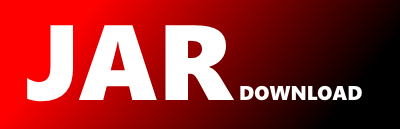
net.gdface.facelog.dborm.device.FlDeviceBean Maven / Gradle / Ivy
// ______________________________________________________
// Generated by sql2java - https://github.com/10km/sql2java-2-6-7 (custom branch)
// modified by guyadong from
// sql2java original version https://sourceforge.net/projects/sql2java/
// JDBC driver used at code generation time: com.mysql.jdbc.Driver
// template: bean.java.vm
// ______________________________________________________
package net.gdface.facelog.dborm.device;
import java.io.Serializable;
import java.util.List;
import java.util.Objects;
import net.gdface.facelog.dborm.Constant;
import net.gdface.facelog.dborm.BaseBean;
import net.gdface.facelog.dborm.CompareToBuilder;
import net.gdface.facelog.dborm.EqualsBuilder;
import net.gdface.facelog.dborm.HashCodeBuilder;
/**
* FlDeviceBean is a mapping of fl_device Table.
*
Meta Data Information (in progress):
*
* - comments: 前端设备基本信息
*
* @author guyadong
*/
public class FlDeviceBean
implements Serializable,BaseBean,Comparable,Constant,Cloneable
{
private static final long serialVersionUID = -1873511050244238973L;
/** NULL {@link FlDeviceBean} bean , IMMUTABLE instance */
public static final FlDeviceBean NULL = new FlDeviceBean().asNULL().asImmutable();
/** comments:设备id */
private Integer id;
/** comments:所属设备组id */
private Integer groupId;
/** comments:设备名称 */
private String name;
/** comments:产品名称 */
private String productName;
/** comments:设备型号 */
private String model;
/** comments:设备供应商 */
private String vendor;
/** comments:设备制造商 */
private String manufacturer;
/** comments:设备生产日期 */
private java.util.Date madeDate;
/** comments:设备版本号 */
private String version;
/** comments:(特征码)算法版本号,用于区分不同人脸识别算法生成的特征数据(允许字母,数字,-,.,_符号) */
private String sdkVersion;
/** comments:设备序列号 */
private String serialNo;
/** comments:6字节MAC地址(HEX) */
private String mac;
/** comments:备注 */
private String remark;
/** comments:应用项目自定义二进制扩展字段(最大64KB) */
private java.nio.ByteBuffer extBin;
/** comments:应用项目自定义文本扩展字段(最大64KB) */
private String extTxt;
private java.util.Date createTime;
private java.util.Date updateTime;
/** flag whether {@code this} can be modified */
private Boolean immutable;
/** columns modified flag */
private int modified;
/** columns initialized flag */
private int initialized;
/** new record flag */
private boolean isNew;
/**
* set immutable status
* @return {@code this}
*/
private FlDeviceBean immutable(Boolean immutable) {
this.immutable = immutable;
return this;
}
/**
* set {@code this} as immutable object
* @return {@code this}
*/
public FlDeviceBean asImmutable() {
return immutable(Boolean.TRUE);
}
/**
* @return {@code true} if {@code this} is a mutable object
*/
public boolean mutable(){
return !Boolean.TRUE.equals(this.immutable);
}
/**
* @return {@code this}
* @throws IllegalStateException if {@code this} is a immutable object
*/
private FlDeviceBean checkMutable(){
if(!mutable()){
throw new IllegalStateException("this is a immutable object");
}
return this;
}
/**
* @return return a new mutable copy of this object.
*/
public FlDeviceBean cloneMutable(){
return clone().immutable(null);
}
@Override
public boolean isNew()
{
return this.isNew;
}
@Override
public void isNew(boolean isNew)
{
this.isNew = isNew;
}
/**
* Specifies to the object if it has been set as new.
*
* @param isNew the boolean value to be assigned to the isNew field
*/
public void setNew(boolean isNew)
{
this.isNew = isNew;
}
/**
* @return the modified status of columns
*/
public int getModified(){
return modified;
}
/**
* @param modified the modified status bit to be assigned to {@link #modified}
*/
public void setModified(int modified){
this.modified = modified;
}
/**
* @return the initialized status of columns
*/
public int getInitialized(){
return initialized;
}
/**
* @param initialized the initialized status bit to be assigned to {@link #initialized}
*/
public void setInitialized(int initialized){
this.initialized = initialized;
}
protected static final >boolean equals(T a, T b) {
return a == b || (a != null && 0==a.compareTo(b));
}
public FlDeviceBean(){
super();
reset();
}
/**
* construct a new instance filled with primary keys
* @param id PK# 1
*/
public FlDeviceBean(Integer id){
this();
setId(id);
}
/**
* Getter method for {@link #id}.
* PRIMARY KEY.
* Meta Data Information (in progress):
*
* - full name: fl_device.id
* - imported key: fl_log.device_id
* - imported key: fl_image.device_id
* - comments: 设备id
* - AUTO_INCREMENT
* - NOT NULL
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of id
*/
public Integer getId(){
return id;
}
/**
* Setter method for {@link #id}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to id
*/
public void setId(Integer newVal)
{
checkMutable();
modified |= FL_DEVICE_ID_ID_MASK;
initialized |= FL_DEVICE_ID_ID_MASK;
if (Objects.equals(newVal, id)) {
return;
}
id = newVal;
}
/**
* Determines if the id has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkIdModified()
{
return 0L != (modified & FL_DEVICE_ID_ID_MASK);
}
/**
* Determines if the id has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkIdInitialized()
{
return 0L != (initialized & FL_DEVICE_ID_ID_MASK);
}
/**
* Getter method for {@link #groupId}.
* Meta Data Information (in progress):
*
* - full name: fl_device.group_id
* - foreign key: fl_device_group.id
* - comments: 所属设备组id
* - default value: '1'
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of groupId
*/
public Integer getGroupId(){
return groupId;
}
/**
* Setter method for {@link #groupId}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to groupId
*/
public void setGroupId(Integer newVal)
{
checkMutable();
modified |= FL_DEVICE_ID_GROUP_ID_MASK;
initialized |= FL_DEVICE_ID_GROUP_ID_MASK;
if (Objects.equals(newVal, groupId)) {
return;
}
groupId = newVal;
}
/**
* Determines if the groupId has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkGroupIdModified()
{
return 0L != (modified & FL_DEVICE_ID_GROUP_ID_MASK);
}
/**
* Determines if the groupId has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkGroupIdInitialized()
{
return 0L != (initialized & FL_DEVICE_ID_GROUP_ID_MASK);
}
/**
* Getter method for {@link #name}.
* Meta Data Information (in progress):
*
* - full name: fl_device.name
* - comments: 设备名称
* - column size: 32
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of name
*/
public String getName(){
return name;
}
/**
* Setter method for {@link #name}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to name
*/
public void setName(String newVal)
{
checkMutable();
modified |= FL_DEVICE_ID_NAME_MASK;
initialized |= FL_DEVICE_ID_NAME_MASK;
if (Objects.equals(newVal, name)) {
return;
}
name = newVal;
}
/**
* Determines if the name has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkNameModified()
{
return 0L != (modified & FL_DEVICE_ID_NAME_MASK);
}
/**
* Determines if the name has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkNameInitialized()
{
return 0L != (initialized & FL_DEVICE_ID_NAME_MASK);
}
/**
* Getter method for {@link #productName}.
* Meta Data Information (in progress):
*
* - full name: fl_device.product_name
* - comments: 产品名称
* - column size: 32
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of productName
*/
public String getProductName(){
return productName;
}
/**
* Setter method for {@link #productName}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to productName
*/
public void setProductName(String newVal)
{
checkMutable();
modified |= FL_DEVICE_ID_PRODUCT_NAME_MASK;
initialized |= FL_DEVICE_ID_PRODUCT_NAME_MASK;
if (Objects.equals(newVal, productName)) {
return;
}
productName = newVal;
}
/**
* Determines if the productName has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkProductNameModified()
{
return 0L != (modified & FL_DEVICE_ID_PRODUCT_NAME_MASK);
}
/**
* Determines if the productName has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkProductNameInitialized()
{
return 0L != (initialized & FL_DEVICE_ID_PRODUCT_NAME_MASK);
}
/**
* Getter method for {@link #model}.
* Meta Data Information (in progress):
*
* - full name: fl_device.model
* - comments: 设备型号
* - column size: 32
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of model
*/
public String getModel(){
return model;
}
/**
* Setter method for {@link #model}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to model
*/
public void setModel(String newVal)
{
checkMutable();
modified |= FL_DEVICE_ID_MODEL_MASK;
initialized |= FL_DEVICE_ID_MODEL_MASK;
if (Objects.equals(newVal, model)) {
return;
}
model = newVal;
}
/**
* Determines if the model has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkModelModified()
{
return 0L != (modified & FL_DEVICE_ID_MODEL_MASK);
}
/**
* Determines if the model has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkModelInitialized()
{
return 0L != (initialized & FL_DEVICE_ID_MODEL_MASK);
}
/**
* Getter method for {@link #vendor}.
* Meta Data Information (in progress):
*
* - full name: fl_device.vendor
* - comments: 设备供应商
* - column size: 32
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of vendor
*/
public String getVendor(){
return vendor;
}
/**
* Setter method for {@link #vendor}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to vendor
*/
public void setVendor(String newVal)
{
checkMutable();
modified |= FL_DEVICE_ID_VENDOR_MASK;
initialized |= FL_DEVICE_ID_VENDOR_MASK;
if (Objects.equals(newVal, vendor)) {
return;
}
vendor = newVal;
}
/**
* Determines if the vendor has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkVendorModified()
{
return 0L != (modified & FL_DEVICE_ID_VENDOR_MASK);
}
/**
* Determines if the vendor has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkVendorInitialized()
{
return 0L != (initialized & FL_DEVICE_ID_VENDOR_MASK);
}
/**
* Getter method for {@link #manufacturer}.
* Meta Data Information (in progress):
*
* - full name: fl_device.manufacturer
* - comments: 设备制造商
* - column size: 32
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of manufacturer
*/
public String getManufacturer(){
return manufacturer;
}
/**
* Setter method for {@link #manufacturer}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to manufacturer
*/
public void setManufacturer(String newVal)
{
checkMutable();
modified |= FL_DEVICE_ID_MANUFACTURER_MASK;
initialized |= FL_DEVICE_ID_MANUFACTURER_MASK;
if (Objects.equals(newVal, manufacturer)) {
return;
}
manufacturer = newVal;
}
/**
* Determines if the manufacturer has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkManufacturerModified()
{
return 0L != (modified & FL_DEVICE_ID_MANUFACTURER_MASK);
}
/**
* Determines if the manufacturer has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkManufacturerInitialized()
{
return 0L != (initialized & FL_DEVICE_ID_MANUFACTURER_MASK);
}
/**
* Getter method for {@link #madeDate}.
* Meta Data Information (in progress):
*
* - full name: fl_device.made_date
* - comments: 设备生产日期
* - column size: 10
* - JDBC type returned by the driver: Types.DATE
*
*
* @return the value of madeDate
*/
public java.util.Date getMadeDate(){
return madeDate;
}
/**
* Setter method for {@link #madeDate}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to madeDate
*/
public void setMadeDate(java.util.Date newVal)
{
checkMutable();
modified |= FL_DEVICE_ID_MADE_DATE_MASK;
initialized |= FL_DEVICE_ID_MADE_DATE_MASK;
if (Objects.equals(newVal, madeDate)) {
return;
}
madeDate = newVal;
}
/**
* Setter method for {@link #madeDate}.
* @param newVal the number of milliseconds since January 1, 1970, 00:00:00 GMT represented by this Date object.
*/
public void setMadeDate(Long newVal)
{
setMadeDate(null == newVal ? null : new java.util.Date(newVal));
}
/**
* Determines if the madeDate has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkMadeDateModified()
{
return 0L != (modified & FL_DEVICE_ID_MADE_DATE_MASK);
}
/**
* Determines if the madeDate has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkMadeDateInitialized()
{
return 0L != (initialized & FL_DEVICE_ID_MADE_DATE_MASK);
}
/**
* Getter method for {@link #version}.
* Meta Data Information (in progress):
*
* - full name: fl_device.version
* - comments: 设备版本号
* - column size: 32
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of version
*/
public String getVersion(){
return version;
}
/**
* Setter method for {@link #version}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to version
*/
public void setVersion(String newVal)
{
checkMutable();
modified |= FL_DEVICE_ID_VERSION_MASK;
initialized |= FL_DEVICE_ID_VERSION_MASK;
if (Objects.equals(newVal, version)) {
return;
}
version = newVal;
}
/**
* Determines if the version has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkVersionModified()
{
return 0L != (modified & FL_DEVICE_ID_VERSION_MASK);
}
/**
* Determines if the version has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkVersionInitialized()
{
return 0L != (initialized & FL_DEVICE_ID_VERSION_MASK);
}
/**
* Getter method for {@link #sdkVersion}.
* Meta Data Information (in progress):
*
* - full name: fl_device.sdk_version
* - comments: (特征码)算法版本号,用于区分不同人脸识别算法生成的特征数据(允许字母,数字,-,.,_符号)
* - NOT NULL
* - column size: 32
* - JDBC type returned by the driver: Types.CHAR
*
*
* @return the value of sdkVersion
*/
public String getSdkVersion(){
return sdkVersion;
}
/**
* Setter method for {@link #sdkVersion}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to sdkVersion
*/
public void setSdkVersion(String newVal)
{
checkMutable();
modified |= FL_DEVICE_ID_SDK_VERSION_MASK;
initialized |= FL_DEVICE_ID_SDK_VERSION_MASK;
if (Objects.equals(newVal, sdkVersion)) {
return;
}
sdkVersion = newVal;
}
/**
* Determines if the sdkVersion has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkSdkVersionModified()
{
return 0L != (modified & FL_DEVICE_ID_SDK_VERSION_MASK);
}
/**
* Determines if the sdkVersion has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkSdkVersionInitialized()
{
return 0L != (initialized & FL_DEVICE_ID_SDK_VERSION_MASK);
}
/**
* Getter method for {@link #serialNo}.
* Meta Data Information (in progress):
*
* - full name: fl_device.serial_no
* - comments: 设备序列号
* - column size: 32
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of serialNo
*/
public String getSerialNo(){
return serialNo;
}
/**
* Setter method for {@link #serialNo}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to serialNo
*/
public void setSerialNo(String newVal)
{
checkMutable();
modified |= FL_DEVICE_ID_SERIAL_NO_MASK;
initialized |= FL_DEVICE_ID_SERIAL_NO_MASK;
if (Objects.equals(newVal, serialNo)) {
return;
}
serialNo = newVal;
}
/**
* Determines if the serialNo has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkSerialNoModified()
{
return 0L != (modified & FL_DEVICE_ID_SERIAL_NO_MASK);
}
/**
* Determines if the serialNo has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkSerialNoInitialized()
{
return 0L != (initialized & FL_DEVICE_ID_SERIAL_NO_MASK);
}
/**
* Getter method for {@link #mac}.
* Meta Data Information (in progress):
*
* - full name: fl_device.mac
* - comments: 6字节MAC地址(HEX)
* - column size: 12
* - JDBC type returned by the driver: Types.CHAR
*
*
* @return the value of mac
*/
public String getMac(){
return mac;
}
/**
* Setter method for {@link #mac}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to mac
*/
public void setMac(String newVal)
{
checkMutable();
modified |= FL_DEVICE_ID_MAC_MASK;
initialized |= FL_DEVICE_ID_MAC_MASK;
if (Objects.equals(newVal, mac)) {
return;
}
mac = newVal;
}
/**
* Determines if the mac has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkMacModified()
{
return 0L != (modified & FL_DEVICE_ID_MAC_MASK);
}
/**
* Determines if the mac has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkMacInitialized()
{
return 0L != (initialized & FL_DEVICE_ID_MAC_MASK);
}
/**
* Getter method for {@link #remark}.
* Meta Data Information (in progress):
*
* - full name: fl_device.remark
* - comments: 备注
* - column size: 256
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of remark
*/
public String getRemark(){
return remark;
}
/**
* Setter method for {@link #remark}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to remark
*/
public void setRemark(String newVal)
{
checkMutable();
modified |= FL_DEVICE_ID_REMARK_MASK;
initialized |= FL_DEVICE_ID_REMARK_MASK;
if (Objects.equals(newVal, remark)) {
return;
}
remark = newVal;
}
/**
* Determines if the remark has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkRemarkModified()
{
return 0L != (modified & FL_DEVICE_ID_REMARK_MASK);
}
/**
* Determines if the remark has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkRemarkInitialized()
{
return 0L != (initialized & FL_DEVICE_ID_REMARK_MASK);
}
/**
* Getter method for {@link #extBin}.
* Meta Data Information (in progress):
*
* - full name: fl_device.ext_bin
* - comments: 应用项目自定义二进制扩展字段(最大64KB)
* - column size: 65535
* - JDBC type returned by the driver: Types.LONGVARBINARY
*
*
* @return the value of extBin
*/
public java.nio.ByteBuffer getExtBin(){
return extBin;
}
/**
* Setter method for {@link #extBin}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to extBin
*/
public void setExtBin(java.nio.ByteBuffer newVal)
{
checkMutable();
modified |= FL_DEVICE_ID_EXT_BIN_MASK;
initialized |= FL_DEVICE_ID_EXT_BIN_MASK;
if (Objects.equals(newVal, extBin)) {
return;
}
extBin = newVal;
}
/**
* Determines if the extBin has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkExtBinModified()
{
return 0L != (modified & FL_DEVICE_ID_EXT_BIN_MASK);
}
/**
* Determines if the extBin has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkExtBinInitialized()
{
return 0L != (initialized & FL_DEVICE_ID_EXT_BIN_MASK);
}
/**
* Getter method for {@link #extTxt}.
* Meta Data Information (in progress):
*
* - full name: fl_device.ext_txt
* - comments: 应用项目自定义文本扩展字段(最大64KB)
* - column size: 65535
* - JDBC type returned by the driver: Types.LONGVARCHAR
*
*
* @return the value of extTxt
*/
public String getExtTxt(){
return extTxt;
}
/**
* Setter method for {@link #extTxt}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to extTxt
*/
public void setExtTxt(String newVal)
{
checkMutable();
modified |= FL_DEVICE_ID_EXT_TXT_MASK;
initialized |= FL_DEVICE_ID_EXT_TXT_MASK;
if (Objects.equals(newVal, extTxt)) {
return;
}
extTxt = newVal;
}
/**
* Determines if the extTxt has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkExtTxtModified()
{
return 0L != (modified & FL_DEVICE_ID_EXT_TXT_MASK);
}
/**
* Determines if the extTxt has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkExtTxtInitialized()
{
return 0L != (initialized & FL_DEVICE_ID_EXT_TXT_MASK);
}
/**
* Getter method for {@link #createTime}.
* Meta Data Information (in progress):
*
* - full name: fl_device.create_time
* - default value: 'CURRENT_TIMESTAMP'
* - NOT NULL
* - column size: 19
* - JDBC type returned by the driver: Types.TIMESTAMP
*
*
* @return the value of createTime
*/
public java.util.Date getCreateTime(){
return createTime;
}
/**
* Setter method for {@link #createTime}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to createTime
*/
public void setCreateTime(java.util.Date newVal)
{
checkMutable();
modified |= FL_DEVICE_ID_CREATE_TIME_MASK;
initialized |= FL_DEVICE_ID_CREATE_TIME_MASK;
if (Objects.equals(newVal, createTime)) {
return;
}
createTime = newVal;
}
/**
* Setter method for {@link #createTime}.
* @param newVal the number of milliseconds since January 1, 1970, 00:00:00 GMT represented by this Date object.
*/
public void setCreateTime(Long newVal)
{
setCreateTime(null == newVal ? null : new java.util.Date(newVal));
}
/**
* Determines if the createTime has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkCreateTimeModified()
{
return 0L != (modified & FL_DEVICE_ID_CREATE_TIME_MASK);
}
/**
* Determines if the createTime has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkCreateTimeInitialized()
{
return 0L != (initialized & FL_DEVICE_ID_CREATE_TIME_MASK);
}
/**
* Getter method for {@link #updateTime}.
* Meta Data Information (in progress):
*
* - full name: fl_device.update_time
* - default value: 'CURRENT_TIMESTAMP'
* - NOT NULL
* - column size: 19
* - JDBC type returned by the driver: Types.TIMESTAMP
*
*
* @return the value of updateTime
*/
public java.util.Date getUpdateTime(){
return updateTime;
}
/**
* Setter method for {@link #updateTime}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to updateTime
*/
public void setUpdateTime(java.util.Date newVal)
{
checkMutable();
modified |= FL_DEVICE_ID_UPDATE_TIME_MASK;
initialized |= FL_DEVICE_ID_UPDATE_TIME_MASK;
if (Objects.equals(newVal, updateTime)) {
return;
}
updateTime = newVal;
}
/**
* Setter method for {@link #updateTime}.
* @param newVal the number of milliseconds since January 1, 1970, 00:00:00 GMT represented by this Date object.
*/
public void setUpdateTime(Long newVal)
{
setUpdateTime(null == newVal ? null : new java.util.Date(newVal));
}
/**
* Determines if the updateTime has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkUpdateTimeModified()
{
return 0L != (modified & FL_DEVICE_ID_UPDATE_TIME_MASK);
}
/**
* Determines if the updateTime has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkUpdateTimeInitialized()
{
return 0L != (initialized & FL_DEVICE_ID_UPDATE_TIME_MASK);
}
//////////////////////////////////////
// referenced bean for FOREIGN KEYS
//////////////////////////////////////
/**
* The referenced {@link FlDeviceGroupBean} by {@link #groupId} .
* FOREIGN KEY (group_id) REFERENCES fl_device_group(id)
*/
private FlDeviceGroupBean referencedByGroupId;
/**
* Getter method for {@link #referencedByGroupId}.
* @return FlDeviceGroupBean
*/
public FlDeviceGroupBean getReferencedByGroupId() {
return this.referencedByGroupId;
}
/**
* Setter method for {@link #referencedByGroupId}.
* @param reference FlDeviceGroupBean
*/
public void setReferencedByGroupId(FlDeviceGroupBean reference) {
this.referencedByGroupId = reference;
}
@Override
public boolean isModified()
{
return 0 != modified;
}
@Override
public boolean isModified(int columnID){
switch ( columnID ){
case FL_DEVICE_ID_ID:
return checkIdModified();
case FL_DEVICE_ID_GROUP_ID:
return checkGroupIdModified();
case FL_DEVICE_ID_NAME:
return checkNameModified();
case FL_DEVICE_ID_PRODUCT_NAME:
return checkProductNameModified();
case FL_DEVICE_ID_MODEL:
return checkModelModified();
case FL_DEVICE_ID_VENDOR:
return checkVendorModified();
case FL_DEVICE_ID_MANUFACTURER:
return checkManufacturerModified();
case FL_DEVICE_ID_MADE_DATE:
return checkMadeDateModified();
case FL_DEVICE_ID_VERSION:
return checkVersionModified();
case FL_DEVICE_ID_SDK_VERSION:
return checkSdkVersionModified();
case FL_DEVICE_ID_SERIAL_NO:
return checkSerialNoModified();
case FL_DEVICE_ID_MAC:
return checkMacModified();
case FL_DEVICE_ID_REMARK:
return checkRemarkModified();
case FL_DEVICE_ID_EXT_BIN:
return checkExtBinModified();
case FL_DEVICE_ID_EXT_TXT:
return checkExtTxtModified();
case FL_DEVICE_ID_CREATE_TIME:
return checkCreateTimeModified();
case FL_DEVICE_ID_UPDATE_TIME:
return checkUpdateTimeModified();
default:
return false;
}
}
@Override
public boolean isInitialized(int columnID){
switch(columnID) {
case FL_DEVICE_ID_ID:
return checkIdInitialized();
case FL_DEVICE_ID_GROUP_ID:
return checkGroupIdInitialized();
case FL_DEVICE_ID_NAME:
return checkNameInitialized();
case FL_DEVICE_ID_PRODUCT_NAME:
return checkProductNameInitialized();
case FL_DEVICE_ID_MODEL:
return checkModelInitialized();
case FL_DEVICE_ID_VENDOR:
return checkVendorInitialized();
case FL_DEVICE_ID_MANUFACTURER:
return checkManufacturerInitialized();
case FL_DEVICE_ID_MADE_DATE:
return checkMadeDateInitialized();
case FL_DEVICE_ID_VERSION:
return checkVersionInitialized();
case FL_DEVICE_ID_SDK_VERSION:
return checkSdkVersionInitialized();
case FL_DEVICE_ID_SERIAL_NO:
return checkSerialNoInitialized();
case FL_DEVICE_ID_MAC:
return checkMacInitialized();
case FL_DEVICE_ID_REMARK:
return checkRemarkInitialized();
case FL_DEVICE_ID_EXT_BIN:
return checkExtBinInitialized();
case FL_DEVICE_ID_EXT_TXT:
return checkExtTxtInitialized();
case FL_DEVICE_ID_CREATE_TIME:
return checkCreateTimeInitialized();
case FL_DEVICE_ID_UPDATE_TIME:
return checkUpdateTimeInitialized();
default:
return false;
}
}
@Override
public boolean isModified(String column){
return isModified(columnIDOf(column));
}
@Override
public boolean isInitialized(String column){
return isInitialized(columnIDOf(column));
}
@Override
public void resetIsModified()
{
checkMutable();
modified = 0;
}
@Override
public void resetPrimaryKeysModified()
{
modified &= (~(FL_DEVICE_ID_ID_MASK));
}
/**
* Resets columns modification status except primary keys to 'not modified'.
*/
public void resetModifiedExceptPrimaryKeys()
{
modified &= (~(FL_DEVICE_ID_GROUP_ID_MASK |
FL_DEVICE_ID_NAME_MASK |
FL_DEVICE_ID_PRODUCT_NAME_MASK |
FL_DEVICE_ID_MODEL_MASK |
FL_DEVICE_ID_VENDOR_MASK |
FL_DEVICE_ID_MANUFACTURER_MASK |
FL_DEVICE_ID_MADE_DATE_MASK |
FL_DEVICE_ID_VERSION_MASK |
FL_DEVICE_ID_SDK_VERSION_MASK |
FL_DEVICE_ID_SERIAL_NO_MASK |
FL_DEVICE_ID_MAC_MASK |
FL_DEVICE_ID_REMARK_MASK |
FL_DEVICE_ID_EXT_BIN_MASK |
FL_DEVICE_ID_EXT_TXT_MASK |
FL_DEVICE_ID_CREATE_TIME_MASK |
FL_DEVICE_ID_UPDATE_TIME_MASK));
}
/**
* Resets the object initialization status to 'not initialized'.
*/
private void resetInitialized()
{
initialized = 0;
}
/** reset all fields to initial value, equal to a new bean */
public void reset(){
checkMutable();
this.id = null;
/* DEFAULT:'1'*/
this.groupId = new Integer(1);
this.name = null;
this.productName = null;
this.model = null;
this.vendor = null;
this.manufacturer = null;
this.madeDate = null;
this.version = null;
this.sdkVersion = null;
this.serialNo = null;
this.mac = null;
this.remark = null;
this.extBin = null;
this.extTxt = null;
/* DEFAULT:'CURRENT_TIMESTAMP'*/
this.createTime = null;
/* DEFAULT:'CURRENT_TIMESTAMP'*/
this.updateTime = null;
this.isNew = true;
this.modified = 0;
this.initialized = (FL_DEVICE_ID_GROUP_ID_MASK);
}
@Override
public boolean equals(Object object)
{
if (!(object instanceof FlDeviceBean)) {
return false;
}
FlDeviceBean obj = (FlDeviceBean) object;
return new EqualsBuilder()
.append(getId(), obj.getId())
.append(getGroupId(), obj.getGroupId())
.append(getName(), obj.getName())
.append(getProductName(), obj.getProductName())
.append(getModel(), obj.getModel())
.append(getVendor(), obj.getVendor())
.append(getManufacturer(), obj.getManufacturer())
.append(getMadeDate(), obj.getMadeDate())
.append(getVersion(), obj.getVersion())
.append(getSdkVersion(), obj.getSdkVersion())
.append(getSerialNo(), obj.getSerialNo())
.append(getMac(), obj.getMac())
.append(getRemark(), obj.getRemark())
.append(getExtBin(), obj.getExtBin())
.append(getExtTxt(), obj.getExtTxt())
.append(getCreateTime(), obj.getCreateTime())
.append(getUpdateTime(), obj.getUpdateTime())
.isEquals();
}
@Override
public int hashCode()
{
return new HashCodeBuilder(-82280557, -700257973)
.append(getId())
.toHashCode();
}
@Override
public String toString() {
return toString(true,false);
}
/**
* cast byte array to HEX string
*
* @param input
* @return {@code null} if {@code input} is null
*/
private static final String toHex(byte[] input) {
if (null == input){
return null;
}
StringBuffer sb = new StringBuffer(input.length * 2);
for (int i = 0; i < input.length; i++) {
sb.append(Character.forDigit((input[i] & 240) >> 4, 16));
sb.append(Character.forDigit(input[i] & 15, 16));
}
return sb.toString();
}
protected static final StringBuilder append(StringBuilder buffer,boolean full,byte[] value){
if(full || null == value){
buffer.append(toHex(value));
}else{
buffer.append(value.length).append(" bytes");
}
return buffer;
}
private static int stringLimit = 64;
private static final int MINIMUM_LIMIT = 16;
protected static final StringBuilder append(StringBuilder buffer,boolean full,String value){
if(full || null == value || value.length() <= stringLimit){
buffer.append(value);
}else{
buffer.append(value.substring(0,stringLimit - 8)).append(" ...").append(value.substring(stringLimit-4,stringLimit));
}
return buffer;
}
protected static final StringBuilder append(StringBuilder buffer,boolean full,T value){
return buffer.append(value);
}
public static final void setStringLimit(int limit){
if(limit < MINIMUM_LIMIT){
throw new IllegalArgumentException(String.format("INVALID limit %d,minimum value %d",limit,MINIMUM_LIMIT));
}
stringLimit = limit;
}
@Override
public String toString(boolean notNull, boolean fullIfStringOrBytes) {
// only output initialized field
StringBuilder builder = new StringBuilder(this.getClass().getName()).append("@").append(Integer.toHexString(this.hashCode())).append("[");
int count = 0;
if(checkIdInitialized()){
if(!notNull || null != getId()){
if(count++ >0){
builder.append(",");
}
builder.append("id=");
append(builder,fullIfStringOrBytes,getId());
}
}
if(checkGroupIdInitialized()){
if(!notNull || null != getGroupId()){
if(count++ >0){
builder.append(",");
}
builder.append("group_id=");
append(builder,fullIfStringOrBytes,getGroupId());
}
}
if(checkNameInitialized()){
if(!notNull || null != getName()){
if(count++ >0){
builder.append(",");
}
builder.append("name=");
append(builder,fullIfStringOrBytes,getName());
}
}
if(checkProductNameInitialized()){
if(!notNull || null != getProductName()){
if(count++ >0){
builder.append(",");
}
builder.append("product_name=");
append(builder,fullIfStringOrBytes,getProductName());
}
}
if(checkModelInitialized()){
if(!notNull || null != getModel()){
if(count++ >0){
builder.append(",");
}
builder.append("model=");
append(builder,fullIfStringOrBytes,getModel());
}
}
if(checkVendorInitialized()){
if(!notNull || null != getVendor()){
if(count++ >0){
builder.append(",");
}
builder.append("vendor=");
append(builder,fullIfStringOrBytes,getVendor());
}
}
if(checkManufacturerInitialized()){
if(!notNull || null != getManufacturer()){
if(count++ >0){
builder.append(",");
}
builder.append("manufacturer=");
append(builder,fullIfStringOrBytes,getManufacturer());
}
}
if(checkMadeDateInitialized()){
if(!notNull || null != getMadeDate()){
if(count++ >0){
builder.append(",");
}
builder.append("made_date=");
append(builder,fullIfStringOrBytes,getMadeDate());
}
}
if(checkVersionInitialized()){
if(!notNull || null != getVersion()){
if(count++ >0){
builder.append(",");
}
builder.append("version=");
append(builder,fullIfStringOrBytes,getVersion());
}
}
if(checkSdkVersionInitialized()){
if(!notNull || null != getSdkVersion()){
if(count++ >0){
builder.append(",");
}
builder.append("sdk_version=");
append(builder,fullIfStringOrBytes,getSdkVersion());
}
}
if(checkSerialNoInitialized()){
if(!notNull || null != getSerialNo()){
if(count++ >0){
builder.append(",");
}
builder.append("serial_no=");
append(builder,fullIfStringOrBytes,getSerialNo());
}
}
if(checkMacInitialized()){
if(!notNull || null != getMac()){
if(count++ >0){
builder.append(",");
}
builder.append("mac=");
append(builder,fullIfStringOrBytes,getMac());
}
}
if(checkRemarkInitialized()){
if(!notNull || null != getRemark()){
if(count++ >0){
builder.append(",");
}
builder.append("remark=");
append(builder,fullIfStringOrBytes,getRemark());
}
}
if(checkExtBinInitialized()){
if(!notNull || null != getExtBin()){
if(count++ >0){
builder.append(",");
}
builder.append("ext_bin=");
append(builder,fullIfStringOrBytes,getExtBin());
}
}
if(checkExtTxtInitialized()){
if(!notNull || null != getExtTxt()){
if(count++ >0){
builder.append(",");
}
builder.append("ext_txt=");
append(builder,fullIfStringOrBytes,getExtTxt());
}
}
if(checkCreateTimeInitialized()){
if(!notNull || null != getCreateTime()){
if(count++ >0){
builder.append(",");
}
builder.append("create_time=");
append(builder,fullIfStringOrBytes,getCreateTime());
}
}
if(checkUpdateTimeInitialized()){
if(!notNull || null != getUpdateTime()){
if(count++ >0){
builder.append(",");
}
builder.append("update_time=");
append(builder,fullIfStringOrBytes,getUpdateTime());
}
}
builder.append("]");
return builder.toString();
}
@Override
public int compareTo(FlDeviceBean object){
return new CompareToBuilder()
.append(getId(), object.getId())
.append(getGroupId(), object.getGroupId())
.append(getName(), object.getName())
.append(getProductName(), object.getProductName())
.append(getModel(), object.getModel())
.append(getVendor(), object.getVendor())
.append(getManufacturer(), object.getManufacturer())
.append(getMadeDate(), object.getMadeDate())
.append(getVersion(), object.getVersion())
.append(getSdkVersion(), object.getSdkVersion())
.append(getSerialNo(), object.getSerialNo())
.append(getMac(), object.getMac())
.append(getRemark(), object.getRemark())
.append(getExtBin(), object.getExtBin())
.append(getExtTxt(), object.getExtTxt())
.append(getCreateTime(), object.getCreateTime())
.append(getUpdateTime(), object.getUpdateTime())
.toComparison();
}
@Override
public FlDeviceBean clone(){
try {
return (FlDeviceBean) super.clone();
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
}
/**
* Make {@code this} to a NULL bean
* set all fields to null, {@link #modified} and {@link #initialized} be set to 0
* @return {@code this} bean
* @author guyadong
*/
public FlDeviceBean asNULL()
{
checkMutable();
setId((Integer)null);
setGroupId((Integer)null);
setName((String)null);
setProductName((String)null);
setModel((String)null);
setVendor((String)null);
setManufacturer((String)null);
setMadeDate((java.util.Date)null);
setVersion((String)null);
setSdkVersion((String)null);
setSerialNo((String)null);
setMac((String)null);
setRemark((String)null);
setExtBin((java.nio.ByteBuffer)null);
setExtTxt((String)null);
setCreateTime((java.util.Date)null);
setUpdateTime((java.util.Date)null);
isNew(true);
resetInitialized();
resetIsModified();
return this;
}
/**
* check whether this bean is a NULL bean
* @return {@code true} if {@link #initialized} be set to zero
* @see #asNULL()
*/
public boolean checkNULL(){
return 0 == getInitialized();
}
/**
* @param source source list
* @return {@code source} replace {@code null} element with null instance({@link #NULL})
*/
public static final List replaceNull(List source){
if(null != source){
for(int i = 0,endIndex = source.size();i replaceNullInstance(List source){
if(null != source){
for(int i = 0,endIndex = source.size();iT getValue(int columnID)
{
switch( columnID ){
case FL_DEVICE_ID_ID:
return (T)getId();
case FL_DEVICE_ID_GROUP_ID:
return (T)getGroupId();
case FL_DEVICE_ID_NAME:
return (T)getName();
case FL_DEVICE_ID_PRODUCT_NAME:
return (T)getProductName();
case FL_DEVICE_ID_MODEL:
return (T)getModel();
case FL_DEVICE_ID_VENDOR:
return (T)getVendor();
case FL_DEVICE_ID_MANUFACTURER:
return (T)getManufacturer();
case FL_DEVICE_ID_MADE_DATE:
return (T)getMadeDate();
case FL_DEVICE_ID_VERSION:
return (T)getVersion();
case FL_DEVICE_ID_SDK_VERSION:
return (T)getSdkVersion();
case FL_DEVICE_ID_SERIAL_NO:
return (T)getSerialNo();
case FL_DEVICE_ID_MAC:
return (T)getMac();
case FL_DEVICE_ID_REMARK:
return (T)getRemark();
case FL_DEVICE_ID_EXT_BIN:
return (T)getExtBin();
case FL_DEVICE_ID_EXT_TXT:
return (T)getExtTxt();
case FL_DEVICE_ID_CREATE_TIME:
return (T)getCreateTime();
case FL_DEVICE_ID_UPDATE_TIME:
return (T)getUpdateTime();
default:
return null;
}
}
@Override
public void setValue(int columnID,T value)
{
switch( columnID ) {
case FL_DEVICE_ID_ID:
setId((Integer)value);
break;
case FL_DEVICE_ID_GROUP_ID:
setGroupId((Integer)value);
break;
case FL_DEVICE_ID_NAME:
setName((String)value);
break;
case FL_DEVICE_ID_PRODUCT_NAME:
setProductName((String)value);
break;
case FL_DEVICE_ID_MODEL:
setModel((String)value);
break;
case FL_DEVICE_ID_VENDOR:
setVendor((String)value);
break;
case FL_DEVICE_ID_MANUFACTURER:
setManufacturer((String)value);
break;
case FL_DEVICE_ID_MADE_DATE:
setMadeDate((java.util.Date)value);
break;
case FL_DEVICE_ID_VERSION:
setVersion((String)value);
break;
case FL_DEVICE_ID_SDK_VERSION:
setSdkVersion((String)value);
break;
case FL_DEVICE_ID_SERIAL_NO:
setSerialNo((String)value);
break;
case FL_DEVICE_ID_MAC:
setMac((String)value);
break;
case FL_DEVICE_ID_REMARK:
setRemark((String)value);
break;
case FL_DEVICE_ID_EXT_BIN:
setExtBin((java.nio.ByteBuffer)value);
break;
case FL_DEVICE_ID_EXT_TXT:
setExtTxt((String)value);
break;
case FL_DEVICE_ID_CREATE_TIME:
setCreateTime((java.util.Date)value);
break;
case FL_DEVICE_ID_UPDATE_TIME:
setUpdateTime((java.util.Date)value);
break;
default:
break;
}
}
@Override
public T getValue(String column)
{
return getValue(columnIDOf(column));
}
@Override
public void setValue(String column,T value)
{
setValue(columnIDOf(column),value);
}
/**
* @param column column name
* @return column id for the given field name or negative if {@code column} is invalid name
*/
public static int columnIDOf(String column){
int index = FL_DEVICE_FIELDS_LIST.indexOf(column);
return index < 0
? FL_DEVICE_JAVA_FIELDS_LIST.indexOf(column)
: index;
}
public static final Builder builder(){
return new Builder().reset();
}
/**
* a builder for FlDeviceBean,the template instance is thread local variable
* a instance of Builder can be reused.
*/
public static final class Builder{
/** FlDeviceBean instance used for template to create new FlDeviceBean instance. */
static final ThreadLocal TEMPLATE = new ThreadLocal(){
@Override
protected FlDeviceBean initialValue() {
return new FlDeviceBean();
}};
private Builder() {}
/**
* reset the bean as template
* @see FlDeviceBean#reset()
*/
public Builder reset(){
TEMPLATE.get().reset();
return this;
}
/** set a bean as template,must not be {@code null} */
public Builder template(FlDeviceBean bean){
if(null == bean){
throw new NullPointerException();
}
TEMPLATE.set(bean);
return this;
}
/** return a clone instance of {@link #TEMPLATE}*/
public FlDeviceBean build(){
return TEMPLATE.get().clone();
}
/**
* fill the field : fl_device.id
* @param id 设备id
* @see FlDeviceBean#getId()
* @see FlDeviceBean#setId(Integer)
*/
public Builder id(Integer id){
TEMPLATE.get().setId(id);
return this;
}
/**
* fill the field : fl_device.group_id
* @param groupId 所属设备组id
* @see FlDeviceBean#getGroupId()
* @see FlDeviceBean#setGroupId(Integer)
*/
public Builder groupId(Integer groupId){
TEMPLATE.get().setGroupId(groupId);
return this;
}
/**
* fill the field : fl_device.name
* @param name 设备名称
* @see FlDeviceBean#getName()
* @see FlDeviceBean#setName(String)
*/
public Builder name(String name){
TEMPLATE.get().setName(name);
return this;
}
/**
* fill the field : fl_device.product_name
* @param productName 产品名称
* @see FlDeviceBean#getProductName()
* @see FlDeviceBean#setProductName(String)
*/
public Builder productName(String productName){
TEMPLATE.get().setProductName(productName);
return this;
}
/**
* fill the field : fl_device.model
* @param model 设备型号
* @see FlDeviceBean#getModel()
* @see FlDeviceBean#setModel(String)
*/
public Builder model(String model){
TEMPLATE.get().setModel(model);
return this;
}
/**
* fill the field : fl_device.vendor
* @param vendor 设备供应商
* @see FlDeviceBean#getVendor()
* @see FlDeviceBean#setVendor(String)
*/
public Builder vendor(String vendor){
TEMPLATE.get().setVendor(vendor);
return this;
}
/**
* fill the field : fl_device.manufacturer
* @param manufacturer 设备制造商
* @see FlDeviceBean#getManufacturer()
* @see FlDeviceBean#setManufacturer(String)
*/
public Builder manufacturer(String manufacturer){
TEMPLATE.get().setManufacturer(manufacturer);
return this;
}
/**
* fill the field : fl_device.made_date
* @param madeDate 设备生产日期
* @see FlDeviceBean#getMadeDate()
* @see FlDeviceBean#setMadeDate(java.util.Date)
*/
public Builder madeDate(java.util.Date madeDate){
TEMPLATE.get().setMadeDate(madeDate);
return this;
}
/**
* fill the field : fl_device.version
* @param version 设备版本号
* @see FlDeviceBean#getVersion()
* @see FlDeviceBean#setVersion(String)
*/
public Builder version(String version){
TEMPLATE.get().setVersion(version);
return this;
}
/**
* fill the field : fl_device.sdk_version
* @param sdkVersion (特征码)算法版本号,用于区分不同人脸识别算法生成的特征数据(允许字母,数字,-,.,_符号)
* @see FlDeviceBean#getSdkVersion()
* @see FlDeviceBean#setSdkVersion(String)
*/
public Builder sdkVersion(String sdkVersion){
TEMPLATE.get().setSdkVersion(sdkVersion);
return this;
}
/**
* fill the field : fl_device.serial_no
* @param serialNo 设备序列号
* @see FlDeviceBean#getSerialNo()
* @see FlDeviceBean#setSerialNo(String)
*/
public Builder serialNo(String serialNo){
TEMPLATE.get().setSerialNo(serialNo);
return this;
}
/**
* fill the field : fl_device.mac
* @param mac 6字节MAC地址(HEX)
* @see FlDeviceBean#getMac()
* @see FlDeviceBean#setMac(String)
*/
public Builder mac(String mac){
TEMPLATE.get().setMac(mac);
return this;
}
/**
* fill the field : fl_device.remark
* @param remark 备注
* @see FlDeviceBean#getRemark()
* @see FlDeviceBean#setRemark(String)
*/
public Builder remark(String remark){
TEMPLATE.get().setRemark(remark);
return this;
}
/**
* fill the field : fl_device.ext_bin
* @param extBin 应用项目自定义二进制扩展字段(最大64KB)
* @see FlDeviceBean#getExtBin()
* @see FlDeviceBean#setExtBin(java.nio.ByteBuffer)
*/
public Builder extBin(java.nio.ByteBuffer extBin){
TEMPLATE.get().setExtBin(extBin);
return this;
}
/**
* fill the field : fl_device.ext_txt
* @param extTxt 应用项目自定义文本扩展字段(最大64KB)
* @see FlDeviceBean#getExtTxt()
* @see FlDeviceBean#setExtTxt(String)
*/
public Builder extTxt(String extTxt){
TEMPLATE.get().setExtTxt(extTxt);
return this;
}
/**
* fill the field : fl_device.create_time
* @param createTime
* @see FlDeviceBean#getCreateTime()
* @see FlDeviceBean#setCreateTime(java.util.Date)
*/
public Builder createTime(java.util.Date createTime){
TEMPLATE.get().setCreateTime(createTime);
return this;
}
/**
* fill the field : fl_device.update_time
* @param updateTime
* @see FlDeviceBean#getUpdateTime()
* @see FlDeviceBean#setUpdateTime(java.util.Date)
*/
public Builder updateTime(java.util.Date updateTime){
TEMPLATE.get().setUpdateTime(updateTime);
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy