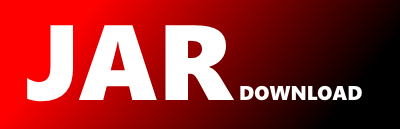
net.gdface.facelog.db.IDeviceManager Maven / Gradle / Ivy
// ______________________________________________________
// Generated by sql2java - https://github.com/10km/sql2java
// JDBC driver used at code generation time: com.mysql.jdbc.Driver
// template: manager.interface.java.vm
// ______________________________________________________
package net.gdface.facelog.db;
import gu.sql2java.TableManager;
import gu.sql2java.exception.ObjectRetrievalException;
import gu.sql2java.exception.RuntimeDaoException;
/**
* Interface to handle database calls (save, load, count, etc...) for the fl_device table.
* Remarks: 前端设备基本信息
* @author guyadong
*/
public interface IDeviceManager extends TableManager
{
//////////////////////////////////////
// PRIMARY KEY METHODS
//////////////////////////////////////
//1
/**
* Loads a {@link DeviceBean} from the fl_device using primary key fields.
*
* @param id Integer - PK# 1
* @return a unique DeviceBean or {@code null} if not found
* @throws RuntimeDaoException
*/
public DeviceBean loadByPrimaryKey(Integer id)throws RuntimeDaoException;
//1.1
/**
* Loads a {@link DeviceBean} from the fl_device using primary key fields.
*
* @param id Integer - PK# 1
* @return a unique DeviceBean
* @throws ObjectRetrievalException if not found
* @throws RuntimeDaoException
*/
public DeviceBean loadByPrimaryKeyChecked(Integer id) throws RuntimeDaoException,ObjectRetrievalException;
//1.4
/**
* check if contains row with primary key fields.
* @param id Integer - PK# 1
* @return true if this fl_device contains row with primary key fields.
* @throws RuntimeDaoException
*/
public boolean existsPrimaryKey(Integer id)throws RuntimeDaoException;
//1.4.1
/**
* Check duplicated row by primary keys,if row exists throw exception
* @param id primary keys
* @return id
* @throws RuntimeDaoException
* @throws ObjectRetrievalException
*/
public Integer checkDuplicate(Integer id)throws RuntimeDaoException,ObjectRetrievalException;
//1.8
/**
* Loads {@link DeviceBean} from the fl_device using primary key fields.
*
* @param keys primary keys array
* @return list of DeviceBean
* @throws RuntimeDaoException
*/
public java.util.List loadByPrimaryKey(Integer... keys)throws RuntimeDaoException;
//1.9
/**
* Loads {@link DeviceBean} from the fl_device using primary key fields.
*
* @param keys primary keys collection
* @return list of DeviceBean
* @throws RuntimeDaoException
*/
public java.util.List loadByPrimaryKey(java.util.Collection keys)throws RuntimeDaoException;
//2
/**
* Delete row according to its primary keys.
* all keys must not be null
*
* @param id Integer - PK# 1
* @return the number of deleted rows
* @throws RuntimeDaoException
*/
public int deleteByPrimaryKey(Integer id)throws RuntimeDaoException;
//2.2
/**
* Delete rows according to primary key.
*
* @param keys primary keys array
* @return the number of deleted rows
* @throws RuntimeDaoException
* @see #delete(gu.sql2java.BaseBean)
*/
public int deleteByPrimaryKey(Integer... keys)throws RuntimeDaoException;
//2.3
/**
* Delete rows according to primary key.
*
* @param keys primary keys collection
* @return the number of deleted rows
* @throws RuntimeDaoException
* @see #delete(gu.sql2java.BaseBean)
*/
public int deleteByPrimaryKey(java.util.Collection keys)throws RuntimeDaoException;
//////////////////////////////////////
// GET/SET IMPORTED KEY BEAN METHOD
//////////////////////////////////////
//3.1 GET IMPORTED
/**
* Retrieves the {@link ErrorLogBean} object from the fl_error_log.device_id field.
* FK_NAME : fl_error_log_ibfk_2
* @param bean the {@link DeviceBean}
* @return the associated {@link ErrorLogBean} beans or {@code null} if {@code bean} is {@code null}
* @throws RuntimeDaoException
*/
public ErrorLogBean[] getErrorLogBeansByDeviceId(DeviceBean bean)throws RuntimeDaoException;
//3.1.2 GET IMPORTED
/**
* Retrieves the {@link ErrorLogBean} object from the fl_error_log.device_id field.
* FK_NAME : fl_error_log_ibfk_2
* @param idOfDevice Integer - PK# 1
* @return the associated {@link ErrorLogBean} beans or {@code null} if {@code bean} is {@code null}
* @throws RuntimeDaoException
*/
public ErrorLogBean[] getErrorLogBeansByDeviceId(Integer idOfDevice)throws RuntimeDaoException;
//3.2 GET IMPORTED
/**
* see also #getErrorLogBeansByDeviceIdAsList(DeviceBean,int,int)
* @param bean
* @return import bean list
* @throws RuntimeDaoException
*/
public java.util.List getErrorLogBeansByDeviceIdAsList(DeviceBean bean)throws RuntimeDaoException;
//3.2.2 GET IMPORTED
/**
* Retrieves the {@link ErrorLogBean} object from fl_error_log.device_id field.
* FK_NAME:fl_error_log_ibfk_2
* @param idOfDevice Integer - PK# 1
* @return the associated {@link ErrorLogBean} beans
* @throws RuntimeDaoException
*/
public java.util.List getErrorLogBeansByDeviceIdAsList(Integer idOfDevice)throws RuntimeDaoException;
//3.2.3 DELETE IMPORTED
/**
* delete the associated {@link ErrorLogBean} objects from fl_error_log.device_id field.
* FK_NAME:fl_error_log_ibfk_2
* @param idOfDevice Integer - PK# 1
* @return the number of deleted rows
* @throws RuntimeDaoException
*/
public int deleteErrorLogBeansByDeviceId(Integer idOfDevice)throws RuntimeDaoException;
//3.2.4 GET IMPORTED
/**
* Retrieves the {@link ErrorLogBean} object from fl_error_log.device_id field.
* FK_NAME:fl_error_log_ibfk_2
* @param bean the {@link DeviceBean}
* @param startRow the start row to be used (first row = 1, last row=-1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @return the associated {@link ErrorLogBean} beans or empty list if {@code bean} is {@code null}
* @throws RuntimeDaoException
*/
public java.util.List getErrorLogBeansByDeviceIdAsList(DeviceBean bean,int startRow,int numRows)throws RuntimeDaoException;
//3.3 SET IMPORTED
/**
* set the {@link ErrorLogBean} object array associate to DeviceBean by the fl_error_log.device_id field.
* FK_NAME : fl_error_log_ibfk_2
* @param bean the referenced {@link DeviceBean}
* @param importedBeans imported beans from fl_error_log
* @return importedBeans always
* @see IErrorLogManager#setReferencedByDeviceId(ErrorLogBean, DeviceBean)
* @throws RuntimeDaoException
*/
public ErrorLogBean[] setErrorLogBeansByDeviceId(DeviceBean bean , ErrorLogBean[] importedBeans)throws RuntimeDaoException;
//3.4 SET IMPORTED
/**
* set the {@link ErrorLogBean} object java.util.Collection associate to DeviceBean by the fl_error_log.device_id field.
* FK_NAME:fl_error_log_ibfk_2
* @param bean the referenced {@link DeviceBean}
* @param importedBeans imported beans from fl_error_log
* @return importedBeans always
* @see IErrorLogManager#setReferencedByDeviceId(ErrorLogBean, DeviceBean)
* @throws RuntimeDaoException
*/
public > C setErrorLogBeansByDeviceId(DeviceBean bean , C importedBeans)throws RuntimeDaoException;
//3.1 GET IMPORTED
/**
* Retrieves the {@link ImageBean} object from the fl_image.device_id field.
* FK_NAME : fl_image_ibfk_1
* @param bean the {@link DeviceBean}
* @return the associated {@link ImageBean} beans or {@code null} if {@code bean} is {@code null}
* @throws RuntimeDaoException
*/
public ImageBean[] getImageBeansByDeviceId(DeviceBean bean)throws RuntimeDaoException;
//3.1.2 GET IMPORTED
/**
* Retrieves the {@link ImageBean} object from the fl_image.device_id field.
* FK_NAME : fl_image_ibfk_1
* @param idOfDevice Integer - PK# 1
* @return the associated {@link ImageBean} beans or {@code null} if {@code bean} is {@code null}
* @throws RuntimeDaoException
*/
public ImageBean[] getImageBeansByDeviceId(Integer idOfDevice)throws RuntimeDaoException;
//3.2 GET IMPORTED
/**
* see also #getImageBeansByDeviceIdAsList(DeviceBean,int,int)
* @param bean
* @return import bean list
* @throws RuntimeDaoException
*/
public java.util.List getImageBeansByDeviceIdAsList(DeviceBean bean)throws RuntimeDaoException;
//3.2.2 GET IMPORTED
/**
* Retrieves the {@link ImageBean} object from fl_image.device_id field.
* FK_NAME:fl_image_ibfk_1
* @param idOfDevice Integer - PK# 1
* @return the associated {@link ImageBean} beans
* @throws RuntimeDaoException
*/
public java.util.List getImageBeansByDeviceIdAsList(Integer idOfDevice)throws RuntimeDaoException;
//3.2.3 DELETE IMPORTED
/**
* delete the associated {@link ImageBean} objects from fl_image.device_id field.
* FK_NAME:fl_image_ibfk_1
* @param idOfDevice Integer - PK# 1
* @return the number of deleted rows
* @throws RuntimeDaoException
*/
public int deleteImageBeansByDeviceId(Integer idOfDevice)throws RuntimeDaoException;
//3.2.4 GET IMPORTED
/**
* Retrieves the {@link ImageBean} object from fl_image.device_id field.
* FK_NAME:fl_image_ibfk_1
* @param bean the {@link DeviceBean}
* @param startRow the start row to be used (first row = 1, last row=-1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @return the associated {@link ImageBean} beans or empty list if {@code bean} is {@code null}
* @throws RuntimeDaoException
*/
public java.util.List getImageBeansByDeviceIdAsList(DeviceBean bean,int startRow,int numRows)throws RuntimeDaoException;
//3.3 SET IMPORTED
/**
* set the {@link ImageBean} object array associate to DeviceBean by the fl_image.device_id field.
* FK_NAME : fl_image_ibfk_1
* @param bean the referenced {@link DeviceBean}
* @param importedBeans imported beans from fl_image
* @return importedBeans always
* @see IImageManager#setReferencedByDeviceId(ImageBean, DeviceBean)
* @throws RuntimeDaoException
*/
public ImageBean[] setImageBeansByDeviceId(DeviceBean bean , ImageBean[] importedBeans)throws RuntimeDaoException;
//3.4 SET IMPORTED
/**
* set the {@link ImageBean} object java.util.Collection associate to DeviceBean by the fl_image.device_id field.
* FK_NAME:fl_image_ibfk_1
* @param bean the referenced {@link DeviceBean}
* @param importedBeans imported beans from fl_image
* @return importedBeans always
* @see IImageManager#setReferencedByDeviceId(ImageBean, DeviceBean)
* @throws RuntimeDaoException
*/
public > C setImageBeansByDeviceId(DeviceBean bean , C importedBeans)throws RuntimeDaoException;
//3.1 GET IMPORTED
/**
* Retrieves the {@link LogBean} object from the fl_log.device_id field.
* FK_NAME : fl_log_ibfk_2
* @param bean the {@link DeviceBean}
* @return the associated {@link LogBean} beans or {@code null} if {@code bean} is {@code null}
* @throws RuntimeDaoException
*/
public LogBean[] getLogBeansByDeviceId(DeviceBean bean)throws RuntimeDaoException;
//3.1.2 GET IMPORTED
/**
* Retrieves the {@link LogBean} object from the fl_log.device_id field.
* FK_NAME : fl_log_ibfk_2
* @param idOfDevice Integer - PK# 1
* @return the associated {@link LogBean} beans or {@code null} if {@code bean} is {@code null}
* @throws RuntimeDaoException
*/
public LogBean[] getLogBeansByDeviceId(Integer idOfDevice)throws RuntimeDaoException;
//3.2 GET IMPORTED
/**
* see also #getLogBeansByDeviceIdAsList(DeviceBean,int,int)
* @param bean
* @return import bean list
* @throws RuntimeDaoException
*/
public java.util.List getLogBeansByDeviceIdAsList(DeviceBean bean)throws RuntimeDaoException;
//3.2.2 GET IMPORTED
/**
* Retrieves the {@link LogBean} object from fl_log.device_id field.
* FK_NAME:fl_log_ibfk_2
* @param idOfDevice Integer - PK# 1
* @return the associated {@link LogBean} beans
* @throws RuntimeDaoException
*/
public java.util.List getLogBeansByDeviceIdAsList(Integer idOfDevice)throws RuntimeDaoException;
//3.2.3 DELETE IMPORTED
/**
* delete the associated {@link LogBean} objects from fl_log.device_id field.
* FK_NAME:fl_log_ibfk_2
* @param idOfDevice Integer - PK# 1
* @return the number of deleted rows
* @throws RuntimeDaoException
*/
public int deleteLogBeansByDeviceId(Integer idOfDevice)throws RuntimeDaoException;
//3.2.4 GET IMPORTED
/**
* Retrieves the {@link LogBean} object from fl_log.device_id field.
* FK_NAME:fl_log_ibfk_2
* @param bean the {@link DeviceBean}
* @param startRow the start row to be used (first row = 1, last row=-1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @return the associated {@link LogBean} beans or empty list if {@code bean} is {@code null}
* @throws RuntimeDaoException
*/
public java.util.List getLogBeansByDeviceIdAsList(DeviceBean bean,int startRow,int numRows)throws RuntimeDaoException;
//3.3 SET IMPORTED
/**
* set the {@link LogBean} object array associate to DeviceBean by the fl_log.device_id field.
* FK_NAME : fl_log_ibfk_2
* @param bean the referenced {@link DeviceBean}
* @param importedBeans imported beans from fl_log
* @return importedBeans always
* @see ILogManager#setReferencedByDeviceId(LogBean, DeviceBean)
* @throws RuntimeDaoException
*/
public LogBean[] setLogBeansByDeviceId(DeviceBean bean , LogBean[] importedBeans)throws RuntimeDaoException;
//3.4 SET IMPORTED
/**
* set the {@link LogBean} object java.util.Collection associate to DeviceBean by the fl_log.device_id field.
* FK_NAME:fl_log_ibfk_2
* @param bean the referenced {@link DeviceBean}
* @param importedBeans imported beans from fl_log
* @return importedBeans always
* @see ILogManager#setReferencedByDeviceId(LogBean, DeviceBean)
* @throws RuntimeDaoException
*/
public > C setLogBeansByDeviceId(DeviceBean bean , C importedBeans)throws RuntimeDaoException;
//3.5 SYNC SAVE
/**
* Save the DeviceBean bean and referenced beans and imported beans into the database.
*
* @param bean the {@link DeviceBean} bean to be saved
* @param refDevicegroupByGroupId the {@link DeviceGroupBean} bean referenced by {@link DeviceBean}
* @param impErrorlogByDeviceId the {@link ErrorLogBean} bean refer to {@link DeviceBean}
* @param impImageByDeviceId the {@link ImageBean} bean refer to {@link DeviceBean}
* @param impLogByDeviceId the {@link LogBean} bean refer to {@link DeviceBean}
* @return the inserted or updated {@link DeviceBean} bean
* @throws RuntimeDaoException
*/
public DeviceBean save(DeviceBean bean
, DeviceGroupBean refDevicegroupByGroupId
, ErrorLogBean[] impErrorlogByDeviceId , ImageBean[] impImageByDeviceId , LogBean[] impLogByDeviceId )throws RuntimeDaoException;
//3.6 SYNC SAVE AS TRANSACTION
/**
* Transaction version for sync save
* see also {@link #save(DeviceBean , DeviceGroupBean , ErrorLogBean[] , ImageBean[] , LogBean[] )}
* @param bean the {@link DeviceBean} bean to be saved
* @param refDevicegroupByGroupId the {@link DeviceGroupBean} bean referenced by {@link DeviceBean}
* @param impErrorlogByDeviceId the {@link ErrorLogBean} bean refer to {@link DeviceBean}
* @param impImageByDeviceId the {@link ImageBean} bean refer to {@link DeviceBean}
* @param impLogByDeviceId the {@link LogBean} bean refer to {@link DeviceBean}
* @return the inserted or updated {@link DeviceBean} bean
* @throws RuntimeDaoException
*/
public DeviceBean saveAsTransaction(final DeviceBean bean
,final DeviceGroupBean refDevicegroupByGroupId
,final ErrorLogBean[] impErrorlogByDeviceId ,final ImageBean[] impImageByDeviceId ,final LogBean[] impLogByDeviceId )throws RuntimeDaoException;
//3.7 SYNC SAVE
/**
* Save the DeviceBean bean and referenced beans and imported beans into the database.
*
* @param bean the {@link DeviceBean} bean to be saved
* @param refDevicegroupByGroupId the {@link DeviceGroupBean} bean referenced by {@link DeviceBean}
* @param impErrorlogByDeviceId the {@link ErrorLogBean} bean refer to {@link DeviceBean}
* @param impImageByDeviceId the {@link ImageBean} bean refer to {@link DeviceBean}
* @param impLogByDeviceId the {@link LogBean} bean refer to {@link DeviceBean}
* @return the inserted or updated {@link DeviceBean} bean
* @throws RuntimeDaoException
*/
public DeviceBean save(DeviceBean bean
, DeviceGroupBean refDevicegroupByGroupId
, java.util.Collection impErrorlogByDeviceId , java.util.Collection impImageByDeviceId , java.util.Collection impLogByDeviceId )throws RuntimeDaoException;
//3.8 SYNC SAVE AS TRANSACTION
/**
* Transaction version for sync save
* see also {@link #save(DeviceBean , DeviceGroupBean , java.util.Collection , java.util.Collection , java.util.Collection )}
* @param bean the {@link DeviceBean} bean to be saved
* @param refDevicegroupByGroupId the {@link DeviceGroupBean} bean referenced by {@link DeviceBean}
* @param impErrorlogByDeviceId the {@link ErrorLogBean} bean refer to {@link DeviceBean}
* @param impImageByDeviceId the {@link ImageBean} bean refer to {@link DeviceBean}
* @param impLogByDeviceId the {@link LogBean} bean refer to {@link DeviceBean}
* @return the inserted or updated {@link DeviceBean} bean
* @throws RuntimeDaoException
*/
public DeviceBean saveAsTransaction(final DeviceBean bean
,final DeviceGroupBean refDevicegroupByGroupId
,final java.util.Collection impErrorlogByDeviceId ,final java.util.Collection impImageByDeviceId ,final java.util.Collection impLogByDeviceId )throws RuntimeDaoException;
//////////////////////////////////////
// GET/SET FOREIGN KEY BEAN METHOD
//////////////////////////////////////
//5.1 GET REFERENCED VALUE
/**
* Retrieves the {@link DeviceGroupBean} object referenced by {@link DeviceBean#getGroupId}() field.
* FK_NAME : fl_device_ibfk_1
* @param bean the {@link DeviceBean}
* @return the associated {@link DeviceGroupBean} bean or {@code null} if {@code bean} is {@code null}
* @throws RuntimeDaoException
*/
public DeviceGroupBean getReferencedByGroupId(DeviceBean bean)throws RuntimeDaoException;
//5.2 SET REFERENCED
/**
* Associates the {@link DeviceBean} object to the {@link DeviceGroupBean} object by {@link DeviceBean#getGroupId}() field.
*
* @param bean the {@link DeviceBean} object to use
* @param beanToSet the {@link DeviceGroupBean} object to associate to the {@link DeviceBean}
* @return always beanToSet saved
* @throws RuntimeDaoException
*/
public DeviceGroupBean setReferencedByGroupId(DeviceBean bean, DeviceGroupBean beanToSet)throws RuntimeDaoException;
//_____________________________________________________________________
//
// USING INDICES
//_____________________________________________________________________
/**
* Retrieves an unique DeviceBean using the mac index.
*
* @param mac the mac column's value filter
* @return an DeviceBean,otherwise null if not found or exists null in input arguments
* @throws RuntimeDaoException
*/
public DeviceBean loadByIndexMac(String mac)throws RuntimeDaoException;
/**
* Retrieves an unique DeviceBean using the mac index.
*
* @param mac the mac column's value filter. must not be null
* @return an DeviceBean
* @throws NullPointerException exists null in input arguments
* @throws ObjectRetrievalException if not found
* @throws RuntimeDaoException
*/
public DeviceBean loadByIndexMacChecked(String mac)throws RuntimeDaoException,ObjectRetrievalException;
/**
* Retrieves an unique DeviceBean for each mac index.
*
* @param indexs index array
* @return an list of DeviceBean
* @throws RuntimeDaoException
*/
public java.util.List loadByIndexMac(String... indexs)throws RuntimeDaoException;
/**
* Retrieves an unique DeviceBean for each mac index.
*
* @param indexs index collection
* @return an list of DeviceBean
* @throws RuntimeDaoException
*/
public java.util.List loadByIndexMac(java.util.Collection indexs)throws RuntimeDaoException;
/**
* Deletes rows for each mac index.
*
* @param indexs index array
* @return the number of deleted rows
* @throws RuntimeDaoException
*/
public int deleteByIndexMac(String... indexs)throws RuntimeDaoException;
/**
* Deletes rows for each mac index.
*
* @param indexs index collection
* @return the number of deleted rows
* @throws RuntimeDaoException
*/
public int deleteByIndexMac(java.util.Collection indexs)throws RuntimeDaoException;
/**
* Deletes rows using the mac index.
*
* @param mac the mac column's value filter.
* @return the number of deleted objects
* @throws RuntimeDaoException
*/
public int deleteByIndexMac(String mac)throws RuntimeDaoException;
/**
* Retrieves an unique DeviceBean using the serial_no index.
*
* @param serialNo the serial_no column's value filter
* @return an DeviceBean,otherwise null if not found or exists null in input arguments
* @throws RuntimeDaoException
*/
public DeviceBean loadByIndexSerialNo(String serialNo)throws RuntimeDaoException;
/**
* Retrieves an unique DeviceBean using the serial_no index.
*
* @param serialNo the serial_no column's value filter. must not be null
* @return an DeviceBean
* @throws NullPointerException exists null in input arguments
* @throws ObjectRetrievalException if not found
* @throws RuntimeDaoException
*/
public DeviceBean loadByIndexSerialNoChecked(String serialNo)throws RuntimeDaoException,ObjectRetrievalException;
/**
* Retrieves an unique DeviceBean for each serial_no index.
*
* @param indexs index array
* @return an list of DeviceBean
* @throws RuntimeDaoException
*/
public java.util.List loadByIndexSerialNo(String... indexs)throws RuntimeDaoException;
/**
* Retrieves an unique DeviceBean for each serial_no index.
*
* @param indexs index collection
* @return an list of DeviceBean
* @throws RuntimeDaoException
*/
public java.util.List loadByIndexSerialNo(java.util.Collection indexs)throws RuntimeDaoException;
/**
* Deletes rows for each serial_no index.
*
* @param indexs index array
* @return the number of deleted rows
* @throws RuntimeDaoException
*/
public int deleteByIndexSerialNo(String... indexs)throws RuntimeDaoException;
/**
* Deletes rows for each serial_no index.
*
* @param indexs index collection
* @return the number of deleted rows
* @throws RuntimeDaoException
*/
public int deleteByIndexSerialNo(java.util.Collection indexs)throws RuntimeDaoException;
/**
* Deletes rows using the serial_no index.
*
* @param serialNo the serial_no column's value filter.
* @return the number of deleted objects
* @throws RuntimeDaoException
*/
public int deleteByIndexSerialNo(String serialNo)throws RuntimeDaoException;
/**
* Retrieves an array of DeviceBean using the group_id index.
*
* @param groupId the group_id column's value filter.
* @return an array of DeviceBean
* @throws RuntimeDaoException
*/
public DeviceBean[] loadByIndexGroupId(Integer groupId)throws RuntimeDaoException;
/**
* Retrieves a list of DeviceBean using the group_id index.
*
* @param groupId the group_id column's value filter.
* @return a list of DeviceBean
* @throws RuntimeDaoException
*/
public java.util.List loadByIndexGroupIdAsList(Integer groupId)throws RuntimeDaoException;
/**
* Deletes rows using the group_id index.
*
* @param groupId the group_id column's value filter.
* @return the number of deleted objects
* @throws RuntimeDaoException
*/
public int deleteByIndexGroupId(Integer groupId)throws RuntimeDaoException;
//45
/**
* return a primary key list from {@link DeviceBean} array
* @param beans
* @return primary key list
*/
public java.util.List toPrimaryKeyList(DeviceBean... beans);
//46
/**
* return a primary key list from {@link DeviceBean} collection
* @param beans
* @return primary key list
*/
public java.util.List toPrimaryKeyList(java.util.Collection beans);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy