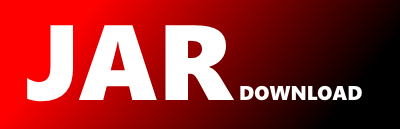
net.gdface.facelog.dborm.person.FlPersonBean Maven / Gradle / Ivy
// ______________________________________________________
// Generated by sql2java - https://github.com/10km/sql2java-2-6-7 (custom branch)
// modified by guyadong from
// sql2java original version https://sourceforge.net/projects/sql2java/
// JDBC driver used at code generation time: com.mysql.jdbc.Driver
// template: bean.java.vm
// ______________________________________________________
package net.gdface.facelog.dborm.person;
import java.io.Serializable;
import java.util.List;
import java.util.Objects;
import net.gdface.facelog.dborm.Constant;
import net.gdface.facelog.dborm.BaseBean;
import net.gdface.facelog.dborm.image.FlImageBean;
import net.gdface.facelog.dborm.CompareToBuilder;
import net.gdface.facelog.dborm.EqualsBuilder;
import net.gdface.facelog.dborm.HashCodeBuilder;
/**
* FlPersonBean is a mapping of fl_person Table.
*
Meta Data Information (in progress):
*
* - comments: 人员基本描述信息
*
* @author guyadong
*/
public class FlPersonBean
implements Serializable,BaseBean,Comparable,Constant,Cloneable
{
private static final long serialVersionUID = -4314407042657759584L;
/** NULL {@link FlPersonBean} bean , IMMUTABLE instance */
public static final FlPersonBean NULL = new FlPersonBean().asNULL().asImmutable();
/** comments:用户id */
private Integer id;
/** comments:所属用户组id */
private Integer groupId;
/** comments:姓名 */
private String name;
/** comments:性别,0:女,1:男,其他:未定义 */
private Integer sex;
/** comments:用户级别,NULL,0:普通用户,2:操作员,3:管理员,其他:未定义 */
private Integer rank;
/** comments:用户密码,MD5 */
private String password;
/** comments:出生日期 */
private java.util.Date birthdate;
/** comments:手机号码 */
private String mobilePhone;
/** comments:证件类型,0:未知,1:身份证,2:护照,3:台胞证,4:港澳通行证,5:军官证,6:外国人居留证,7:员工卡,8:其他 */
private Integer papersType;
/** comments:证件号码 */
private String papersNum;
/** comments:用户默认照片(证件照,标准照)的md5校验码,外键 */
private String imageMd5;
/** comments:验证有效期限(超过期限不能通过验证),为NULL永久有效 */
private java.util.Date expiryDate;
/** comments:备注 */
private String remark;
/** comments:应用项目自定义二进制扩展字段(最大64KB) */
private java.nio.ByteBuffer extBin;
/** comments:应用项目自定义文本扩展字段(最大64KB) */
private String extTxt;
private java.util.Date createTime;
private java.util.Date updateTime;
/** flag whether {@code this} can be modified */
private Boolean immutable;
/** columns modified flag */
private int modified;
/** columns initialized flag */
private int initialized;
/** new record flag */
private boolean isNew;
/**
* set immutable status
* @return {@code this}
*/
private FlPersonBean immutable(Boolean immutable) {
this.immutable = immutable;
return this;
}
/**
* set {@code this} as immutable object
* @return {@code this}
*/
public FlPersonBean asImmutable() {
return immutable(Boolean.TRUE);
}
/**
* @return {@code true} if {@code this} is a mutable object
*/
public boolean mutable(){
return !Boolean.TRUE.equals(this.immutable);
}
/**
* @return {@code this}
* @throws IllegalStateException if {@code this} is a immutable object
*/
private FlPersonBean checkMutable(){
if(!mutable()){
throw new IllegalStateException("this is a immutable object");
}
return this;
}
/**
* @return return a new mutable copy of this object.
*/
public FlPersonBean cloneMutable(){
return clone().immutable(null);
}
@Override
public boolean isNew()
{
return this.isNew;
}
@Override
public void isNew(boolean isNew)
{
this.isNew = isNew;
}
/**
* Specifies to the object if it has been set as new.
*
* @param isNew the boolean value to be assigned to the isNew field
*/
public void setNew(boolean isNew)
{
this.isNew = isNew;
}
/**
* @return the modified status of columns
*/
public int getModified(){
return modified;
}
/**
* @param modified the modified status bit to be assigned to {@link #modified}
*/
public void setModified(int modified){
this.modified = modified;
}
/**
* @return the initialized status of columns
*/
public int getInitialized(){
return initialized;
}
/**
* @param initialized the initialized status bit to be assigned to {@link #initialized}
*/
public void setInitialized(int initialized){
this.initialized = initialized;
}
protected static final >boolean equals(T a, T b) {
return a == b || (a != null && 0==a.compareTo(b));
}
public FlPersonBean(){
super();
reset();
}
/**
* construct a new instance filled with primary keys
* @param id PK# 1
*/
public FlPersonBean(Integer id){
this();
setId(id);
}
/**
* Getter method for {@link #id}.
* PRIMARY KEY.
* Meta Data Information (in progress):
*
* - full name: fl_person.id
* - imported key: fl_log.person_id
* - imported key: fl_feature.person_id
* - comments: 用户id
* - AUTO_INCREMENT
* - NOT NULL
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of id
*/
public Integer getId(){
return id;
}
/**
* Setter method for {@link #id}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to id
*/
public void setId(Integer newVal)
{
checkMutable();
modified |= FL_PERSON_ID_ID_MASK;
initialized |= FL_PERSON_ID_ID_MASK;
if (Objects.equals(newVal, id)) {
return;
}
id = newVal;
}
/**
* Determines if the id has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkIdModified()
{
return 0L != (modified & FL_PERSON_ID_ID_MASK);
}
/**
* Determines if the id has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkIdInitialized()
{
return 0L != (initialized & FL_PERSON_ID_ID_MASK);
}
/**
* Getter method for {@link #groupId}.
* Meta Data Information (in progress):
*
* - full name: fl_person.group_id
* - foreign key: fl_person_group.id
* - comments: 所属用户组id
* - default value: '1'
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of groupId
*/
public Integer getGroupId(){
return groupId;
}
/**
* Setter method for {@link #groupId}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to groupId
*/
public void setGroupId(Integer newVal)
{
checkMutable();
modified |= FL_PERSON_ID_GROUP_ID_MASK;
initialized |= FL_PERSON_ID_GROUP_ID_MASK;
if (Objects.equals(newVal, groupId)) {
return;
}
groupId = newVal;
}
/**
* Determines if the groupId has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkGroupIdModified()
{
return 0L != (modified & FL_PERSON_ID_GROUP_ID_MASK);
}
/**
* Determines if the groupId has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkGroupIdInitialized()
{
return 0L != (initialized & FL_PERSON_ID_GROUP_ID_MASK);
}
/**
* Getter method for {@link #name}.
* Meta Data Information (in progress):
*
* - full name: fl_person.name
* - comments: 姓名
* - NOT NULL
* - column size: 32
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of name
*/
public String getName(){
return name;
}
/**
* Setter method for {@link #name}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to name
*/
public void setName(String newVal)
{
checkMutable();
modified |= FL_PERSON_ID_NAME_MASK;
initialized |= FL_PERSON_ID_NAME_MASK;
if (Objects.equals(newVal, name)) {
return;
}
name = newVal;
}
/**
* Determines if the name has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkNameModified()
{
return 0L != (modified & FL_PERSON_ID_NAME_MASK);
}
/**
* Determines if the name has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkNameInitialized()
{
return 0L != (initialized & FL_PERSON_ID_NAME_MASK);
}
/**
* Getter method for {@link #sex}.
* Meta Data Information (in progress):
*
* - full name: fl_person.sex
* - comments: 性别,0:女,1:男,其他:未定义
* - column size: 3
* - JDBC type returned by the driver: Types.TINYINT
*
*
* @return the value of sex
*/
public Integer getSex(){
return sex;
}
/**
* Setter method for {@link #sex}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to sex
*/
public void setSex(Integer newVal)
{
checkMutable();
modified |= FL_PERSON_ID_SEX_MASK;
initialized |= FL_PERSON_ID_SEX_MASK;
if (Objects.equals(newVal, sex)) {
return;
}
sex = newVal;
}
/**
* Determines if the sex has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkSexModified()
{
return 0L != (modified & FL_PERSON_ID_SEX_MASK);
}
/**
* Determines if the sex has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkSexInitialized()
{
return 0L != (initialized & FL_PERSON_ID_SEX_MASK);
}
/**
* Getter method for {@link #rank}.
* Meta Data Information (in progress):
*
* - full name: fl_person.rank
* - comments: 用户级别,NULL,0:普通用户,2:操作员,3:管理员,其他:未定义
* - column size: 3
* - JDBC type returned by the driver: Types.TINYINT
*
*
* @return the value of rank
*/
public Integer getRank(){
return rank;
}
/**
* Setter method for {@link #rank}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to rank
*/
public void setRank(Integer newVal)
{
checkMutable();
modified |= FL_PERSON_ID_RANK_MASK;
initialized |= FL_PERSON_ID_RANK_MASK;
if (Objects.equals(newVal, rank)) {
return;
}
rank = newVal;
}
/**
* Determines if the rank has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkRankModified()
{
return 0L != (modified & FL_PERSON_ID_RANK_MASK);
}
/**
* Determines if the rank has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkRankInitialized()
{
return 0L != (initialized & FL_PERSON_ID_RANK_MASK);
}
/**
* Getter method for {@link #password}.
* Meta Data Information (in progress):
*
* - full name: fl_person.password
* - comments: 用户密码,MD5
* - column size: 32
* - JDBC type returned by the driver: Types.CHAR
*
*
* @return the value of password
*/
public String getPassword(){
return password;
}
/**
* Setter method for {@link #password}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to password
*/
public void setPassword(String newVal)
{
checkMutable();
modified |= FL_PERSON_ID_PASSWORD_MASK;
initialized |= FL_PERSON_ID_PASSWORD_MASK;
if (Objects.equals(newVal, password)) {
return;
}
password = newVal;
}
/**
* Determines if the password has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkPasswordModified()
{
return 0L != (modified & FL_PERSON_ID_PASSWORD_MASK);
}
/**
* Determines if the password has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkPasswordInitialized()
{
return 0L != (initialized & FL_PERSON_ID_PASSWORD_MASK);
}
/**
* Getter method for {@link #birthdate}.
* Meta Data Information (in progress):
*
* - full name: fl_person.birthdate
* - comments: 出生日期
* - column size: 10
* - JDBC type returned by the driver: Types.DATE
*
*
* @return the value of birthdate
*/
public java.util.Date getBirthdate(){
return birthdate;
}
/**
* Setter method for {@link #birthdate}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to birthdate
*/
public void setBirthdate(java.util.Date newVal)
{
checkMutable();
modified |= FL_PERSON_ID_BIRTHDATE_MASK;
initialized |= FL_PERSON_ID_BIRTHDATE_MASK;
if (Objects.equals(newVal, birthdate)) {
return;
}
birthdate = newVal;
}
/**
* Setter method for {@link #birthdate}.
* @param newVal the number of milliseconds since January 1, 1970, 00:00:00 GMT represented by this Date object.
*/
public void setBirthdate(Long newVal)
{
setBirthdate(null == newVal ? null : new java.util.Date(newVal));
}
/**
* Determines if the birthdate has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkBirthdateModified()
{
return 0L != (modified & FL_PERSON_ID_BIRTHDATE_MASK);
}
/**
* Determines if the birthdate has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkBirthdateInitialized()
{
return 0L != (initialized & FL_PERSON_ID_BIRTHDATE_MASK);
}
/**
* Getter method for {@link #mobilePhone}.
* Meta Data Information (in progress):
*
* - full name: fl_person.mobile_phone
* - comments: 手机号码
* - column size: 11
* - JDBC type returned by the driver: Types.CHAR
*
*
* @return the value of mobilePhone
*/
public String getMobilePhone(){
return mobilePhone;
}
/**
* Setter method for {@link #mobilePhone}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to mobilePhone
*/
public void setMobilePhone(String newVal)
{
checkMutable();
modified |= FL_PERSON_ID_MOBILE_PHONE_MASK;
initialized |= FL_PERSON_ID_MOBILE_PHONE_MASK;
if (Objects.equals(newVal, mobilePhone)) {
return;
}
mobilePhone = newVal;
}
/**
* Determines if the mobilePhone has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkMobilePhoneModified()
{
return 0L != (modified & FL_PERSON_ID_MOBILE_PHONE_MASK);
}
/**
* Determines if the mobilePhone has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkMobilePhoneInitialized()
{
return 0L != (initialized & FL_PERSON_ID_MOBILE_PHONE_MASK);
}
/**
* Getter method for {@link #papersType}.
* Meta Data Information (in progress):
*
* - full name: fl_person.papers_type
* - comments: 证件类型,0:未知,1:身份证,2:护照,3:台胞证,4:港澳通行证,5:军官证,6:外国人居留证,7:员工卡,8:其他
* - column size: 3
* - JDBC type returned by the driver: Types.TINYINT
*
*
* @return the value of papersType
*/
public Integer getPapersType(){
return papersType;
}
/**
* Setter method for {@link #papersType}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to papersType
*/
public void setPapersType(Integer newVal)
{
checkMutable();
modified |= FL_PERSON_ID_PAPERS_TYPE_MASK;
initialized |= FL_PERSON_ID_PAPERS_TYPE_MASK;
if (Objects.equals(newVal, papersType)) {
return;
}
papersType = newVal;
}
/**
* Determines if the papersType has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkPapersTypeModified()
{
return 0L != (modified & FL_PERSON_ID_PAPERS_TYPE_MASK);
}
/**
* Determines if the papersType has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkPapersTypeInitialized()
{
return 0L != (initialized & FL_PERSON_ID_PAPERS_TYPE_MASK);
}
/**
* Getter method for {@link #papersNum}.
* Meta Data Information (in progress):
*
* - full name: fl_person.papers_num
* - comments: 证件号码
* - column size: 32
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of papersNum
*/
public String getPapersNum(){
return papersNum;
}
/**
* Setter method for {@link #papersNum}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to papersNum
*/
public void setPapersNum(String newVal)
{
checkMutable();
modified |= FL_PERSON_ID_PAPERS_NUM_MASK;
initialized |= FL_PERSON_ID_PAPERS_NUM_MASK;
if (Objects.equals(newVal, papersNum)) {
return;
}
papersNum = newVal;
}
/**
* Determines if the papersNum has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkPapersNumModified()
{
return 0L != (modified & FL_PERSON_ID_PAPERS_NUM_MASK);
}
/**
* Determines if the papersNum has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkPapersNumInitialized()
{
return 0L != (initialized & FL_PERSON_ID_PAPERS_NUM_MASK);
}
/**
* Getter method for {@link #imageMd5}.
* Meta Data Information (in progress):
*
* - full name: fl_person.image_md5
* - foreign key: fl_image.md5
* - comments: 用户默认照片(证件照,标准照)的md5校验码,外键
* - column size: 32
* - JDBC type returned by the driver: Types.CHAR
*
*
* @return the value of imageMd5
*/
public String getImageMd5(){
return imageMd5;
}
/**
* Setter method for {@link #imageMd5}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to imageMd5
*/
public void setImageMd5(String newVal)
{
checkMutable();
modified |= FL_PERSON_ID_IMAGE_MD5_MASK;
initialized |= FL_PERSON_ID_IMAGE_MD5_MASK;
if (Objects.equals(newVal, imageMd5)) {
return;
}
imageMd5 = newVal;
}
/**
* Determines if the imageMd5 has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkImageMd5Modified()
{
return 0L != (modified & FL_PERSON_ID_IMAGE_MD5_MASK);
}
/**
* Determines if the imageMd5 has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkImageMd5Initialized()
{
return 0L != (initialized & FL_PERSON_ID_IMAGE_MD5_MASK);
}
/**
* Getter method for {@link #expiryDate}.
* Meta Data Information (in progress):
*
* - full name: fl_person.expiry_date
* - comments: 验证有效期限(超过期限不能通过验证),为NULL永久有效
* - default value: '2050-12-31'
* - column size: 10
* - JDBC type returned by the driver: Types.DATE
*
*
* @return the value of expiryDate
*/
public java.util.Date getExpiryDate(){
return expiryDate;
}
/**
* Setter method for {@link #expiryDate}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to expiryDate
*/
public void setExpiryDate(java.util.Date newVal)
{
checkMutable();
modified |= FL_PERSON_ID_EXPIRY_DATE_MASK;
initialized |= FL_PERSON_ID_EXPIRY_DATE_MASK;
if (Objects.equals(newVal, expiryDate)) {
return;
}
expiryDate = newVal;
}
/**
* Setter method for {@link #expiryDate}.
* @param newVal the number of milliseconds since January 1, 1970, 00:00:00 GMT represented by this Date object.
*/
public void setExpiryDate(Long newVal)
{
setExpiryDate(null == newVal ? null : new java.util.Date(newVal));
}
/**
* Determines if the expiryDate has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkExpiryDateModified()
{
return 0L != (modified & FL_PERSON_ID_EXPIRY_DATE_MASK);
}
/**
* Determines if the expiryDate has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkExpiryDateInitialized()
{
return 0L != (initialized & FL_PERSON_ID_EXPIRY_DATE_MASK);
}
/**
* Getter method for {@link #remark}.
* Meta Data Information (in progress):
*
* - full name: fl_person.remark
* - comments: 备注
* - column size: 256
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of remark
*/
public String getRemark(){
return remark;
}
/**
* Setter method for {@link #remark}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to remark
*/
public void setRemark(String newVal)
{
checkMutable();
modified |= FL_PERSON_ID_REMARK_MASK;
initialized |= FL_PERSON_ID_REMARK_MASK;
if (Objects.equals(newVal, remark)) {
return;
}
remark = newVal;
}
/**
* Determines if the remark has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkRemarkModified()
{
return 0L != (modified & FL_PERSON_ID_REMARK_MASK);
}
/**
* Determines if the remark has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkRemarkInitialized()
{
return 0L != (initialized & FL_PERSON_ID_REMARK_MASK);
}
/**
* Getter method for {@link #extBin}.
* Meta Data Information (in progress):
*
* - full name: fl_person.ext_bin
* - comments: 应用项目自定义二进制扩展字段(最大64KB)
* - column size: 65535
* - JDBC type returned by the driver: Types.LONGVARBINARY
*
*
* @return the value of extBin
*/
public java.nio.ByteBuffer getExtBin(){
return extBin;
}
/**
* Setter method for {@link #extBin}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to extBin
*/
public void setExtBin(java.nio.ByteBuffer newVal)
{
checkMutable();
modified |= FL_PERSON_ID_EXT_BIN_MASK;
initialized |= FL_PERSON_ID_EXT_BIN_MASK;
if (Objects.equals(newVal, extBin)) {
return;
}
extBin = newVal;
}
/**
* Determines if the extBin has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkExtBinModified()
{
return 0L != (modified & FL_PERSON_ID_EXT_BIN_MASK);
}
/**
* Determines if the extBin has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkExtBinInitialized()
{
return 0L != (initialized & FL_PERSON_ID_EXT_BIN_MASK);
}
/**
* Getter method for {@link #extTxt}.
* Meta Data Information (in progress):
*
* - full name: fl_person.ext_txt
* - comments: 应用项目自定义文本扩展字段(最大64KB)
* - column size: 65535
* - JDBC type returned by the driver: Types.LONGVARCHAR
*
*
* @return the value of extTxt
*/
public String getExtTxt(){
return extTxt;
}
/**
* Setter method for {@link #extTxt}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to extTxt
*/
public void setExtTxt(String newVal)
{
checkMutable();
modified |= FL_PERSON_ID_EXT_TXT_MASK;
initialized |= FL_PERSON_ID_EXT_TXT_MASK;
if (Objects.equals(newVal, extTxt)) {
return;
}
extTxt = newVal;
}
/**
* Determines if the extTxt has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkExtTxtModified()
{
return 0L != (modified & FL_PERSON_ID_EXT_TXT_MASK);
}
/**
* Determines if the extTxt has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkExtTxtInitialized()
{
return 0L != (initialized & FL_PERSON_ID_EXT_TXT_MASK);
}
/**
* Getter method for {@link #createTime}.
* Meta Data Information (in progress):
*
* - full name: fl_person.create_time
* - default value: 'CURRENT_TIMESTAMP'
* - NOT NULL
* - column size: 19
* - JDBC type returned by the driver: Types.TIMESTAMP
*
*
* @return the value of createTime
*/
public java.util.Date getCreateTime(){
return createTime;
}
/**
* Setter method for {@link #createTime}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to createTime
*/
public void setCreateTime(java.util.Date newVal)
{
checkMutable();
modified |= FL_PERSON_ID_CREATE_TIME_MASK;
initialized |= FL_PERSON_ID_CREATE_TIME_MASK;
if (Objects.equals(newVal, createTime)) {
return;
}
createTime = newVal;
}
/**
* Setter method for {@link #createTime}.
* @param newVal the number of milliseconds since January 1, 1970, 00:00:00 GMT represented by this Date object.
*/
public void setCreateTime(Long newVal)
{
setCreateTime(null == newVal ? null : new java.util.Date(newVal));
}
/**
* Determines if the createTime has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkCreateTimeModified()
{
return 0L != (modified & FL_PERSON_ID_CREATE_TIME_MASK);
}
/**
* Determines if the createTime has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkCreateTimeInitialized()
{
return 0L != (initialized & FL_PERSON_ID_CREATE_TIME_MASK);
}
/**
* Getter method for {@link #updateTime}.
* Meta Data Information (in progress):
*
* - full name: fl_person.update_time
* - default value: 'CURRENT_TIMESTAMP'
* - NOT NULL
* - column size: 19
* - JDBC type returned by the driver: Types.TIMESTAMP
*
*
* @return the value of updateTime
*/
public java.util.Date getUpdateTime(){
return updateTime;
}
/**
* Setter method for {@link #updateTime}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to updateTime
*/
public void setUpdateTime(java.util.Date newVal)
{
checkMutable();
modified |= FL_PERSON_ID_UPDATE_TIME_MASK;
initialized |= FL_PERSON_ID_UPDATE_TIME_MASK;
if (Objects.equals(newVal, updateTime)) {
return;
}
updateTime = newVal;
}
/**
* Setter method for {@link #updateTime}.
* @param newVal the number of milliseconds since January 1, 1970, 00:00:00 GMT represented by this Date object.
*/
public void setUpdateTime(Long newVal)
{
setUpdateTime(null == newVal ? null : new java.util.Date(newVal));
}
/**
* Determines if the updateTime has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkUpdateTimeModified()
{
return 0L != (modified & FL_PERSON_ID_UPDATE_TIME_MASK);
}
/**
* Determines if the updateTime has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkUpdateTimeInitialized()
{
return 0L != (initialized & FL_PERSON_ID_UPDATE_TIME_MASK);
}
//////////////////////////////////////
// referenced bean for FOREIGN KEYS
//////////////////////////////////////
/**
* The referenced {@link FlImageBean} by {@link #imageMd5} .
* FOREIGN KEY (image_md5) REFERENCES fl_image(md5)
*/
private FlImageBean referencedByImageMd5;
/**
* Getter method for {@link #referencedByImageMd5}.
* @return FlImageBean
*/
public FlImageBean getReferencedByImageMd5() {
return this.referencedByImageMd5;
}
/**
* Setter method for {@link #referencedByImageMd5}.
* @param reference FlImageBean
*/
public void setReferencedByImageMd5(FlImageBean reference) {
this.referencedByImageMd5 = reference;
}
/**
* The referenced {@link FlPersonGroupBean} by {@link #groupId} .
* FOREIGN KEY (group_id) REFERENCES fl_person_group(id)
*/
private FlPersonGroupBean referencedByGroupId;
/**
* Getter method for {@link #referencedByGroupId}.
* @return FlPersonGroupBean
*/
public FlPersonGroupBean getReferencedByGroupId() {
return this.referencedByGroupId;
}
/**
* Setter method for {@link #referencedByGroupId}.
* @param reference FlPersonGroupBean
*/
public void setReferencedByGroupId(FlPersonGroupBean reference) {
this.referencedByGroupId = reference;
}
@Override
public boolean isModified()
{
return 0 != modified;
}
@Override
public boolean isModified(int columnID){
switch ( columnID ){
case FL_PERSON_ID_ID:
return checkIdModified();
case FL_PERSON_ID_GROUP_ID:
return checkGroupIdModified();
case FL_PERSON_ID_NAME:
return checkNameModified();
case FL_PERSON_ID_SEX:
return checkSexModified();
case FL_PERSON_ID_RANK:
return checkRankModified();
case FL_PERSON_ID_PASSWORD:
return checkPasswordModified();
case FL_PERSON_ID_BIRTHDATE:
return checkBirthdateModified();
case FL_PERSON_ID_MOBILE_PHONE:
return checkMobilePhoneModified();
case FL_PERSON_ID_PAPERS_TYPE:
return checkPapersTypeModified();
case FL_PERSON_ID_PAPERS_NUM:
return checkPapersNumModified();
case FL_PERSON_ID_IMAGE_MD5:
return checkImageMd5Modified();
case FL_PERSON_ID_EXPIRY_DATE:
return checkExpiryDateModified();
case FL_PERSON_ID_REMARK:
return checkRemarkModified();
case FL_PERSON_ID_EXT_BIN:
return checkExtBinModified();
case FL_PERSON_ID_EXT_TXT:
return checkExtTxtModified();
case FL_PERSON_ID_CREATE_TIME:
return checkCreateTimeModified();
case FL_PERSON_ID_UPDATE_TIME:
return checkUpdateTimeModified();
default:
return false;
}
}
@Override
public boolean isInitialized(int columnID){
switch(columnID) {
case FL_PERSON_ID_ID:
return checkIdInitialized();
case FL_PERSON_ID_GROUP_ID:
return checkGroupIdInitialized();
case FL_PERSON_ID_NAME:
return checkNameInitialized();
case FL_PERSON_ID_SEX:
return checkSexInitialized();
case FL_PERSON_ID_RANK:
return checkRankInitialized();
case FL_PERSON_ID_PASSWORD:
return checkPasswordInitialized();
case FL_PERSON_ID_BIRTHDATE:
return checkBirthdateInitialized();
case FL_PERSON_ID_MOBILE_PHONE:
return checkMobilePhoneInitialized();
case FL_PERSON_ID_PAPERS_TYPE:
return checkPapersTypeInitialized();
case FL_PERSON_ID_PAPERS_NUM:
return checkPapersNumInitialized();
case FL_PERSON_ID_IMAGE_MD5:
return checkImageMd5Initialized();
case FL_PERSON_ID_EXPIRY_DATE:
return checkExpiryDateInitialized();
case FL_PERSON_ID_REMARK:
return checkRemarkInitialized();
case FL_PERSON_ID_EXT_BIN:
return checkExtBinInitialized();
case FL_PERSON_ID_EXT_TXT:
return checkExtTxtInitialized();
case FL_PERSON_ID_CREATE_TIME:
return checkCreateTimeInitialized();
case FL_PERSON_ID_UPDATE_TIME:
return checkUpdateTimeInitialized();
default:
return false;
}
}
@Override
public boolean isModified(String column){
return isModified(columnIDOf(column));
}
@Override
public boolean isInitialized(String column){
return isInitialized(columnIDOf(column));
}
@Override
public void resetIsModified()
{
checkMutable();
modified = 0;
}
@Override
public void resetPrimaryKeysModified()
{
modified &= (~(FL_PERSON_ID_ID_MASK));
}
/**
* Resets columns modification status except primary keys to 'not modified'.
*/
public void resetModifiedExceptPrimaryKeys()
{
modified &= (~(FL_PERSON_ID_GROUP_ID_MASK |
FL_PERSON_ID_NAME_MASK |
FL_PERSON_ID_SEX_MASK |
FL_PERSON_ID_RANK_MASK |
FL_PERSON_ID_PASSWORD_MASK |
FL_PERSON_ID_BIRTHDATE_MASK |
FL_PERSON_ID_MOBILE_PHONE_MASK |
FL_PERSON_ID_PAPERS_TYPE_MASK |
FL_PERSON_ID_PAPERS_NUM_MASK |
FL_PERSON_ID_IMAGE_MD5_MASK |
FL_PERSON_ID_EXPIRY_DATE_MASK |
FL_PERSON_ID_REMARK_MASK |
FL_PERSON_ID_EXT_BIN_MASK |
FL_PERSON_ID_EXT_TXT_MASK |
FL_PERSON_ID_CREATE_TIME_MASK |
FL_PERSON_ID_UPDATE_TIME_MASK));
}
/**
* Resets the object initialization status to 'not initialized'.
*/
private void resetInitialized()
{
initialized = 0;
}
/** reset all fields to initial value, equal to a new bean */
public void reset(){
checkMutable();
this.id = null;
/* DEFAULT:'1'*/
this.groupId = new Integer(1);
this.name = null;
this.sex = null;
this.rank = null;
this.password = null;
this.birthdate = null;
this.mobilePhone = null;
this.papersType = null;
this.papersNum = null;
this.imageMd5 = null;
/* DEFAULT:'2050-12-31'*/
this.expiryDate = new java.text.SimpleDateFormat("yyyy-MM-dd").parse("2050-12-31",new java.text.ParsePosition(0));
this.remark = null;
this.extBin = null;
this.extTxt = null;
/* DEFAULT:'CURRENT_TIMESTAMP'*/
this.createTime = null;
/* DEFAULT:'CURRENT_TIMESTAMP'*/
this.updateTime = null;
this.isNew = true;
this.modified = 0;
this.initialized = (FL_PERSON_ID_GROUP_ID_MASK | FL_PERSON_ID_EXPIRY_DATE_MASK);
}
@Override
public boolean equals(Object object)
{
if (!(object instanceof FlPersonBean)) {
return false;
}
FlPersonBean obj = (FlPersonBean) object;
return new EqualsBuilder()
.append(getId(), obj.getId())
.append(getGroupId(), obj.getGroupId())
.append(getName(), obj.getName())
.append(getSex(), obj.getSex())
.append(getRank(), obj.getRank())
.append(getPassword(), obj.getPassword())
.append(getBirthdate(), obj.getBirthdate())
.append(getMobilePhone(), obj.getMobilePhone())
.append(getPapersType(), obj.getPapersType())
.append(getPapersNum(), obj.getPapersNum())
.append(getImageMd5(), obj.getImageMd5())
.append(getExpiryDate(), obj.getExpiryDate())
.append(getRemark(), obj.getRemark())
.append(getExtBin(), obj.getExtBin())
.append(getExtTxt(), obj.getExtTxt())
.append(getCreateTime(), obj.getCreateTime())
.append(getUpdateTime(), obj.getUpdateTime())
.isEquals();
}
@Override
public int hashCode()
{
return new HashCodeBuilder(-82280557, -700257973)
.append(getId())
.toHashCode();
}
@Override
public String toString() {
return toString(true,false);
}
/**
* cast byte array to HEX string
*
* @param input
* @return {@code null} if {@code input} is null
*/
private static final String toHex(byte[] input) {
if (null == input){
return null;
}
StringBuffer sb = new StringBuffer(input.length * 2);
for (int i = 0; i < input.length; i++) {
sb.append(Character.forDigit((input[i] & 240) >> 4, 16));
sb.append(Character.forDigit(input[i] & 15, 16));
}
return sb.toString();
}
protected static final StringBuilder append(StringBuilder buffer,boolean full,byte[] value){
if(full || null == value){
buffer.append(toHex(value));
}else{
buffer.append(value.length).append(" bytes");
}
return buffer;
}
private static int stringLimit = 64;
private static final int MINIMUM_LIMIT = 16;
protected static final StringBuilder append(StringBuilder buffer,boolean full,String value){
if(full || null == value || value.length() <= stringLimit){
buffer.append(value);
}else{
buffer.append(value.substring(0,stringLimit - 8)).append(" ...").append(value.substring(stringLimit-4,stringLimit));
}
return buffer;
}
protected static final StringBuilder append(StringBuilder buffer,boolean full,T value){
return buffer.append(value);
}
public static final void setStringLimit(int limit){
if(limit < MINIMUM_LIMIT){
throw new IllegalArgumentException(String.format("INVALID limit %d,minimum value %d",limit,MINIMUM_LIMIT));
}
stringLimit = limit;
}
@Override
public String toString(boolean notNull, boolean fullIfStringOrBytes) {
// only output initialized field
StringBuilder builder = new StringBuilder(this.getClass().getName()).append("@").append(Integer.toHexString(this.hashCode())).append("[");
int count = 0;
if(checkIdInitialized()){
if(!notNull || null != getId()){
if(count++ >0){
builder.append(",");
}
builder.append("id=");
append(builder,fullIfStringOrBytes,getId());
}
}
if(checkGroupIdInitialized()){
if(!notNull || null != getGroupId()){
if(count++ >0){
builder.append(",");
}
builder.append("group_id=");
append(builder,fullIfStringOrBytes,getGroupId());
}
}
if(checkNameInitialized()){
if(!notNull || null != getName()){
if(count++ >0){
builder.append(",");
}
builder.append("name=");
append(builder,fullIfStringOrBytes,getName());
}
}
if(checkSexInitialized()){
if(!notNull || null != getSex()){
if(count++ >0){
builder.append(",");
}
builder.append("sex=");
append(builder,fullIfStringOrBytes,getSex());
}
}
if(checkRankInitialized()){
if(!notNull || null != getRank()){
if(count++ >0){
builder.append(",");
}
builder.append("rank=");
append(builder,fullIfStringOrBytes,getRank());
}
}
if(checkPasswordInitialized()){
if(!notNull || null != getPassword()){
if(count++ >0){
builder.append(",");
}
builder.append("password=");
append(builder,fullIfStringOrBytes,getPassword());
}
}
if(checkBirthdateInitialized()){
if(!notNull || null != getBirthdate()){
if(count++ >0){
builder.append(",");
}
builder.append("birthdate=");
append(builder,fullIfStringOrBytes,getBirthdate());
}
}
if(checkMobilePhoneInitialized()){
if(!notNull || null != getMobilePhone()){
if(count++ >0){
builder.append(",");
}
builder.append("mobile_phone=");
append(builder,fullIfStringOrBytes,getMobilePhone());
}
}
if(checkPapersTypeInitialized()){
if(!notNull || null != getPapersType()){
if(count++ >0){
builder.append(",");
}
builder.append("papers_type=");
append(builder,fullIfStringOrBytes,getPapersType());
}
}
if(checkPapersNumInitialized()){
if(!notNull || null != getPapersNum()){
if(count++ >0){
builder.append(",");
}
builder.append("papers_num=");
append(builder,fullIfStringOrBytes,getPapersNum());
}
}
if(checkImageMd5Initialized()){
if(!notNull || null != getImageMd5()){
if(count++ >0){
builder.append(",");
}
builder.append("image_md5=");
append(builder,fullIfStringOrBytes,getImageMd5());
}
}
if(checkExpiryDateInitialized()){
if(!notNull || null != getExpiryDate()){
if(count++ >0){
builder.append(",");
}
builder.append("expiry_date=");
append(builder,fullIfStringOrBytes,getExpiryDate());
}
}
if(checkRemarkInitialized()){
if(!notNull || null != getRemark()){
if(count++ >0){
builder.append(",");
}
builder.append("remark=");
append(builder,fullIfStringOrBytes,getRemark());
}
}
if(checkExtBinInitialized()){
if(!notNull || null != getExtBin()){
if(count++ >0){
builder.append(",");
}
builder.append("ext_bin=");
append(builder,fullIfStringOrBytes,getExtBin());
}
}
if(checkExtTxtInitialized()){
if(!notNull || null != getExtTxt()){
if(count++ >0){
builder.append(",");
}
builder.append("ext_txt=");
append(builder,fullIfStringOrBytes,getExtTxt());
}
}
if(checkCreateTimeInitialized()){
if(!notNull || null != getCreateTime()){
if(count++ >0){
builder.append(",");
}
builder.append("create_time=");
append(builder,fullIfStringOrBytes,getCreateTime());
}
}
if(checkUpdateTimeInitialized()){
if(!notNull || null != getUpdateTime()){
if(count++ >0){
builder.append(",");
}
builder.append("update_time=");
append(builder,fullIfStringOrBytes,getUpdateTime());
}
}
builder.append("]");
return builder.toString();
}
@Override
public int compareTo(FlPersonBean object){
return new CompareToBuilder()
.append(getId(), object.getId())
.append(getGroupId(), object.getGroupId())
.append(getName(), object.getName())
.append(getSex(), object.getSex())
.append(getRank(), object.getRank())
.append(getPassword(), object.getPassword())
.append(getBirthdate(), object.getBirthdate())
.append(getMobilePhone(), object.getMobilePhone())
.append(getPapersType(), object.getPapersType())
.append(getPapersNum(), object.getPapersNum())
.append(getImageMd5(), object.getImageMd5())
.append(getExpiryDate(), object.getExpiryDate())
.append(getRemark(), object.getRemark())
.append(getExtBin(), object.getExtBin())
.append(getExtTxt(), object.getExtTxt())
.append(getCreateTime(), object.getCreateTime())
.append(getUpdateTime(), object.getUpdateTime())
.toComparison();
}
@Override
public FlPersonBean clone(){
try {
return (FlPersonBean) super.clone();
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
}
/**
* Make {@code this} to a NULL bean
* set all fields to null, {@link #modified} and {@link #initialized} be set to 0
* @return {@code this} bean
* @author guyadong
*/
public FlPersonBean asNULL()
{
checkMutable();
setId((Integer)null);
setGroupId((Integer)null);
setName((String)null);
setSex((Integer)null);
setRank((Integer)null);
setPassword((String)null);
setBirthdate((java.util.Date)null);
setMobilePhone((String)null);
setPapersType((Integer)null);
setPapersNum((String)null);
setImageMd5((String)null);
setExpiryDate((java.util.Date)null);
setRemark((String)null);
setExtBin((java.nio.ByteBuffer)null);
setExtTxt((String)null);
setCreateTime((java.util.Date)null);
setUpdateTime((java.util.Date)null);
isNew(true);
resetInitialized();
resetIsModified();
return this;
}
/**
* check whether this bean is a NULL bean
* @return {@code true} if {@link #initialized} be set to zero
* @see #asNULL()
*/
public boolean checkNULL(){
return 0 == getInitialized();
}
/**
* @param source source list
* @return {@code source} replace {@code null} element with null instance({@link #NULL})
*/
public static final List replaceNull(List source){
if(null != source){
for(int i = 0,endIndex = source.size();i replaceNullInstance(List source){
if(null != source){
for(int i = 0,endIndex = source.size();iT getValue(int columnID)
{
switch( columnID ){
case FL_PERSON_ID_ID:
return (T)getId();
case FL_PERSON_ID_GROUP_ID:
return (T)getGroupId();
case FL_PERSON_ID_NAME:
return (T)getName();
case FL_PERSON_ID_SEX:
return (T)getSex();
case FL_PERSON_ID_RANK:
return (T)getRank();
case FL_PERSON_ID_PASSWORD:
return (T)getPassword();
case FL_PERSON_ID_BIRTHDATE:
return (T)getBirthdate();
case FL_PERSON_ID_MOBILE_PHONE:
return (T)getMobilePhone();
case FL_PERSON_ID_PAPERS_TYPE:
return (T)getPapersType();
case FL_PERSON_ID_PAPERS_NUM:
return (T)getPapersNum();
case FL_PERSON_ID_IMAGE_MD5:
return (T)getImageMd5();
case FL_PERSON_ID_EXPIRY_DATE:
return (T)getExpiryDate();
case FL_PERSON_ID_REMARK:
return (T)getRemark();
case FL_PERSON_ID_EXT_BIN:
return (T)getExtBin();
case FL_PERSON_ID_EXT_TXT:
return (T)getExtTxt();
case FL_PERSON_ID_CREATE_TIME:
return (T)getCreateTime();
case FL_PERSON_ID_UPDATE_TIME:
return (T)getUpdateTime();
default:
return null;
}
}
@Override
public void setValue(int columnID,T value)
{
switch( columnID ) {
case FL_PERSON_ID_ID:
setId((Integer)value);
break;
case FL_PERSON_ID_GROUP_ID:
setGroupId((Integer)value);
break;
case FL_PERSON_ID_NAME:
setName((String)value);
break;
case FL_PERSON_ID_SEX:
setSex((Integer)value);
break;
case FL_PERSON_ID_RANK:
setRank((Integer)value);
break;
case FL_PERSON_ID_PASSWORD:
setPassword((String)value);
break;
case FL_PERSON_ID_BIRTHDATE:
setBirthdate((java.util.Date)value);
break;
case FL_PERSON_ID_MOBILE_PHONE:
setMobilePhone((String)value);
break;
case FL_PERSON_ID_PAPERS_TYPE:
setPapersType((Integer)value);
break;
case FL_PERSON_ID_PAPERS_NUM:
setPapersNum((String)value);
break;
case FL_PERSON_ID_IMAGE_MD5:
setImageMd5((String)value);
break;
case FL_PERSON_ID_EXPIRY_DATE:
setExpiryDate((java.util.Date)value);
break;
case FL_PERSON_ID_REMARK:
setRemark((String)value);
break;
case FL_PERSON_ID_EXT_BIN:
setExtBin((java.nio.ByteBuffer)value);
break;
case FL_PERSON_ID_EXT_TXT:
setExtTxt((String)value);
break;
case FL_PERSON_ID_CREATE_TIME:
setCreateTime((java.util.Date)value);
break;
case FL_PERSON_ID_UPDATE_TIME:
setUpdateTime((java.util.Date)value);
break;
default:
break;
}
}
@Override
public T getValue(String column)
{
return getValue(columnIDOf(column));
}
@Override
public void setValue(String column,T value)
{
setValue(columnIDOf(column),value);
}
/**
* @param column column name
* @return column id for the given field name or negative if {@code column} is invalid name
*/
public static int columnIDOf(String column){
int index = FL_PERSON_FIELDS_LIST.indexOf(column);
return index < 0
? FL_PERSON_JAVA_FIELDS_LIST.indexOf(column)
: index;
}
public static final Builder builder(){
return new Builder().reset();
}
/**
* a builder for FlPersonBean,the template instance is thread local variable
* a instance of Builder can be reused.
*/
public static final class Builder{
/** FlPersonBean instance used for template to create new FlPersonBean instance. */
static final ThreadLocal TEMPLATE = new ThreadLocal(){
@Override
protected FlPersonBean initialValue() {
return new FlPersonBean();
}};
private Builder() {}
/**
* reset the bean as template
* @see FlPersonBean#reset()
*/
public Builder reset(){
TEMPLATE.get().reset();
return this;
}
/** set a bean as template,must not be {@code null} */
public Builder template(FlPersonBean bean){
if(null == bean){
throw new NullPointerException();
}
TEMPLATE.set(bean);
return this;
}
/** return a clone instance of {@link #TEMPLATE}*/
public FlPersonBean build(){
return TEMPLATE.get().clone();
}
/**
* fill the field : fl_person.id
* @param id 用户id
* @see FlPersonBean#getId()
* @see FlPersonBean#setId(Integer)
*/
public Builder id(Integer id){
TEMPLATE.get().setId(id);
return this;
}
/**
* fill the field : fl_person.group_id
* @param groupId 所属用户组id
* @see FlPersonBean#getGroupId()
* @see FlPersonBean#setGroupId(Integer)
*/
public Builder groupId(Integer groupId){
TEMPLATE.get().setGroupId(groupId);
return this;
}
/**
* fill the field : fl_person.name
* @param name 姓名
* @see FlPersonBean#getName()
* @see FlPersonBean#setName(String)
*/
public Builder name(String name){
TEMPLATE.get().setName(name);
return this;
}
/**
* fill the field : fl_person.sex
* @param sex 性别,0:女,1:男,其他:未定义
* @see FlPersonBean#getSex()
* @see FlPersonBean#setSex(Integer)
*/
public Builder sex(Integer sex){
TEMPLATE.get().setSex(sex);
return this;
}
/**
* fill the field : fl_person.rank
* @param rank 用户级别,NULL,0:普通用户,2:操作员,3:管理员,其他:未定义
* @see FlPersonBean#getRank()
* @see FlPersonBean#setRank(Integer)
*/
public Builder rank(Integer rank){
TEMPLATE.get().setRank(rank);
return this;
}
/**
* fill the field : fl_person.password
* @param password 用户密码,MD5
* @see FlPersonBean#getPassword()
* @see FlPersonBean#setPassword(String)
*/
public Builder password(String password){
TEMPLATE.get().setPassword(password);
return this;
}
/**
* fill the field : fl_person.birthdate
* @param birthdate 出生日期
* @see FlPersonBean#getBirthdate()
* @see FlPersonBean#setBirthdate(java.util.Date)
*/
public Builder birthdate(java.util.Date birthdate){
TEMPLATE.get().setBirthdate(birthdate);
return this;
}
/**
* fill the field : fl_person.mobile_phone
* @param mobilePhone 手机号码
* @see FlPersonBean#getMobilePhone()
* @see FlPersonBean#setMobilePhone(String)
*/
public Builder mobilePhone(String mobilePhone){
TEMPLATE.get().setMobilePhone(mobilePhone);
return this;
}
/**
* fill the field : fl_person.papers_type
* @param papersType 证件类型,0:未知,1:身份证,2:护照,3:台胞证,4:港澳通行证,5:军官证,6:外国人居留证,7:员工卡,8:其他
* @see FlPersonBean#getPapersType()
* @see FlPersonBean#setPapersType(Integer)
*/
public Builder papersType(Integer papersType){
TEMPLATE.get().setPapersType(papersType);
return this;
}
/**
* fill the field : fl_person.papers_num
* @param papersNum 证件号码
* @see FlPersonBean#getPapersNum()
* @see FlPersonBean#setPapersNum(String)
*/
public Builder papersNum(String papersNum){
TEMPLATE.get().setPapersNum(papersNum);
return this;
}
/**
* fill the field : fl_person.image_md5
* @param imageMd5 用户默认照片(证件照,标准照)的md5校验码,外键
* @see FlPersonBean#getImageMd5()
* @see FlPersonBean#setImageMd5(String)
*/
public Builder imageMd5(String imageMd5){
TEMPLATE.get().setImageMd5(imageMd5);
return this;
}
/**
* fill the field : fl_person.expiry_date
* @param expiryDate 验证有效期限(超过期限不能通过验证),为NULL永久有效
* @see FlPersonBean#getExpiryDate()
* @see FlPersonBean#setExpiryDate(java.util.Date)
*/
public Builder expiryDate(java.util.Date expiryDate){
TEMPLATE.get().setExpiryDate(expiryDate);
return this;
}
/**
* fill the field : fl_person.remark
* @param remark 备注
* @see FlPersonBean#getRemark()
* @see FlPersonBean#setRemark(String)
*/
public Builder remark(String remark){
TEMPLATE.get().setRemark(remark);
return this;
}
/**
* fill the field : fl_person.ext_bin
* @param extBin 应用项目自定义二进制扩展字段(最大64KB)
* @see FlPersonBean#getExtBin()
* @see FlPersonBean#setExtBin(java.nio.ByteBuffer)
*/
public Builder extBin(java.nio.ByteBuffer extBin){
TEMPLATE.get().setExtBin(extBin);
return this;
}
/**
* fill the field : fl_person.ext_txt
* @param extTxt 应用项目自定义文本扩展字段(最大64KB)
* @see FlPersonBean#getExtTxt()
* @see FlPersonBean#setExtTxt(String)
*/
public Builder extTxt(String extTxt){
TEMPLATE.get().setExtTxt(extTxt);
return this;
}
/**
* fill the field : fl_person.create_time
* @param createTime
* @see FlPersonBean#getCreateTime()
* @see FlPersonBean#setCreateTime(java.util.Date)
*/
public Builder createTime(java.util.Date createTime){
TEMPLATE.get().setCreateTime(createTime);
return this;
}
/**
* fill the field : fl_person.update_time
* @param updateTime
* @see FlPersonBean#getUpdateTime()
* @see FlPersonBean#setUpdateTime(java.util.Date)
*/
public Builder updateTime(java.util.Date updateTime){
TEMPLATE.get().setUpdateTime(updateTime);
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy