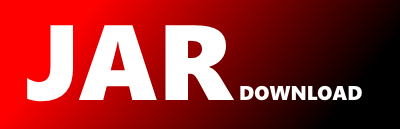
net.gdface.facelog.db.LogLightBean Maven / Gradle / Ivy
// ______________________________________________________
// Generated by sql2java - https://github.com/10km/sql2java
// JDBC driver used at code generation time: com.mysql.jdbc.Driver
// template: bean.java.vm
// ______________________________________________________
package net.gdface.facelog.db;
import java.io.Serializable;
import java.util.Objects;
import gu.sql2java.BaseRow;
import com.facebook.swift.codec.ThriftStruct;
import com.facebook.swift.codec.ThriftField;
import com.facebook.swift.codec.ThriftField.Requiredness;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonIgnore;
import net.gdface.annotation.CodegenLength;
import net.gdface.annotation.CodegenRequired;
import net.gdface.annotation.CodegenDefaultvalue;
import net.gdface.annotation.CodegenInvalidValue;
/**
* LogLightBean is a mapping of fl_log_light Table.
*
Meta Data Information (in progress):
*
* - comments: VIEW
*
* @author guyadong
*/
@ThriftStruct
@ApiModel(description="VIEW")
public final class LogLightBean extends BaseRow
implements Serializable,Constant
{
private static final long serialVersionUID = 4419196843551738129L;
/** comments:日志id */
@ApiModelProperty(value = "日志id" ,dataType="Integer")
@CodegenDefaultvalue("0")@CodegenInvalidValue("-1")
private Integer id;
/** comments:用户id */
@ApiModelProperty(value = "用户id" ,dataType="Integer")
@CodegenDefaultvalue("0")@CodegenInvalidValue("-1")
private Integer personId;
/** comments:姓名 */
@ApiModelProperty(value = "姓名" ,required=true ,dataType="String")
@CodegenLength(max=32,prealloc=true)@CodegenRequired@CodegenInvalidValue
private String name;
/** comments:证件类型,0:未知,1:身份证,2:护照,3:台胞证,4:港澳通行证,5:军官证,6:外国人居留证,7:员工卡,8:其他 */
@ApiModelProperty(value = "证件类型,0:未知,1:身份证,2:护照,3:台胞证,4:港澳通行证,5:军官证,6:外国人居留证,7:员工卡,8:其他" ,dataType="Integer")
@CodegenInvalidValue("-1")
private Integer papersType;
/** comments:证件号码 */
@ApiModelProperty(value = "证件号码" ,dataType="String")
@CodegenLength(max=32,prealloc=true)@CodegenInvalidValue
private String papersNum;
/** comments:外键,日志来源设备id */
@ApiModelProperty(value = "外键,日志来源设备id" ,dataType="Integer")
@CodegenInvalidValue("-1")
private Integer deviceId;
/** comments:身份认证方式,NULL,0:人脸,1:指纹,2:密码,3:刷卡 */
@ApiModelProperty(value = "身份认证方式,NULL,0:人脸,1:指纹,2:密码,3:刷卡" ,dataType="Integer")
@CodegenDefaultvalue("0")@CodegenInvalidValue("-1")
private Integer verifyType;
/** comments:验证时间(可能由前端设备提供时间) */
@ApiModelProperty(value = "验证时间(可能由前端设备提供时间)" ,dataType="Date")
@CodegenDefaultvalue("0000-00-00 00:00:00")@CodegenInvalidValue("0")
private java.util.Date verifyTime;
/** comments:通行方向,NULL,0:入口,1:出口,默认0 */
@ApiModelProperty(value = "通行方向,NULL,0:入口,1:出口,默认0" ,dataType="Integer")
@CodegenInvalidValue("-1")
private Integer direction;
/** comments:体温(摄氏) */
@ApiModelProperty(value = "体温(摄氏)" ,dataType="Double")
@CodegenInvalidValue("-1")
private Double temperature;
/** comments:外键,现场采集人脸图像id */
@ApiModelProperty(value = "外键,现场采集人脸图像id" ,dataType="String")
@CodegenLength(value=32,prealloc=true)@CodegenInvalidValue
private String imageMd5;
/** comments:JSON格式的扩展字段(最大64KB),用于定义附加验证信息 */
@ApiModelProperty(value = "JSON格式的扩展字段(最大64KB),用于定义附加验证信息" ,dataType="String")
@CodegenLength(max=65535)@CodegenInvalidValue
private String props;
/** columns modified flag */
@ApiModelProperty(value="columns modified flag",dataType="int",required=true)
private int modified;
/** columns initialized flag */
@ApiModelProperty(value="columns initialized flag",dataType="int",required=true)
private int initialized;
/** new record flag */
@ApiModelProperty(value="new record flag",dataType="boolean",required=true)
private boolean isNew;
@ThriftField(value=1,name="_new",requiredness=Requiredness.REQUIRED)
@Override
public boolean isNew()
{
return this.isNew;
}
/**
* Specifies to the object if it has been set as new.
*
* @param isNew the boolean value to be assigned to the isNew field
*/
@ThriftField()
@Override
public void setNew(boolean isNew)
{
this.isNew = isNew;
}
/**
* @return the modified status of columns
*/
@ThriftField(value=2,requiredness=Requiredness.REQUIRED)
@Override
public int getModified(){
return modified;
}
/**
* @param modified the modified status bit to be assigned to {@link #modified}
*/
@ThriftField()
@Override
public void setModified(int modified){
this.modified = modified;
}
/**
* @return the initialized status of columns
*/
@ThriftField(value=3,requiredness=Requiredness.REQUIRED)
@Override
public int getInitialized(){
return initialized;
}
/**
* @param initialized the initialized status bit to be assigned to {@link #initialized}
*/
@ThriftField()
@Override
public void setInitialized(int initialized){
this.initialized = initialized;
}
public LogLightBean(){
reset();
}
/**
* Getter method for {@link #id}.
* Meta Data Information (in progress):
*
* - full name: fl_log_light.id
* - comments: 日志id
* - default value: '0'
* - NOT NULL
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of id
*/
@ThriftField(value=4)
@JsonProperty("id")
public Integer getId(){
return id;
}
/**
* Setter method for {@link #id}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to id
*/
@ThriftField(name="id")
@JsonProperty("id")
public void setId(Integer newVal)
{
modified |= FL_LOG_LIGHT_ID_ID_MASK;
initialized |= FL_LOG_LIGHT_ID_ID_MASK;
if (Objects.equals(newVal, id)) {
return;
}
id = newVal;
}
/**
* Setter method for {@link #id}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to id
*/
@JsonIgnore
public void setId(int newVal)
{
setId(new Integer(newVal));
}
/**
* Determines if the id has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkIdModified()
{
return 0 != (modified & FL_LOG_LIGHT_ID_ID_MASK);
}
/**
* Determines if the id has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkIdInitialized()
{
return 0 != (initialized & FL_LOG_LIGHT_ID_ID_MASK);
}
/**
* Getter method for {@link #personId}.
* Meta Data Information (in progress):
*
* - full name: fl_log_light.person_id
* - comments: 用户id
* - default value: '0'
* - NOT NULL
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of personId
*/
@ThriftField(value=5)
@JsonProperty("personId")
public Integer getPersonId(){
return personId;
}
/**
* Setter method for {@link #personId}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to personId
*/
@ThriftField(name="personId")
@JsonProperty("personId")
public void setPersonId(Integer newVal)
{
modified |= FL_LOG_LIGHT_ID_PERSON_ID_MASK;
initialized |= FL_LOG_LIGHT_ID_PERSON_ID_MASK;
if (Objects.equals(newVal, personId)) {
return;
}
personId = newVal;
}
/**
* Setter method for {@link #personId}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to personId
*/
@JsonIgnore
public void setPersonId(int newVal)
{
setPersonId(new Integer(newVal));
}
/**
* Determines if the personId has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkPersonIdModified()
{
return 0 != (modified & FL_LOG_LIGHT_ID_PERSON_ID_MASK);
}
/**
* Determines if the personId has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkPersonIdInitialized()
{
return 0 != (initialized & FL_LOG_LIGHT_ID_PERSON_ID_MASK);
}
/**
* Getter method for {@link #name}.
* Meta Data Information (in progress):
*
* - full name: fl_log_light.name
* - comments: 姓名
* - NOT NULL
* - column size: 32
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of name
*/
@ThriftField(value=6)
@JsonProperty("name")
public String getName(){
return name;
}
/**
* Setter method for {@link #name}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to name
*/
@ThriftField(name="name")
@JsonProperty("name")
public void setName(String newVal)
{
modified |= FL_LOG_LIGHT_ID_NAME_MASK;
initialized |= FL_LOG_LIGHT_ID_NAME_MASK;
if (Objects.equals(newVal, name)) {
return;
}
name = newVal;
}
/**
* Determines if the name has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkNameModified()
{
return 0 != (modified & FL_LOG_LIGHT_ID_NAME_MASK);
}
/**
* Determines if the name has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkNameInitialized()
{
return 0 != (initialized & FL_LOG_LIGHT_ID_NAME_MASK);
}
/**
* Getter method for {@link #papersType}.
* Meta Data Information (in progress):
*
* - full name: fl_log_light.papers_type
* - comments: 证件类型,0:未知,1:身份证,2:护照,3:台胞证,4:港澳通行证,5:军官证,6:外国人居留证,7:员工卡,8:其他
* - column size: 3
* - JDBC type returned by the driver: Types.TINYINT
*
*
* @return the value of papersType
*/
@ThriftField(value=7)
@JsonProperty("papersType")
public Integer getPapersType(){
return papersType;
}
/**
* Setter method for {@link #papersType}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to papersType
*/
@ThriftField(name="papersType")
@JsonProperty("papersType")
public void setPapersType(Integer newVal)
{
modified |= FL_LOG_LIGHT_ID_PAPERS_TYPE_MASK;
initialized |= FL_LOG_LIGHT_ID_PAPERS_TYPE_MASK;
if (Objects.equals(newVal, papersType)) {
return;
}
papersType = newVal;
}
/**
* Setter method for {@link #papersType}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to papersType
*/
@JsonIgnore
public void setPapersType(int newVal)
{
setPapersType(new Integer(newVal));
}
/**
* Determines if the papersType has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkPapersTypeModified()
{
return 0 != (modified & FL_LOG_LIGHT_ID_PAPERS_TYPE_MASK);
}
/**
* Determines if the papersType has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkPapersTypeInitialized()
{
return 0 != (initialized & FL_LOG_LIGHT_ID_PAPERS_TYPE_MASK);
}
/**
* Getter method for {@link #papersNum}.
* Meta Data Information (in progress):
*
* - full name: fl_log_light.papers_num
* - comments: 证件号码
* - column size: 32
* - JDBC type returned by the driver: Types.VARCHAR
*
*
* @return the value of papersNum
*/
@ThriftField(value=8)
@JsonProperty("papersNum")
public String getPapersNum(){
return papersNum;
}
/**
* Setter method for {@link #papersNum}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to papersNum
*/
@ThriftField(name="papersNum")
@JsonProperty("papersNum")
public void setPapersNum(String newVal)
{
modified |= FL_LOG_LIGHT_ID_PAPERS_NUM_MASK;
initialized |= FL_LOG_LIGHT_ID_PAPERS_NUM_MASK;
if (Objects.equals(newVal, papersNum)) {
return;
}
papersNum = newVal;
}
/**
* Determines if the papersNum has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkPapersNumModified()
{
return 0 != (modified & FL_LOG_LIGHT_ID_PAPERS_NUM_MASK);
}
/**
* Determines if the papersNum has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkPapersNumInitialized()
{
return 0 != (initialized & FL_LOG_LIGHT_ID_PAPERS_NUM_MASK);
}
/**
* Getter method for {@link #deviceId}.
* Meta Data Information (in progress):
*
* - full name: fl_log_light.device_id
* - comments: 外键,日志来源设备id
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of deviceId
*/
@ThriftField(value=9)
@JsonProperty("deviceId")
public Integer getDeviceId(){
return deviceId;
}
/**
* Setter method for {@link #deviceId}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to deviceId
*/
@ThriftField(name="deviceId")
@JsonProperty("deviceId")
public void setDeviceId(Integer newVal)
{
modified |= FL_LOG_LIGHT_ID_DEVICE_ID_MASK;
initialized |= FL_LOG_LIGHT_ID_DEVICE_ID_MASK;
if (Objects.equals(newVal, deviceId)) {
return;
}
deviceId = newVal;
}
/**
* Setter method for {@link #deviceId}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to deviceId
*/
@JsonIgnore
public void setDeviceId(int newVal)
{
setDeviceId(new Integer(newVal));
}
/**
* Determines if the deviceId has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkDeviceIdModified()
{
return 0 != (modified & FL_LOG_LIGHT_ID_DEVICE_ID_MASK);
}
/**
* Determines if the deviceId has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkDeviceIdInitialized()
{
return 0 != (initialized & FL_LOG_LIGHT_ID_DEVICE_ID_MASK);
}
/**
* Getter method for {@link #verifyType}.
* Meta Data Information (in progress):
*
* - full name: fl_log_light.verify_type
* - comments: 身份认证方式,NULL,0:人脸,1:指纹,2:密码,3:刷卡
* - default value: '0'
* - column size: 3
* - JDBC type returned by the driver: Types.TINYINT
*
*
* @return the value of verifyType
*/
@ThriftField(value=10)
@JsonProperty("verifyType")
public Integer getVerifyType(){
return verifyType;
}
/**
* Setter method for {@link #verifyType}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to verifyType
*/
@ThriftField(name="verifyType")
@JsonProperty("verifyType")
public void setVerifyType(Integer newVal)
{
modified |= FL_LOG_LIGHT_ID_VERIFY_TYPE_MASK;
initialized |= FL_LOG_LIGHT_ID_VERIFY_TYPE_MASK;
if (Objects.equals(newVal, verifyType)) {
return;
}
verifyType = newVal;
}
/**
* Setter method for {@link #verifyType}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to verifyType
*/
@JsonIgnore
public void setVerifyType(int newVal)
{
setVerifyType(new Integer(newVal));
}
/**
* Determines if the verifyType has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkVerifyTypeModified()
{
return 0 != (modified & FL_LOG_LIGHT_ID_VERIFY_TYPE_MASK);
}
/**
* Determines if the verifyType has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkVerifyTypeInitialized()
{
return 0 != (initialized & FL_LOG_LIGHT_ID_VERIFY_TYPE_MASK);
}
/**
* Getter method for {@link #verifyTime}.
* Meta Data Information (in progress):
*
* - full name: fl_log_light.verify_time
* - comments: 验证时间(可能由前端设备提供时间)
* - default value: '0000-00-00 00:00:00'
* - NOT NULL
* - column size: 19
* - JDBC type returned by the driver: Types.TIMESTAMP
*
*
* @return the value of verifyTime
*/
@JsonIgnore
public java.util.Date getVerifyTime(){
return verifyTime;
}
/**
* use Long to represent date type for thrift:swift support
* @see #getVerifyTime()
*/
@ThriftField(value=11,name="verifyTime")
@JsonProperty("verifyTime")
public Long readVerifyTime(){
return null == verifyTime ? null:verifyTime.getTime();
}
/**
* Setter method for {@link #verifyTime}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value( NOT NULL) to be assigned to verifyTime
*/
@JsonIgnore
public void setVerifyTime(java.util.Date newVal)
{
modified |= FL_LOG_LIGHT_ID_VERIFY_TIME_MASK;
initialized |= FL_LOG_LIGHT_ID_VERIFY_TIME_MASK;
if (Objects.equals(newVal, verifyTime)) {
return;
}
verifyTime = newVal;
}
/**
* setter for thrift:swift OR jackson support
* without modification for {@link #modified} and {@link #initialized}
* NOTE:DO NOT use the method in your code
*/
@ThriftField(name="verifyTime")
@JsonProperty("verifyTime")
public void writeVerifyTime(Long newVal){
setVerifyTime(null == newVal?null:new java.util.Date(newVal));
}
/**
* Setter method for {@link #verifyTime}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to verifyTime
*/
@JsonIgnore
public void setVerifyTime(long newVal)
{
setVerifyTime(new java.util.Date(newVal));
}
/**
* Setter method for {@link #verifyTime}.
* @param newVal the number of milliseconds since January 1, 1970, 00:00:00 GMT represented by this Date object.
*/
@JsonIgnore
public void setVerifyTime(Long newVal)
{
setVerifyTime(null == newVal ? null : new java.util.Date(newVal));
}
/**
* Determines if the verifyTime has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkVerifyTimeModified()
{
return 0 != (modified & FL_LOG_LIGHT_ID_VERIFY_TIME_MASK);
}
/**
* Determines if the verifyTime has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkVerifyTimeInitialized()
{
return 0 != (initialized & FL_LOG_LIGHT_ID_VERIFY_TIME_MASK);
}
/**
* Getter method for {@link #direction}.
* Meta Data Information (in progress):
*
* - full name: fl_log_light.direction
* - comments: 通行方向,NULL,0:入口,1:出口,默认0
* - column size: 10
* - JDBC type returned by the driver: Types.INTEGER
*
*
* @return the value of direction
*/
@ThriftField(value=12)
@JsonProperty("direction")
public Integer getDirection(){
return direction;
}
/**
* Setter method for {@link #direction}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to direction
*/
@ThriftField(name="direction")
@JsonProperty("direction")
public void setDirection(Integer newVal)
{
modified |= FL_LOG_LIGHT_ID_DIRECTION_MASK;
initialized |= FL_LOG_LIGHT_ID_DIRECTION_MASK;
if (Objects.equals(newVal, direction)) {
return;
}
direction = newVal;
}
/**
* Setter method for {@link #direction}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to direction
*/
@JsonIgnore
public void setDirection(int newVal)
{
setDirection(new Integer(newVal));
}
/**
* Determines if the direction has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkDirectionModified()
{
return 0 != (modified & FL_LOG_LIGHT_ID_DIRECTION_MASK);
}
/**
* Determines if the direction has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkDirectionInitialized()
{
return 0 != (initialized & FL_LOG_LIGHT_ID_DIRECTION_MASK);
}
/**
* Getter method for {@link #temperature}.
* Meta Data Information (in progress):
*
* - full name: fl_log_light.temperature
* - comments: 体温(摄氏)
* - column size: 4
* - JDBC type returned by the driver: Types.DOUBLE
*
*
* @return the value of temperature
*/
@ThriftField(value=13)
@JsonProperty("temperature")
public Double getTemperature(){
return temperature;
}
/**
* Setter method for {@link #temperature}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to temperature
*/
@ThriftField(name="temperature")
@JsonProperty("temperature")
public void setTemperature(Double newVal)
{
modified |= FL_LOG_LIGHT_ID_TEMPERATURE_MASK;
initialized |= FL_LOG_LIGHT_ID_TEMPERATURE_MASK;
if (Objects.equals(newVal, temperature)) {
return;
}
temperature = newVal;
}
/**
* Setter method for {@link #temperature}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to temperature
*/
@JsonIgnore
public void setTemperature(double newVal)
{
setTemperature(new Double(newVal));
}
/**
* Determines if the temperature has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkTemperatureModified()
{
return 0 != (modified & FL_LOG_LIGHT_ID_TEMPERATURE_MASK);
}
/**
* Determines if the temperature has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkTemperatureInitialized()
{
return 0 != (initialized & FL_LOG_LIGHT_ID_TEMPERATURE_MASK);
}
/**
* Getter method for {@link #imageMd5}.
* Meta Data Information (in progress):
*
* - full name: fl_log_light.image_md5
* - comments: 外键,现场采集人脸图像id
* - column size: 32
* - JDBC type returned by the driver: Types.CHAR
*
*
* @return the value of imageMd5
*/
@ThriftField(value=14)
@JsonProperty("imageMd5")
public String getImageMd5(){
return imageMd5;
}
/**
* Setter method for {@link #imageMd5}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to imageMd5
*/
@ThriftField(name="imageMd5")
@JsonProperty("imageMd5")
public void setImageMd5(String newVal)
{
modified |= FL_LOG_LIGHT_ID_IMAGE_MD5_MASK;
initialized |= FL_LOG_LIGHT_ID_IMAGE_MD5_MASK;
if (Objects.equals(newVal, imageMd5)) {
return;
}
imageMd5 = newVal;
}
/**
* Determines if the imageMd5 has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkImageMd5Modified()
{
return 0 != (modified & FL_LOG_LIGHT_ID_IMAGE_MD5_MASK);
}
/**
* Determines if the imageMd5 has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkImageMd5Initialized()
{
return 0 != (initialized & FL_LOG_LIGHT_ID_IMAGE_MD5_MASK);
}
/**
* Getter method for {@link #props}.
* Meta Data Information (in progress):
*
* - full name: fl_log_light.props
* - comments: JSON格式的扩展字段(最大64KB),用于定义附加验证信息
* - column size: 65535
* - JDBC type returned by the driver: Types.LONGVARCHAR
*
*
* @return the value of props
*/
@ThriftField(value=15)
@JsonProperty("props")
public String getProps(){
return props;
}
/**
* Setter method for {@link #props}.
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
*
* @param newVal the new value to be assigned to props
*/
@ThriftField(name="props")
@JsonProperty("props")
public void setProps(String newVal)
{
modified |= FL_LOG_LIGHT_ID_PROPS_MASK;
initialized |= FL_LOG_LIGHT_ID_PROPS_MASK;
if (Objects.equals(newVal, props)) {
return;
}
props = newVal;
}
/**
* Determines if the props has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean checkPropsModified()
{
return 0 != (modified & FL_LOG_LIGHT_ID_PROPS_MASK);
}
/**
* Determines if the props has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean checkPropsInitialized()
{
return 0 != (initialized & FL_LOG_LIGHT_ID_PROPS_MASK);
}
@Override
public boolean beModified()
{
return 0 != modified;
}
@Override
public boolean isModified(int columnID){
return columnID>=0 && columnID < metaData.columnCount && 0 != (modified & (1 << columnID));
}
@Override
public boolean isInitialized(int columnID){
return columnID>=0 && columnID < metaData.columnCount && 0 != (initialized & (1 << columnID));
}
@Override
public void resetIsModified()
{
modified = 0;
}
@Override
public void resetPrimaryKeysModified()
{
// columns is null or empty;
}
/**
* Resets columns modification status except primary keys to 'not modified'.
*/
public void resetModifiedExceptPrimaryKeys()
{
modified &= (~(FL_LOG_LIGHT_ID_ID_MASK |
FL_LOG_LIGHT_ID_PERSON_ID_MASK |
FL_LOG_LIGHT_ID_NAME_MASK |
FL_LOG_LIGHT_ID_PAPERS_TYPE_MASK |
FL_LOG_LIGHT_ID_PAPERS_NUM_MASK |
FL_LOG_LIGHT_ID_DEVICE_ID_MASK |
FL_LOG_LIGHT_ID_VERIFY_TYPE_MASK |
FL_LOG_LIGHT_ID_VERIFY_TIME_MASK |
FL_LOG_LIGHT_ID_DIRECTION_MASK |
FL_LOG_LIGHT_ID_TEMPERATURE_MASK |
FL_LOG_LIGHT_ID_IMAGE_MD5_MASK |
FL_LOG_LIGHT_ID_PROPS_MASK));
}
/** reset all fields to initial value, equal to a new bean */
public void reset(){
/* DEFAULT:'0'*/
this.id = new Integer(0);
/* DEFAULT:'0'*/
this.personId = new Integer(0);
this.name = null;
this.papersType = null;
this.papersNum = null;
this.deviceId = null;
/* DEFAULT:'0'*/
this.verifyType = new Integer(0);
/* DEFAULT:'0000-00-00 00:00:00'*/
this.verifyTime = null;
this.direction = null;
this.temperature = null;
this.imageMd5 = null;
this.props = null;
this.isNew = true;
this.modified = 0;
this.initialized = (FL_LOG_LIGHT_ID_ID_MASK | FL_LOG_LIGHT_ID_PERSON_ID_MASK | FL_LOG_LIGHT_ID_VERIFY_TYPE_MASK);
}
@Override
public LogLightBean clone(){
return (LogLightBean) super.clone();
}
public static final Builder builder(){
return new Builder().reset();
}
/**
* a builder for LogLightBean,the template instance is thread local variable
* a instance of Builder can be reused.
*/
public static final class Builder{
/** LogLightBean instance used for template to create new LogLightBean instance. */
static final ThreadLocal TEMPLATE = new ThreadLocal(){
@Override
protected LogLightBean initialValue() {
return new LogLightBean();
}};
private Builder() {}
/**
* reset the bean as template
* @see LogLightBean#reset()
*/
public Builder reset(){
TEMPLATE.get().reset();
return this;
}
/** set a bean as template,must not be {@code null} */
public Builder template(LogLightBean bean){
if(null == bean){
throw new NullPointerException();
}
TEMPLATE.set(bean);
return this;
}
/** return a clone instance of {@link #TEMPLATE}*/
public LogLightBean build(){
return TEMPLATE.get().clone();
}
/**
* fill the field : fl_log_light.id
* @param id 日志id
* @see LogLightBean#getId()
* @see LogLightBean#setId(Integer)
*/
public Builder id(Integer id){
TEMPLATE.get().setId(id);
return this;
}
/**
* fill the field : fl_log_light.person_id
* @param personId 用户id
* @see LogLightBean#getPersonId()
* @see LogLightBean#setPersonId(Integer)
*/
public Builder personId(Integer personId){
TEMPLATE.get().setPersonId(personId);
return this;
}
/**
* fill the field : fl_log_light.name
* @param name 姓名
* @see LogLightBean#getName()
* @see LogLightBean#setName(String)
*/
public Builder name(String name){
TEMPLATE.get().setName(name);
return this;
}
/**
* fill the field : fl_log_light.papers_type
* @param papersType 证件类型,0:未知,1:身份证,2:护照,3:台胞证,4:港澳通行证,5:军官证,6:外国人居留证,7:员工卡,8:其他
* @see LogLightBean#getPapersType()
* @see LogLightBean#setPapersType(Integer)
*/
public Builder papersType(Integer papersType){
TEMPLATE.get().setPapersType(papersType);
return this;
}
/**
* fill the field : fl_log_light.papers_num
* @param papersNum 证件号码
* @see LogLightBean#getPapersNum()
* @see LogLightBean#setPapersNum(String)
*/
public Builder papersNum(String papersNum){
TEMPLATE.get().setPapersNum(papersNum);
return this;
}
/**
* fill the field : fl_log_light.device_id
* @param deviceId 外键,日志来源设备id
* @see LogLightBean#getDeviceId()
* @see LogLightBean#setDeviceId(Integer)
*/
public Builder deviceId(Integer deviceId){
TEMPLATE.get().setDeviceId(deviceId);
return this;
}
/**
* fill the field : fl_log_light.verify_type
* @param verifyType 身份认证方式,NULL,0:人脸,1:指纹,2:密码,3:刷卡
* @see LogLightBean#getVerifyType()
* @see LogLightBean#setVerifyType(Integer)
*/
public Builder verifyType(Integer verifyType){
TEMPLATE.get().setVerifyType(verifyType);
return this;
}
/**
* fill the field : fl_log_light.verify_time
* @param verifyTime 验证时间(可能由前端设备提供时间)
* @see LogLightBean#getVerifyTime()
* @see LogLightBean#setVerifyTime(java.util.Date)
*/
public Builder verifyTime(java.util.Date verifyTime){
TEMPLATE.get().setVerifyTime(verifyTime);
return this;
}
/**
* fill the field : fl_log_light.direction
* @param direction 通行方向,NULL,0:入口,1:出口,默认0
* @see LogLightBean#getDirection()
* @see LogLightBean#setDirection(Integer)
*/
public Builder direction(Integer direction){
TEMPLATE.get().setDirection(direction);
return this;
}
/**
* fill the field : fl_log_light.temperature
* @param temperature 体温(摄氏)
* @see LogLightBean#getTemperature()
* @see LogLightBean#setTemperature(Double)
*/
public Builder temperature(Double temperature){
TEMPLATE.get().setTemperature(temperature);
return this;
}
/**
* fill the field : fl_log_light.image_md5
* @param imageMd5 外键,现场采集人脸图像id
* @see LogLightBean#getImageMd5()
* @see LogLightBean#setImageMd5(String)
*/
public Builder imageMd5(String imageMd5){
TEMPLATE.get().setImageMd5(imageMd5);
return this;
}
/**
* fill the field : fl_log_light.props
* @param props JSON格式的扩展字段(最大64KB),用于定义附加验证信息
* @see LogLightBean#getProps()
* @see LogLightBean#setProps(String)
*/
public Builder props(String props){
TEMPLATE.get().setProps(props);
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy