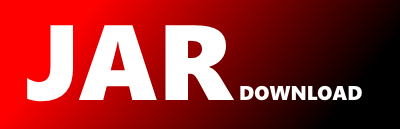
net.gdface.facelog.dborm.face.FlFaceBean Maven / Gradle / Ivy
// ______________________________________________________
// Generated by sql2java - https://github.com/10km/sql2java-2-6-7 (custom branch)
// modified by guyadong from
// sql2java original version https://sourceforge.net/projects/sql2java/
// JDBC driver used at code generation time: com.mysql.jdbc.Driver
// template: bean.java.vm
// ______________________________________________________
package net.gdface.facelog.dborm.face;
import java.io.Serializable;
import java.util.List;
import java.util.Objects;
import net.gdface.facelog.dborm.Constant;
import net.gdface.facelog.dborm.BaseBean;
import net.gdface.facelog.dborm.image.FlImageBean;
import net.gdface.facelog.dborm.CompareToBuilder;
import net.gdface.facelog.dborm.EqualsBuilder;
import net.gdface.facelog.dborm.HashCodeBuilder;
/**
* FlFaceBean is a mapping of fl_face Table.
*
Meta Data Information (in progress):
*
* - comments: 人脸检测信息数据表,用于保存检测到的人脸的所有信息(特征数据除外)
*
* @author guyadong
*/
public class FlFaceBean
implements Serializable,BaseBean,Comparable,Constant,Cloneable
{
private static final long serialVersionUID = 2979758335038585995L;
/** NULL {@link FlFaceBean} bean , IMMUTABLE instance */
public static final FlFaceBean NULL = new FlFaceBean().asNULL().asImmutable();
/** comments:主键 */
private Integer id;
/** comments:外键,所属图像id */
private String imageMd5;
/** comments:人脸位置矩形:x */
private Integer faceLeft;
/** comments:人脸位置矩形:y */
private Integer faceTop;
/** comments:人脸位置矩形:width */
private Integer faceWidth;
/** comments:人脸位置矩形:height */
private Integer faceHeight;
/** comments:左眼位置:x */
private Integer eyeLeftx;
/** comments:左眼位置:y */
private Integer eyeLefty;
/** comments:右眼位置:x */
private Integer eyeRightx;
/** comments:右眼位置:y */
private Integer eyeRighty;
/** comments:嘴巴位置:x */
private Integer mouthX;
/** comments:嘴巴位置:y */
private Integer mouthY;
/** comments:鼻子位置:x */
private Integer noseX;
/** comments:鼻子位置:y */
private Integer noseY;
/** comments:人脸姿态:偏航角 */
private Integer angleYaw;
/** comments:人脸姿态:俯仰角 */
private Integer anglePitch;
/** comments:人脸姿态:滚转角 */
private Integer angleRoll;
/** comments:扩展字段,保存人脸检测基本信息之外的其他数据,内容由SDK负责解析 */
private java.nio.ByteBuffer extInfo;
/** comments:外键,人脸特征数据MD5 id */
private String featureMd5;
/** flag whether {@code this} can be modified */
private Boolean immutable;
/** columns modified flag */
private int modified;
/** columns initialized flag */
private int initialized;
/** new record flag */
private boolean isNew;
/**
* set immutable status
* @return {@code this}
*/
private FlFaceBean immutable(Boolean immutable) {
this.immutable = immutable;
return this;
}
/**
* set {@code this} as immutable object
* @return {@code this}
*/
public FlFaceBean asImmutable() {
return immutable(Boolean.TRUE);
}
/**
* @return {@code true} if {@code this} is a mutable object
*/
public boolean mutable(){
return !Boolean.TRUE.equals(this.immutable);
}
/**
* @return {@code this}
* @throws IllegalStateException if {@code this} is a immutable object
*/
private FlFaceBean checkMutable(){
if(!mutable()){
throw new IllegalStateException("this is a immutable object");
}
return this;
}
/**
* @return return a new mutable copy of this object.
*/
public FlFaceBean cloneMutable(){
return clone().immutable(null);
}
@Override
public boolean isNew()
{
return this.isNew;
}
@Override
public void isNew(boolean isNew)
{
this.isNew = isNew;
}
/**
* Specifies to the object if it has been set as new.
*
* @param isNew the boolean value to be assigned to the isNew field
*/
public void setNew(boolean isNew)
{
this.isNew = isNew;
}
/**
* @return the modified status of columns
*/
public int getModified(){
return modified;
}
/**
* @param modified the modified status bit to be assigned to {@link #modified}
*/
public void setModified(int modified){
this.modified = modified;
}
/**
* @return the initialized status of columns
*/
public int getInitialized(){
return initialized;
}
/**
* @param initialized the initialized status bit to be assigned to {@link #initialized}
*/
public void setInitialized(int initialized){
this.initialized = initialized;
}
protected static final >boolean equals(T a, T b) {
return a == b || (a != null && 0==a.compareTo(b));
}
public FlFaceBean(){
super();
reset();
}
/**
* construct a new instance filled with primary keys
* @param id PK# 1
*/
public FlFaceBean(Integer id){
this();
setId(id);
}
/**
* Getter method for {@link #id}.
* PRIMARY KEY.
* Meta Data Information (in progress):
*
* - full name: fl_face.id
* - imported key: fl_log.compare_face
* - comments: 主键
* - AUTO_INCREMENT
* - NOT NULL
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of id
*/
public Integer getId(){
return id;
}
/**
* Setter method for {@link #id}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to id
*/
public void setId(Integer newVal)
{
checkMutable();
modified |= FL_FACE_ID_ID_MASK;
initialized |= FL_FACE_ID_ID_MASK;
if (Objects.equals(newVal, id)) {
return;
}
id = newVal;
}
/**
* Determines if the id has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkIdModified()
{
return 0L != (modified & FL_FACE_ID_ID_MASK);
}
/**
* Determines if the id has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkIdInitialized()
{
return 0L != (initialized & FL_FACE_ID_ID_MASK);
}
/**
* Getter method for {@link #imageMd5}.
* Meta Data Information (in progress):
*
* - full name: fl_face.image_md5
* - foreign key: fl_image.md5
* - comments: 外键,所属图像id
* - NOT NULL
* - column size: 32
* - JDBC type returned by the driver: Types.CHAR
*
*
* @return the value of imageMd5
*/
public String getImageMd5(){
return imageMd5;
}
/**
* Setter method for {@link #imageMd5}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to imageMd5
*/
public void setImageMd5(String newVal)
{
checkMutable();
modified |= FL_FACE_ID_IMAGE_MD5_MASK;
initialized |= FL_FACE_ID_IMAGE_MD5_MASK;
if (Objects.equals(newVal, imageMd5)) {
return;
}
imageMd5 = newVal;
}
/**
* Determines if the imageMd5 has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkImageMd5Modified()
{
return 0L != (modified & FL_FACE_ID_IMAGE_MD5_MASK);
}
/**
* Determines if the imageMd5 has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkImageMd5Initialized()
{
return 0L != (initialized & FL_FACE_ID_IMAGE_MD5_MASK);
}
/**
* Getter method for {@link #faceLeft}.
* Meta Data Information (in progress):
*
* - full name: fl_face.face_left
* - comments: 人脸位置矩形:x
* - NOT NULL
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of faceLeft
*/
public Integer getFaceLeft(){
return faceLeft;
}
/**
* Setter method for {@link #faceLeft}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to faceLeft
*/
public void setFaceLeft(Integer newVal)
{
checkMutable();
modified |= FL_FACE_ID_FACE_LEFT_MASK;
initialized |= FL_FACE_ID_FACE_LEFT_MASK;
if (Objects.equals(newVal, faceLeft)) {
return;
}
faceLeft = newVal;
}
/**
* Determines if the faceLeft has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkFaceLeftModified()
{
return 0L != (modified & FL_FACE_ID_FACE_LEFT_MASK);
}
/**
* Determines if the faceLeft has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkFaceLeftInitialized()
{
return 0L != (initialized & FL_FACE_ID_FACE_LEFT_MASK);
}
/**
* Getter method for {@link #faceTop}.
* Meta Data Information (in progress):
*
* - full name: fl_face.face_top
* - comments: 人脸位置矩形:y
* - NOT NULL
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of faceTop
*/
public Integer getFaceTop(){
return faceTop;
}
/**
* Setter method for {@link #faceTop}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to faceTop
*/
public void setFaceTop(Integer newVal)
{
checkMutable();
modified |= FL_FACE_ID_FACE_TOP_MASK;
initialized |= FL_FACE_ID_FACE_TOP_MASK;
if (Objects.equals(newVal, faceTop)) {
return;
}
faceTop = newVal;
}
/**
* Determines if the faceTop has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkFaceTopModified()
{
return 0L != (modified & FL_FACE_ID_FACE_TOP_MASK);
}
/**
* Determines if the faceTop has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkFaceTopInitialized()
{
return 0L != (initialized & FL_FACE_ID_FACE_TOP_MASK);
}
/**
* Getter method for {@link #faceWidth}.
* Meta Data Information (in progress):
*
* - full name: fl_face.face_width
* - comments: 人脸位置矩形:width
* - NOT NULL
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of faceWidth
*/
public Integer getFaceWidth(){
return faceWidth;
}
/**
* Setter method for {@link #faceWidth}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to faceWidth
*/
public void setFaceWidth(Integer newVal)
{
checkMutable();
modified |= FL_FACE_ID_FACE_WIDTH_MASK;
initialized |= FL_FACE_ID_FACE_WIDTH_MASK;
if (Objects.equals(newVal, faceWidth)) {
return;
}
faceWidth = newVal;
}
/**
* Determines if the faceWidth has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkFaceWidthModified()
{
return 0L != (modified & FL_FACE_ID_FACE_WIDTH_MASK);
}
/**
* Determines if the faceWidth has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkFaceWidthInitialized()
{
return 0L != (initialized & FL_FACE_ID_FACE_WIDTH_MASK);
}
/**
* Getter method for {@link #faceHeight}.
* Meta Data Information (in progress):
*
* - full name: fl_face.face_height
* - comments: 人脸位置矩形:height
* - NOT NULL
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of faceHeight
*/
public Integer getFaceHeight(){
return faceHeight;
}
/**
* Setter method for {@link #faceHeight}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to faceHeight
*/
public void setFaceHeight(Integer newVal)
{
checkMutable();
modified |= FL_FACE_ID_FACE_HEIGHT_MASK;
initialized |= FL_FACE_ID_FACE_HEIGHT_MASK;
if (Objects.equals(newVal, faceHeight)) {
return;
}
faceHeight = newVal;
}
/**
* Determines if the faceHeight has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkFaceHeightModified()
{
return 0L != (modified & FL_FACE_ID_FACE_HEIGHT_MASK);
}
/**
* Determines if the faceHeight has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkFaceHeightInitialized()
{
return 0L != (initialized & FL_FACE_ID_FACE_HEIGHT_MASK);
}
/**
* Getter method for {@link #eyeLeftx}.
* Meta Data Information (in progress):
*
* - full name: fl_face.eye_leftx
* - comments: 左眼位置:x
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of eyeLeftx
*/
public Integer getEyeLeftx(){
return eyeLeftx;
}
/**
* Setter method for {@link #eyeLeftx}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to eyeLeftx
*/
public void setEyeLeftx(Integer newVal)
{
checkMutable();
modified |= FL_FACE_ID_EYE_LEFTX_MASK;
initialized |= FL_FACE_ID_EYE_LEFTX_MASK;
if (Objects.equals(newVal, eyeLeftx)) {
return;
}
eyeLeftx = newVal;
}
/**
* Determines if the eyeLeftx has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkEyeLeftxModified()
{
return 0L != (modified & FL_FACE_ID_EYE_LEFTX_MASK);
}
/**
* Determines if the eyeLeftx has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkEyeLeftxInitialized()
{
return 0L != (initialized & FL_FACE_ID_EYE_LEFTX_MASK);
}
/**
* Getter method for {@link #eyeLefty}.
* Meta Data Information (in progress):
*
* - full name: fl_face.eye_lefty
* - comments: 左眼位置:y
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of eyeLefty
*/
public Integer getEyeLefty(){
return eyeLefty;
}
/**
* Setter method for {@link #eyeLefty}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to eyeLefty
*/
public void setEyeLefty(Integer newVal)
{
checkMutable();
modified |= FL_FACE_ID_EYE_LEFTY_MASK;
initialized |= FL_FACE_ID_EYE_LEFTY_MASK;
if (Objects.equals(newVal, eyeLefty)) {
return;
}
eyeLefty = newVal;
}
/**
* Determines if the eyeLefty has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkEyeLeftyModified()
{
return 0L != (modified & FL_FACE_ID_EYE_LEFTY_MASK);
}
/**
* Determines if the eyeLefty has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkEyeLeftyInitialized()
{
return 0L != (initialized & FL_FACE_ID_EYE_LEFTY_MASK);
}
/**
* Getter method for {@link #eyeRightx}.
* Meta Data Information (in progress):
*
* - full name: fl_face.eye_rightx
* - comments: 右眼位置:x
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of eyeRightx
*/
public Integer getEyeRightx(){
return eyeRightx;
}
/**
* Setter method for {@link #eyeRightx}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to eyeRightx
*/
public void setEyeRightx(Integer newVal)
{
checkMutable();
modified |= FL_FACE_ID_EYE_RIGHTX_MASK;
initialized |= FL_FACE_ID_EYE_RIGHTX_MASK;
if (Objects.equals(newVal, eyeRightx)) {
return;
}
eyeRightx = newVal;
}
/**
* Determines if the eyeRightx has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkEyeRightxModified()
{
return 0L != (modified & FL_FACE_ID_EYE_RIGHTX_MASK);
}
/**
* Determines if the eyeRightx has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkEyeRightxInitialized()
{
return 0L != (initialized & FL_FACE_ID_EYE_RIGHTX_MASK);
}
/**
* Getter method for {@link #eyeRighty}.
* Meta Data Information (in progress):
*
* - full name: fl_face.eye_righty
* - comments: 右眼位置:y
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of eyeRighty
*/
public Integer getEyeRighty(){
return eyeRighty;
}
/**
* Setter method for {@link #eyeRighty}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to eyeRighty
*/
public void setEyeRighty(Integer newVal)
{
checkMutable();
modified |= FL_FACE_ID_EYE_RIGHTY_MASK;
initialized |= FL_FACE_ID_EYE_RIGHTY_MASK;
if (Objects.equals(newVal, eyeRighty)) {
return;
}
eyeRighty = newVal;
}
/**
* Determines if the eyeRighty has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkEyeRightyModified()
{
return 0L != (modified & FL_FACE_ID_EYE_RIGHTY_MASK);
}
/**
* Determines if the eyeRighty has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkEyeRightyInitialized()
{
return 0L != (initialized & FL_FACE_ID_EYE_RIGHTY_MASK);
}
/**
* Getter method for {@link #mouthX}.
* Meta Data Information (in progress):
*
* - full name: fl_face.mouth_x
* - comments: 嘴巴位置:x
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of mouthX
*/
public Integer getMouthX(){
return mouthX;
}
/**
* Setter method for {@link #mouthX}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to mouthX
*/
public void setMouthX(Integer newVal)
{
checkMutable();
modified |= FL_FACE_ID_MOUTH_X_MASK;
initialized |= FL_FACE_ID_MOUTH_X_MASK;
if (Objects.equals(newVal, mouthX)) {
return;
}
mouthX = newVal;
}
/**
* Determines if the mouthX has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkMouthXModified()
{
return 0L != (modified & FL_FACE_ID_MOUTH_X_MASK);
}
/**
* Determines if the mouthX has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkMouthXInitialized()
{
return 0L != (initialized & FL_FACE_ID_MOUTH_X_MASK);
}
/**
* Getter method for {@link #mouthY}.
* Meta Data Information (in progress):
*
* - full name: fl_face.mouth_y
* - comments: 嘴巴位置:y
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of mouthY
*/
public Integer getMouthY(){
return mouthY;
}
/**
* Setter method for {@link #mouthY}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to mouthY
*/
public void setMouthY(Integer newVal)
{
checkMutable();
modified |= FL_FACE_ID_MOUTH_Y_MASK;
initialized |= FL_FACE_ID_MOUTH_Y_MASK;
if (Objects.equals(newVal, mouthY)) {
return;
}
mouthY = newVal;
}
/**
* Determines if the mouthY has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkMouthYModified()
{
return 0L != (modified & FL_FACE_ID_MOUTH_Y_MASK);
}
/**
* Determines if the mouthY has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkMouthYInitialized()
{
return 0L != (initialized & FL_FACE_ID_MOUTH_Y_MASK);
}
/**
* Getter method for {@link #noseX}.
* Meta Data Information (in progress):
*
* - full name: fl_face.nose_x
* - comments: 鼻子位置:x
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of noseX
*/
public Integer getNoseX(){
return noseX;
}
/**
* Setter method for {@link #noseX}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to noseX
*/
public void setNoseX(Integer newVal)
{
checkMutable();
modified |= FL_FACE_ID_NOSE_X_MASK;
initialized |= FL_FACE_ID_NOSE_X_MASK;
if (Objects.equals(newVal, noseX)) {
return;
}
noseX = newVal;
}
/**
* Determines if the noseX has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkNoseXModified()
{
return 0L != (modified & FL_FACE_ID_NOSE_X_MASK);
}
/**
* Determines if the noseX has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkNoseXInitialized()
{
return 0L != (initialized & FL_FACE_ID_NOSE_X_MASK);
}
/**
* Getter method for {@link #noseY}.
* Meta Data Information (in progress):
*
* - full name: fl_face.nose_y
* - comments: 鼻子位置:y
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of noseY
*/
public Integer getNoseY(){
return noseY;
}
/**
* Setter method for {@link #noseY}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to noseY
*/
public void setNoseY(Integer newVal)
{
checkMutable();
modified |= FL_FACE_ID_NOSE_Y_MASK;
initialized |= FL_FACE_ID_NOSE_Y_MASK;
if (Objects.equals(newVal, noseY)) {
return;
}
noseY = newVal;
}
/**
* Determines if the noseY has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkNoseYModified()
{
return 0L != (modified & FL_FACE_ID_NOSE_Y_MASK);
}
/**
* Determines if the noseY has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkNoseYInitialized()
{
return 0L != (initialized & FL_FACE_ID_NOSE_Y_MASK);
}
/**
* Getter method for {@link #angleYaw}.
* Meta Data Information (in progress):
*
* - full name: fl_face.angle_yaw
* - comments: 人脸姿态:偏航角
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of angleYaw
*/
public Integer getAngleYaw(){
return angleYaw;
}
/**
* Setter method for {@link #angleYaw}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to angleYaw
*/
public void setAngleYaw(Integer newVal)
{
checkMutable();
modified |= FL_FACE_ID_ANGLE_YAW_MASK;
initialized |= FL_FACE_ID_ANGLE_YAW_MASK;
if (Objects.equals(newVal, angleYaw)) {
return;
}
angleYaw = newVal;
}
/**
* Determines if the angleYaw has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkAngleYawModified()
{
return 0L != (modified & FL_FACE_ID_ANGLE_YAW_MASK);
}
/**
* Determines if the angleYaw has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkAngleYawInitialized()
{
return 0L != (initialized & FL_FACE_ID_ANGLE_YAW_MASK);
}
/**
* Getter method for {@link #anglePitch}.
* Meta Data Information (in progress):
*
* - full name: fl_face.angle_pitch
* - comments: 人脸姿态:俯仰角
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of anglePitch
*/
public Integer getAnglePitch(){
return anglePitch;
}
/**
* Setter method for {@link #anglePitch}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to anglePitch
*/
public void setAnglePitch(Integer newVal)
{
checkMutable();
modified |= FL_FACE_ID_ANGLE_PITCH_MASK;
initialized |= FL_FACE_ID_ANGLE_PITCH_MASK;
if (Objects.equals(newVal, anglePitch)) {
return;
}
anglePitch = newVal;
}
/**
* Determines if the anglePitch has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkAnglePitchModified()
{
return 0L != (modified & FL_FACE_ID_ANGLE_PITCH_MASK);
}
/**
* Determines if the anglePitch has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkAnglePitchInitialized()
{
return 0L != (initialized & FL_FACE_ID_ANGLE_PITCH_MASK);
}
/**
* Getter method for {@link #angleRoll}.
* Meta Data Information (in progress):
*
* - full name: fl_face.angle_roll
* - comments: 人脸姿态:滚转角
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of angleRoll
*/
public Integer getAngleRoll(){
return angleRoll;
}
/**
* Setter method for {@link #angleRoll}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to angleRoll
*/
public void setAngleRoll(Integer newVal)
{
checkMutable();
modified |= FL_FACE_ID_ANGLE_ROLL_MASK;
initialized |= FL_FACE_ID_ANGLE_ROLL_MASK;
if (Objects.equals(newVal, angleRoll)) {
return;
}
angleRoll = newVal;
}
/**
* Determines if the angleRoll has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkAngleRollModified()
{
return 0L != (modified & FL_FACE_ID_ANGLE_ROLL_MASK);
}
/**
* Determines if the angleRoll has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkAngleRollInitialized()
{
return 0L != (initialized & FL_FACE_ID_ANGLE_ROLL_MASK);
}
/**
* Getter method for {@link #extInfo}.
* Meta Data Information (in progress):
*
* - full name: fl_face.ext_info
* - comments: 扩展字段,保存人脸检测基本信息之外的其他数据,内容由SDK负责解析
* - column size: 65535
* - JDBC type returned by the driver: Types.LONGVARBINARY
*
*
* @return the value of extInfo
*/
public java.nio.ByteBuffer getExtInfo(){
return extInfo;
}
/**
* Setter method for {@link #extInfo}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to extInfo
*/
public void setExtInfo(java.nio.ByteBuffer newVal)
{
checkMutable();
modified |= FL_FACE_ID_EXT_INFO_MASK;
initialized |= FL_FACE_ID_EXT_INFO_MASK;
if (Objects.equals(newVal, extInfo)) {
return;
}
extInfo = newVal;
}
/**
* Determines if the extInfo has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkExtInfoModified()
{
return 0L != (modified & FL_FACE_ID_EXT_INFO_MASK);
}
/**
* Determines if the extInfo has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkExtInfoInitialized()
{
return 0L != (initialized & FL_FACE_ID_EXT_INFO_MASK);
}
/**
* Getter method for {@link #featureMd5}.
* Meta Data Information (in progress):
*
* - full name: fl_face.feature_md5
* - foreign key: fl_feature.md5
* - comments: 外键,人脸特征数据MD5 id
* - column size: 32
* - JDBC type returned by the driver: Types.CHAR
*
*
* @return the value of featureMd5
*/
public String getFeatureMd5(){
return featureMd5;
}
/**
* Setter method for {@link #featureMd5}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to featureMd5
*/
public void setFeatureMd5(String newVal)
{
checkMutable();
modified |= FL_FACE_ID_FEATURE_MD5_MASK;
initialized |= FL_FACE_ID_FEATURE_MD5_MASK;
if (Objects.equals(newVal, featureMd5)) {
return;
}
featureMd5 = newVal;
}
/**
* Determines if the featureMd5 has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkFeatureMd5Modified()
{
return 0L != (modified & FL_FACE_ID_FEATURE_MD5_MASK);
}
/**
* Determines if the featureMd5 has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkFeatureMd5Initialized()
{
return 0L != (initialized & FL_FACE_ID_FEATURE_MD5_MASK);
}
//////////////////////////////////////
// referenced bean for FOREIGN KEYS
//////////////////////////////////////
/**
* The referenced {@link FlFeatureBean} by {@link #featureMd5} .
* FOREIGN KEY (feature_md5) REFERENCES fl_feature(md5)
*/
private FlFeatureBean referencedByFeatureMd5;
/**
* Getter method for {@link #referencedByFeatureMd5}.
* @return FlFeatureBean
*/
public FlFeatureBean getReferencedByFeatureMd5() {
return this.referencedByFeatureMd5;
}
/**
* Setter method for {@link #referencedByFeatureMd5}.
* @param reference FlFeatureBean
*/
public void setReferencedByFeatureMd5(FlFeatureBean reference) {
this.referencedByFeatureMd5 = reference;
}
/**
* The referenced {@link FlImageBean} by {@link #imageMd5} .
* FOREIGN KEY (image_md5) REFERENCES fl_image(md5)
*/
private FlImageBean referencedByImageMd5;
/**
* Getter method for {@link #referencedByImageMd5}.
* @return FlImageBean
*/
public FlImageBean getReferencedByImageMd5() {
return this.referencedByImageMd5;
}
/**
* Setter method for {@link #referencedByImageMd5}.
* @param reference FlImageBean
*/
public void setReferencedByImageMd5(FlImageBean reference) {
this.referencedByImageMd5 = reference;
}
@Override
public boolean isModified()
{
return 0 != modified;
}
@Override
public boolean isModified(int columnID){
switch ( columnID ){
case FL_FACE_ID_ID:
return checkIdModified();
case FL_FACE_ID_IMAGE_MD5:
return checkImageMd5Modified();
case FL_FACE_ID_FACE_LEFT:
return checkFaceLeftModified();
case FL_FACE_ID_FACE_TOP:
return checkFaceTopModified();
case FL_FACE_ID_FACE_WIDTH:
return checkFaceWidthModified();
case FL_FACE_ID_FACE_HEIGHT:
return checkFaceHeightModified();
case FL_FACE_ID_EYE_LEFTX:
return checkEyeLeftxModified();
case FL_FACE_ID_EYE_LEFTY:
return checkEyeLeftyModified();
case FL_FACE_ID_EYE_RIGHTX:
return checkEyeRightxModified();
case FL_FACE_ID_EYE_RIGHTY:
return checkEyeRightyModified();
case FL_FACE_ID_MOUTH_X:
return checkMouthXModified();
case FL_FACE_ID_MOUTH_Y:
return checkMouthYModified();
case FL_FACE_ID_NOSE_X:
return checkNoseXModified();
case FL_FACE_ID_NOSE_Y:
return checkNoseYModified();
case FL_FACE_ID_ANGLE_YAW:
return checkAngleYawModified();
case FL_FACE_ID_ANGLE_PITCH:
return checkAnglePitchModified();
case FL_FACE_ID_ANGLE_ROLL:
return checkAngleRollModified();
case FL_FACE_ID_EXT_INFO:
return checkExtInfoModified();
case FL_FACE_ID_FEATURE_MD5:
return checkFeatureMd5Modified();
default:
return false;
}
}
@Override
public boolean isInitialized(int columnID){
switch(columnID) {
case FL_FACE_ID_ID:
return checkIdInitialized();
case FL_FACE_ID_IMAGE_MD5:
return checkImageMd5Initialized();
case FL_FACE_ID_FACE_LEFT:
return checkFaceLeftInitialized();
case FL_FACE_ID_FACE_TOP:
return checkFaceTopInitialized();
case FL_FACE_ID_FACE_WIDTH:
return checkFaceWidthInitialized();
case FL_FACE_ID_FACE_HEIGHT:
return checkFaceHeightInitialized();
case FL_FACE_ID_EYE_LEFTX:
return checkEyeLeftxInitialized();
case FL_FACE_ID_EYE_LEFTY:
return checkEyeLeftyInitialized();
case FL_FACE_ID_EYE_RIGHTX:
return checkEyeRightxInitialized();
case FL_FACE_ID_EYE_RIGHTY:
return checkEyeRightyInitialized();
case FL_FACE_ID_MOUTH_X:
return checkMouthXInitialized();
case FL_FACE_ID_MOUTH_Y:
return checkMouthYInitialized();
case FL_FACE_ID_NOSE_X:
return checkNoseXInitialized();
case FL_FACE_ID_NOSE_Y:
return checkNoseYInitialized();
case FL_FACE_ID_ANGLE_YAW:
return checkAngleYawInitialized();
case FL_FACE_ID_ANGLE_PITCH:
return checkAnglePitchInitialized();
case FL_FACE_ID_ANGLE_ROLL:
return checkAngleRollInitialized();
case FL_FACE_ID_EXT_INFO:
return checkExtInfoInitialized();
case FL_FACE_ID_FEATURE_MD5:
return checkFeatureMd5Initialized();
default:
return false;
}
}
@Override
public boolean isModified(String column){
return isModified(columnIDOf(column));
}
@Override
public boolean isInitialized(String column){
return isInitialized(columnIDOf(column));
}
@Override
public void resetIsModified()
{
checkMutable();
modified = 0;
}
@Override
public void resetPrimaryKeysModified()
{
modified &= (~(FL_FACE_ID_ID_MASK));
}
/**
* Resets columns modification status except primary keys to 'not modified'.
*/
public void resetModifiedExceptPrimaryKeys()
{
modified &= (~(FL_FACE_ID_IMAGE_MD5_MASK |
FL_FACE_ID_FACE_LEFT_MASK |
FL_FACE_ID_FACE_TOP_MASK |
FL_FACE_ID_FACE_WIDTH_MASK |
FL_FACE_ID_FACE_HEIGHT_MASK |
FL_FACE_ID_EYE_LEFTX_MASK |
FL_FACE_ID_EYE_LEFTY_MASK |
FL_FACE_ID_EYE_RIGHTX_MASK |
FL_FACE_ID_EYE_RIGHTY_MASK |
FL_FACE_ID_MOUTH_X_MASK |
FL_FACE_ID_MOUTH_Y_MASK |
FL_FACE_ID_NOSE_X_MASK |
FL_FACE_ID_NOSE_Y_MASK |
FL_FACE_ID_ANGLE_YAW_MASK |
FL_FACE_ID_ANGLE_PITCH_MASK |
FL_FACE_ID_ANGLE_ROLL_MASK |
FL_FACE_ID_EXT_INFO_MASK |
FL_FACE_ID_FEATURE_MD5_MASK));
}
/**
* Resets the object initialization status to 'not initialized'.
*/
private void resetInitialized()
{
initialized = 0;
}
/** reset all fields to initial value, equal to a new bean */
public void reset(){
checkMutable();
this.id = null;
this.imageMd5 = null;
this.faceLeft = null;
this.faceTop = null;
this.faceWidth = null;
this.faceHeight = null;
this.eyeLeftx = null;
this.eyeLefty = null;
this.eyeRightx = null;
this.eyeRighty = null;
this.mouthX = null;
this.mouthY = null;
this.noseX = null;
this.noseY = null;
this.angleYaw = null;
this.anglePitch = null;
this.angleRoll = null;
this.extInfo = null;
this.featureMd5 = null;
this.isNew = true;
this.modified = 0;
this.initialized = 0;
}
@Override
public boolean equals(Object object)
{
if (!(object instanceof FlFaceBean)) {
return false;
}
FlFaceBean obj = (FlFaceBean) object;
return new EqualsBuilder()
.append(getId(), obj.getId())
.append(getImageMd5(), obj.getImageMd5())
.append(getFaceLeft(), obj.getFaceLeft())
.append(getFaceTop(), obj.getFaceTop())
.append(getFaceWidth(), obj.getFaceWidth())
.append(getFaceHeight(), obj.getFaceHeight())
.append(getEyeLeftx(), obj.getEyeLeftx())
.append(getEyeLefty(), obj.getEyeLefty())
.append(getEyeRightx(), obj.getEyeRightx())
.append(getEyeRighty(), obj.getEyeRighty())
.append(getMouthX(), obj.getMouthX())
.append(getMouthY(), obj.getMouthY())
.append(getNoseX(), obj.getNoseX())
.append(getNoseY(), obj.getNoseY())
.append(getAngleYaw(), obj.getAngleYaw())
.append(getAnglePitch(), obj.getAnglePitch())
.append(getAngleRoll(), obj.getAngleRoll())
.append(getExtInfo(), obj.getExtInfo())
.append(getFeatureMd5(), obj.getFeatureMd5())
.isEquals();
}
@Override
public int hashCode()
{
return new HashCodeBuilder(-82280557, -700257973)
.append(getId())
.toHashCode();
}
@Override
public String toString() {
return toString(true,false);
}
/**
* cast byte array to HEX string
*
* @param input
* @return {@code null} if {@code input} is null
*/
private static final String toHex(byte[] input) {
if (null == input){
return null;
}
StringBuffer sb = new StringBuffer(input.length * 2);
for (int i = 0; i < input.length; i++) {
sb.append(Character.forDigit((input[i] & 240) >> 4, 16));
sb.append(Character.forDigit(input[i] & 15, 16));
}
return sb.toString();
}
protected static final StringBuilder append(StringBuilder buffer,boolean full,byte[] value){
if(full || null == value){
buffer.append(toHex(value));
}else{
buffer.append(value.length).append(" bytes");
}
return buffer;
}
private static int stringLimit = 64;
private static final int MINIMUM_LIMIT = 16;
protected static final StringBuilder append(StringBuilder buffer,boolean full,String value){
if(full || null == value || value.length() <= stringLimit){
buffer.append(value);
}else{
buffer.append(value.substring(0,stringLimit - 8)).append(" ...").append(value.substring(stringLimit-4,stringLimit));
}
return buffer;
}
protected static final StringBuilder append(StringBuilder buffer,boolean full,T value){
return buffer.append(value);
}
public static final void setStringLimit(int limit){
if(limit < MINIMUM_LIMIT){
throw new IllegalArgumentException(String.format("INVALID limit %d,minimum value %d",limit,MINIMUM_LIMIT));
}
stringLimit = limit;
}
@Override
public String toString(boolean notNull, boolean fullIfStringOrBytes) {
// only output initialized field
StringBuilder builder = new StringBuilder(this.getClass().getName()).append("@").append(Integer.toHexString(this.hashCode())).append("[");
int count = 0;
if(checkIdInitialized()){
if(!notNull || null != getId()){
if(count++ >0){
builder.append(",");
}
builder.append("id=");
append(builder,fullIfStringOrBytes,getId());
}
}
if(checkImageMd5Initialized()){
if(!notNull || null != getImageMd5()){
if(count++ >0){
builder.append(",");
}
builder.append("image_md5=");
append(builder,fullIfStringOrBytes,getImageMd5());
}
}
if(checkFaceLeftInitialized()){
if(!notNull || null != getFaceLeft()){
if(count++ >0){
builder.append(",");
}
builder.append("face_left=");
append(builder,fullIfStringOrBytes,getFaceLeft());
}
}
if(checkFaceTopInitialized()){
if(!notNull || null != getFaceTop()){
if(count++ >0){
builder.append(",");
}
builder.append("face_top=");
append(builder,fullIfStringOrBytes,getFaceTop());
}
}
if(checkFaceWidthInitialized()){
if(!notNull || null != getFaceWidth()){
if(count++ >0){
builder.append(",");
}
builder.append("face_width=");
append(builder,fullIfStringOrBytes,getFaceWidth());
}
}
if(checkFaceHeightInitialized()){
if(!notNull || null != getFaceHeight()){
if(count++ >0){
builder.append(",");
}
builder.append("face_height=");
append(builder,fullIfStringOrBytes,getFaceHeight());
}
}
if(checkEyeLeftxInitialized()){
if(!notNull || null != getEyeLeftx()){
if(count++ >0){
builder.append(",");
}
builder.append("eye_leftx=");
append(builder,fullIfStringOrBytes,getEyeLeftx());
}
}
if(checkEyeLeftyInitialized()){
if(!notNull || null != getEyeLefty()){
if(count++ >0){
builder.append(",");
}
builder.append("eye_lefty=");
append(builder,fullIfStringOrBytes,getEyeLefty());
}
}
if(checkEyeRightxInitialized()){
if(!notNull || null != getEyeRightx()){
if(count++ >0){
builder.append(",");
}
builder.append("eye_rightx=");
append(builder,fullIfStringOrBytes,getEyeRightx());
}
}
if(checkEyeRightyInitialized()){
if(!notNull || null != getEyeRighty()){
if(count++ >0){
builder.append(",");
}
builder.append("eye_righty=");
append(builder,fullIfStringOrBytes,getEyeRighty());
}
}
if(checkMouthXInitialized()){
if(!notNull || null != getMouthX()){
if(count++ >0){
builder.append(",");
}
builder.append("mouth_x=");
append(builder,fullIfStringOrBytes,getMouthX());
}
}
if(checkMouthYInitialized()){
if(!notNull || null != getMouthY()){
if(count++ >0){
builder.append(",");
}
builder.append("mouth_y=");
append(builder,fullIfStringOrBytes,getMouthY());
}
}
if(checkNoseXInitialized()){
if(!notNull || null != getNoseX()){
if(count++ >0){
builder.append(",");
}
builder.append("nose_x=");
append(builder,fullIfStringOrBytes,getNoseX());
}
}
if(checkNoseYInitialized()){
if(!notNull || null != getNoseY()){
if(count++ >0){
builder.append(",");
}
builder.append("nose_y=");
append(builder,fullIfStringOrBytes,getNoseY());
}
}
if(checkAngleYawInitialized()){
if(!notNull || null != getAngleYaw()){
if(count++ >0){
builder.append(",");
}
builder.append("angle_yaw=");
append(builder,fullIfStringOrBytes,getAngleYaw());
}
}
if(checkAnglePitchInitialized()){
if(!notNull || null != getAnglePitch()){
if(count++ >0){
builder.append(",");
}
builder.append("angle_pitch=");
append(builder,fullIfStringOrBytes,getAnglePitch());
}
}
if(checkAngleRollInitialized()){
if(!notNull || null != getAngleRoll()){
if(count++ >0){
builder.append(",");
}
builder.append("angle_roll=");
append(builder,fullIfStringOrBytes,getAngleRoll());
}
}
if(checkExtInfoInitialized()){
if(!notNull || null != getExtInfo()){
if(count++ >0){
builder.append(",");
}
builder.append("ext_info=");
append(builder,fullIfStringOrBytes,getExtInfo());
}
}
if(checkFeatureMd5Initialized()){
if(!notNull || null != getFeatureMd5()){
if(count++ >0){
builder.append(",");
}
builder.append("feature_md5=");
append(builder,fullIfStringOrBytes,getFeatureMd5());
}
}
builder.append("]");
return builder.toString();
}
@Override
public int compareTo(FlFaceBean object){
return new CompareToBuilder()
.append(getId(), object.getId())
.append(getImageMd5(), object.getImageMd5())
.append(getFaceLeft(), object.getFaceLeft())
.append(getFaceTop(), object.getFaceTop())
.append(getFaceWidth(), object.getFaceWidth())
.append(getFaceHeight(), object.getFaceHeight())
.append(getEyeLeftx(), object.getEyeLeftx())
.append(getEyeLefty(), object.getEyeLefty())
.append(getEyeRightx(), object.getEyeRightx())
.append(getEyeRighty(), object.getEyeRighty())
.append(getMouthX(), object.getMouthX())
.append(getMouthY(), object.getMouthY())
.append(getNoseX(), object.getNoseX())
.append(getNoseY(), object.getNoseY())
.append(getAngleYaw(), object.getAngleYaw())
.append(getAnglePitch(), object.getAnglePitch())
.append(getAngleRoll(), object.getAngleRoll())
.append(getExtInfo(), object.getExtInfo())
.append(getFeatureMd5(), object.getFeatureMd5())
.toComparison();
}
@Override
public FlFaceBean clone(){
try {
return (FlFaceBean) super.clone();
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
}
/**
* Make {@code this} to a NULL bean
* set all fields to null, {@link #modified} and {@link #initialized} be set to 0
* @return {@code this} bean
* @author guyadong
*/
public FlFaceBean asNULL()
{
checkMutable();
setId((Integer)null);
setImageMd5((String)null);
setFaceLeft((Integer)null);
setFaceTop((Integer)null);
setFaceWidth((Integer)null);
setFaceHeight((Integer)null);
setEyeLeftx((Integer)null);
setEyeLefty((Integer)null);
setEyeRightx((Integer)null);
setEyeRighty((Integer)null);
setMouthX((Integer)null);
setMouthY((Integer)null);
setNoseX((Integer)null);
setNoseY((Integer)null);
setAngleYaw((Integer)null);
setAnglePitch((Integer)null);
setAngleRoll((Integer)null);
setExtInfo((java.nio.ByteBuffer)null);
setFeatureMd5((String)null);
isNew(true);
resetInitialized();
resetIsModified();
return this;
}
/**
* check whether this bean is a NULL bean
* @return {@code true} if {@link #initialized} be set to zero
* @see #asNULL()
*/
public boolean checkNULL(){
return 0 == getInitialized();
}
/**
* @param source source list
* @return {@code source} replace {@code null} element with null instance({@link #NULL})
*/
public static final List replaceNull(List source){
if(null != source){
for(int i = 0,endIndex = source.size();i replaceNullInstance(List source){
if(null != source){
for(int i = 0,endIndex = source.size();iT getValue(int columnID)
{
switch( columnID ){
case FL_FACE_ID_ID:
return (T)getId();
case FL_FACE_ID_IMAGE_MD5:
return (T)getImageMd5();
case FL_FACE_ID_FACE_LEFT:
return (T)getFaceLeft();
case FL_FACE_ID_FACE_TOP:
return (T)getFaceTop();
case FL_FACE_ID_FACE_WIDTH:
return (T)getFaceWidth();
case FL_FACE_ID_FACE_HEIGHT:
return (T)getFaceHeight();
case FL_FACE_ID_EYE_LEFTX:
return (T)getEyeLeftx();
case FL_FACE_ID_EYE_LEFTY:
return (T)getEyeLefty();
case FL_FACE_ID_EYE_RIGHTX:
return (T)getEyeRightx();
case FL_FACE_ID_EYE_RIGHTY:
return (T)getEyeRighty();
case FL_FACE_ID_MOUTH_X:
return (T)getMouthX();
case FL_FACE_ID_MOUTH_Y:
return (T)getMouthY();
case FL_FACE_ID_NOSE_X:
return (T)getNoseX();
case FL_FACE_ID_NOSE_Y:
return (T)getNoseY();
case FL_FACE_ID_ANGLE_YAW:
return (T)getAngleYaw();
case FL_FACE_ID_ANGLE_PITCH:
return (T)getAnglePitch();
case FL_FACE_ID_ANGLE_ROLL:
return (T)getAngleRoll();
case FL_FACE_ID_EXT_INFO:
return (T)getExtInfo();
case FL_FACE_ID_FEATURE_MD5:
return (T)getFeatureMd5();
default:
return null;
}
}
@Override
public void setValue(int columnID,T value)
{
switch( columnID ) {
case FL_FACE_ID_ID:
setId((Integer)value);
break;
case FL_FACE_ID_IMAGE_MD5:
setImageMd5((String)value);
break;
case FL_FACE_ID_FACE_LEFT:
setFaceLeft((Integer)value);
break;
case FL_FACE_ID_FACE_TOP:
setFaceTop((Integer)value);
break;
case FL_FACE_ID_FACE_WIDTH:
setFaceWidth((Integer)value);
break;
case FL_FACE_ID_FACE_HEIGHT:
setFaceHeight((Integer)value);
break;
case FL_FACE_ID_EYE_LEFTX:
setEyeLeftx((Integer)value);
break;
case FL_FACE_ID_EYE_LEFTY:
setEyeLefty((Integer)value);
break;
case FL_FACE_ID_EYE_RIGHTX:
setEyeRightx((Integer)value);
break;
case FL_FACE_ID_EYE_RIGHTY:
setEyeRighty((Integer)value);
break;
case FL_FACE_ID_MOUTH_X:
setMouthX((Integer)value);
break;
case FL_FACE_ID_MOUTH_Y:
setMouthY((Integer)value);
break;
case FL_FACE_ID_NOSE_X:
setNoseX((Integer)value);
break;
case FL_FACE_ID_NOSE_Y:
setNoseY((Integer)value);
break;
case FL_FACE_ID_ANGLE_YAW:
setAngleYaw((Integer)value);
break;
case FL_FACE_ID_ANGLE_PITCH:
setAnglePitch((Integer)value);
break;
case FL_FACE_ID_ANGLE_ROLL:
setAngleRoll((Integer)value);
break;
case FL_FACE_ID_EXT_INFO:
setExtInfo((java.nio.ByteBuffer)value);
break;
case FL_FACE_ID_FEATURE_MD5:
setFeatureMd5((String)value);
break;
default:
break;
}
}
@Override
public T getValue(String column)
{
return getValue(columnIDOf(column));
}
@Override
public void setValue(String column,T value)
{
setValue(columnIDOf(column),value);
}
/**
* @param column column name
* @return column id for the given field name or negative if {@code column} is invalid name
*/
public static int columnIDOf(String column){
int index = FL_FACE_FIELDS_LIST.indexOf(column);
return index < 0
? FL_FACE_JAVA_FIELDS_LIST.indexOf(column)
: index;
}
public static final Builder builder(){
return new Builder().reset();
}
/**
* a builder for FlFaceBean,the template instance is thread local variable
* a instance of Builder can be reused.
*/
public static final class Builder{
/** FlFaceBean instance used for template to create new FlFaceBean instance. */
static final ThreadLocal TEMPLATE = new ThreadLocal(){
@Override
protected FlFaceBean initialValue() {
return new FlFaceBean();
}};
private Builder() {}
/**
* reset the bean as template
* @see FlFaceBean#reset()
*/
public Builder reset(){
TEMPLATE.get().reset();
return this;
}
/** set a bean as template,must not be {@code null} */
public Builder template(FlFaceBean bean){
if(null == bean){
throw new NullPointerException();
}
TEMPLATE.set(bean);
return this;
}
/** return a clone instance of {@link #TEMPLATE}*/
public FlFaceBean build(){
return TEMPLATE.get().clone();
}
/**
* fill the field : fl_face.id
* @param id 主键
* @see FlFaceBean#getId()
* @see FlFaceBean#setId(Integer)
*/
public Builder id(Integer id){
TEMPLATE.get().setId(id);
return this;
}
/**
* fill the field : fl_face.image_md5
* @param imageMd5 外键,所属图像id
* @see FlFaceBean#getImageMd5()
* @see FlFaceBean#setImageMd5(String)
*/
public Builder imageMd5(String imageMd5){
TEMPLATE.get().setImageMd5(imageMd5);
return this;
}
/**
* fill the field : fl_face.face_left
* @param faceLeft 人脸位置矩形:x
* @see FlFaceBean#getFaceLeft()
* @see FlFaceBean#setFaceLeft(Integer)
*/
public Builder faceLeft(Integer faceLeft){
TEMPLATE.get().setFaceLeft(faceLeft);
return this;
}
/**
* fill the field : fl_face.face_top
* @param faceTop 人脸位置矩形:y
* @see FlFaceBean#getFaceTop()
* @see FlFaceBean#setFaceTop(Integer)
*/
public Builder faceTop(Integer faceTop){
TEMPLATE.get().setFaceTop(faceTop);
return this;
}
/**
* fill the field : fl_face.face_width
* @param faceWidth 人脸位置矩形:width
* @see FlFaceBean#getFaceWidth()
* @see FlFaceBean#setFaceWidth(Integer)
*/
public Builder faceWidth(Integer faceWidth){
TEMPLATE.get().setFaceWidth(faceWidth);
return this;
}
/**
* fill the field : fl_face.face_height
* @param faceHeight 人脸位置矩形:height
* @see FlFaceBean#getFaceHeight()
* @see FlFaceBean#setFaceHeight(Integer)
*/
public Builder faceHeight(Integer faceHeight){
TEMPLATE.get().setFaceHeight(faceHeight);
return this;
}
/**
* fill the field : fl_face.eye_leftx
* @param eyeLeftx 左眼位置:x
* @see FlFaceBean#getEyeLeftx()
* @see FlFaceBean#setEyeLeftx(Integer)
*/
public Builder eyeLeftx(Integer eyeLeftx){
TEMPLATE.get().setEyeLeftx(eyeLeftx);
return this;
}
/**
* fill the field : fl_face.eye_lefty
* @param eyeLefty 左眼位置:y
* @see FlFaceBean#getEyeLefty()
* @see FlFaceBean#setEyeLefty(Integer)
*/
public Builder eyeLefty(Integer eyeLefty){
TEMPLATE.get().setEyeLefty(eyeLefty);
return this;
}
/**
* fill the field : fl_face.eye_rightx
* @param eyeRightx 右眼位置:x
* @see FlFaceBean#getEyeRightx()
* @see FlFaceBean#setEyeRightx(Integer)
*/
public Builder eyeRightx(Integer eyeRightx){
TEMPLATE.get().setEyeRightx(eyeRightx);
return this;
}
/**
* fill the field : fl_face.eye_righty
* @param eyeRighty 右眼位置:y
* @see FlFaceBean#getEyeRighty()
* @see FlFaceBean#setEyeRighty(Integer)
*/
public Builder eyeRighty(Integer eyeRighty){
TEMPLATE.get().setEyeRighty(eyeRighty);
return this;
}
/**
* fill the field : fl_face.mouth_x
* @param mouthX 嘴巴位置:x
* @see FlFaceBean#getMouthX()
* @see FlFaceBean#setMouthX(Integer)
*/
public Builder mouthX(Integer mouthX){
TEMPLATE.get().setMouthX(mouthX);
return this;
}
/**
* fill the field : fl_face.mouth_y
* @param mouthY 嘴巴位置:y
* @see FlFaceBean#getMouthY()
* @see FlFaceBean#setMouthY(Integer)
*/
public Builder mouthY(Integer mouthY){
TEMPLATE.get().setMouthY(mouthY);
return this;
}
/**
* fill the field : fl_face.nose_x
* @param noseX 鼻子位置:x
* @see FlFaceBean#getNoseX()
* @see FlFaceBean#setNoseX(Integer)
*/
public Builder noseX(Integer noseX){
TEMPLATE.get().setNoseX(noseX);
return this;
}
/**
* fill the field : fl_face.nose_y
* @param noseY 鼻子位置:y
* @see FlFaceBean#getNoseY()
* @see FlFaceBean#setNoseY(Integer)
*/
public Builder noseY(Integer noseY){
TEMPLATE.get().setNoseY(noseY);
return this;
}
/**
* fill the field : fl_face.angle_yaw
* @param angleYaw 人脸姿态:偏航角
* @see FlFaceBean#getAngleYaw()
* @see FlFaceBean#setAngleYaw(Integer)
*/
public Builder angleYaw(Integer angleYaw){
TEMPLATE.get().setAngleYaw(angleYaw);
return this;
}
/**
* fill the field : fl_face.angle_pitch
* @param anglePitch 人脸姿态:俯仰角
* @see FlFaceBean#getAnglePitch()
* @see FlFaceBean#setAnglePitch(Integer)
*/
public Builder anglePitch(Integer anglePitch){
TEMPLATE.get().setAnglePitch(anglePitch);
return this;
}
/**
* fill the field : fl_face.angle_roll
* @param angleRoll 人脸姿态:滚转角
* @see FlFaceBean#getAngleRoll()
* @see FlFaceBean#setAngleRoll(Integer)
*/
public Builder angleRoll(Integer angleRoll){
TEMPLATE.get().setAngleRoll(angleRoll);
return this;
}
/**
* fill the field : fl_face.ext_info
* @param extInfo 扩展字段,保存人脸检测基本信息之外的其他数据,内容由SDK负责解析
* @see FlFaceBean#getExtInfo()
* @see FlFaceBean#setExtInfo(java.nio.ByteBuffer)
*/
public Builder extInfo(java.nio.ByteBuffer extInfo){
TEMPLATE.get().setExtInfo(extInfo);
return this;
}
/**
* fill the field : fl_face.feature_md5
* @param featureMd5 外键,人脸特征数据MD5 id
* @see FlFaceBean#getFeatureMd5()
* @see FlFaceBean#setFeatureMd5(String)
*/
public Builder featureMd5(String featureMd5){
TEMPLATE.get().setFeatureMd5(featureMd5);
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy