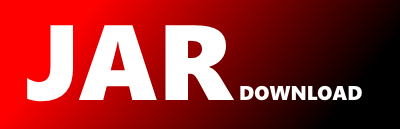
net.gdface.facelog.dborm.face.FlFeatureManager Maven / Gradle / Ivy
// ______________________________________________________
// Generated by sql2java - https://github.com/10km/sql2java-2-6-7 (custom branch)
// modified by guyadong from
// sql2java original version https://sourceforge.net/projects/sql2java/
// JDBC driver used at code generation time: com.mysql.jdbc.Driver
// template: manager.java.vm
// ______________________________________________________
package net.gdface.facelog.dborm.face;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.sql.Types;
import java.util.List;
import java.util.concurrent.Callable;
import java.util.concurrent.atomic.AtomicInteger;
import net.gdface.facelog.dborm.Constant;
import net.gdface.facelog.dborm.Manager;
import net.gdface.facelog.dborm.TableListener;
import net.gdface.facelog.dborm.TableManager;
import net.gdface.facelog.dborm.exception.DaoException;
import net.gdface.facelog.dborm.exception.DataAccessException;
import net.gdface.facelog.dborm.exception.DataRetrievalException;
import net.gdface.facelog.dborm.exception.ObjectRetrievalException;
import net.gdface.facelog.dborm.log.FlLogBean;
import net.gdface.facelog.dborm.log.FlLogManager;
import net.gdface.facelog.dborm.person.FlPersonBean;
import net.gdface.facelog.dborm.person.FlPersonManager;
/**
* Handles database calls (save, load, count, etc...) for the fl_feature table.
* Remarks: 用于验证身份的人脸特征数据表
* @author sql2java
*/
public class FlFeatureManager extends TableManager.BaseAdapter
{
/**
* Tablename.
*/
public static final String TABLE_NAME="fl_feature";
/**
* Contains all the primary key fields of the fl_feature table.
*/
public static final String[] PRIMARYKEY_NAMES =
{
"md5"
};
@Override
public String getTableName() {
return TABLE_NAME;
}
@Override
public String getFields() {
return FL_FEATURE_FIELDS;
}
@Override
public String getFullFields() {
return FL_FEATURE_FULL_FIELDS;
}
@Override
public String[] getPrimarykeyNames() {
return PRIMARYKEY_NAMES;
}
private static FlFeatureManager singleton = new FlFeatureManager();
protected FlFeatureManager(){}
/**
* Get the FlFeatureManager singleton.
*
* @return FlFeatureManager
*/
public static FlFeatureManager getInstance()
{
return singleton;
}
/**
* Creates a new FlFeatureBean instance.
*
* @return the new FlFeatureBean
*/
public FlFeatureBean createBean()
{
return new FlFeatureBean();
}
@Override
protected Class beanType(){
return FlFeatureBean.class;
}
protected FlFaceManager instanceOfFlFaceManager(){
return FlFaceManager.getInstance();
}
protected FlLogManager instanceOfFlLogManager(){
return FlLogManager.getInstance();
}
protected FlPersonManager instanceOfFlPersonManager(){
return FlPersonManager.getInstance();
}
//////////////////////////////////////
// PRIMARY KEY METHODS
//////////////////////////////////////
//1
/**
* Loads a {@link FlFeatureBean} from the fl_feature using primary key fields.
*
* @param md5 String - PK# 1
* @return a unique FlFeatureBean or {@code null} if not found or have null argument
* @throws DaoException
*/
public FlFeatureBean loadByPrimaryKey(String md5) throws DaoException
{
try{
return loadByPrimaryKeyChecked(md5);
}catch(ObjectRetrievalException e){
// not found
return null;
}
}
//1.1
/**
* Loads a {@link FlFeatureBean} from the fl_feature using primary key fields.
*
* @param md5 String - PK# 1
* @return a unique FlFeatureBean
* @throws ObjectRetrievalException if not found
* @throws DaoException
*/
@SuppressWarnings("unused")
public FlFeatureBean loadByPrimaryKeyChecked(String md5) throws DaoException
{
if(null == md5){
throw new ObjectRetrievalException(new NullPointerException());
}
Connection c = null;
PreparedStatement ps = null;
try
{
c = this.getConnection();
StringBuilder sql = new StringBuilder("SELECT " + FL_FEATURE_FIELDS + " FROM fl_feature WHERE md5=?");
// System.out.println("loadByPrimaryKey: " + sql);
ps = c.prepareStatement(sql.toString(),
ResultSet.TYPE_SCROLL_INSENSITIVE,
ResultSet.CONCUR_READ_ONLY);
if (md5 == null) { ps.setNull(FL_FEATURE_ID_MD5 + 1, Types.CHAR); } else { ps.setString(FL_FEATURE_ID_MD5 + 1, md5); }
List pReturn = this.loadByPreparedStatementAsList(ps);
if (1 == pReturn.size()) {
return pReturn.get(0);
} else {
throw new ObjectRetrievalException();
}
}
catch(ObjectRetrievalException e)
{
throw e;
}
catch(SQLException e)
{
throw new DataRetrievalException(e);
}
finally
{
this.getManager().close(ps);
this.freeConnection(c);
}
}
//1.2
@Override
public FlFeatureBean loadByPrimaryKey(FlFeatureBean bean) throws DaoException
{
return bean==null?null:loadByPrimaryKey(bean.getMd5());
}
//1.2.2
@Override
public FlFeatureBean loadByPrimaryKeyChecked(FlFeatureBean bean) throws DaoException
{
if(null == bean){
throw new NullPointerException();
}
return loadByPrimaryKeyChecked(bean.getMd5());
}
//1.3
/**
* Loads a {@link FlFeatureBean} from the fl_feature using primary key fields.
* @param keys primary keys value:
* @return a unique {@link FlFeatureBean} or {@code null} if not found
* @see #loadByPrimaryKey(String md5)
*/
@Override
public FlFeatureBean loadByPrimaryKey(Object ...keys) throws DaoException{
if(null == keys){
throw new NullPointerException();
}
if(keys.length != FL_FEATURE_PK_COUNT){
throw new IllegalArgumentException("argument number mismatch with primary key number");
}
if(null == keys[0]){
return null;
}
return loadByPrimaryKey((String)keys[0]);
}
//1.3.2
@Override
public FlFeatureBean loadByPrimaryKeyChecked(Object ...keys) throws DaoException{
if(null == keys){
throw new NullPointerException();
}
if(keys.length != FL_FEATURE_PK_COUNT){
throw new IllegalArgumentException("argument number mismatch with primary key number");
}
if(! (keys[0] instanceof String)){
throw new IllegalArgumentException("invalid type for the No.1 argument,expected type:String");
}
return loadByPrimaryKeyChecked((String)keys[0]);
}
//1.4
/**
* Returns true if this fl_feature contains row with primary key fields.
* @param md5 String - PK# 1
* @throws DaoException
*/
@SuppressWarnings("unused")
public boolean existsPrimaryKey(String md5) throws DaoException
{
if(null == md5){
return false;
}
Connection c = null;
PreparedStatement ps = null;
try{
c = this.getConnection();
StringBuilder sql = new StringBuilder("SELECT COUNT(*) AS MCOUNT FROM fl_feature WHERE md5=?");
// System.out.println("loadByPrimaryKey: " + sql);
ps = c.prepareStatement(sql.toString(),
ResultSet.TYPE_SCROLL_INSENSITIVE,
ResultSet.CONCUR_READ_ONLY);
if (md5 == null) { ps.setNull(FL_FEATURE_ID_MD5 + 1, Types.CHAR); } else { ps.setString(FL_FEATURE_ID_MD5 + 1, md5); }
return 1 == this.countByPreparedStatement(ps);
}catch(SQLException e){
throw new ObjectRetrievalException(e);
}finally{
this.getManager().close(ps);
this.freeConnection(c);
}
}
//1.6
/**
* Return true if this fl_feature contains row with primary key fields.
* @param bean
* @throws DaoException
* @return false if primary kes has null
*/
@Override
public boolean existsByPrimaryKey(FlFeatureBean bean) throws DaoException
{
if(null == bean || null == bean.getMd5()){
return false;
}
int modified = bean.getModified();
try{
bean.resetModifiedExceptPrimaryKeys();
return 1 == countUsingTemplate(bean);
}finally{
bean.setModified(modified);
}
}
//1.7
@Override
public FlFeatureBean checkDuplicate(FlFeatureBean bean) throws DaoException{
if(existsByPrimaryKey(bean)){
throw new ObjectRetrievalException("Duplicate entry ("+ bean.getMd5() +") for key 'PRIMARY'");
}
return bean;
}
//1.4.1
/**
* Check duplicated row by primary keys,if row exists throw {@link ObjectRetrievalException}
* @param md5 String
* @throws DaoException
* @see #existsPrimaryKey(String md5)
*/
public String checkDuplicate(String md5) throws DaoException
{
if(existsPrimaryKey(md5)){
throw new ObjectRetrievalException("Duplicate entry '"+ md5 +"' for key 'PRIMARY'");
}
return md5;
}
//2
/**
* Delete row according to its primary keys.
* all keys must not be null
*
* @param md5 String - PK# 1
* @return the number of deleted rows
* @throws DaoException
* @see #delete(FlFeatureBean)
*/
public int deleteByPrimaryKey(String md5) throws DaoException
{
FlFeatureBean bean=createBean();
bean.setMd5(md5);
return this.delete(bean);
}
//2.2
/**
* Delete row according to primary keys of bean.
*
* @param bean will be deleted ,all keys must not be null
* @return the number of deleted rows,0 returned if bean is null
* @throws DaoException
*/
@Override
public int delete(FlFeatureBean bean) throws DaoException
{
if(null == bean || null == bean.getMd5()){
return 0;
}
Connection c = null;
PreparedStatement ps = null;
try
{
// listener callback
this.listenerContainer.beforeDelete(bean);
c = this.getConnection();
StringBuilder sql = new StringBuilder("DELETE FROM fl_feature WHERE md5=?");
// System.out.println("deleteByPrimaryKey: " + sql);
ps = c.prepareStatement(sql.toString(),
ResultSet.TYPE_SCROLL_INSENSITIVE,
ResultSet.CONCUR_READ_ONLY);
if (bean.getMd5() == null) { ps.setNull(FL_FEATURE_ID_MD5 + 1, Types.CHAR); } else { ps.setString(FL_FEATURE_ID_MD5 + 1, bean.getMd5()); }
int rows=ps.executeUpdate();
if(rows>0){
// listener callback
this.listenerContainer.afterDelete(bean);
}
return rows;
}
catch(SQLException e)
{
throw new DataAccessException(e);
}
finally
{
// listener callback
this.listenerContainer.done();
this.getManager().close(ps);
this.freeConnection(c);
}
}
//2.1
/**
* Delete row according to its primary keys.
*
* @param keys primary keys value:
* @return the number of deleted rows
* @see #delete(FlFeatureBean)
*/
@Override
public int deleteByPrimaryKey(Object ...keys) throws DaoException{
if(null == keys){
throw new NullPointerException();
}
if(keys.length != FL_FEATURE_PK_COUNT){
throw new IllegalArgumentException("argument number mismatch with primary key number");
}
FlFeatureBean bean = createBean();
if(null != keys[0] && !(keys[0] instanceof String)){
throw new IllegalArgumentException("invalid type for the No.1 argument,expected type:String");
}
bean.setMd5((String)keys[0]);
return delete(bean);
}
//////////////////////////////////////
// IMPORT KEY GENERIC METHOD
//////////////////////////////////////
private static final Class>[] IMPORTED_BEAN_TYPES = new Class>[]{FlFaceBean.class,FlLogBean.class};
/**
* @see #getImportedBeansAsList(FlFeatureBean,int)
*/
@SuppressWarnings("unchecked")
@Override
public > T[] getImportedBeans(FlFeatureBean bean, int ikIndex) throws DaoException {
return getImportedBeansAsList(bean, ikIndex).toArray((T[])java.lang.reflect.Array.newInstance(IMPORTED_BEAN_TYPES[ikIndex],0));
}
/**
* Retrieves imported T objects by ikIndex.
* @param
*
* - {@link Constant#FL_FEATURE_IK_FL_FACE_FEATURE_MD5} - {@link FlFaceBean}
* - {@link Constant#FL_FEATURE_IK_FL_LOG_VERIFY_FEATURE} - {@link FlLogBean}
*
* @param bean the {@link FlFeatureBean} object to use
* @param ikIndex valid values: {@link Constant#FL_FEATURE_IK_FL_FACE_FEATURE_MD5},{@link Constant#FL_FEATURE_IK_FL_LOG_VERIFY_FEATURE}
* @return the associated T beans or {@code null} if {@code bean} is {@code null}
* @throws DaoException
*/
@SuppressWarnings("unchecked")
@Override
public > List getImportedBeansAsList(FlFeatureBean bean,int ikIndex)throws DaoException{
switch(ikIndex){
case FL_FEATURE_IK_FL_FACE_FEATURE_MD5:
return (List)this.getFaceBeansByFeatureMd5AsList(bean);
case FL_FEATURE_IK_FL_LOG_VERIFY_FEATURE:
return (List)this.getLogBeansByVerifyFeatureAsList(bean);
default:
throw new IllegalArgumentException(String.format("invalid ikIndex %d", ikIndex));
}
}
/**
* Set the T objects as imported beans of bean object by ikIndex.
* @param see also {@link #getImportedBeansAsList(FlFeatureBean,int)}
* @param bean the {@link FlFeatureBean} object to use
* @param importedBeans the FlLogBean array to associate to the {@link FlFeatureBean}
* @param ikIndex valid values: see also {@link #getImportedBeansAsList(FlFeatureBean,int)}
* @return importedBeans always
* @throws DaoException
*/
@SuppressWarnings("unchecked")
@Override
public > T[] setImportedBeans(FlFeatureBean bean,T[] importedBeans,int ikIndex)throws DaoException{
switch(ikIndex){
case FL_FEATURE_IK_FL_FACE_FEATURE_MD5:
return (T[])setFaceBeansByFeatureMd5(bean,(FlFaceBean[])importedBeans);
case FL_FEATURE_IK_FL_LOG_VERIFY_FEATURE:
return (T[])setLogBeansByVerifyFeature(bean,(FlLogBean[])importedBeans);
default:
throw new IllegalArgumentException(String.format("invalid ikIndex %d", ikIndex));
}
}
/**
* Set the importedBeans associates to the bean by ikIndex
* @param see also {@link #getImportedBeansAsList(FlFeatureBean,int)}
* @param bean the {@link FlFeatureBean} object to use
* @param importedBeans the T object to associate to the {@link FlFeatureBean}
* @param ikIndex valid values: see also {@link #getImportedBeansAsList(FlFeatureBean,int)}
* @return importedBeans always
* @throws DaoException
*/
@SuppressWarnings("unchecked")
@Override
public ,C extends java.util.Collection> C setImportedBeans(FlFeatureBean bean,C importedBeans,int ikIndex)throws DaoException{
switch(ikIndex){
case FL_FEATURE_IK_FL_FACE_FEATURE_MD5:
return (C)setFaceBeansByFeatureMd5(bean,(java.util.Collection)importedBeans);
case FL_FEATURE_IK_FL_LOG_VERIFY_FEATURE:
return (C)setLogBeansByVerifyFeature(bean,(java.util.Collection)importedBeans);
default:
throw new IllegalArgumentException(String.format("invalid ikIndex %d", ikIndex));
}
}
//////////////////////////////////////
// GET/SET IMPORTED KEY BEAN METHOD
//////////////////////////////////////
//3.1 GET IMPORTED
/**
* Retrieves the {@link FlFaceBean} object from the fl_face.feature_md5 field.
* FK_NAME : fl_face_ibfk_2
* @param bean the {@link FlFeatureBean}
* @return the associated {@link FlFaceBean} beans or {@code null} if {@code bean} is {@code null}
* @throws DaoException
*/
public FlFaceBean[] getFaceBeansByFeatureMd5(FlFeatureBean bean) throws DaoException
{
return getFaceBeansByFeatureMd5AsList(bean).toArray(new FlFaceBean[0]);
}
//3.1.2 GET IMPORTED
/**
* Retrieves the {@link FlFaceBean} object from the fl_face.feature_md5 field.
* FK_NAME : fl_face_ibfk_2
* @param md5OfFeature String - PK# 1
* @return the associated {@link FlFaceBean} beans or {@code null} if {@code bean} is {@code null}
* @throws DaoException
*/
public FlFaceBean[] getFaceBeansByFeatureMd5(String md5OfFeature) throws DaoException
{
FlFeatureBean bean = createBean();
bean.setMd5(md5OfFeature);
return getFaceBeansByFeatureMd5(bean);
}
//3.2 GET IMPORTED
/**
* Retrieves the {@link FlFaceBean} object from fl_face.feature_md5 field.
* FK_NAME:fl_face_ibfk_2
* @param bean the {@link FlFeatureBean}
* @return the associated {@link FlFaceBean} beans
* @throws DaoException
*/
public List getFaceBeansByFeatureMd5AsList(FlFeatureBean bean) throws DaoException
{
return getFaceBeansByFeatureMd5AsList(bean,1,-1);
}
//3.2.2 GET IMPORTED
/**
* Retrieves the {@link FlFaceBean} object from fl_face.feature_md5 field.
* FK_NAME:fl_face_ibfk_2
* @param md5OfFeature String - PK# 1
* @return the associated {@link FlFaceBean} beans
* @throws DaoException
*/
public List getFaceBeansByFeatureMd5AsList(String md5OfFeature) throws DaoException
{
FlFeatureBean bean = createBean();
bean.setMd5(md5OfFeature);
return getFaceBeansByFeatureMd5AsList(bean);
}
//3.2.4 GET IMPORTED
/**
* Retrieves the {@link FlFaceBean} object from fl_face.feature_md5 field,
* given the start row and number of rows.
* FK_NAME:fl_face_ibfk_2
* @param bean the {@link FlFeatureBean}
* @param startRow the start row to be used (first row = 1, last row=-1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @return the associated {@link FlFaceBean} beans
* @throws DaoException
*/
public List getFaceBeansByFeatureMd5AsList(FlFeatureBean bean,int startRow, int numRows) throws DaoException
{
if(null == bean){
return new java.util.ArrayList();
}
FlFaceBean other = new FlFaceBean();
other.setFeatureMd5(bean.getMd5());
return instanceOfFlFaceManager().loadUsingTemplateAsList(other,startRow,numRows);
}
//3.3 SET IMPORTED
/**
* set the {@link FlFaceBean} object array associate to FlFeatureBean by the fl_face.feature_md5 field.
* FK_NAME : fl_face_ibfk_2
* @param bean the referenced {@link FlFeatureBean}
* @param importedBeans imported beans from fl_face
* @return importedBeans always
* @throws DaoException
* @see FlFaceManager#setReferencedByFeatureMd5(FlFaceBean, FlFeatureBean)
*/
public FlFaceBean[] setFaceBeansByFeatureMd5(FlFeatureBean bean , FlFaceBean[] importedBeans) throws DaoException
{
if(null != importedBeans){
for( FlFaceBean importBean : importedBeans ){
instanceOfFlFaceManager().setReferencedByFeatureMd5(importBean , bean);
}
}
return importedBeans;
}
//3.4 SET IMPORTED
/**
* set the {@link FlFaceBean} object collection associate to FlFeatureBean by the fl_face.feature_md5 field.
* FK_NAME:fl_face_ibfk_2
* @param bean the referenced {@link FlFeatureBean}
* @param importedBeans imported beans from fl_face
* @return importedBeans always
* @throws DaoException
* @see FlFaceManager#setReferencedByFeatureMd5(FlFaceBean, FlFeatureBean)
*/
public > C setFaceBeansByFeatureMd5(FlFeatureBean bean , C importedBeans) throws DaoException
{
if(null != importedBeans){
for( FlFaceBean importBean : importedBeans ){
instanceOfFlFaceManager().setReferencedByFeatureMd5(importBean , bean);
}
}
return importedBeans;
}
//3.1 GET IMPORTED
/**
* Retrieves the {@link FlLogBean} object from the fl_log.verify_feature field.
* FK_NAME : fl_log_ibfk_3
* @param bean the {@link FlFeatureBean}
* @return the associated {@link FlLogBean} beans or {@code null} if {@code bean} is {@code null}
* @throws DaoException
*/
public FlLogBean[] getLogBeansByVerifyFeature(FlFeatureBean bean) throws DaoException
{
return getLogBeansByVerifyFeatureAsList(bean).toArray(new FlLogBean[0]);
}
//3.1.2 GET IMPORTED
/**
* Retrieves the {@link FlLogBean} object from the fl_log.verify_feature field.
* FK_NAME : fl_log_ibfk_3
* @param md5OfFeature String - PK# 1
* @return the associated {@link FlLogBean} beans or {@code null} if {@code bean} is {@code null}
* @throws DaoException
*/
public FlLogBean[] getLogBeansByVerifyFeature(String md5OfFeature) throws DaoException
{
FlFeatureBean bean = createBean();
bean.setMd5(md5OfFeature);
return getLogBeansByVerifyFeature(bean);
}
//3.2 GET IMPORTED
/**
* Retrieves the {@link FlLogBean} object from fl_log.verify_feature field.
* FK_NAME:fl_log_ibfk_3
* @param bean the {@link FlFeatureBean}
* @return the associated {@link FlLogBean} beans
* @throws DaoException
*/
public List getLogBeansByVerifyFeatureAsList(FlFeatureBean bean) throws DaoException
{
return getLogBeansByVerifyFeatureAsList(bean,1,-1);
}
//3.2.2 GET IMPORTED
/**
* Retrieves the {@link FlLogBean} object from fl_log.verify_feature field.
* FK_NAME:fl_log_ibfk_3
* @param md5OfFeature String - PK# 1
* @return the associated {@link FlLogBean} beans
* @throws DaoException
*/
public List getLogBeansByVerifyFeatureAsList(String md5OfFeature) throws DaoException
{
FlFeatureBean bean = createBean();
bean.setMd5(md5OfFeature);
return getLogBeansByVerifyFeatureAsList(bean);
}
//3.2.4 GET IMPORTED
/**
* Retrieves the {@link FlLogBean} object from fl_log.verify_feature field,
* given the start row and number of rows.
* FK_NAME:fl_log_ibfk_3
* @param bean the {@link FlFeatureBean}
* @param startRow the start row to be used (first row = 1, last row=-1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @return the associated {@link FlLogBean} beans
* @throws DaoException
*/
public List getLogBeansByVerifyFeatureAsList(FlFeatureBean bean,int startRow, int numRows) throws DaoException
{
if(null == bean){
return new java.util.ArrayList();
}
FlLogBean other = new FlLogBean();
other.setVerifyFeature(bean.getMd5());
return instanceOfFlLogManager().loadUsingTemplateAsList(other,startRow,numRows);
}
//3.3 SET IMPORTED
/**
* set the {@link FlLogBean} object array associate to FlFeatureBean by the fl_log.verify_feature field.
* FK_NAME : fl_log_ibfk_3
* @param bean the referenced {@link FlFeatureBean}
* @param importedBeans imported beans from fl_log
* @return importedBeans always
* @throws DaoException
* @see FlLogManager#setReferencedByVerifyFeature(FlLogBean, FlFeatureBean)
*/
public FlLogBean[] setLogBeansByVerifyFeature(FlFeatureBean bean , FlLogBean[] importedBeans) throws DaoException
{
if(null != importedBeans){
for( FlLogBean importBean : importedBeans ){
instanceOfFlLogManager().setReferencedByVerifyFeature(importBean , bean);
}
}
return importedBeans;
}
//3.4 SET IMPORTED
/**
* set the {@link FlLogBean} object collection associate to FlFeatureBean by the fl_log.verify_feature field.
* FK_NAME:fl_log_ibfk_3
* @param bean the referenced {@link FlFeatureBean}
* @param importedBeans imported beans from fl_log
* @return importedBeans always
* @throws DaoException
* @see FlLogManager#setReferencedByVerifyFeature(FlLogBean, FlFeatureBean)
*/
public > C setLogBeansByVerifyFeature(FlFeatureBean bean , C importedBeans) throws DaoException
{
if(null != importedBeans){
for( FlLogBean importBean : importedBeans ){
instanceOfFlLogManager().setReferencedByVerifyFeature(importBean , bean);
}
}
return importedBeans;
}
//3.5 SYNC SAVE
/**
* Save the FlFeatureBean bean and referenced beans and imported beans into the database.
*
* @param bean the {@link FlFeatureBean} bean to be saved
* @param refPersonByPersonId the {@link FlPersonBean} bean referenced by {@link FlFeatureBean}
* @param impFaceByFeatureMd5 the {@link FlFaceBean} beans refer to {@link FlFeatureBean}
* @param impLogByVerifyFeature the {@link FlLogBean} beans refer to {@link FlFeatureBean}
* @return the inserted or updated {@link FlFeatureBean} bean
* @throws DaoException
*/
public FlFeatureBean save(FlFeatureBean bean
, FlPersonBean refPersonByPersonId
, FlFaceBean[] impFaceByFeatureMd5 , FlLogBean[] impLogByVerifyFeature ) throws DaoException
{
if(null == bean) {
return null;
}
if(null != refPersonByPersonId){
this.setReferencedByPersonId(bean,refPersonByPersonId);
}
bean = this.save( bean );
if(null != impFaceByFeatureMd5){
this.setFaceBeansByFeatureMd5(bean,impFaceByFeatureMd5);
instanceOfFlFaceManager().save( impFaceByFeatureMd5 );
}
if(null != impLogByVerifyFeature){
this.setLogBeansByVerifyFeature(bean,impLogByVerifyFeature);
instanceOfFlLogManager().save( impLogByVerifyFeature );
}
return bean;
}
//3.6 SYNC SAVE AS TRANSACTION
/**
* Transaction version for sync save
* @see #save(FlFeatureBean , FlPersonBean , FlFaceBean[] , FlLogBean[] )
*/
public FlFeatureBean saveAsTransaction(final FlFeatureBean bean
,final FlPersonBean refPersonByPersonId
,final FlFaceBean[] impFaceByFeatureMd5 ,final FlLogBean[] impLogByVerifyFeature ) throws DaoException
{
return this.runAsTransaction(new Callable(){
@Override
public FlFeatureBean call() throws Exception {
return save(bean , refPersonByPersonId , impFaceByFeatureMd5 , impLogByVerifyFeature );
}});
}
//3.7 SYNC SAVE
/**
* Save the FlFeatureBean bean and referenced beans and imported beans into the database.
*
* @param bean the {@link FlFeatureBean} bean to be saved
* @param refPersonByPersonId the {@link FlPersonBean} bean referenced by {@link FlFeatureBean}
* @param impFaceByFeatureMd5 the {@link FlFaceBean} bean refer to {@link FlFeatureBean}
* @param impLogByVerifyFeature the {@link FlLogBean} bean refer to {@link FlFeatureBean}
* @return the inserted or updated {@link FlFeatureBean} bean
* @throws DaoException
*/
public FlFeatureBean save(FlFeatureBean bean
, FlPersonBean refPersonByPersonId
, java.util.Collection impFaceByFeatureMd5 , java.util.Collection impLogByVerifyFeature ) throws DaoException
{
if(null == bean) {
return null;
}
if(null != refPersonByPersonId){
this.setReferencedByPersonId(bean,refPersonByPersonId);
}
bean = this.save( bean );
if(null != impFaceByFeatureMd5){
this.setFaceBeansByFeatureMd5(bean,impFaceByFeatureMd5);
instanceOfFlFaceManager().save( impFaceByFeatureMd5 );
}
if(null != impLogByVerifyFeature){
this.setLogBeansByVerifyFeature(bean,impLogByVerifyFeature);
instanceOfFlLogManager().save( impLogByVerifyFeature );
}
return bean;
}
//3.8 SYNC SAVE AS TRANSACTION
/**
* Transaction version for sync save
* @see #save(FlFeatureBean , FlPersonBean , java.util.Collection , java.util.Collection )
* @throws DaoException
*/
public FlFeatureBean saveAsTransaction(final FlFeatureBean bean
,final FlPersonBean refPersonByPersonId
,final java.util.Collection impFaceByFeatureMd5 ,final java.util.Collection impLogByVerifyFeature ) throws DaoException
{
return this.runAsTransaction(new Callable(){
@Override
public FlFeatureBean call() throws Exception {
return save(bean , refPersonByPersonId , impFaceByFeatureMd5 , impLogByVerifyFeature );
}});
}
private static final int SYNC_SAVE_ARG_LEN = 3;
private static final int SYNC_SAVE_ARG_0 = 0;
private static final int SYNC_SAVE_ARG_1 = 1;
private static final int SYNC_SAVE_ARG_2 = 2;
//3.9 SYNC SAVE
/**
* Save the FlFeatureBean bean and referenced beans and imported beans (array) into the database.
*
* @param bean the {@link FlFeatureBean} bean to be saved
* @param inputs referenced beans or imported beans
* see also {@link #save(FlFeatureBean , FlPersonBean , FlFaceBean[] , FlLogBean[] )}
* @return the inserted or updated {@link FlFeatureBean} bean
* @throws DaoException
*/
@Override
public FlFeatureBean save(FlFeatureBean bean,Object ...inputs) throws DaoException
{
if(null == inputs){
return save(bean);
}
if(inputs.length > SYNC_SAVE_ARG_LEN){
throw new IllegalArgumentException("too many dynamic arguments,max dynamic arguments number: 3");
}
Object[] args = new Object[SYNC_SAVE_ARG_LEN];
System.arraycopy(inputs, 0, args, 0, inputs.length);
if( null != args[SYNC_SAVE_ARG_0] && !(args[SYNC_SAVE_ARG_0] instanceof FlPersonBean)){
throw new IllegalArgumentException("invalid type for the No.1 dynamic argument,expected type:FlPersonBean");
}
if( null != args[SYNC_SAVE_ARG_1] && !(args[SYNC_SAVE_ARG_1] instanceof FlFaceBean[])){
throw new IllegalArgumentException("invalid type for the No.2 dynamic argument,expected type:FlFaceBean[]");
}
if( null != args[SYNC_SAVE_ARG_2] && !(args[SYNC_SAVE_ARG_2] instanceof FlLogBean[])){
throw new IllegalArgumentException("invalid type for the No.3 dynamic argument,expected type:FlLogBean[]");
}
return save(bean,
(FlPersonBean)args[SYNC_SAVE_ARG_0],
(FlFaceBean[])args[SYNC_SAVE_ARG_1],
(FlLogBean[])args[SYNC_SAVE_ARG_2]);
}
//3.10 SYNC SAVE
/**
* Save the FlFeatureBean bean and referenced beans and imported beans (collection) into the database.
*
* @param bean the {@link FlFeatureBean} bean to be saved
* @param inputs referenced beans or imported beans
* see also {@link #save(FlFeatureBean , FlPersonBean , java.util.Collection , java.util.Collection )}
* @return the inserted or updated {@link FlFeatureBean} bean
* @throws DaoException
*/
@SuppressWarnings("unchecked")
@Override
public FlFeatureBean saveCollection(FlFeatureBean bean,Object ...inputs) throws DaoException
{
if(null == inputs){
return save(bean);
}
if(inputs.length > SYNC_SAVE_ARG_LEN){
throw new IllegalArgumentException("too many dynamic arguments,max dynamic arguments number: 3");
}
Object[] args = new Object[SYNC_SAVE_ARG_LEN];
System.arraycopy(inputs, 0, args, 0, inputs.length);
if( null != args[SYNC_SAVE_ARG_0] && !(args[SYNC_SAVE_ARG_0] instanceof FlPersonBean)){
throw new IllegalArgumentException("invalid type for the No.1 argument,expected type:FlPersonBean");
}
if( null != args[SYNC_SAVE_ARG_1] && !(args[SYNC_SAVE_ARG_1] instanceof java.util.Collection)){
throw new IllegalArgumentException("invalid type for the No.2 argument,expected type:java.util.Collection");
}
if( null != args[SYNC_SAVE_ARG_2] && !(args[SYNC_SAVE_ARG_2] instanceof java.util.Collection)){
throw new IllegalArgumentException("invalid type for the No.3 argument,expected type:java.util.Collection");
}
return save(bean,
(FlPersonBean)args[SYNC_SAVE_ARG_0],
(java.util.Collection)args[SYNC_SAVE_ARG_1],
(java.util.Collection)args[SYNC_SAVE_ARG_2]);
}
//////////////////////////////////////
// FOREIGN KEY GENERIC METHOD
//////////////////////////////////////
/**
* Retrieves the bean object referenced by fkIndex.
* @param
*
* - {@link Constant#FL_FEATURE_FK_PERSON_ID} - {@link FlPersonBean}
*
* @param bean the {@link FlFeatureBean} object to use
* @param fkIndex valid values:
* {@link Constant#FL_FEATURE_FK_PERSON_ID}
* @return the associated T bean or {@code null} if {@code bean} or {@code beanToSet} is {@code null}
* @throws DaoException
*/
@SuppressWarnings("unchecked")
@Override
public > T getReferencedBean(FlFeatureBean bean,int fkIndex)throws DaoException{
switch(fkIndex){
case FL_FEATURE_FK_PERSON_ID:
return (T)this.getReferencedByPersonId(bean);
default:
throw new IllegalArgumentException(String.format("invalid fkIndex %d", fkIndex));
}
}
/**
* Associates the {@link FlFeatureBean} object to the bean object by fkIndex field.
*
* @param see also {@link #getReferencedBean(FlFeatureBean,int)}
* @param bean the {@link FlFeatureBean} object to use
* @param beanToSet the T object to associate to the {@link FlFeatureBean}
* @param fkIndex valid values: see also {@link #getReferencedBean(FlFeatureBean,int)}
* @return always beanToSet saved
* @throws DaoException
*/
@SuppressWarnings("unchecked")
@Override
public > T setReferencedBean(FlFeatureBean bean,T beanToSet,int fkIndex)throws DaoException{
switch(fkIndex){
case FL_FEATURE_FK_PERSON_ID:
return (T)this.setReferencedByPersonId(bean, (FlPersonBean)beanToSet);
default:
throw new IllegalArgumentException(String.format("invalid fkIndex %d", fkIndex));
}
}
//////////////////////////////////////
// GET/SET FOREIGN KEY BEAN METHOD
//////////////////////////////////////
//5.1 GET REFERENCED VALUE
/**
* Retrieves the {@link FlPersonBean} object referenced by {@link FlFeatureBean#getPersonId}() field.
* FK_NAME : fl_feature_ibfk_1
* @param bean the {@link FlFeatureBean}
* @return the associated {@link FlPersonBean} bean or {@code null} if {@code bean} is {@code null}
* @throws DaoException
*/
public FlPersonBean getReferencedByPersonId(FlFeatureBean bean) throws DaoException
{
if(null == bean){
return null;
}
bean.setReferencedByPersonId(instanceOfFlPersonManager().loadByPrimaryKey(bean.getPersonId()));
return bean.getReferencedByPersonId();
}
//5.2 SET REFERENCED
/**
* Associates the {@link FlFeatureBean} object to the {@link FlPersonBean} object by {@link FlFeatureBean#getPersonId}() field.
*
* @param bean the {@link FlFeatureBean} object to use
* @param beanToSet the {@link FlPersonBean} object to associate to the {@link FlFeatureBean} .
* @return always beanToSet saved
* @throws DaoException
*/
public FlPersonBean setReferencedByPersonId(FlFeatureBean bean, FlPersonBean beanToSet) throws DaoException
{
if(null != bean){
instanceOfFlPersonManager().save(beanToSet);
bean.setReferencedByPersonId(beanToSet);
if( null == beanToSet){
bean.setPersonId(null);
}else{
bean.setPersonId(beanToSet.getId());
}
}
return beanToSet;
}
//////////////////////////////////////
// SQL 'WHERE' METHOD
//////////////////////////////////////
//11
/**
* Deletes rows from the fl_feature table using a 'where' clause.
* It is up to you to pass the 'WHERE' in your where clauses.
*
Attention, if 'WHERE' is omitted it will delete all records.
*
* @param where the sql 'where' clause
* @return the number of deleted rows
* @throws DaoException
*/
@Override
public int deleteByWhere(String where) throws DaoException
{
if( !this.listenerContainer.isEmpty()){
final DeleteBeanAction action = new DeleteBeanAction();
this.loadByWhere(where,action);
return action.getCount();
}
Connection c = null;
PreparedStatement ps = null;
try
{
c = this.getConnection();
StringBuilder sql = new StringBuilder("DELETE FROM fl_feature " + where);
// System.out.println("deleteByWhere: " + sql);
ps = c.prepareStatement(sql.toString());
return ps.executeUpdate();
}
catch(SQLException e)
{
throw new DataAccessException(e);
}
finally
{
this.getManager().close(ps);
this.freeConnection(c);
}
}
//_____________________________________________________________________
//
// SAVE
//_____________________________________________________________________
//13
@Override
public FlFeatureBean insert(FlFeatureBean bean) throws DaoException
{
// mini checks
if (null == bean || !bean.isModified()) {
return bean;
}
if (!bean.isNew()){
return this.update(bean);
}
Connection c = null;
PreparedStatement ps = null;
StringBuilder sql = null;
try
{
c = this.getConnection();
// listener callback
this.listenerContainer.beforeInsert(bean);
int dirtyCount = 0;
sql = new StringBuilder("INSERT into fl_feature (");
if (bean.checkMd5Modified()) {
if (dirtyCount>0) {
sql.append(",");
}
sql.append("md5");
dirtyCount++;
}
if (bean.checkSdkVersionModified()) {
if (dirtyCount>0) {
sql.append(",");
}
sql.append("sdk_version");
dirtyCount++;
}
if (bean.checkPersonIdModified()) {
if (dirtyCount>0) {
sql.append(",");
}
sql.append("person_id");
dirtyCount++;
}
if (bean.checkFeatureModified()) {
if (dirtyCount>0) {
sql.append(",");
}
sql.append("feature");
dirtyCount++;
}
if (bean.checkUpdateTimeModified()) {
if (dirtyCount>0) {
sql.append(",");
}
sql.append("update_time");
dirtyCount++;
}
sql.append(") values (");
if(dirtyCount > 0) {
sql.append("?");
for(int i = 1; i < dirtyCount; i++) {
sql.append(",?");
}
}
sql.append(")");
// System.out.println("insert : " + sql.toString());
ps = c.prepareStatement(sql.toString(), ResultSet.TYPE_SCROLL_INSENSITIVE, ResultSet.CONCUR_READ_ONLY);
this.fillPreparedStatement(ps, bean, SEARCH_EXACT,true);
ps.executeUpdate();
bean.isNew(false);
bean.resetIsModified();
// listener callback
this.listenerContainer.afterInsert(bean);
return bean;
}
catch(SQLException e)
{
throw new DataAccessException(e);
}
finally
{
// listener callback
this.listenerContainer.done();
sql = null;
this.getManager().close(ps);
this.freeConnection(c);
}
}
//14
@Override
public FlFeatureBean update(FlFeatureBean bean) throws DaoException
{
// mini checks
if (null == bean || !bean.isModified()) {
return bean;
}
if (bean.isNew()){
return this.insert(bean);
}
Connection c = null;
PreparedStatement ps = null;
StringBuilder sql = null;
try
{
c = this.getConnection();
// listener callback
this.listenerContainer.beforeUpdate(bean);
sql = new StringBuilder("UPDATE fl_feature SET ");
boolean useComma=false;
if (bean.checkMd5Modified()) {
if (useComma) {
sql.append(", ");
} else {
useComma=true;
}
sql.append("md5=?");
}
if (bean.checkSdkVersionModified()) {
if (useComma) {
sql.append(", ");
} else {
useComma=true;
}
sql.append("sdk_version=?");
}
if (bean.checkPersonIdModified()) {
if (useComma) {
sql.append(", ");
} else {
useComma=true;
}
sql.append("person_id=?");
}
if (bean.checkFeatureModified()) {
if (useComma) {
sql.append(", ");
} else {
useComma=true;
}
sql.append("feature=?");
}
if (bean.checkUpdateTimeModified()) {
if (useComma) {
sql.append(", ");
} else {
useComma=true;
}
sql.append("update_time=?");
}
sql.append(" WHERE ");
sql.append("md5=?");
// System.out.println("update : " + sql.toString());
ps = c.prepareStatement(sql.toString(),
ResultSet.TYPE_SCROLL_INSENSITIVE,
ResultSet.CONCUR_READ_ONLY);
int dirtyCount = this.fillPreparedStatement(ps, bean, SEARCH_EXACT,true);
if (dirtyCount == 0) {
// System.out.println("The bean to look is not initialized... do not update.");
return bean;
}
if (bean.getMd5() == null) { ps.setNull(++dirtyCount, Types.CHAR); } else { ps.setString(++dirtyCount, bean.getMd5()); }
ps.executeUpdate();
// listener callback
this.listenerContainer.afterUpdate(bean);
bean.resetIsModified();
return bean;
}
catch(SQLException e)
{
throw new DataAccessException(e);
}
finally
{
// listener callback
this.listenerContainer.done();
sql = null;
this.getManager().close(ps);
this.freeConnection(c);
}
}
//_____________________________________________________________________
//
// USING TEMPLATE
//_____________________________________________________________________
//18
@Override
public FlFeatureBean loadUniqueUsingTemplate(FlFeatureBean bean) throws DaoException
{
List beans = this.loadUsingTemplateAsList(bean);
switch(beans.size()){
case 0:
return null;
case 1:
return beans.get(0);
default:
throw new ObjectRetrievalException("More than one element !!");
}
}
//18-1
@Override
public FlFeatureBean loadUniqueUsingTemplateChecked(FlFeatureBean bean) throws DaoException
{
List beans = this.loadUsingTemplateAsList(bean);
switch(beans.size()){
case 0:
throw new ObjectRetrievalException("Not found element !!");
case 1:
return beans.get(0);
default:
throw new ObjectRetrievalException("More than one element !!");
}
}
//20-5
@Override
public int loadUsingTemplate(FlFeatureBean bean, int[] fieldList, int startRow, int numRows,int searchType, Action action) throws DaoException
{
// System.out.println("loadUsingTemplate startRow:" + startRow + ", numRows:" + numRows + ", searchType:" + searchType);
StringBuilder sqlWhere = new StringBuilder("");
String sql=createSelectSql(fieldList,this.fillWhere(sqlWhere, bean, searchType) > 0?" WHERE "+sqlWhere.toString():null);
PreparedStatement ps = null;
Connection connection = null;
try {
connection = this.getConnection();
ps = connection.prepareStatement(sql,
ResultSet.TYPE_FORWARD_ONLY,
ResultSet.CONCUR_READ_ONLY);
this.fillPreparedStatement(ps, bean, searchType,false);
return this.loadByPreparedStatement(ps, fieldList, startRow, numRows, action);
} catch (DaoException e) {
throw e;
}catch (SQLException e) {
throw new DataAccessException(e);
} finally {
this.getManager().close(ps);
this.freeConnection(connection);
}
}
//21
@Override
public int deleteUsingTemplate(FlFeatureBean bean) throws DaoException
{
if(bean.checkMd5Initialized() && null != bean.getMd5()){
return this.deleteByPrimaryKey(bean.getMd5());
}
if( !this.listenerContainer.isEmpty()){
final DeleteBeanAction action=new DeleteBeanAction();
this.loadUsingTemplate(bean,action);
return action.getCount();
}
Connection c = null;
PreparedStatement ps = null;
StringBuilder sql = new StringBuilder("DELETE FROM fl_feature ");
StringBuilder sqlWhere = new StringBuilder("");
try
{
if (this.fillWhere(sqlWhere, bean, SEARCH_EXACT) > 0)
{
sql.append(" WHERE ").append(sqlWhere);
}
else
{
// System.out.println("The bean to look is not initialized... deleting all");
}
// System.out.println("deleteUsingTemplate: " + sql.toString());
c = this.getConnection();
ps = c.prepareStatement(sql.toString(),
ResultSet.TYPE_SCROLL_INSENSITIVE,
ResultSet.CONCUR_READ_ONLY);
this.fillPreparedStatement(ps, bean, SEARCH_EXACT, false);
return ps.executeUpdate();
}
catch(SQLException e)
{
throw new DataAccessException(e);
}
finally
{
this.getManager().close(ps);
this.freeConnection(c);
sql = null;
sqlWhere = null;
}
}
//_____________________________________________________________________
//
// USING INDICES
//_____________________________________________________________________
/**
* Retrieves an array of FlFeatureBean using the person_id index.
*
* @param personId the person_id column's value filter.
* @return an array of FlFeatureBean
* @throws DaoException
*/
public FlFeatureBean[] loadByIndexPersonId(Integer personId) throws DaoException
{
return (FlFeatureBean[])this.loadByIndexPersonIdAsList(personId).toArray(new FlFeatureBean[0]);
}
/**
* Retrieves a list of FlFeatureBean using the person_id index.
*
* @param personId the person_id column's value filter.
* @return a list of FlFeatureBean
* @throws DaoException
*/
public List loadByIndexPersonIdAsList(Integer personId) throws DaoException
{
FlFeatureBean bean = this.createBean();
bean.setPersonId(personId);
return loadUsingTemplateAsList(bean);
}
/**
* Deletes rows using the person_id index.
*
* @param personId the person_id column's value filter.
* @return the number of deleted objects
* @throws DaoException
*/
public int deleteByIndexPersonId(Integer personId) throws DaoException
{
FlFeatureBean bean = this.createBean();
bean.setPersonId(personId);
return deleteUsingTemplate(bean);
}
/**
* Retrieves an array of FlFeatureBean using the sdk_version index.
*
* @param sdkVersion the sdk_version column's value filter.
* @return an array of FlFeatureBean
* @throws DaoException
*/
public FlFeatureBean[] loadByIndexSdkVersion(String sdkVersion) throws DaoException
{
return (FlFeatureBean[])this.loadByIndexSdkVersionAsList(sdkVersion).toArray(new FlFeatureBean[0]);
}
/**
* Retrieves a list of FlFeatureBean using the sdk_version index.
*
* @param sdkVersion the sdk_version column's value filter.
* @return a list of FlFeatureBean
* @throws DaoException
*/
public List loadByIndexSdkVersionAsList(String sdkVersion) throws DaoException
{
FlFeatureBean bean = this.createBean();
bean.setSdkVersion(sdkVersion);
return loadUsingTemplateAsList(bean);
}
/**
* Deletes rows using the sdk_version index.
*
* @param sdkVersion the sdk_version column's value filter.
* @return the number of deleted objects
* @throws DaoException
*/
public int deleteByIndexSdkVersion(String sdkVersion) throws DaoException
{
FlFeatureBean bean = this.createBean();
bean.setSdkVersion(sdkVersion);
return deleteUsingTemplate(bean);
}
/**
* Retrieves a list of FlFeatureBean using the index specified by keyIndex.
* @param keyIndex valid values:
* {@link Constant#FL_FEATURE_INDEX_PERSON_ID},{@link Constant#FL_FEATURE_INDEX_SDK_VERSION}
* @param keys key values of index
* @return a list of FlFeatureBean
* @throws DaoException
*/
@Override
public List loadByIndexAsList(int keyIndex,Object ...keys)throws DaoException
{
if(null == keys){
throw new NullPointerException();
}
switch(keyIndex){
case FL_FEATURE_INDEX_PERSON_ID:{
if(keys.length != 1){
throw new IllegalArgumentException("argument number mismatch with index 'person_id' column number");
}
if(null != keys[0] && !(keys[0] instanceof Integer)){
throw new IllegalArgumentException("invalid type for the No.1 argument,expected type:Integer");
}
return this.loadByIndexPersonIdAsList((Integer)keys[0]);
}
case FL_FEATURE_INDEX_SDK_VERSION:{
if(keys.length != 1){
throw new IllegalArgumentException("argument number mismatch with index 'sdk_version' column number");
}
if(null != keys[0] && !(keys[0] instanceof String)){
throw new IllegalArgumentException("invalid type for the No.1 argument,expected type:String");
}
return this.loadByIndexSdkVersionAsList((String)keys[0]);
}
default:
throw new IllegalArgumentException(String.format("invalid keyIndex %d", keyIndex));
}
}
/**
* Deletes rows using key.
* @param keyIndex valid values:
* {@link Constant#FL_FEATURE_INDEX_PERSON_ID},{@link Constant#FL_FEATURE_INDEX_SDK_VERSION}
* @param keys key values of index
* @return the number of deleted objects
* @throws DaoException
*/
@Override
public int deleteByIndex(int keyIndex,Object ...keys)throws DaoException
{
if(null == keys){
throw new NullPointerException();
}
switch(keyIndex){
case FL_FEATURE_INDEX_PERSON_ID:{
if(keys.length != 1){
throw new IllegalArgumentException("argument number mismatch with index 'person_id' column number");
}
if(null != keys[0] && !(keys[0] instanceof Integer)){
throw new IllegalArgumentException("invalid type for the No.1 argument,expected type:Integer");
}
return this.deleteByIndexPersonId((Integer)keys[0]);
}
case FL_FEATURE_INDEX_SDK_VERSION:{
if(keys.length != 1){
throw new IllegalArgumentException("argument number mismatch with index 'sdk_version' column number");
}
if(null != keys[0] && !(keys[0] instanceof String)){
throw new IllegalArgumentException("invalid type for the No.1 argument,expected type:String");
}
return this.deleteByIndexSdkVersion((String)keys[0]);
}
default:
throw new IllegalArgumentException(String.format("invalid keyIndex %d", keyIndex));
}
}
//_____________________________________________________________________
//
// COUNT
//_____________________________________________________________________
//25
@Override
public int countWhere(String where) throws DaoException
{
String sql = new StringBuffer("SELECT COUNT(*) AS MCOUNT FROM fl_feature ")
.append(null == where ? "" : where).toString();
// System.out.println("countWhere: " + sql);
Connection c = null;
Statement st = null;
ResultSet rs = null;
try
{
int iReturn = -1;
c = this.getConnection();
st = c.createStatement();
rs = st.executeQuery(sql);
if (rs.next())
{
iReturn = rs.getInt("MCOUNT");
}
if (iReturn != -1) {
return iReturn;
}
}
catch(SQLException e)
{
throw new DataAccessException(e);
}
finally
{
this.getManager().close(st, rs);
this.freeConnection(c);
sql = null;
}
throw new DataAccessException("Error in countWhere where=[" + where + "]");
}
//26
/**
* Retrieves the number of rows of the table fl_feature with a prepared statement.
*
* @param ps the PreparedStatement to be used
* @return the number of rows returned
* @throws DaoException
*/
private int countByPreparedStatement(PreparedStatement ps) throws DaoException
{
ResultSet rs = null;
try
{
int iReturn = -1;
rs = ps.executeQuery();
if (rs.next()) {
iReturn = rs.getInt("MCOUNT");
}
if (iReturn != -1) {
return iReturn;
}
}
catch(SQLException e)
{
throw new DataAccessException(e);
}
finally
{
this.getManager().close(rs);
}
throw new DataAccessException("Error in countByPreparedStatement");
}
//20
/**
* count the number of elements of a specific FlFeatureBean bean given the search type
*
* @param bean the FlFeatureBean template to look for
* @param searchType exact ? like ? starting like ?
* @return the number of rows returned
* @throws DaoException
*/
@Override
public int countUsingTemplate(FlFeatureBean bean, int searchType) throws DaoException
{
Connection c = null;
PreparedStatement ps = null;
StringBuilder sql = new StringBuilder("SELECT COUNT(*) AS MCOUNT FROM fl_feature");
StringBuilder sqlWhere = new StringBuilder("");
try
{
if (this.fillWhere(sqlWhere, bean, SEARCH_EXACT) > 0)
{
sql.append(" WHERE ").append(sqlWhere);
}
else
{
// System.out.println("The bean to look is not initialized... counting all...");
}
// System.out.println("countUsingTemplate: " + sql.toString());
c = this.getConnection();
ps = c.prepareStatement(sql.toString(),
ResultSet.TYPE_SCROLL_INSENSITIVE,
ResultSet.CONCUR_READ_ONLY);
this.fillPreparedStatement(ps, bean, searchType,false);
return this.countByPreparedStatement(ps);
}
catch(SQLException e)
{
throw new DataAccessException(e);
}
finally
{
this.getManager().close(ps);
this.freeConnection(c);
sql = null;
sqlWhere = null;
}
}
/**
* fills the given StringBuilder with the sql where clauses constructed using the bean and the search type
* @param sqlWhere the StringBuilder that will be filled
* @param bean the bean to use for creating the where clauses
* @param searchType exact ? like ? starting like ?
* @return the number of clauses returned
*/
protected int fillWhere(StringBuilder sqlWhere, FlFeatureBean bean, int searchType)
{
if (bean == null) {
return 0;
}
int dirtyCount = 0;
String sqlEqualsOperation = searchType == SEARCH_EXACT ? "=" : " like ";
try
{
if (bean.checkMd5Modified()) {
dirtyCount ++;
if (bean.getMd5() == null) {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("md5 IS NULL");
} else {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("md5 ").append(sqlEqualsOperation).append("?");
}
}
if (bean.checkSdkVersionModified()) {
dirtyCount ++;
if (bean.getSdkVersion() == null) {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("sdk_version IS NULL");
} else {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("sdk_version ").append(sqlEqualsOperation).append("?");
}
}
if (bean.checkPersonIdModified()) {
dirtyCount ++;
if (bean.getPersonId() == null) {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("person_id IS NULL");
} else {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("person_id = ?");
}
}
if (bean.checkFeatureModified()) {
dirtyCount ++;
if (bean.getFeature() == null) {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("feature IS NULL");
} else {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("feature = ?");
}
}
if (bean.checkUpdateTimeModified()) {
dirtyCount ++;
if (bean.getUpdateTime() == null) {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("update_time IS NULL");
} else {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("update_time = ?");
}
}
}
finally
{
sqlEqualsOperation = null;
}
return dirtyCount;
}
/**
* fill the given prepared statement with the bean values and a search type
* @param ps the PreparedStatement that will be filled
* @param bean the bean to use for creating the where clauses
* @param searchType exact ? like ? starting like ?
* @param fillNull wether fill null for null field
* @return the number of clauses returned
* @throws DaoException
*/
protected int fillPreparedStatement(PreparedStatement ps, FlFeatureBean bean, int searchType,boolean fillNull) throws DaoException
{
if (bean == null) {
return 0;
}
int dirtyCount = 0;
try
{
if (bean.checkMd5Modified()) {
switch (searchType) {
case SEARCH_EXACT:
// System.out.println("Setting for " + dirtyCount + " [" + bean.getMd5() + "]");
if (bean.getMd5() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.CHAR);} } else { ps.setString(++dirtyCount, bean.getMd5()); }
break;
case SEARCH_LIKE:
// System.out.println("Setting for " + dirtyCount + " [%" + bean.getMd5() + "%]");
if ( bean.getMd5() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.CHAR);} } else { ps.setString(++dirtyCount, SQL_LIKE_WILDCARD + bean.getMd5() + SQL_LIKE_WILDCARD); }
break;
case SEARCH_STARTING_LIKE:
// System.out.println("Setting for " + dirtyCount + " [%" + bean.getMd5() + "]");
if ( bean.getMd5() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.CHAR);} } else { ps.setString(++dirtyCount, SQL_LIKE_WILDCARD + bean.getMd5()); }
break;
case SEARCH_ENDING_LIKE:
// System.out.println("Setting for " + dirtyCount + " [" + bean.getMd5() + "%]");
if (bean.getMd5() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.CHAR);} } else { ps.setString(++dirtyCount, bean.getMd5() + SQL_LIKE_WILDCARD); }
break;
default:
throw new DaoException("Unknown search type " + searchType);
}
}
if (bean.checkSdkVersionModified()) {
switch (searchType) {
case SEARCH_EXACT:
// System.out.println("Setting for " + dirtyCount + " [" + bean.getSdkVersion() + "]");
if (bean.getSdkVersion() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.CHAR);} } else { ps.setString(++dirtyCount, bean.getSdkVersion()); }
break;
case SEARCH_LIKE:
// System.out.println("Setting for " + dirtyCount + " [%" + bean.getSdkVersion() + "%]");
if ( bean.getSdkVersion() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.CHAR);} } else { ps.setString(++dirtyCount, SQL_LIKE_WILDCARD + bean.getSdkVersion() + SQL_LIKE_WILDCARD); }
break;
case SEARCH_STARTING_LIKE:
// System.out.println("Setting for " + dirtyCount + " [%" + bean.getSdkVersion() + "]");
if ( bean.getSdkVersion() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.CHAR);} } else { ps.setString(++dirtyCount, SQL_LIKE_WILDCARD + bean.getSdkVersion()); }
break;
case SEARCH_ENDING_LIKE:
// System.out.println("Setting for " + dirtyCount + " [" + bean.getSdkVersion() + "%]");
if (bean.getSdkVersion() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.CHAR);} } else { ps.setString(++dirtyCount, bean.getSdkVersion() + SQL_LIKE_WILDCARD); }
break;
default:
throw new DaoException("Unknown search type " + searchType);
}
}
if (bean.checkPersonIdModified()) {
// System.out.println("Setting for " + dirtyCount + " [" + bean.getPersonId() + "]");
if (bean.getPersonId() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.INTEGER);} } else { Manager.setInteger(ps, ++dirtyCount, bean.getPersonId()); }
}
if (bean.checkFeatureModified()) {
// System.out.println("Setting for " + dirtyCount + " [" + bean.getFeature() + "]");
if (bean.getFeature() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.LONGVARBINARY);} } else { Manager.setBytes(Types.LONGVARBINARY,ps, ++dirtyCount, bean.getFeature()); }
}
if (bean.checkUpdateTimeModified()) {
// System.out.println("Setting for " + dirtyCount + " [" + bean.getUpdateTime() + "]");
if (bean.getUpdateTime() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.TIMESTAMP);} } else { ps.setTimestamp(++dirtyCount, new java.sql.Timestamp(bean.getUpdateTime().getTime())); }
}
}
catch(SQLException e)
{
throw new DataAccessException(e);
}
return dirtyCount;
}
//_____________________________________________________________________
//
// DECODE RESULT SET
//_____________________________________________________________________
//28
/**
* decode a resultset in an array of FlFeatureBean objects
*
* @param rs the resultset to decode
* @param fieldList table of the field's associated constants
* @param startRow the start row to be used (first row = 1, last row = -1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @return the resulting FlFeatureBean table
* @throws DaoException
*/
public FlFeatureBean[] decodeResultSet(ResultSet rs, int[] fieldList, int startRow, int numRows) throws DaoException
{
return this.decodeResultSetAsList(rs, fieldList, startRow, numRows).toArray(new FlFeatureBean[0]);
}
//28-1
/**
* decode a resultset in a list of FlFeatureBean objects
*
* @param rs the resultset to decode
* @param fieldList table of the field's associated constants
* @param startRow the start row to be used (first row = 1, last row = -1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @return the resulting FlFeatureBean table
* @throws DaoException
*/
public List decodeResultSetAsList(ResultSet rs, int[] fieldList, int startRow, int numRows) throws DaoException
{
ListAction action = new ListAction();
actionOnResultSet(rs, fieldList, numRows, numRows, action);
return action.getList();
}
//28-2
/** decode a resultset and call action
* @param rs the resultset to decode
* @param fieldList table of the field's associated constants
* @param startRow the start row to be used (first row = 1, last row = -1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @param action interface obj for do something
* @return the count dealt by action
* @throws DaoException
* @throws IllegalArgumentException
*/
public int actionOnResultSet(ResultSet rs, int[] fieldList, int startRow, int numRows, Action action) throws DaoException{
try{
int count = 0;
if(0!=numRows){
if( startRow<1 ){
throw new IllegalArgumentException("invalid argument:startRow (must >=1)");
}
if( null==action || null==rs ){
throw new IllegalArgumentException("invalid argument:action OR rs (must not be null)");
}
for(;startRow > 1 && rs.next();){
--startRow;
//skip to last of startRow
}
if (fieldList == null) {
if(numRows<0){
for(;rs.next();++count){
action.call(decodeRow(rs, action.getBean()));
}
}else{
for(;rs.next() && count loadByPreparedStatementAsList(PreparedStatement ps) throws DaoException
{
return this.loadByPreparedStatementAsList(ps, null);
}
//33
/**
* Loads all the elements using a prepared statement specifying a list of fields to be retrieved.
*
* @param ps the PreparedStatement to be used
* @param fieldList table of the field's associated constants
* @return an array of FlFeatureBean
* @throws DaoException
*/
public FlFeatureBean[] loadByPreparedStatement(PreparedStatement ps, int[] fieldList) throws DaoException
{
return this.loadByPreparedStatementAsList(ps, fieldList).toArray(new FlFeatureBean[0]);
}
//33
/**
* Loads all the elements using a prepared statement specifying a list of fields to be retrieved.
*
* @param ps the PreparedStatement to be used
* @param fieldList table of the field's associated constants
* @return an array of FlFeatureBean
* @throws DaoException
*/
public List loadByPreparedStatementAsList(PreparedStatement ps, int[] fieldList) throws DaoException
{
return loadByPreparedStatementAsList(ps,fieldList,1,-1);
}
//34
/**
* Loads all the elements using a prepared statement specifying a list of fields to be retrieved,
* and specifying the start row and the number of rows.
*
* @param ps the PreparedStatement to be used
* @param startRow the start row to be used (first row = 1, last row = -1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @param fieldList table of the field's associated constants
* @return an array of FlFeatureBean
* @throws DaoException
*/
public FlFeatureBean[] loadByPreparedStatement(PreparedStatement ps, int[] fieldList, int startRow, int numRows) throws DaoException
{
return loadByPreparedStatementAsList(ps,fieldList,startRow,numRows).toArray(new FlFeatureBean[0]);
}
//34-1
/**
* Loads all the elements using a prepared statement specifying a list of fields to be retrieved,
* and specifying the start row and the number of rows.
*
* @param ps the PreparedStatement to be used
* @param startRow the start row to be used (first row = 1, last row = -1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @param fieldList table of the field's associated constants
* @return an array of FlFeatureBean
* @throws DaoException
*/
public List loadByPreparedStatementAsList(PreparedStatement ps, int[] fieldList, int startRow, int numRows) throws DaoException
{
ListAction action = new ListAction();
loadByPreparedStatement(ps,fieldList,startRow,numRows,action);
return action.getList();
}
//34-2
/**
* Loads each element using a prepared statement specifying a list of fields to be retrieved,
* and specifying the start row and the number of rows
* and dealt by action.
*
* @param ps the PreparedStatement to be used
* @param startRow the start row to be used (first row = 1, last row = -1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @param fieldList table of the field's associated constants
* @param action Action object for do something(not null)
* @return the count dealt by action
* @throws DaoException
*/
public int loadByPreparedStatement(PreparedStatement ps, int[] fieldList, int startRow, int numRows,Action action) throws DaoException
{
ResultSet rs = null;
try {
ps.setFetchSize(100);
rs = ps.executeQuery();
return this.actionOnResultSet(rs, fieldList, startRow, numRows, action);
} catch (DaoException e) {
throw e;
} catch (SQLException e) {
throw new DataAccessException(e);
} finally {
this.getManager().close(rs);
}
}
//_____________________________________________________________________
//
// LISTENER
//_____________________________________________________________________
private final TableListener.ListenerContainer listenerContainer = new TableListener.ListenerContainer();
//35
@Override
public TableListener registerListener(TableListener listener)
{
this.listenerContainer.add(listener);
return listener;
}
//36
/**
* remove listener.
*/
@Override
public void unregisterListener(TableListener listener)
{
this.listenerContainer.remove(listener);
}
//37
@Override
public void fire(TableListener.Event event, FlFeatureBean bean) throws DaoException{
if(null == event){
throw new NullPointerException();
}
event.fire(listenerContainer, bean);
}
//37-1
@Override
public void fire(int event, FlFeatureBean bean) throws DaoException{
try{
fire(TableListener.Event.values()[event],bean);
}catch(ArrayIndexOutOfBoundsException e){
throw new IllegalArgumentException("invalid event id " + event);
}
}
/** foreign key listener for DEELTE RULE : CASCADE */
private final net.gdface.facelog.dborm.BaseForeignKeyListener foreignKeyListenerByPersonId =
new net.gdface.facelog.dborm.BaseForeignKeyListener(){
@Override
protected List getImportedBeans(FlPersonBean bean) throws DaoException {
return listenerContainer.isEmpty()
? java.util.Collections.emptyList()
: instanceOfFlPersonManager().getFeatureBeansByPersonIdAsList(bean);
}
@Override
protected void onRemove(List effectBeans) throws DaoException {
for(FlFeatureBean bean:effectBeans){
Event.DELETE.fire(listenerContainer, bean);
}
}};
//37-2
/**
* bind foreign key listener to foreign table:
* DELETE RULE : CASCADE {@code fl_feature(person_id)- fl_person(id)}
*/
public void bindForeignKeyListenerForDeleteRule(){
instanceOfFlPersonManager().registerListener(foreignKeyListenerByPersonId);
}
//37-3
/**
* unbind foreign key listener from all of foreign tables
* @see #bindForeignKeyListenerForDeleteRule()
*/
public void unbindForeignKeyListenerForDeleteRule(){
instanceOfFlPersonManager().unregisterListener(foreignKeyListenerByPersonId);
}
//_____________________________________________________________________
//
// UTILS
//_____________________________________________________________________
//40
/**
* Retrieves the manager object used to get connections.
*
* @return the manager used
*/
private Manager getManager()
{
return Manager.getInstance();
}
//41
/**
* Frees the connection.
*
* @param c the connection to release
*/
private void freeConnection(Connection c)
{
// back to pool
this.getManager().releaseConnection(c);
}
//42
/**
* Gets the connection.
*/
private Connection getConnection() throws DaoException
{
try
{
return this.getManager().getConnection();
}
catch(SQLException e)
{
throw new DataAccessException(e);
}
}
//43
@Override
public boolean isPrimaryKey(String column){
for(String c:PRIMARYKEY_NAMES){
if(c.equalsIgnoreCase(column)){
return true;
}
}
return false;
}
/**
* Fill the given prepared statement with the values in argList
* @param ps the PreparedStatement that will be filled
* @param argList the arguments to use fill given prepared statement
* @throws DaoException
*/
private void fillPrepareStatement(PreparedStatement ps, Object[] argList) throws DaoException{
try {
if (!(argList == null || ps == null)) {
for (int i = 0; i < argList.length; i++) {
if (argList[i].getClass().equals(byte[].class)) {
ps.setBytes(i + 1, (byte[]) argList[i]);
} else {
ps.setObject(i + 1, argList[i]);
}
}
}
} catch (SQLException e) {
throw new DaoException(e);
}
}
@Override
public int loadBySqlForAction(String sql, Object[] argList, int[] fieldList,int startRow, int numRows,Action action) throws DaoException{
PreparedStatement ps = null;
Connection connection = null;
// logger.debug("sql string:\n" + sql + "\n");
try {
connection = this.getConnection();
ps = connection.prepareStatement(sql,
ResultSet.TYPE_FORWARD_ONLY,
ResultSet.CONCUR_READ_ONLY);
fillPrepareStatement(ps, argList);
return this.loadByPreparedStatement(ps, fieldList, startRow, numRows, action);
} catch (DaoException e) {
throw e;
}catch (SQLException e) {
throw new DataAccessException(e);
} finally {
this.getManager().close(ps);
this.freeConnection(connection);
}
}
@Override
public T runAsTransaction(Callable fun) throws DaoException{
return Manager.getInstance().runAsTransaction(fun);
}
class DeleteBeanAction extends Action.BaseAdapter{
private final AtomicInteger count=new AtomicInteger(0);
@Override
public void call(FlFeatureBean bean) throws DaoException {
FlFeatureManager.this.delete(bean);
count.incrementAndGet();
}
int getCount(){
return count.get();
}
}
//45
/**
* return a primary key list from {@link FlFeatureBean} array
* @param array
*/
public List toPrimaryKeyList(FlFeatureBean... array){
if(null == array){
return new java.util.ArrayList();
}
java.util.ArrayList list = new java.util.ArrayList(array.length);
for(FlFeatureBean bean:array){
list.add(null == bean ? null : bean.getMd5());
}
return list;
}
//46
/**
* return a primary key list from {@link FlFeatureBean} collection
* @param collection
*/
public List toPrimaryKeyList(java.util.Collection collection){
if(null == collection){
return new java.util.ArrayList();
}
java.util.ArrayList list = new java.util.ArrayList(collection.size());
for(FlFeatureBean bean:collection){
list.add(null == bean ? null : bean.getMd5());
}
return list;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy