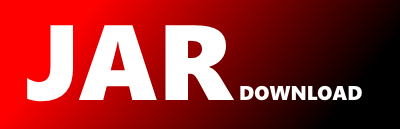
net.gdface.facelog.dborm.log.FlLogLightBean Maven / Gradle / Ivy
// ______________________________________________________
// Generated by sql2java - https://github.com/10km/sql2java-2-6-7 (custom branch)
// modified by guyadong from
// sql2java original version https://sourceforge.net/projects/sql2java/
// JDBC driver used at code generation time: com.mysql.jdbc.Driver
// template: bean.java.vm
// ______________________________________________________
package net.gdface.facelog.dborm.log;
import java.io.Serializable;
import java.util.List;
import java.util.Objects;
import net.gdface.facelog.dborm.Constant;
import net.gdface.facelog.dborm.BaseBean;
import net.gdface.facelog.dborm.CompareToBuilder;
import net.gdface.facelog.dborm.EqualsBuilder;
import net.gdface.facelog.dborm.HashCodeBuilder;
/**
* FlLogLightBean is a mapping of fl_log_light Table.
*
Meta Data Information (in progress):
*
* - comments: VIEW
*
* @author guyadong
*/
public class FlLogLightBean
implements Serializable,BaseBean,Comparable,Constant,Cloneable
{
private static final long serialVersionUID = -8629124492169570652L;
/** NULL {@link FlLogLightBean} bean , IMMUTABLE instance */
public static final FlLogLightBean NULL = new FlLogLightBean().asNULL().asImmutable();
/** comments:日志id */
private Integer id;
/** comments:用户id */
private Integer personId;
/** comments:姓名 */
private String name;
/** comments:证件类型,0:未知,1:身份证,2:护照,3:台胞证,4:港澳通行证,5:军官证,6:外国人居留证,7:员工卡,8:其他 */
private Integer papersType;
/** comments:证件号码 */
private String papersNum;
/** comments:验证时间(可能由前端设备提供时间) */
private java.util.Date verifyTime;
/** flag whether {@code this} can be modified */
private Boolean immutable;
/** columns modified flag */
private int modified;
/** columns initialized flag */
private int initialized;
/** new record flag */
private boolean isNew;
/**
* set immutable status
* @return {@code this}
*/
private FlLogLightBean immutable(Boolean immutable) {
this.immutable = immutable;
return this;
}
/**
* set {@code this} as immutable object
* @return {@code this}
*/
public FlLogLightBean asImmutable() {
return immutable(Boolean.TRUE);
}
/**
* @return {@code true} if {@code this} is a mutable object
*/
public boolean mutable(){
return !Boolean.TRUE.equals(this.immutable);
}
/**
* @return {@code this}
* @throws IllegalStateException if {@code this} is a immutable object
*/
private FlLogLightBean checkMutable(){
if(!mutable()){
throw new IllegalStateException("this is a immutable object");
}
return this;
}
/**
* @return return a new mutable copy of this object.
*/
public FlLogLightBean cloneMutable(){
return clone().immutable(null);
}
@Override
public boolean isNew()
{
return this.isNew;
}
@Override
public void isNew(boolean isNew)
{
this.isNew = isNew;
}
/**
* Specifies to the object if it has been set as new.
*
* @param isNew the boolean value to be assigned to the isNew field
*/
public void setNew(boolean isNew)
{
this.isNew = isNew;
}
/**
* @return the modified status of columns
*/
public int getModified(){
return modified;
}
/**
* @param modified the modified status bit to be assigned to {@link #modified}
*/
public void setModified(int modified){
this.modified = modified;
}
/**
* @return the initialized status of columns
*/
public int getInitialized(){
return initialized;
}
/**
* @param initialized the initialized status bit to be assigned to {@link #initialized}
*/
public void setInitialized(int initialized){
this.initialized = initialized;
}
protected static final >boolean equals(T a, T b) {
return a == b || (a != null && 0==a.compareTo(b));
}
public FlLogLightBean(){
super();
reset();
}
/**
* Getter method for {@link #id}.
* Meta Data Information (in progress):
*
* - full name: fl_log_light.id
* - comments: 日志id
* - default value: '0'
* - NOT NULL
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of id
*/
public Integer getId(){
return id;
}
/**
* Setter method for {@link #id}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to id
*/
public void setId(Integer newVal)
{
checkMutable();
modified |= FL_LOG_LIGHT_ID_ID_MASK;
initialized |= FL_LOG_LIGHT_ID_ID_MASK;
if (Objects.equals(newVal, id)) {
return;
}
id = newVal;
}
/**
* Determines if the id has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkIdModified()
{
return 0L != (modified & FL_LOG_LIGHT_ID_ID_MASK);
}
/**
* Determines if the id has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkIdInitialized()
{
return 0L != (initialized & FL_LOG_LIGHT_ID_ID_MASK);
}
/**
* Getter method for {@link #personId}.
* Meta Data Information (in progress):
*
* - full name: fl_log_light.person_id
* - comments: 用户id
* - default value: '0'
* - NOT NULL
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of personId
*/
public Integer getPersonId(){
return personId;
}
/**
* Setter method for {@link #personId}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to personId
*/
public void setPersonId(Integer newVal)
{
checkMutable();
modified |= FL_LOG_LIGHT_ID_PERSON_ID_MASK;
initialized |= FL_LOG_LIGHT_ID_PERSON_ID_MASK;
if (Objects.equals(newVal, personId)) {
return;
}
personId = newVal;
}
/**
* Determines if the personId has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkPersonIdModified()
{
return 0L != (modified & FL_LOG_LIGHT_ID_PERSON_ID_MASK);
}
/**
* Determines if the personId has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkPersonIdInitialized()
{
return 0L != (initialized & FL_LOG_LIGHT_ID_PERSON_ID_MASK);
}
/**
* Getter method for {@link #name}.
* Meta Data Information (in progress):
*
* - full name: fl_log_light.name
* - comments: 姓名
* - NOT NULL
* - column size: 32
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of name
*/
public String getName(){
return name;
}
/**
* Setter method for {@link #name}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to name
*/
public void setName(String newVal)
{
checkMutable();
modified |= FL_LOG_LIGHT_ID_NAME_MASK;
initialized |= FL_LOG_LIGHT_ID_NAME_MASK;
if (Objects.equals(newVal, name)) {
return;
}
name = newVal;
}
/**
* Determines if the name has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkNameModified()
{
return 0L != (modified & FL_LOG_LIGHT_ID_NAME_MASK);
}
/**
* Determines if the name has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkNameInitialized()
{
return 0L != (initialized & FL_LOG_LIGHT_ID_NAME_MASK);
}
/**
* Getter method for {@link #papersType}.
* Meta Data Information (in progress):
*
* - full name: fl_log_light.papers_type
* - comments: 证件类型,0:未知,1:身份证,2:护照,3:台胞证,4:港澳通行证,5:军官证,6:外国人居留证,7:员工卡,8:其他
* - column size: 3
* - JDBC type returned by the driver: Types.TINYINT
*
*
* @return the value of papersType
*/
public Integer getPapersType(){
return papersType;
}
/**
* Setter method for {@link #papersType}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to papersType
*/
public void setPapersType(Integer newVal)
{
checkMutable();
modified |= FL_LOG_LIGHT_ID_PAPERS_TYPE_MASK;
initialized |= FL_LOG_LIGHT_ID_PAPERS_TYPE_MASK;
if (Objects.equals(newVal, papersType)) {
return;
}
papersType = newVal;
}
/**
* Determines if the papersType has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkPapersTypeModified()
{
return 0L != (modified & FL_LOG_LIGHT_ID_PAPERS_TYPE_MASK);
}
/**
* Determines if the papersType has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkPapersTypeInitialized()
{
return 0L != (initialized & FL_LOG_LIGHT_ID_PAPERS_TYPE_MASK);
}
/**
* Getter method for {@link #papersNum}.
* Meta Data Information (in progress):
*
* - full name: fl_log_light.papers_num
* - comments: 证件号码
* - column size: 32
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of papersNum
*/
public String getPapersNum(){
return papersNum;
}
/**
* Setter method for {@link #papersNum}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to papersNum
*/
public void setPapersNum(String newVal)
{
checkMutable();
modified |= FL_LOG_LIGHT_ID_PAPERS_NUM_MASK;
initialized |= FL_LOG_LIGHT_ID_PAPERS_NUM_MASK;
if (Objects.equals(newVal, papersNum)) {
return;
}
papersNum = newVal;
}
/**
* Determines if the papersNum has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkPapersNumModified()
{
return 0L != (modified & FL_LOG_LIGHT_ID_PAPERS_NUM_MASK);
}
/**
* Determines if the papersNum has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkPapersNumInitialized()
{
return 0L != (initialized & FL_LOG_LIGHT_ID_PAPERS_NUM_MASK);
}
/**
* Getter method for {@link #verifyTime}.
* Meta Data Information (in progress):
*
* - full name: fl_log_light.verify_time
* - comments: 验证时间(可能由前端设备提供时间)
* - default value: '0000-00-00 00:00:00'
* - NOT NULL
* - column size: 19
* - JDBC type returned by the driver: Types.TIMESTAMP
*
*
* @return the value of verifyTime
*/
public java.util.Date getVerifyTime(){
return verifyTime;
}
/**
* Setter method for {@link #verifyTime}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to verifyTime
*/
public void setVerifyTime(java.util.Date newVal)
{
checkMutable();
modified |= FL_LOG_LIGHT_ID_VERIFY_TIME_MASK;
initialized |= FL_LOG_LIGHT_ID_VERIFY_TIME_MASK;
if (Objects.equals(newVal, verifyTime)) {
return;
}
verifyTime = newVal;
}
/**
* Setter method for {@link #verifyTime}.
* @param newVal the number of milliseconds since January 1, 1970, 00:00:00 GMT represented by this Date object.
*/
public void setVerifyTime(Long newVal)
{
setVerifyTime(null == newVal ? null : new java.util.Date(newVal));
}
/**
* Determines if the verifyTime has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkVerifyTimeModified()
{
return 0L != (modified & FL_LOG_LIGHT_ID_VERIFY_TIME_MASK);
}
/**
* Determines if the verifyTime has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkVerifyTimeInitialized()
{
return 0L != (initialized & FL_LOG_LIGHT_ID_VERIFY_TIME_MASK);
}
@Override
public boolean isModified()
{
return 0 != modified;
}
@Override
public boolean isModified(int columnID){
switch ( columnID ){
case FL_LOG_LIGHT_ID_ID:
return checkIdModified();
case FL_LOG_LIGHT_ID_PERSON_ID:
return checkPersonIdModified();
case FL_LOG_LIGHT_ID_NAME:
return checkNameModified();
case FL_LOG_LIGHT_ID_PAPERS_TYPE:
return checkPapersTypeModified();
case FL_LOG_LIGHT_ID_PAPERS_NUM:
return checkPapersNumModified();
case FL_LOG_LIGHT_ID_VERIFY_TIME:
return checkVerifyTimeModified();
default:
return false;
}
}
@Override
public boolean isInitialized(int columnID){
switch(columnID) {
case FL_LOG_LIGHT_ID_ID:
return checkIdInitialized();
case FL_LOG_LIGHT_ID_PERSON_ID:
return checkPersonIdInitialized();
case FL_LOG_LIGHT_ID_NAME:
return checkNameInitialized();
case FL_LOG_LIGHT_ID_PAPERS_TYPE:
return checkPapersTypeInitialized();
case FL_LOG_LIGHT_ID_PAPERS_NUM:
return checkPapersNumInitialized();
case FL_LOG_LIGHT_ID_VERIFY_TIME:
return checkVerifyTimeInitialized();
default:
return false;
}
}
@Override
public boolean isModified(String column){
return isModified(columnIDOf(column));
}
@Override
public boolean isInitialized(String column){
return isInitialized(columnIDOf(column));
}
@Override
public void resetIsModified()
{
checkMutable();
modified = 0;
}
@Override
public void resetPrimaryKeysModified()
{
// columns is null or empty;
}
/**
* Resets columns modification status except primary keys to 'not modified'.
*/
public void resetModifiedExceptPrimaryKeys()
{
modified &= (~(FL_LOG_LIGHT_ID_ID_MASK |
FL_LOG_LIGHT_ID_PERSON_ID_MASK |
FL_LOG_LIGHT_ID_NAME_MASK |
FL_LOG_LIGHT_ID_PAPERS_TYPE_MASK |
FL_LOG_LIGHT_ID_PAPERS_NUM_MASK |
FL_LOG_LIGHT_ID_VERIFY_TIME_MASK));
}
/**
* Resets the object initialization status to 'not initialized'.
*/
private void resetInitialized()
{
initialized = 0;
}
/** reset all fields to initial value, equal to a new bean */
public void reset(){
checkMutable();
/* DEFAULT:'0'*/
this.id = new Integer(0);
/* DEFAULT:'0'*/
this.personId = new Integer(0);
this.name = null;
this.papersType = null;
this.papersNum = null;
/* DEFAULT:'0000-00-00 00:00:00'*/
this.verifyTime = null;
this.isNew = true;
this.modified = 0;
this.initialized = (FL_LOG_LIGHT_ID_ID_MASK | FL_LOG_LIGHT_ID_PERSON_ID_MASK);
}
@Override
public boolean equals(Object object)
{
if (!(object instanceof FlLogLightBean)) {
return false;
}
FlLogLightBean obj = (FlLogLightBean) object;
return new EqualsBuilder()
.append(getId(), obj.getId())
.append(getPersonId(), obj.getPersonId())
.append(getName(), obj.getName())
.append(getPapersType(), obj.getPapersType())
.append(getPapersNum(), obj.getPapersNum())
.append(getVerifyTime(), obj.getVerifyTime())
.isEquals();
}
@Override
public int hashCode()
{
return new HashCodeBuilder(-82280557, -700257973)
.append(getId())
.append(getPersonId())
.append(getName())
.append(getPapersType())
.append(getPapersNum())
.append(getVerifyTime())
.toHashCode();
}
@Override
public String toString() {
return toString(true,false);
}
/**
* cast byte array to HEX string
*
* @param input
* @return {@code null} if {@code input} is null
*/
private static final String toHex(byte[] input) {
if (null == input){
return null;
}
StringBuffer sb = new StringBuffer(input.length * 2);
for (int i = 0; i < input.length; i++) {
sb.append(Character.forDigit((input[i] & 240) >> 4, 16));
sb.append(Character.forDigit(input[i] & 15, 16));
}
return sb.toString();
}
protected static final StringBuilder append(StringBuilder buffer,boolean full,byte[] value){
if(full || null == value){
buffer.append(toHex(value));
}else{
buffer.append(value.length).append(" bytes");
}
return buffer;
}
private static int stringLimit = 64;
private static final int MINIMUM_LIMIT = 16;
protected static final StringBuilder append(StringBuilder buffer,boolean full,String value){
if(full || null == value || value.length() <= stringLimit){
buffer.append(value);
}else{
buffer.append(value.substring(0,stringLimit - 8)).append(" ...").append(value.substring(stringLimit-4,stringLimit));
}
return buffer;
}
protected static final StringBuilder append(StringBuilder buffer,boolean full,T value){
return buffer.append(value);
}
public static final void setStringLimit(int limit){
if(limit < MINIMUM_LIMIT){
throw new IllegalArgumentException(String.format("INVALID limit %d,minimum value %d",limit,MINIMUM_LIMIT));
}
stringLimit = limit;
}
@Override
public String toString(boolean notNull, boolean fullIfStringOrBytes) {
// only output initialized field
StringBuilder builder = new StringBuilder(this.getClass().getName()).append("@").append(Integer.toHexString(this.hashCode())).append("[");
int count = 0;
if(checkIdInitialized()){
if(!notNull || null != getId()){
if(count++ >0){
builder.append(",");
}
builder.append("id=");
append(builder,fullIfStringOrBytes,getId());
}
}
if(checkPersonIdInitialized()){
if(!notNull || null != getPersonId()){
if(count++ >0){
builder.append(",");
}
builder.append("person_id=");
append(builder,fullIfStringOrBytes,getPersonId());
}
}
if(checkNameInitialized()){
if(!notNull || null != getName()){
if(count++ >0){
builder.append(",");
}
builder.append("name=");
append(builder,fullIfStringOrBytes,getName());
}
}
if(checkPapersTypeInitialized()){
if(!notNull || null != getPapersType()){
if(count++ >0){
builder.append(",");
}
builder.append("papers_type=");
append(builder,fullIfStringOrBytes,getPapersType());
}
}
if(checkPapersNumInitialized()){
if(!notNull || null != getPapersNum()){
if(count++ >0){
builder.append(",");
}
builder.append("papers_num=");
append(builder,fullIfStringOrBytes,getPapersNum());
}
}
if(checkVerifyTimeInitialized()){
if(!notNull || null != getVerifyTime()){
if(count++ >0){
builder.append(",");
}
builder.append("verify_time=");
append(builder,fullIfStringOrBytes,getVerifyTime());
}
}
builder.append("]");
return builder.toString();
}
@Override
public int compareTo(FlLogLightBean object){
return new CompareToBuilder()
.append(getId(), object.getId())
.append(getPersonId(), object.getPersonId())
.append(getName(), object.getName())
.append(getPapersType(), object.getPapersType())
.append(getPapersNum(), object.getPapersNum())
.append(getVerifyTime(), object.getVerifyTime())
.toComparison();
}
@Override
public FlLogLightBean clone(){
try {
return (FlLogLightBean) super.clone();
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
}
/**
* Make {@code this} to a NULL bean
* set all fields to null, {@link #modified} and {@link #initialized} be set to 0
* @return {@code this} bean
* @author guyadong
*/
public FlLogLightBean asNULL()
{
checkMutable();
setId((Integer)null);
setPersonId((Integer)null);
setName((String)null);
setPapersType((Integer)null);
setPapersNum((String)null);
setVerifyTime((java.util.Date)null);
isNew(true);
resetInitialized();
resetIsModified();
return this;
}
/**
* check whether this bean is a NULL bean
* @return {@code true} if {@link #initialized} be set to zero
* @see #asNULL()
*/
public boolean checkNULL(){
return 0 == getInitialized();
}
/**
* @param source source list
* @return {@code source} replace {@code null} element with null instance({@link #NULL})
*/
public static final List replaceNull(List source){
if(null != source){
for(int i = 0,endIndex = source.size();i replaceNullInstance(List source){
if(null != source){
for(int i = 0,endIndex = source.size();iT getValue(int columnID)
{
switch( columnID ){
case FL_LOG_LIGHT_ID_ID:
return (T)getId();
case FL_LOG_LIGHT_ID_PERSON_ID:
return (T)getPersonId();
case FL_LOG_LIGHT_ID_NAME:
return (T)getName();
case FL_LOG_LIGHT_ID_PAPERS_TYPE:
return (T)getPapersType();
case FL_LOG_LIGHT_ID_PAPERS_NUM:
return (T)getPapersNum();
case FL_LOG_LIGHT_ID_VERIFY_TIME:
return (T)getVerifyTime();
default:
return null;
}
}
@Override
public void setValue(int columnID,T value)
{
switch( columnID ) {
case FL_LOG_LIGHT_ID_ID:
setId((Integer)value);
break;
case FL_LOG_LIGHT_ID_PERSON_ID:
setPersonId((Integer)value);
break;
case FL_LOG_LIGHT_ID_NAME:
setName((String)value);
break;
case FL_LOG_LIGHT_ID_PAPERS_TYPE:
setPapersType((Integer)value);
break;
case FL_LOG_LIGHT_ID_PAPERS_NUM:
setPapersNum((String)value);
break;
case FL_LOG_LIGHT_ID_VERIFY_TIME:
setVerifyTime((java.util.Date)value);
break;
default:
break;
}
}
@Override
public T getValue(String column)
{
return getValue(columnIDOf(column));
}
@Override
public void setValue(String column,T value)
{
setValue(columnIDOf(column),value);
}
/**
* @param column column name
* @return column id for the given field name or negative if {@code column} is invalid name
*/
public static int columnIDOf(String column){
int index = FL_LOG_LIGHT_FIELDS_LIST.indexOf(column);
return index < 0
? FL_LOG_LIGHT_JAVA_FIELDS_LIST.indexOf(column)
: index;
}
public static final Builder builder(){
return new Builder().reset();
}
/**
* a builder for FlLogLightBean,the template instance is thread local variable
* a instance of Builder can be reused.
*/
public static final class Builder{
/** FlLogLightBean instance used for template to create new FlLogLightBean instance. */
static final ThreadLocal TEMPLATE = new ThreadLocal(){
@Override
protected FlLogLightBean initialValue() {
return new FlLogLightBean();
}};
private Builder() {}
/**
* reset the bean as template
* @see FlLogLightBean#reset()
*/
public Builder reset(){
TEMPLATE.get().reset();
return this;
}
/** set a bean as template,must not be {@code null} */
public Builder template(FlLogLightBean bean){
if(null == bean){
throw new NullPointerException();
}
TEMPLATE.set(bean);
return this;
}
/** return a clone instance of {@link #TEMPLATE}*/
public FlLogLightBean build(){
return TEMPLATE.get().clone();
}
/**
* fill the field : fl_log_light.id
* @param id 日志id
* @see FlLogLightBean#getId()
* @see FlLogLightBean#setId(Integer)
*/
public Builder id(Integer id){
TEMPLATE.get().setId(id);
return this;
}
/**
* fill the field : fl_log_light.person_id
* @param personId 用户id
* @see FlLogLightBean#getPersonId()
* @see FlLogLightBean#setPersonId(Integer)
*/
public Builder personId(Integer personId){
TEMPLATE.get().setPersonId(personId);
return this;
}
/**
* fill the field : fl_log_light.name
* @param name 姓名
* @see FlLogLightBean#getName()
* @see FlLogLightBean#setName(String)
*/
public Builder name(String name){
TEMPLATE.get().setName(name);
return this;
}
/**
* fill the field : fl_log_light.papers_type
* @param papersType 证件类型,0:未知,1:身份证,2:护照,3:台胞证,4:港澳通行证,5:军官证,6:外国人居留证,7:员工卡,8:其他
* @see FlLogLightBean#getPapersType()
* @see FlLogLightBean#setPapersType(Integer)
*/
public Builder papersType(Integer papersType){
TEMPLATE.get().setPapersType(papersType);
return this;
}
/**
* fill the field : fl_log_light.papers_num
* @param papersNum 证件号码
* @see FlLogLightBean#getPapersNum()
* @see FlLogLightBean#setPapersNum(String)
*/
public Builder papersNum(String papersNum){
TEMPLATE.get().setPapersNum(papersNum);
return this;
}
/**
* fill the field : fl_log_light.verify_time
* @param verifyTime 验证时间(可能由前端设备提供时间)
* @see FlLogLightBean#getVerifyTime()
* @see FlLogLightBean#setVerifyTime(java.util.Date)
*/
public Builder verifyTime(java.util.Date verifyTime){
TEMPLATE.get().setVerifyTime(verifyTime);
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy