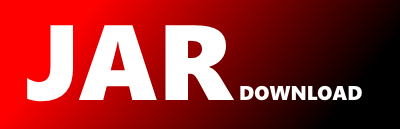
net.gdface.facelog.db.DeviceBean Maven / Gradle / Ivy
// ______________________________________________________
// Generated by sql2java - https://github.com/10km/sql2java
// JDBC driver used at code generation time: com.mysql.jdbc.Driver
// template: bean.java.vm
// ______________________________________________________
package net.gdface.facelog.db;
import java.io.Serializable;
import java.util.Objects;
import gu.sql2java.BaseRow;
import com.facebook.swift.codec.ThriftStruct;
import com.facebook.swift.codec.ThriftField;
import com.facebook.swift.codec.ThriftField.Requiredness;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonIgnore;
import net.gdface.annotation.CodegenLength;
import net.gdface.annotation.CodegenRequired;
import net.gdface.annotation.CodegenDefaultvalue;
import net.gdface.annotation.CodegenInvalidValue;
/**
* DeviceBean is a mapping of fl_device Table.
*
Meta Data Information (in progress):
*
* - comments: 前端设备基本信息
*
* @author guyadong
*/
@ThriftStruct
@ApiModel(description="前端设备基本信息")
public final class DeviceBean extends BaseRow
implements Serializable,Constant
{
private static final long serialVersionUID = -1983784566394161565L;
/** comments:设备id */
@ApiModelProperty(value = "设备id" ,required=true ,dataType="Integer")
@CodegenRequired@CodegenInvalidValue("0")
private Integer id;
/** comments:所属设备组id */
@ApiModelProperty(value = "所属设备组id" ,dataType="Integer")
@CodegenDefaultvalue("1")@CodegenInvalidValue("0")
private Integer groupId;
/** comments:设备名称 */
@ApiModelProperty(value = "设备名称" ,dataType="String")
@CodegenLength(max=32,prealloc=true)@CodegenInvalidValue
private String name;
/** comments:产品名称 */
@ApiModelProperty(value = "产品名称" ,dataType="String")
@CodegenLength(max=32,prealloc=true)@CodegenInvalidValue
private String productName;
/** comments:设备型号 */
@ApiModelProperty(value = "设备型号" ,dataType="String")
@CodegenLength(max=32,prealloc=true)@CodegenInvalidValue
private String model;
/** comments:设备供应商 */
@ApiModelProperty(value = "设备供应商" ,dataType="String")
@CodegenLength(max=32,prealloc=true)@CodegenInvalidValue
private String vendor;
/** comments:设备制造商 */
@ApiModelProperty(value = "设备制造商" ,dataType="String")
@CodegenLength(max=32,prealloc=true)@CodegenInvalidValue
private String manufacturer;
/** comments:设备生产日期 */
@ApiModelProperty(value = "设备生产日期" ,dataType="Date")
@CodegenInvalidValue("0")
private java.util.Date madeDate;
/** comments:设备版本号 */
@ApiModelProperty(value = "设备版本号" ,dataType="String")
@CodegenLength(max=32,prealloc=true)@CodegenInvalidValue
private String version;
/** comments:支持的特征码(算法)版本号列表(逗号分隔),特征版本号用于区分不同人脸识别算法生成的特征数据(SDK版本号命名允许字母,数字,-,.,_符号) */
@ApiModelProperty(value = "支持的特征码(算法)版本号列表(逗号分隔),特征版本号用于区分不同人脸识别算法生成的特征数据(SDK版本号命名允许字母,数字,-,.,_符号)" ,dataType="String")
@CodegenLength(max=128,prealloc=true)@CodegenInvalidValue
private String usedSdks;
/** comments:设备序列号 */
@ApiModelProperty(value = "设备序列号" ,dataType="String")
@CodegenLength(max=32,prealloc=true)@CodegenInvalidValue
private String serialNo;
/** comments:6字节MAC地址(HEX) */
@ApiModelProperty(value = "6字节MAC地址(HEX)" ,dataType="String")
@CodegenLength(value=12,prealloc=true)@CodegenInvalidValue
private String mac;
/** comments:通行方向,NULL,0:入口,1:出口,默认0 */
@ApiModelProperty(value = "通行方向,NULL,0:入口,1:出口,默认0" ,dataType="Integer")
@CodegenInvalidValue("-1")
private Integer direction;
/** comments:备注 */
@ApiModelProperty(value = "备注" ,dataType="String")
@CodegenLength(max=256)@CodegenInvalidValue
private String remark;
/** comments:应用项目自定义二进制扩展字段(最大64KB) */
@ApiModelProperty(value = "应用项目自定义二进制扩展字段(最大64KB)" ,dataType="ByteBuffer")
@CodegenLength(max=65535)@CodegenInvalidValue
private java.nio.ByteBuffer extBin;
/** comments:应用项目自定义文本扩展字段(最大64KB) */
@ApiModelProperty(value = "应用项目自定义文本扩展字段(最大64KB)" ,dataType="String")
@CodegenLength(max=65535)@CodegenInvalidValue
private String extTxt;
@ApiModelProperty(value = "create_time" ,dataType="Date")
@CodegenInvalidValue("0")
private java.util.Date createTime;
@ApiModelProperty(value = "update_time" ,dataType="Date")
@CodegenInvalidValue("0")
private java.util.Date updateTime;
/** comments:设备状态,设备端向上汇报设备状态(设备端写入,应用端只读),JSON格式字符串,由应用项目定义 */
@ApiModelProperty(value = "设备状态,设备端向上汇报设备状态(设备端写入,应用端只读),JSON格式字符串,由应用项目定义" ,dataType="String")
@CodegenLength(max=512)@CodegenInvalidValue
private String status;
/** comments:设备开关,应用端设置设备运行参数,设备端根据相关设置改变运行状态(双向读写),JSON格式字符串,由应用项目定义 */
@ApiModelProperty(value = "设备开关,应用端设置设备运行参数,设备端根据相关设置改变运行状态(双向读写),JSON格式字符串,由应用项目定义" ,dataType="String")
@CodegenLength(max=512)@CodegenInvalidValue
private String options;
/** columns modified flag */
@ApiModelProperty(value="columns modified flag",dataType="int",required=true)
private int modified;
/** columns initialized flag */
@ApiModelProperty(value="columns initialized flag",dataType="int",required=true)
private int initialized;
/** new record flag */
@ApiModelProperty(value="new record flag",dataType="boolean",required=true)
private boolean isNew;
@ThriftField(value=1,name="_new",requiredness=Requiredness.REQUIRED)
@Override
public boolean isNew()
{
return this.isNew;
}
/**
* Specifies to the object if it has been set as new.
*
* @param isNew the boolean value to be assigned to the isNew field
*/
@ThriftField()
@Override
public void setNew(boolean isNew)
{
this.isNew = isNew;
}
/**
* @return the modified status of columns
*/
@ThriftField(value=2,requiredness=Requiredness.REQUIRED)
@Override
public int getModified(){
return modified;
}
/**
* @param modified the modified status bit to be assigned to {@link #modified}
*/
@ThriftField()
@Override
public void setModified(int modified){
this.modified = modified;
}
/**
* @return the initialized status of columns
*/
@ThriftField(value=3,requiredness=Requiredness.REQUIRED)
@Override
public int getInitialized(){
return initialized;
}
/**
* @param initialized the initialized status bit to be assigned to {@link #initialized}
*/
@ThriftField()
@Override
public void setInitialized(int initialized){
this.initialized = initialized;
}
public DeviceBean(){
reset();
}
/**
* construct a new instance filled with primary keys
* @param id PK# 1
*/
public DeviceBean(Integer id){
setId(id);
}
/**
* Getter method for {@link #id}.
* PRIMARY KEY.
* Meta Data Information (in progress):
*
* - full name: fl_device.id
* - imported key: fl_log.device_id
* - imported key: fl_error_log.device_id
* - imported key: fl_image.device_id
* - comments: 设备id
* - AUTO_INCREMENT
* - NOT NULL
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of id
*/
@ThriftField(value=4)
@JsonProperty("id")
public Integer getId(){
return id;
}
/**
* Setter method for {@link #id}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to id
*/
@ThriftField(name="id")
@JsonProperty("id")
public void setId(Integer newVal)
{
modified |= FL_DEVICE_ID_ID_MASK;
initialized |= FL_DEVICE_ID_ID_MASK;
if (Objects.equals(newVal, id)) {
return;
}
id = newVal;
}
/**
* Setter method for {@link #id}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to id
*/
@JsonIgnore
public void setId(int newVal)
{
setId(new Integer(newVal));
}
/**
* Determines if the id has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkIdModified()
{
return 0 != (modified & FL_DEVICE_ID_ID_MASK);
}
/**
* Determines if the id has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkIdInitialized()
{
return 0 != (initialized & FL_DEVICE_ID_ID_MASK);
}
/**
* Getter method for {@link #groupId}.
* Meta Data Information (in progress):
*
* - full name: fl_device.group_id
* - foreign key: fl_device_group.id
* - comments: 所属设备组id
* - default value: '1'
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of groupId
*/
@ThriftField(value=5)
@JsonProperty("groupId")
public Integer getGroupId(){
return groupId;
}
/**
* Setter method for {@link #groupId}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to groupId
*/
@ThriftField(name="groupId")
@JsonProperty("groupId")
public void setGroupId(Integer newVal)
{
modified |= FL_DEVICE_ID_GROUP_ID_MASK;
initialized |= FL_DEVICE_ID_GROUP_ID_MASK;
if (Objects.equals(newVal, groupId)) {
return;
}
groupId = newVal;
}
/**
* Setter method for {@link #groupId}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to groupId
*/
@JsonIgnore
public void setGroupId(int newVal)
{
setGroupId(new Integer(newVal));
}
/**
* Determines if the groupId has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkGroupIdModified()
{
return 0 != (modified & FL_DEVICE_ID_GROUP_ID_MASK);
}
/**
* Determines if the groupId has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkGroupIdInitialized()
{
return 0 != (initialized & FL_DEVICE_ID_GROUP_ID_MASK);
}
/**
* Getter method for {@link #name}.
* Meta Data Information (in progress):
*
* - full name: fl_device.name
* - comments: 设备名称
* - column size: 32
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of name
*/
@ThriftField(value=6)
@JsonProperty("name")
public String getName(){
return name;
}
/**
* Setter method for {@link #name}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to name
*/
@ThriftField(name="name")
@JsonProperty("name")
public void setName(String newVal)
{
modified |= FL_DEVICE_ID_NAME_MASK;
initialized |= FL_DEVICE_ID_NAME_MASK;
if (Objects.equals(newVal, name)) {
return;
}
name = newVal;
}
/**
* Determines if the name has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkNameModified()
{
return 0 != (modified & FL_DEVICE_ID_NAME_MASK);
}
/**
* Determines if the name has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkNameInitialized()
{
return 0 != (initialized & FL_DEVICE_ID_NAME_MASK);
}
/**
* Getter method for {@link #productName}.
* Meta Data Information (in progress):
*
* - full name: fl_device.product_name
* - comments: 产品名称
* - column size: 32
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of productName
*/
@ThriftField(value=7)
@JsonProperty("productName")
public String getProductName(){
return productName;
}
/**
* Setter method for {@link #productName}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to productName
*/
@ThriftField(name="productName")
@JsonProperty("productName")
public void setProductName(String newVal)
{
modified |= FL_DEVICE_ID_PRODUCT_NAME_MASK;
initialized |= FL_DEVICE_ID_PRODUCT_NAME_MASK;
if (Objects.equals(newVal, productName)) {
return;
}
productName = newVal;
}
/**
* Determines if the productName has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkProductNameModified()
{
return 0 != (modified & FL_DEVICE_ID_PRODUCT_NAME_MASK);
}
/**
* Determines if the productName has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkProductNameInitialized()
{
return 0 != (initialized & FL_DEVICE_ID_PRODUCT_NAME_MASK);
}
/**
* Getter method for {@link #model}.
* Meta Data Information (in progress):
*
* - full name: fl_device.model
* - comments: 设备型号
* - column size: 32
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of model
*/
@ThriftField(value=8)
@JsonProperty("model")
public String getModel(){
return model;
}
/**
* Setter method for {@link #model}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to model
*/
@ThriftField(name="model")
@JsonProperty("model")
public void setModel(String newVal)
{
modified |= FL_DEVICE_ID_MODEL_MASK;
initialized |= FL_DEVICE_ID_MODEL_MASK;
if (Objects.equals(newVal, model)) {
return;
}
model = newVal;
}
/**
* Determines if the model has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkModelModified()
{
return 0 != (modified & FL_DEVICE_ID_MODEL_MASK);
}
/**
* Determines if the model has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkModelInitialized()
{
return 0 != (initialized & FL_DEVICE_ID_MODEL_MASK);
}
/**
* Getter method for {@link #vendor}.
* Meta Data Information (in progress):
*
* - full name: fl_device.vendor
* - comments: 设备供应商
* - column size: 32
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of vendor
*/
@ThriftField(value=9)
@JsonProperty("vendor")
public String getVendor(){
return vendor;
}
/**
* Setter method for {@link #vendor}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to vendor
*/
@ThriftField(name="vendor")
@JsonProperty("vendor")
public void setVendor(String newVal)
{
modified |= FL_DEVICE_ID_VENDOR_MASK;
initialized |= FL_DEVICE_ID_VENDOR_MASK;
if (Objects.equals(newVal, vendor)) {
return;
}
vendor = newVal;
}
/**
* Determines if the vendor has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkVendorModified()
{
return 0 != (modified & FL_DEVICE_ID_VENDOR_MASK);
}
/**
* Determines if the vendor has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkVendorInitialized()
{
return 0 != (initialized & FL_DEVICE_ID_VENDOR_MASK);
}
/**
* Getter method for {@link #manufacturer}.
* Meta Data Information (in progress):
*
* - full name: fl_device.manufacturer
* - comments: 设备制造商
* - column size: 32
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of manufacturer
*/
@ThriftField(value=10)
@JsonProperty("manufacturer")
public String getManufacturer(){
return manufacturer;
}
/**
* Setter method for {@link #manufacturer}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to manufacturer
*/
@ThriftField(name="manufacturer")
@JsonProperty("manufacturer")
public void setManufacturer(String newVal)
{
modified |= FL_DEVICE_ID_MANUFACTURER_MASK;
initialized |= FL_DEVICE_ID_MANUFACTURER_MASK;
if (Objects.equals(newVal, manufacturer)) {
return;
}
manufacturer = newVal;
}
/**
* Determines if the manufacturer has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkManufacturerModified()
{
return 0 != (modified & FL_DEVICE_ID_MANUFACTURER_MASK);
}
/**
* Determines if the manufacturer has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkManufacturerInitialized()
{
return 0 != (initialized & FL_DEVICE_ID_MANUFACTURER_MASK);
}
/**
* Getter method for {@link #madeDate}.
* Meta Data Information (in progress):
*
* - full name: fl_device.made_date
* - comments: 设备生产日期
* - column size: 10
* - JDBC type returned by the driver: Types.DATE
*
*
* @return the value of madeDate
*/
@JsonIgnore
public java.util.Date getMadeDate(){
return madeDate;
}
/**
* use Long to represent date type for thrift:swift support
* @see #getMadeDate()
*/
@ThriftField(value=11,name="madeDate")
@JsonProperty("madeDate")
public Long readMadeDate(){
return null == madeDate ? null:madeDate.getTime();
}
/**
* Setter method for {@link #madeDate}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to madeDate
*/
@JsonIgnore
public void setMadeDate(java.util.Date newVal)
{
modified |= FL_DEVICE_ID_MADE_DATE_MASK;
initialized |= FL_DEVICE_ID_MADE_DATE_MASK;
if (Objects.equals(newVal, madeDate)) {
return;
}
madeDate = newVal;
}
/**
* setter for thrift:swift OR jackson support
* without modification for {@link #modified} and {@link #initialized}
* NOTE:DO NOT use the method in your code
*/
@ThriftField(name="madeDate")
@JsonProperty("madeDate")
public void writeMadeDate(Long newVal){
setMadeDate(null == newVal?null:new java.util.Date(newVal));
}
/**
* Setter method for {@link #madeDate}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to madeDate
*/
@JsonIgnore
public void setMadeDate(long newVal)
{
setMadeDate(new java.util.Date(newVal));
}
/**
* Setter method for {@link #madeDate}.
* @param newVal the number of milliseconds since January 1, 1970, 00:00:00 GMT represented by this Date object.
*/
@JsonIgnore
public void setMadeDate(Long newVal)
{
setMadeDate(null == newVal ? null : new java.util.Date(newVal));
}
/**
* Determines if the madeDate has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkMadeDateModified()
{
return 0 != (modified & FL_DEVICE_ID_MADE_DATE_MASK);
}
/**
* Determines if the madeDate has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkMadeDateInitialized()
{
return 0 != (initialized & FL_DEVICE_ID_MADE_DATE_MASK);
}
/**
* Getter method for {@link #version}.
* Meta Data Information (in progress):
*
* - full name: fl_device.version
* - comments: 设备版本号
* - column size: 32
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of version
*/
@ThriftField(value=12)
@JsonProperty("version")
public String getVersion(){
return version;
}
/**
* Setter method for {@link #version}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to version
*/
@ThriftField(name="version")
@JsonProperty("version")
public void setVersion(String newVal)
{
modified |= FL_DEVICE_ID_VERSION_MASK;
initialized |= FL_DEVICE_ID_VERSION_MASK;
if (Objects.equals(newVal, version)) {
return;
}
version = newVal;
}
/**
* Determines if the version has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkVersionModified()
{
return 0 != (modified & FL_DEVICE_ID_VERSION_MASK);
}
/**
* Determines if the version has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkVersionInitialized()
{
return 0 != (initialized & FL_DEVICE_ID_VERSION_MASK);
}
/**
* Getter method for {@link #usedSdks}.
* Meta Data Information (in progress):
*
* - full name: fl_device.used_sdks
* - comments: 支持的特征码(算法)版本号列表(逗号分隔),特征版本号用于区分不同人脸识别算法生成的特征数据(SDK版本号命名允许字母,数字,-,.,_符号)
* - column size: 128
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of usedSdks
*/
@ThriftField(value=13)
@JsonProperty("usedSdks")
public String getUsedSdks(){
return usedSdks;
}
/**
* Setter method for {@link #usedSdks}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to usedSdks
*/
@ThriftField(name="usedSdks")
@JsonProperty("usedSdks")
public void setUsedSdks(String newVal)
{
modified |= FL_DEVICE_ID_USED_SDKS_MASK;
initialized |= FL_DEVICE_ID_USED_SDKS_MASK;
if (Objects.equals(newVal, usedSdks)) {
return;
}
usedSdks = newVal;
}
/**
* Determines if the usedSdks has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkUsedSdksModified()
{
return 0 != (modified & FL_DEVICE_ID_USED_SDKS_MASK);
}
/**
* Determines if the usedSdks has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkUsedSdksInitialized()
{
return 0 != (initialized & FL_DEVICE_ID_USED_SDKS_MASK);
}
/**
* Getter method for {@link #serialNo}.
* Meta Data Information (in progress):
*
* - full name: fl_device.serial_no
* - comments: 设备序列号
* - column size: 32
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of serialNo
*/
@ThriftField(value=14)
@JsonProperty("serialNo")
public String getSerialNo(){
return serialNo;
}
/**
* Setter method for {@link #serialNo}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to serialNo
*/
@ThriftField(name="serialNo")
@JsonProperty("serialNo")
public void setSerialNo(String newVal)
{
modified |= FL_DEVICE_ID_SERIAL_NO_MASK;
initialized |= FL_DEVICE_ID_SERIAL_NO_MASK;
if (Objects.equals(newVal, serialNo)) {
return;
}
serialNo = newVal;
}
/**
* Determines if the serialNo has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkSerialNoModified()
{
return 0 != (modified & FL_DEVICE_ID_SERIAL_NO_MASK);
}
/**
* Determines if the serialNo has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkSerialNoInitialized()
{
return 0 != (initialized & FL_DEVICE_ID_SERIAL_NO_MASK);
}
/**
* Getter method for {@link #mac}.
* Meta Data Information (in progress):
*
* - full name: fl_device.mac
* - comments: 6字节MAC地址(HEX)
* - column size: 12
* - JDBC type returned by the driver: Types.CHAR
*
*
* @return the value of mac
*/
@ThriftField(value=15)
@JsonProperty("mac")
public String getMac(){
return mac;
}
/**
* Setter method for {@link #mac}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to mac
*/
@ThriftField(name="mac")
@JsonProperty("mac")
public void setMac(String newVal)
{
modified |= FL_DEVICE_ID_MAC_MASK;
initialized |= FL_DEVICE_ID_MAC_MASK;
if (Objects.equals(newVal, mac)) {
return;
}
mac = newVal;
}
/**
* Determines if the mac has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkMacModified()
{
return 0 != (modified & FL_DEVICE_ID_MAC_MASK);
}
/**
* Determines if the mac has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkMacInitialized()
{
return 0 != (initialized & FL_DEVICE_ID_MAC_MASK);
}
/**
* Getter method for {@link #direction}.
* Meta Data Information (in progress):
*
* - full name: fl_device.direction
* - comments: 通行方向,NULL,0:入口,1:出口,默认0
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of direction
*/
@ThriftField(value=16)
@JsonProperty("direction")
public Integer getDirection(){
return direction;
}
/**
* Setter method for {@link #direction}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to direction
*/
@ThriftField(name="direction")
@JsonProperty("direction")
public void setDirection(Integer newVal)
{
modified |= FL_DEVICE_ID_DIRECTION_MASK;
initialized |= FL_DEVICE_ID_DIRECTION_MASK;
if (Objects.equals(newVal, direction)) {
return;
}
direction = newVal;
}
/**
* Setter method for {@link #direction}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to direction
*/
@JsonIgnore
public void setDirection(int newVal)
{
setDirection(new Integer(newVal));
}
/**
* Determines if the direction has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkDirectionModified()
{
return 0 != (modified & FL_DEVICE_ID_DIRECTION_MASK);
}
/**
* Determines if the direction has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkDirectionInitialized()
{
return 0 != (initialized & FL_DEVICE_ID_DIRECTION_MASK);
}
/**
* Getter method for {@link #remark}.
* Meta Data Information (in progress):
*
* - full name: fl_device.remark
* - comments: 备注
* - column size: 256
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of remark
*/
@ThriftField(value=17)
@JsonProperty("remark")
public String getRemark(){
return remark;
}
/**
* Setter method for {@link #remark}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to remark
*/
@ThriftField(name="remark")
@JsonProperty("remark")
public void setRemark(String newVal)
{
modified |= FL_DEVICE_ID_REMARK_MASK;
initialized |= FL_DEVICE_ID_REMARK_MASK;
if (Objects.equals(newVal, remark)) {
return;
}
remark = newVal;
}
/**
* Determines if the remark has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkRemarkModified()
{
return 0 != (modified & FL_DEVICE_ID_REMARK_MASK);
}
/**
* Determines if the remark has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkRemarkInitialized()
{
return 0 != (initialized & FL_DEVICE_ID_REMARK_MASK);
}
/**
* Getter method for {@link #extBin}.
* Meta Data Information (in progress):
*
* - full name: fl_device.ext_bin
* - comments: 应用项目自定义二进制扩展字段(最大64KB)
* - column size: 65535
* - JDBC type returned by the driver: Types.LONGVARBINARY
*
*
* @return the value of extBin
*/
@ThriftField(value=18)
@JsonProperty("extBin")
public java.nio.ByteBuffer getExtBin(){
return extBin;
}
/**
* Setter method for {@link #extBin}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to extBin
*/
@ThriftField(name="extBin")
@JsonProperty("extBin")
public void setExtBin(java.nio.ByteBuffer newVal)
{
modified |= FL_DEVICE_ID_EXT_BIN_MASK;
initialized |= FL_DEVICE_ID_EXT_BIN_MASK;
if (Objects.equals(newVal, extBin)) {
return;
}
extBin = newVal;
}
/**
* Determines if the extBin has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkExtBinModified()
{
return 0 != (modified & FL_DEVICE_ID_EXT_BIN_MASK);
}
/**
* Determines if the extBin has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkExtBinInitialized()
{
return 0 != (initialized & FL_DEVICE_ID_EXT_BIN_MASK);
}
/**
* Getter method for {@link #extTxt}.
* Meta Data Information (in progress):
*
* - full name: fl_device.ext_txt
* - comments: 应用项目自定义文本扩展字段(最大64KB)
* - column size: 65535
* - JDBC type returned by the driver: Types.LONGVARCHAR
*
*
* @return the value of extTxt
*/
@ThriftField(value=19)
@JsonProperty("extTxt")
public String getExtTxt(){
return extTxt;
}
/**
* Setter method for {@link #extTxt}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to extTxt
*/
@ThriftField(name="extTxt")
@JsonProperty("extTxt")
public void setExtTxt(String newVal)
{
modified |= FL_DEVICE_ID_EXT_TXT_MASK;
initialized |= FL_DEVICE_ID_EXT_TXT_MASK;
if (Objects.equals(newVal, extTxt)) {
return;
}
extTxt = newVal;
}
/**
* Determines if the extTxt has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkExtTxtModified()
{
return 0 != (modified & FL_DEVICE_ID_EXT_TXT_MASK);
}
/**
* Determines if the extTxt has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkExtTxtInitialized()
{
return 0 != (initialized & FL_DEVICE_ID_EXT_TXT_MASK);
}
/**
* Getter method for {@link #createTime}.
* Meta Data Information (in progress):
*
* - full name: fl_device.create_time
* - default value: 'CURRENT_TIMESTAMP'
* - NOT NULL
* - column size: 19
* - JDBC type returned by the driver: Types.TIMESTAMP
*
*
* @return the value of createTime
*/
@JsonIgnore
public java.util.Date getCreateTime(){
return createTime;
}
/**
* use Long to represent date type for thrift:swift support
* @see #getCreateTime()
*/
@ThriftField(value=20,name="createTime")
@JsonProperty("createTime")
public Long readCreateTime(){
return null == createTime ? null:createTime.getTime();
}
/**
* Setter method for {@link #createTime}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to createTime
*/
@JsonIgnore
public void setCreateTime(java.util.Date newVal)
{
modified |= FL_DEVICE_ID_CREATE_TIME_MASK;
initialized |= FL_DEVICE_ID_CREATE_TIME_MASK;
if (Objects.equals(newVal, createTime)) {
return;
}
createTime = newVal;
}
/**
* setter for thrift:swift OR jackson support
* without modification for {@link #modified} and {@link #initialized}
* NOTE:DO NOT use the method in your code
*/
@ThriftField(name="createTime")
@JsonProperty("createTime")
public void writeCreateTime(Long newVal){
setCreateTime(null == newVal?null:new java.util.Date(newVal));
}
/**
* Setter method for {@link #createTime}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to createTime
*/
@JsonIgnore
public void setCreateTime(long newVal)
{
setCreateTime(new java.util.Date(newVal));
}
/**
* Setter method for {@link #createTime}.
* @param newVal the number of milliseconds since January 1, 1970, 00:00:00 GMT represented by this Date object.
*/
@JsonIgnore
public void setCreateTime(Long newVal)
{
setCreateTime(null == newVal ? null : new java.util.Date(newVal));
}
/**
* Determines if the createTime has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkCreateTimeModified()
{
return 0 != (modified & FL_DEVICE_ID_CREATE_TIME_MASK);
}
/**
* Determines if the createTime has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkCreateTimeInitialized()
{
return 0 != (initialized & FL_DEVICE_ID_CREATE_TIME_MASK);
}
/**
* Getter method for {@link #updateTime}.
* Meta Data Information (in progress):
*
* - full name: fl_device.update_time
* - default value: 'CURRENT_TIMESTAMP'
* - NOT NULL
* - column size: 19
* - JDBC type returned by the driver: Types.TIMESTAMP
*
*
* @return the value of updateTime
*/
@JsonIgnore
public java.util.Date getUpdateTime(){
return updateTime;
}
/**
* use Long to represent date type for thrift:swift support
* @see #getUpdateTime()
*/
@ThriftField(value=21,name="updateTime")
@JsonProperty("updateTime")
public Long readUpdateTime(){
return null == updateTime ? null:updateTime.getTime();
}
/**
* Setter method for {@link #updateTime}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to updateTime
*/
@JsonIgnore
public void setUpdateTime(java.util.Date newVal)
{
modified |= FL_DEVICE_ID_UPDATE_TIME_MASK;
initialized |= FL_DEVICE_ID_UPDATE_TIME_MASK;
if (Objects.equals(newVal, updateTime)) {
return;
}
updateTime = newVal;
}
/**
* setter for thrift:swift OR jackson support
* without modification for {@link #modified} and {@link #initialized}
* NOTE:DO NOT use the method in your code
*/
@ThriftField(name="updateTime")
@JsonProperty("updateTime")
public void writeUpdateTime(Long newVal){
setUpdateTime(null == newVal?null:new java.util.Date(newVal));
}
/**
* Setter method for {@link #updateTime}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to updateTime
*/
@JsonIgnore
public void setUpdateTime(long newVal)
{
setUpdateTime(new java.util.Date(newVal));
}
/**
* Setter method for {@link #updateTime}.
* @param newVal the number of milliseconds since January 1, 1970, 00:00:00 GMT represented by this Date object.
*/
@JsonIgnore
public void setUpdateTime(Long newVal)
{
setUpdateTime(null == newVal ? null : new java.util.Date(newVal));
}
/**
* Determines if the updateTime has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkUpdateTimeModified()
{
return 0 != (modified & FL_DEVICE_ID_UPDATE_TIME_MASK);
}
/**
* Determines if the updateTime has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkUpdateTimeInitialized()
{
return 0 != (initialized & FL_DEVICE_ID_UPDATE_TIME_MASK);
}
/**
* Getter method for {@link #status}.
* Meta Data Information (in progress):
*
* - full name: fl_device.status
* - comments: 设备状态,设备端向上汇报设备状态(设备端写入,应用端只读),JSON格式字符串,由应用项目定义
* - column size: 512
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of status
*/
@ThriftField(value=22)
@JsonProperty("status")
public String getStatus(){
return status;
}
/**
* Setter method for {@link #status}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to status
*/
@ThriftField(name="status")
@JsonProperty("status")
public void setStatus(String newVal)
{
modified |= FL_DEVICE_ID_STATUS_MASK;
initialized |= FL_DEVICE_ID_STATUS_MASK;
if (Objects.equals(newVal, status)) {
return;
}
status = newVal;
}
/**
* Determines if the status has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkStatusModified()
{
return 0 != (modified & FL_DEVICE_ID_STATUS_MASK);
}
/**
* Determines if the status has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkStatusInitialized()
{
return 0 != (initialized & FL_DEVICE_ID_STATUS_MASK);
}
/**
* Getter method for {@link #options}.
* Meta Data Information (in progress):
*
* - full name: fl_device.options
* - comments: 设备开关,应用端设置设备运行参数,设备端根据相关设置改变运行状态(双向读写),JSON格式字符串,由应用项目定义
* - column size: 512
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of options
*/
@ThriftField(value=23)
@JsonProperty("options")
public String getOptions(){
return options;
}
/**
* Setter method for {@link #options}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to options
*/
@ThriftField(name="options")
@JsonProperty("options")
public void setOptions(String newVal)
{
modified |= FL_DEVICE_ID_OPTIONS_MASK;
initialized |= FL_DEVICE_ID_OPTIONS_MASK;
if (Objects.equals(newVal, options)) {
return;
}
options = newVal;
}
/**
* Determines if the options has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkOptionsModified()
{
return 0 != (modified & FL_DEVICE_ID_OPTIONS_MASK);
}
/**
* Determines if the options has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkOptionsInitialized()
{
return 0 != (initialized & FL_DEVICE_ID_OPTIONS_MASK);
}
@Override
public boolean beModified()
{
return 0 != modified;
}
@Override
public boolean isModified(int columnID){
return columnID>=0 && columnID < metaData.columnCount && 0 != (modified & (1 << columnID));
}
@Override
public boolean isInitialized(int columnID){
return columnID>=0 && columnID < metaData.columnCount && 0 != (initialized & (1 << columnID));
}
@Override
public void resetIsModified()
{
modified = 0;
}
@Override
public void resetPrimaryKeysModified()
{
modified &= (~(FL_DEVICE_ID_ID_MASK));
}
/**
* Resets columns modification status except primary keys to 'not modified'.
*/
public void resetModifiedExceptPrimaryKeys()
{
modified &= (~(FL_DEVICE_ID_GROUP_ID_MASK |
FL_DEVICE_ID_NAME_MASK |
FL_DEVICE_ID_PRODUCT_NAME_MASK |
FL_DEVICE_ID_MODEL_MASK |
FL_DEVICE_ID_VENDOR_MASK |
FL_DEVICE_ID_MANUFACTURER_MASK |
FL_DEVICE_ID_MADE_DATE_MASK |
FL_DEVICE_ID_VERSION_MASK |
FL_DEVICE_ID_USED_SDKS_MASK |
FL_DEVICE_ID_SERIAL_NO_MASK |
FL_DEVICE_ID_MAC_MASK |
FL_DEVICE_ID_DIRECTION_MASK |
FL_DEVICE_ID_REMARK_MASK |
FL_DEVICE_ID_EXT_BIN_MASK |
FL_DEVICE_ID_EXT_TXT_MASK |
FL_DEVICE_ID_CREATE_TIME_MASK |
FL_DEVICE_ID_UPDATE_TIME_MASK |
FL_DEVICE_ID_STATUS_MASK |
FL_DEVICE_ID_OPTIONS_MASK));
}
/** reset all fields to initial value, equal to a new bean */
public void reset(){
this.id = null;
/* DEFAULT:'1'*/
this.groupId = new Integer(1);
this.name = null;
this.productName = null;
this.model = null;
this.vendor = null;
this.manufacturer = null;
this.madeDate = null;
this.version = null;
this.usedSdks = null;
this.serialNo = null;
this.mac = null;
this.direction = null;
this.remark = null;
this.extBin = null;
this.extTxt = null;
/* DEFAULT:'CURRENT_TIMESTAMP'*/
this.createTime = null;
/* DEFAULT:'CURRENT_TIMESTAMP'*/
this.updateTime = null;
this.status = null;
this.options = null;
this.isNew = true;
this.modified = 0;
this.initialized = (FL_DEVICE_ID_GROUP_ID_MASK);
}
@Override
public DeviceBean clone(){
return (DeviceBean) super.clone();
}
public static final Builder builder(){
return new Builder().reset();
}
/**
* a builder for DeviceBean,the template instance is thread local variable
* a instance of Builder can be reused.
*/
public static final class Builder{
/** DeviceBean instance used for template to create new DeviceBean instance. */
static final ThreadLocal TEMPLATE = new ThreadLocal(){
@Override
protected DeviceBean initialValue() {
return new DeviceBean();
}};
private Builder() {}
/**
* reset the bean as template
* @see DeviceBean#reset()
*/
public Builder reset(){
TEMPLATE.get().reset();
return this;
}
/** set a bean as template,must not be {@code null} */
public Builder template(DeviceBean bean){
if(null == bean){
throw new NullPointerException();
}
TEMPLATE.set(bean);
return this;
}
/** return a clone instance of {@link #TEMPLATE}*/
public DeviceBean build(){
return TEMPLATE.get().clone();
}
/**
* fill the field : fl_device.id
* @param id 设备id
* @see DeviceBean#getId()
* @see DeviceBean#setId(Integer)
*/
public Builder id(Integer id){
TEMPLATE.get().setId(id);
return this;
}
/**
* fill the field : fl_device.group_id
* @param groupId 所属设备组id
* @see DeviceBean#getGroupId()
* @see DeviceBean#setGroupId(Integer)
*/
public Builder groupId(Integer groupId){
TEMPLATE.get().setGroupId(groupId);
return this;
}
/**
* fill the field : fl_device.name
* @param name 设备名称
* @see DeviceBean#getName()
* @see DeviceBean#setName(String)
*/
public Builder name(String name){
TEMPLATE.get().setName(name);
return this;
}
/**
* fill the field : fl_device.product_name
* @param productName 产品名称
* @see DeviceBean#getProductName()
* @see DeviceBean#setProductName(String)
*/
public Builder productName(String productName){
TEMPLATE.get().setProductName(productName);
return this;
}
/**
* fill the field : fl_device.model
* @param model 设备型号
* @see DeviceBean#getModel()
* @see DeviceBean#setModel(String)
*/
public Builder model(String model){
TEMPLATE.get().setModel(model);
return this;
}
/**
* fill the field : fl_device.vendor
* @param vendor 设备供应商
* @see DeviceBean#getVendor()
* @see DeviceBean#setVendor(String)
*/
public Builder vendor(String vendor){
TEMPLATE.get().setVendor(vendor);
return this;
}
/**
* fill the field : fl_device.manufacturer
* @param manufacturer 设备制造商
* @see DeviceBean#getManufacturer()
* @see DeviceBean#setManufacturer(String)
*/
public Builder manufacturer(String manufacturer){
TEMPLATE.get().setManufacturer(manufacturer);
return this;
}
/**
* fill the field : fl_device.made_date
* @param madeDate 设备生产日期
* @see DeviceBean#getMadeDate()
* @see DeviceBean#setMadeDate(java.util.Date)
*/
public Builder madeDate(java.util.Date madeDate){
TEMPLATE.get().setMadeDate(madeDate);
return this;
}
/**
* fill the field : fl_device.version
* @param version 设备版本号
* @see DeviceBean#getVersion()
* @see DeviceBean#setVersion(String)
*/
public Builder version(String version){
TEMPLATE.get().setVersion(version);
return this;
}
/**
* fill the field : fl_device.used_sdks
* @param usedSdks 支持的特征码(算法)版本号列表(逗号分隔),特征版本号用于区分不同人脸识别算法生成的特征数据(SDK版本号命名允许字母,数字,-,.,_符号)
* @see DeviceBean#getUsedSdks()
* @see DeviceBean#setUsedSdks(String)
*/
public Builder usedSdks(String usedSdks){
TEMPLATE.get().setUsedSdks(usedSdks);
return this;
}
/**
* fill the field : fl_device.serial_no
* @param serialNo 设备序列号
* @see DeviceBean#getSerialNo()
* @see DeviceBean#setSerialNo(String)
*/
public Builder serialNo(String serialNo){
TEMPLATE.get().setSerialNo(serialNo);
return this;
}
/**
* fill the field : fl_device.mac
* @param mac 6字节MAC地址(HEX)
* @see DeviceBean#getMac()
* @see DeviceBean#setMac(String)
*/
public Builder mac(String mac){
TEMPLATE.get().setMac(mac);
return this;
}
/**
* fill the field : fl_device.direction
* @param direction 通行方向,NULL,0:入口,1:出口,默认0
* @see DeviceBean#getDirection()
* @see DeviceBean#setDirection(Integer)
*/
public Builder direction(Integer direction){
TEMPLATE.get().setDirection(direction);
return this;
}
/**
* fill the field : fl_device.remark
* @param remark 备注
* @see DeviceBean#getRemark()
* @see DeviceBean#setRemark(String)
*/
public Builder remark(String remark){
TEMPLATE.get().setRemark(remark);
return this;
}
/**
* fill the field : fl_device.ext_bin
* @param extBin 应用项目自定义二进制扩展字段(最大64KB)
* @see DeviceBean#getExtBin()
* @see DeviceBean#setExtBin(java.nio.ByteBuffer)
*/
public Builder extBin(java.nio.ByteBuffer extBin){
TEMPLATE.get().setExtBin(extBin);
return this;
}
/**
* fill the field : fl_device.ext_txt
* @param extTxt 应用项目自定义文本扩展字段(最大64KB)
* @see DeviceBean#getExtTxt()
* @see DeviceBean#setExtTxt(String)
*/
public Builder extTxt(String extTxt){
TEMPLATE.get().setExtTxt(extTxt);
return this;
}
/**
* fill the field : fl_device.create_time
* @param createTime
* @see DeviceBean#getCreateTime()
* @see DeviceBean#setCreateTime(java.util.Date)
*/
public Builder createTime(java.util.Date createTime){
TEMPLATE.get().setCreateTime(createTime);
return this;
}
/**
* fill the field : fl_device.update_time
* @param updateTime
* @see DeviceBean#getUpdateTime()
* @see DeviceBean#setUpdateTime(java.util.Date)
*/
public Builder updateTime(java.util.Date updateTime){
TEMPLATE.get().setUpdateTime(updateTime);
return this;
}
/**
* fill the field : fl_device.status
* @param status 设备状态,设备端向上汇报设备状态(设备端写入,应用端只读),JSON格式字符串,由应用项目定义
* @see DeviceBean#getStatus()
* @see DeviceBean#setStatus(String)
*/
public Builder status(String status){
TEMPLATE.get().setStatus(status);
return this;
}
/**
* fill the field : fl_device.options
* @param options 设备开关,应用端设置设备运行参数,设备端根据相关设置改变运行状态(双向读写),JSON格式字符串,由应用项目定义
* @see DeviceBean#getOptions()
* @see DeviceBean#setOptions(String)
*/
public Builder options(String options){
TEMPLATE.get().setOptions(options);
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy