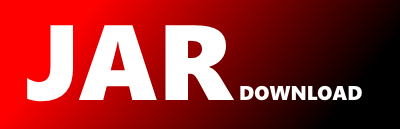
net.gdface.facelog.dborm.permit.FlPermitComparator Maven / Gradle / Ivy
// ______________________________________________________
// Generated by sql2java - https://github.com/10km/sql2java-2-6-7 (custom branch)
// modified by guyadong from
// sql2java original version https://sourceforge.net/projects/sql2java/
// JDBC driver used at code generation time: com.mysql.jdbc.Driver
// template: comparator.java.vm
// ______________________________________________________
package net.gdface.facelog.dborm.permit;
import java.util.Comparator;
import net.gdface.facelog.dborm.Constant;
/**
* Comparator class is used to sort the FlPermitBean objects.
* @author sql2java
*/
public class FlPermitComparator implements Comparator,Constant
{
/**
* Holds the field on which the comparison is performed.
*/
private int iType;
/**
* Value that will contain the information about the order of the sort: normal or reversal.
*/
private boolean bReverse;
/**
* Constructor class for FlPermitComparator.
*
* Example:
*
* Arrays.sort(pArray, new FlPermitComparator(Constant.FL_PERMIT_ID_DEVICE_GROUP_ID, bReverse));
*
* @param iType the field from which you want to sort
*
* Possible values are:
*
* - {@link Constant#FL_PERMIT_ID_DEVICE_GROUP_ID}
*
- {@link Constant#FL_PERMIT_ID_PERSON_GROUP_ID}
*
- {@link Constant#FL_PERMIT_ID_REMARK}
*
- {@link Constant#FL_PERMIT_ID_EXT_BIN}
*
- {@link Constant#FL_PERMIT_ID_EXT_TXT}
*
- {@link Constant#FL_PERMIT_ID_CREATE_TIME}
*
*/
public FlPermitComparator(int iType)
{
this(iType, false);
}
/**
* Constructor class for FlPermitComparator.
*
* Example:
*
* Arrays.sort(pArray, new FlPermitComparator(Constant.FL_PERMIT_ID_DEVICE_GROUP_ID, bReverse));
*
* @param iType the field from which you want to sort.
*
* Possible values are:
*
* - {@link Constant#FL_PERMIT_ID_DEVICE_GROUP_ID})
*
- {@link Constant#FL_PERMIT_ID_PERSON_GROUP_ID})
*
- {@link Constant#FL_PERMIT_ID_REMARK})
*
- {@link Constant#FL_PERMIT_ID_EXT_BIN})
*
- {@link Constant#FL_PERMIT_ID_EXT_TXT})
*
- {@link Constant#FL_PERMIT_ID_CREATE_TIME})
*
*
* @param bReverse set this value to true, if you want to reverse the sorting results
*/
public FlPermitComparator(int iType, boolean bReverse)
{
this.iType = iType;
this.bReverse = bReverse;
}
@Override
public int compare(FlPermitBean b1, FlPermitBean b2)
{
int iReturn = 0;
switch(iType)
{
case FL_PERMIT_ID_DEVICE_GROUP_ID:
if (b1.getDeviceGroupId() == null && b2.getDeviceGroupId() != null) {
iReturn = -1;
} else if (b1.getDeviceGroupId() == null && b2.getDeviceGroupId() == null) {
iReturn = 0;
} else if (b1.getDeviceGroupId() != null && b2.getDeviceGroupId() == null) {
iReturn = 1;
} else {
iReturn = b1.getDeviceGroupId().compareTo(b2.getDeviceGroupId());
}
break;
case FL_PERMIT_ID_PERSON_GROUP_ID:
if (b1.getPersonGroupId() == null && b2.getPersonGroupId() != null) {
iReturn = -1;
} else if (b1.getPersonGroupId() == null && b2.getPersonGroupId() == null) {
iReturn = 0;
} else if (b1.getPersonGroupId() != null && b2.getPersonGroupId() == null) {
iReturn = 1;
} else {
iReturn = b1.getPersonGroupId().compareTo(b2.getPersonGroupId());
}
break;
case FL_PERMIT_ID_REMARK:
if (b1.getRemark() == null && b2.getRemark() != null) {
iReturn = -1;
} else if (b1.getRemark() == null && b2.getRemark() == null) {
iReturn = 0;
} else if (b1.getRemark() != null && b2.getRemark() == null) {
iReturn = 1;
} else {
iReturn = b1.getRemark().compareTo(b2.getRemark());
}
break;
case FL_PERMIT_ID_EXT_BIN:
if (b1.getExtBin() == null && b2.getExtBin() != null) {
iReturn = -1;
} else if (b1.getExtBin() == null && b2.getExtBin() == null) {
iReturn = 0;
} else if (b1.getExtBin() != null && b2.getExtBin() == null) {
iReturn = 1;
} else {
iReturn = b1.getExtBin().compareTo(b2.getExtBin());
}
break;
case FL_PERMIT_ID_EXT_TXT:
if (b1.getExtTxt() == null && b2.getExtTxt() != null) {
iReturn = -1;
} else if (b1.getExtTxt() == null && b2.getExtTxt() == null) {
iReturn = 0;
} else if (b1.getExtTxt() != null && b2.getExtTxt() == null) {
iReturn = 1;
} else {
iReturn = b1.getExtTxt().compareTo(b2.getExtTxt());
}
break;
case FL_PERMIT_ID_CREATE_TIME:
if (b1.getCreateTime() == null && b2.getCreateTime() != null) {
iReturn = -1;
} else if (b1.getCreateTime() == null && b2.getCreateTime() == null) {
iReturn = 0;
} else if (b1.getCreateTime() != null && b2.getCreateTime() == null) {
iReturn = 1;
} else {
iReturn = b1.getCreateTime().compareTo(b2.getCreateTime());
}
break;
default:
throw new IllegalArgumentException("Type passed for the field is not supported");
}
return bReverse ? (-1 * iReturn) : iReturn;
}}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy