IDeviceGroupManager
@Override
public DeviceBean[] getDeviceBeansByGroupId(DeviceGroupBean bean)
{
return this.getDeviceBeansByGroupIdAsList(bean).toArray(new DeviceBean[0]);
}
//3.1.2 GET IMPORTED override IDeviceGroupManager
@Override
public DeviceBean[] getDeviceBeansByGroupId(Integer idOfDeviceGroup)
{
DeviceGroupBean bean = new DeviceGroupBean();
bean.setId(idOfDeviceGroup);
return getDeviceBeansByGroupId(bean);
}
//3.2 GET IMPORTED override IDeviceGroupManager
@Override
public java.util.List getDeviceBeansByGroupIdAsList(DeviceGroupBean bean)
{
return getDeviceBeansByGroupIdAsList(bean,1,-1);
}
//3.2.2 GET IMPORTED override IDeviceGroupManager
@Override
public java.util.List getDeviceBeansByGroupIdAsList(Integer idOfDeviceGroup)
{
DeviceGroupBean bean = new DeviceGroupBean();
bean.setId(idOfDeviceGroup);
return getDeviceBeansByGroupIdAsList(bean);
}
//3.2.3 DELETE IMPORTED override IDeviceGroupManager
@Override
public int deleteDeviceBeansByGroupId(Integer idOfDeviceGroup)
{
java.util.List list =getDeviceBeansByGroupIdAsList(idOfDeviceGroup);
return instanceOfDeviceManager().delete(list);
}
//3.2.4 GET IMPORTED override IDeviceGroupManager
@Override
public java.util.List getDeviceBeansByGroupIdAsList(DeviceGroupBean bean,int startRow, int numRows)
{
try {
return this.dbConverter.getDeviceBeanConverter().fromRight(nativeManager.getDeviceBeansByGroupIdAsList( this.beanConverter.toRight(bean),startRow,numRows));
}
catch(DaoException e)
{
throw new RuntimeDaoException(e);
}
}
//3.3 SET IMPORTED override IDeviceGroupManager
@Override
public DeviceBean[] setDeviceBeansByGroupId(DeviceGroupBean bean , DeviceBean[] importedBeans)
{
if(null != importedBeans){
for( DeviceBean importBean : importedBeans ){
instanceOfDeviceManager().setReferencedByGroupId(importBean , bean);
}
}
return importedBeans;
}
//3.4 SET IMPORTED override IDeviceGroupManager
@Override
public > C setDeviceBeansByGroupId(DeviceGroupBean bean , C importedBeans)
{
if(null != importedBeans){
for( DeviceBean importBean : importedBeans ){
instanceOfDeviceManager().setReferencedByGroupId(importBean , bean);
}
}
return importedBeans;
}
//3.1 GET IMPORTED override IDeviceGroupManager
@Override
public DeviceGroupBean[] getDeviceGroupBeansByParent(DeviceGroupBean bean)
{
return this.getDeviceGroupBeansByParentAsList(bean).toArray(new DeviceGroupBean[0]);
}
//3.1.2 GET IMPORTED override IDeviceGroupManager
@Override
public DeviceGroupBean[] getDeviceGroupBeansByParent(Integer idOfDeviceGroup)
{
DeviceGroupBean bean = new DeviceGroupBean();
bean.setId(idOfDeviceGroup);
return getDeviceGroupBeansByParent(bean);
}
//3.2 GET IMPORTED override IDeviceGroupManager
@Override
public java.util.List getDeviceGroupBeansByParentAsList(DeviceGroupBean bean)
{
return getDeviceGroupBeansByParentAsList(bean,1,-1);
}
//3.2.2 GET IMPORTED override IDeviceGroupManager
@Override
public java.util.List getDeviceGroupBeansByParentAsList(Integer idOfDeviceGroup)
{
DeviceGroupBean bean = new DeviceGroupBean();
bean.setId(idOfDeviceGroup);
return getDeviceGroupBeansByParentAsList(bean);
}
//3.2.3 DELETE IMPORTED override IDeviceGroupManager
@Override
public int deleteDeviceGroupBeansByParent(Integer idOfDeviceGroup)
{
java.util.List list =getDeviceGroupBeansByParentAsList(idOfDeviceGroup);
return instanceOfDeviceGroupManager().delete(list);
}
//3.2.4 GET IMPORTED override IDeviceGroupManager
@Override
public java.util.List getDeviceGroupBeansByParentAsList(DeviceGroupBean bean,int startRow, int numRows)
{
try {
return this.dbConverter.getDeviceGroupBeanConverter().fromRight(nativeManager.getDeviceGroupBeansByParentAsList( this.beanConverter.toRight(bean),startRow,numRows));
}
catch(DaoException e)
{
throw new RuntimeDaoException(e);
}
}
//3.3 SET IMPORTED override IDeviceGroupManager
@Override
public DeviceGroupBean[] setDeviceGroupBeansByParent(DeviceGroupBean bean , DeviceGroupBean[] importedBeans)
{
if(null != importedBeans){
for( DeviceGroupBean importBean : importedBeans ){
instanceOfDeviceGroupManager().setReferencedByParent(importBean , bean);
}
}
return importedBeans;
}
//3.4 SET IMPORTED override IDeviceGroupManager
@Override
public > C setDeviceGroupBeansByParent(DeviceGroupBean bean , C importedBeans)
{
if(null != importedBeans){
for( DeviceGroupBean importBean : importedBeans ){
instanceOfDeviceGroupManager().setReferencedByParent(importBean , bean);
}
}
return importedBeans;
}
//3.1 GET IMPORTED override IDeviceGroupManager
@Override
public PermitBean[] getPermitBeansByDeviceGroupId(DeviceGroupBean bean)
{
return this.getPermitBeansByDeviceGroupIdAsList(bean).toArray(new PermitBean[0]);
}
//3.1.2 GET IMPORTED override IDeviceGroupManager
@Override
public PermitBean[] getPermitBeansByDeviceGroupId(Integer idOfDeviceGroup)
{
DeviceGroupBean bean = new DeviceGroupBean();
bean.setId(idOfDeviceGroup);
return getPermitBeansByDeviceGroupId(bean);
}
//3.2 GET IMPORTED override IDeviceGroupManager
@Override
public java.util.List getPermitBeansByDeviceGroupIdAsList(DeviceGroupBean bean)
{
return getPermitBeansByDeviceGroupIdAsList(bean,1,-1);
}
//3.2.2 GET IMPORTED override IDeviceGroupManager
@Override
public java.util.List getPermitBeansByDeviceGroupIdAsList(Integer idOfDeviceGroup)
{
DeviceGroupBean bean = new DeviceGroupBean();
bean.setId(idOfDeviceGroup);
return getPermitBeansByDeviceGroupIdAsList(bean);
}
//3.2.3 DELETE IMPORTED override IDeviceGroupManager
@Override
public int deletePermitBeansByDeviceGroupId(Integer idOfDeviceGroup)
{
java.util.List list =getPermitBeansByDeviceGroupIdAsList(idOfDeviceGroup);
return instanceOfPermitManager().delete(list);
}
//3.2.4 GET IMPORTED override IDeviceGroupManager
@Override
public java.util.List getPermitBeansByDeviceGroupIdAsList(DeviceGroupBean bean,int startRow, int numRows)
{
try {
return this.dbConverter.getPermitBeanConverter().fromRight(nativeManager.getPermitBeansByDeviceGroupIdAsList( this.beanConverter.toRight(bean),startRow,numRows));
}
catch(DaoException e)
{
throw new RuntimeDaoException(e);
}
}
//3.3 SET IMPORTED override IDeviceGroupManager
@Override
public PermitBean[] setPermitBeansByDeviceGroupId(DeviceGroupBean bean , PermitBean[] importedBeans)
{
if(null != importedBeans){
for( PermitBean importBean : importedBeans ){
instanceOfPermitManager().setReferencedByDeviceGroupId(importBean , bean);
}
}
return importedBeans;
}
//3.4 SET IMPORTED override IDeviceGroupManager
@Override
public > C setPermitBeansByDeviceGroupId(DeviceGroupBean bean , C importedBeans)
{
if(null != importedBeans){
for( PermitBean importBean : importedBeans ){
instanceOfPermitManager().setReferencedByDeviceGroupId(importBean , bean);
}
}
return importedBeans;
}
//3.5 SYNC SAVE override IDeviceGroupManager
@Override
public DeviceGroupBean save(DeviceGroupBean bean
, DeviceGroupBean refDevicegroupByParent
, DeviceBean[] impDeviceByGroupId , DeviceGroupBean[] impDevicegroupByParent , PermitBean[] impPermitByDeviceGroupId )
{
if(null == bean){
return null;
}
if(null != refDevicegroupByParent){
this.setReferencedByParent(bean,refDevicegroupByParent);
}
bean = this.save( bean );
if(null != impDeviceByGroupId){
this.setDeviceBeansByGroupId(bean,impDeviceByGroupId);
instanceOfDeviceManager().save( impDeviceByGroupId );
}
if(null != impDevicegroupByParent){
this.setDeviceGroupBeansByParent(bean,impDevicegroupByParent);
instanceOfDeviceGroupManager().save( impDevicegroupByParent );
}
if(null != impPermitByDeviceGroupId){
this.setPermitBeansByDeviceGroupId(bean,impPermitByDeviceGroupId);
instanceOfPermitManager().save( impPermitByDeviceGroupId );
}
return bean;
}
//3.6 SYNC SAVE AS TRANSACTION override IDeviceGroupManager
@Override
public DeviceGroupBean saveAsTransaction(final DeviceGroupBean bean
,final DeviceGroupBean refDevicegroupByParent
,final DeviceBean[] impDeviceByGroupId ,final DeviceGroupBean[] impDevicegroupByParent ,final PermitBean[] impPermitByDeviceGroupId )
{
return this.runAsTransaction(new Callable(){
@Override
public DeviceGroupBean call() throws Exception {
return save(bean , refDevicegroupByParent , impDeviceByGroupId , impDevicegroupByParent , impPermitByDeviceGroupId );
}});
}
//3.7 SYNC SAVE override IDeviceGroupManager
@Override
public DeviceGroupBean save(DeviceGroupBean bean
, DeviceGroupBean refDevicegroupByParent
, java.util.Collection impDeviceByGroupId , java.util.Collection impDevicegroupByParent , java.util.Collection impPermitByDeviceGroupId )
{
if(null == bean){
return null;
}
if(null != refDevicegroupByParent){
this.setReferencedByParent(bean,refDevicegroupByParent);
}
bean = this.save( bean );
if(null != impDeviceByGroupId){
this.setDeviceBeansByGroupId(bean,impDeviceByGroupId);
instanceOfDeviceManager().save( impDeviceByGroupId );
}
if(null != impDevicegroupByParent){
this.setDeviceGroupBeansByParent(bean,impDevicegroupByParent);
instanceOfDeviceGroupManager().save( impDevicegroupByParent );
}
if(null != impPermitByDeviceGroupId){
this.setPermitBeansByDeviceGroupId(bean,impPermitByDeviceGroupId);
instanceOfPermitManager().save( impPermitByDeviceGroupId );
}
return bean;
}
//3.8 SYNC SAVE AS TRANSACTION override IDeviceGroupManager
@Override
public DeviceGroupBean saveAsTransaction(final DeviceGroupBean bean
,final DeviceGroupBean refDevicegroupByParent
,final java.util.Collection impDeviceByGroupId ,final java.util.Collection impDevicegroupByParent ,final java.util.Collection impPermitByDeviceGroupId )
{
return this.runAsTransaction(new Callable(){
@Override
public DeviceGroupBean call() throws Exception {
return save(bean , refDevicegroupByParent , impDeviceByGroupId , impDevicegroupByParent , impPermitByDeviceGroupId );
}});
}
private static final int SYNC_SAVE_ARG_LEN = 4;
private static final int SYNC_SAVE_ARG_0 = 0;
private static final int SYNC_SAVE_ARG_1 = 1;
private static final int SYNC_SAVE_ARG_2 = 2;
private static final int SYNC_SAVE_ARG_3 = 3;
//3.9 SYNC SAVE
/**
* Save the {@link DeviceGroupBean} bean and referenced beans and imported beans into the database.
*
* @param bean the {@link DeviceGroupBean} bean to be saved
* @param inputs referenced beans or imported beans
* see also {@link #save(DeviceGroupBean , DeviceGroupBean , DeviceBean[] , DeviceGroupBean[] , PermitBean[] )}
* @return the inserted or updated {@link DeviceGroupBean} bean
*/
@Override
public DeviceGroupBean save(DeviceGroupBean bean,Object ...inputs)
{
if(null == inputs){
return save(bean);
}
if(inputs.length > SYNC_SAVE_ARG_LEN){
throw new IllegalArgumentException("too many dynamic arguments,max dynamic arguments number: 4");
}
Object[] args = new Object[SYNC_SAVE_ARG_LEN];
System.arraycopy(inputs, 0, args, 0, inputs.length);
if( null != args[SYNC_SAVE_ARG_0] && !(args[SYNC_SAVE_ARG_0] instanceof DeviceGroupBean)){
throw new IllegalArgumentException("invalid type for the No.1 dynamic argument,expected type:DeviceGroupBean");
}
if( null != args[SYNC_SAVE_ARG_1] && !(args[SYNC_SAVE_ARG_1] instanceof DeviceBean[])){
throw new IllegalArgumentException("invalid type for the No.2 argument,expected type:DeviceBean[]");
}
if( null != args[SYNC_SAVE_ARG_2] && !(args[SYNC_SAVE_ARG_2] instanceof DeviceGroupBean[])){
throw new IllegalArgumentException("invalid type for the No.3 argument,expected type:DeviceGroupBean[]");
}
if( null != args[SYNC_SAVE_ARG_3] && !(args[SYNC_SAVE_ARG_3] instanceof PermitBean[])){
throw new IllegalArgumentException("invalid type for the No.4 argument,expected type:PermitBean[]");
}
return save(bean,
(DeviceGroupBean)args[SYNC_SAVE_ARG_0],
(DeviceBean[])args[SYNC_SAVE_ARG_1],
(DeviceGroupBean[])args[SYNC_SAVE_ARG_2],
(PermitBean[])args[SYNC_SAVE_ARG_3]);
}
//3.10 SYNC SAVE
/**
* Save the {@link DeviceGroupBean} bean and referenced beans and imported beans into the database.
*
* @param bean the {@link DeviceGroupBean} bean to be saved
* @param inputs referenced beans or imported beans
* see also {@link #save(DeviceGroupBean , DeviceGroupBean , java.util.Collection , java.util.Collection , java.util.Collection )}
* @return the inserted or updated {@link DeviceGroupBean} bean
*/
@SuppressWarnings("unchecked")
@Override
public DeviceGroupBean saveCollection(DeviceGroupBean bean,Object ...inputs)
{
if(null == inputs){
return save(bean);
}
if(inputs.length > SYNC_SAVE_ARG_LEN){
throw new IllegalArgumentException("too many dynamic arguments,max dynamic arguments number: 4");
}
Object[] args = new Object[SYNC_SAVE_ARG_LEN];
System.arraycopy(inputs, 0, args, 0, inputs.length);
if( null != args[SYNC_SAVE_ARG_0] && !(args[SYNC_SAVE_ARG_0] instanceof DeviceGroupBean)){
throw new IllegalArgumentException("invalid type for the No.1 dynamic argument,expected type:DeviceGroupBean");
}
if( null != args[SYNC_SAVE_ARG_1] && !(args[SYNC_SAVE_ARG_1] instanceof java.util.Collection)){
throw new IllegalArgumentException("invalid type for the No.2 argument,expected type:java.util.Collection");
}
if( null != args[SYNC_SAVE_ARG_2] && !(args[SYNC_SAVE_ARG_2] instanceof java.util.Collection)){
throw new IllegalArgumentException("invalid type for the No.3 argument,expected type:java.util.Collection");
}
if( null != args[SYNC_SAVE_ARG_3] && !(args[SYNC_SAVE_ARG_3] instanceof java.util.Collection)){
throw new IllegalArgumentException("invalid type for the No.4 argument,expected type:java.util.Collection");
}
return save(bean,
(DeviceGroupBean)args[SYNC_SAVE_ARG_0],
(java.util.Collection)args[SYNC_SAVE_ARG_1],
(java.util.Collection)args[SYNC_SAVE_ARG_2],
(java.util.Collection)args[SYNC_SAVE_ARG_3]);
}
//////////////////////////////////////
// FOREIGN KEY GENERIC METHOD
//////////////////////////////////////
/**
* Retrieves the bean object referenced by fkIndex.
* @param
*
* - {@link Constant#FL_DEVICE_GROUP_FK_PARENT} TO {@link DeviceGroupBean}
*
* @param bean the {@link DeviceGroupBean} object to use
* @param fkIndex valid values:
* {@link Constant#FL_DEVICE_GROUP_FK_PARENT}
* @return the associated T bean or {@code null} if {@code bean} or {@code beanToSet} is {@code null}
*/
@SuppressWarnings("unchecked")
@Override
public > T getReferencedBean(DeviceGroupBean bean,int fkIndex){
switch(fkIndex){
case FL_DEVICE_GROUP_FK_PARENT:
return (T)this.getReferencedByParent(bean);
default:
throw new IllegalArgumentException(String.format("invalid fkIndex %d", fkIndex));
}
}
/**
* Associates the {@link DeviceGroupBean} object to the bean object by fkIndex field.
*
* @param see also {@link #getReferencedBean(DeviceGroupBean,int)}
* @param bean the {@link DeviceGroupBean} object to use
* @param beanToSet the T object to associate to the {@link DeviceGroupBean}
* @param fkIndex valid values: see also {@link #getReferencedBean(DeviceGroupBean,int)}
* @return always beanToSet saved
*/
@SuppressWarnings("unchecked")
@Override
public > T setReferencedBean(DeviceGroupBean bean,T beanToSet,int fkIndex){
switch(fkIndex){
case FL_DEVICE_GROUP_FK_PARENT:
return (T)this.setReferencedByParent(bean, (DeviceGroupBean)beanToSet);
default:
throw new IllegalArgumentException(String.format("invalid fkIndex %d", fkIndex));
}
}
//////////////////////////////////////
// GET/SET FOREIGN KEY BEAN METHOD
//////////////////////////////////////
//5.1 GET REFERENCED VALUE override IDeviceGroupManager
@Override
public DeviceGroupBean getReferencedByParent(DeviceGroupBean bean)
{
if(null == bean){
return null;
}
bean.setReferencedByParent(instanceOfDeviceGroupManager().loadByPrimaryKey(bean.getParent()));
return bean.getReferencedByParent();
}
//5.2 SET REFERENCED override IDeviceGroupManager
@Override
public DeviceGroupBean setReferencedByParent(DeviceGroupBean bean, DeviceGroupBean beanToSet)
{
try{
net.gdface.facelog.dborm.device.FlDeviceGroupBean nativeBean = this.beanConverter.toRight(bean);
IBeanConverter foreignConverter = this.dbConverter.getDeviceGroupBeanConverter();
net.gdface.facelog.dborm.device.FlDeviceGroupBean foreignNativeBean = foreignConverter.toRight(beanToSet);
this.nativeManager.setReferencedByParent(nativeBean,foreignNativeBean);
this.beanConverter.fromRight(bean, nativeBean);
foreignConverter.fromRight(beanToSet,foreignNativeBean);
return beanToSet;
}
catch(DaoException e)
{
throw new RuntimeDaoException(e);
}
}
//////////////////////////////////////
// SQL 'WHERE' METHOD
//////////////////////////////////////
//11
@Override
public int deleteByWhere(String where)
{
try{
return this.nativeManager.deleteByWhere(where);
}
catch(DaoException e)
{
throw new RuntimeDaoException(e);
}
}
//_____________________________________________________________________
//
// SAVE
//_____________________________________________________________________
//13
@Override
protected DeviceGroupBean insert(DeviceGroupBean bean)
{
try{
return this.beanConverter.fromRight(bean,this.nativeManager.insert(this.beanConverter.toRight(bean)));
}
catch(DaoException e)
{
throw new RuntimeDaoException(e);
}
}
//14
@Override
protected DeviceGroupBean update(DeviceGroupBean bean)
{
try{
return this.beanConverter.fromRight(bean,this.nativeManager.update(this.beanConverter.toRight(bean)));
}
catch(DaoException e)
{
throw new RuntimeDaoException(e);
}
}
//_____________________________________________________________________
//
// USING TEMPLATE
//_____________________________________________________________________
//18
@Override
public DeviceGroupBean loadUniqueUsingTemplate(DeviceGroupBean bean)
{
try{
return this.beanConverter.fromRight(this.nativeManager.loadUniqueUsingTemplate(this.beanConverter.toRight(bean)));
}
catch(DaoException e)
{
throw new RuntimeDaoException(e);
}
}
//18-1
@Override
public DeviceGroupBean loadUniqueUsingTemplateChecked(DeviceGroupBean bean) throws ObjectRetrievalException
{
try{
return this.beanConverter.fromRight(this.nativeManager.loadUniqueUsingTemplateChecked(this.beanConverter.toRight(bean)));
}
catch(net.gdface.facelog.dborm.exception.ObjectRetrievalException e)
{
throw new ObjectRetrievalException();
}
catch(DaoException e)
{
throw new RuntimeDaoException(e);
}
}
//20-5
@Override
public int loadUsingTemplate(DeviceGroupBean bean, int[] fieldList, int startRow, int numRows,int searchType, Action action)
{
try {
return this.nativeManager.loadUsingTemplate(this.beanConverter.toRight(bean),fieldList,startRow,numRows,searchType,this.toNative(action));
}
catch(DaoException e)
{
throw new RuntimeDaoException(e);
}
}
//21
@Override
public int deleteUsingTemplate(DeviceGroupBean bean)
{
try{
return this.nativeManager.deleteUsingTemplate(this.beanConverter.toRight(bean));
}
catch(DaoException e)
{
throw new RuntimeDaoException(e);
}
}
//_____________________________________________________________________
//
// USING INDICES
//_____________________________________________________________________
// override IDeviceGroupManager
@Override
public DeviceGroupBean[] loadByIndexParent(Integer parent)
{
return this.loadByIndexParentAsList(parent).toArray(new DeviceGroupBean[0]);
}
// override IDeviceGroupManager
@Override
public java.util.List loadByIndexParentAsList(Integer parent)
{
try{
return this.beanConverter.fromRight(this.nativeManager.loadByIndexParentAsList(parent));
}
catch(DaoException e)
{
throw new RuntimeDaoException(e);
}
}
// override IDeviceGroupManager
@Override
public int deleteByIndexParent(Integer parent)
{
try{
return this.nativeManager.deleteByIndexParent(parent);
}
catch(DaoException e)
{
throw new RuntimeDaoException(e);
}
}
/**
* Retrieves a list of DeviceGroupBean using the index specified by keyIndex.
* @param keyIndex valid values:
* {@link Constant#FL_DEVICE_GROUP_INDEX_PARENT}
* @param keys key values of index
* @return a list of DeviceGroupBean
*/
@Override
public java.util.List loadByIndexAsList(int keyIndex,Object ...keys)
{
try{
return this.beanConverter.fromRight(this.nativeManager.loadByIndexAsList(keyIndex,keys));
}catch(DaoException e){
throw new RuntimeDaoException(e);
}
}
/**
* Deletes rows using key.
* @param keyIndex valid values:
* {@link Constant#FL_DEVICE_GROUP_INDEX_PARENT}
* @param keys key values of index
* @return the number of deleted objects
*/
@Override
public int deleteByIndex(int keyIndex,Object ...keys)
{
try{
return this.nativeManager.deleteByIndex(keyIndex,keys);
}catch(DaoException e){
throw new RuntimeDaoException(e);
}
}
//_____________________________________________________________________
//
// MANY TO MANY: LOAD OTHER BEAN VIA JUNCTION TABLE
//_____________________________________________________________________
//22 MANY TO MANY override IDeviceGroupManager
@Override
public java.util.List loadViaPermitAsList(PersonGroupBean bean)
{
return this.loadViaPermitAsList(bean, 1, -1);
}
//23 MANY TO MANY override IDeviceGroupManager
@Override
public java.util.List loadViaPermitAsList(PersonGroupBean bean, int startRow, int numRows)
{
try{
return this.beanConverter.fromRight(
this.nativeManager.loadViaPermitAsList(
this.dbConverter.getPersonGroupBeanConverter().toRight(bean),
startRow,
numRows));
}catch(DaoException e){
throw new RuntimeDaoException(e);
}
}
//23.2 MANY TO MANY override IDeviceGroupManager
@Override
public void addJunction(DeviceGroupBean bean,PersonGroupBean linked){
if(null == bean || null == bean.getId()){
return ;
}
if(null == linked || null ==bean.getId()){
return ;
}
if(!instanceOfPermitManager().existsPrimaryKey(bean.getId(),linked.getId())){
PermitBean junction = new PermitBean();
junction.setDeviceGroupId(bean.getId());
junction.setPersonGroupId(linked.getId());
instanceOfPermitManager().save(junction);
}
}
//23.3 MANY TO MANY override IDeviceGroupManager
@Override
public int deleteJunction(DeviceGroupBean bean,PersonGroupBean linked){
if(null == bean || null == bean.getId()){
return 0;
}
if(null == linked || null ==bean.getId()){
return 0;
}
return instanceOfPermitManager().deleteByPrimaryKey(bean.getId(),linked.getId());
}
//23.4 MANY TO MANY override IDeviceGroupManager
@Override
public void addJunction(DeviceGroupBean bean,PersonGroupBean... linkedBeans){
if(null != linkedBeans){
for(PersonGroupBean linked:linkedBeans){
addJunction(bean,linked);
}
}
}
//23.5 MANY TO MANY override IDeviceGroupManager
@Override
public void addJunction(DeviceGroupBean bean,java.util.Collection linkedBeans){
if(null != linkedBeans){
for(PersonGroupBean linked:linkedBeans){
addJunction(bean,linked);
}
}
}
//23.6 MANY TO MANY override IDeviceGroupManager
@Override
public int deleteJunction(DeviceGroupBean bean,PersonGroupBean... linkedBeans){
int count = 0;
if(null != linkedBeans){
for(PersonGroupBean linked:linkedBeans){
count += deleteJunction(bean,linked);
}
}
return count;
}
//23.7 MANY TO MANY override IDeviceGroupManager
@Override
public int deleteJunction(DeviceGroupBean bean,java.util.Collection linkedBeans){
int count = 0;
if(null != linkedBeans){
for(PersonGroupBean linked:linkedBeans){
count += deleteJunction(bean,linked);
}
}
return count;
}
//_____________________________________________________________________
//
// COUNT
//_____________________________________________________________________
//25
@Override
public int countWhere(String where)
{
try{
return this.nativeManager.countWhere(where);
}
catch(DaoException e)
{
throw new RuntimeDaoException(e);
}
}
//20
@Override
public int countUsingTemplate(DeviceGroupBean bean, int searchType)
{
try{
return this.nativeManager.countUsingTemplate(this.beanConverter.toRight(bean),searchType);
}
catch(DaoException e)
{
throw new RuntimeDaoException(e);
}
}
//_____________________________________________________________________
//
// LISTENER
//_____________________________________________________________________
//35
/**
* @return {@link WrapListener} instance
*/
@Override
public TableListener registerListener(TableListener listener)
{
WrapListener wrapListener;
if(listener instanceof WrapListener){
wrapListener = (WrapListener)listener;
this.nativeManager.registerListener(wrapListener.nativeListener);
}else{
wrapListener = new WrapListener(listener);
this.nativeManager.registerListener(wrapListener.nativeListener);
}
return wrapListener;
}
//36
@Override
public void unregisterListener(TableListener listener)
{
if(!(listener instanceof WrapListener)){
throw new IllegalArgumentException("invalid listener type: " + WrapListener.class.getName() +" required");
}
this.nativeManager.unregisterListener(((WrapListener)listener).nativeListener);
}
//37
@Override
public void fire(TableListener.Event event, DeviceGroupBean bean){
fire(event.ordinal(), bean);
}
//37-1
@Override
public void fire(int event, DeviceGroupBean bean){
try{
this.nativeManager.fire(event, this.beanConverter.toRight(bean));
}
catch(DaoException e)
{
throw new RuntimeDaoException(e);
}
}
//37-2
/**
* bind foreign key listener to foreign table for DELETE RULE
*/
void bindForeignKeyListenerForDeleteRule(){
this.nativeManager.bindForeignKeyListenerForDeleteRule();
}
//37-3
/**
* unbind foreign key listener from all of foreign tables
* @see #bindForeignKeyListenerForDeleteRule()
*/
void unbindForeignKeyListenerForDeleteRule(){
this.nativeManager.unbindForeignKeyListenerForDeleteRule();
}
/**
* wrap {@code TableListener} as native listener
*/
public class WrapListener implements TableListener{
private final TableListener listener;
private final net.gdface.facelog.dborm.TableListener nativeListener;
private WrapListener(final TableListener listener) {
if(null == listener){
throw new NullPointerException();
}
this.listener = listener;
this.nativeListener = new net.gdface.facelog.dborm.TableListener (){
@Override
public void beforeInsert(net.gdface.facelog.dborm.device.FlDeviceGroupBean bean) throws DaoException {
listener.beforeInsert(DeviceGroupManager.this.beanConverter.fromRight(bean));
}
@Override
public void afterInsert(net.gdface.facelog.dborm.device.FlDeviceGroupBean bean) throws DaoException {
listener.afterInsert(DeviceGroupManager.this.beanConverter.fromRight(bean));
}
@Override
public void beforeUpdate(net.gdface.facelog.dborm.device.FlDeviceGroupBean bean) throws DaoException {
listener.beforeUpdate(DeviceGroupManager.this.beanConverter.fromRight(bean));
}
@Override
public void afterUpdate(net.gdface.facelog.dborm.device.FlDeviceGroupBean bean) throws DaoException {
listener.afterUpdate(DeviceGroupManager.this.beanConverter.fromRight(bean));
}
@Override
public void beforeDelete(net.gdface.facelog.dborm.device.FlDeviceGroupBean bean) throws DaoException {
listener.beforeDelete(DeviceGroupManager.this.beanConverter.fromRight(bean));
}
@Override
public void afterDelete(net.gdface.facelog.dborm.device.FlDeviceGroupBean bean) throws DaoException {
listener.afterDelete(DeviceGroupManager.this.beanConverter.fromRight(bean));
}
@Override
public void done() throws DaoException {
listener.done();
}};
}
@Override
public void beforeInsert(DeviceGroupBean bean) {
listener.beforeInsert(bean);
}
@Override
public void afterInsert(DeviceGroupBean bean) {
listener.afterInsert(bean);
}
@Override
public void beforeUpdate(DeviceGroupBean bean) {
listener.beforeUpdate(bean);
}
@Override
public void afterUpdate(DeviceGroupBean bean) {
listener.afterUpdate(bean);
}
@Override
public void beforeDelete(DeviceGroupBean bean) {
listener.beforeDelete(bean);
}
@Override
public void afterDelete(DeviceGroupBean bean) {
listener.afterDelete(bean);
}
@Override
public void done() {
listener.done();
}
}
//_____________________________________________________________________
//
// UTILS
//_____________________________________________________________________
//43
@Override
public boolean isPrimaryKey(String column){
return this.nativeManager.isPrimaryKey(column);
}
@Override
public int loadBySqlForAction(String sql, Object[] argList, int[] fieldList,int startRow, int numRows,Action action){
try{
return this.nativeManager.loadBySqlForAction(sql,argList,fieldList,startRow,numRows,this.toNative(action));
}
catch(DaoException e)
{
throw new RuntimeDaoException(e);
}
}
@Override
public T runAsTransaction(Callable fun) {
try{
return this.nativeManager.runAsTransaction(fun);
}
catch(DaoException e)
{
throw new RuntimeDaoException(e);
}
}
@Override
public void runAsTransaction(Runnable fun) {
try{
this.nativeManager.runAsTransaction(fun);
}
catch(DaoException e)
{
throw new RuntimeDaoException(e);
}
}
private net.gdface.facelog.dborm.TableManager.Action toNative(final Action action){
if(null == action){
throw new NullPointerException();
}
return new net.gdface.facelog.dborm.TableManager.Action(){
@Override
public void call(net.gdface.facelog.dborm.device.FlDeviceGroupBean bean) {
action.call(DeviceGroupManager.this.beanConverter.fromRight(bean));
}
@Override
public net.gdface.facelog.dborm.device.FlDeviceGroupBean getBean() {
return DeviceGroupManager.this.beanConverter.toRight(action.getBean());
}};
}
//45 override IDeviceGroupManager
@Override
public java.util.List toPrimaryKeyList(DeviceGroupBean... beans){
if(null == beans){
return new java.util.ArrayList();
}
java.util.ArrayList list = new java.util.ArrayList(beans.length);
for(DeviceGroupBean bean:beans){
list.add(null == bean ? null : bean.getId());
}
return list;
}
//46 override IDeviceGroupManager
@Override
public java.util.List toPrimaryKeyList(java.util.Collection beans){
if(null == beans){
return new java.util.ArrayList();
}
java.util.ArrayList list = new java.util.ArrayList(beans.size());
for(DeviceGroupBean bean:beans){
list.add(null == bean ? null : bean.getId());
}
return list;
}
//_____________________________________________________________________
//
// SELF-REFERENCE
//_____________________________________________________________________
//47 IDeviceGroupManager
@Override
public java.util.List listOfParent(Integer id){
java.util.List list = new java.util.ArrayList();
for(DeviceGroupBean parent = loadByPrimaryKey(id)
; null != parent
; parent = loadByPrimaryKey(parent.getParent())){
list.add(parent);
if( (parent.getId().equals(parent.getParent()))
|| (list.size() > 1 && parent.getId().equals(id))){
// cycle reference
break;
}
}
java.util.Collections.reverse(list);
return list;
}
//48 IDeviceGroupManager
@Override
public java.util.List listOfParent(DeviceGroupBean bean){
return null == bean
? java.util.Collections.emptyList()
: listOfParent(bean.getId());
}
//49 IDeviceGroupManager
@Override
public int levelOfParent(Integer id){
int count = 0 ;
for(DeviceGroupBean parent = loadByPrimaryKey(id)
; null != parent
; ++count,parent = loadByPrimaryKey(parent.getParent())){
if( (parent.getId().equals(parent.getParent()))
|| (count > 0 && parent.getId().equals(id))){
// cycle reference
return -1;
}
}
return count;
}
//50 IDeviceGroupManager
@Override
public int levelOfParent(DeviceGroupBean bean){
return null == bean
? 0
: levelOfParent(bean.getId());
}
//51 IDeviceGroupManager
@Override
public boolean isCycleOnParent(Integer id){
return levelOfParent(id) < 0;
}
//52 IDeviceGroupManager
@Override
public boolean isCycleOnParent(DeviceGroupBean bean){
return levelOfParent(bean) < 0;
}
//53 IDeviceGroupManager
@Override
public DeviceGroupBean topOfParent(Integer id){
if(null == id){
throw new NullPointerException();
}
DeviceGroupBean parent = loadByPrimaryKey(id);
int count = 0 ;
for(;null != parent && null != parent.getParent();){
if( (parent.getId().equals(parent.getParent()))
|| (++count > 1 && parent.getId().equals(id))){
// cycle reference
throw new IllegalStateException("cycle on field: " + "parent");
}
parent = loadByPrimaryKeyChecked(parent.getParent());
}
return parent;
}
//54 IDeviceGroupManager
@Override
public DeviceGroupBean topOfParent(DeviceGroupBean bean){
if(null == bean){
throw new NullPointerException();
}
return topOfParent(bean.getId());
}
//55 IDeviceGroupManager
@Override
public Integer checkCycleOfParent(Integer id){
if(isCycleOnParent(id)){
throw new IllegalStateException("cycle on field: " + "parent");
}
return id;
}
//56 IDeviceGroupManager
@Override
public DeviceGroupBean checkCycleOfParent(DeviceGroupBean bean){
if(isCycleOnParent(bean)){
throw new IllegalStateException("cycle on field: " + "parent");
}
return bean;
}
//57 IDeviceGroupManager
@Override
public java.util.List childListByParent(Integer id){
java.util.LinkedHashSet set = new java.util.LinkedHashSet();
return new java.util.ArrayList(doListOfChild(id,set));
}
//58 IDeviceGroupManager
@Override
public java.util.List childListByParent(DeviceGroupBean bean){
return null == bean
? java.util.Collections.emptyList()
: childListByParent(bean.getId());
}
/**
* get all of child recursively
* @param id PK# 1
* @param set child set for output
*/
private java.util.LinkedHashSet doListOfChild(Integer id, java.util.LinkedHashSet set){
DeviceGroupBean bean = loadByPrimaryKey(id);
if(bean != null){
if(set.contains(bean)){
throw new IllegalStateException("cycle on field: " + "parent");
}
set.add(bean);
DeviceGroupBean tmpl = DeviceGroupBean.builder().parent(bean.getId()).build();
java.util.List childs = loadUsingTemplateAsList(tmpl);
for(DeviceGroupBean c:childs){
doListOfChild(c.getId(),set);
}
}
return set;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy