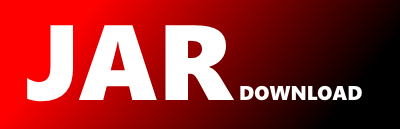
net.gdface.facelog.dborm.image.FlImageBean Maven / Gradle / Ivy
// ______________________________________________________
// Generated by sql2java - https://github.com/10km/sql2java-2-6-7 (custom branch)
// modified by guyadong from
// sql2java original version https://sourceforge.net/projects/sql2java/
// JDBC driver used at code generation time: com.mysql.jdbc.Driver
// template: bean.java.vm
// ______________________________________________________
package net.gdface.facelog.dborm.image;
import java.io.Serializable;
import java.util.List;
import java.util.Objects;
import net.gdface.facelog.dborm.Constant;
import net.gdface.facelog.dborm.BaseBean;
import net.gdface.facelog.dborm.device.FlDeviceBean;
import net.gdface.facelog.dborm.CompareToBuilder;
import net.gdface.facelog.dborm.EqualsBuilder;
import net.gdface.facelog.dborm.HashCodeBuilder;
/**
* FlImageBean is a mapping of fl_image Table.
*
Meta Data Information (in progress):
*
* - comments: 图像信息存储表,用于存储系统中所有用到的图像数据,表中只包含图像基本信息,图像二进制源数据存在在fl_store中(md5对应)
*
* @author guyadong
*/
public class FlImageBean
implements Serializable,BaseBean,Comparable,Constant,Cloneable
{
private static final long serialVersionUID = 646979810912117585L;
/** NULL {@link FlImageBean} bean , IMMUTABLE instance */
public static final FlImageBean NULL = new FlImageBean().asNULL().asImmutable();
/** comments:主键,图像md5检验码,同时也是从 fl_store 获取图像数据的key */
private String md5;
/** comments:图像格式 */
private String format;
/** comments:图像宽度 */
private Integer width;
/** comments:图像高度 */
private Integer height;
/** comments:通道数 */
private Integer depth;
/** comments:图像中的人脸数目 */
private Integer faceNum;
/** comments:缩略图md5,图像数据存储在 fl_imae_store(md5) */
private String thumbMd5;
/** comments:外键,图像来源设备 */
private Integer deviceId;
/** flag whether {@code this} can be modified */
private Boolean immutable;
/** columns modified flag */
private int modified;
/** columns initialized flag */
private int initialized;
/** new record flag */
private boolean isNew;
/**
* set immutable status
* @return {@code this}
*/
private FlImageBean immutable(Boolean immutable) {
this.immutable = immutable;
return this;
}
/**
* set {@code this} as immutable object
* @return {@code this}
*/
public FlImageBean asImmutable() {
return immutable(Boolean.TRUE);
}
/**
* @return {@code true} if {@code this} is a mutable object
*/
public boolean mutable(){
return !Boolean.TRUE.equals(this.immutable);
}
/**
* @return {@code this}
* @throws IllegalStateException if {@code this} is a immutable object
*/
private FlImageBean checkMutable(){
if(!mutable()){
throw new IllegalStateException("this is a immutable object");
}
return this;
}
/**
* @return return a new mutable copy of this object.
*/
public FlImageBean cloneMutable(){
return clone().immutable(null);
}
@Override
public boolean isNew()
{
return this.isNew;
}
@Override
public void isNew(boolean isNew)
{
this.isNew = isNew;
}
/**
* Specifies to the object if it has been set as new.
*
* @param isNew the boolean value to be assigned to the isNew field
*/
public void setNew(boolean isNew)
{
this.isNew = isNew;
}
/**
* @return the modified status of columns
*/
public int getModified(){
return modified;
}
/**
* @param modified the modified status bit to be assigned to {@link #modified}
*/
public void setModified(int modified){
this.modified = modified;
}
/**
* @return the initialized status of columns
*/
public int getInitialized(){
return initialized;
}
/**
* @param initialized the initialized status bit to be assigned to {@link #initialized}
*/
public void setInitialized(int initialized){
this.initialized = initialized;
}
protected static final >boolean equals(T a, T b) {
return a == b || (a != null && 0==a.compareTo(b));
}
public FlImageBean(){
super();
reset();
}
/**
* construct a new instance filled with primary keys
* @param md5 PK# 1
*/
public FlImageBean(String md5){
this();
setMd5(md5);
}
/**
* Getter method for {@link #md5}.
* PRIMARY KEY.
* Meta Data Information (in progress):
*
* - full name: fl_image.md5
* - imported key: fl_face.image_md5
* - imported key: fl_person.image_md5
* - comments: 主键,图像md5检验码,同时也是从 fl_store 获取图像数据的key
* - NOT NULL
* - column size: 32
* - JDBC type returned by the driver: Types.CHAR
*
*
* @return the value of md5
*/
public String getMd5(){
return md5;
}
/**
* Setter method for {@link #md5}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to md5
*/
public void setMd5(String newVal)
{
checkMutable();
modified |= FL_IMAGE_ID_MD5_MASK;
initialized |= FL_IMAGE_ID_MD5_MASK;
if (Objects.equals(newVal, md5)) {
return;
}
md5 = newVal;
}
/**
* Determines if the md5 has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkMd5Modified()
{
return 0L != (modified & FL_IMAGE_ID_MD5_MASK);
}
/**
* Determines if the md5 has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkMd5Initialized()
{
return 0L != (initialized & FL_IMAGE_ID_MD5_MASK);
}
/**
* Getter method for {@link #format}.
* Meta Data Information (in progress):
*
* - full name: fl_image.format
* - comments: 图像格式
* - column size: 32
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of format
*/
public String getFormat(){
return format;
}
/**
* Setter method for {@link #format}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to format
*/
public void setFormat(String newVal)
{
checkMutable();
modified |= FL_IMAGE_ID_FORMAT_MASK;
initialized |= FL_IMAGE_ID_FORMAT_MASK;
if (Objects.equals(newVal, format)) {
return;
}
format = newVal;
}
/**
* Determines if the format has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkFormatModified()
{
return 0L != (modified & FL_IMAGE_ID_FORMAT_MASK);
}
/**
* Determines if the format has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkFormatInitialized()
{
return 0L != (initialized & FL_IMAGE_ID_FORMAT_MASK);
}
/**
* Getter method for {@link #width}.
* Meta Data Information (in progress):
*
* - full name: fl_image.width
* - comments: 图像宽度
* - NOT NULL
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of width
*/
public Integer getWidth(){
return width;
}
/**
* Setter method for {@link #width}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to width
*/
public void setWidth(Integer newVal)
{
checkMutable();
modified |= FL_IMAGE_ID_WIDTH_MASK;
initialized |= FL_IMAGE_ID_WIDTH_MASK;
if (Objects.equals(newVal, width)) {
return;
}
width = newVal;
}
/**
* Determines if the width has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkWidthModified()
{
return 0L != (modified & FL_IMAGE_ID_WIDTH_MASK);
}
/**
* Determines if the width has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkWidthInitialized()
{
return 0L != (initialized & FL_IMAGE_ID_WIDTH_MASK);
}
/**
* Getter method for {@link #height}.
* Meta Data Information (in progress):
*
* - full name: fl_image.height
* - comments: 图像高度
* - NOT NULL
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of height
*/
public Integer getHeight(){
return height;
}
/**
* Setter method for {@link #height}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to height
*/
public void setHeight(Integer newVal)
{
checkMutable();
modified |= FL_IMAGE_ID_HEIGHT_MASK;
initialized |= FL_IMAGE_ID_HEIGHT_MASK;
if (Objects.equals(newVal, height)) {
return;
}
height = newVal;
}
/**
* Determines if the height has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkHeightModified()
{
return 0L != (modified & FL_IMAGE_ID_HEIGHT_MASK);
}
/**
* Determines if the height has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkHeightInitialized()
{
return 0L != (initialized & FL_IMAGE_ID_HEIGHT_MASK);
}
/**
* Getter method for {@link #depth}.
* Meta Data Information (in progress):
*
* - full name: fl_image.depth
* - comments: 通道数
* - default value: '0'
* - NOT NULL
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of depth
*/
public Integer getDepth(){
return depth;
}
/**
* Setter method for {@link #depth}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to depth
*/
public void setDepth(Integer newVal)
{
checkMutable();
modified |= FL_IMAGE_ID_DEPTH_MASK;
initialized |= FL_IMAGE_ID_DEPTH_MASK;
if (Objects.equals(newVal, depth)) {
return;
}
depth = newVal;
}
/**
* Determines if the depth has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkDepthModified()
{
return 0L != (modified & FL_IMAGE_ID_DEPTH_MASK);
}
/**
* Determines if the depth has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkDepthInitialized()
{
return 0L != (initialized & FL_IMAGE_ID_DEPTH_MASK);
}
/**
* Getter method for {@link #faceNum}.
* Meta Data Information (in progress):
*
* - full name: fl_image.face_num
* - comments: 图像中的人脸数目
* - default value: '0'
* - NOT NULL
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of faceNum
*/
public Integer getFaceNum(){
return faceNum;
}
/**
* Setter method for {@link #faceNum}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to faceNum
*/
public void setFaceNum(Integer newVal)
{
checkMutable();
modified |= FL_IMAGE_ID_FACE_NUM_MASK;
initialized |= FL_IMAGE_ID_FACE_NUM_MASK;
if (Objects.equals(newVal, faceNum)) {
return;
}
faceNum = newVal;
}
/**
* Determines if the faceNum has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkFaceNumModified()
{
return 0L != (modified & FL_IMAGE_ID_FACE_NUM_MASK);
}
/**
* Determines if the faceNum has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkFaceNumInitialized()
{
return 0L != (initialized & FL_IMAGE_ID_FACE_NUM_MASK);
}
/**
* Getter method for {@link #thumbMd5}.
* Meta Data Information (in progress):
*
* - full name: fl_image.thumb_md5
* - comments: 缩略图md5,图像数据存储在 fl_imae_store(md5)
* - column size: 32
* - JDBC type returned by the driver: Types.CHAR
*
*
* @return the value of thumbMd5
*/
public String getThumbMd5(){
return thumbMd5;
}
/**
* Setter method for {@link #thumbMd5}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to thumbMd5
*/
public void setThumbMd5(String newVal)
{
checkMutable();
modified |= FL_IMAGE_ID_THUMB_MD5_MASK;
initialized |= FL_IMAGE_ID_THUMB_MD5_MASK;
if (Objects.equals(newVal, thumbMd5)) {
return;
}
thumbMd5 = newVal;
}
/**
* Determines if the thumbMd5 has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkThumbMd5Modified()
{
return 0L != (modified & FL_IMAGE_ID_THUMB_MD5_MASK);
}
/**
* Determines if the thumbMd5 has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkThumbMd5Initialized()
{
return 0L != (initialized & FL_IMAGE_ID_THUMB_MD5_MASK);
}
/**
* Getter method for {@link #deviceId}.
* Meta Data Information (in progress):
*
* - full name: fl_image.device_id
* - foreign key: fl_device.id
* - comments: 外键,图像来源设备
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of deviceId
*/
public Integer getDeviceId(){
return deviceId;
}
/**
* Setter method for {@link #deviceId}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to deviceId
*/
public void setDeviceId(Integer newVal)
{
checkMutable();
modified |= FL_IMAGE_ID_DEVICE_ID_MASK;
initialized |= FL_IMAGE_ID_DEVICE_ID_MASK;
if (Objects.equals(newVal, deviceId)) {
return;
}
deviceId = newVal;
}
/**
* Determines if the deviceId has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkDeviceIdModified()
{
return 0L != (modified & FL_IMAGE_ID_DEVICE_ID_MASK);
}
/**
* Determines if the deviceId has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkDeviceIdInitialized()
{
return 0L != (initialized & FL_IMAGE_ID_DEVICE_ID_MASK);
}
//////////////////////////////////////
// referenced bean for FOREIGN KEYS
//////////////////////////////////////
/**
* The referenced {@link FlDeviceBean} by {@link #deviceId} .
* FOREIGN KEY (device_id) REFERENCES fl_device(id)
*/
private FlDeviceBean referencedByDeviceId;
/**
* Getter method for {@link #referencedByDeviceId}.
* @return FlDeviceBean
*/
public FlDeviceBean getReferencedByDeviceId() {
return this.referencedByDeviceId;
}
/**
* Setter method for {@link #referencedByDeviceId}.
* @param reference FlDeviceBean
*/
public void setReferencedByDeviceId(FlDeviceBean reference) {
this.referencedByDeviceId = reference;
}
@Override
public boolean isModified()
{
return 0 != modified;
}
@Override
public boolean isModified(int columnID){
switch ( columnID ){
case FL_IMAGE_ID_MD5:
return checkMd5Modified();
case FL_IMAGE_ID_FORMAT:
return checkFormatModified();
case FL_IMAGE_ID_WIDTH:
return checkWidthModified();
case FL_IMAGE_ID_HEIGHT:
return checkHeightModified();
case FL_IMAGE_ID_DEPTH:
return checkDepthModified();
case FL_IMAGE_ID_FACE_NUM:
return checkFaceNumModified();
case FL_IMAGE_ID_THUMB_MD5:
return checkThumbMd5Modified();
case FL_IMAGE_ID_DEVICE_ID:
return checkDeviceIdModified();
default:
return false;
}
}
@Override
public boolean isInitialized(int columnID){
switch(columnID) {
case FL_IMAGE_ID_MD5:
return checkMd5Initialized();
case FL_IMAGE_ID_FORMAT:
return checkFormatInitialized();
case FL_IMAGE_ID_WIDTH:
return checkWidthInitialized();
case FL_IMAGE_ID_HEIGHT:
return checkHeightInitialized();
case FL_IMAGE_ID_DEPTH:
return checkDepthInitialized();
case FL_IMAGE_ID_FACE_NUM:
return checkFaceNumInitialized();
case FL_IMAGE_ID_THUMB_MD5:
return checkThumbMd5Initialized();
case FL_IMAGE_ID_DEVICE_ID:
return checkDeviceIdInitialized();
default:
return false;
}
}
@Override
public boolean isModified(String column){
return isModified(columnIDOf(column));
}
@Override
public boolean isInitialized(String column){
return isInitialized(columnIDOf(column));
}
@Override
public void resetIsModified()
{
checkMutable();
modified = 0;
}
@Override
public void resetPrimaryKeysModified()
{
modified &= (~(FL_IMAGE_ID_MD5_MASK));
}
/**
* Resets columns modification status except primary keys to 'not modified'.
*/
public void resetModifiedExceptPrimaryKeys()
{
modified &= (~(FL_IMAGE_ID_FORMAT_MASK |
FL_IMAGE_ID_WIDTH_MASK |
FL_IMAGE_ID_HEIGHT_MASK |
FL_IMAGE_ID_DEPTH_MASK |
FL_IMAGE_ID_FACE_NUM_MASK |
FL_IMAGE_ID_THUMB_MD5_MASK |
FL_IMAGE_ID_DEVICE_ID_MASK));
}
/**
* Resets the object initialization status to 'not initialized'.
*/
private void resetInitialized()
{
initialized = 0;
}
/** reset all fields to initial value, equal to a new bean */
public void reset(){
checkMutable();
this.md5 = null;
this.format = null;
this.width = null;
this.height = null;
/* DEFAULT:'0'*/
this.depth = new Integer(0);
/* DEFAULT:'0'*/
this.faceNum = new Integer(0);
this.thumbMd5 = null;
this.deviceId = null;
this.isNew = true;
this.modified = 0;
this.initialized = (FL_IMAGE_ID_DEPTH_MASK | FL_IMAGE_ID_FACE_NUM_MASK);
}
@Override
public boolean equals(Object object)
{
if (!(object instanceof FlImageBean)) {
return false;
}
FlImageBean obj = (FlImageBean) object;
return new EqualsBuilder()
.append(getMd5(), obj.getMd5())
.append(getFormat(), obj.getFormat())
.append(getWidth(), obj.getWidth())
.append(getHeight(), obj.getHeight())
.append(getDepth(), obj.getDepth())
.append(getFaceNum(), obj.getFaceNum())
.append(getThumbMd5(), obj.getThumbMd5())
.append(getDeviceId(), obj.getDeviceId())
.isEquals();
}
@Override
public int hashCode()
{
return new HashCodeBuilder(-82280557, -700257973)
.append(getMd5())
.toHashCode();
}
@Override
public String toString() {
return toString(true,false);
}
/**
* cast byte array to HEX string
*
* @param input
* @return {@code null} if {@code input} is null
*/
private static final String toHex(byte[] input) {
if (null == input){
return null;
}
StringBuffer sb = new StringBuffer(input.length * 2);
for (int i = 0; i < input.length; i++) {
sb.append(Character.forDigit((input[i] & 240) >> 4, 16));
sb.append(Character.forDigit(input[i] & 15, 16));
}
return sb.toString();
}
protected static final StringBuilder append(StringBuilder buffer,boolean full,byte[] value){
if(full || null == value){
buffer.append(toHex(value));
}else{
buffer.append(value.length).append(" bytes");
}
return buffer;
}
private static int stringLimit = 64;
private static final int MINIMUM_LIMIT = 16;
protected static final StringBuilder append(StringBuilder buffer,boolean full,String value){
if(full || null == value || value.length() <= stringLimit){
buffer.append(value);
}else{
buffer.append(value.substring(0,stringLimit - 8)).append(" ...").append(value.substring(stringLimit-4,stringLimit));
}
return buffer;
}
protected static final StringBuilder append(StringBuilder buffer,boolean full,T value){
return buffer.append(value);
}
public static final void setStringLimit(int limit){
if(limit < MINIMUM_LIMIT){
throw new IllegalArgumentException(String.format("INVALID limit %d,minimum value %d",limit,MINIMUM_LIMIT));
}
stringLimit = limit;
}
@Override
public String toString(boolean notNull, boolean fullIfStringOrBytes) {
// only output initialized field
StringBuilder builder = new StringBuilder(this.getClass().getName()).append("@").append(Integer.toHexString(this.hashCode())).append("[");
int count = 0;
if(checkMd5Initialized()){
if(!notNull || null != getMd5()){
if(count++ >0){
builder.append(",");
}
builder.append("md5=");
append(builder,fullIfStringOrBytes,getMd5());
}
}
if(checkFormatInitialized()){
if(!notNull || null != getFormat()){
if(count++ >0){
builder.append(",");
}
builder.append("format=");
append(builder,fullIfStringOrBytes,getFormat());
}
}
if(checkWidthInitialized()){
if(!notNull || null != getWidth()){
if(count++ >0){
builder.append(",");
}
builder.append("width=");
append(builder,fullIfStringOrBytes,getWidth());
}
}
if(checkHeightInitialized()){
if(!notNull || null != getHeight()){
if(count++ >0){
builder.append(",");
}
builder.append("height=");
append(builder,fullIfStringOrBytes,getHeight());
}
}
if(checkDepthInitialized()){
if(!notNull || null != getDepth()){
if(count++ >0){
builder.append(",");
}
builder.append("depth=");
append(builder,fullIfStringOrBytes,getDepth());
}
}
if(checkFaceNumInitialized()){
if(!notNull || null != getFaceNum()){
if(count++ >0){
builder.append(",");
}
builder.append("face_num=");
append(builder,fullIfStringOrBytes,getFaceNum());
}
}
if(checkThumbMd5Initialized()){
if(!notNull || null != getThumbMd5()){
if(count++ >0){
builder.append(",");
}
builder.append("thumb_md5=");
append(builder,fullIfStringOrBytes,getThumbMd5());
}
}
if(checkDeviceIdInitialized()){
if(!notNull || null != getDeviceId()){
if(count++ >0){
builder.append(",");
}
builder.append("device_id=");
append(builder,fullIfStringOrBytes,getDeviceId());
}
}
builder.append("]");
return builder.toString();
}
@Override
public int compareTo(FlImageBean object){
return new CompareToBuilder()
.append(getMd5(), object.getMd5())
.append(getFormat(), object.getFormat())
.append(getWidth(), object.getWidth())
.append(getHeight(), object.getHeight())
.append(getDepth(), object.getDepth())
.append(getFaceNum(), object.getFaceNum())
.append(getThumbMd5(), object.getThumbMd5())
.append(getDeviceId(), object.getDeviceId())
.toComparison();
}
@Override
public FlImageBean clone(){
try {
return (FlImageBean) super.clone();
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
}
/**
* Make {@code this} to a NULL bean
* set all fields to null, {@link #modified} and {@link #initialized} be set to 0
* @return {@code this} bean
* @author guyadong
*/
public FlImageBean asNULL()
{
checkMutable();
setMd5((String)null);
setFormat((String)null);
setWidth((Integer)null);
setHeight((Integer)null);
setDepth((Integer)null);
setFaceNum((Integer)null);
setThumbMd5((String)null);
setDeviceId((Integer)null);
isNew(true);
resetInitialized();
resetIsModified();
return this;
}
/**
* check whether this bean is a NULL bean
* @return {@code true} if {@link #initialized} be set to zero
* @see #asNULL()
*/
public boolean checkNULL(){
return 0 == getInitialized();
}
/**
* @param source source list
* @return {@code source} replace {@code null} element with null instance({@link #NULL})
*/
public static final List replaceNull(List source){
if(null != source){
for(int i = 0,endIndex = source.size();i replaceNullInstance(List source){
if(null != source){
for(int i = 0,endIndex = source.size();iT getValue(int columnID)
{
switch( columnID ){
case FL_IMAGE_ID_MD5:
return (T)getMd5();
case FL_IMAGE_ID_FORMAT:
return (T)getFormat();
case FL_IMAGE_ID_WIDTH:
return (T)getWidth();
case FL_IMAGE_ID_HEIGHT:
return (T)getHeight();
case FL_IMAGE_ID_DEPTH:
return (T)getDepth();
case FL_IMAGE_ID_FACE_NUM:
return (T)getFaceNum();
case FL_IMAGE_ID_THUMB_MD5:
return (T)getThumbMd5();
case FL_IMAGE_ID_DEVICE_ID:
return (T)getDeviceId();
default:
return null;
}
}
@Override
public void setValue(int columnID,T value)
{
switch( columnID ) {
case FL_IMAGE_ID_MD5:
setMd5((String)value);
break;
case FL_IMAGE_ID_FORMAT:
setFormat((String)value);
break;
case FL_IMAGE_ID_WIDTH:
setWidth((Integer)value);
break;
case FL_IMAGE_ID_HEIGHT:
setHeight((Integer)value);
break;
case FL_IMAGE_ID_DEPTH:
setDepth((Integer)value);
break;
case FL_IMAGE_ID_FACE_NUM:
setFaceNum((Integer)value);
break;
case FL_IMAGE_ID_THUMB_MD5:
setThumbMd5((String)value);
break;
case FL_IMAGE_ID_DEVICE_ID:
setDeviceId((Integer)value);
break;
default:
break;
}
}
@Override
public T getValue(String column)
{
return getValue(columnIDOf(column));
}
@Override
public void setValue(String column,T value)
{
setValue(columnIDOf(column),value);
}
/**
* @param column column name
* @return column id for the given field name or negative if {@code column} is invalid name
*/
public static int columnIDOf(String column){
int index = FL_IMAGE_FIELDS_LIST.indexOf(column);
return index < 0
? FL_IMAGE_JAVA_FIELDS_LIST.indexOf(column)
: index;
}
public static final Builder builder(){
return new Builder().reset();
}
/**
* a builder for FlImageBean,the template instance is thread local variable
* a instance of Builder can be reused.
*/
public static final class Builder{
/** FlImageBean instance used for template to create new FlImageBean instance. */
static final ThreadLocal TEMPLATE = new ThreadLocal(){
@Override
protected FlImageBean initialValue() {
return new FlImageBean();
}};
private Builder() {}
/**
* reset the bean as template
* @see FlImageBean#reset()
*/
public Builder reset(){
TEMPLATE.get().reset();
return this;
}
/** set a bean as template,must not be {@code null} */
public Builder template(FlImageBean bean){
if(null == bean){
throw new NullPointerException();
}
TEMPLATE.set(bean);
return this;
}
/** return a clone instance of {@link #TEMPLATE}*/
public FlImageBean build(){
return TEMPLATE.get().clone();
}
/**
* fill the field : fl_image.md5
* @param md5 主键,图像md5检验码,同时也是从 fl_store 获取图像数据的key
* @see FlImageBean#getMd5()
* @see FlImageBean#setMd5(String)
*/
public Builder md5(String md5){
TEMPLATE.get().setMd5(md5);
return this;
}
/**
* fill the field : fl_image.format
* @param format 图像格式
* @see FlImageBean#getFormat()
* @see FlImageBean#setFormat(String)
*/
public Builder format(String format){
TEMPLATE.get().setFormat(format);
return this;
}
/**
* fill the field : fl_image.width
* @param width 图像宽度
* @see FlImageBean#getWidth()
* @see FlImageBean#setWidth(Integer)
*/
public Builder width(Integer width){
TEMPLATE.get().setWidth(width);
return this;
}
/**
* fill the field : fl_image.height
* @param height 图像高度
* @see FlImageBean#getHeight()
* @see FlImageBean#setHeight(Integer)
*/
public Builder height(Integer height){
TEMPLATE.get().setHeight(height);
return this;
}
/**
* fill the field : fl_image.depth
* @param depth 通道数
* @see FlImageBean#getDepth()
* @see FlImageBean#setDepth(Integer)
*/
public Builder depth(Integer depth){
TEMPLATE.get().setDepth(depth);
return this;
}
/**
* fill the field : fl_image.face_num
* @param faceNum 图像中的人脸数目
* @see FlImageBean#getFaceNum()
* @see FlImageBean#setFaceNum(Integer)
*/
public Builder faceNum(Integer faceNum){
TEMPLATE.get().setFaceNum(faceNum);
return this;
}
/**
* fill the field : fl_image.thumb_md5
* @param thumbMd5 缩略图md5,图像数据存储在 fl_imae_store(md5)
* @see FlImageBean#getThumbMd5()
* @see FlImageBean#setThumbMd5(String)
*/
public Builder thumbMd5(String thumbMd5){
TEMPLATE.get().setThumbMd5(thumbMd5);
return this;
}
/**
* fill the field : fl_image.device_id
* @param deviceId 外键,图像来源设备
* @see FlImageBean#getDeviceId()
* @see FlImageBean#setDeviceId(Integer)
*/
public Builder deviceId(Integer deviceId){
TEMPLATE.get().setDeviceId(deviceId);
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy