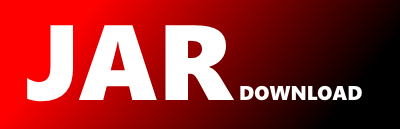
net.gdface.facelog.db.IFeatureManager Maven / Gradle / Ivy
// ______________________________________________________
// Generated by sql2java - https://github.com/10km/sql2java
// JDBC driver used at code generation time: com.mysql.jdbc.Driver
// template: manager.interface.java.vm
// ______________________________________________________
package net.gdface.facelog.db;
import gu.sql2java.TableManager;
import gu.sql2java.exception.ObjectRetrievalException;
import gu.sql2java.exception.RuntimeDaoException;
/**
* Interface to handle database calls (save, load, count, etc...) for the fl_feature table.
* Remarks: 用于验证身份的人脸特征数据表
* @author guyadong
*/
public interface IFeatureManager extends TableManager
{
//////////////////////////////////////
// PRIMARY KEY METHODS
//////////////////////////////////////
//1
/**
* Loads a {@link FeatureBean} from the fl_feature using primary key fields.
*
* @param md5 String - PK# 1
* @return a unique FeatureBean or {@code null} if not found
* @throws RuntimeDaoException
*/
public FeatureBean loadByPrimaryKey(String md5)throws RuntimeDaoException;
//1.1
/**
* Loads a {@link FeatureBean} from the fl_feature using primary key fields.
*
* @param md5 String - PK# 1
* @return a unique FeatureBean
* @throws ObjectRetrievalException if not found
* @throws RuntimeDaoException
*/
public FeatureBean loadByPrimaryKeyChecked(String md5) throws RuntimeDaoException,ObjectRetrievalException;
//1.4
/**
* check if contains row with primary key fields.
* @param md5 String - PK# 1
* @return true if this fl_feature contains row with primary key fields.
* @throws RuntimeDaoException
*/
public boolean existsPrimaryKey(String md5)throws RuntimeDaoException;
//1.4.1
/**
* Check duplicated row by primary keys,if row exists throw exception
* @param md5 primary keys
* @return md5
* @throws RuntimeDaoException
* @throws ObjectRetrievalException
*/
public String checkDuplicate(String md5)throws RuntimeDaoException,ObjectRetrievalException;
//1.8
/**
* Loads {@link FeatureBean} from the fl_feature using primary key fields.
*
* @param keys primary keys array
* @return list of FeatureBean
* @throws RuntimeDaoException
*/
public java.util.List loadByPrimaryKey(String... keys)throws RuntimeDaoException;
//1.9
/**
* Loads {@link FeatureBean} from the fl_feature using primary key fields.
*
* @param keys primary keys collection
* @return list of FeatureBean
* @throws RuntimeDaoException
*/
public java.util.List loadByPrimaryKey(java.util.Collection keys)throws RuntimeDaoException;
//2
/**
* Delete row according to its primary keys.
* all keys must not be null
*
* @param md5 String - PK# 1
* @return the number of deleted rows
* @throws RuntimeDaoException
*/
public int deleteByPrimaryKey(String md5)throws RuntimeDaoException;
//2.2
/**
* Delete rows according to primary key.
*
* @param keys primary keys array
* @return the number of deleted rows
* @throws RuntimeDaoException
* @see #delete(gu.sql2java.BaseBean)
*/
public int deleteByPrimaryKey(String... keys)throws RuntimeDaoException;
//2.3
/**
* Delete rows according to primary key.
*
* @param keys primary keys collection
* @return the number of deleted rows
* @throws RuntimeDaoException
* @see #delete(gu.sql2java.BaseBean)
*/
public int deleteByPrimaryKey(java.util.Collection keys)throws RuntimeDaoException;
//////////////////////////////////////
// GET/SET IMPORTED KEY BEAN METHOD
//////////////////////////////////////
//3.1 GET IMPORTED
/**
* Retrieves the {@link FaceBean} object from the fl_face.feature_md5 field.
* FK_NAME : fl_face_ibfk_2
* @param bean the {@link FeatureBean}
* @return the associated {@link FaceBean} beans or {@code null} if {@code bean} is {@code null}
* @throws RuntimeDaoException
*/
public FaceBean[] getFaceBeansByFeatureMd5(FeatureBean bean)throws RuntimeDaoException;
//3.1.2 GET IMPORTED
/**
* Retrieves the {@link FaceBean} object from the fl_face.feature_md5 field.
* FK_NAME : fl_face_ibfk_2
* @param md5OfFeature String - PK# 1
* @return the associated {@link FaceBean} beans or {@code null} if {@code bean} is {@code null}
* @throws RuntimeDaoException
*/
public FaceBean[] getFaceBeansByFeatureMd5(String md5OfFeature)throws RuntimeDaoException;
//3.2 GET IMPORTED
/**
* see also #getFaceBeansByFeatureMd5AsList(FeatureBean,int,int)
* @param bean
* @return import bean list
* @throws RuntimeDaoException
*/
public java.util.List getFaceBeansByFeatureMd5AsList(FeatureBean bean)throws RuntimeDaoException;
//3.2.2 GET IMPORTED
/**
* Retrieves the {@link FaceBean} object from fl_face.feature_md5 field.
* FK_NAME:fl_face_ibfk_2
* @param md5OfFeature String - PK# 1
* @return the associated {@link FaceBean} beans
* @throws RuntimeDaoException
*/
public java.util.List getFaceBeansByFeatureMd5AsList(String md5OfFeature)throws RuntimeDaoException;
//3.2.3 DELETE IMPORTED
/**
* delete the associated {@link FaceBean} objects from fl_face.feature_md5 field.
* FK_NAME:fl_face_ibfk_2
* @param md5OfFeature String - PK# 1
* @return the number of deleted rows
* @throws RuntimeDaoException
*/
public int deleteFaceBeansByFeatureMd5(String md5OfFeature)throws RuntimeDaoException;
//3.2.4 GET IMPORTED
/**
* Retrieves the {@link FaceBean} object from fl_face.feature_md5 field.
* FK_NAME:fl_face_ibfk_2
* @param bean the {@link FeatureBean}
* @param startRow the start row to be used (first row = 1, last row=-1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @return the associated {@link FaceBean} beans or empty list if {@code bean} is {@code null}
* @throws RuntimeDaoException
*/
public java.util.List getFaceBeansByFeatureMd5AsList(FeatureBean bean,int startRow,int numRows)throws RuntimeDaoException;
//3.3 SET IMPORTED
/**
* set the {@link FaceBean} object array associate to FeatureBean by the fl_face.feature_md5 field.
* FK_NAME : fl_face_ibfk_2
* @param bean the referenced {@link FeatureBean}
* @param importedBeans imported beans from fl_face
* @return importedBeans always
* @see IFaceManager#setReferencedByFeatureMd5(FaceBean, FeatureBean)
* @throws RuntimeDaoException
*/
public FaceBean[] setFaceBeansByFeatureMd5(FeatureBean bean , FaceBean[] importedBeans)throws RuntimeDaoException;
//3.4 SET IMPORTED
/**
* set the {@link FaceBean} object java.util.Collection associate to FeatureBean by the fl_face.feature_md5 field.
* FK_NAME:fl_face_ibfk_2
* @param bean the referenced {@link FeatureBean}
* @param importedBeans imported beans from fl_face
* @return importedBeans always
* @see IFaceManager#setReferencedByFeatureMd5(FaceBean, FeatureBean)
* @throws RuntimeDaoException
*/
public > C setFaceBeansByFeatureMd5(FeatureBean bean , C importedBeans)throws RuntimeDaoException;
//3.1 GET IMPORTED
/**
* Retrieves the {@link LogBean} object from the fl_log.verify_feature field.
* FK_NAME : fl_log_ibfk_3
* @param bean the {@link FeatureBean}
* @return the associated {@link LogBean} beans or {@code null} if {@code bean} is {@code null}
* @throws RuntimeDaoException
*/
public LogBean[] getLogBeansByVerifyFeature(FeatureBean bean)throws RuntimeDaoException;
//3.1.2 GET IMPORTED
/**
* Retrieves the {@link LogBean} object from the fl_log.verify_feature field.
* FK_NAME : fl_log_ibfk_3
* @param md5OfFeature String - PK# 1
* @return the associated {@link LogBean} beans or {@code null} if {@code bean} is {@code null}
* @throws RuntimeDaoException
*/
public LogBean[] getLogBeansByVerifyFeature(String md5OfFeature)throws RuntimeDaoException;
//3.2 GET IMPORTED
/**
* see also #getLogBeansByVerifyFeatureAsList(FeatureBean,int,int)
* @param bean
* @return import bean list
* @throws RuntimeDaoException
*/
public java.util.List getLogBeansByVerifyFeatureAsList(FeatureBean bean)throws RuntimeDaoException;
//3.2.2 GET IMPORTED
/**
* Retrieves the {@link LogBean} object from fl_log.verify_feature field.
* FK_NAME:fl_log_ibfk_3
* @param md5OfFeature String - PK# 1
* @return the associated {@link LogBean} beans
* @throws RuntimeDaoException
*/
public java.util.List getLogBeansByVerifyFeatureAsList(String md5OfFeature)throws RuntimeDaoException;
//3.2.3 DELETE IMPORTED
/**
* delete the associated {@link LogBean} objects from fl_log.verify_feature field.
* FK_NAME:fl_log_ibfk_3
* @param md5OfFeature String - PK# 1
* @return the number of deleted rows
* @throws RuntimeDaoException
*/
public int deleteLogBeansByVerifyFeature(String md5OfFeature)throws RuntimeDaoException;
//3.2.4 GET IMPORTED
/**
* Retrieves the {@link LogBean} object from fl_log.verify_feature field.
* FK_NAME:fl_log_ibfk_3
* @param bean the {@link FeatureBean}
* @param startRow the start row to be used (first row = 1, last row=-1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @return the associated {@link LogBean} beans or empty list if {@code bean} is {@code null}
* @throws RuntimeDaoException
*/
public java.util.List getLogBeansByVerifyFeatureAsList(FeatureBean bean,int startRow,int numRows)throws RuntimeDaoException;
//3.3 SET IMPORTED
/**
* set the {@link LogBean} object array associate to FeatureBean by the fl_log.verify_feature field.
* FK_NAME : fl_log_ibfk_3
* @param bean the referenced {@link FeatureBean}
* @param importedBeans imported beans from fl_log
* @return importedBeans always
* @see ILogManager#setReferencedByVerifyFeature(LogBean, FeatureBean)
* @throws RuntimeDaoException
*/
public LogBean[] setLogBeansByVerifyFeature(FeatureBean bean , LogBean[] importedBeans)throws RuntimeDaoException;
//3.4 SET IMPORTED
/**
* set the {@link LogBean} object java.util.Collection associate to FeatureBean by the fl_log.verify_feature field.
* FK_NAME:fl_log_ibfk_3
* @param bean the referenced {@link FeatureBean}
* @param importedBeans imported beans from fl_log
* @return importedBeans always
* @see ILogManager#setReferencedByVerifyFeature(LogBean, FeatureBean)
* @throws RuntimeDaoException
*/
public > C setLogBeansByVerifyFeature(FeatureBean bean , C importedBeans)throws RuntimeDaoException;
//3.5 SYNC SAVE
/**
* Save the FeatureBean bean and referenced beans and imported beans into the database.
*
* @param bean the {@link FeatureBean} bean to be saved
* @param refPersonByPersonId the {@link PersonBean} bean referenced by {@link FeatureBean}
* @param impFaceByFeatureMd5 the {@link FaceBean} bean refer to {@link FeatureBean}
* @param impLogByVerifyFeature the {@link LogBean} bean refer to {@link FeatureBean}
* @return the inserted or updated {@link FeatureBean} bean
* @throws RuntimeDaoException
*/
public FeatureBean save(FeatureBean bean
, PersonBean refPersonByPersonId
, FaceBean[] impFaceByFeatureMd5 , LogBean[] impLogByVerifyFeature )throws RuntimeDaoException;
//3.6 SYNC SAVE AS TRANSACTION
/**
* Transaction version for sync save
* see also {@link #save(FeatureBean , PersonBean , FaceBean[] , LogBean[] )}
* @param bean the {@link FeatureBean} bean to be saved
* @param refPersonByPersonId the {@link PersonBean} bean referenced by {@link FeatureBean}
* @param impFaceByFeatureMd5 the {@link FaceBean} bean refer to {@link FeatureBean}
* @param impLogByVerifyFeature the {@link LogBean} bean refer to {@link FeatureBean}
* @return the inserted or updated {@link FeatureBean} bean
* @throws RuntimeDaoException
*/
public FeatureBean saveAsTransaction(final FeatureBean bean
,final PersonBean refPersonByPersonId
,final FaceBean[] impFaceByFeatureMd5 ,final LogBean[] impLogByVerifyFeature )throws RuntimeDaoException;
//3.7 SYNC SAVE
/**
* Save the FeatureBean bean and referenced beans and imported beans into the database.
*
* @param bean the {@link FeatureBean} bean to be saved
* @param refPersonByPersonId the {@link PersonBean} bean referenced by {@link FeatureBean}
* @param impFaceByFeatureMd5 the {@link FaceBean} bean refer to {@link FeatureBean}
* @param impLogByVerifyFeature the {@link LogBean} bean refer to {@link FeatureBean}
* @return the inserted or updated {@link FeatureBean} bean
* @throws RuntimeDaoException
*/
public FeatureBean save(FeatureBean bean
, PersonBean refPersonByPersonId
, java.util.Collection impFaceByFeatureMd5 , java.util.Collection impLogByVerifyFeature )throws RuntimeDaoException;
//3.8 SYNC SAVE AS TRANSACTION
/**
* Transaction version for sync save
* see also {@link #save(FeatureBean , PersonBean , java.util.Collection , java.util.Collection )}
* @param bean the {@link FeatureBean} bean to be saved
* @param refPersonByPersonId the {@link PersonBean} bean referenced by {@link FeatureBean}
* @param impFaceByFeatureMd5 the {@link FaceBean} bean refer to {@link FeatureBean}
* @param impLogByVerifyFeature the {@link LogBean} bean refer to {@link FeatureBean}
* @return the inserted or updated {@link FeatureBean} bean
* @throws RuntimeDaoException
*/
public FeatureBean saveAsTransaction(final FeatureBean bean
,final PersonBean refPersonByPersonId
,final java.util.Collection impFaceByFeatureMd5 ,final java.util.Collection impLogByVerifyFeature )throws RuntimeDaoException;
//////////////////////////////////////
// GET/SET FOREIGN KEY BEAN METHOD
//////////////////////////////////////
//5.1 GET REFERENCED VALUE
/**
* Retrieves the {@link PersonBean} object referenced by {@link FeatureBean#getPersonId}() field.
* FK_NAME : fl_feature_ibfk_1
* @param bean the {@link FeatureBean}
* @return the associated {@link PersonBean} bean or {@code null} if {@code bean} is {@code null}
* @throws RuntimeDaoException
*/
public PersonBean getReferencedByPersonId(FeatureBean bean)throws RuntimeDaoException;
//5.2 SET REFERENCED
/**
* Associates the {@link FeatureBean} object to the {@link PersonBean} object by {@link FeatureBean#getPersonId}() field.
*
* @param bean the {@link FeatureBean} object to use
* @param beanToSet the {@link PersonBean} object to associate to the {@link FeatureBean}
* @return always beanToSet saved
* @throws RuntimeDaoException
*/
public PersonBean setReferencedByPersonId(FeatureBean bean, PersonBean beanToSet)throws RuntimeDaoException;
//_____________________________________________________________________
//
// USING INDICES
//_____________________________________________________________________
/**
* Retrieves an array of FeatureBean using the feature_version index.
*
* @param version the version column's value filter.
* @return an array of FeatureBean
* @throws RuntimeDaoException
*/
public FeatureBean[] loadByIndexVersion(String version)throws RuntimeDaoException;
/**
* Retrieves a list of FeatureBean using the feature_version index.
*
* @param version the version column's value filter.
* @return a list of FeatureBean
* @throws RuntimeDaoException
*/
public java.util.List loadByIndexVersionAsList(String version)throws RuntimeDaoException;
/**
* Deletes rows using the feature_version index.
*
* @param version the version column's value filter.
* @return the number of deleted objects
* @throws RuntimeDaoException
*/
public int deleteByIndexVersion(String version)throws RuntimeDaoException;
/**
* Retrieves an array of FeatureBean using the person_id index.
*
* @param personId the person_id column's value filter.
* @return an array of FeatureBean
* @throws RuntimeDaoException
*/
public FeatureBean[] loadByIndexPersonId(Integer personId)throws RuntimeDaoException;
/**
* Retrieves a list of FeatureBean using the person_id index.
*
* @param personId the person_id column's value filter.
* @return a list of FeatureBean
* @throws RuntimeDaoException
*/
public java.util.List loadByIndexPersonIdAsList(Integer personId)throws RuntimeDaoException;
/**
* Deletes rows using the person_id index.
*
* @param personId the person_id column's value filter.
* @return the number of deleted objects
* @throws RuntimeDaoException
*/
public int deleteByIndexPersonId(Integer personId)throws RuntimeDaoException;
//45
/**
* return a primary key list from {@link FeatureBean} array
* @param beans
* @return primary key list
*/
public java.util.List toPrimaryKeyList(FeatureBean... beans);
//46
/**
* return a primary key list from {@link FeatureBean} collection
* @param beans
* @return primary key list
*/
public java.util.List toPrimaryKeyList(java.util.Collection beans);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy