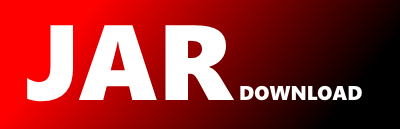
net.gdface.facelog.dborm.log.FlLogLightManager Maven / Gradle / Ivy
// ______________________________________________________
// Generated by sql2java - https://github.com/10km/sql2java-2-6-7 (custom branch)
// modified by guyadong from
// sql2java original version https://sourceforge.net/projects/sql2java/
// JDBC driver used at code generation time: com.mysql.jdbc.Driver
// template: manager.java.vm
// ______________________________________________________
package net.gdface.facelog.dborm.log;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.sql.Types;
import java.util.List;
import java.util.concurrent.Callable;
import java.util.concurrent.atomic.AtomicInteger;
import net.gdface.facelog.dborm.Constant;
import net.gdface.facelog.dborm.Manager;
import net.gdface.facelog.dborm.TableListener;
import net.gdface.facelog.dborm.TableManager;
import net.gdface.facelog.dborm.exception.DaoException;
import net.gdface.facelog.dborm.exception.DataAccessException;
import net.gdface.facelog.dborm.exception.DataRetrievalException;
import net.gdface.facelog.dborm.exception.ObjectRetrievalException;
/**
* Handles database calls (save, load, count, etc...) for the fl_log_light table.
* Remarks: VIEW
* @author sql2java
*/
public class FlLogLightManager extends TableManager.BaseAdapter
{
/**
* Tablename.
*/
public static final String TABLE_NAME="fl_log_light";
/**
* Contains all the primary key fields of the fl_log_light table.
*/
public static final String[] PRIMARYKEY_NAMES =
{
};
@Override
public String getTableName() {
return TABLE_NAME;
}
@Override
public String getFields() {
return FL_LOG_LIGHT_FIELDS;
}
@Override
public String getFullFields() {
return FL_LOG_LIGHT_FULL_FIELDS;
}
@Override
public String[] getPrimarykeyNames() {
return PRIMARYKEY_NAMES;
}
private static FlLogLightManager singleton = new FlLogLightManager();
protected FlLogLightManager(){}
/**
* Get the FlLogLightManager singleton.
*
* @return FlLogLightManager
*/
public static FlLogLightManager getInstance()
{
return singleton;
}
/**
* Creates a new FlLogLightBean instance.
*
* @return the new FlLogLightBean
*/
public FlLogLightBean createBean()
{
return new FlLogLightBean();
}
@Override
protected Class beanType(){
return FlLogLightBean.class;
}
//////////////////////////////////////
// SQL 'WHERE' METHOD
//////////////////////////////////////
//11
/**
* Deletes rows from the fl_log_light table using a 'where' clause.
* It is up to you to pass the 'WHERE' in your where clauses.
*
Attention, if 'WHERE' is omitted it will delete all records.
*
* @param where the sql 'where' clause
* @return the number of deleted rows
* @throws DaoException
*/
@Override
public int deleteByWhere(String where) throws DaoException
{
if( !this.listenerContainer.isEmpty()){
final DeleteBeanAction action = new DeleteBeanAction();
this.loadByWhere(where,action);
return action.getCount();
}
Connection c = null;
PreparedStatement ps = null;
try
{
c = this.getConnection();
StringBuilder sql = new StringBuilder("DELETE FROM fl_log_light " + where);
// System.out.println("deleteByWhere: " + sql);
ps = c.prepareStatement(sql.toString());
return ps.executeUpdate();
}
catch(SQLException e)
{
throw new DataAccessException(e);
}
finally
{
this.getManager().close(ps);
this.freeConnection(c);
}
}
//_____________________________________________________________________
//
// SAVE
//_____________________________________________________________________
//13
@Override
public FlLogLightBean insert(FlLogLightBean bean) throws DaoException
{
// mini checks
if (null == bean || !bean.isModified()) {
return bean;
}
if (!bean.isNew()){
return this.update(bean);
}
Connection c = null;
PreparedStatement ps = null;
StringBuilder sql = null;
try
{
c = this.getConnection();
// listener callback
this.listenerContainer.beforeInsert(bean);
int dirtyCount = 0;
sql = new StringBuilder("INSERT into fl_log_light (");
if (bean.checkIdModified()) {
if (dirtyCount>0) {
sql.append(",");
}
sql.append("id");
dirtyCount++;
}
if (bean.checkPersonIdModified()) {
if (dirtyCount>0) {
sql.append(",");
}
sql.append("person_id");
dirtyCount++;
}
if (bean.checkNameModified()) {
if (dirtyCount>0) {
sql.append(",");
}
sql.append("name");
dirtyCount++;
}
if (bean.checkPapersTypeModified()) {
if (dirtyCount>0) {
sql.append(",");
}
sql.append("papers_type");
dirtyCount++;
}
if (bean.checkPapersNumModified()) {
if (dirtyCount>0) {
sql.append(",");
}
sql.append("papers_num");
dirtyCount++;
}
if (bean.checkVerifyTimeModified()) {
if (dirtyCount>0) {
sql.append(",");
}
sql.append("verify_time");
dirtyCount++;
}
sql.append(") values (");
if(dirtyCount > 0) {
sql.append("?");
for(int i = 1; i < dirtyCount; i++) {
sql.append(",?");
}
}
sql.append(")");
// System.out.println("insert : " + sql.toString());
ps = c.prepareStatement(sql.toString(), ResultSet.TYPE_SCROLL_INSENSITIVE, ResultSet.CONCUR_READ_ONLY);
this.fillPreparedStatement(ps, bean, SEARCH_EXACT,true);
ps.executeUpdate();
bean.isNew(false);
bean.resetIsModified();
// listener callback
this.listenerContainer.afterInsert(bean);
return bean;
}
catch(SQLException e)
{
throw new DataAccessException(e);
}
finally
{
// listener callback
this.listenerContainer.done();
sql = null;
this.getManager().close(ps);
this.freeConnection(c);
}
}
//14
@Override
public FlLogLightBean update(FlLogLightBean bean) throws DaoException
{
// mini checks
if (null == bean || !bean.isModified()) {
return bean;
}
if (bean.isNew()){
return this.insert(bean);
}
Connection c = null;
PreparedStatement ps = null;
StringBuilder sql = null;
try
{
c = this.getConnection();
// listener callback
this.listenerContainer.beforeUpdate(bean);
sql = new StringBuilder("UPDATE fl_log_light SET ");
boolean useComma=false;
if (bean.checkIdModified()) {
if (useComma) {
sql.append(", ");
} else {
useComma=true;
}
sql.append("id=?");
}
if (bean.checkPersonIdModified()) {
if (useComma) {
sql.append(", ");
} else {
useComma=true;
}
sql.append("person_id=?");
}
if (bean.checkNameModified()) {
if (useComma) {
sql.append(", ");
} else {
useComma=true;
}
sql.append("name=?");
}
if (bean.checkPapersTypeModified()) {
if (useComma) {
sql.append(", ");
} else {
useComma=true;
}
sql.append("papers_type=?");
}
if (bean.checkPapersNumModified()) {
if (useComma) {
sql.append(", ");
} else {
useComma=true;
}
sql.append("papers_num=?");
}
if (bean.checkVerifyTimeModified()) {
if (useComma) {
sql.append(", ");
} else {
useComma=true;
}
sql.append("verify_time=?");
}
sql.append("");
// System.out.println("update : " + sql.toString());
ps = c.prepareStatement(sql.toString(),
ResultSet.TYPE_SCROLL_INSENSITIVE,
ResultSet.CONCUR_READ_ONLY);
int dirtyCount = this.fillPreparedStatement(ps, bean, SEARCH_EXACT,true);
if (dirtyCount == 0) {
// System.out.println("The bean to look is not initialized... do not update.");
return bean;
}
ps.executeUpdate();
// listener callback
this.listenerContainer.afterUpdate(bean);
bean.resetIsModified();
return bean;
}
catch(SQLException e)
{
throw new DataAccessException(e);
}
finally
{
// listener callback
this.listenerContainer.done();
sql = null;
this.getManager().close(ps);
this.freeConnection(c);
}
}
//_____________________________________________________________________
//
// USING TEMPLATE
//_____________________________________________________________________
//18
@Override
public FlLogLightBean loadUniqueUsingTemplate(FlLogLightBean bean) throws DaoException
{
List beans = this.loadUsingTemplateAsList(bean);
switch(beans.size()){
case 0:
return null;
case 1:
return beans.get(0);
default:
throw new ObjectRetrievalException("More than one element !!");
}
}
//18-1
@Override
public FlLogLightBean loadUniqueUsingTemplateChecked(FlLogLightBean bean) throws DaoException
{
List beans = this.loadUsingTemplateAsList(bean);
switch(beans.size()){
case 0:
throw new ObjectRetrievalException("Not found element !!");
case 1:
return beans.get(0);
default:
throw new ObjectRetrievalException("More than one element !!");
}
}
//20-5
@Override
public int loadUsingTemplate(FlLogLightBean bean, int[] fieldList, int startRow, int numRows,int searchType, Action action) throws DaoException
{
// System.out.println("loadUsingTemplate startRow:" + startRow + ", numRows:" + numRows + ", searchType:" + searchType);
StringBuilder sqlWhere = new StringBuilder("");
String sql=createSelectSql(fieldList,this.fillWhere(sqlWhere, bean, searchType) > 0?" WHERE "+sqlWhere.toString():null);
PreparedStatement ps = null;
Connection connection = null;
try {
connection = this.getConnection();
ps = connection.prepareStatement(sql,
ResultSet.TYPE_FORWARD_ONLY,
ResultSet.CONCUR_READ_ONLY);
this.fillPreparedStatement(ps, bean, searchType,false);
return this.loadByPreparedStatement(ps, fieldList, startRow, numRows, action);
} catch (DaoException e) {
throw e;
}catch (SQLException e) {
throw new DataAccessException(e);
} finally {
this.getManager().close(ps);
this.freeConnection(connection);
}
}
//21
@Override
public int deleteUsingTemplate(FlLogLightBean bean) throws DaoException
{
if( !this.listenerContainer.isEmpty()){
final DeleteBeanAction action=new DeleteBeanAction();
this.loadUsingTemplate(bean,action);
return action.getCount();
}
Connection c = null;
PreparedStatement ps = null;
StringBuilder sql = new StringBuilder("DELETE FROM fl_log_light ");
StringBuilder sqlWhere = new StringBuilder("");
try
{
if (this.fillWhere(sqlWhere, bean, SEARCH_EXACT) > 0)
{
sql.append(" WHERE ").append(sqlWhere);
}
else
{
// System.out.println("The bean to look is not initialized... deleting all");
}
// System.out.println("deleteUsingTemplate: " + sql.toString());
c = this.getConnection();
ps = c.prepareStatement(sql.toString(),
ResultSet.TYPE_SCROLL_INSENSITIVE,
ResultSet.CONCUR_READ_ONLY);
this.fillPreparedStatement(ps, bean, SEARCH_EXACT, false);
return ps.executeUpdate();
}
catch(SQLException e)
{
throw new DataAccessException(e);
}
finally
{
this.getManager().close(ps);
this.freeConnection(c);
sql = null;
sqlWhere = null;
}
}
//_____________________________________________________________________
//
// COUNT
//_____________________________________________________________________
//25
@Override
public int countWhere(String where) throws DaoException
{
String sql = new StringBuffer("SELECT COUNT(*) AS MCOUNT FROM fl_log_light ")
.append(null == where ? "" : where).toString();
// System.out.println("countWhere: " + sql);
Connection c = null;
Statement st = null;
ResultSet rs = null;
try
{
int iReturn = -1;
c = this.getConnection();
st = c.createStatement();
rs = st.executeQuery(sql);
if (rs.next())
{
iReturn = rs.getInt("MCOUNT");
}
if (iReturn != -1) {
return iReturn;
}
}
catch(SQLException e)
{
throw new DataAccessException(e);
}
finally
{
this.getManager().close(st, rs);
this.freeConnection(c);
sql = null;
}
throw new DataAccessException("Error in countWhere where=[" + where + "]");
}
//26
/**
* Retrieves the number of rows of the table fl_log_light with a prepared statement.
*
* @param ps the PreparedStatement to be used
* @return the number of rows returned
* @throws DaoException
*/
private int countByPreparedStatement(PreparedStatement ps) throws DaoException
{
ResultSet rs = null;
try
{
int iReturn = -1;
rs = ps.executeQuery();
if (rs.next()) {
iReturn = rs.getInt("MCOUNT");
}
if (iReturn != -1) {
return iReturn;
}
}
catch(SQLException e)
{
throw new DataAccessException(e);
}
finally
{
this.getManager().close(rs);
}
throw new DataAccessException("Error in countByPreparedStatement");
}
//20
/**
* count the number of elements of a specific FlLogLightBean bean given the search type
*
* @param bean the FlLogLightBean template to look for
* @param searchType exact ? like ? starting like ?
* @return the number of rows returned
* @throws DaoException
*/
@Override
public int countUsingTemplate(FlLogLightBean bean, int searchType) throws DaoException
{
Connection c = null;
PreparedStatement ps = null;
StringBuilder sql = new StringBuilder("SELECT COUNT(*) AS MCOUNT FROM fl_log_light");
StringBuilder sqlWhere = new StringBuilder("");
try
{
if (this.fillWhere(sqlWhere, bean, SEARCH_EXACT) > 0)
{
sql.append(" WHERE ").append(sqlWhere);
}
else
{
// System.out.println("The bean to look is not initialized... counting all...");
}
// System.out.println("countUsingTemplate: " + sql.toString());
c = this.getConnection();
ps = c.prepareStatement(sql.toString(),
ResultSet.TYPE_SCROLL_INSENSITIVE,
ResultSet.CONCUR_READ_ONLY);
this.fillPreparedStatement(ps, bean, searchType,false);
return this.countByPreparedStatement(ps);
}
catch(SQLException e)
{
throw new DataAccessException(e);
}
finally
{
this.getManager().close(ps);
this.freeConnection(c);
sql = null;
sqlWhere = null;
}
}
/**
* fills the given StringBuilder with the sql where clauses constructed using the bean and the search type
* @param sqlWhere the StringBuilder that will be filled
* @param bean the bean to use for creating the where clauses
* @param searchType exact ? like ? starting like ?
* @return the number of clauses returned
*/
protected int fillWhere(StringBuilder sqlWhere, FlLogLightBean bean, int searchType)
{
if (bean == null) {
return 0;
}
int dirtyCount = 0;
String sqlEqualsOperation = searchType == SEARCH_EXACT ? "=" : " like ";
try
{
if (bean.checkIdModified()) {
dirtyCount ++;
if (bean.getId() == null) {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("id IS NULL");
} else {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("id = ?");
}
}
if (bean.checkPersonIdModified()) {
dirtyCount ++;
if (bean.getPersonId() == null) {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("person_id IS NULL");
} else {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("person_id = ?");
}
}
if (bean.checkNameModified()) {
dirtyCount ++;
if (bean.getName() == null) {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("name IS NULL");
} else {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("name ").append(sqlEqualsOperation).append("?");
}
}
if (bean.checkPapersTypeModified()) {
dirtyCount ++;
if (bean.getPapersType() == null) {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("papers_type IS NULL");
} else {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("papers_type = ?");
}
}
if (bean.checkPapersNumModified()) {
dirtyCount ++;
if (bean.getPapersNum() == null) {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("papers_num IS NULL");
} else {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("papers_num ").append(sqlEqualsOperation).append("?");
}
}
if (bean.checkVerifyTimeModified()) {
dirtyCount ++;
if (bean.getVerifyTime() == null) {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("verify_time IS NULL");
} else {
sqlWhere.append((sqlWhere.length() == 0) ? " " : " AND ").append("verify_time = ?");
}
}
}
finally
{
sqlEqualsOperation = null;
}
return dirtyCount;
}
/**
* fill the given prepared statement with the bean values and a search type
* @param ps the PreparedStatement that will be filled
* @param bean the bean to use for creating the where clauses
* @param searchType exact ? like ? starting like ?
* @param fillNull wether fill null for null field
* @return the number of clauses returned
* @throws DaoException
*/
protected int fillPreparedStatement(PreparedStatement ps, FlLogLightBean bean, int searchType,boolean fillNull) throws DaoException
{
if (bean == null) {
return 0;
}
int dirtyCount = 0;
try
{
if (bean.checkIdModified()) {
// System.out.println("Setting for " + dirtyCount + " [" + bean.getId() + "]");
if (bean.getId() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.INTEGER);} } else { Manager.setInteger(ps, ++dirtyCount, bean.getId()); }
}
if (bean.checkPersonIdModified()) {
// System.out.println("Setting for " + dirtyCount + " [" + bean.getPersonId() + "]");
if (bean.getPersonId() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.INTEGER);} } else { Manager.setInteger(ps, ++dirtyCount, bean.getPersonId()); }
}
if (bean.checkNameModified()) {
switch (searchType) {
case SEARCH_EXACT:
// System.out.println("Setting for " + dirtyCount + " [" + bean.getName() + "]");
if (bean.getName() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.VARCHAR);} } else { ps.setString(++dirtyCount, bean.getName()); }
break;
case SEARCH_LIKE:
// System.out.println("Setting for " + dirtyCount + " [%" + bean.getName() + "%]");
if ( bean.getName() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.VARCHAR);} } else { ps.setString(++dirtyCount, SQL_LIKE_WILDCARD + bean.getName() + SQL_LIKE_WILDCARD); }
break;
case SEARCH_STARTING_LIKE:
// System.out.println("Setting for " + dirtyCount + " [%" + bean.getName() + "]");
if ( bean.getName() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.VARCHAR);} } else { ps.setString(++dirtyCount, SQL_LIKE_WILDCARD + bean.getName()); }
break;
case SEARCH_ENDING_LIKE:
// System.out.println("Setting for " + dirtyCount + " [" + bean.getName() + "%]");
if (bean.getName() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.VARCHAR);} } else { ps.setString(++dirtyCount, bean.getName() + SQL_LIKE_WILDCARD); }
break;
default:
throw new DaoException("Unknown search type " + searchType);
}
}
if (bean.checkPapersTypeModified()) {
// System.out.println("Setting for " + dirtyCount + " [" + bean.getPapersType() + "]");
if (bean.getPapersType() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.TINYINT);} } else { Manager.setInteger(ps, ++dirtyCount, bean.getPapersType()); }
}
if (bean.checkPapersNumModified()) {
switch (searchType) {
case SEARCH_EXACT:
// System.out.println("Setting for " + dirtyCount + " [" + bean.getPapersNum() + "]");
if (bean.getPapersNum() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.VARCHAR);} } else { ps.setString(++dirtyCount, bean.getPapersNum()); }
break;
case SEARCH_LIKE:
// System.out.println("Setting for " + dirtyCount + " [%" + bean.getPapersNum() + "%]");
if ( bean.getPapersNum() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.VARCHAR);} } else { ps.setString(++dirtyCount, SQL_LIKE_WILDCARD + bean.getPapersNum() + SQL_LIKE_WILDCARD); }
break;
case SEARCH_STARTING_LIKE:
// System.out.println("Setting for " + dirtyCount + " [%" + bean.getPapersNum() + "]");
if ( bean.getPapersNum() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.VARCHAR);} } else { ps.setString(++dirtyCount, SQL_LIKE_WILDCARD + bean.getPapersNum()); }
break;
case SEARCH_ENDING_LIKE:
// System.out.println("Setting for " + dirtyCount + " [" + bean.getPapersNum() + "%]");
if (bean.getPapersNum() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.VARCHAR);} } else { ps.setString(++dirtyCount, bean.getPapersNum() + SQL_LIKE_WILDCARD); }
break;
default:
throw new DaoException("Unknown search type " + searchType);
}
}
if (bean.checkVerifyTimeModified()) {
// System.out.println("Setting for " + dirtyCount + " [" + bean.getVerifyTime() + "]");
if (bean.getVerifyTime() == null) {if(fillNull){ ps.setNull(++dirtyCount, Types.TIMESTAMP);} } else { ps.setTimestamp(++dirtyCount, new java.sql.Timestamp(bean.getVerifyTime().getTime())); }
}
}
catch(SQLException e)
{
throw new DataAccessException(e);
}
return dirtyCount;
}
//_____________________________________________________________________
//
// DECODE RESULT SET
//_____________________________________________________________________
//28
/**
* decode a resultset in an array of FlLogLightBean objects
*
* @param rs the resultset to decode
* @param fieldList table of the field's associated constants
* @param startRow the start row to be used (first row = 1, last row = -1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @return the resulting FlLogLightBean table
* @throws DaoException
*/
public FlLogLightBean[] decodeResultSet(ResultSet rs, int[] fieldList, int startRow, int numRows) throws DaoException
{
return this.decodeResultSetAsList(rs, fieldList, startRow, numRows).toArray(new FlLogLightBean[0]);
}
//28-1
/**
* decode a resultset in a list of FlLogLightBean objects
*
* @param rs the resultset to decode
* @param fieldList table of the field's associated constants
* @param startRow the start row to be used (first row = 1, last row = -1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @return the resulting FlLogLightBean table
* @throws DaoException
*/
public List decodeResultSetAsList(ResultSet rs, int[] fieldList, int startRow, int numRows) throws DaoException
{
ListAction action = new ListAction();
actionOnResultSet(rs, fieldList, numRows, numRows, action);
return action.getList();
}
//28-2
/** decode a resultset and call action
* @param rs the resultset to decode
* @param fieldList table of the field's associated constants
* @param startRow the start row to be used (first row = 1, last row = -1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @param action interface obj for do something
* @return the count dealt by action
* @throws DaoException
* @throws IllegalArgumentException
*/
public int actionOnResultSet(ResultSet rs, int[] fieldList, int startRow, int numRows, Action action) throws DaoException{
try{
int count = 0;
if(0!=numRows){
if( startRow<1 ){
throw new IllegalArgumentException("invalid argument:startRow (must >=1)");
}
if( null==action || null==rs ){
throw new IllegalArgumentException("invalid argument:action OR rs (must not be null)");
}
for(;startRow > 1 && rs.next();){
--startRow;
//skip to last of startRow
}
if (fieldList == null) {
if(numRows<0){
for(;rs.next();++count){
action.call(decodeRow(rs, action.getBean()));
}
}else{
for(;rs.next() && count loadByPreparedStatementAsList(PreparedStatement ps) throws DaoException
{
return this.loadByPreparedStatementAsList(ps, null);
}
//33
/**
* Loads all the elements using a prepared statement specifying a list of fields to be retrieved.
*
* @param ps the PreparedStatement to be used
* @param fieldList table of the field's associated constants
* @return an array of FlLogLightBean
* @throws DaoException
*/
public FlLogLightBean[] loadByPreparedStatement(PreparedStatement ps, int[] fieldList) throws DaoException
{
return this.loadByPreparedStatementAsList(ps, fieldList).toArray(new FlLogLightBean[0]);
}
//33
/**
* Loads all the elements using a prepared statement specifying a list of fields to be retrieved.
*
* @param ps the PreparedStatement to be used
* @param fieldList table of the field's associated constants
* @return an array of FlLogLightBean
* @throws DaoException
*/
public List loadByPreparedStatementAsList(PreparedStatement ps, int[] fieldList) throws DaoException
{
return loadByPreparedStatementAsList(ps,fieldList,1,-1);
}
//34
/**
* Loads all the elements using a prepared statement specifying a list of fields to be retrieved,
* and specifying the start row and the number of rows.
*
* @param ps the PreparedStatement to be used
* @param startRow the start row to be used (first row = 1, last row = -1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @param fieldList table of the field's associated constants
* @return an array of FlLogLightBean
* @throws DaoException
*/
public FlLogLightBean[] loadByPreparedStatement(PreparedStatement ps, int[] fieldList, int startRow, int numRows) throws DaoException
{
return loadByPreparedStatementAsList(ps,fieldList,startRow,numRows).toArray(new FlLogLightBean[0]);
}
//34-1
/**
* Loads all the elements using a prepared statement specifying a list of fields to be retrieved,
* and specifying the start row and the number of rows.
*
* @param ps the PreparedStatement to be used
* @param startRow the start row to be used (first row = 1, last row = -1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @param fieldList table of the field's associated constants
* @return an array of FlLogLightBean
* @throws DaoException
*/
public List loadByPreparedStatementAsList(PreparedStatement ps, int[] fieldList, int startRow, int numRows) throws DaoException
{
ListAction action = new ListAction();
loadByPreparedStatement(ps,fieldList,startRow,numRows,action);
return action.getList();
}
//34-2
/**
* Loads each element using a prepared statement specifying a list of fields to be retrieved,
* and specifying the start row and the number of rows
* and dealt by action.
*
* @param ps the PreparedStatement to be used
* @param startRow the start row to be used (first row = 1, last row = -1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @param fieldList table of the field's associated constants
* @param action Action object for do something(not null)
* @return the count dealt by action
* @throws DaoException
*/
public int loadByPreparedStatement(PreparedStatement ps, int[] fieldList, int startRow, int numRows,Action action) throws DaoException
{
ResultSet rs = null;
try {
ps.setFetchSize(100);
rs = ps.executeQuery();
return this.actionOnResultSet(rs, fieldList, startRow, numRows, action);
} catch (DaoException e) {
throw e;
} catch (SQLException e) {
throw new DataAccessException(e);
} finally {
this.getManager().close(rs);
}
}
//_____________________________________________________________________
//
// LISTENER
//_____________________________________________________________________
private final TableListener.ListenerContainer listenerContainer = new TableListener.ListenerContainer();
//35
@Override
public TableListener registerListener(TableListener listener)
{
this.listenerContainer.add(listener);
return listener;
}
//36
/**
* remove listener.
*/
@Override
public void unregisterListener(TableListener listener)
{
this.listenerContainer.remove(listener);
}
//37
@Override
public void fire(TableListener.Event event, FlLogLightBean bean) throws DaoException{
if(null == event){
throw new NullPointerException();
}
event.fire(listenerContainer, bean);
}
//37-1
@Override
public void fire(int event, FlLogLightBean bean) throws DaoException{
try{
fire(TableListener.Event.values()[event],bean);
}catch(ArrayIndexOutOfBoundsException e){
throw new IllegalArgumentException("invalid event id " + event);
}
}
//37-2
/**
* bind foreign key listener to foreign table:
*/
public void bindForeignKeyListenerForDeleteRule(){
}
//37-3
/**
* unbind foreign key listener from all of foreign tables
* @see #bindForeignKeyListenerForDeleteRule()
*/
public void unbindForeignKeyListenerForDeleteRule(){
}
//_____________________________________________________________________
//
// UTILS
//_____________________________________________________________________
//40
/**
* Retrieves the manager object used to get connections.
*
* @return the manager used
*/
private Manager getManager()
{
return Manager.getInstance();
}
//41
/**
* Frees the connection.
*
* @param c the connection to release
*/
private void freeConnection(Connection c)
{
// back to pool
this.getManager().releaseConnection(c);
}
//42
/**
* Gets the connection.
*/
private Connection getConnection() throws DaoException
{
try
{
return this.getManager().getConnection();
}
catch(SQLException e)
{
throw new DataAccessException(e);
}
}
//43
@Override
public boolean isPrimaryKey(String column){
for(String c:PRIMARYKEY_NAMES){
if(c.equalsIgnoreCase(column)){
return true;
}
}
return false;
}
/**
* Fill the given prepared statement with the values in argList
* @param ps the PreparedStatement that will be filled
* @param argList the arguments to use fill given prepared statement
* @throws DaoException
*/
private void fillPrepareStatement(PreparedStatement ps, Object[] argList) throws DaoException{
try {
if (!(argList == null || ps == null)) {
for (int i = 0; i < argList.length; i++) {
if (argList[i].getClass().equals(byte[].class)) {
ps.setBytes(i + 1, (byte[]) argList[i]);
} else {
ps.setObject(i + 1, argList[i]);
}
}
}
} catch (SQLException e) {
throw new DaoException(e);
}
}
@Override
public int loadBySqlForAction(String sql, Object[] argList, int[] fieldList,int startRow, int numRows,Action action) throws DaoException{
PreparedStatement ps = null;
Connection connection = null;
// logger.debug("sql string:\n" + sql + "\n");
try {
connection = this.getConnection();
ps = connection.prepareStatement(sql,
ResultSet.TYPE_FORWARD_ONLY,
ResultSet.CONCUR_READ_ONLY);
fillPrepareStatement(ps, argList);
return this.loadByPreparedStatement(ps, fieldList, startRow, numRows, action);
} catch (DaoException e) {
throw e;
}catch (SQLException e) {
throw new DataAccessException(e);
} finally {
this.getManager().close(ps);
this.freeConnection(connection);
}
}
@Override
public T runAsTransaction(Callable fun) throws DaoException{
return Manager.getInstance().runAsTransaction(fun);
}
class DeleteBeanAction extends Action.BaseAdapter{
private final AtomicInteger count=new AtomicInteger(0);
@Override
public void call(FlLogLightBean bean) throws DaoException {
FlLogLightManager.this.delete(bean);
count.incrementAndGet();
}
int getCount(){
return count.get();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy