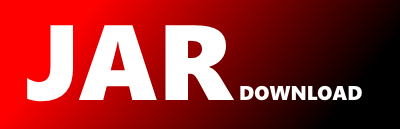
net.gdface.facelog.db.IErrorLogManager Maven / Gradle / Ivy
// ______________________________________________________
// Generated by sql2java - https://github.com/10km/sql2java
// JDBC driver used at code generation time: com.mysql.jdbc.Driver
// template: manager.interface.java.vm
// ______________________________________________________
package net.gdface.facelog.db;
import gu.sql2java.TableManager;
import gu.sql2java.exception.ObjectRetrievalException;
import gu.sql2java.exception.RuntimeDaoException;
/**
* Interface to handle database calls (save, load, count, etc...) for the fl_error_log table.
* Remarks: 系统错误日志,记录系统产生错误
* @author guyadong
*/
public interface IErrorLogManager extends TableManager
{
//////////////////////////////////////
// PRIMARY KEY METHODS
//////////////////////////////////////
//1
/**
* Loads a {@link ErrorLogBean} from the fl_error_log using primary key fields.
*
* @param id Integer - PK# 1
* @return a unique ErrorLogBean or {@code null} if not found
* @throws RuntimeDaoException
*/
public ErrorLogBean loadByPrimaryKey(Integer id)throws RuntimeDaoException;
//1.1
/**
* Loads a {@link ErrorLogBean} from the fl_error_log using primary key fields.
*
* @param id Integer - PK# 1
* @return a unique ErrorLogBean
* @throws ObjectRetrievalException if not found
* @throws RuntimeDaoException
*/
public ErrorLogBean loadByPrimaryKeyChecked(Integer id) throws RuntimeDaoException,ObjectRetrievalException;
//1.4
/**
* check if contains row with primary key fields.
* @param id Integer - PK# 1
* @return true if this fl_error_log contains row with primary key fields.
* @throws RuntimeDaoException
*/
public boolean existsPrimaryKey(Integer id)throws RuntimeDaoException;
//1.4.1
/**
* Check duplicated row by primary keys,if row exists throw exception
* @param id primary keys
* @return id
* @throws RuntimeDaoException
* @throws ObjectRetrievalException
*/
public Integer checkDuplicate(Integer id)throws RuntimeDaoException,ObjectRetrievalException;
//1.8
/**
* Loads {@link ErrorLogBean} from the fl_error_log using primary key fields.
*
* @param keys primary keys array
* @return list of ErrorLogBean
* @throws RuntimeDaoException
*/
public java.util.List loadByPrimaryKey(Integer... keys)throws RuntimeDaoException;
//1.9
/**
* Loads {@link ErrorLogBean} from the fl_error_log using primary key fields.
*
* @param keys primary keys collection
* @return list of ErrorLogBean
* @throws RuntimeDaoException
*/
public java.util.List loadByPrimaryKey(java.util.Collection keys)throws RuntimeDaoException;
//2
/**
* Delete row according to its primary keys.
* all keys must not be null
*
* @param id Integer - PK# 1
* @return the number of deleted rows
* @throws RuntimeDaoException
*/
public int deleteByPrimaryKey(Integer id)throws RuntimeDaoException;
//2.2
/**
* Delete rows according to primary key.
*
* @param keys primary keys array
* @return the number of deleted rows
* @throws RuntimeDaoException
* @see #delete(gu.sql2java.BaseBean)
*/
public int deleteByPrimaryKey(Integer... keys)throws RuntimeDaoException;
//2.3
/**
* Delete rows according to primary key.
*
* @param keys primary keys collection
* @return the number of deleted rows
* @throws RuntimeDaoException
* @see #delete(gu.sql2java.BaseBean)
*/
public int deleteByPrimaryKey(java.util.Collection keys)throws RuntimeDaoException;
//3.5 SYNC SAVE
/**
* Save the ErrorLogBean bean and referenced beans and imported beans into the database.
*
* @param bean the {@link ErrorLogBean} bean to be saved
* @param refDeviceByDeviceId the {@link DeviceBean} bean referenced by {@link ErrorLogBean}
* @param refPersonByPersonId the {@link PersonBean} bean referenced by {@link ErrorLogBean}
* @return the inserted or updated {@link ErrorLogBean} bean
* @throws RuntimeDaoException
*/
public ErrorLogBean save(ErrorLogBean bean
, DeviceBean refDeviceByDeviceId , PersonBean refPersonByPersonId
)throws RuntimeDaoException;
//3.6 SYNC SAVE AS TRANSACTION
/**
* Transaction version for sync save
* see also {@link #save(ErrorLogBean , DeviceBean , PersonBean )}
* @param bean the {@link ErrorLogBean} bean to be saved
* @param refDeviceByDeviceId the {@link DeviceBean} bean referenced by {@link ErrorLogBean}
* @param refPersonByPersonId the {@link PersonBean} bean referenced by {@link ErrorLogBean}
* @return the inserted or updated {@link ErrorLogBean} bean
* @throws RuntimeDaoException
*/
public ErrorLogBean saveAsTransaction(final ErrorLogBean bean
,final DeviceBean refDeviceByDeviceId ,final PersonBean refPersonByPersonId
)throws RuntimeDaoException;
//////////////////////////////////////
// GET/SET FOREIGN KEY BEAN METHOD
//////////////////////////////////////
//5.1 GET REFERENCED VALUE
/**
* Retrieves the {@link DeviceBean} object referenced by {@link ErrorLogBean#getDeviceId}() field.
* FK_NAME : fl_error_log_ibfk_2
* @param bean the {@link ErrorLogBean}
* @return the associated {@link DeviceBean} bean or {@code null} if {@code bean} is {@code null}
* @throws RuntimeDaoException
*/
public DeviceBean getReferencedByDeviceId(ErrorLogBean bean)throws RuntimeDaoException;
//5.2 SET REFERENCED
/**
* Associates the {@link ErrorLogBean} object to the {@link DeviceBean} object by {@link ErrorLogBean#getDeviceId}() field.
*
* @param bean the {@link ErrorLogBean} object to use
* @param beanToSet the {@link DeviceBean} object to associate to the {@link ErrorLogBean}
* @return always beanToSet saved
* @throws RuntimeDaoException
*/
public DeviceBean setReferencedByDeviceId(ErrorLogBean bean, DeviceBean beanToSet)throws RuntimeDaoException;
//5.1 GET REFERENCED VALUE
/**
* Retrieves the {@link PersonBean} object referenced by {@link ErrorLogBean#getPersonId}() field.
* FK_NAME : fl_error_log_ibfk_1
* @param bean the {@link ErrorLogBean}
* @return the associated {@link PersonBean} bean or {@code null} if {@code bean} is {@code null}
* @throws RuntimeDaoException
*/
public PersonBean getReferencedByPersonId(ErrorLogBean bean)throws RuntimeDaoException;
//5.2 SET REFERENCED
/**
* Associates the {@link ErrorLogBean} object to the {@link PersonBean} object by {@link ErrorLogBean#getPersonId}() field.
*
* @param bean the {@link ErrorLogBean} object to use
* @param beanToSet the {@link PersonBean} object to associate to the {@link ErrorLogBean}
* @return always beanToSet saved
* @throws RuntimeDaoException
*/
public PersonBean setReferencedByPersonId(ErrorLogBean bean, PersonBean beanToSet)throws RuntimeDaoException;
//_____________________________________________________________________
//
// USING INDICES
//_____________________________________________________________________
/**
* Retrieves an array of ErrorLogBean using the catalog index.
*
* @param catalog the catalog column's value filter.
* @return an array of ErrorLogBean
* @throws RuntimeDaoException
*/
public ErrorLogBean[] loadByIndexCatalog(String catalog)throws RuntimeDaoException;
/**
* Retrieves a list of ErrorLogBean using the catalog index.
*
* @param catalog the catalog column's value filter.
* @return a list of ErrorLogBean
* @throws RuntimeDaoException
*/
public java.util.List loadByIndexCatalogAsList(String catalog)throws RuntimeDaoException;
/**
* Deletes rows using the catalog index.
*
* @param catalog the catalog column's value filter.
* @return the number of deleted objects
* @throws RuntimeDaoException
*/
public int deleteByIndexCatalog(String catalog)throws RuntimeDaoException;
/**
* Retrieves an array of ErrorLogBean using the device_id index.
*
* @param deviceId the device_id column's value filter.
* @return an array of ErrorLogBean
* @throws RuntimeDaoException
*/
public ErrorLogBean[] loadByIndexDeviceId(Integer deviceId)throws RuntimeDaoException;
/**
* Retrieves a list of ErrorLogBean using the device_id index.
*
* @param deviceId the device_id column's value filter.
* @return a list of ErrorLogBean
* @throws RuntimeDaoException
*/
public java.util.List loadByIndexDeviceIdAsList(Integer deviceId)throws RuntimeDaoException;
/**
* Deletes rows using the device_id index.
*
* @param deviceId the device_id column's value filter.
* @return the number of deleted objects
* @throws RuntimeDaoException
*/
public int deleteByIndexDeviceId(Integer deviceId)throws RuntimeDaoException;
/**
* Retrieves an array of ErrorLogBean using the person_id index.
*
* @param personId the person_id column's value filter.
* @return an array of ErrorLogBean
* @throws RuntimeDaoException
*/
public ErrorLogBean[] loadByIndexPersonId(Integer personId)throws RuntimeDaoException;
/**
* Retrieves a list of ErrorLogBean using the person_id index.
*
* @param personId the person_id column's value filter.
* @return a list of ErrorLogBean
* @throws RuntimeDaoException
*/
public java.util.List loadByIndexPersonIdAsList(Integer personId)throws RuntimeDaoException;
/**
* Deletes rows using the person_id index.
*
* @param personId the person_id column's value filter.
* @return the number of deleted objects
* @throws RuntimeDaoException
*/
public int deleteByIndexPersonId(Integer personId)throws RuntimeDaoException;
//45
/**
* return a primary key list from {@link ErrorLogBean} array
* @param beans
* @return primary key list
*/
public java.util.List toPrimaryKeyList(ErrorLogBean... beans);
//46
/**
* return a primary key list from {@link ErrorLogBean} collection
* @param beans
* @return primary key list
*/
public java.util.List toPrimaryKeyList(java.util.Collection beans);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy