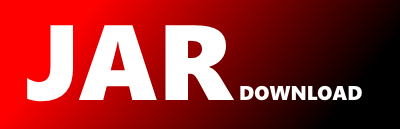
net.gdface.facelog.db.LogBean Maven / Gradle / Ivy
// ______________________________________________________
// Generated by sql2java - https://github.com/10km/sql2java
// JDBC driver used at code generation time: com.mysql.jdbc.Driver
// template: bean.java.vm
// ______________________________________________________
package net.gdface.facelog.db;
import java.io.Serializable;
import java.util.Objects;
import gu.sql2java.BaseRow;
import com.facebook.swift.codec.ThriftStruct;
import com.facebook.swift.codec.ThriftField;
import com.facebook.swift.codec.ThriftField.Requiredness;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonIgnore;
import net.gdface.annotation.CodegenLength;
import net.gdface.annotation.CodegenRequired;
import net.gdface.annotation.CodegenDefaultvalue;
import net.gdface.annotation.CodegenInvalidValue;
/**
* LogBean is a mapping of fl_log Table.
*
Meta Data Information (in progress):
*
* - comments: 人脸验证日志,记录所有通过验证的人员
*
* @author guyadong
*/
@ThriftStruct
@ApiModel(description="人脸验证日志,记录所有通过验证的人员")
public final class LogBean extends BaseRow
implements Serializable,Constant
{
private static final long serialVersionUID = 46995545005652737L;
/** comments:日志id */
@ApiModelProperty(value = "日志id" ,required=true ,dataType="Integer")
@CodegenRequired@CodegenInvalidValue("0")
private Integer id;
/** comments:外键,用户id */
@ApiModelProperty(value = "外键,用户id" ,required=true ,dataType="Integer")
@CodegenRequired@CodegenInvalidValue("0")
private Integer personId;
/** comments:外键,日志来源设备id */
@ApiModelProperty(value = "外键,日志来源设备id" ,dataType="Integer")
@CodegenInvalidValue("0")
private Integer deviceId;
/** comments:外键,用于验证身份的人脸特征数据MD5 id */
@ApiModelProperty(value = "外键,用于验证身份的人脸特征数据MD5 id" ,dataType="String")
@CodegenLength(value=32,prealloc=true)@CodegenInvalidValue
private String verifyFeature;
/** comments:外键,现场采集人脸图像id */
@ApiModelProperty(value = "外键,现场采集人脸图像id" ,dataType="String")
@CodegenLength(value=32,prealloc=true)@CodegenInvalidValue
private String imageMd5;
/** comments:外键,现场采集的人脸信息记录id */
@ApiModelProperty(value = "外键,现场采集的人脸信息记录id" ,dataType="Integer")
@CodegenInvalidValue("0")
private Integer compareFace;
/** comments:验证状态,NULL,0:允许通过,其他:拒绝 */
@ApiModelProperty(value = "验证状态,NULL,0:允许通过,其他:拒绝" ,dataType="Integer")
@CodegenInvalidValue("-1")
private Integer verifyStatus;
/** comments:验证相似度 */
@ApiModelProperty(value = "验证相似度" ,dataType="Double")
@CodegenInvalidValue("-1")
private Double similarty;
/** comments:通行方向,NULL,0:入口,1:出口,默认0 */
@ApiModelProperty(value = "通行方向,NULL,0:入口,1:出口,默认0" ,dataType="Integer")
@CodegenInvalidValue("-1")
private Integer direction;
/** comments:体温(摄氏) */
@ApiModelProperty(value = "体温(摄氏)" ,dataType="Double")
@CodegenInvalidValue("-1")
private Double temperature;
/** comments:JSON格式的扩展字段(最大64KB),用于定义附加验证信息 */
@ApiModelProperty(value = "JSON格式的扩展字段(最大64KB),用于定义附加验证信息" ,dataType="String")
@CodegenLength(max=65535)@CodegenInvalidValue
private String props;
/** comments:身份认证方式,NULL,0:人脸,1:指纹,2:密码,3:刷卡 */
@ApiModelProperty(value = "身份认证方式,NULL,0:人脸,1:指纹,2:密码,3:刷卡" ,dataType="Integer")
@CodegenDefaultvalue("0")@CodegenInvalidValue("-1")
private Integer verifyType;
/** comments:验证时间(可能由前端设备提供时间) */
@ApiModelProperty(value = "验证时间(可能由前端设备提供时间)" ,dataType="Date")
@CodegenInvalidValue("0")
private java.util.Date verifyTime;
@ApiModelProperty(value = "create_time" ,dataType="Date")
@CodegenInvalidValue("0")
private java.util.Date createTime;
/** columns modified flag */
@ApiModelProperty(value="columns modified flag",dataType="int",required=true)
private int modified;
/** columns initialized flag */
@ApiModelProperty(value="columns initialized flag",dataType="int",required=true)
private int initialized;
/** new record flag */
@ApiModelProperty(value="new record flag",dataType="boolean",required=true)
private boolean isNew;
@ThriftField(value=1,name="_new",requiredness=Requiredness.REQUIRED)
@Override
public boolean isNew()
{
return this.isNew;
}
/**
* Specifies to the object if it has been set as new.
*
* @param isNew the boolean value to be assigned to the isNew field
*/
@ThriftField()
@Override
public void setNew(boolean isNew)
{
this.isNew = isNew;
}
/**
* @return the modified status of columns
*/
@ThriftField(value=2,requiredness=Requiredness.REQUIRED)
@Override
public int getModified(){
return modified;
}
/**
* @param modified the modified status bit to be assigned to {@link #modified}
*/
@ThriftField()
@Override
public void setModified(int modified){
this.modified = modified;
}
/**
* @return the initialized status of columns
*/
@ThriftField(value=3,requiredness=Requiredness.REQUIRED)
@Override
public int getInitialized(){
return initialized;
}
/**
* @param initialized the initialized status bit to be assigned to {@link #initialized}
*/
@ThriftField()
@Override
public void setInitialized(int initialized){
this.initialized = initialized;
}
public LogBean(){
reset();
}
/**
* construct a new instance filled with primary keys
* @param id PK# 1
*/
public LogBean(Integer id){
setId(id);
}
/**
* Getter method for {@link #id}.
* PRIMARY KEY.
* Meta Data Information (in progress):
*
* - full name: fl_log.id
* - comments: 日志id
* - AUTO_INCREMENT
* - NOT NULL
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of id
*/
@ThriftField(value=4)
@JsonProperty("id")
public Integer getId(){
return id;
}
/**
* Setter method for {@link #id}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to id
*/
@ThriftField(name="id")
@JsonProperty("id")
public void setId(Integer newVal)
{
modified |= FL_LOG_ID_ID_MASK;
initialized |= FL_LOG_ID_ID_MASK;
if (Objects.equals(newVal, id)) {
return;
}
id = newVal;
}
/**
* Setter method for {@link #id}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to id
*/
@JsonIgnore
public void setId(int newVal)
{
setId(new Integer(newVal));
}
/**
* Determines if the id has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkIdModified()
{
return 0 != (modified & FL_LOG_ID_ID_MASK);
}
/**
* Determines if the id has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkIdInitialized()
{
return 0 != (initialized & FL_LOG_ID_ID_MASK);
}
/**
* Getter method for {@link #personId}.
* Meta Data Information (in progress):
*
* - full name: fl_log.person_id
* - foreign key: fl_person.id
* - comments: 外键,用户id
* - NOT NULL
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of personId
*/
@ThriftField(value=5)
@JsonProperty("personId")
public Integer getPersonId(){
return personId;
}
/**
* Setter method for {@link #personId}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to personId
*/
@ThriftField(name="personId")
@JsonProperty("personId")
public void setPersonId(Integer newVal)
{
modified |= FL_LOG_ID_PERSON_ID_MASK;
initialized |= FL_LOG_ID_PERSON_ID_MASK;
if (Objects.equals(newVal, personId)) {
return;
}
personId = newVal;
}
/**
* Setter method for {@link #personId}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to personId
*/
@JsonIgnore
public void setPersonId(int newVal)
{
setPersonId(new Integer(newVal));
}
/**
* Determines if the personId has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkPersonIdModified()
{
return 0 != (modified & FL_LOG_ID_PERSON_ID_MASK);
}
/**
* Determines if the personId has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkPersonIdInitialized()
{
return 0 != (initialized & FL_LOG_ID_PERSON_ID_MASK);
}
/**
* Getter method for {@link #deviceId}.
* Meta Data Information (in progress):
*
* - full name: fl_log.device_id
* - foreign key: fl_device.id
* - comments: 外键,日志来源设备id
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of deviceId
*/
@ThriftField(value=6)
@JsonProperty("deviceId")
public Integer getDeviceId(){
return deviceId;
}
/**
* Setter method for {@link #deviceId}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to deviceId
*/
@ThriftField(name="deviceId")
@JsonProperty("deviceId")
public void setDeviceId(Integer newVal)
{
modified |= FL_LOG_ID_DEVICE_ID_MASK;
initialized |= FL_LOG_ID_DEVICE_ID_MASK;
if (Objects.equals(newVal, deviceId)) {
return;
}
deviceId = newVal;
}
/**
* Setter method for {@link #deviceId}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to deviceId
*/
@JsonIgnore
public void setDeviceId(int newVal)
{
setDeviceId(new Integer(newVal));
}
/**
* Determines if the deviceId has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkDeviceIdModified()
{
return 0 != (modified & FL_LOG_ID_DEVICE_ID_MASK);
}
/**
* Determines if the deviceId has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkDeviceIdInitialized()
{
return 0 != (initialized & FL_LOG_ID_DEVICE_ID_MASK);
}
/**
* Getter method for {@link #verifyFeature}.
* Meta Data Information (in progress):
*
* - full name: fl_log.verify_feature
* - foreign key: fl_feature.md5
* - comments: 外键,用于验证身份的人脸特征数据MD5 id
* - column size: 32
* - JDBC type returned by the driver: Types.CHAR
*
*
* @return the value of verifyFeature
*/
@ThriftField(value=7)
@JsonProperty("verifyFeature")
public String getVerifyFeature(){
return verifyFeature;
}
/**
* Setter method for {@link #verifyFeature}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to verifyFeature
*/
@ThriftField(name="verifyFeature")
@JsonProperty("verifyFeature")
public void setVerifyFeature(String newVal)
{
modified |= FL_LOG_ID_VERIFY_FEATURE_MASK;
initialized |= FL_LOG_ID_VERIFY_FEATURE_MASK;
if (Objects.equals(newVal, verifyFeature)) {
return;
}
verifyFeature = newVal;
}
/**
* Determines if the verifyFeature has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkVerifyFeatureModified()
{
return 0 != (modified & FL_LOG_ID_VERIFY_FEATURE_MASK);
}
/**
* Determines if the verifyFeature has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkVerifyFeatureInitialized()
{
return 0 != (initialized & FL_LOG_ID_VERIFY_FEATURE_MASK);
}
/**
* Getter method for {@link #imageMd5}.
* Meta Data Information (in progress):
*
* - full name: fl_log.image_md5
* - foreign key: fl_image.md5
* - comments: 外键,现场采集人脸图像id
* - column size: 32
* - JDBC type returned by the driver: Types.CHAR
*
*
* @return the value of imageMd5
*/
@ThriftField(value=8)
@JsonProperty("imageMd5")
public String getImageMd5(){
return imageMd5;
}
/**
* Setter method for {@link #imageMd5}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to imageMd5
*/
@ThriftField(name="imageMd5")
@JsonProperty("imageMd5")
public void setImageMd5(String newVal)
{
modified |= FL_LOG_ID_IMAGE_MD5_MASK;
initialized |= FL_LOG_ID_IMAGE_MD5_MASK;
if (Objects.equals(newVal, imageMd5)) {
return;
}
imageMd5 = newVal;
}
/**
* Determines if the imageMd5 has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkImageMd5Modified()
{
return 0 != (modified & FL_LOG_ID_IMAGE_MD5_MASK);
}
/**
* Determines if the imageMd5 has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkImageMd5Initialized()
{
return 0 != (initialized & FL_LOG_ID_IMAGE_MD5_MASK);
}
/**
* Getter method for {@link #compareFace}.
* Meta Data Information (in progress):
*
* - full name: fl_log.compare_face
* - foreign key: fl_face.id
* - comments: 外键,现场采集的人脸信息记录id
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of compareFace
*/
@ThriftField(value=9)
@JsonProperty("compareFace")
public Integer getCompareFace(){
return compareFace;
}
/**
* Setter method for {@link #compareFace}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to compareFace
*/
@ThriftField(name="compareFace")
@JsonProperty("compareFace")
public void setCompareFace(Integer newVal)
{
modified |= FL_LOG_ID_COMPARE_FACE_MASK;
initialized |= FL_LOG_ID_COMPARE_FACE_MASK;
if (Objects.equals(newVal, compareFace)) {
return;
}
compareFace = newVal;
}
/**
* Setter method for {@link #compareFace}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to compareFace
*/
@JsonIgnore
public void setCompareFace(int newVal)
{
setCompareFace(new Integer(newVal));
}
/**
* Determines if the compareFace has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkCompareFaceModified()
{
return 0 != (modified & FL_LOG_ID_COMPARE_FACE_MASK);
}
/**
* Determines if the compareFace has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkCompareFaceInitialized()
{
return 0 != (initialized & FL_LOG_ID_COMPARE_FACE_MASK);
}
/**
* Getter method for {@link #verifyStatus}.
* Meta Data Information (in progress):
*
* - full name: fl_log.verify_status
* - comments: 验证状态,NULL,0:允许通过,其他:拒绝
* - column size: 3
* - JDBC type returned by the driver: Types.TINYINT
*
*
* @return the value of verifyStatus
*/
@ThriftField(value=10)
@JsonProperty("verifyStatus")
public Integer getVerifyStatus(){
return verifyStatus;
}
/**
* Setter method for {@link #verifyStatus}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to verifyStatus
*/
@ThriftField(name="verifyStatus")
@JsonProperty("verifyStatus")
public void setVerifyStatus(Integer newVal)
{
modified |= FL_LOG_ID_VERIFY_STATUS_MASK;
initialized |= FL_LOG_ID_VERIFY_STATUS_MASK;
if (Objects.equals(newVal, verifyStatus)) {
return;
}
verifyStatus = newVal;
}
/**
* Setter method for {@link #verifyStatus}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to verifyStatus
*/
@JsonIgnore
public void setVerifyStatus(int newVal)
{
setVerifyStatus(new Integer(newVal));
}
/**
* Determines if the verifyStatus has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkVerifyStatusModified()
{
return 0 != (modified & FL_LOG_ID_VERIFY_STATUS_MASK);
}
/**
* Determines if the verifyStatus has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkVerifyStatusInitialized()
{
return 0 != (initialized & FL_LOG_ID_VERIFY_STATUS_MASK);
}
/**
* Getter method for {@link #similarty}.
* Meta Data Information (in progress):
*
* - full name: fl_log.similarty
* - comments: 验证相似度
* - column size: 22
* - JDBC type returned by the driver: Types.DOUBLE
*
*
* @return the value of similarty
*/
@ThriftField(value=11)
@JsonProperty("similarty")
public Double getSimilarty(){
return similarty;
}
/**
* Setter method for {@link #similarty}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to similarty
*/
@ThriftField(name="similarty")
@JsonProperty("similarty")
public void setSimilarty(Double newVal)
{
modified |= FL_LOG_ID_SIMILARTY_MASK;
initialized |= FL_LOG_ID_SIMILARTY_MASK;
if (Objects.equals(newVal, similarty)) {
return;
}
similarty = newVal;
}
/**
* Setter method for {@link #similarty}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to similarty
*/
@JsonIgnore
public void setSimilarty(double newVal)
{
setSimilarty(new Double(newVal));
}
/**
* Determines if the similarty has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkSimilartyModified()
{
return 0 != (modified & FL_LOG_ID_SIMILARTY_MASK);
}
/**
* Determines if the similarty has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkSimilartyInitialized()
{
return 0 != (initialized & FL_LOG_ID_SIMILARTY_MASK);
}
/**
* Getter method for {@link #direction}.
* Meta Data Information (in progress):
*
* - full name: fl_log.direction
* - comments: 通行方向,NULL,0:入口,1:出口,默认0
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of direction
*/
@ThriftField(value=12)
@JsonProperty("direction")
public Integer getDirection(){
return direction;
}
/**
* Setter method for {@link #direction}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to direction
*/
@ThriftField(name="direction")
@JsonProperty("direction")
public void setDirection(Integer newVal)
{
modified |= FL_LOG_ID_DIRECTION_MASK;
initialized |= FL_LOG_ID_DIRECTION_MASK;
if (Objects.equals(newVal, direction)) {
return;
}
direction = newVal;
}
/**
* Setter method for {@link #direction}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to direction
*/
@JsonIgnore
public void setDirection(int newVal)
{
setDirection(new Integer(newVal));
}
/**
* Determines if the direction has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkDirectionModified()
{
return 0 != (modified & FL_LOG_ID_DIRECTION_MASK);
}
/**
* Determines if the direction has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkDirectionInitialized()
{
return 0 != (initialized & FL_LOG_ID_DIRECTION_MASK);
}
/**
* Getter method for {@link #temperature}.
* Meta Data Information (in progress):
*
* - full name: fl_log.temperature
* - comments: 体温(摄氏)
* - column size: 4
* - JDBC type returned by the driver: Types.DOUBLE
*
*
* @return the value of temperature
*/
@ThriftField(value=13)
@JsonProperty("temperature")
public Double getTemperature(){
return temperature;
}
/**
* Setter method for {@link #temperature}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to temperature
*/
@ThriftField(name="temperature")
@JsonProperty("temperature")
public void setTemperature(Double newVal)
{
modified |= FL_LOG_ID_TEMPERATURE_MASK;
initialized |= FL_LOG_ID_TEMPERATURE_MASK;
if (Objects.equals(newVal, temperature)) {
return;
}
temperature = newVal;
}
/**
* Setter method for {@link #temperature}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to temperature
*/
@JsonIgnore
public void setTemperature(double newVal)
{
setTemperature(new Double(newVal));
}
/**
* Determines if the temperature has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkTemperatureModified()
{
return 0 != (modified & FL_LOG_ID_TEMPERATURE_MASK);
}
/**
* Determines if the temperature has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkTemperatureInitialized()
{
return 0 != (initialized & FL_LOG_ID_TEMPERATURE_MASK);
}
/**
* Getter method for {@link #props}.
* Meta Data Information (in progress):
*
* - full name: fl_log.props
* - comments: JSON格式的扩展字段(最大64KB),用于定义附加验证信息
* - column size: 65535
* - JDBC type returned by the driver: Types.LONGVARCHAR
*
*
* @return the value of props
*/
@ThriftField(value=14)
@JsonProperty("props")
public String getProps(){
return props;
}
/**
* Setter method for {@link #props}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to props
*/
@ThriftField(name="props")
@JsonProperty("props")
public void setProps(String newVal)
{
modified |= FL_LOG_ID_PROPS_MASK;
initialized |= FL_LOG_ID_PROPS_MASK;
if (Objects.equals(newVal, props)) {
return;
}
props = newVal;
}
/**
* Determines if the props has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkPropsModified()
{
return 0 != (modified & FL_LOG_ID_PROPS_MASK);
}
/**
* Determines if the props has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkPropsInitialized()
{
return 0 != (initialized & FL_LOG_ID_PROPS_MASK);
}
/**
* Getter method for {@link #verifyType}.
* Meta Data Information (in progress):
*
* - full name: fl_log.verify_type
* - comments: 身份认证方式,NULL,0:人脸,1:指纹,2:密码,3:刷卡
* - default value: '0'
* - column size: 3
* - JDBC type returned by the driver: Types.TINYINT
*
*
* @return the value of verifyType
*/
@ThriftField(value=15)
@JsonProperty("verifyType")
public Integer getVerifyType(){
return verifyType;
}
/**
* Setter method for {@link #verifyType}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to verifyType
*/
@ThriftField(name="verifyType")
@JsonProperty("verifyType")
public void setVerifyType(Integer newVal)
{
modified |= FL_LOG_ID_VERIFY_TYPE_MASK;
initialized |= FL_LOG_ID_VERIFY_TYPE_MASK;
if (Objects.equals(newVal, verifyType)) {
return;
}
verifyType = newVal;
}
/**
* Setter method for {@link #verifyType}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to verifyType
*/
@JsonIgnore
public void setVerifyType(int newVal)
{
setVerifyType(new Integer(newVal));
}
/**
* Determines if the verifyType has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkVerifyTypeModified()
{
return 0 != (modified & FL_LOG_ID_VERIFY_TYPE_MASK);
}
/**
* Determines if the verifyType has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkVerifyTypeInitialized()
{
return 0 != (initialized & FL_LOG_ID_VERIFY_TYPE_MASK);
}
/**
* Getter method for {@link #verifyTime}.
* Meta Data Information (in progress):
*
* - full name: fl_log.verify_time
* - comments: 验证时间(可能由前端设备提供时间)
* - default value: 'CURRENT_TIMESTAMP'
* - NOT NULL
* - column size: 19
* - JDBC type returned by the driver: Types.TIMESTAMP
*
*
* @return the value of verifyTime
*/
@JsonIgnore
public java.util.Date getVerifyTime(){
return verifyTime;
}
/**
* use Long to represent date type for thrift:swift support
* @see #getVerifyTime()
*/
@ThriftField(value=16,name="verifyTime")
@JsonProperty("verifyTime")
public Long readVerifyTime(){
return null == verifyTime ? null:verifyTime.getTime();
}
/**
* Setter method for {@link #verifyTime}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to verifyTime
*/
@JsonIgnore
public void setVerifyTime(java.util.Date newVal)
{
modified |= FL_LOG_ID_VERIFY_TIME_MASK;
initialized |= FL_LOG_ID_VERIFY_TIME_MASK;
if (Objects.equals(newVal, verifyTime)) {
return;
}
verifyTime = newVal;
}
/**
* setter for thrift:swift OR jackson support
* without modification for {@link #modified} and {@link #initialized}
* NOTE:DO NOT use the method in your code
*/
@ThriftField(name="verifyTime")
@JsonProperty("verifyTime")
public void writeVerifyTime(Long newVal){
setVerifyTime(null == newVal?null:new java.util.Date(newVal));
}
/**
* Setter method for {@link #verifyTime}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to verifyTime
*/
@JsonIgnore
public void setVerifyTime(long newVal)
{
setVerifyTime(new java.util.Date(newVal));
}
/**
* Setter method for {@link #verifyTime}.
* @param newVal the number of milliseconds since January 1, 1970, 00:00:00 GMT represented by this Date object.
*/
@JsonIgnore
public void setVerifyTime(Long newVal)
{
setVerifyTime(null == newVal ? null : new java.util.Date(newVal));
}
/**
* Determines if the verifyTime has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkVerifyTimeModified()
{
return 0 != (modified & FL_LOG_ID_VERIFY_TIME_MASK);
}
/**
* Determines if the verifyTime has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkVerifyTimeInitialized()
{
return 0 != (initialized & FL_LOG_ID_VERIFY_TIME_MASK);
}
/**
* Getter method for {@link #createTime}.
* Meta Data Information (in progress):
*
* - full name: fl_log.create_time
* - default value: 'CURRENT_TIMESTAMP'
* - NOT NULL
* - column size: 19
* - JDBC type returned by the driver: Types.TIMESTAMP
*
*
* @return the value of createTime
*/
@JsonIgnore
public java.util.Date getCreateTime(){
return createTime;
}
/**
* use Long to represent date type for thrift:swift support
* @see #getCreateTime()
*/
@ThriftField(value=17,name="createTime")
@JsonProperty("createTime")
public Long readCreateTime(){
return null == createTime ? null:createTime.getTime();
}
/**
* Setter method for {@link #createTime}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to createTime
*/
@JsonIgnore
public void setCreateTime(java.util.Date newVal)
{
modified |= FL_LOG_ID_CREATE_TIME_MASK;
initialized |= FL_LOG_ID_CREATE_TIME_MASK;
if (Objects.equals(newVal, createTime)) {
return;
}
createTime = newVal;
}
/**
* setter for thrift:swift OR jackson support
* without modification for {@link #modified} and {@link #initialized}
* NOTE:DO NOT use the method in your code
*/
@ThriftField(name="createTime")
@JsonProperty("createTime")
public void writeCreateTime(Long newVal){
setCreateTime(null == newVal?null:new java.util.Date(newVal));
}
/**
* Setter method for {@link #createTime}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to createTime
*/
@JsonIgnore
public void setCreateTime(long newVal)
{
setCreateTime(new java.util.Date(newVal));
}
/**
* Setter method for {@link #createTime}.
* @param newVal the number of milliseconds since January 1, 1970, 00:00:00 GMT represented by this Date object.
*/
@JsonIgnore
public void setCreateTime(Long newVal)
{
setCreateTime(null == newVal ? null : new java.util.Date(newVal));
}
/**
* Determines if the createTime has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkCreateTimeModified()
{
return 0 != (modified & FL_LOG_ID_CREATE_TIME_MASK);
}
/**
* Determines if the createTime has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkCreateTimeInitialized()
{
return 0 != (initialized & FL_LOG_ID_CREATE_TIME_MASK);
}
@Override
public boolean beModified()
{
return 0 != modified;
}
@Override
public boolean isModified(int columnID){
return columnID>=0 && columnID < metaData.columnCount && 0 != (modified & (1 << columnID));
}
@Override
public boolean isInitialized(int columnID){
return columnID>=0 && columnID < metaData.columnCount && 0 != (initialized & (1 << columnID));
}
@Override
public void resetIsModified()
{
modified = 0;
}
@Override
public void resetPrimaryKeysModified()
{
modified &= (~(FL_LOG_ID_ID_MASK));
}
/**
* Resets columns modification status except primary keys to 'not modified'.
*/
public void resetModifiedExceptPrimaryKeys()
{
modified &= (~(FL_LOG_ID_PERSON_ID_MASK |
FL_LOG_ID_DEVICE_ID_MASK |
FL_LOG_ID_VERIFY_FEATURE_MASK |
FL_LOG_ID_IMAGE_MD5_MASK |
FL_LOG_ID_COMPARE_FACE_MASK |
FL_LOG_ID_VERIFY_STATUS_MASK |
FL_LOG_ID_SIMILARTY_MASK |
FL_LOG_ID_DIRECTION_MASK |
FL_LOG_ID_TEMPERATURE_MASK |
FL_LOG_ID_PROPS_MASK |
FL_LOG_ID_VERIFY_TYPE_MASK |
FL_LOG_ID_VERIFY_TIME_MASK |
FL_LOG_ID_CREATE_TIME_MASK));
}
/** reset all fields to initial value, equal to a new bean */
public void reset(){
this.id = null;
this.personId = null;
this.deviceId = null;
this.verifyFeature = null;
this.imageMd5 = null;
this.compareFace = null;
this.verifyStatus = null;
this.similarty = null;
this.direction = null;
this.temperature = null;
this.props = null;
/* DEFAULT:'0'*/
this.verifyType = new Integer(0);
/* DEFAULT:'CURRENT_TIMESTAMP'*/
this.verifyTime = null;
/* DEFAULT:'CURRENT_TIMESTAMP'*/
this.createTime = null;
this.isNew = true;
this.modified = 0;
this.initialized = (FL_LOG_ID_VERIFY_TYPE_MASK);
}
@Override
public LogBean clone(){
return (LogBean) super.clone();
}
public static final Builder builder(){
return new Builder().reset();
}
/**
* a builder for LogBean,the template instance is thread local variable
* a instance of Builder can be reused.
*/
public static final class Builder{
/** LogBean instance used for template to create new LogBean instance. */
static final ThreadLocal TEMPLATE = new ThreadLocal(){
@Override
protected LogBean initialValue() {
return new LogBean();
}};
private Builder() {}
/**
* reset the bean as template
* @see LogBean#reset()
*/
public Builder reset(){
TEMPLATE.get().reset();
return this;
}
/** set a bean as template,must not be {@code null} */
public Builder template(LogBean bean){
if(null == bean){
throw new NullPointerException();
}
TEMPLATE.set(bean);
return this;
}
/** return a clone instance of {@link #TEMPLATE}*/
public LogBean build(){
return TEMPLATE.get().clone();
}
/**
* fill the field : fl_log.id
* @param id 日志id
* @see LogBean#getId()
* @see LogBean#setId(Integer)
*/
public Builder id(Integer id){
TEMPLATE.get().setId(id);
return this;
}
/**
* fill the field : fl_log.person_id
* @param personId 外键,用户id
* @see LogBean#getPersonId()
* @see LogBean#setPersonId(Integer)
*/
public Builder personId(Integer personId){
TEMPLATE.get().setPersonId(personId);
return this;
}
/**
* fill the field : fl_log.device_id
* @param deviceId 外键,日志来源设备id
* @see LogBean#getDeviceId()
* @see LogBean#setDeviceId(Integer)
*/
public Builder deviceId(Integer deviceId){
TEMPLATE.get().setDeviceId(deviceId);
return this;
}
/**
* fill the field : fl_log.verify_feature
* @param verifyFeature 外键,用于验证身份的人脸特征数据MD5 id
* @see LogBean#getVerifyFeature()
* @see LogBean#setVerifyFeature(String)
*/
public Builder verifyFeature(String verifyFeature){
TEMPLATE.get().setVerifyFeature(verifyFeature);
return this;
}
/**
* fill the field : fl_log.image_md5
* @param imageMd5 外键,现场采集人脸图像id
* @see LogBean#getImageMd5()
* @see LogBean#setImageMd5(String)
*/
public Builder imageMd5(String imageMd5){
TEMPLATE.get().setImageMd5(imageMd5);
return this;
}
/**
* fill the field : fl_log.compare_face
* @param compareFace 外键,现场采集的人脸信息记录id
* @see LogBean#getCompareFace()
* @see LogBean#setCompareFace(Integer)
*/
public Builder compareFace(Integer compareFace){
TEMPLATE.get().setCompareFace(compareFace);
return this;
}
/**
* fill the field : fl_log.verify_status
* @param verifyStatus 验证状态,NULL,0:允许通过,其他:拒绝
* @see LogBean#getVerifyStatus()
* @see LogBean#setVerifyStatus(Integer)
*/
public Builder verifyStatus(Integer verifyStatus){
TEMPLATE.get().setVerifyStatus(verifyStatus);
return this;
}
/**
* fill the field : fl_log.similarty
* @param similarty 验证相似度
* @see LogBean#getSimilarty()
* @see LogBean#setSimilarty(Double)
*/
public Builder similarty(Double similarty){
TEMPLATE.get().setSimilarty(similarty);
return this;
}
/**
* fill the field : fl_log.direction
* @param direction 通行方向,NULL,0:入口,1:出口,默认0
* @see LogBean#getDirection()
* @see LogBean#setDirection(Integer)
*/
public Builder direction(Integer direction){
TEMPLATE.get().setDirection(direction);
return this;
}
/**
* fill the field : fl_log.temperature
* @param temperature 体温(摄氏)
* @see LogBean#getTemperature()
* @see LogBean#setTemperature(Double)
*/
public Builder temperature(Double temperature){
TEMPLATE.get().setTemperature(temperature);
return this;
}
/**
* fill the field : fl_log.props
* @param props JSON格式的扩展字段(最大64KB),用于定义附加验证信息
* @see LogBean#getProps()
* @see LogBean#setProps(String)
*/
public Builder props(String props){
TEMPLATE.get().setProps(props);
return this;
}
/**
* fill the field : fl_log.verify_type
* @param verifyType 身份认证方式,NULL,0:人脸,1:指纹,2:密码,3:刷卡
* @see LogBean#getVerifyType()
* @see LogBean#setVerifyType(Integer)
*/
public Builder verifyType(Integer verifyType){
TEMPLATE.get().setVerifyType(verifyType);
return this;
}
/**
* fill the field : fl_log.verify_time
* @param verifyTime 验证时间(可能由前端设备提供时间)
* @see LogBean#getVerifyTime()
* @see LogBean#setVerifyTime(java.util.Date)
*/
public Builder verifyTime(java.util.Date verifyTime){
TEMPLATE.get().setVerifyTime(verifyTime);
return this;
}
/**
* fill the field : fl_log.create_time
* @param createTime
* @see LogBean#getCreateTime()
* @see LogBean#setCreateTime(java.util.Date)
*/
public Builder createTime(java.util.Date createTime){
TEMPLATE.get().setCreateTime(createTime);
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy