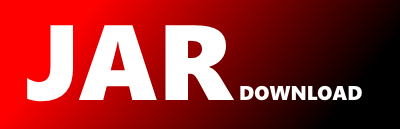
net.gdface.facelog.db.IDeviceGroupManager Maven / Gradle / Ivy
// ______________________________________________________
// Generated by sql2java - https://github.com/10km/sql2java
// JDBC driver used at code generation time: com.mysql.jdbc.Driver
// template: manager.interface.java.vm
// ______________________________________________________
package net.gdface.facelog.db;
import gu.sql2java.TableManager;
import gu.sql2java.exception.ObjectRetrievalException;
import gu.sql2java.exception.RuntimeDaoException;
/**
* Interface to handle database calls (save, load, count, etc...) for the fl_device_group table.
* Remarks: 设备组信息
* @author guyadong
*/
public interface IDeviceGroupManager extends TableManager
{
//////////////////////////////////////
// PRIMARY KEY METHODS
//////////////////////////////////////
//1
/**
* Loads a {@link DeviceGroupBean} from the fl_device_group using primary key fields.
*
* @param id Integer - PK# 1
* @return a unique DeviceGroupBean or {@code null} if not found
* @throws RuntimeDaoException
*/
public DeviceGroupBean loadByPrimaryKey(Integer id)throws RuntimeDaoException;
//1.1
/**
* Loads a {@link DeviceGroupBean} from the fl_device_group using primary key fields.
*
* @param id Integer - PK# 1
* @return a unique DeviceGroupBean
* @throws ObjectRetrievalException if not found
* @throws RuntimeDaoException
*/
public DeviceGroupBean loadByPrimaryKeyChecked(Integer id) throws RuntimeDaoException,ObjectRetrievalException;
//1.4
/**
* check if contains row with primary key fields.
* @param id Integer - PK# 1
* @return true if this fl_device_group contains row with primary key fields.
* @throws RuntimeDaoException
*/
public boolean existsPrimaryKey(Integer id)throws RuntimeDaoException;
//1.4.1
/**
* Check duplicated row by primary keys,if row exists throw exception
* @param id primary keys
* @return id
* @throws RuntimeDaoException
* @throws ObjectRetrievalException
*/
public Integer checkDuplicate(Integer id)throws RuntimeDaoException,ObjectRetrievalException;
//1.8
/**
* Loads {@link DeviceGroupBean} from the fl_device_group using primary key fields.
*
* @param keys primary keys array
* @return list of DeviceGroupBean
* @throws RuntimeDaoException
*/
public java.util.List loadByPrimaryKey(Integer... keys)throws RuntimeDaoException;
//1.9
/**
* Loads {@link DeviceGroupBean} from the fl_device_group using primary key fields.
*
* @param keys primary keys collection
* @return list of DeviceGroupBean
* @throws RuntimeDaoException
*/
public java.util.List loadByPrimaryKey(java.util.Collection keys)throws RuntimeDaoException;
//2
/**
* Delete row according to its primary keys.
* all keys must not be null
*
* @param id Integer - PK# 1
* @return the number of deleted rows
* @throws RuntimeDaoException
*/
public int deleteByPrimaryKey(Integer id)throws RuntimeDaoException;
//2.2
/**
* Delete rows according to primary key.
*
* @param keys primary keys array
* @return the number of deleted rows
* @throws RuntimeDaoException
* @see #delete(gu.sql2java.BaseBean)
*/
public int deleteByPrimaryKey(Integer... keys)throws RuntimeDaoException;
//2.3
/**
* Delete rows according to primary key.
*
* @param keys primary keys collection
* @return the number of deleted rows
* @throws RuntimeDaoException
* @see #delete(gu.sql2java.BaseBean)
*/
public int deleteByPrimaryKey(java.util.Collection keys)throws RuntimeDaoException;
//////////////////////////////////////
// GET/SET IMPORTED KEY BEAN METHOD
//////////////////////////////////////
//3.1 GET IMPORTED
/**
* Retrieves the {@link DeviceBean} object from the fl_device.group_id field.
* FK_NAME : fl_device_ibfk_1
* @param bean the {@link DeviceGroupBean}
* @return the associated {@link DeviceBean} beans or {@code null} if {@code bean} is {@code null}
* @throws RuntimeDaoException
*/
public DeviceBean[] getDeviceBeansByGroupId(DeviceGroupBean bean)throws RuntimeDaoException;
//3.1.2 GET IMPORTED
/**
* Retrieves the {@link DeviceBean} object from the fl_device.group_id field.
* FK_NAME : fl_device_ibfk_1
* @param idOfDeviceGroup Integer - PK# 1
* @return the associated {@link DeviceBean} beans or {@code null} if {@code bean} is {@code null}
* @throws RuntimeDaoException
*/
public DeviceBean[] getDeviceBeansByGroupId(Integer idOfDeviceGroup)throws RuntimeDaoException;
//3.2 GET IMPORTED
/**
* see also #getDeviceBeansByGroupIdAsList(DeviceGroupBean,int,int)
* @param bean
* @return import bean list
* @throws RuntimeDaoException
*/
public java.util.List getDeviceBeansByGroupIdAsList(DeviceGroupBean bean)throws RuntimeDaoException;
//3.2.2 GET IMPORTED
/**
* Retrieves the {@link DeviceBean} object from fl_device.group_id field.
* FK_NAME:fl_device_ibfk_1
* @param idOfDeviceGroup Integer - PK# 1
* @return the associated {@link DeviceBean} beans
* @throws RuntimeDaoException
*/
public java.util.List getDeviceBeansByGroupIdAsList(Integer idOfDeviceGroup)throws RuntimeDaoException;
//3.2.3 DELETE IMPORTED
/**
* delete the associated {@link DeviceBean} objects from fl_device.group_id field.
* FK_NAME:fl_device_ibfk_1
* @param idOfDeviceGroup Integer - PK# 1
* @return the number of deleted rows
* @throws RuntimeDaoException
*/
public int deleteDeviceBeansByGroupId(Integer idOfDeviceGroup)throws RuntimeDaoException;
//3.2.4 GET IMPORTED
/**
* Retrieves the {@link DeviceBean} object from fl_device.group_id field.
* FK_NAME:fl_device_ibfk_1
* @param bean the {@link DeviceGroupBean}
* @param startRow the start row to be used (first row = 1, last row=-1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @return the associated {@link DeviceBean} beans or empty list if {@code bean} is {@code null}
* @throws RuntimeDaoException
*/
public java.util.List getDeviceBeansByGroupIdAsList(DeviceGroupBean bean,int startRow,int numRows)throws RuntimeDaoException;
//3.3 SET IMPORTED
/**
* set the {@link DeviceBean} object array associate to DeviceGroupBean by the fl_device.group_id field.
* FK_NAME : fl_device_ibfk_1
* @param bean the referenced {@link DeviceGroupBean}
* @param importedBeans imported beans from fl_device
* @return importedBeans always
* @see IDeviceManager#setReferencedByGroupId(DeviceBean, DeviceGroupBean)
* @throws RuntimeDaoException
*/
public DeviceBean[] setDeviceBeansByGroupId(DeviceGroupBean bean , DeviceBean[] importedBeans)throws RuntimeDaoException;
//3.4 SET IMPORTED
/**
* set the {@link DeviceBean} object java.util.Collection associate to DeviceGroupBean by the fl_device.group_id field.
* FK_NAME:fl_device_ibfk_1
* @param bean the referenced {@link DeviceGroupBean}
* @param importedBeans imported beans from fl_device
* @return importedBeans always
* @see IDeviceManager#setReferencedByGroupId(DeviceBean, DeviceGroupBean)
* @throws RuntimeDaoException
*/
public > C setDeviceBeansByGroupId(DeviceGroupBean bean , C importedBeans)throws RuntimeDaoException;
//3.1 GET IMPORTED
/**
* Retrieves the {@link DeviceGroupBean} object from the fl_device_group.parent field.
* FK_NAME : fl_device_group_ibfk_1
* @param bean the {@link DeviceGroupBean}
* @return the associated {@link DeviceGroupBean} beans or {@code null} if {@code bean} is {@code null}
* @throws RuntimeDaoException
*/
public DeviceGroupBean[] getDeviceGroupBeansByParent(DeviceGroupBean bean)throws RuntimeDaoException;
//3.1.2 GET IMPORTED
/**
* Retrieves the {@link DeviceGroupBean} object from the fl_device_group.parent field.
* FK_NAME : fl_device_group_ibfk_1
* @param idOfDeviceGroup Integer - PK# 1
* @return the associated {@link DeviceGroupBean} beans or {@code null} if {@code bean} is {@code null}
* @throws RuntimeDaoException
*/
public DeviceGroupBean[] getDeviceGroupBeansByParent(Integer idOfDeviceGroup)throws RuntimeDaoException;
//3.2 GET IMPORTED
/**
* see also #getDeviceGroupBeansByParentAsList(DeviceGroupBean,int,int)
* @param bean
* @return import bean list
* @throws RuntimeDaoException
*/
public java.util.List getDeviceGroupBeansByParentAsList(DeviceGroupBean bean)throws RuntimeDaoException;
//3.2.2 GET IMPORTED
/**
* Retrieves the {@link DeviceGroupBean} object from fl_device_group.parent field.
* FK_NAME:fl_device_group_ibfk_1
* @param idOfDeviceGroup Integer - PK# 1
* @return the associated {@link DeviceGroupBean} beans
* @throws RuntimeDaoException
*/
public java.util.List getDeviceGroupBeansByParentAsList(Integer idOfDeviceGroup)throws RuntimeDaoException;
//3.2.3 DELETE IMPORTED
/**
* delete the associated {@link DeviceGroupBean} objects from fl_device_group.parent field.
* FK_NAME:fl_device_group_ibfk_1
* @param idOfDeviceGroup Integer - PK# 1
* @return the number of deleted rows
* @throws RuntimeDaoException
*/
public int deleteDeviceGroupBeansByParent(Integer idOfDeviceGroup)throws RuntimeDaoException;
//3.2.4 GET IMPORTED
/**
* Retrieves the {@link DeviceGroupBean} object from fl_device_group.parent field.
* FK_NAME:fl_device_group_ibfk_1
* @param bean the {@link DeviceGroupBean}
* @param startRow the start row to be used (first row = 1, last row=-1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @return the associated {@link DeviceGroupBean} beans or empty list if {@code bean} is {@code null}
* @throws RuntimeDaoException
*/
public java.util.List getDeviceGroupBeansByParentAsList(DeviceGroupBean bean,int startRow,int numRows)throws RuntimeDaoException;
//3.3 SET IMPORTED
/**
* set the {@link DeviceGroupBean} object array associate to DeviceGroupBean by the fl_device_group.parent field.
* FK_NAME : fl_device_group_ibfk_1
* @param bean the referenced {@link DeviceGroupBean}
* @param importedBeans imported beans from fl_device_group
* @return importedBeans always
* @see IDeviceGroupManager#setReferencedByParent(DeviceGroupBean, DeviceGroupBean)
* @throws RuntimeDaoException
*/
public DeviceGroupBean[] setDeviceGroupBeansByParent(DeviceGroupBean bean , DeviceGroupBean[] importedBeans)throws RuntimeDaoException;
//3.4 SET IMPORTED
/**
* set the {@link DeviceGroupBean} object java.util.Collection associate to DeviceGroupBean by the fl_device_group.parent field.
* FK_NAME:fl_device_group_ibfk_1
* @param bean the referenced {@link DeviceGroupBean}
* @param importedBeans imported beans from fl_device_group
* @return importedBeans always
* @see IDeviceGroupManager#setReferencedByParent(DeviceGroupBean, DeviceGroupBean)
* @throws RuntimeDaoException
*/
public > C setDeviceGroupBeansByParent(DeviceGroupBean bean , C importedBeans)throws RuntimeDaoException;
//3.1 GET IMPORTED
/**
* Retrieves the {@link PermitBean} object from the fl_permit.device_group_id field.
* FK_NAME : fl_permit_ibfk_1
* @param bean the {@link DeviceGroupBean}
* @return the associated {@link PermitBean} beans or {@code null} if {@code bean} is {@code null}
* @throws RuntimeDaoException
*/
public PermitBean[] getPermitBeansByDeviceGroupId(DeviceGroupBean bean)throws RuntimeDaoException;
//3.1.2 GET IMPORTED
/**
* Retrieves the {@link PermitBean} object from the fl_permit.device_group_id field.
* FK_NAME : fl_permit_ibfk_1
* @param idOfDeviceGroup Integer - PK# 1
* @return the associated {@link PermitBean} beans or {@code null} if {@code bean} is {@code null}
* @throws RuntimeDaoException
*/
public PermitBean[] getPermitBeansByDeviceGroupId(Integer idOfDeviceGroup)throws RuntimeDaoException;
//3.2 GET IMPORTED
/**
* see also #getPermitBeansByDeviceGroupIdAsList(DeviceGroupBean,int,int)
* @param bean
* @return import bean list
* @throws RuntimeDaoException
*/
public java.util.List getPermitBeansByDeviceGroupIdAsList(DeviceGroupBean bean)throws RuntimeDaoException;
//3.2.2 GET IMPORTED
/**
* Retrieves the {@link PermitBean} object from fl_permit.device_group_id field.
* FK_NAME:fl_permit_ibfk_1
* @param idOfDeviceGroup Integer - PK# 1
* @return the associated {@link PermitBean} beans
* @throws RuntimeDaoException
*/
public java.util.List getPermitBeansByDeviceGroupIdAsList(Integer idOfDeviceGroup)throws RuntimeDaoException;
//3.2.3 DELETE IMPORTED
/**
* delete the associated {@link PermitBean} objects from fl_permit.device_group_id field.
* FK_NAME:fl_permit_ibfk_1
* @param idOfDeviceGroup Integer - PK# 1
* @return the number of deleted rows
* @throws RuntimeDaoException
*/
public int deletePermitBeansByDeviceGroupId(Integer idOfDeviceGroup)throws RuntimeDaoException;
//3.2.4 GET IMPORTED
/**
* Retrieves the {@link PermitBean} object from fl_permit.device_group_id field.
* FK_NAME:fl_permit_ibfk_1
* @param bean the {@link DeviceGroupBean}
* @param startRow the start row to be used (first row = 1, last row=-1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @return the associated {@link PermitBean} beans or empty list if {@code bean} is {@code null}
* @throws RuntimeDaoException
*/
public java.util.List getPermitBeansByDeviceGroupIdAsList(DeviceGroupBean bean,int startRow,int numRows)throws RuntimeDaoException;
//3.3 SET IMPORTED
/**
* set the {@link PermitBean} object array associate to DeviceGroupBean by the fl_permit.device_group_id field.
* FK_NAME : fl_permit_ibfk_1
* @param bean the referenced {@link DeviceGroupBean}
* @param importedBeans imported beans from fl_permit
* @return importedBeans always
* @see IPermitManager#setReferencedByDeviceGroupId(PermitBean, DeviceGroupBean)
* @throws RuntimeDaoException
*/
public PermitBean[] setPermitBeansByDeviceGroupId(DeviceGroupBean bean , PermitBean[] importedBeans)throws RuntimeDaoException;
//3.4 SET IMPORTED
/**
* set the {@link PermitBean} object java.util.Collection associate to DeviceGroupBean by the fl_permit.device_group_id field.
* FK_NAME:fl_permit_ibfk_1
* @param bean the referenced {@link DeviceGroupBean}
* @param importedBeans imported beans from fl_permit
* @return importedBeans always
* @see IPermitManager#setReferencedByDeviceGroupId(PermitBean, DeviceGroupBean)
* @throws RuntimeDaoException
*/
public > C setPermitBeansByDeviceGroupId(DeviceGroupBean bean , C importedBeans)throws RuntimeDaoException;
//3.5 SYNC SAVE
/**
* Save the DeviceGroupBean bean and referenced beans and imported beans into the database.
*
* @param bean the {@link DeviceGroupBean} bean to be saved
* @param refDevicegroupByParent the {@link DeviceGroupBean} bean referenced by {@link DeviceGroupBean}
* @param impDeviceByGroupId the {@link DeviceBean} bean refer to {@link DeviceGroupBean}
* @param impDevicegroupByParent the {@link DeviceGroupBean} bean refer to {@link DeviceGroupBean}
* @param impPermitByDeviceGroupId the {@link PermitBean} bean refer to {@link DeviceGroupBean}
* @return the inserted or updated {@link DeviceGroupBean} bean
* @throws RuntimeDaoException
*/
public DeviceGroupBean save(DeviceGroupBean bean
, DeviceGroupBean refDevicegroupByParent
, DeviceBean[] impDeviceByGroupId , DeviceGroupBean[] impDevicegroupByParent , PermitBean[] impPermitByDeviceGroupId )throws RuntimeDaoException;
//3.6 SYNC SAVE AS TRANSACTION
/**
* Transaction version for sync save
* see also {@link #save(DeviceGroupBean , DeviceGroupBean , DeviceBean[] , DeviceGroupBean[] , PermitBean[] )}
* @param bean the {@link DeviceGroupBean} bean to be saved
* @param refDevicegroupByParent the {@link DeviceGroupBean} bean referenced by {@link DeviceGroupBean}
* @param impDeviceByGroupId the {@link DeviceBean} bean refer to {@link DeviceGroupBean}
* @param impDevicegroupByParent the {@link DeviceGroupBean} bean refer to {@link DeviceGroupBean}
* @param impPermitByDeviceGroupId the {@link PermitBean} bean refer to {@link DeviceGroupBean}
* @return the inserted or updated {@link DeviceGroupBean} bean
* @throws RuntimeDaoException
*/
public DeviceGroupBean saveAsTransaction(final DeviceGroupBean bean
,final DeviceGroupBean refDevicegroupByParent
,final DeviceBean[] impDeviceByGroupId ,final DeviceGroupBean[] impDevicegroupByParent ,final PermitBean[] impPermitByDeviceGroupId )throws RuntimeDaoException;
//3.7 SYNC SAVE
/**
* Save the DeviceGroupBean bean and referenced beans and imported beans into the database.
*
* @param bean the {@link DeviceGroupBean} bean to be saved
* @param refDevicegroupByParent the {@link DeviceGroupBean} bean referenced by {@link DeviceGroupBean}
* @param impDeviceByGroupId the {@link DeviceBean} bean refer to {@link DeviceGroupBean}
* @param impDevicegroupByParent the {@link DeviceGroupBean} bean refer to {@link DeviceGroupBean}
* @param impPermitByDeviceGroupId the {@link PermitBean} bean refer to {@link DeviceGroupBean}
* @return the inserted or updated {@link DeviceGroupBean} bean
* @throws RuntimeDaoException
*/
public DeviceGroupBean save(DeviceGroupBean bean
, DeviceGroupBean refDevicegroupByParent
, java.util.Collection impDeviceByGroupId , java.util.Collection impDevicegroupByParent , java.util.Collection impPermitByDeviceGroupId )throws RuntimeDaoException;
//3.8 SYNC SAVE AS TRANSACTION
/**
* Transaction version for sync save
* see also {@link #save(DeviceGroupBean , DeviceGroupBean , java.util.Collection , java.util.Collection , java.util.Collection )}
* @param bean the {@link DeviceGroupBean} bean to be saved
* @param refDevicegroupByParent the {@link DeviceGroupBean} bean referenced by {@link DeviceGroupBean}
* @param impDeviceByGroupId the {@link DeviceBean} bean refer to {@link DeviceGroupBean}
* @param impDevicegroupByParent the {@link DeviceGroupBean} bean refer to {@link DeviceGroupBean}
* @param impPermitByDeviceGroupId the {@link PermitBean} bean refer to {@link DeviceGroupBean}
* @return the inserted or updated {@link DeviceGroupBean} bean
* @throws RuntimeDaoException
*/
public DeviceGroupBean saveAsTransaction(final DeviceGroupBean bean
,final DeviceGroupBean refDevicegroupByParent
,final java.util.Collection impDeviceByGroupId ,final java.util.Collection impDevicegroupByParent ,final java.util.Collection impPermitByDeviceGroupId )throws RuntimeDaoException;
//////////////////////////////////////
// GET/SET FOREIGN KEY BEAN METHOD
//////////////////////////////////////
//5.1 GET REFERENCED VALUE
/**
* Retrieves the {@link DeviceGroupBean} object referenced by {@link DeviceGroupBean#getParent}() field.
* FK_NAME : fl_device_group_ibfk_1
* @param bean the {@link DeviceGroupBean}
* @return the associated {@link DeviceGroupBean} bean or {@code null} if {@code bean} is {@code null}
* @throws RuntimeDaoException
*/
public DeviceGroupBean getReferencedByParent(DeviceGroupBean bean)throws RuntimeDaoException;
//5.2 SET REFERENCED
/**
* Associates the {@link DeviceGroupBean} object to the {@link DeviceGroupBean} object by {@link DeviceGroupBean#getParent}() field.
*
* @param bean the {@link DeviceGroupBean} object to use
* @param beanToSet the {@link DeviceGroupBean} object to associate to the {@link DeviceGroupBean}
* @return always beanToSet saved
* @throws RuntimeDaoException
*/
public DeviceGroupBean setReferencedByParent(DeviceGroupBean bean, DeviceGroupBean beanToSet)throws RuntimeDaoException;
//_____________________________________________________________________
//
// USING INDICES
//_____________________________________________________________________
/**
* Retrieves an array of DeviceGroupBean using the parent index.
*
* @param parent the parent column's value filter.
* @return an array of DeviceGroupBean
* @throws RuntimeDaoException
*/
public DeviceGroupBean[] loadByIndexParent(Integer parent)throws RuntimeDaoException;
/**
* Retrieves a list of DeviceGroupBean using the parent index.
*
* @param parent the parent column's value filter.
* @return a list of DeviceGroupBean
* @throws RuntimeDaoException
*/
public java.util.List loadByIndexParentAsList(Integer parent)throws RuntimeDaoException;
/**
* Deletes rows using the parent index.
*
* @param parent the parent column's value filter.
* @return the number of deleted objects
* @throws RuntimeDaoException
*/
public int deleteByIndexParent(Integer parent)throws RuntimeDaoException;
//45
/**
* return a primary key list from {@link DeviceGroupBean} array
* @param beans
* @return primary key list
*/
public java.util.List toPrimaryKeyList(DeviceGroupBean... beans);
//46
/**
* return a primary key list from {@link DeviceGroupBean} collection
* @param beans
* @return primary key list
*/
public java.util.List toPrimaryKeyList(java.util.Collection beans);
//_____________________________________________________________________
//
// MANY TO MANY: LOAD OTHER BEAN VIA JUNCTION TABLE
//_____________________________________________________________________
//22 MANY TO MANY
/**
* see also #loadViaPermitAsList(DeviceGroupBean,int,int)
* @param bean
* @return DeviceGroupBean list
* @throws RuntimeDaoException
*/
public java.util.List loadViaPermitAsList(PersonGroupBean bean)throws RuntimeDaoException;
//23 MANY TO MANY
/**
* Retrieves an list of DeviceGroupBean using the junction table Permit, given a PersonGroupBean,
* specifying the start row and the number of rows.
*
* @param bean the PersonGroupBean bean to be used
* @param startRow the start row to be used (first row = 1, last row = -1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @return a list of DeviceGroupBean
* @throws RuntimeDaoException
*/
public java.util.List loadViaPermitAsList(PersonGroupBean bean, int startRow, int numRows)throws RuntimeDaoException;
//23.2 MANY TO MANY
/**
* add junction between {@link DeviceGroupBean} and {@link PersonGroupBean} if junction not exists
* @param bean
* @param linked
* @throws RuntimeDaoException
*/
public void addJunction(DeviceGroupBean bean,PersonGroupBean linked)throws RuntimeDaoException;
//23.3 MANY TO MANY
/**
* remove junction between {@link DeviceGroupBean} and {@link PersonGroupBean}
* @param bean
* @param linked
* @return deleted rows count
* @throws RuntimeDaoException
*/
public int deleteJunction(DeviceGroupBean bean,PersonGroupBean linked)throws RuntimeDaoException;
//23.4 MANY TO MANY
/**
* see also {@link #addJunction(DeviceGroupBean,PersonGroupBean)}
* @param bean
* @param linkedBeans
* @throws RuntimeDaoException
*/
public void addJunction(DeviceGroupBean bean,PersonGroupBean... linkedBeans)throws RuntimeDaoException;
//23.5 MANY TO MANY
/**
* see also {@link #addJunction(DeviceGroupBean,PersonGroupBean)}
* @param bean
* @param linkedBeans
* @throws RuntimeDaoException
*/
public void addJunctionWithPersonGroup(DeviceGroupBean bean,java.util.Collection linkedBeans)throws RuntimeDaoException;
//23.6 MANY TO MANY
/**
* see also {@link #deleteJunction(DeviceGroupBean,PersonGroupBean)}
* @param bean
* @param linkedBeans
* @return count of deleted rows
* @throws RuntimeDaoException
*/
public int deleteJunction(DeviceGroupBean bean,PersonGroupBean... linkedBeans)throws RuntimeDaoException;
//23.7 MANY TO MANY
/**
* see also {@link #deleteJunction(DeviceGroupBean,PersonGroupBean)}
* @param bean
* @param linkedBeans
* @return count of deleted rows
* @throws RuntimeDaoException
*/
public int deleteJunctionWithPersonGroup(DeviceGroupBean bean,java.util.Collection linkedBeans)throws RuntimeDaoException;
//_____________________________________________________________________
//
// SELF-REFERENCE
//_____________________________________________________________________
//47
/**
* return bean list ( include bean specified by primary keys ) by the self-reference field : {@code fl_device_group(parent) }
* first element is top bean
* @param id PK# 1
* @return empty list if input primary key is {@code null}
* first element equal last if self-reference field is cycle
* @throws RuntimeDaoException
*/
public java.util.List listOfParent(Integer id)throws RuntimeDaoException;
//48
/**
* see also {@link #listOfParent(Integer)}
* @param bean
* @return DeviceGroupBean list
* @throws RuntimeDaoException
*/
public java.util.List listOfParent(DeviceGroupBean bean)throws RuntimeDaoException;
//49
/**
* get level count on the self-reference field : {@code fl_device_group(parent) }
* @param id PK# 1
* @return 0 if input primary key is {@code null}
* -1 if self-reference field is cycle
* @throws RuntimeDaoException
*/
public int levelOfParent(Integer id)throws RuntimeDaoException;
//50
/**
* see also {@link #levelOfParent(Integer)}
* @param bean
* @return level count
* @throws RuntimeDaoException
*/
public int levelOfParent(DeviceGroupBean bean)throws RuntimeDaoException;
//51
/**
* test whether the self-reference field is cycle : {@code fl_device_group(parent) }
* @param id PK# 1
* @see #levelOfParent(DeviceGroupBean)
* @return true if the self-reference field is cycle
* @throws RuntimeDaoException
*/
public boolean isCycleOnParent(Integer id)throws RuntimeDaoException;
//52
/**
* test whether the self-reference field is cycle : {@code fl_device_group(parent) }
* @param bean
* @return true if the self-reference field is cycle
* @throws RuntimeDaoException
* @see #levelOfParent(DeviceGroupBean)
*/
public boolean isCycleOnParent(DeviceGroupBean bean)throws RuntimeDaoException;
//53
/**
* return top bean that with {@code null} self-reference field : {@code fl_device_group(parent) }
* @param id PK# 1
* @return top bean
* @throws NullPointerException if input primary key is {@code null}
* @throws IllegalStateException if self-reference field is cycle
* @throws ObjectRetrievalException not found record by primary key
* @throws RuntimeDaoException
*/
public DeviceGroupBean topOfParent(Integer id)throws RuntimeDaoException;
//54
/**
* see also {@link #topOfParent(Integer)}
* @param bean
* @return top bean
* @throws NullPointerException if input primary key is {@code null}
* @throws IllegalStateException if self-reference field is cycle
* @throws ObjectRetrievalException not found record by primary key
* @throws RuntimeDaoException
*/
public DeviceGroupBean topOfParent(DeviceGroupBean bean)throws RuntimeDaoException;
//55
/**
* Ensures the self-reference field is not cycle : {@code fl_device_group(parent) }
* @param id PK# 1
* @return always {@code id}
* @throws IllegalStateException if self-reference field is cycle
* @throws RuntimeDaoException
* @see #isCycleOnParent(Integer)
*/
public Integer checkCycleOfParent(Integer id)throws RuntimeDaoException;
//56
/**
* Ensures the self-reference field is not cycle : {@code fl_device_group(parent) }
* @param bean
* @return always {@code bean}
* @throws IllegalStateException if self-reference field is cycle
* @throws RuntimeDaoException
* @see #isCycleOnParent(DeviceGroupBean)
*/
public DeviceGroupBean checkCycleOfParent(DeviceGroupBean bean)throws RuntimeDaoException;
//57
/**
* return child bean list (self included) by the self-reference field : {@code fl_device_group(parent) }
* throw {@link RuntimeDaoException} if self-reference field is cycle
* @param id PK# 1
* @return child bean list,empty list if not found record
* @throws IllegalStateException if self-reference field is cycle
* @throws RuntimeDaoException
*/
public java.util.List childListByParent(Integer id)throws RuntimeDaoException;
//58
/**
* see also {@link #childListByParent(Integer)}
* @param bean
* @return child bean list,empty list if not found record
* @throws RuntimeDaoException
*/
public java.util.List childListByParent(DeviceGroupBean bean)throws RuntimeDaoException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy