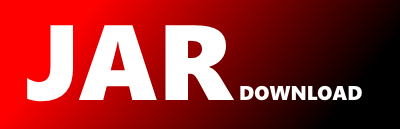
net.gdface.facelog.dborm.image.FlImageManager Maven / Gradle / Ivy
// ______________________________________________________
// Generated by sql2java - https://github.com/10km/sql2java-2-6-7 (custom branch)
// modified by guyadong from
// sql2java original version https://sourceforge.net/projects/sql2java/
// JDBC driver used at code generation time: com.mysql.jdbc.Driver
// template: manager.java.vm
// ______________________________________________________
package net.gdface.facelog.dborm.image;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.sql.Types;
import java.util.List;
import java.util.concurrent.Callable;
import java.util.concurrent.atomic.AtomicInteger;
import net.gdface.facelog.dborm.Constant;
import net.gdface.facelog.dborm.Manager;
import net.gdface.facelog.dborm.TableListener;
import net.gdface.facelog.dborm.TableManager;
import net.gdface.facelog.dborm.exception.DaoException;
import net.gdface.facelog.dborm.exception.DataAccessException;
import net.gdface.facelog.dborm.exception.DataRetrievalException;
import net.gdface.facelog.dborm.exception.ObjectRetrievalException;
import net.gdface.facelog.dborm.face.FlFaceBean;
import net.gdface.facelog.dborm.face.FlFaceManager;
import net.gdface.facelog.dborm.person.FlPersonBean;
import net.gdface.facelog.dborm.person.FlPersonManager;
import net.gdface.facelog.dborm.device.FlDeviceBean;
import net.gdface.facelog.dborm.device.FlDeviceManager;
/**
* Handles database calls (save, load, count, etc...) for the fl_image table.
* Remarks: 图像信息存储表,用于存储系统中所有用到的图像数据,表中只包含图像基本信息,图像二进制源数据存在在fl_store中(md5对应)
* @author sql2java
*/
public class FlImageManager extends TableManager.BaseAdapter
{
/**
* Tablename.
*/
public static final String TABLE_NAME="fl_image";
/**
* Contains all the primary key fields of the fl_image table.
*/
public static final String[] PRIMARYKEY_NAMES =
{
"md5"
};
@Override
public String getTableName() {
return TABLE_NAME;
}
@Override
public String getFields() {
return FL_IMAGE_FIELDS;
}
@Override
public String getFullFields() {
return FL_IMAGE_FULL_FIELDS;
}
@Override
public String[] getPrimarykeyNames() {
return PRIMARYKEY_NAMES;
}
private static FlImageManager singleton = new FlImageManager();
protected FlImageManager(){}
/**
* Get the FlImageManager singleton.
*
* @return FlImageManager
*/
public static FlImageManager getInstance()
{
return singleton;
}
/**
* Creates a new FlImageBean instance.
*
* @return the new FlImageBean
*/
public FlImageBean createBean()
{
return new FlImageBean();
}
@Override
protected Class beanType(){
return FlImageBean.class;
}
protected FlFaceManager instanceOfFlFaceManager(){
return FlFaceManager.getInstance();
}
protected FlPersonManager instanceOfFlPersonManager(){
return FlPersonManager.getInstance();
}
protected FlDeviceManager instanceOfFlDeviceManager(){
return FlDeviceManager.getInstance();
}
//////////////////////////////////////
// PRIMARY KEY METHODS
//////////////////////////////////////
//1
/**
* Loads a {@link FlImageBean} from the fl_image using primary key fields.
*
* @param md5 String - PK# 1
* @return a unique FlImageBean or {@code null} if not found or have null argument
* @throws DaoException
*/
public FlImageBean loadByPrimaryKey(String md5) throws DaoException
{
try{
return loadByPrimaryKeyChecked(md5);
}catch(ObjectRetrievalException e){
// not found
return null;
}
}
//1.1
/**
* Loads a {@link FlImageBean} from the fl_image using primary key fields.
*
* @param md5 String - PK# 1
* @return a unique FlImageBean
* @throws ObjectRetrievalException if not found
* @throws DaoException
*/
@SuppressWarnings("unused")
public FlImageBean loadByPrimaryKeyChecked(String md5) throws DaoException
{
if(null == md5){
throw new ObjectRetrievalException(new NullPointerException());
}
Connection c = null;
PreparedStatement ps = null;
try
{
c = this.getConnection();
StringBuilder sql = new StringBuilder("SELECT " + FL_IMAGE_FIELDS + " FROM fl_image WHERE md5=?");
// System.out.println("loadByPrimaryKey: " + sql);
ps = c.prepareStatement(sql.toString(),
ResultSet.TYPE_SCROLL_INSENSITIVE,
ResultSet.CONCUR_READ_ONLY);
if (md5 == null) { ps.setNull(FL_IMAGE_ID_MD5 + 1, Types.CHAR); } else { ps.setString(FL_IMAGE_ID_MD5 + 1, md5); }
List pReturn = this.loadByPreparedStatementAsList(ps);
if (1 == pReturn.size()) {
return pReturn.get(0);
} else {
throw new ObjectRetrievalException();
}
}
catch(ObjectRetrievalException e)
{
throw e;
}
catch(SQLException e)
{
throw new DataRetrievalException(e);
}
finally
{
this.getManager().close(ps);
this.freeConnection(c);
}
}
//1.2
@Override
public FlImageBean loadByPrimaryKey(FlImageBean bean) throws DaoException
{
return bean==null?null:loadByPrimaryKey(bean.getMd5());
}
//1.2.2
@Override
public FlImageBean loadByPrimaryKeyChecked(FlImageBean bean) throws DaoException
{
if(null == bean){
throw new NullPointerException();
}
return loadByPrimaryKeyChecked(bean.getMd5());
}
//1.3
/**
* Loads a {@link FlImageBean} from the fl_image using primary key fields.
* @param keys primary keys value:
* @return a unique {@link FlImageBean} or {@code null} if not found
* @see #loadByPrimaryKey(String md5)
*/
@Override
public FlImageBean loadByPrimaryKey(Object ...keys) throws DaoException{
if(null == keys){
throw new NullPointerException();
}
if(keys.length != FL_IMAGE_PK_COUNT){
throw new IllegalArgumentException("argument number mismatch with primary key number");
}
if(null == keys[0]){
return null;
}
return loadByPrimaryKey((String)keys[0]);
}
//1.3.2
@Override
public FlImageBean loadByPrimaryKeyChecked(Object ...keys) throws DaoException{
if(null == keys){
throw new NullPointerException();
}
if(keys.length != FL_IMAGE_PK_COUNT){
throw new IllegalArgumentException("argument number mismatch with primary key number");
}
if(! (keys[0] instanceof String)){
throw new IllegalArgumentException("invalid type for the No.1 argument,expected type:String");
}
return loadByPrimaryKeyChecked((String)keys[0]);
}
//1.4
/**
* Returns true if this fl_image contains row with primary key fields.
* @param md5 String - PK# 1
* @throws DaoException
*/
@SuppressWarnings("unused")
public boolean existsPrimaryKey(String md5) throws DaoException
{
if(null == md5){
return false;
}
Connection c = null;
PreparedStatement ps = null;
try{
c = this.getConnection();
StringBuilder sql = new StringBuilder("SELECT COUNT(*) AS MCOUNT FROM fl_image WHERE md5=?");
// System.out.println("loadByPrimaryKey: " + sql);
ps = c.prepareStatement(sql.toString(),
ResultSet.TYPE_SCROLL_INSENSITIVE,
ResultSet.CONCUR_READ_ONLY);
if (md5 == null) { ps.setNull(FL_IMAGE_ID_MD5 + 1, Types.CHAR); } else { ps.setString(FL_IMAGE_ID_MD5 + 1, md5); }
return 1 == this.countByPreparedStatement(ps);
}catch(SQLException e){
throw new ObjectRetrievalException(e);
}finally{
this.getManager().close(ps);
this.freeConnection(c);
}
}
//1.6
/**
* Return true if this fl_image contains row with primary key fields.
* @param bean
* @throws DaoException
* @return false if primary kes has null
*/
@Override
public boolean existsByPrimaryKey(FlImageBean bean) throws DaoException
{
if(null == bean || null == bean.getMd5()){
return false;
}
int modified = bean.getModified();
try{
bean.resetModifiedExceptPrimaryKeys();
return 1 == countUsingTemplate(bean);
}finally{
bean.setModified(modified);
}
}
//1.7
@Override
public FlImageBean checkDuplicate(FlImageBean bean) throws DaoException{
if(existsByPrimaryKey(bean)){
throw new ObjectRetrievalException("Duplicate entry ("+ bean.getMd5() +") for key 'PRIMARY'");
}
return bean;
}
//1.4.1
/**
* Check duplicated row by primary keys,if row exists throw {@link ObjectRetrievalException}
* @param md5 String
* @throws DaoException
* @see #existsPrimaryKey(String md5)
*/
public String checkDuplicate(String md5) throws DaoException
{
if(existsPrimaryKey(md5)){
throw new ObjectRetrievalException("Duplicate entry '"+ md5 +"' for key 'PRIMARY'");
}
return md5;
}
//2
/**
* Delete row according to its primary keys.
* all keys must not be null
*
* @param md5 String - PK# 1
* @return the number of deleted rows
* @throws DaoException
* @see #delete(FlImageBean)
*/
public int deleteByPrimaryKey(String md5) throws DaoException
{
FlImageBean bean=createBean();
bean.setMd5(md5);
return this.delete(bean);
}
//2.2
/**
* Delete row according to primary keys of bean.
*
* @param bean will be deleted ,all keys must not be null
* @return the number of deleted rows,0 returned if bean is null
* @throws DaoException
*/
@Override
public int delete(FlImageBean bean) throws DaoException
{
if(null == bean || null == bean.getMd5()){
return 0;
}
Connection c = null;
PreparedStatement ps = null;
try
{
// listener callback
this.listenerContainer.beforeDelete(bean);
c = this.getConnection();
StringBuilder sql = new StringBuilder("DELETE FROM fl_image WHERE md5=?");
// System.out.println("deleteByPrimaryKey: " + sql);
ps = c.prepareStatement(sql.toString(),
ResultSet.TYPE_SCROLL_INSENSITIVE,
ResultSet.CONCUR_READ_ONLY);
if (bean.getMd5() == null) { ps.setNull(FL_IMAGE_ID_MD5 + 1, Types.CHAR); } else { ps.setString(FL_IMAGE_ID_MD5 + 1, bean.getMd5()); }
int rows=ps.executeUpdate();
if(rows>0){
// listener callback
this.listenerContainer.afterDelete(bean);
}
return rows;
}
catch(SQLException e)
{
throw new DataAccessException(e);
}
finally
{
// listener callback
this.listenerContainer.done();
this.getManager().close(ps);
this.freeConnection(c);
}
}
//2.1
/**
* Delete row according to its primary keys.
*
* @param keys primary keys value:
* @return the number of deleted rows
* @see #delete(FlImageBean)
*/
@Override
public int deleteByPrimaryKey(Object ...keys) throws DaoException{
if(null == keys){
throw new NullPointerException();
}
if(keys.length != FL_IMAGE_PK_COUNT){
throw new IllegalArgumentException("argument number mismatch with primary key number");
}
FlImageBean bean = createBean();
if(null != keys[0] && !(keys[0] instanceof String)){
throw new IllegalArgumentException("invalid type for the No.1 argument,expected type:String");
}
bean.setMd5((String)keys[0]);
return delete(bean);
}
//////////////////////////////////////
// IMPORT KEY GENERIC METHOD
//////////////////////////////////////
private static final Class>[] IMPORTED_BEAN_TYPES = new Class>[]{FlFaceBean.class,FlPersonBean.class};
/**
* @see #getImportedBeansAsList(FlImageBean,int)
*/
@SuppressWarnings("unchecked")
@Override
public > T[] getImportedBeans(FlImageBean bean, int ikIndex) throws DaoException {
return getImportedBeansAsList(bean, ikIndex).toArray((T[])java.lang.reflect.Array.newInstance(IMPORTED_BEAN_TYPES[ikIndex],0));
}
/**
* Retrieves imported T objects by ikIndex.
* @param
*
* - {@link Constant#FL_IMAGE_IK_FL_FACE_IMAGE_MD5} - {@link FlFaceBean}
* - {@link Constant#FL_IMAGE_IK_FL_PERSON_IMAGE_MD5} - {@link FlPersonBean}
*
* @param bean the {@link FlImageBean} object to use
* @param ikIndex valid values: {@link Constant#FL_IMAGE_IK_FL_FACE_IMAGE_MD5},{@link Constant#FL_IMAGE_IK_FL_PERSON_IMAGE_MD5}
* @return the associated T beans or {@code null} if {@code bean} is {@code null}
* @throws DaoException
*/
@SuppressWarnings("unchecked")
@Override
public > List getImportedBeansAsList(FlImageBean bean,int ikIndex)throws DaoException{
switch(ikIndex){
case FL_IMAGE_IK_FL_FACE_IMAGE_MD5:
return (List)this.getFaceBeansByImageMd5AsList(bean);
case FL_IMAGE_IK_FL_PERSON_IMAGE_MD5:
return (List)this.getPersonBeansByImageMd5AsList(bean);
default:
throw new IllegalArgumentException(String.format("invalid ikIndex %d", ikIndex));
}
}
/**
* Set the T objects as imported beans of bean object by ikIndex.
* @param see also {@link #getImportedBeansAsList(FlImageBean,int)}
* @param bean the {@link FlImageBean} object to use
* @param importedBeans the FlPersonBean array to associate to the {@link FlImageBean}
* @param ikIndex valid values: see also {@link #getImportedBeansAsList(FlImageBean,int)}
* @return importedBeans always
* @throws DaoException
*/
@SuppressWarnings("unchecked")
@Override
public > T[] setImportedBeans(FlImageBean bean,T[] importedBeans,int ikIndex)throws DaoException{
switch(ikIndex){
case FL_IMAGE_IK_FL_FACE_IMAGE_MD5:
return (T[])setFaceBeansByImageMd5(bean,(FlFaceBean[])importedBeans);
case FL_IMAGE_IK_FL_PERSON_IMAGE_MD5:
return (T[])setPersonBeansByImageMd5(bean,(FlPersonBean[])importedBeans);
default:
throw new IllegalArgumentException(String.format("invalid ikIndex %d", ikIndex));
}
}
/**
* Set the importedBeans associates to the bean by ikIndex
* @param see also {@link #getImportedBeansAsList(FlImageBean,int)}
* @param bean the {@link FlImageBean} object to use
* @param importedBeans the T object to associate to the {@link FlImageBean}
* @param ikIndex valid values: see also {@link #getImportedBeansAsList(FlImageBean,int)}
* @return importedBeans always
* @throws DaoException
*/
@SuppressWarnings("unchecked")
@Override
public ,C extends java.util.Collection> C setImportedBeans(FlImageBean bean,C importedBeans,int ikIndex)throws DaoException{
switch(ikIndex){
case FL_IMAGE_IK_FL_FACE_IMAGE_MD5:
return (C)setFaceBeansByImageMd5(bean,(java.util.Collection)importedBeans);
case FL_IMAGE_IK_FL_PERSON_IMAGE_MD5:
return (C)setPersonBeansByImageMd5(bean,(java.util.Collection)importedBeans);
default:
throw new IllegalArgumentException(String.format("invalid ikIndex %d", ikIndex));
}
}
//////////////////////////////////////
// GET/SET IMPORTED KEY BEAN METHOD
//////////////////////////////////////
//3.1 GET IMPORTED
/**
* Retrieves the {@link FlFaceBean} object from the fl_face.image_md5 field.
* FK_NAME : fl_face_ibfk_1
* @param bean the {@link FlImageBean}
* @return the associated {@link FlFaceBean} beans or {@code null} if {@code bean} is {@code null}
* @throws DaoException
*/
public FlFaceBean[] getFaceBeansByImageMd5(FlImageBean bean) throws DaoException
{
return getFaceBeansByImageMd5AsList(bean).toArray(new FlFaceBean[0]);
}
//3.1.2 GET IMPORTED
/**
* Retrieves the {@link FlFaceBean} object from the fl_face.image_md5 field.
* FK_NAME : fl_face_ibfk_1
* @param md5OfImage String - PK# 1
* @return the associated {@link FlFaceBean} beans or {@code null} if {@code bean} is {@code null}
* @throws DaoException
*/
public FlFaceBean[] getFaceBeansByImageMd5(String md5OfImage) throws DaoException
{
FlImageBean bean = createBean();
bean.setMd5(md5OfImage);
return getFaceBeansByImageMd5(bean);
}
//3.2 GET IMPORTED
/**
* Retrieves the {@link FlFaceBean} object from fl_face.image_md5 field.
* FK_NAME:fl_face_ibfk_1
* @param bean the {@link FlImageBean}
* @return the associated {@link FlFaceBean} beans
* @throws DaoException
*/
public List getFaceBeansByImageMd5AsList(FlImageBean bean) throws DaoException
{
return getFaceBeansByImageMd5AsList(bean,1,-1);
}
//3.2.2 GET IMPORTED
/**
* Retrieves the {@link FlFaceBean} object from fl_face.image_md5 field.
* FK_NAME:fl_face_ibfk_1
* @param md5OfImage String - PK# 1
* @return the associated {@link FlFaceBean} beans
* @throws DaoException
*/
public List getFaceBeansByImageMd5AsList(String md5OfImage) throws DaoException
{
FlImageBean bean = createBean();
bean.setMd5(md5OfImage);
return getFaceBeansByImageMd5AsList(bean);
}
//3.2.4 GET IMPORTED
/**
* Retrieves the {@link FlFaceBean} object from fl_face.image_md5 field,
* given the start row and number of rows.
* FK_NAME:fl_face_ibfk_1
* @param bean the {@link FlImageBean}
* @param startRow the start row to be used (first row = 1, last row=-1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @return the associated {@link FlFaceBean} beans
* @throws DaoException
*/
public List getFaceBeansByImageMd5AsList(FlImageBean bean,int startRow, int numRows) throws DaoException
{
if(null == bean){
return new java.util.ArrayList();
}
FlFaceBean other = new FlFaceBean();
other.setImageMd5(bean.getMd5());
return instanceOfFlFaceManager().loadUsingTemplateAsList(other,startRow,numRows);
}
//3.3 SET IMPORTED
/**
* set the {@link FlFaceBean} object array associate to FlImageBean by the fl_face.image_md5 field.
* FK_NAME : fl_face_ibfk_1
* @param bean the referenced {@link FlImageBean}
* @param importedBeans imported beans from fl_face
* @return importedBeans always
* @throws DaoException
* @see FlFaceManager#setReferencedByImageMd5(FlFaceBean, FlImageBean)
*/
public FlFaceBean[] setFaceBeansByImageMd5(FlImageBean bean , FlFaceBean[] importedBeans) throws DaoException
{
if(null != importedBeans){
for( FlFaceBean importBean : importedBeans ){
instanceOfFlFaceManager().setReferencedByImageMd5(importBean , bean);
}
}
return importedBeans;
}
//3.4 SET IMPORTED
/**
* set the {@link FlFaceBean} object collection associate to FlImageBean by the fl_face.image_md5 field.
* FK_NAME:fl_face_ibfk_1
* @param bean the referenced {@link FlImageBean}
* @param importedBeans imported beans from fl_face
* @return importedBeans always
* @throws DaoException
* @see FlFaceManager#setReferencedByImageMd5(FlFaceBean, FlImageBean)
*/
public > C setFaceBeansByImageMd5(FlImageBean bean , C importedBeans) throws DaoException
{
if(null != importedBeans){
for( FlFaceBean importBean : importedBeans ){
instanceOfFlFaceManager().setReferencedByImageMd5(importBean , bean);
}
}
return importedBeans;
}
//3.1 GET IMPORTED
/**
* Retrieves the {@link FlPersonBean} object from the fl_person.image_md5 field.
* FK_NAME : fl_person_ibfk_2
* @param bean the {@link FlImageBean}
* @return the associated {@link FlPersonBean} beans or {@code null} if {@code bean} is {@code null}
* @throws DaoException
*/
public FlPersonBean[] getPersonBeansByImageMd5(FlImageBean bean) throws DaoException
{
return getPersonBeansByImageMd5AsList(bean).toArray(new FlPersonBean[0]);
}
//3.1.2 GET IMPORTED
/**
* Retrieves the {@link FlPersonBean} object from the fl_person.image_md5 field.
* FK_NAME : fl_person_ibfk_2
* @param md5OfImage String - PK# 1
* @return the associated {@link FlPersonBean} beans or {@code null} if {@code bean} is {@code null}
* @throws DaoException
*/
public FlPersonBean[] getPersonBeansByImageMd5(String md5OfImage) throws DaoException
{
FlImageBean bean = createBean();
bean.setMd5(md5OfImage);
return getPersonBeansByImageMd5(bean);
}
//3.2 GET IMPORTED
/**
* Retrieves the {@link FlPersonBean} object from fl_person.image_md5 field.
* FK_NAME:fl_person_ibfk_2
* @param bean the {@link FlImageBean}
* @return the associated {@link FlPersonBean} beans
* @throws DaoException
*/
public List getPersonBeansByImageMd5AsList(FlImageBean bean) throws DaoException
{
return getPersonBeansByImageMd5AsList(bean,1,-1);
}
//3.2.2 GET IMPORTED
/**
* Retrieves the {@link FlPersonBean} object from fl_person.image_md5 field.
* FK_NAME:fl_person_ibfk_2
* @param md5OfImage String - PK# 1
* @return the associated {@link FlPersonBean} beans
* @throws DaoException
*/
public List getPersonBeansByImageMd5AsList(String md5OfImage) throws DaoException
{
FlImageBean bean = createBean();
bean.setMd5(md5OfImage);
return getPersonBeansByImageMd5AsList(bean);
}
//3.2.4 GET IMPORTED
/**
* Retrieves the {@link FlPersonBean} object from fl_person.image_md5 field,
* given the start row and number of rows.
* FK_NAME:fl_person_ibfk_2
* @param bean the {@link FlImageBean}
* @param startRow the start row to be used (first row = 1, last row=-1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @return the associated {@link FlPersonBean} beans
* @throws DaoException
*/
public List getPersonBeansByImageMd5AsList(FlImageBean bean,int startRow, int numRows) throws DaoException
{
if(null == bean){
return new java.util.ArrayList();
}
FlPersonBean other = new FlPersonBean();
other.setImageMd5(bean.getMd5());
return instanceOfFlPersonManager().loadUsingTemplateAsList(other,startRow,numRows);
}
//3.3 SET IMPORTED
/**
* set the {@link FlPersonBean} object array associate to FlImageBean by the fl_person.image_md5 field.
* FK_NAME : fl_person_ibfk_2
* @param bean the referenced {@link FlImageBean}
* @param importedBeans imported beans from fl_person
* @return importedBeans always
* @throws DaoException
* @see FlPersonManager#setReferencedByImageMd5(FlPersonBean, FlImageBean)
*/
public FlPersonBean[] setPersonBeansByImageMd5(FlImageBean bean , FlPersonBean[] importedBeans) throws DaoException
{
if(null != importedBeans){
for( FlPersonBean importBean : importedBeans ){
instanceOfFlPersonManager().setReferencedByImageMd5(importBean , bean);
}
}
return importedBeans;
}
//3.4 SET IMPORTED
/**
* set the {@link FlPersonBean} object collection associate to FlImageBean by the fl_person.image_md5 field.
* FK_NAME:fl_person_ibfk_2
* @param bean the referenced {@link FlImageBean}
* @param importedBeans imported beans from fl_person
* @return importedBeans always
* @throws DaoException
* @see FlPersonManager#setReferencedByImageMd5(FlPersonBean, FlImageBean)
*/
public > C setPersonBeansByImageMd5(FlImageBean bean , C importedBeans) throws DaoException
{
if(null != importedBeans){
for( FlPersonBean importBean : importedBeans ){
instanceOfFlPersonManager().setReferencedByImageMd5(importBean , bean);
}
}
return importedBeans;
}
//3.5 SYNC SAVE
/**
* Save the FlImageBean bean and referenced beans and imported beans into the database.
*
* @param bean the {@link FlImageBean} bean to be saved
* @param refDeviceByDeviceId the {@link FlDeviceBean} bean referenced by {@link FlImageBean}
* @param impFaceByImageMd5 the {@link FlFaceBean} beans refer to {@link FlImageBean}
* @param impPersonByImageMd5 the {@link FlPersonBean} beans refer to {@link FlImageBean}
* @return the inserted or updated {@link FlImageBean} bean
* @throws DaoException
*/
public FlImageBean save(FlImageBean bean
, FlDeviceBean refDeviceByDeviceId
, FlFaceBean[] impFaceByImageMd5 , FlPersonBean[] impPersonByImageMd5 ) throws DaoException
{
if(null == bean) {
return null;
}
if(null != refDeviceByDeviceId){
this.setReferencedByDeviceId(bean,refDeviceByDeviceId);
}
bean = this.save( bean );
if(null != impFaceByImageMd5){
this.setFaceBeansByImageMd5(bean,impFaceByImageMd5);
instanceOfFlFaceManager().save( impFaceByImageMd5 );
}
if(null != impPersonByImageMd5){
this.setPersonBeansByImageMd5(bean,impPersonByImageMd5);
instanceOfFlPersonManager().save( impPersonByImageMd5 );
}
return bean;
}
//3.6 SYNC SAVE AS TRANSACTION
/**
* Transaction version for sync save
* @see #save(FlImageBean , FlDeviceBean , FlFaceBean[] , FlPersonBean[] )
*/
public FlImageBean saveAsTransaction(final FlImageBean bean
,final FlDeviceBean refDeviceByDeviceId
,final FlFaceBean[] impFaceByImageMd5 ,final FlPersonBean[] impPersonByImageMd5 ) throws DaoException
{
return this.runAsTransaction(new Callable(){
@Override
public FlImageBean call() throws Exception {
return save(bean , refDeviceByDeviceId , impFaceByImageMd5 , impPersonByImageMd5 );
}});
}
//3.7 SYNC SAVE
/**
* Save the FlImageBean bean and referenced beans and imported beans into the database.
*
* @param bean the {@link FlImageBean} bean to be saved
* @param refDeviceByDeviceId the {@link FlDeviceBean} bean referenced by {@link FlImageBean}
* @param impFaceByImageMd5 the {@link FlFaceBean} bean refer to {@link FlImageBean}
* @param impPersonByImageMd5 the {@link FlPersonBean} bean refer to {@link FlImageBean}
* @return the inserted or updated {@link FlImageBean} bean
* @throws DaoException
*/
public FlImageBean save(FlImageBean bean
, FlDeviceBean refDeviceByDeviceId
, java.util.Collection impFaceByImageMd5 , java.util.Collection impPersonByImageMd5 ) throws DaoException
{
if(null == bean) {
return null;
}
if(null != refDeviceByDeviceId){
this.setReferencedByDeviceId(bean,refDeviceByDeviceId);
}
bean = this.save( bean );
if(null != impFaceByImageMd5){
this.setFaceBeansByImageMd5(bean,impFaceByImageMd5);
instanceOfFlFaceManager().save( impFaceByImageMd5 );
}
if(null != impPersonByImageMd5){
this.setPersonBeansByImageMd5(bean,impPersonByImageMd5);
instanceOfFlPersonManager().save( impPersonByImageMd5 );
}
return bean;
}
//3.8 SYNC SAVE AS TRANSACTION
/**
* Transaction version for sync save
* @see #save(FlImageBean , FlDeviceBean , java.util.Collection , java.util.Collection )
* @throws DaoException
*/
public FlImageBean saveAsTransaction(final FlImageBean bean
,final FlDeviceBean refDeviceByDeviceId
,final java.util.Collection impFaceByImageMd5 ,final java.util.Collection impPersonByImageMd5 ) throws DaoException
{
return this.runAsTransaction(new Callable(){
@Override
public FlImageBean call() throws Exception {
return save(bean , refDeviceByDeviceId , impFaceByImageMd5 , impPersonByImageMd5 );
}});
}
private static final int SYNC_SAVE_ARG_LEN = 3;
private static final int SYNC_SAVE_ARG_0 = 0;
private static final int SYNC_SAVE_ARG_1 = 1;
private static final int SYNC_SAVE_ARG_2 = 2;
//3.9 SYNC SAVE
/**
* Save the FlImageBean bean and referenced beans and imported beans (array) into the database.
*
* @param bean the {@link FlImageBean} bean to be saved
* @param inputs referenced beans or imported beans
* see also {@link #save(FlImageBean , FlDeviceBean , FlFaceBean[] , FlPersonBean[] )}
* @return the inserted or updated {@link FlImageBean} bean
* @throws DaoException
*/
@Override
public FlImageBean save(FlImageBean bean,Object ...inputs) throws DaoException
{
if(null == inputs){
return save(bean);
}
if(inputs.length > SYNC_SAVE_ARG_LEN){
throw new IllegalArgumentException("too many dynamic arguments,max dynamic arguments number: 3");
}
Object[] args = new Object[SYNC_SAVE_ARG_LEN];
System.arraycopy(inputs, 0, args, 0, inputs.length);
if( null != args[SYNC_SAVE_ARG_0] && !(args[SYNC_SAVE_ARG_0] instanceof FlDeviceBean)){
throw new IllegalArgumentException("invalid type for the No.1 dynamic argument,expected type:FlDeviceBean");
}
if( null != args[SYNC_SAVE_ARG_1] && !(args[SYNC_SAVE_ARG_1] instanceof FlFaceBean[])){
throw new IllegalArgumentException("invalid type for the No.2 dynamic argument,expected type:FlFaceBean[]");
}
if( null != args[SYNC_SAVE_ARG_2] && !(args[SYNC_SAVE_ARG_2] instanceof FlPersonBean[])){
throw new IllegalArgumentException("invalid type for the No.3 dynamic argument,expected type:FlPersonBean[]");
}
return save(bean,
(FlDeviceBean)args[SYNC_SAVE_ARG_0],
(FlFaceBean[])args[SYNC_SAVE_ARG_1],
(FlPersonBean[])args[SYNC_SAVE_ARG_2]);
}
//3.10 SYNC SAVE
/**
* Save the FlImageBean bean and referenced beans and imported beans (collection) into the database.
*
* @param bean the {@link FlImageBean} bean to be saved
* @param inputs referenced beans or imported beans
* see also {@link #save(FlImageBean , FlDeviceBean , java.util.Collection , java.util.Collection )}
* @return the inserted or updated {@link FlImageBean} bean
* @throws DaoException
*/
@SuppressWarnings("unchecked")
@Override
public FlImageBean saveCollection(FlImageBean bean,Object ...inputs) throws DaoException
{
if(null == inputs){
return save(bean);
}
if(inputs.length > SYNC_SAVE_ARG_LEN){
throw new IllegalArgumentException("too many dynamic arguments,max dynamic arguments number: 3");
}
Object[] args = new Object[SYNC_SAVE_ARG_LEN];
System.arraycopy(inputs, 0, args, 0, inputs.length);
if( null != args[SYNC_SAVE_ARG_0] && !(args[SYNC_SAVE_ARG_0] instanceof FlDeviceBean)){
throw new IllegalArgumentException("invalid type for the No.1 argument,expected type:FlDeviceBean");
}
if( null != args[SYNC_SAVE_ARG_1] && !(args[SYNC_SAVE_ARG_1] instanceof java.util.Collection)){
throw new IllegalArgumentException("invalid type for the No.2 argument,expected type:java.util.Collection");
}
if( null != args[SYNC_SAVE_ARG_2] && !(args[SYNC_SAVE_ARG_2] instanceof java.util.Collection)){
throw new IllegalArgumentException("invalid type for the No.3 argument,expected type:java.util.Collection");
}
return save(bean,
(FlDeviceBean)args[SYNC_SAVE_ARG_0],
(java.util.Collection)args[SYNC_SAVE_ARG_1],
(java.util.Collection)args[SYNC_SAVE_ARG_2]);
}
//////////////////////////////////////
// FOREIGN KEY GENERIC METHOD
//////////////////////////////////////
/**
* Retrieves the bean object referenced by fkIndex.
* @param
*
* - {@link Constant#FL_IMAGE_FK_DEVICE_ID} - {@link FlDeviceBean}
*
* @param bean the {@link FlImageBean} object to use
* @param fkIndex valid values:
* {@link Constant#FL_IMAGE_FK_DEVICE_ID}
* @return the associated T bean or {@code null} if {@code bean} or {@code beanToSet} is {@code null}
* @throws DaoException
*/
@SuppressWarnings("unchecked")
@Override
public > T getReferencedBean(FlImageBean bean,int fkIndex)throws DaoException{
switch(fkIndex){
case FL_IMAGE_FK_DEVICE_ID:
return (T)this.getReferencedByDeviceId(bean);
default:
throw new IllegalArgumentException(String.format("invalid fkIndex %d", fkIndex));
}
}
/**
* Associates the {@link FlImageBean} object to the bean object by fkIndex field.
*
* @param see also {@link #getReferencedBean(FlImageBean,int)}
* @param bean the {@link FlImageBean} object to use
* @param beanToSet the T object to associate to the {@link FlImageBean}
* @param fkIndex valid values: see also {@link #getReferencedBean(FlImageBean,int)}
* @return always beanToSet saved
* @throws DaoException
*/
@SuppressWarnings("unchecked")
@Override
public > T setReferencedBean(FlImageBean bean,T beanToSet,int fkIndex)throws DaoException{
switch(fkIndex){
case FL_IMAGE_FK_DEVICE_ID:
return (T)this.setReferencedByDeviceId(bean, (FlDeviceBean)beanToSet);
default:
throw new IllegalArgumentException(String.format("invalid fkIndex %d", fkIndex));
}
}
//////////////////////////////////////
// GET/SET FOREIGN KEY BEAN METHOD
//////////////////////////////////////
//5.1 GET REFERENCED VALUE
/**
* Retrieves the {@link FlDeviceBean} object referenced by {@link FlImageBean#getDeviceId}() field.
* FK_NAME : fl_image_ibfk_1
* @param bean the {@link FlImageBean}
* @return the associated {@link FlDeviceBean} bean or {@code null} if {@code bean} is {@code null}
* @throws DaoException
*/
public FlDeviceBean getReferencedByDeviceId(FlImageBean bean) throws DaoException
{
if(null == bean){
return null;
}
bean.setReferencedByDeviceId(instanceOfFlDeviceManager().loadByPrimaryKey(bean.getDeviceId()));
return bean.getReferencedByDeviceId();
}
//5.2 SET REFERENCED
/**
* Associates the {@link FlImageBean} object to the {@link FlDeviceBean} object by {@link FlImageBean#getDeviceId}() field.
*
* @param bean the {@link FlImageBean} object to use
* @param beanToSet the {@link FlDeviceBean} object to associate to the {@link FlImageBean} .
* @return always beanToSet saved
* @throws DaoException
*/
public FlDeviceBean setReferencedByDeviceId(FlImageBean bean, FlDeviceBean beanToSet) throws DaoException
{
if(null != bean){
instanceOfFlDeviceManager().save(beanToSet);
bean.setReferencedByDeviceId(beanToSet);
if( null == beanToSet){
bean.setDeviceId(null);
}else{
bean.setDeviceId(beanToSet.getId());
}
}
return beanToSet;
}
//////////////////////////////////////
// SQL 'WHERE' METHOD
//////////////////////////////////////
//11
/**
* Deletes rows from the fl_image table using a 'where' clause.
* It is up to you to pass the 'WHERE' in your where clauses.
*
Attention, if 'WHERE' is omitted it will delete all records.
*
* @param where the sql 'where' clause
* @return the number of deleted rows
* @throws DaoException
*/
@Override
public int deleteByWhere(String where) throws DaoException
{
if( !this.listenerContainer.isEmpty()){
final DeleteBeanAction action = new DeleteBeanAction();
this.loadByWhere(where,action);
return action.getCount();
}
Connection c = null;
PreparedStatement ps = null;
try
{
c = this.getConnection();
StringBuilder sql = new StringBuilder("DELETE FROM fl_image " + where);
// System.out.println("deleteByWhere: " + sql);
ps = c.prepareStatement(sql.toString());
return ps.executeUpdate();
}
catch(SQLException e)
{
throw new DataAccessException(e);
}
finally
{
this.getManager().close(ps);
this.freeConnection(c);
}
}
//_____________________________________________________________________
//
// SAVE
//_____________________________________________________________________
//13
@Override
public FlImageBean insert(FlImageBean bean) throws DaoException
{
// mini checks
if (null == bean || !bean.isModified()) {
return bean;
}
if (!bean.isNew()){
return this.update(bean);
}
Connection c = null;
PreparedStatement ps = null;
StringBuilder sql = null;
try
{
c = this.getConnection();
// listener callback
this.listenerContainer.beforeInsert(bean);
int dirtyCount = 0;
sql = new StringBuilder("INSERT into fl_image (");
if (bean.checkMd5Modified()) {
if (dirtyCount>0) {
sql.append(",");
}
sql.append("md5");
dirtyCount++;
}
if (bean.checkFormatModified()) {
if (dirtyCount>0) {
sql.append(",");
}
sql.append("format");
dirtyCount++;
}
if (bean.checkWidthModified()) {
if (dirtyCount>0) {
sql.append(",");
}
sql.append("width");
dirtyCount++;
}
if (bean.checkHeightModified()) {
if (dirtyCount>0) {
sql.append(",");
}
sql.append("height");
dirtyCount++;
}
if (bean.checkDepthModified()) {
if (dirtyCount>0) {
sql.append(",");
}
sql.append("depth");
dirtyCount++;
}
if (bean.checkFaceNumModified()) {
if (dirtyCount>0) {
sql.append(",");
}
sql.append("face_num");
dirtyCount++;
}
if (bean.checkThumbMd5Modified()) {
if (dirtyCount>0) {
sql.append(",");
}
sql.append("thumb_md5");
dirtyCount++;
}
if (bean.checkDeviceIdModified()) {
if (dirtyCount>0) {
sql.append(",");
}
sql.append("device_id");
dirtyCount++;
}
sql.append(") values (");
if(dirtyCount > 0) {
sql.append("?");
for(int i = 1; i < dirtyCount; i++) {
sql.append(",?");
}
}
sql.append(")");
// System.out.println("insert : " + sql.toString());
ps = c.prepareStatement(sql.toString(), ResultSet.TYPE_SCROLL_INSENSITIVE, ResultSet.CONCUR_READ_ONLY);
this.fillPreparedStatement(ps, bean, SEARCH_EXACT,true);
ps.executeUpdate();
bean.isNew(false);
bean.resetIsModified();
// listener callback
this.listenerContainer.afterInsert(bean);
return bean;
}
catch(SQLException e)
{
throw new DataAccessException(e);
}
finally
{
// listener callback
this.listenerContainer.done();
sql = null;
this.getManager().close(ps);
this.freeConnection(c);
}
}
//14
@Override
public FlImageBean update(FlImageBean bean) throws DaoException
{
// mini checks
if (null == bean || !bean.isModified()) {
return bean;
}
if (bean.isNew()){
return this.insert(bean);
}
Connection c = null;
PreparedStatement ps = null;
StringBuilder sql = null;
try
{
c = this.getConnection();
// listener callback
this.listenerContainer.beforeUpdate(bean);
sql = new StringBuilder("UPDATE fl_image SET ");
boolean useComma=false;
if (bean.checkMd5Modified()) {
if (useComma) {
sql.append(", ");
} else {
useComma=true;
}
sql.append("md5=?");
}
if (bean.checkFormatModified()) {
if (useComma) {
sql.append(", ");
} else {
useComma=true;
}
sql.append("format=?");
}
if (bean.checkWidthModified()) {
if (useComma) {
sql.append(", ");
} else {
useComma=true;
}
sql.append("width=?");
}
if (bean.checkHeightModified()) {
if (useComma) {
sql.append(", ");
} else {
useComma=true;
}
sql.append("height=?");
}
if (bean.checkDepthModified()) {
if (useComma) {
sql.append(", ");
} else {
useComma=true;
}
sql.append("depth=?");
}
if (bean.checkFaceNumModified()) {
if (useComma) {
sql.append(", ");
} else {
useComma=true;
}
sql.append("face_num=?");
}
if (bean.checkThumbMd5Modified()) {
if (useComma) {
sql.append(", ");
} else {
useComma=true;
}
sql.append("thumb_md5=?");
}
if (bean.checkDeviceIdModified()) {
if (useComma) {
sql.append(", ");
} else {
useComma=true;
}
sql.append("device_id=?");
}
sql.append(" WHERE ");
sql.append("md5=?");
// System.out.println("update : " + sql.toString());
ps = c.prepareStatement(sql.toString(),
ResultSet.TYPE_SCROLL_INSENSITIVE,
ResultSet.CONCUR_READ_ONLY);
int dirtyCount = this.fillPreparedStatement(ps, bean, SEARCH_EXACT,true);
if (dirtyCount == 0) {
// System.out.println("The bean to look is not initialized... do not update.");
return bean;
}
if (bean.getMd5() == null) { ps.setNull(++dirtyCount, Types.CHAR); } else { ps.setString(++dirtyCount, bean.getMd5()); }
ps.executeUpdate();
// listener callback
this.listenerContainer.afterUpdate(bean);
bean.resetIsModified();
return bean;
}
catch(SQLException e)
{
throw new DataAccessException(e);
}
finally
{
// listener callback
this.listenerContainer.done();
sql = null;
this.getManager().close(ps);
this.freeConnection(c);
}
}
//_____________________________________________________________________
//
// USING TEMPLATE
//_____________________________________________________________________
//18
@Override
public FlImageBean loadUniqueUsingTemplate(FlImageBean bean) throws DaoException
{
List beans = this.loadUsingTemplateAsList(bean);
switch(beans.size()){
case 0:
return null;
case 1:
return beans.get(0);
default:
throw new ObjectRetrievalException("More than one element !!");
}
}
//18-1
@Override
public FlImageBean loadUniqueUsingTemplateChecked(FlImageBean bean) throws DaoException
{
List beans = this.loadUsingTemplateAsList(bean);
switch(beans.size()){
case 0:
throw new ObjectRetrievalException("Not found element !!");
case 1:
return beans.get(0);
default:
throw new ObjectRetrievalException("More than one element !!");
}
}
//20-5
@Override
public int loadUsingTemplate(FlImageBean bean, int[] fieldList, int startRow, int numRows,int searchType, Action action) throws DaoException
{
// System.out.println("loadUsingTemplate startRow:" + startRow + ", numRows:" + numRows + ", searchType:" + searchType);
StringBuilder sqlWhere = new StringBuilder("");
String sql=createSelectSql(fieldList,this.fillWhere(sqlWhere, bean, searchType) > 0?" WHERE "+sqlWhere.toString():null);
PreparedStatement ps = null;
Connection connection = null;
try {
connection = this.getConnection();
ps = connection.prepareStatement(sql,
ResultSet.TYPE_FORWARD_ONLY,
ResultSet.CONCUR_READ_ONLY);
this.fillPreparedStatement(ps, bean, searchType,false);
return this.loadByPreparedStatement(ps, fieldList, startRow, numRows, action);
} catch (DaoException e) {
throw e;
}catch (SQLException e) {
throw new DataAccessException(e);
} finally {
this.getManager().close(ps);
this.freeConnection(connection);
}
}
//21
@Override
public int deleteUsingTemplate(FlImageBean bean) throws DaoException
{
if(bean.checkMd5Initialized() && null != bean.getMd5()){
return this.deleteByPrimaryKey(bean.getMd5());
}
if( !this.listenerContainer.isEmpty()){
final DeleteBeanAction action=new DeleteBeanAction();
this.loadUsingTemplate(bean,action);
return action.getCount();
}
Connection c = null;
PreparedStatement ps = null;
StringBuilder sql = new StringBuilder("DELETE FROM fl_image ");
StringBuilder sqlWhere = new StringBuilder("");
try
{
if (this.fillWhere(sqlWhere, bean, SEARCH_EXACT) > 0)
{
sql.append(" WHERE ").append(sqlWhere);
}
else
{
// System.out.println("The bean to look is not initialized... deleting all");
}
// System.out.println("deleteUsingTemplate: " + sql.toString());
c = this.getConnection();
ps = c.prepareStatement(sql.toString(),
ResultSet.TYPE_SCROLL_INSENSITIVE,
ResultSet.CONCUR_READ_ONLY);
this.fillPreparedStatement(ps, bean, SEARCH_EXACT, false);
return ps.executeUpdate();
}
catch(SQLException e)
{
throw new DataAccessException(e);
}
finally
{
this.getManager().close(ps);
this.freeConnection(c);
sql = null;
sqlWhere = null;
}
}
//_____________________________________________________________________
//
// USING INDICES
//_____________________________________________________________________
/**
* Retrieves an array of FlImageBean using the device_id index.
*
* @param deviceId the device_id column's value filter.
* @return an array of FlImageBean
* @throws DaoException
*/
public FlImageBean[] loadByIndexDeviceId(Integer deviceId) throws DaoException
{
return (FlImageBean[])this.loadByIndexDeviceIdAsList(deviceId).toArray(new FlImageBean[0]);
}
/**
* Retrieves a list of FlImageBean using the device_id index.
*
* @param deviceId the device_id column's value filter.
* @return a list of FlImageBean
* @throws DaoException
*/
public List loadByIndexDeviceIdAsList(Integer deviceId) throws DaoException
{
FlImageBean bean = this.createBean();
bean.setDeviceId(deviceId);
return loadUsingTemplateAsList(bean);
}
/**
* Deletes rows using the device_id index.
*
* @param deviceId the device_id column's value filter.
* @return the number of deleted objects
* @throws DaoException
*/
public int deleteByIndexDeviceId(Integer deviceId) throws DaoException
{
FlImageBean bean = this.createBean();
bean.setDeviceId(deviceId);
return deleteUsingTemplate(bean);
}
/**
* Retrieves a list of FlImageBean using the index specified by keyIndex.
* @param keyIndex valid values:
* {@link Constant#FL_IMAGE_INDEX_DEVICE_ID}
* @param keys key values of index
* @return a list of FlImageBean
* @throws DaoException
*/
@Override
public List loadByIndexAsList(int keyIndex,Object ...keys)throws DaoException
{
if(null == keys){
throw new NullPointerException();
}
switch(keyIndex){
case FL_IMAGE_INDEX_DEVICE_ID:{
if(keys.length != 1){
throw new IllegalArgumentException("argument number mismatch with index 'device_id' column number");
}
if(null != keys[0] && !(keys[0] instanceof Integer)){
throw new IllegalArgumentException("invalid type for the No.1 argument,expected type:Integer");
}
return this.loadByIndexDeviceIdAsList((Integer)keys[0]);
}
default:
throw new IllegalArgumentException(String.format("invalid keyIndex %d", keyIndex));
}
}
/**
* Deletes rows using key.
* @param keyIndex valid values:
* {@link Constant#FL_IMAGE_INDEX_DEVICE_ID}
* @param keys key values of index
* @return the number of deleted objects
* @throws DaoException
*/
@Override
public int deleteByIndex(int keyIndex,Object ...keys)throws DaoException
{
if(null == keys){
throw new NullPointerException();
}
switch(keyIndex){
case FL_IMAGE_INDEX_DEVICE_ID:{
if(keys.length != 1){
throw new IllegalArgumentException("argument number mismatch with index 'device_id' column number");
}
if(null != keys[0] && !(keys[0] instanceof Integer)){
throw new IllegalArgumentException("invalid type for the No.1 argument,expected type:Integer");
}
return this.deleteByIndexDeviceId((Integer)keys[0]);
}
default:
throw new IllegalArgumentException(String.format("invalid keyIndex %d", keyIndex));
}
}
//_____________________________________________________________________
//
// COUNT
//_____________________________________________________________________
//25
@Override
public int countWhere(String where) throws DaoException
{
String sql = new StringBuffer("SELECT COUNT(*) AS MCOUNT FROM fl_image ")
.append(null == where ? "" : where).toString();
// System.out.println("countWhere: " + sql);
Connection c = null;
Statement st = null;
ResultSet rs = null;
try
{
int iReturn = -1;
c = this.getConnection();
st = c.createStatement();
rs = st.executeQuery(sql);
if (rs.next())
{
iReturn = rs.getInt("MCOUNT");
}
if (iReturn != -1) {
return iReturn;
}
}
catch(SQLException e)
{
throw new DataAccessException(e);
}
finally
{
this.getManager().close(st, rs);
this.freeConnection(c);
sql = null;
}
throw new DataAccessException("Error in countWhere where=[" + where + "]");
}
//26
/**
* Retrieves the number of rows of the table fl_image with a prepared statement.
*
* @param ps the PreparedStatement to be used
* @return the number of rows returned
* @throws DaoException
*/
private int countByPreparedStatement(PreparedStatement ps) throws DaoException
{
ResultSet rs = null;
try
{
int iReturn = -1;
rs = ps.executeQuery();
if (rs.next()) {
iReturn = rs.getInt("MCOUNT");
}
if (iReturn != -1) {
return iReturn;
}
}
catch(SQLException e)
{
throw new DataAccessException(e);
}
finally
{
this.getManager().close(rs);
}
throw new DataAccessException("Error in countByPreparedStatement");
}
//20
/**
* count the number of elements of a specific FlImageBean bean given the search type
*
* @param bean the FlImageBean template to look for
* @param searchType exact ? like ? starting like ?
* @return the number of rows returned
* @throws DaoException
*/
@Override
public int countUsingTemplate(FlImageBean bean, int searchType) throws DaoException
{
Connection c = null;
PreparedStatement ps = null;
StringBuilder sql = new StringBuilder("SELECT COUNT(*) AS MCOUNT FROM fl_image");
StringBuilder sqlWhere = new StringBuilder("");
try
{
if (this.fillWhere(sqlWhere, bean, SEARCH_EXACT) > 0)
{
sql.append(" WHERE ").append(sqlWhere);
}
else
{
// System.out.println("The bean to look is not initialized... counting all...");
}
// System.out.println("countUsingTemplate: " + sql.toString());
c = this.getConnection();
ps = c.prepareStatement(sql.toString(),
ResultSet.TYPE_SCROLL_INSENSITIVE,
ResultSet.CONCUR_READ_ONLY);
this.fillPreparedStatement(ps, bean, searchType,false);
return this.countByPreparedStatement(ps);
}
catch(SQLException e)
{
throw new DataAccessException(e);
}
finally
{
this.getManager().close(ps);
this.freeConnection(c);
sql = null;
sqlWhere = null;
}
}
/**
* fills the given StringBuilder with the sql where clauses constructed using the bean and the search type
* @param sqlWhere the StringBuilder that will be filled
* @param bean the bean to use for creating the where clauses
* @param searchType exact ? like ? starting like ?
* @return the number of clauses returned
*/
protected int fillWhere(StringBuilder sqlWhere, FlImageBean bean, int searchType)
{
if (bean == null) {
return 0;
}
int dirtyCount = 0;
String sqlEqualsOperation = searchType == SEARCH_EXACT ? "=" : " like ";
try
{
if (bean.checkMd5Modified()) {
dirtyCount ++;
if (bean.getMd5() == null) {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("md5 IS NULL");
} else {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("md5 ").append(sqlEqualsOperation).append("?");
}
}
if (bean.checkFormatModified()) {
dirtyCount ++;
if (bean.getFormat() == null) {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("format IS NULL");
} else {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("format ").append(sqlEqualsOperation).append("?");
}
}
if (bean.checkWidthModified()) {
dirtyCount ++;
if (bean.getWidth() == null) {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("width IS NULL");
} else {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("width = ?");
}
}
if (bean.checkHeightModified()) {
dirtyCount ++;
if (bean.getHeight() == null) {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("height IS NULL");
} else {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("height = ?");
}
}
if (bean.checkDepthModified()) {
dirtyCount ++;
if (bean.getDepth() == null) {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("depth IS NULL");
} else {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("depth = ?");
}
}
if (bean.checkFaceNumModified()) {
dirtyCount ++;
if (bean.getFaceNum() == null) {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("face_num IS NULL");
} else {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("face_num = ?");
}
}
if (bean.checkThumbMd5Modified()) {
dirtyCount ++;
if (bean.getThumbMd5() == null) {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("thumb_md5 IS NULL");
} else {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("thumb_md5 ").append(sqlEqualsOperation).append("?");
}
}
if (bean.checkDeviceIdModified()) {
dirtyCount ++;
if (bean.getDeviceId() == null) {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("device_id IS NULL");
} else {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("device_id = ?");
}
}
}
finally
{
sqlEqualsOperation = null;
}
return dirtyCount;
}
/**
* fill the given prepared statement with the bean values and a search type
* @param ps the PreparedStatement that will be filled
* @param bean the bean to use for creating the where clauses
* @param searchType exact ? like ? starting like ?
* @param fillNull wether fill null for null field
* @return the number of clauses returned
* @throws DaoException
*/
protected int fillPreparedStatement(PreparedStatement ps, FlImageBean bean, int searchType,boolean fillNull) throws DaoException
{
if (bean == null) {
return 0;
}
int dirtyCount = 0;
try
{
if (bean.checkMd5Modified()) {
switch (searchType) {
case SEARCH_EXACT:
// System.out.println("Setting for " + dirtyCount + " [" + bean.getMd5() + "]");
if (bean.getMd5() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.CHAR);} } else { ps.setString(++dirtyCount, bean.getMd5()); }
break;
case SEARCH_LIKE:
// System.out.println("Setting for " + dirtyCount + " [%" + bean.getMd5() + "%]");
if ( bean.getMd5() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.CHAR);} } else { ps.setString(++dirtyCount, SQL_LIKE_WILDCARD + bean.getMd5() + SQL_LIKE_WILDCARD); }
break;
case SEARCH_STARTING_LIKE:
// System.out.println("Setting for " + dirtyCount + " [%" + bean.getMd5() + "]");
if ( bean.getMd5() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.CHAR);} } else { ps.setString(++dirtyCount, SQL_LIKE_WILDCARD + bean.getMd5()); }
break;
case SEARCH_ENDING_LIKE:
// System.out.println("Setting for " + dirtyCount + " [" + bean.getMd5() + "%]");
if (bean.getMd5() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.CHAR);} } else { ps.setString(++dirtyCount, bean.getMd5() + SQL_LIKE_WILDCARD); }
break;
default:
throw new DaoException("Unknown search type " + searchType);
}
}
if (bean.checkFormatModified()) {
switch (searchType) {
case SEARCH_EXACT:
// System.out.println("Setting for " + dirtyCount + " [" + bean.getFormat() + "]");
if (bean.getFormat() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.VARCHAR);} } else { ps.setString(++dirtyCount, bean.getFormat()); }
break;
case SEARCH_LIKE:
// System.out.println("Setting for " + dirtyCount + " [%" + bean.getFormat() + "%]");
if ( bean.getFormat() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.VARCHAR);} } else { ps.setString(++dirtyCount, SQL_LIKE_WILDCARD + bean.getFormat() + SQL_LIKE_WILDCARD); }
break;
case SEARCH_STARTING_LIKE:
// System.out.println("Setting for " + dirtyCount + " [%" + bean.getFormat() + "]");
if ( bean.getFormat() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.VARCHAR);} } else { ps.setString(++dirtyCount, SQL_LIKE_WILDCARD + bean.getFormat()); }
break;
case SEARCH_ENDING_LIKE:
// System.out.println("Setting for " + dirtyCount + " [" + bean.getFormat() + "%]");
if (bean.getFormat() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.VARCHAR);} } else { ps.setString(++dirtyCount, bean.getFormat() + SQL_LIKE_WILDCARD); }
break;
default:
throw new DaoException("Unknown search type " + searchType);
}
}
if (bean.checkWidthModified()) {
// System.out.println("Setting for " + dirtyCount + " [" + bean.getWidth() + "]");
if (bean.getWidth() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.INTEGER);} } else { Manager.setInteger(ps, ++dirtyCount, bean.getWidth()); }
}
if (bean.checkHeightModified()) {
// System.out.println("Setting for " + dirtyCount + " [" + bean.getHeight() + "]");
if (bean.getHeight() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.INTEGER);} } else { Manager.setInteger(ps, ++dirtyCount, bean.getHeight()); }
}
if (bean.checkDepthModified()) {
// System.out.println("Setting for " + dirtyCount + " [" + bean.getDepth() + "]");
if (bean.getDepth() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.INTEGER);} } else { Manager.setInteger(ps, ++dirtyCount, bean.getDepth()); }
}
if (bean.checkFaceNumModified()) {
// System.out.println("Setting for " + dirtyCount + " [" + bean.getFaceNum() + "]");
if (bean.getFaceNum() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.INTEGER);} } else { Manager.setInteger(ps, ++dirtyCount, bean.getFaceNum()); }
}
if (bean.checkThumbMd5Modified()) {
switch (searchType) {
case SEARCH_EXACT:
// System.out.println("Setting for " + dirtyCount + " [" + bean.getThumbMd5() + "]");
if (bean.getThumbMd5() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.CHAR);} } else { ps.setString(++dirtyCount, bean.getThumbMd5()); }
break;
case SEARCH_LIKE:
// System.out.println("Setting for " + dirtyCount + " [%" + bean.getThumbMd5() + "%]");
if ( bean.getThumbMd5() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.CHAR);} } else { ps.setString(++dirtyCount, SQL_LIKE_WILDCARD + bean.getThumbMd5() + SQL_LIKE_WILDCARD); }
break;
case SEARCH_STARTING_LIKE:
// System.out.println("Setting for " + dirtyCount + " [%" + bean.getThumbMd5() + "]");
if ( bean.getThumbMd5() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.CHAR);} } else { ps.setString(++dirtyCount, SQL_LIKE_WILDCARD + bean.getThumbMd5()); }
break;
case SEARCH_ENDING_LIKE:
// System.out.println("Setting for " + dirtyCount + " [" + bean.getThumbMd5() + "%]");
if (bean.getThumbMd5() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.CHAR);} } else { ps.setString(++dirtyCount, bean.getThumbMd5() + SQL_LIKE_WILDCARD); }
break;
default:
throw new DaoException("Unknown search type " + searchType);
}
}
if (bean.checkDeviceIdModified()) {
// System.out.println("Setting for " + dirtyCount + " [" + bean.getDeviceId() + "]");
if (bean.getDeviceId() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.INTEGER);} } else { Manager.setInteger(ps, ++dirtyCount, bean.getDeviceId()); }
}
}
catch(SQLException e)
{
throw new DataAccessException(e);
}
return dirtyCount;
}
//_____________________________________________________________________
//
// DECODE RESULT SET
//_____________________________________________________________________
//28
/**
* decode a resultset in an array of FlImageBean objects
*
* @param rs the resultset to decode
* @param fieldList table of the field's associated constants
* @param startRow the start row to be used (first row = 1, last row = -1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @return the resulting FlImageBean table
* @throws DaoException
*/
public FlImageBean[] decodeResultSet(ResultSet rs, int[] fieldList, int startRow, int numRows) throws DaoException
{
return this.decodeResultSetAsList(rs, fieldList, startRow, numRows).toArray(new FlImageBean[0]);
}
//28-1
/**
* decode a resultset in a list of FlImageBean objects
*
* @param rs the resultset to decode
* @param fieldList table of the field's associated constants
* @param startRow the start row to be used (first row = 1, last row = -1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @return the resulting FlImageBean table
* @throws DaoException
*/
public List decodeResultSetAsList(ResultSet rs, int[] fieldList, int startRow, int numRows) throws DaoException
{
ListAction action = new ListAction();
actionOnResultSet(rs, fieldList, numRows, numRows, action);
return action.getList();
}
//28-2
/** decode a resultset and call action
* @param rs the resultset to decode
* @param fieldList table of the field's associated constants
* @param startRow the start row to be used (first row = 1, last row = -1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @param action interface obj for do something
* @return the count dealt by action
* @throws DaoException
* @throws IllegalArgumentException
*/
public int actionOnResultSet(ResultSet rs, int[] fieldList, int startRow, int numRows, Action action) throws DaoException{
try{
int count = 0;
if(0!=numRows){
if( startRow<1 ){
throw new IllegalArgumentException("invalid argument:startRow (must >=1)");
}
if( null==action || null==rs ){
throw new IllegalArgumentException("invalid argument:action OR rs (must not be null)");
}
for(;startRow > 1 && rs.next();){
--startRow;
//skip to last of startRow
}
if (fieldList == null) {
if(numRows<0){
for(;rs.next();++count){
action.call(decodeRow(rs, action.getBean()));
}
}else{
for(;rs.next() && count loadByPreparedStatementAsList(PreparedStatement ps) throws DaoException
{
return this.loadByPreparedStatementAsList(ps, null);
}
//33
/**
* Loads all the elements using a prepared statement specifying a list of fields to be retrieved.
*
* @param ps the PreparedStatement to be used
* @param fieldList table of the field's associated constants
* @return an array of FlImageBean
* @throws DaoException
*/
public FlImageBean[] loadByPreparedStatement(PreparedStatement ps, int[] fieldList) throws DaoException
{
return this.loadByPreparedStatementAsList(ps, fieldList).toArray(new FlImageBean[0]);
}
//33
/**
* Loads all the elements using a prepared statement specifying a list of fields to be retrieved.
*
* @param ps the PreparedStatement to be used
* @param fieldList table of the field's associated constants
* @return an array of FlImageBean
* @throws DaoException
*/
public List loadByPreparedStatementAsList(PreparedStatement ps, int[] fieldList) throws DaoException
{
return loadByPreparedStatementAsList(ps,fieldList,1,-1);
}
//34
/**
* Loads all the elements using a prepared statement specifying a list of fields to be retrieved,
* and specifying the start row and the number of rows.
*
* @param ps the PreparedStatement to be used
* @param startRow the start row to be used (first row = 1, last row = -1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @param fieldList table of the field's associated constants
* @return an array of FlImageBean
* @throws DaoException
*/
public FlImageBean[] loadByPreparedStatement(PreparedStatement ps, int[] fieldList, int startRow, int numRows) throws DaoException
{
return loadByPreparedStatementAsList(ps,fieldList,startRow,numRows).toArray(new FlImageBean[0]);
}
//34-1
/**
* Loads all the elements using a prepared statement specifying a list of fields to be retrieved,
* and specifying the start row and the number of rows.
*
* @param ps the PreparedStatement to be used
* @param startRow the start row to be used (first row = 1, last row = -1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @param fieldList table of the field's associated constants
* @return an array of FlImageBean
* @throws DaoException
*/
public List loadByPreparedStatementAsList(PreparedStatement ps, int[] fieldList, int startRow, int numRows) throws DaoException
{
ListAction action = new ListAction();
loadByPreparedStatement(ps,fieldList,startRow,numRows,action);
return action.getList();
}
//34-2
/**
* Loads each element using a prepared statement specifying a list of fields to be retrieved,
* and specifying the start row and the number of rows
* and dealt by action.
*
* @param ps the PreparedStatement to be used
* @param startRow the start row to be used (first row = 1, last row = -1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @param fieldList table of the field's associated constants
* @param action Action object for do something(not null)
* @return the count dealt by action
* @throws DaoException
*/
public int loadByPreparedStatement(PreparedStatement ps, int[] fieldList, int startRow, int numRows,Action action) throws DaoException
{
ResultSet rs = null;
try {
ps.setFetchSize(100);
rs = ps.executeQuery();
return this.actionOnResultSet(rs, fieldList, startRow, numRows, action);
} catch (DaoException e) {
throw e;
} catch (SQLException e) {
throw new DataAccessException(e);
} finally {
this.getManager().close(rs);
}
}
//_____________________________________________________________________
//
// LISTENER
//_____________________________________________________________________
private final TableListener.ListenerContainer listenerContainer = new TableListener.ListenerContainer();
//35
@Override
public TableListener registerListener(TableListener listener)
{
this.listenerContainer.add(listener);
return listener;
}
//36
/**
* remove listener.
*/
@Override
public void unregisterListener(TableListener listener)
{
this.listenerContainer.remove(listener);
}
//37
@Override
public void fire(TableListener.Event event, FlImageBean bean) throws DaoException{
if(null == event){
throw new NullPointerException();
}
event.fire(listenerContainer, bean);
}
//37-1
@Override
public void fire(int event, FlImageBean bean) throws DaoException{
try{
fire(TableListener.Event.values()[event],bean);
}catch(ArrayIndexOutOfBoundsException e){
throw new IllegalArgumentException("invalid event id " + event);
}
}
/** foreign key listener for DEELTE RULE : SET_NULL */
private final net.gdface.facelog.dborm.BaseForeignKeyListener foreignKeyListenerByDeviceId =
new net.gdface.facelog.dborm.BaseForeignKeyListener(){
@Override
protected List getImportedBeans(FlDeviceBean bean) throws DaoException {
return listenerContainer.isEmpty()
? java.util.Collections.emptyList()
: instanceOfFlDeviceManager().getImageBeansByDeviceIdAsList(bean);
}
@Override
protected void onRemove(List effectBeans) throws DaoException {
for(FlImageBean bean:effectBeans){
bean.setDeviceId(null);
Event.UPDATE.fire(listenerContainer, bean);
bean.resetIsModified();
}
}};
//37-2
/**
* bind foreign key listener to foreign table:
* DELETE RULE : SET_NULL {@code fl_image(device_id)- fl_device(id)}
*/
public void bindForeignKeyListenerForDeleteRule(){
instanceOfFlDeviceManager().registerListener(foreignKeyListenerByDeviceId);
}
//37-3
/**
* unbind foreign key listener from all of foreign tables
* @see #bindForeignKeyListenerForDeleteRule()
*/
public void unbindForeignKeyListenerForDeleteRule(){
instanceOfFlDeviceManager().unregisterListener(foreignKeyListenerByDeviceId);
}
//_____________________________________________________________________
//
// UTILS
//_____________________________________________________________________
//40
/**
* Retrieves the manager object used to get connections.
*
* @return the manager used
*/
private Manager getManager()
{
return Manager.getInstance();
}
//41
/**
* Frees the connection.
*
* @param c the connection to release
*/
private void freeConnection(Connection c)
{
// back to pool
this.getManager().releaseConnection(c);
}
//42
/**
* Gets the connection.
*/
private Connection getConnection() throws DaoException
{
try
{
return this.getManager().getConnection();
}
catch(SQLException e)
{
throw new DataAccessException(e);
}
}
//43
@Override
public boolean isPrimaryKey(String column){
for(String c:PRIMARYKEY_NAMES){
if(c.equalsIgnoreCase(column)){
return true;
}
}
return false;
}
/**
* Fill the given prepared statement with the values in argList
* @param ps the PreparedStatement that will be filled
* @param argList the arguments to use fill given prepared statement
* @throws DaoException
*/
private void fillPrepareStatement(PreparedStatement ps, Object[] argList) throws DaoException{
try {
if (!(argList == null || ps == null)) {
for (int i = 0; i < argList.length; i++) {
if (argList[i].getClass().equals(byte[].class)) {
ps.setBytes(i + 1, (byte[]) argList[i]);
} else {
ps.setObject(i + 1, argList[i]);
}
}
}
} catch (SQLException e) {
throw new DaoException(e);
}
}
@Override
public int loadBySqlForAction(String sql, Object[] argList, int[] fieldList,int startRow, int numRows,Action action) throws DaoException{
PreparedStatement ps = null;
Connection connection = null;
// logger.debug("sql string:\n" + sql + "\n");
try {
connection = this.getConnection();
ps = connection.prepareStatement(sql,
ResultSet.TYPE_FORWARD_ONLY,
ResultSet.CONCUR_READ_ONLY);
fillPrepareStatement(ps, argList);
return this.loadByPreparedStatement(ps, fieldList, startRow, numRows, action);
} catch (DaoException e) {
throw e;
}catch (SQLException e) {
throw new DataAccessException(e);
} finally {
this.getManager().close(ps);
this.freeConnection(connection);
}
}
@Override
public T runAsTransaction(Callable fun) throws DaoException{
return Manager.getInstance().runAsTransaction(fun);
}
class DeleteBeanAction extends Action.BaseAdapter{
private final AtomicInteger count=new AtomicInteger(0);
@Override
public void call(FlImageBean bean) throws DaoException {
FlImageManager.this.delete(bean);
count.incrementAndGet();
}
int getCount(){
return count.get();
}
}
//45
/**
* return a primary key list from {@link FlImageBean} array
* @param array
*/
public List toPrimaryKeyList(FlImageBean... array){
if(null == array){
return new java.util.ArrayList();
}
java.util.ArrayList list = new java.util.ArrayList(array.length);
for(FlImageBean bean:array){
list.add(null == bean ? null : bean.getMd5());
}
return list;
}
//46
/**
* return a primary key list from {@link FlImageBean} collection
* @param collection
*/
public List toPrimaryKeyList(java.util.Collection collection){
if(null == collection){
return new java.util.ArrayList();
}
java.util.ArrayList list = new java.util.ArrayList(collection.size());
for(FlImageBean bean:collection){
list.add(null == bean ? null : bean.getMd5());
}
return list;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy