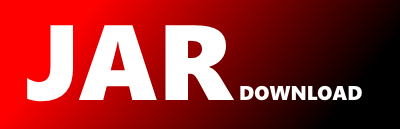
net.gdface.facelog.db.ErrorLogBean Maven / Gradle / Ivy
// ______________________________________________________
// Generated by sql2java - https://github.com/10km/sql2java
// JDBC driver used at code generation time: com.mysql.jdbc.Driver
// template: bean.java.vm
// ______________________________________________________
package net.gdface.facelog.db;
import java.io.Serializable;
import java.util.Objects;
import gu.sql2java.BaseRow;
import com.facebook.swift.codec.ThriftStruct;
import com.facebook.swift.codec.ThriftField;
import com.facebook.swift.codec.ThriftField.Requiredness;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonIgnore;
import net.gdface.annotation.CodegenLength;
import net.gdface.annotation.CodegenRequired;
import net.gdface.annotation.CodegenInvalidValue;
/**
* ErrorLogBean is a mapping of fl_error_log Table.
*
Meta Data Information (in progress):
*
* - comments: 系统错误日志,记录系统产生错误
*
* @author guyadong
*/
@ThriftStruct
@ApiModel(description="系统错误日志,记录系统产生错误")
public final class ErrorLogBean extends BaseRow
implements Serializable,Constant
{
private static final long serialVersionUID = -2413123417676212793L;
/** comments:日志id */
@ApiModelProperty(value = "日志id" ,required=true ,dataType="Integer")
@CodegenRequired@CodegenInvalidValue("0")
private Integer id;
/** comments:错误类型分类,以/分隔的多级类型定义,应用程序可自定义,顶级分类:service(服务异常),device(设备异常),application(应用异常) */
@ApiModelProperty(value = "错误类型分类,以/分隔的多级类型定义,应用程序可自定义,顶级分类:service(服务异常),device(设备异常),application(应用异常)" ,required=true ,dataType="String")
@CodegenLength(max=64,prealloc=true)@CodegenRequired@CodegenInvalidValue
private String catalog;
/** comments:外键,日志来源用户id */
@ApiModelProperty(value = "外键,日志来源用户id" ,dataType="Integer")
@CodegenInvalidValue("0")
private Integer personId;
/** comments:外键,日志来源设备id */
@ApiModelProperty(value = "外键,日志来源设备id" ,dataType="Integer")
@CodegenInvalidValue("0")
private Integer deviceId;
/** comments:异常类名,such as java.io.IOException */
@ApiModelProperty(value = "异常类名,such as java.io.IOException" ,dataType="String")
@CodegenLength(max=256)@CodegenInvalidValue
private String exceptionClass;
/** comments:错误信息 */
@ApiModelProperty(value = "错误信息" ,dataType="String")
@CodegenLength(max=1024)@CodegenInvalidValue
private String message;
/** comments:异常堆栈信息(最大64KB) */
@ApiModelProperty(value = "异常堆栈信息(最大64KB)" ,dataType="String")
@CodegenLength(max=65535)@CodegenInvalidValue
private String stackTrace;
@ApiModelProperty(value = "create_time" ,dataType="Date")
@CodegenInvalidValue("0")
private java.util.Date createTime;
/** columns modified flag */
@ApiModelProperty(value="columns modified flag",dataType="int",required=true)
private int modified;
/** columns initialized flag */
@ApiModelProperty(value="columns initialized flag",dataType="int",required=true)
private int initialized;
/** new record flag */
@ApiModelProperty(value="new record flag",dataType="boolean",required=true)
private boolean isNew;
@ThriftField(value=1,name="_new",requiredness=Requiredness.REQUIRED)
@Override
public boolean isNew()
{
return this.isNew;
}
/**
* Specifies to the object if it has been set as new.
*
* @param isNew the boolean value to be assigned to the isNew field
*/
@ThriftField()
@Override
public void setNew(boolean isNew)
{
this.isNew = isNew;
}
/**
* @return the modified status of columns
*/
@ThriftField(value=2,requiredness=Requiredness.REQUIRED)
@Override
public int getModified(){
return modified;
}
/**
* @param modified the modified status bit to be assigned to {@link #modified}
*/
@ThriftField()
@Override
public void setModified(int modified){
this.modified = modified;
}
/**
* @return the initialized status of columns
*/
@ThriftField(value=3,requiredness=Requiredness.REQUIRED)
@Override
public int getInitialized(){
return initialized;
}
/**
* @param initialized the initialized status bit to be assigned to {@link #initialized}
*/
@ThriftField()
@Override
public void setInitialized(int initialized){
this.initialized = initialized;
}
public ErrorLogBean(){
reset();
}
/**
* construct a new instance filled with primary keys
* @param id PK# 1
*/
public ErrorLogBean(Integer id){
setId(id);
}
/**
* Getter method for {@link #id}.
* PRIMARY KEY.
* Meta Data Information (in progress):
*
* - full name: fl_error_log.id
* - comments: 日志id
* - AUTO_INCREMENT
* - NOT NULL
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of id
*/
@ThriftField(value=4)
@JsonProperty("id")
public Integer getId(){
return id;
}
/**
* Setter method for {@link #id}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to id
*/
@ThriftField(name="id")
@JsonProperty("id")
public void setId(Integer newVal)
{
modified |= FL_ERROR_LOG_ID_ID_MASK;
initialized |= FL_ERROR_LOG_ID_ID_MASK;
if (Objects.equals(newVal, id)) {
return;
}
id = newVal;
}
/**
* Setter method for {@link #id}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to id
*/
@JsonIgnore
public void setId(int newVal)
{
setId(new Integer(newVal));
}
/**
* Determines if the id has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkIdModified()
{
return 0 != (modified & FL_ERROR_LOG_ID_ID_MASK);
}
/**
* Determines if the id has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkIdInitialized()
{
return 0 != (initialized & FL_ERROR_LOG_ID_ID_MASK);
}
/**
* Getter method for {@link #catalog}.
* Meta Data Information (in progress):
*
* - full name: fl_error_log.catalog
* - comments: 错误类型分类,以/分隔的多级类型定义,应用程序可自定义,顶级分类:service(服务异常),device(设备异常),application(应用异常)
* - NOT NULL
* - column size: 64
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of catalog
*/
@ThriftField(value=5)
@JsonProperty("catalog")
public String getCatalog(){
return catalog;
}
/**
* Setter method for {@link #catalog}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to catalog
*/
@ThriftField(name="catalog")
@JsonProperty("catalog")
public void setCatalog(String newVal)
{
modified |= FL_ERROR_LOG_ID_CATALOG_MASK;
initialized |= FL_ERROR_LOG_ID_CATALOG_MASK;
if (Objects.equals(newVal, catalog)) {
return;
}
catalog = newVal;
}
/**
* Determines if the catalog has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkCatalogModified()
{
return 0 != (modified & FL_ERROR_LOG_ID_CATALOG_MASK);
}
/**
* Determines if the catalog has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkCatalogInitialized()
{
return 0 != (initialized & FL_ERROR_LOG_ID_CATALOG_MASK);
}
/**
* Getter method for {@link #personId}.
* Meta Data Information (in progress):
*
* - full name: fl_error_log.person_id
* - foreign key: fl_person.id
* - comments: 外键,日志来源用户id
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of personId
*/
@ThriftField(value=6)
@JsonProperty("personId")
public Integer getPersonId(){
return personId;
}
/**
* Setter method for {@link #personId}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to personId
*/
@ThriftField(name="personId")
@JsonProperty("personId")
public void setPersonId(Integer newVal)
{
modified |= FL_ERROR_LOG_ID_PERSON_ID_MASK;
initialized |= FL_ERROR_LOG_ID_PERSON_ID_MASK;
if (Objects.equals(newVal, personId)) {
return;
}
personId = newVal;
}
/**
* Setter method for {@link #personId}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to personId
*/
@JsonIgnore
public void setPersonId(int newVal)
{
setPersonId(new Integer(newVal));
}
/**
* Determines if the personId has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkPersonIdModified()
{
return 0 != (modified & FL_ERROR_LOG_ID_PERSON_ID_MASK);
}
/**
* Determines if the personId has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkPersonIdInitialized()
{
return 0 != (initialized & FL_ERROR_LOG_ID_PERSON_ID_MASK);
}
/**
* Getter method for {@link #deviceId}.
* Meta Data Information (in progress):
*
* - full name: fl_error_log.device_id
* - foreign key: fl_device.id
* - comments: 外键,日志来源设备id
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of deviceId
*/
@ThriftField(value=7)
@JsonProperty("deviceId")
public Integer getDeviceId(){
return deviceId;
}
/**
* Setter method for {@link #deviceId}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to deviceId
*/
@ThriftField(name="deviceId")
@JsonProperty("deviceId")
public void setDeviceId(Integer newVal)
{
modified |= FL_ERROR_LOG_ID_DEVICE_ID_MASK;
initialized |= FL_ERROR_LOG_ID_DEVICE_ID_MASK;
if (Objects.equals(newVal, deviceId)) {
return;
}
deviceId = newVal;
}
/**
* Setter method for {@link #deviceId}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to deviceId
*/
@JsonIgnore
public void setDeviceId(int newVal)
{
setDeviceId(new Integer(newVal));
}
/**
* Determines if the deviceId has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkDeviceIdModified()
{
return 0 != (modified & FL_ERROR_LOG_ID_DEVICE_ID_MASK);
}
/**
* Determines if the deviceId has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkDeviceIdInitialized()
{
return 0 != (initialized & FL_ERROR_LOG_ID_DEVICE_ID_MASK);
}
/**
* Getter method for {@link #exceptionClass}.
* Meta Data Information (in progress):
*
* - full name: fl_error_log.exception_class
* - comments: 异常类名,such as java.io.IOException
* - column size: 256
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of exceptionClass
*/
@ThriftField(value=8)
@JsonProperty("exceptionClass")
public String getExceptionClass(){
return exceptionClass;
}
/**
* Setter method for {@link #exceptionClass}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to exceptionClass
*/
@ThriftField(name="exceptionClass")
@JsonProperty("exceptionClass")
public void setExceptionClass(String newVal)
{
modified |= FL_ERROR_LOG_ID_EXCEPTION_CLASS_MASK;
initialized |= FL_ERROR_LOG_ID_EXCEPTION_CLASS_MASK;
if (Objects.equals(newVal, exceptionClass)) {
return;
}
exceptionClass = newVal;
}
/**
* Determines if the exceptionClass has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkExceptionClassModified()
{
return 0 != (modified & FL_ERROR_LOG_ID_EXCEPTION_CLASS_MASK);
}
/**
* Determines if the exceptionClass has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkExceptionClassInitialized()
{
return 0 != (initialized & FL_ERROR_LOG_ID_EXCEPTION_CLASS_MASK);
}
/**
* Getter method for {@link #message}.
* Meta Data Information (in progress):
*
* - full name: fl_error_log.message
* - comments: 错误信息
* - column size: 1024
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of message
*/
@ThriftField(value=9)
@JsonProperty("message")
public String getMessage(){
return message;
}
/**
* Setter method for {@link #message}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to message
*/
@ThriftField(name="message")
@JsonProperty("message")
public void setMessage(String newVal)
{
modified |= FL_ERROR_LOG_ID_MESSAGE_MASK;
initialized |= FL_ERROR_LOG_ID_MESSAGE_MASK;
if (Objects.equals(newVal, message)) {
return;
}
message = newVal;
}
/**
* Determines if the message has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkMessageModified()
{
return 0 != (modified & FL_ERROR_LOG_ID_MESSAGE_MASK);
}
/**
* Determines if the message has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkMessageInitialized()
{
return 0 != (initialized & FL_ERROR_LOG_ID_MESSAGE_MASK);
}
/**
* Getter method for {@link #stackTrace}.
* Meta Data Information (in progress):
*
* - full name: fl_error_log.stack_trace
* - comments: 异常堆栈信息(最大64KB)
* - column size: 65535
* - JDBC type returned by the driver: Types.LONGVARCHAR
*
*
* @return the value of stackTrace
*/
@ThriftField(value=10)
@JsonProperty("stackTrace")
public String getStackTrace(){
return stackTrace;
}
/**
* Setter method for {@link #stackTrace}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to stackTrace
*/
@ThriftField(name="stackTrace")
@JsonProperty("stackTrace")
public void setStackTrace(String newVal)
{
modified |= FL_ERROR_LOG_ID_STACK_TRACE_MASK;
initialized |= FL_ERROR_LOG_ID_STACK_TRACE_MASK;
if (Objects.equals(newVal, stackTrace)) {
return;
}
stackTrace = newVal;
}
/**
* Determines if the stackTrace has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkStackTraceModified()
{
return 0 != (modified & FL_ERROR_LOG_ID_STACK_TRACE_MASK);
}
/**
* Determines if the stackTrace has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkStackTraceInitialized()
{
return 0 != (initialized & FL_ERROR_LOG_ID_STACK_TRACE_MASK);
}
/**
* Getter method for {@link #createTime}.
* Meta Data Information (in progress):
*
* - full name: fl_error_log.create_time
* - default value: 'CURRENT_TIMESTAMP'
* - NOT NULL
* - column size: 19
* - JDBC type returned by the driver: Types.TIMESTAMP
*
*
* @return the value of createTime
*/
@JsonIgnore
public java.util.Date getCreateTime(){
return createTime;
}
/**
* use Long to represent date type for thrift:swift support
* @see #getCreateTime()
*/
@ThriftField(value=11,name="createTime")
@JsonProperty("createTime")
public Long readCreateTime(){
return null == createTime ? null:createTime.getTime();
}
/**
* Setter method for {@link #createTime}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to createTime
*/
@JsonIgnore
public void setCreateTime(java.util.Date newVal)
{
modified |= FL_ERROR_LOG_ID_CREATE_TIME_MASK;
initialized |= FL_ERROR_LOG_ID_CREATE_TIME_MASK;
if (Objects.equals(newVal, createTime)) {
return;
}
createTime = newVal;
}
/**
* setter for thrift:swift OR jackson support
* without modification for {@link #modified} and {@link #initialized}
* NOTE:DO NOT use the method in your code
*/
@ThriftField(name="createTime")
@JsonProperty("createTime")
public void writeCreateTime(Long newVal){
setCreateTime(null == newVal?null:new java.util.Date(newVal));
}
/**
* Setter method for {@link #createTime}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to createTime
*/
@JsonIgnore
public void setCreateTime(long newVal)
{
setCreateTime(new java.util.Date(newVal));
}
/**
* Setter method for {@link #createTime}.
* @param newVal the number of milliseconds since January 1, 1970, 00:00:00 GMT represented by this Date object.
*/
@JsonIgnore
public void setCreateTime(Long newVal)
{
setCreateTime(null == newVal ? null : new java.util.Date(newVal));
}
/**
* Determines if the createTime has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkCreateTimeModified()
{
return 0 != (modified & FL_ERROR_LOG_ID_CREATE_TIME_MASK);
}
/**
* Determines if the createTime has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkCreateTimeInitialized()
{
return 0 != (initialized & FL_ERROR_LOG_ID_CREATE_TIME_MASK);
}
@Override
public boolean beModified()
{
return 0 != modified;
}
@Override
public boolean isModified(int columnID){
return columnID>=0 && columnID < metaData.columnCount && 0 != (modified & (1 << columnID));
}
@Override
public boolean isInitialized(int columnID){
return columnID>=0 && columnID < metaData.columnCount && 0 != (initialized & (1 << columnID));
}
@Override
public void resetIsModified()
{
modified = 0;
}
@Override
public void resetPrimaryKeysModified()
{
modified &= (~(FL_ERROR_LOG_ID_ID_MASK));
}
/**
* Resets columns modification status except primary keys to 'not modified'.
*/
public void resetModifiedExceptPrimaryKeys()
{
modified &= (~(FL_ERROR_LOG_ID_CATALOG_MASK |
FL_ERROR_LOG_ID_PERSON_ID_MASK |
FL_ERROR_LOG_ID_DEVICE_ID_MASK |
FL_ERROR_LOG_ID_EXCEPTION_CLASS_MASK |
FL_ERROR_LOG_ID_MESSAGE_MASK |
FL_ERROR_LOG_ID_STACK_TRACE_MASK |
FL_ERROR_LOG_ID_CREATE_TIME_MASK));
}
/** reset all fields to initial value, equal to a new bean */
public void reset(){
this.id = null;
this.catalog = null;
this.personId = null;
this.deviceId = null;
this.exceptionClass = null;
this.message = null;
this.stackTrace = null;
/* DEFAULT:'CURRENT_TIMESTAMP'*/
this.createTime = null;
this.isNew = true;
this.modified = 0;
this.initialized = 0;
}
@Override
public ErrorLogBean clone(){
return (ErrorLogBean) super.clone();
}
public static final Builder builder(){
return new Builder().reset();
}
/**
* a builder for ErrorLogBean,the template instance is thread local variable
* a instance of Builder can be reused.
*/
public static final class Builder{
/** ErrorLogBean instance used for template to create new ErrorLogBean instance. */
static final ThreadLocal TEMPLATE = new ThreadLocal(){
@Override
protected ErrorLogBean initialValue() {
return new ErrorLogBean();
}};
private Builder() {}
/**
* reset the bean as template
* @see ErrorLogBean#reset()
*/
public Builder reset(){
TEMPLATE.get().reset();
return this;
}
/** set a bean as template,must not be {@code null} */
public Builder template(ErrorLogBean bean){
if(null == bean){
throw new NullPointerException();
}
TEMPLATE.set(bean);
return this;
}
/** return a clone instance of {@link #TEMPLATE}*/
public ErrorLogBean build(){
return TEMPLATE.get().clone();
}
/**
* fill the field : fl_error_log.id
* @param id 日志id
* @see ErrorLogBean#getId()
* @see ErrorLogBean#setId(Integer)
*/
public Builder id(Integer id){
TEMPLATE.get().setId(id);
return this;
}
/**
* fill the field : fl_error_log.catalog
* @param catalog 错误类型分类,以/分隔的多级类型定义,应用程序可自定义,顶级分类:service(服务异常),device(设备异常),application(应用异常)
* @see ErrorLogBean#getCatalog()
* @see ErrorLogBean#setCatalog(String)
*/
public Builder catalog(String catalog){
TEMPLATE.get().setCatalog(catalog);
return this;
}
/**
* fill the field : fl_error_log.person_id
* @param personId 外键,日志来源用户id
* @see ErrorLogBean#getPersonId()
* @see ErrorLogBean#setPersonId(Integer)
*/
public Builder personId(Integer personId){
TEMPLATE.get().setPersonId(personId);
return this;
}
/**
* fill the field : fl_error_log.device_id
* @param deviceId 外键,日志来源设备id
* @see ErrorLogBean#getDeviceId()
* @see ErrorLogBean#setDeviceId(Integer)
*/
public Builder deviceId(Integer deviceId){
TEMPLATE.get().setDeviceId(deviceId);
return this;
}
/**
* fill the field : fl_error_log.exception_class
* @param exceptionClass 异常类名,such as java.io.IOException
* @see ErrorLogBean#getExceptionClass()
* @see ErrorLogBean#setExceptionClass(String)
*/
public Builder exceptionClass(String exceptionClass){
TEMPLATE.get().setExceptionClass(exceptionClass);
return this;
}
/**
* fill the field : fl_error_log.message
* @param message 错误信息
* @see ErrorLogBean#getMessage()
* @see ErrorLogBean#setMessage(String)
*/
public Builder message(String message){
TEMPLATE.get().setMessage(message);
return this;
}
/**
* fill the field : fl_error_log.stack_trace
* @param stackTrace 异常堆栈信息(最大64KB)
* @see ErrorLogBean#getStackTrace()
* @see ErrorLogBean#setStackTrace(String)
*/
public Builder stackTrace(String stackTrace){
TEMPLATE.get().setStackTrace(stackTrace);
return this;
}
/**
* fill the field : fl_error_log.create_time
* @param createTime
* @see ErrorLogBean#getCreateTime()
* @see ErrorLogBean#setCreateTime(java.util.Date)
*/
public Builder createTime(java.util.Date createTime){
TEMPLATE.get().setCreateTime(createTime);
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy