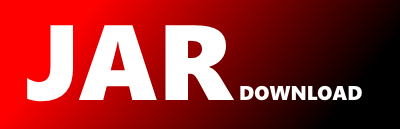
net.gdface.facelog.dborm.person.FlPersonComparator Maven / Gradle / Ivy
// ______________________________________________________
// Generated by sql2java - https://github.com/10km/sql2java-2-6-7 (custom branch)
// modified by guyadong from
// sql2java original version https://sourceforge.net/projects/sql2java/
// JDBC driver used at code generation time: com.mysql.jdbc.Driver
// template: comparator.java.vm
// ______________________________________________________
package net.gdface.facelog.dborm.person;
import java.util.Comparator;
import net.gdface.facelog.dborm.Constant;
/**
* Comparator class is used to sort the FlPersonBean objects.
* @author sql2java
*/
public class FlPersonComparator implements Comparator,Constant
{
/**
* Holds the field on which the comparison is performed.
*/
private int iType;
/**
* Value that will contain the information about the order of the sort: normal or reversal.
*/
private boolean bReverse;
/**
* Constructor class for FlPersonComparator.
*
* Example:
*
* Arrays.sort(pArray, new FlPersonComparator(Constant.FL_PERSON_ID_ID, bReverse));
*
* @param iType the field from which you want to sort
*
* Possible values are:
*
* - {@link Constant#FL_PERSON_ID_ID}
*
- {@link Constant#FL_PERSON_ID_GROUP_ID}
*
- {@link Constant#FL_PERSON_ID_NAME}
*
- {@link Constant#FL_PERSON_ID_SEX}
*
- {@link Constant#FL_PERSON_ID_RANK}
*
- {@link Constant#FL_PERSON_ID_PASSWORD}
*
- {@link Constant#FL_PERSON_ID_BIRTHDATE}
*
- {@link Constant#FL_PERSON_ID_MOBILE_PHONE}
*
- {@link Constant#FL_PERSON_ID_PAPERS_TYPE}
*
- {@link Constant#FL_PERSON_ID_PAPERS_NUM}
*
- {@link Constant#FL_PERSON_ID_IMAGE_MD5}
*
- {@link Constant#FL_PERSON_ID_EXPIRY_DATE}
*
- {@link Constant#FL_PERSON_ID_REMARK}
*
- {@link Constant#FL_PERSON_ID_EXT_BIN}
*
- {@link Constant#FL_PERSON_ID_EXT_TXT}
*
- {@link Constant#FL_PERSON_ID_CREATE_TIME}
*
- {@link Constant#FL_PERSON_ID_UPDATE_TIME}
*
*/
public FlPersonComparator(int iType)
{
this(iType, false);
}
/**
* Constructor class for FlPersonComparator.
*
* Example:
*
* Arrays.sort(pArray, new FlPersonComparator(Constant.FL_PERSON_ID_ID, bReverse));
*
* @param iType the field from which you want to sort.
*
* Possible values are:
*
* - {@link Constant#FL_PERSON_ID_ID})
*
- {@link Constant#FL_PERSON_ID_GROUP_ID})
*
- {@link Constant#FL_PERSON_ID_NAME})
*
- {@link Constant#FL_PERSON_ID_SEX})
*
- {@link Constant#FL_PERSON_ID_RANK})
*
- {@link Constant#FL_PERSON_ID_PASSWORD})
*
- {@link Constant#FL_PERSON_ID_BIRTHDATE})
*
- {@link Constant#FL_PERSON_ID_MOBILE_PHONE})
*
- {@link Constant#FL_PERSON_ID_PAPERS_TYPE})
*
- {@link Constant#FL_PERSON_ID_PAPERS_NUM})
*
- {@link Constant#FL_PERSON_ID_IMAGE_MD5})
*
- {@link Constant#FL_PERSON_ID_EXPIRY_DATE})
*
- {@link Constant#FL_PERSON_ID_REMARK})
*
- {@link Constant#FL_PERSON_ID_EXT_BIN})
*
- {@link Constant#FL_PERSON_ID_EXT_TXT})
*
- {@link Constant#FL_PERSON_ID_CREATE_TIME})
*
- {@link Constant#FL_PERSON_ID_UPDATE_TIME})
*
*
* @param bReverse set this value to true, if you want to reverse the sorting results
*/
public FlPersonComparator(int iType, boolean bReverse)
{
this.iType = iType;
this.bReverse = bReverse;
}
@Override
public int compare(FlPersonBean b1, FlPersonBean b2)
{
int iReturn = 0;
switch(iType)
{
case FL_PERSON_ID_ID:
if (b1.getId() == null && b2.getId() != null) {
iReturn = -1;
} else if (b1.getId() == null && b2.getId() == null) {
iReturn = 0;
} else if (b1.getId() != null && b2.getId() == null) {
iReturn = 1;
} else {
iReturn = b1.getId().compareTo(b2.getId());
}
break;
case FL_PERSON_ID_GROUP_ID:
if (b1.getGroupId() == null && b2.getGroupId() != null) {
iReturn = -1;
} else if (b1.getGroupId() == null && b2.getGroupId() == null) {
iReturn = 0;
} else if (b1.getGroupId() != null && b2.getGroupId() == null) {
iReturn = 1;
} else {
iReturn = b1.getGroupId().compareTo(b2.getGroupId());
}
break;
case FL_PERSON_ID_NAME:
if (b1.getName() == null && b2.getName() != null) {
iReturn = -1;
} else if (b1.getName() == null && b2.getName() == null) {
iReturn = 0;
} else if (b1.getName() != null && b2.getName() == null) {
iReturn = 1;
} else {
iReturn = b1.getName().compareTo(b2.getName());
}
break;
case FL_PERSON_ID_SEX:
if (b1.getSex() == null && b2.getSex() != null) {
iReturn = -1;
} else if (b1.getSex() == null && b2.getSex() == null) {
iReturn = 0;
} else if (b1.getSex() != null && b2.getSex() == null) {
iReturn = 1;
} else {
iReturn = b1.getSex().compareTo(b2.getSex());
}
break;
case FL_PERSON_ID_RANK:
if (b1.getRank() == null && b2.getRank() != null) {
iReturn = -1;
} else if (b1.getRank() == null && b2.getRank() == null) {
iReturn = 0;
} else if (b1.getRank() != null && b2.getRank() == null) {
iReturn = 1;
} else {
iReturn = b1.getRank().compareTo(b2.getRank());
}
break;
case FL_PERSON_ID_PASSWORD:
if (b1.getPassword() == null && b2.getPassword() != null) {
iReturn = -1;
} else if (b1.getPassword() == null && b2.getPassword() == null) {
iReturn = 0;
} else if (b1.getPassword() != null && b2.getPassword() == null) {
iReturn = 1;
} else {
iReturn = b1.getPassword().compareTo(b2.getPassword());
}
break;
case FL_PERSON_ID_BIRTHDATE:
if (b1.getBirthdate() == null && b2.getBirthdate() != null) {
iReturn = -1;
} else if (b1.getBirthdate() == null && b2.getBirthdate() == null) {
iReturn = 0;
} else if (b1.getBirthdate() != null && b2.getBirthdate() == null) {
iReturn = 1;
} else {
iReturn = b1.getBirthdate().compareTo(b2.getBirthdate());
}
break;
case FL_PERSON_ID_MOBILE_PHONE:
if (b1.getMobilePhone() == null && b2.getMobilePhone() != null) {
iReturn = -1;
} else if (b1.getMobilePhone() == null && b2.getMobilePhone() == null) {
iReturn = 0;
} else if (b1.getMobilePhone() != null && b2.getMobilePhone() == null) {
iReturn = 1;
} else {
iReturn = b1.getMobilePhone().compareTo(b2.getMobilePhone());
}
break;
case FL_PERSON_ID_PAPERS_TYPE:
if (b1.getPapersType() == null && b2.getPapersType() != null) {
iReturn = -1;
} else if (b1.getPapersType() == null && b2.getPapersType() == null) {
iReturn = 0;
} else if (b1.getPapersType() != null && b2.getPapersType() == null) {
iReturn = 1;
} else {
iReturn = b1.getPapersType().compareTo(b2.getPapersType());
}
break;
case FL_PERSON_ID_PAPERS_NUM:
if (b1.getPapersNum() == null && b2.getPapersNum() != null) {
iReturn = -1;
} else if (b1.getPapersNum() == null && b2.getPapersNum() == null) {
iReturn = 0;
} else if (b1.getPapersNum() != null && b2.getPapersNum() == null) {
iReturn = 1;
} else {
iReturn = b1.getPapersNum().compareTo(b2.getPapersNum());
}
break;
case FL_PERSON_ID_IMAGE_MD5:
if (b1.getImageMd5() == null && b2.getImageMd5() != null) {
iReturn = -1;
} else if (b1.getImageMd5() == null && b2.getImageMd5() == null) {
iReturn = 0;
} else if (b1.getImageMd5() != null && b2.getImageMd5() == null) {
iReturn = 1;
} else {
iReturn = b1.getImageMd5().compareTo(b2.getImageMd5());
}
break;
case FL_PERSON_ID_EXPIRY_DATE:
if (b1.getExpiryDate() == null && b2.getExpiryDate() != null) {
iReturn = -1;
} else if (b1.getExpiryDate() == null && b2.getExpiryDate() == null) {
iReturn = 0;
} else if (b1.getExpiryDate() != null && b2.getExpiryDate() == null) {
iReturn = 1;
} else {
iReturn = b1.getExpiryDate().compareTo(b2.getExpiryDate());
}
break;
case FL_PERSON_ID_REMARK:
if (b1.getRemark() == null && b2.getRemark() != null) {
iReturn = -1;
} else if (b1.getRemark() == null && b2.getRemark() == null) {
iReturn = 0;
} else if (b1.getRemark() != null && b2.getRemark() == null) {
iReturn = 1;
} else {
iReturn = b1.getRemark().compareTo(b2.getRemark());
}
break;
case FL_PERSON_ID_EXT_BIN:
if (b1.getExtBin() == null && b2.getExtBin() != null) {
iReturn = -1;
} else if (b1.getExtBin() == null && b2.getExtBin() == null) {
iReturn = 0;
} else if (b1.getExtBin() != null && b2.getExtBin() == null) {
iReturn = 1;
} else {
iReturn = b1.getExtBin().compareTo(b2.getExtBin());
}
break;
case FL_PERSON_ID_EXT_TXT:
if (b1.getExtTxt() == null && b2.getExtTxt() != null) {
iReturn = -1;
} else if (b1.getExtTxt() == null && b2.getExtTxt() == null) {
iReturn = 0;
} else if (b1.getExtTxt() != null && b2.getExtTxt() == null) {
iReturn = 1;
} else {
iReturn = b1.getExtTxt().compareTo(b2.getExtTxt());
}
break;
case FL_PERSON_ID_CREATE_TIME:
if (b1.getCreateTime() == null && b2.getCreateTime() != null) {
iReturn = -1;
} else if (b1.getCreateTime() == null && b2.getCreateTime() == null) {
iReturn = 0;
} else if (b1.getCreateTime() != null && b2.getCreateTime() == null) {
iReturn = 1;
} else {
iReturn = b1.getCreateTime().compareTo(b2.getCreateTime());
}
break;
case FL_PERSON_ID_UPDATE_TIME:
if (b1.getUpdateTime() == null && b2.getUpdateTime() != null) {
iReturn = -1;
} else if (b1.getUpdateTime() == null && b2.getUpdateTime() == null) {
iReturn = 0;
} else if (b1.getUpdateTime() != null && b2.getUpdateTime() == null) {
iReturn = 1;
} else {
iReturn = b1.getUpdateTime().compareTo(b2.getUpdateTime());
}
break;
default:
throw new IllegalArgumentException("Type passed for the field is not supported");
}
return bReverse ? (-1 * iReturn) : iReturn;
}}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy