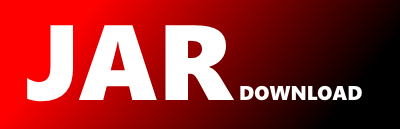
net.gdface.facelog.db.FaceBean Maven / Gradle / Ivy
// ______________________________________________________
// Generated by sql2java - https://github.com/10km/sql2java
// JDBC driver used at code generation time: com.mysql.jdbc.Driver
// template: bean.java.vm
// ______________________________________________________
package net.gdface.facelog.db;
import java.io.Serializable;
import java.util.Objects;
import gu.sql2java.BaseRow;
import com.facebook.swift.codec.ThriftStruct;
import com.facebook.swift.codec.ThriftField;
import com.facebook.swift.codec.ThriftField.Requiredness;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonIgnore;
import net.gdface.annotation.CodegenLength;
import net.gdface.annotation.CodegenRequired;
import net.gdface.annotation.CodegenInvalidValue;
/**
* FaceBean is a mapping of fl_face Table.
*
Meta Data Information (in progress):
*
* - comments: 人脸检测信息数据表,用于保存检测到的人脸的所有信息(特征数据除外)
*
* @author guyadong
*/
@ThriftStruct
@ApiModel(description="人脸检测信息数据表,用于保存检测到的人脸的所有信息(特征数据除外)")
public final class FaceBean extends BaseRow
implements Serializable,Constant
{
private static final long serialVersionUID = -1428389659131258505L;
/** comments:主键 */
@ApiModelProperty(value = "主键" ,required=true ,dataType="Integer")
@CodegenRequired@CodegenInvalidValue("0")
private Integer id;
/** comments:外键,所属图像id */
@ApiModelProperty(value = "外键,所属图像id" ,required=true ,dataType="String")
@CodegenLength(value=32,prealloc=true)@CodegenRequired@CodegenInvalidValue
private String imageMd5;
/** comments:人脸位置矩形:x */
@ApiModelProperty(value = "人脸位置矩形:x" ,required=true ,dataType="Integer")
@CodegenRequired@CodegenInvalidValue("-1")
private Integer faceLeft;
/** comments:人脸位置矩形:y */
@ApiModelProperty(value = "人脸位置矩形:y" ,required=true ,dataType="Integer")
@CodegenRequired@CodegenInvalidValue("-1")
private Integer faceTop;
/** comments:人脸位置矩形:width */
@ApiModelProperty(value = "人脸位置矩形:width" ,required=true ,dataType="Integer")
@CodegenRequired@CodegenInvalidValue("-1")
private Integer faceWidth;
/** comments:人脸位置矩形:height */
@ApiModelProperty(value = "人脸位置矩形:height" ,required=true ,dataType="Integer")
@CodegenRequired@CodegenInvalidValue("-1")
private Integer faceHeight;
/** comments:左眼位置:x */
@ApiModelProperty(value = "左眼位置:x" ,dataType="Integer")
@CodegenInvalidValue("-1")
private Integer eyeLeftx;
/** comments:左眼位置:y */
@ApiModelProperty(value = "左眼位置:y" ,dataType="Integer")
@CodegenInvalidValue("-1")
private Integer eyeLefty;
/** comments:右眼位置:x */
@ApiModelProperty(value = "右眼位置:x" ,dataType="Integer")
@CodegenInvalidValue("-1")
private Integer eyeRightx;
/** comments:右眼位置:y */
@ApiModelProperty(value = "右眼位置:y" ,dataType="Integer")
@CodegenInvalidValue("-1")
private Integer eyeRighty;
/** comments:嘴巴位置:x */
@ApiModelProperty(value = "嘴巴位置:x" ,dataType="Integer")
@CodegenInvalidValue("-1")
private Integer mouthX;
/** comments:嘴巴位置:y */
@ApiModelProperty(value = "嘴巴位置:y" ,dataType="Integer")
@CodegenInvalidValue("-1")
private Integer mouthY;
/** comments:鼻子位置:x */
@ApiModelProperty(value = "鼻子位置:x" ,dataType="Integer")
@CodegenInvalidValue("-1")
private Integer noseX;
/** comments:鼻子位置:y */
@ApiModelProperty(value = "鼻子位置:y" ,dataType="Integer")
@CodegenInvalidValue("-1")
private Integer noseY;
/** comments:人脸姿态:偏航角 */
@ApiModelProperty(value = "人脸姿态:偏航角" ,dataType="Integer")
@CodegenInvalidValue("-1")
private Integer angleYaw;
/** comments:人脸姿态:俯仰角 */
@ApiModelProperty(value = "人脸姿态:俯仰角" ,dataType="Integer")
@CodegenInvalidValue("-1")
private Integer anglePitch;
/** comments:人脸姿态:滚转角 */
@ApiModelProperty(value = "人脸姿态:滚转角" ,dataType="Integer")
@CodegenInvalidValue("-1")
private Integer angleRoll;
/** comments:扩展字段,保存人脸检测基本信息之外的其他数据,内容由SDK负责解析 */
@ApiModelProperty(value = "扩展字段,保存人脸检测基本信息之外的其他数据,内容由SDK负责解析" ,dataType="ByteBuffer")
@CodegenLength(max=65535)@CodegenInvalidValue
private java.nio.ByteBuffer extInfo;
/** comments:外键,人脸特征数据MD5 id */
@ApiModelProperty(value = "外键,人脸特征数据MD5 id" ,dataType="String")
@CodegenLength(value=32,prealloc=true)@CodegenInvalidValue
private String featureMd5;
/** columns modified flag */
@ApiModelProperty(value="columns modified flag",dataType="int",required=true)
private int modified;
/** columns initialized flag */
@ApiModelProperty(value="columns initialized flag",dataType="int",required=true)
private int initialized;
/** new record flag */
@ApiModelProperty(value="new record flag",dataType="boolean",required=true)
private boolean isNew;
@ThriftField(value=1,name="_new",requiredness=Requiredness.REQUIRED)
@Override
public boolean isNew()
{
return this.isNew;
}
/**
* Specifies to the object if it has been set as new.
*
* @param isNew the boolean value to be assigned to the isNew field
*/
@ThriftField()
@Override
public void setNew(boolean isNew)
{
this.isNew = isNew;
}
/**
* @return the modified status of columns
*/
@ThriftField(value=2,requiredness=Requiredness.REQUIRED)
@Override
public int getModified(){
return modified;
}
/**
* @param modified the modified status bit to be assigned to {@link #modified}
*/
@ThriftField()
@Override
public void setModified(int modified){
this.modified = modified;
}
/**
* @return the initialized status of columns
*/
@ThriftField(value=3,requiredness=Requiredness.REQUIRED)
@Override
public int getInitialized(){
return initialized;
}
/**
* @param initialized the initialized status bit to be assigned to {@link #initialized}
*/
@ThriftField()
@Override
public void setInitialized(int initialized){
this.initialized = initialized;
}
public FaceBean(){
reset();
}
/**
* construct a new instance filled with primary keys
* @param id PK# 1
*/
public FaceBean(Integer id){
setId(id);
}
/**
* Getter method for {@link #id}.
* PRIMARY KEY.
* Meta Data Information (in progress):
*
* - full name: fl_face.id
* - imported key: fl_log.compare_face
* - comments: 主键
* - AUTO_INCREMENT
* - NOT NULL
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of id
*/
@ThriftField(value=4)
@JsonProperty("id")
public Integer getId(){
return id;
}
/**
* Setter method for {@link #id}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to id
*/
@ThriftField(name="id")
@JsonProperty("id")
public void setId(Integer newVal)
{
modified |= FL_FACE_ID_ID_MASK;
initialized |= FL_FACE_ID_ID_MASK;
if (Objects.equals(newVal, id)) {
return;
}
id = newVal;
}
/**
* Setter method for {@link #id}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to id
*/
@JsonIgnore
public void setId(int newVal)
{
setId(new Integer(newVal));
}
/**
* Determines if the id has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkIdModified()
{
return 0 != (modified & FL_FACE_ID_ID_MASK);
}
/**
* Determines if the id has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkIdInitialized()
{
return 0 != (initialized & FL_FACE_ID_ID_MASK);
}
/**
* Getter method for {@link #imageMd5}.
* Meta Data Information (in progress):
*
* - full name: fl_face.image_md5
* - foreign key: fl_image.md5
* - comments: 外键,所属图像id
* - NOT NULL
* - column size: 32
* - JDBC type returned by the driver: Types.CHAR
*
*
* @return the value of imageMd5
*/
@ThriftField(value=5)
@JsonProperty("imageMd5")
public String getImageMd5(){
return imageMd5;
}
/**
* Setter method for {@link #imageMd5}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to imageMd5
*/
@ThriftField(name="imageMd5")
@JsonProperty("imageMd5")
public void setImageMd5(String newVal)
{
modified |= FL_FACE_ID_IMAGE_MD5_MASK;
initialized |= FL_FACE_ID_IMAGE_MD5_MASK;
if (Objects.equals(newVal, imageMd5)) {
return;
}
imageMd5 = newVal;
}
/**
* Determines if the imageMd5 has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkImageMd5Modified()
{
return 0 != (modified & FL_FACE_ID_IMAGE_MD5_MASK);
}
/**
* Determines if the imageMd5 has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkImageMd5Initialized()
{
return 0 != (initialized & FL_FACE_ID_IMAGE_MD5_MASK);
}
/**
* Getter method for {@link #faceLeft}.
* Meta Data Information (in progress):
*
* - full name: fl_face.face_left
* - comments: 人脸位置矩形:x
* - NOT NULL
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of faceLeft
*/
@ThriftField(value=6)
@JsonProperty("faceLeft")
public Integer getFaceLeft(){
return faceLeft;
}
/**
* Setter method for {@link #faceLeft}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to faceLeft
*/
@ThriftField(name="faceLeft")
@JsonProperty("faceLeft")
public void setFaceLeft(Integer newVal)
{
modified |= FL_FACE_ID_FACE_LEFT_MASK;
initialized |= FL_FACE_ID_FACE_LEFT_MASK;
if (Objects.equals(newVal, faceLeft)) {
return;
}
faceLeft = newVal;
}
/**
* Setter method for {@link #faceLeft}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to faceLeft
*/
@JsonIgnore
public void setFaceLeft(int newVal)
{
setFaceLeft(new Integer(newVal));
}
/**
* Determines if the faceLeft has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkFaceLeftModified()
{
return 0 != (modified & FL_FACE_ID_FACE_LEFT_MASK);
}
/**
* Determines if the faceLeft has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkFaceLeftInitialized()
{
return 0 != (initialized & FL_FACE_ID_FACE_LEFT_MASK);
}
/**
* Getter method for {@link #faceTop}.
* Meta Data Information (in progress):
*
* - full name: fl_face.face_top
* - comments: 人脸位置矩形:y
* - NOT NULL
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of faceTop
*/
@ThriftField(value=7)
@JsonProperty("faceTop")
public Integer getFaceTop(){
return faceTop;
}
/**
* Setter method for {@link #faceTop}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to faceTop
*/
@ThriftField(name="faceTop")
@JsonProperty("faceTop")
public void setFaceTop(Integer newVal)
{
modified |= FL_FACE_ID_FACE_TOP_MASK;
initialized |= FL_FACE_ID_FACE_TOP_MASK;
if (Objects.equals(newVal, faceTop)) {
return;
}
faceTop = newVal;
}
/**
* Setter method for {@link #faceTop}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to faceTop
*/
@JsonIgnore
public void setFaceTop(int newVal)
{
setFaceTop(new Integer(newVal));
}
/**
* Determines if the faceTop has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkFaceTopModified()
{
return 0 != (modified & FL_FACE_ID_FACE_TOP_MASK);
}
/**
* Determines if the faceTop has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkFaceTopInitialized()
{
return 0 != (initialized & FL_FACE_ID_FACE_TOP_MASK);
}
/**
* Getter method for {@link #faceWidth}.
* Meta Data Information (in progress):
*
* - full name: fl_face.face_width
* - comments: 人脸位置矩形:width
* - NOT NULL
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of faceWidth
*/
@ThriftField(value=8)
@JsonProperty("faceWidth")
public Integer getFaceWidth(){
return faceWidth;
}
/**
* Setter method for {@link #faceWidth}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to faceWidth
*/
@ThriftField(name="faceWidth")
@JsonProperty("faceWidth")
public void setFaceWidth(Integer newVal)
{
modified |= FL_FACE_ID_FACE_WIDTH_MASK;
initialized |= FL_FACE_ID_FACE_WIDTH_MASK;
if (Objects.equals(newVal, faceWidth)) {
return;
}
faceWidth = newVal;
}
/**
* Setter method for {@link #faceWidth}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to faceWidth
*/
@JsonIgnore
public void setFaceWidth(int newVal)
{
setFaceWidth(new Integer(newVal));
}
/**
* Determines if the faceWidth has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkFaceWidthModified()
{
return 0 != (modified & FL_FACE_ID_FACE_WIDTH_MASK);
}
/**
* Determines if the faceWidth has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkFaceWidthInitialized()
{
return 0 != (initialized & FL_FACE_ID_FACE_WIDTH_MASK);
}
/**
* Getter method for {@link #faceHeight}.
* Meta Data Information (in progress):
*
* - full name: fl_face.face_height
* - comments: 人脸位置矩形:height
* - NOT NULL
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of faceHeight
*/
@ThriftField(value=9)
@JsonProperty("faceHeight")
public Integer getFaceHeight(){
return faceHeight;
}
/**
* Setter method for {@link #faceHeight}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to faceHeight
*/
@ThriftField(name="faceHeight")
@JsonProperty("faceHeight")
public void setFaceHeight(Integer newVal)
{
modified |= FL_FACE_ID_FACE_HEIGHT_MASK;
initialized |= FL_FACE_ID_FACE_HEIGHT_MASK;
if (Objects.equals(newVal, faceHeight)) {
return;
}
faceHeight = newVal;
}
/**
* Setter method for {@link #faceHeight}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to faceHeight
*/
@JsonIgnore
public void setFaceHeight(int newVal)
{
setFaceHeight(new Integer(newVal));
}
/**
* Determines if the faceHeight has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkFaceHeightModified()
{
return 0 != (modified & FL_FACE_ID_FACE_HEIGHT_MASK);
}
/**
* Determines if the faceHeight has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkFaceHeightInitialized()
{
return 0 != (initialized & FL_FACE_ID_FACE_HEIGHT_MASK);
}
/**
* Getter method for {@link #eyeLeftx}.
* Meta Data Information (in progress):
*
* - full name: fl_face.eye_leftx
* - comments: 左眼位置:x
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of eyeLeftx
*/
@ThriftField(value=10)
@JsonProperty("eyeLeftx")
public Integer getEyeLeftx(){
return eyeLeftx;
}
/**
* Setter method for {@link #eyeLeftx}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to eyeLeftx
*/
@ThriftField(name="eyeLeftx")
@JsonProperty("eyeLeftx")
public void setEyeLeftx(Integer newVal)
{
modified |= FL_FACE_ID_EYE_LEFTX_MASK;
initialized |= FL_FACE_ID_EYE_LEFTX_MASK;
if (Objects.equals(newVal, eyeLeftx)) {
return;
}
eyeLeftx = newVal;
}
/**
* Setter method for {@link #eyeLeftx}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to eyeLeftx
*/
@JsonIgnore
public void setEyeLeftx(int newVal)
{
setEyeLeftx(new Integer(newVal));
}
/**
* Determines if the eyeLeftx has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkEyeLeftxModified()
{
return 0 != (modified & FL_FACE_ID_EYE_LEFTX_MASK);
}
/**
* Determines if the eyeLeftx has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkEyeLeftxInitialized()
{
return 0 != (initialized & FL_FACE_ID_EYE_LEFTX_MASK);
}
/**
* Getter method for {@link #eyeLefty}.
* Meta Data Information (in progress):
*
* - full name: fl_face.eye_lefty
* - comments: 左眼位置:y
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of eyeLefty
*/
@ThriftField(value=11)
@JsonProperty("eyeLefty")
public Integer getEyeLefty(){
return eyeLefty;
}
/**
* Setter method for {@link #eyeLefty}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to eyeLefty
*/
@ThriftField(name="eyeLefty")
@JsonProperty("eyeLefty")
public void setEyeLefty(Integer newVal)
{
modified |= FL_FACE_ID_EYE_LEFTY_MASK;
initialized |= FL_FACE_ID_EYE_LEFTY_MASK;
if (Objects.equals(newVal, eyeLefty)) {
return;
}
eyeLefty = newVal;
}
/**
* Setter method for {@link #eyeLefty}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to eyeLefty
*/
@JsonIgnore
public void setEyeLefty(int newVal)
{
setEyeLefty(new Integer(newVal));
}
/**
* Determines if the eyeLefty has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkEyeLeftyModified()
{
return 0 != (modified & FL_FACE_ID_EYE_LEFTY_MASK);
}
/**
* Determines if the eyeLefty has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkEyeLeftyInitialized()
{
return 0 != (initialized & FL_FACE_ID_EYE_LEFTY_MASK);
}
/**
* Getter method for {@link #eyeRightx}.
* Meta Data Information (in progress):
*
* - full name: fl_face.eye_rightx
* - comments: 右眼位置:x
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of eyeRightx
*/
@ThriftField(value=12)
@JsonProperty("eyeRightx")
public Integer getEyeRightx(){
return eyeRightx;
}
/**
* Setter method for {@link #eyeRightx}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to eyeRightx
*/
@ThriftField(name="eyeRightx")
@JsonProperty("eyeRightx")
public void setEyeRightx(Integer newVal)
{
modified |= FL_FACE_ID_EYE_RIGHTX_MASK;
initialized |= FL_FACE_ID_EYE_RIGHTX_MASK;
if (Objects.equals(newVal, eyeRightx)) {
return;
}
eyeRightx = newVal;
}
/**
* Setter method for {@link #eyeRightx}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to eyeRightx
*/
@JsonIgnore
public void setEyeRightx(int newVal)
{
setEyeRightx(new Integer(newVal));
}
/**
* Determines if the eyeRightx has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkEyeRightxModified()
{
return 0 != (modified & FL_FACE_ID_EYE_RIGHTX_MASK);
}
/**
* Determines if the eyeRightx has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkEyeRightxInitialized()
{
return 0 != (initialized & FL_FACE_ID_EYE_RIGHTX_MASK);
}
/**
* Getter method for {@link #eyeRighty}.
* Meta Data Information (in progress):
*
* - full name: fl_face.eye_righty
* - comments: 右眼位置:y
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of eyeRighty
*/
@ThriftField(value=13)
@JsonProperty("eyeRighty")
public Integer getEyeRighty(){
return eyeRighty;
}
/**
* Setter method for {@link #eyeRighty}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to eyeRighty
*/
@ThriftField(name="eyeRighty")
@JsonProperty("eyeRighty")
public void setEyeRighty(Integer newVal)
{
modified |= FL_FACE_ID_EYE_RIGHTY_MASK;
initialized |= FL_FACE_ID_EYE_RIGHTY_MASK;
if (Objects.equals(newVal, eyeRighty)) {
return;
}
eyeRighty = newVal;
}
/**
* Setter method for {@link #eyeRighty}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to eyeRighty
*/
@JsonIgnore
public void setEyeRighty(int newVal)
{
setEyeRighty(new Integer(newVal));
}
/**
* Determines if the eyeRighty has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkEyeRightyModified()
{
return 0 != (modified & FL_FACE_ID_EYE_RIGHTY_MASK);
}
/**
* Determines if the eyeRighty has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkEyeRightyInitialized()
{
return 0 != (initialized & FL_FACE_ID_EYE_RIGHTY_MASK);
}
/**
* Getter method for {@link #mouthX}.
* Meta Data Information (in progress):
*
* - full name: fl_face.mouth_x
* - comments: 嘴巴位置:x
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of mouthX
*/
@ThriftField(value=14)
@JsonProperty("mouthX")
public Integer getMouthX(){
return mouthX;
}
/**
* Setter method for {@link #mouthX}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to mouthX
*/
@ThriftField(name="mouthX")
@JsonProperty("mouthX")
public void setMouthX(Integer newVal)
{
modified |= FL_FACE_ID_MOUTH_X_MASK;
initialized |= FL_FACE_ID_MOUTH_X_MASK;
if (Objects.equals(newVal, mouthX)) {
return;
}
mouthX = newVal;
}
/**
* Setter method for {@link #mouthX}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to mouthX
*/
@JsonIgnore
public void setMouthX(int newVal)
{
setMouthX(new Integer(newVal));
}
/**
* Determines if the mouthX has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkMouthXModified()
{
return 0 != (modified & FL_FACE_ID_MOUTH_X_MASK);
}
/**
* Determines if the mouthX has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkMouthXInitialized()
{
return 0 != (initialized & FL_FACE_ID_MOUTH_X_MASK);
}
/**
* Getter method for {@link #mouthY}.
* Meta Data Information (in progress):
*
* - full name: fl_face.mouth_y
* - comments: 嘴巴位置:y
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of mouthY
*/
@ThriftField(value=15)
@JsonProperty("mouthY")
public Integer getMouthY(){
return mouthY;
}
/**
* Setter method for {@link #mouthY}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to mouthY
*/
@ThriftField(name="mouthY")
@JsonProperty("mouthY")
public void setMouthY(Integer newVal)
{
modified |= FL_FACE_ID_MOUTH_Y_MASK;
initialized |= FL_FACE_ID_MOUTH_Y_MASK;
if (Objects.equals(newVal, mouthY)) {
return;
}
mouthY = newVal;
}
/**
* Setter method for {@link #mouthY}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to mouthY
*/
@JsonIgnore
public void setMouthY(int newVal)
{
setMouthY(new Integer(newVal));
}
/**
* Determines if the mouthY has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkMouthYModified()
{
return 0 != (modified & FL_FACE_ID_MOUTH_Y_MASK);
}
/**
* Determines if the mouthY has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkMouthYInitialized()
{
return 0 != (initialized & FL_FACE_ID_MOUTH_Y_MASK);
}
/**
* Getter method for {@link #noseX}.
* Meta Data Information (in progress):
*
* - full name: fl_face.nose_x
* - comments: 鼻子位置:x
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of noseX
*/
@ThriftField(value=16)
@JsonProperty("noseX")
public Integer getNoseX(){
return noseX;
}
/**
* Setter method for {@link #noseX}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to noseX
*/
@ThriftField(name="noseX")
@JsonProperty("noseX")
public void setNoseX(Integer newVal)
{
modified |= FL_FACE_ID_NOSE_X_MASK;
initialized |= FL_FACE_ID_NOSE_X_MASK;
if (Objects.equals(newVal, noseX)) {
return;
}
noseX = newVal;
}
/**
* Setter method for {@link #noseX}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to noseX
*/
@JsonIgnore
public void setNoseX(int newVal)
{
setNoseX(new Integer(newVal));
}
/**
* Determines if the noseX has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkNoseXModified()
{
return 0 != (modified & FL_FACE_ID_NOSE_X_MASK);
}
/**
* Determines if the noseX has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkNoseXInitialized()
{
return 0 != (initialized & FL_FACE_ID_NOSE_X_MASK);
}
/**
* Getter method for {@link #noseY}.
* Meta Data Information (in progress):
*
* - full name: fl_face.nose_y
* - comments: 鼻子位置:y
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of noseY
*/
@ThriftField(value=17)
@JsonProperty("noseY")
public Integer getNoseY(){
return noseY;
}
/**
* Setter method for {@link #noseY}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to noseY
*/
@ThriftField(name="noseY")
@JsonProperty("noseY")
public void setNoseY(Integer newVal)
{
modified |= FL_FACE_ID_NOSE_Y_MASK;
initialized |= FL_FACE_ID_NOSE_Y_MASK;
if (Objects.equals(newVal, noseY)) {
return;
}
noseY = newVal;
}
/**
* Setter method for {@link #noseY}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to noseY
*/
@JsonIgnore
public void setNoseY(int newVal)
{
setNoseY(new Integer(newVal));
}
/**
* Determines if the noseY has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkNoseYModified()
{
return 0 != (modified & FL_FACE_ID_NOSE_Y_MASK);
}
/**
* Determines if the noseY has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkNoseYInitialized()
{
return 0 != (initialized & FL_FACE_ID_NOSE_Y_MASK);
}
/**
* Getter method for {@link #angleYaw}.
* Meta Data Information (in progress):
*
* - full name: fl_face.angle_yaw
* - comments: 人脸姿态:偏航角
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of angleYaw
*/
@ThriftField(value=18)
@JsonProperty("angleYaw")
public Integer getAngleYaw(){
return angleYaw;
}
/**
* Setter method for {@link #angleYaw}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to angleYaw
*/
@ThriftField(name="angleYaw")
@JsonProperty("angleYaw")
public void setAngleYaw(Integer newVal)
{
modified |= FL_FACE_ID_ANGLE_YAW_MASK;
initialized |= FL_FACE_ID_ANGLE_YAW_MASK;
if (Objects.equals(newVal, angleYaw)) {
return;
}
angleYaw = newVal;
}
/**
* Setter method for {@link #angleYaw}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to angleYaw
*/
@JsonIgnore
public void setAngleYaw(int newVal)
{
setAngleYaw(new Integer(newVal));
}
/**
* Determines if the angleYaw has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkAngleYawModified()
{
return 0 != (modified & FL_FACE_ID_ANGLE_YAW_MASK);
}
/**
* Determines if the angleYaw has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkAngleYawInitialized()
{
return 0 != (initialized & FL_FACE_ID_ANGLE_YAW_MASK);
}
/**
* Getter method for {@link #anglePitch}.
* Meta Data Information (in progress):
*
* - full name: fl_face.angle_pitch
* - comments: 人脸姿态:俯仰角
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of anglePitch
*/
@ThriftField(value=19)
@JsonProperty("anglePitch")
public Integer getAnglePitch(){
return anglePitch;
}
/**
* Setter method for {@link #anglePitch}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to anglePitch
*/
@ThriftField(name="anglePitch")
@JsonProperty("anglePitch")
public void setAnglePitch(Integer newVal)
{
modified |= FL_FACE_ID_ANGLE_PITCH_MASK;
initialized |= FL_FACE_ID_ANGLE_PITCH_MASK;
if (Objects.equals(newVal, anglePitch)) {
return;
}
anglePitch = newVal;
}
/**
* Setter method for {@link #anglePitch}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to anglePitch
*/
@JsonIgnore
public void setAnglePitch(int newVal)
{
setAnglePitch(new Integer(newVal));
}
/**
* Determines if the anglePitch has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkAnglePitchModified()
{
return 0 != (modified & FL_FACE_ID_ANGLE_PITCH_MASK);
}
/**
* Determines if the anglePitch has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkAnglePitchInitialized()
{
return 0 != (initialized & FL_FACE_ID_ANGLE_PITCH_MASK);
}
/**
* Getter method for {@link #angleRoll}.
* Meta Data Information (in progress):
*
* - full name: fl_face.angle_roll
* - comments: 人脸姿态:滚转角
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of angleRoll
*/
@ThriftField(value=20)
@JsonProperty("angleRoll")
public Integer getAngleRoll(){
return angleRoll;
}
/**
* Setter method for {@link #angleRoll}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to angleRoll
*/
@ThriftField(name="angleRoll")
@JsonProperty("angleRoll")
public void setAngleRoll(Integer newVal)
{
modified |= FL_FACE_ID_ANGLE_ROLL_MASK;
initialized |= FL_FACE_ID_ANGLE_ROLL_MASK;
if (Objects.equals(newVal, angleRoll)) {
return;
}
angleRoll = newVal;
}
/**
* Setter method for {@link #angleRoll}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to angleRoll
*/
@JsonIgnore
public void setAngleRoll(int newVal)
{
setAngleRoll(new Integer(newVal));
}
/**
* Determines if the angleRoll has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkAngleRollModified()
{
return 0 != (modified & FL_FACE_ID_ANGLE_ROLL_MASK);
}
/**
* Determines if the angleRoll has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkAngleRollInitialized()
{
return 0 != (initialized & FL_FACE_ID_ANGLE_ROLL_MASK);
}
/**
* Getter method for {@link #extInfo}.
* Meta Data Information (in progress):
*
* - full name: fl_face.ext_info
* - comments: 扩展字段,保存人脸检测基本信息之外的其他数据,内容由SDK负责解析
* - column size: 65535
* - JDBC type returned by the driver: Types.LONGVARBINARY
*
*
* @return the value of extInfo
*/
@ThriftField(value=21)
@JsonProperty("extInfo")
public java.nio.ByteBuffer getExtInfo(){
return extInfo;
}
/**
* Setter method for {@link #extInfo}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to extInfo
*/
@ThriftField(name="extInfo")
@JsonProperty("extInfo")
public void setExtInfo(java.nio.ByteBuffer newVal)
{
modified |= FL_FACE_ID_EXT_INFO_MASK;
initialized |= FL_FACE_ID_EXT_INFO_MASK;
if (Objects.equals(newVal, extInfo)) {
return;
}
extInfo = newVal;
}
/**
* Determines if the extInfo has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkExtInfoModified()
{
return 0 != (modified & FL_FACE_ID_EXT_INFO_MASK);
}
/**
* Determines if the extInfo has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkExtInfoInitialized()
{
return 0 != (initialized & FL_FACE_ID_EXT_INFO_MASK);
}
/**
* Getter method for {@link #featureMd5}.
* Meta Data Information (in progress):
*
* - full name: fl_face.feature_md5
* - foreign key: fl_feature.md5
* - comments: 外键,人脸特征数据MD5 id
* - column size: 32
* - JDBC type returned by the driver: Types.CHAR
*
*
* @return the value of featureMd5
*/
@ThriftField(value=22)
@JsonProperty("featureMd5")
public String getFeatureMd5(){
return featureMd5;
}
/**
* Setter method for {@link #featureMd5}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to featureMd5
*/
@ThriftField(name="featureMd5")
@JsonProperty("featureMd5")
public void setFeatureMd5(String newVal)
{
modified |= FL_FACE_ID_FEATURE_MD5_MASK;
initialized |= FL_FACE_ID_FEATURE_MD5_MASK;
if (Objects.equals(newVal, featureMd5)) {
return;
}
featureMd5 = newVal;
}
/**
* Determines if the featureMd5 has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkFeatureMd5Modified()
{
return 0 != (modified & FL_FACE_ID_FEATURE_MD5_MASK);
}
/**
* Determines if the featureMd5 has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkFeatureMd5Initialized()
{
return 0 != (initialized & FL_FACE_ID_FEATURE_MD5_MASK);
}
@Override
public boolean beModified()
{
return 0 != modified;
}
@Override
public boolean isModified(int columnID){
return columnID>=0 && columnID < metaData.columnCount && 0 != (modified & (1 << columnID));
}
@Override
public boolean isInitialized(int columnID){
return columnID>=0 && columnID < metaData.columnCount && 0 != (initialized & (1 << columnID));
}
@Override
public void resetIsModified()
{
modified = 0;
}
@Override
public void resetPrimaryKeysModified()
{
modified &= (~(FL_FACE_ID_ID_MASK));
}
/**
* Resets columns modification status except primary keys to 'not modified'.
*/
public void resetModifiedExceptPrimaryKeys()
{
modified &= (~(FL_FACE_ID_IMAGE_MD5_MASK |
FL_FACE_ID_FACE_LEFT_MASK |
FL_FACE_ID_FACE_TOP_MASK |
FL_FACE_ID_FACE_WIDTH_MASK |
FL_FACE_ID_FACE_HEIGHT_MASK |
FL_FACE_ID_EYE_LEFTX_MASK |
FL_FACE_ID_EYE_LEFTY_MASK |
FL_FACE_ID_EYE_RIGHTX_MASK |
FL_FACE_ID_EYE_RIGHTY_MASK |
FL_FACE_ID_MOUTH_X_MASK |
FL_FACE_ID_MOUTH_Y_MASK |
FL_FACE_ID_NOSE_X_MASK |
FL_FACE_ID_NOSE_Y_MASK |
FL_FACE_ID_ANGLE_YAW_MASK |
FL_FACE_ID_ANGLE_PITCH_MASK |
FL_FACE_ID_ANGLE_ROLL_MASK |
FL_FACE_ID_EXT_INFO_MASK |
FL_FACE_ID_FEATURE_MD5_MASK));
}
/** reset all fields to initial value, equal to a new bean */
public void reset(){
this.id = null;
this.imageMd5 = null;
this.faceLeft = null;
this.faceTop = null;
this.faceWidth = null;
this.faceHeight = null;
this.eyeLeftx = null;
this.eyeLefty = null;
this.eyeRightx = null;
this.eyeRighty = null;
this.mouthX = null;
this.mouthY = null;
this.noseX = null;
this.noseY = null;
this.angleYaw = null;
this.anglePitch = null;
this.angleRoll = null;
this.extInfo = null;
this.featureMd5 = null;
this.isNew = true;
this.modified = 0;
this.initialized = 0;
}
@Override
public FaceBean clone(){
return (FaceBean) super.clone();
}
public static final Builder builder(){
return new Builder().reset();
}
/**
* a builder for FaceBean,the template instance is thread local variable
* a instance of Builder can be reused.
*/
public static final class Builder{
/** FaceBean instance used for template to create new FaceBean instance. */
static final ThreadLocal TEMPLATE = new ThreadLocal(){
@Override
protected FaceBean initialValue() {
return new FaceBean();
}};
private Builder() {}
/**
* reset the bean as template
* @see FaceBean#reset()
*/
public Builder reset(){
TEMPLATE.get().reset();
return this;
}
/** set a bean as template,must not be {@code null} */
public Builder template(FaceBean bean){
if(null == bean){
throw new NullPointerException();
}
TEMPLATE.set(bean);
return this;
}
/** return a clone instance of {@link #TEMPLATE}*/
public FaceBean build(){
return TEMPLATE.get().clone();
}
/**
* fill the field : fl_face.id
* @param id 主键
* @see FaceBean#getId()
* @see FaceBean#setId(Integer)
*/
public Builder id(Integer id){
TEMPLATE.get().setId(id);
return this;
}
/**
* fill the field : fl_face.image_md5
* @param imageMd5 外键,所属图像id
* @see FaceBean#getImageMd5()
* @see FaceBean#setImageMd5(String)
*/
public Builder imageMd5(String imageMd5){
TEMPLATE.get().setImageMd5(imageMd5);
return this;
}
/**
* fill the field : fl_face.face_left
* @param faceLeft 人脸位置矩形:x
* @see FaceBean#getFaceLeft()
* @see FaceBean#setFaceLeft(Integer)
*/
public Builder faceLeft(Integer faceLeft){
TEMPLATE.get().setFaceLeft(faceLeft);
return this;
}
/**
* fill the field : fl_face.face_top
* @param faceTop 人脸位置矩形:y
* @see FaceBean#getFaceTop()
* @see FaceBean#setFaceTop(Integer)
*/
public Builder faceTop(Integer faceTop){
TEMPLATE.get().setFaceTop(faceTop);
return this;
}
/**
* fill the field : fl_face.face_width
* @param faceWidth 人脸位置矩形:width
* @see FaceBean#getFaceWidth()
* @see FaceBean#setFaceWidth(Integer)
*/
public Builder faceWidth(Integer faceWidth){
TEMPLATE.get().setFaceWidth(faceWidth);
return this;
}
/**
* fill the field : fl_face.face_height
* @param faceHeight 人脸位置矩形:height
* @see FaceBean#getFaceHeight()
* @see FaceBean#setFaceHeight(Integer)
*/
public Builder faceHeight(Integer faceHeight){
TEMPLATE.get().setFaceHeight(faceHeight);
return this;
}
/**
* fill the field : fl_face.eye_leftx
* @param eyeLeftx 左眼位置:x
* @see FaceBean#getEyeLeftx()
* @see FaceBean#setEyeLeftx(Integer)
*/
public Builder eyeLeftx(Integer eyeLeftx){
TEMPLATE.get().setEyeLeftx(eyeLeftx);
return this;
}
/**
* fill the field : fl_face.eye_lefty
* @param eyeLefty 左眼位置:y
* @see FaceBean#getEyeLefty()
* @see FaceBean#setEyeLefty(Integer)
*/
public Builder eyeLefty(Integer eyeLefty){
TEMPLATE.get().setEyeLefty(eyeLefty);
return this;
}
/**
* fill the field : fl_face.eye_rightx
* @param eyeRightx 右眼位置:x
* @see FaceBean#getEyeRightx()
* @see FaceBean#setEyeRightx(Integer)
*/
public Builder eyeRightx(Integer eyeRightx){
TEMPLATE.get().setEyeRightx(eyeRightx);
return this;
}
/**
* fill the field : fl_face.eye_righty
* @param eyeRighty 右眼位置:y
* @see FaceBean#getEyeRighty()
* @see FaceBean#setEyeRighty(Integer)
*/
public Builder eyeRighty(Integer eyeRighty){
TEMPLATE.get().setEyeRighty(eyeRighty);
return this;
}
/**
* fill the field : fl_face.mouth_x
* @param mouthX 嘴巴位置:x
* @see FaceBean#getMouthX()
* @see FaceBean#setMouthX(Integer)
*/
public Builder mouthX(Integer mouthX){
TEMPLATE.get().setMouthX(mouthX);
return this;
}
/**
* fill the field : fl_face.mouth_y
* @param mouthY 嘴巴位置:y
* @see FaceBean#getMouthY()
* @see FaceBean#setMouthY(Integer)
*/
public Builder mouthY(Integer mouthY){
TEMPLATE.get().setMouthY(mouthY);
return this;
}
/**
* fill the field : fl_face.nose_x
* @param noseX 鼻子位置:x
* @see FaceBean#getNoseX()
* @see FaceBean#setNoseX(Integer)
*/
public Builder noseX(Integer noseX){
TEMPLATE.get().setNoseX(noseX);
return this;
}
/**
* fill the field : fl_face.nose_y
* @param noseY 鼻子位置:y
* @see FaceBean#getNoseY()
* @see FaceBean#setNoseY(Integer)
*/
public Builder noseY(Integer noseY){
TEMPLATE.get().setNoseY(noseY);
return this;
}
/**
* fill the field : fl_face.angle_yaw
* @param angleYaw 人脸姿态:偏航角
* @see FaceBean#getAngleYaw()
* @see FaceBean#setAngleYaw(Integer)
*/
public Builder angleYaw(Integer angleYaw){
TEMPLATE.get().setAngleYaw(angleYaw);
return this;
}
/**
* fill the field : fl_face.angle_pitch
* @param anglePitch 人脸姿态:俯仰角
* @see FaceBean#getAnglePitch()
* @see FaceBean#setAnglePitch(Integer)
*/
public Builder anglePitch(Integer anglePitch){
TEMPLATE.get().setAnglePitch(anglePitch);
return this;
}
/**
* fill the field : fl_face.angle_roll
* @param angleRoll 人脸姿态:滚转角
* @see FaceBean#getAngleRoll()
* @see FaceBean#setAngleRoll(Integer)
*/
public Builder angleRoll(Integer angleRoll){
TEMPLATE.get().setAngleRoll(angleRoll);
return this;
}
/**
* fill the field : fl_face.ext_info
* @param extInfo 扩展字段,保存人脸检测基本信息之外的其他数据,内容由SDK负责解析
* @see FaceBean#getExtInfo()
* @see FaceBean#setExtInfo(java.nio.ByteBuffer)
*/
public Builder extInfo(java.nio.ByteBuffer extInfo){
TEMPLATE.get().setExtInfo(extInfo);
return this;
}
/**
* fill the field : fl_face.feature_md5
* @param featureMd5 外键,人脸特征数据MD5 id
* @see FaceBean#getFeatureMd5()
* @see FaceBean#setFeatureMd5(String)
*/
public Builder featureMd5(String featureMd5){
TEMPLATE.get().setFeatureMd5(featureMd5);
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy