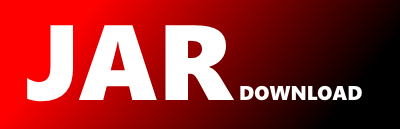
net.gdface.facelog.db.ImageBean Maven / Gradle / Ivy
// ______________________________________________________
// Generated by sql2java - https://github.com/10km/sql2java
// JDBC driver used at code generation time: com.mysql.jdbc.Driver
// template: bean.java.vm
// ______________________________________________________
package net.gdface.facelog.db;
import java.io.Serializable;
import java.util.Objects;
import gu.sql2java.BaseRow;
import com.facebook.swift.codec.ThriftStruct;
import com.facebook.swift.codec.ThriftField;
import com.facebook.swift.codec.ThriftField.Requiredness;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonIgnore;
import net.gdface.annotation.CodegenLength;
import net.gdface.annotation.CodegenRequired;
import net.gdface.annotation.CodegenDefaultvalue;
import net.gdface.annotation.CodegenInvalidValue;
/**
* ImageBean is a mapping of fl_image Table.
*
Meta Data Information (in progress):
*
* - comments: 图像信息存储表,用于存储系统中所有用到的图像数据,表中只包含图像基本信息,图像二进制源数据存在在fl_store中(md5对应)
*
* @author guyadong
*/
@ThriftStruct
@ApiModel(description="图像信息存储表,用于存储系统中所有用到的图像数据,表中只包含图像基本信息,图像二进制源数据存在在fl_store中(md5对应)")
public final class ImageBean extends BaseRow
implements Serializable,Constant
{
private static final long serialVersionUID = -5491214114261088424L;
/** comments:主键,图像md5检验码,同时也是从 fl_store 获取图像数据的key */
@ApiModelProperty(value = "主键,图像md5检验码,同时也是从 fl_store 获取图像数据的key" ,required=true ,dataType="String")
@CodegenLength(value=32,prealloc=true)@CodegenRequired@CodegenInvalidValue
private String md5;
/** comments:图像格式 */
@ApiModelProperty(value = "图像格式" ,dataType="String")
@CodegenLength(max=32,prealloc=true)@CodegenInvalidValue
private String format;
/** comments:图像宽度 */
@ApiModelProperty(value = "图像宽度" ,required=true ,dataType="Integer")
@CodegenRequired@CodegenInvalidValue("-1")
private Integer width;
/** comments:图像高度 */
@ApiModelProperty(value = "图像高度" ,required=true ,dataType="Integer")
@CodegenRequired@CodegenInvalidValue("-1")
private Integer height;
/** comments:通道数 */
@ApiModelProperty(value = "通道数" ,dataType="Integer")
@CodegenDefaultvalue("0")@CodegenInvalidValue("-1")
private Integer depth;
/** comments:图像中的人脸数目 */
@ApiModelProperty(value = "图像中的人脸数目" ,dataType="Integer")
@CodegenDefaultvalue("0")@CodegenInvalidValue("-1")
private Integer faceNum;
/** comments:缩略图md5,图像数据存储在 fl_imae_store(md5) */
@ApiModelProperty(value = "缩略图md5,图像数据存储在 fl_imae_store(md5)" ,dataType="String")
@CodegenLength(value=32,prealloc=true)@CodegenInvalidValue
private String thumbMd5;
/** comments:外键,图像来源设备 */
@ApiModelProperty(value = "外键,图像来源设备" ,dataType="Integer")
@CodegenInvalidValue("0")
private Integer deviceId;
/** columns modified flag */
@ApiModelProperty(value="columns modified flag",dataType="int",required=true)
private int modified;
/** columns initialized flag */
@ApiModelProperty(value="columns initialized flag",dataType="int",required=true)
private int initialized;
/** new record flag */
@ApiModelProperty(value="new record flag",dataType="boolean",required=true)
private boolean isNew;
@ThriftField(value=1,name="_new",requiredness=Requiredness.REQUIRED)
@Override
public boolean isNew()
{
return this.isNew;
}
/**
* Specifies to the object if it has been set as new.
*
* @param isNew the boolean value to be assigned to the isNew field
*/
@ThriftField()
@Override
public void setNew(boolean isNew)
{
this.isNew = isNew;
}
/**
* @return the modified status of columns
*/
@ThriftField(value=2,requiredness=Requiredness.REQUIRED)
@Override
public int getModified(){
return modified;
}
/**
* @param modified the modified status bit to be assigned to {@link #modified}
*/
@ThriftField()
@Override
public void setModified(int modified){
this.modified = modified;
}
/**
* @return the initialized status of columns
*/
@ThriftField(value=3,requiredness=Requiredness.REQUIRED)
@Override
public int getInitialized(){
return initialized;
}
/**
* @param initialized the initialized status bit to be assigned to {@link #initialized}
*/
@ThriftField()
@Override
public void setInitialized(int initialized){
this.initialized = initialized;
}
public ImageBean(){
reset();
}
/**
* construct a new instance filled with primary keys
* @param md5 PK# 1
*/
public ImageBean(String md5){
setMd5(md5);
}
/**
* Getter method for {@link #md5}.
* PRIMARY KEY.
* Meta Data Information (in progress):
*
* - full name: fl_image.md5
* - imported key: fl_face.image_md5
* - imported key: fl_log.image_md5
* - imported key: fl_person.image_md5
* - comments: 主键,图像md5检验码,同时也是从 fl_store 获取图像数据的key
* - NOT NULL
* - column size: 32
* - JDBC type returned by the driver: Types.CHAR
*
*
* @return the value of md5
*/
@ThriftField(value=4)
@JsonProperty("md5")
public String getMd5(){
return md5;
}
/**
* Setter method for {@link #md5}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to md5
*/
@ThriftField(name="md5")
@JsonProperty("md5")
public void setMd5(String newVal)
{
modified |= FL_IMAGE_ID_MD5_MASK;
initialized |= FL_IMAGE_ID_MD5_MASK;
if (Objects.equals(newVal, md5)) {
return;
}
md5 = newVal;
}
/**
* Determines if the md5 has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkMd5Modified()
{
return 0 != (modified & FL_IMAGE_ID_MD5_MASK);
}
/**
* Determines if the md5 has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkMd5Initialized()
{
return 0 != (initialized & FL_IMAGE_ID_MD5_MASK);
}
/**
* Getter method for {@link #format}.
* Meta Data Information (in progress):
*
* - full name: fl_image.format
* - comments: 图像格式
* - column size: 32
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of format
*/
@ThriftField(value=5)
@JsonProperty("format")
public String getFormat(){
return format;
}
/**
* Setter method for {@link #format}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to format
*/
@ThriftField(name="format")
@JsonProperty("format")
public void setFormat(String newVal)
{
modified |= FL_IMAGE_ID_FORMAT_MASK;
initialized |= FL_IMAGE_ID_FORMAT_MASK;
if (Objects.equals(newVal, format)) {
return;
}
format = newVal;
}
/**
* Determines if the format has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkFormatModified()
{
return 0 != (modified & FL_IMAGE_ID_FORMAT_MASK);
}
/**
* Determines if the format has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkFormatInitialized()
{
return 0 != (initialized & FL_IMAGE_ID_FORMAT_MASK);
}
/**
* Getter method for {@link #width}.
* Meta Data Information (in progress):
*
* - full name: fl_image.width
* - comments: 图像宽度
* - NOT NULL
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of width
*/
@ThriftField(value=6)
@JsonProperty("width")
public Integer getWidth(){
return width;
}
/**
* Setter method for {@link #width}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to width
*/
@ThriftField(name="width")
@JsonProperty("width")
public void setWidth(Integer newVal)
{
modified |= FL_IMAGE_ID_WIDTH_MASK;
initialized |= FL_IMAGE_ID_WIDTH_MASK;
if (Objects.equals(newVal, width)) {
return;
}
width = newVal;
}
/**
* Setter method for {@link #width}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to width
*/
@JsonIgnore
public void setWidth(int newVal)
{
setWidth(new Integer(newVal));
}
/**
* Determines if the width has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkWidthModified()
{
return 0 != (modified & FL_IMAGE_ID_WIDTH_MASK);
}
/**
* Determines if the width has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkWidthInitialized()
{
return 0 != (initialized & FL_IMAGE_ID_WIDTH_MASK);
}
/**
* Getter method for {@link #height}.
* Meta Data Information (in progress):
*
* - full name: fl_image.height
* - comments: 图像高度
* - NOT NULL
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of height
*/
@ThriftField(value=7)
@JsonProperty("height")
public Integer getHeight(){
return height;
}
/**
* Setter method for {@link #height}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to height
*/
@ThriftField(name="height")
@JsonProperty("height")
public void setHeight(Integer newVal)
{
modified |= FL_IMAGE_ID_HEIGHT_MASK;
initialized |= FL_IMAGE_ID_HEIGHT_MASK;
if (Objects.equals(newVal, height)) {
return;
}
height = newVal;
}
/**
* Setter method for {@link #height}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to height
*/
@JsonIgnore
public void setHeight(int newVal)
{
setHeight(new Integer(newVal));
}
/**
* Determines if the height has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkHeightModified()
{
return 0 != (modified & FL_IMAGE_ID_HEIGHT_MASK);
}
/**
* Determines if the height has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkHeightInitialized()
{
return 0 != (initialized & FL_IMAGE_ID_HEIGHT_MASK);
}
/**
* Getter method for {@link #depth}.
* Meta Data Information (in progress):
*
* - full name: fl_image.depth
* - comments: 通道数
* - default value: '0'
* - NOT NULL
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of depth
*/
@ThriftField(value=8)
@JsonProperty("depth")
public Integer getDepth(){
return depth;
}
/**
* Setter method for {@link #depth}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to depth
*/
@ThriftField(name="depth")
@JsonProperty("depth")
public void setDepth(Integer newVal)
{
modified |= FL_IMAGE_ID_DEPTH_MASK;
initialized |= FL_IMAGE_ID_DEPTH_MASK;
if (Objects.equals(newVal, depth)) {
return;
}
depth = newVal;
}
/**
* Setter method for {@link #depth}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to depth
*/
@JsonIgnore
public void setDepth(int newVal)
{
setDepth(new Integer(newVal));
}
/**
* Determines if the depth has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkDepthModified()
{
return 0 != (modified & FL_IMAGE_ID_DEPTH_MASK);
}
/**
* Determines if the depth has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkDepthInitialized()
{
return 0 != (initialized & FL_IMAGE_ID_DEPTH_MASK);
}
/**
* Getter method for {@link #faceNum}.
* Meta Data Information (in progress):
*
* - full name: fl_image.face_num
* - comments: 图像中的人脸数目
* - default value: '0'
* - NOT NULL
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of faceNum
*/
@ThriftField(value=9)
@JsonProperty("faceNum")
public Integer getFaceNum(){
return faceNum;
}
/**
* Setter method for {@link #faceNum}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to faceNum
*/
@ThriftField(name="faceNum")
@JsonProperty("faceNum")
public void setFaceNum(Integer newVal)
{
modified |= FL_IMAGE_ID_FACE_NUM_MASK;
initialized |= FL_IMAGE_ID_FACE_NUM_MASK;
if (Objects.equals(newVal, faceNum)) {
return;
}
faceNum = newVal;
}
/**
* Setter method for {@link #faceNum}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to faceNum
*/
@JsonIgnore
public void setFaceNum(int newVal)
{
setFaceNum(new Integer(newVal));
}
/**
* Determines if the faceNum has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkFaceNumModified()
{
return 0 != (modified & FL_IMAGE_ID_FACE_NUM_MASK);
}
/**
* Determines if the faceNum has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkFaceNumInitialized()
{
return 0 != (initialized & FL_IMAGE_ID_FACE_NUM_MASK);
}
/**
* Getter method for {@link #thumbMd5}.
* Meta Data Information (in progress):
*
* - full name: fl_image.thumb_md5
* - comments: 缩略图md5,图像数据存储在 fl_imae_store(md5)
* - column size: 32
* - JDBC type returned by the driver: Types.CHAR
*
*
* @return the value of thumbMd5
*/
@ThriftField(value=10)
@JsonProperty("thumbMd5")
public String getThumbMd5(){
return thumbMd5;
}
/**
* Setter method for {@link #thumbMd5}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to thumbMd5
*/
@ThriftField(name="thumbMd5")
@JsonProperty("thumbMd5")
public void setThumbMd5(String newVal)
{
modified |= FL_IMAGE_ID_THUMB_MD5_MASK;
initialized |= FL_IMAGE_ID_THUMB_MD5_MASK;
if (Objects.equals(newVal, thumbMd5)) {
return;
}
thumbMd5 = newVal;
}
/**
* Determines if the thumbMd5 has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkThumbMd5Modified()
{
return 0 != (modified & FL_IMAGE_ID_THUMB_MD5_MASK);
}
/**
* Determines if the thumbMd5 has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkThumbMd5Initialized()
{
return 0 != (initialized & FL_IMAGE_ID_THUMB_MD5_MASK);
}
/**
* Getter method for {@link #deviceId}.
* Meta Data Information (in progress):
*
* - full name: fl_image.device_id
* - foreign key: fl_device.id
* - comments: 外键,图像来源设备
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of deviceId
*/
@ThriftField(value=11)
@JsonProperty("deviceId")
public Integer getDeviceId(){
return deviceId;
}
/**
* Setter method for {@link #deviceId}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to deviceId
*/
@ThriftField(name="deviceId")
@JsonProperty("deviceId")
public void setDeviceId(Integer newVal)
{
modified |= FL_IMAGE_ID_DEVICE_ID_MASK;
initialized |= FL_IMAGE_ID_DEVICE_ID_MASK;
if (Objects.equals(newVal, deviceId)) {
return;
}
deviceId = newVal;
}
/**
* Setter method for {@link #deviceId}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to deviceId
*/
@JsonIgnore
public void setDeviceId(int newVal)
{
setDeviceId(new Integer(newVal));
}
/**
* Determines if the deviceId has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkDeviceIdModified()
{
return 0 != (modified & FL_IMAGE_ID_DEVICE_ID_MASK);
}
/**
* Determines if the deviceId has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkDeviceIdInitialized()
{
return 0 != (initialized & FL_IMAGE_ID_DEVICE_ID_MASK);
}
@Override
public boolean beModified()
{
return 0 != modified;
}
@Override
public boolean isModified(int columnID){
return columnID>=0 && columnID < metaData.columnCount && 0 != (modified & (1 << columnID));
}
@Override
public boolean isInitialized(int columnID){
return columnID>=0 && columnID < metaData.columnCount && 0 != (initialized & (1 << columnID));
}
@Override
public void resetIsModified()
{
modified = 0;
}
@Override
public void resetPrimaryKeysModified()
{
modified &= (~(FL_IMAGE_ID_MD5_MASK));
}
/**
* Resets columns modification status except primary keys to 'not modified'.
*/
public void resetModifiedExceptPrimaryKeys()
{
modified &= (~(FL_IMAGE_ID_FORMAT_MASK |
FL_IMAGE_ID_WIDTH_MASK |
FL_IMAGE_ID_HEIGHT_MASK |
FL_IMAGE_ID_DEPTH_MASK |
FL_IMAGE_ID_FACE_NUM_MASK |
FL_IMAGE_ID_THUMB_MD5_MASK |
FL_IMAGE_ID_DEVICE_ID_MASK));
}
/** reset all fields to initial value, equal to a new bean */
public void reset(){
this.md5 = null;
this.format = null;
this.width = null;
this.height = null;
/* DEFAULT:'0'*/
this.depth = new Integer(0);
/* DEFAULT:'0'*/
this.faceNum = new Integer(0);
this.thumbMd5 = null;
this.deviceId = null;
this.isNew = true;
this.modified = 0;
this.initialized = (FL_IMAGE_ID_DEPTH_MASK | FL_IMAGE_ID_FACE_NUM_MASK);
}
@Override
public ImageBean clone(){
return (ImageBean) super.clone();
}
public static final Builder builder(){
return new Builder().reset();
}
/**
* a builder for ImageBean,the template instance is thread local variable
* a instance of Builder can be reused.
*/
public static final class Builder{
/** ImageBean instance used for template to create new ImageBean instance. */
static final ThreadLocal TEMPLATE = new ThreadLocal(){
@Override
protected ImageBean initialValue() {
return new ImageBean();
}};
private Builder() {}
/**
* reset the bean as template
* @see ImageBean#reset()
*/
public Builder reset(){
TEMPLATE.get().reset();
return this;
}
/** set a bean as template,must not be {@code null} */
public Builder template(ImageBean bean){
if(null == bean){
throw new NullPointerException();
}
TEMPLATE.set(bean);
return this;
}
/** return a clone instance of {@link #TEMPLATE}*/
public ImageBean build(){
return TEMPLATE.get().clone();
}
/**
* fill the field : fl_image.md5
* @param md5 主键,图像md5检验码,同时也是从 fl_store 获取图像数据的key
* @see ImageBean#getMd5()
* @see ImageBean#setMd5(String)
*/
public Builder md5(String md5){
TEMPLATE.get().setMd5(md5);
return this;
}
/**
* fill the field : fl_image.format
* @param format 图像格式
* @see ImageBean#getFormat()
* @see ImageBean#setFormat(String)
*/
public Builder format(String format){
TEMPLATE.get().setFormat(format);
return this;
}
/**
* fill the field : fl_image.width
* @param width 图像宽度
* @see ImageBean#getWidth()
* @see ImageBean#setWidth(Integer)
*/
public Builder width(Integer width){
TEMPLATE.get().setWidth(width);
return this;
}
/**
* fill the field : fl_image.height
* @param height 图像高度
* @see ImageBean#getHeight()
* @see ImageBean#setHeight(Integer)
*/
public Builder height(Integer height){
TEMPLATE.get().setHeight(height);
return this;
}
/**
* fill the field : fl_image.depth
* @param depth 通道数
* @see ImageBean#getDepth()
* @see ImageBean#setDepth(Integer)
*/
public Builder depth(Integer depth){
TEMPLATE.get().setDepth(depth);
return this;
}
/**
* fill the field : fl_image.face_num
* @param faceNum 图像中的人脸数目
* @see ImageBean#getFaceNum()
* @see ImageBean#setFaceNum(Integer)
*/
public Builder faceNum(Integer faceNum){
TEMPLATE.get().setFaceNum(faceNum);
return this;
}
/**
* fill the field : fl_image.thumb_md5
* @param thumbMd5 缩略图md5,图像数据存储在 fl_imae_store(md5)
* @see ImageBean#getThumbMd5()
* @see ImageBean#setThumbMd5(String)
*/
public Builder thumbMd5(String thumbMd5){
TEMPLATE.get().setThumbMd5(thumbMd5);
return this;
}
/**
* fill the field : fl_image.device_id
* @param deviceId 外键,图像来源设备
* @see ImageBean#getDeviceId()
* @see ImageBean#setDeviceId(Integer)
*/
public Builder deviceId(Integer deviceId){
TEMPLATE.get().setDeviceId(deviceId);
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy