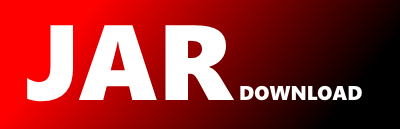
net.gdface.facelog.TempPwdManagement Maven / Gradle / Ivy
package net.gdface.facelog;
import static com.google.common.base.Preconditions.checkNotNull;
import static com.google.common.base.Preconditions.checkArgument;
import java.util.Calendar;
import java.util.Date;
import java.util.List;
import java.util.Random;
import java.util.Set;
import com.google.common.base.Strings;
import com.google.common.collect.Collections2;
import com.google.common.collect.ImmutableList;
import gu.simplemq.redis.JedisPoolLazy;
import gu.simplemq.redis.RedisFactory;
import gu.simplemq.redis.RedisTable;
import gu.sql2java.exception.ObjectRetrievalException;
import net.gdface.facelog.db.DeviceBean;
import net.gdface.facelog.db.DeviceGroupBean;
import net.gdface.facelog.db.PersonBean;
import net.gdface.facelog.db.PersonGroupBean;
/**
* 临时密码管理
* @author guyadong
*
*/
public class TempPwdManagement implements ServiceConstant {
private final DaoManagement dm;
private final RedisTable tempPwdTable;
private final RedisTable tempPwdTabler;
public TempPwdManagement(DaoManagement dao) {
this.dm = checkNotNull(dao,"dao is null");
this.tempPwdTable = RedisFactory.getTable(TABLE_TMP_PWD, JedisPoolLazy.getDefaultInstance());
this.tempPwdTabler = RedisFactory.getTable(TABLE_TMP_PWD_R, JedisPoolLazy.getDefaultInstance());
}
/**
* 根据持续时间(分钟)加上当前时间计算出到期时间
* @param duration 持续时间(分钟)
* @return 到期时间
*/
public static Date getExpiryDate(int duration){
checkArgument(duration >0,"duration must > 0");
Date date = new Date(); //取时间
Calendar calendar = Calendar.getInstance();
calendar.setTime(date);
calendar.add(Calendar.MINUTE,duration);
return calendar.getTime();
}
/**
* 为targetId指定的目标创建临时密码对象
* @param targetId 目标ID
* @param targetType 目标类型
* @param expiryDate 临时密码到期时间
* @return
*/
@SuppressWarnings("unused")
synchronized String createTempPwd(int targetId,TmpPwdTargetType targetType,Date expiryDate){
try {
switch (checkNotNull(targetType,"targetType is null")) {
case USER_GROUP_ID:
{
PersonGroupBean bean = dm.daoGetPersonGroupChecked(targetId);
break;
}
case DEVICE_GROUP_ID:
{
DeviceGroupBean bean = dm.daoGetDeviceGroupChecked(targetId);
break;
}
case USER_ID:
{
PersonBean bean = dm.daoGetPersonChecked(targetId);
break;
}
case DEVICE_ID:
{
DeviceBean bean = dm.daoGetDeviceChecked(targetId);
break;
}
default:
throw new IllegalArgumentException(String.format("UNSUPPORTED target type %s", targetType));
}
if(null == expiryDate){
expiryDate = getExpiryDate(DEFAULT_TEMP_PWD_DURATION);
}
TmpwdTargetInfo target = new TmpwdTargetInfo(targetType, targetId, expiryDate);
String targetKey = target.toString();
// 旧密码
String oldpwd = tempPwdTabler.get(targetKey);
if(oldpwd != null){
// 如果存在旧密码则删除旧密码
tempPwdTable.remove(oldpwd);
tempPwdTabler.remove(targetKey);
}
Random random = new Random();
// 6位数字密码
String pwd = String.format("%06d",random.nextInt(1000000));
while (tempPwdTable.containsKey(pwd)){
pwd = String.format("%06d",random.nextInt(1000000));
}
tempPwdTable.set(pwd, target, true);
tempPwdTabler.set(targetKey,pwd, true);
// 设置过期时间
tempPwdTable.expire(pwd,expiryDate);
tempPwdTabler.expire(targetKey,expiryDate);
return pwd;
} catch (ObjectRetrievalException e) {
throw new IllegalArgumentException(String.format("INVALID targetId %d for %s",targetId,targetType));
}
}
synchronized TmpwdTargetInfo getTempPwd(String pwd){
return Strings.isNullOrEmpty(pwd) ? null : tempPwdTable.get(pwd);
}
private boolean belong(int deviceGroupId,int deviceId){
DeviceBean deviceBean = dm.daoGetDevice(deviceId);
List parents = null == deviceBean
? ImmutableList.of()
: dm.daoToPrimaryKeyListFromDeviceGroups(dm.daoListOfParentForDeviceGroup(deviceBean.getGroupId()));
return parents.contains(deviceGroupId);
}
/**
* 判断指定用户是否可以在指定设备上通行
* @param personId
* @param deviceId
* @return 允许通行返回{@code true},否则返回{@code false}
*/
private boolean permitPerson(int personId,int deviceId){
Set features = dm.daoGetPersonsPermittedOnDevice(deviceId, false, null, null);
return Collections2.transform(features, dm.daoCastPersonToPk).contains(personId);
}
private boolean permitPersonGroup(int personGroupId,int deviceId){
DeviceBean deviceBean = dm.daoGetDevice(deviceId);
if(null != deviceBean){
return dm.daoGetPersonGroupsPermittedBy(deviceBean.getGroupId(),false).contains(personGroupId);
}
return false;
}
TmpwdTargetInfo getTargetInfo4PwdOnDevice(String pwd,int deviceId){
TmpwdTargetInfo targetInfo = getTempPwd(pwd);
boolean permit = false;
if(targetInfo != null){
switch(targetInfo.getTargetType()){
case USER_GROUP_ID:
permit = permitPersonGroup(targetInfo.getTargetId(),deviceId);
break;
case DEVICE_GROUP_ID:
permit = belong(targetInfo.getTargetId(),deviceId);
break;
case USER_ID:
permit = permitPerson(targetInfo.getTargetId(), deviceId);
break;
case DEVICE_ID:
permit = targetInfo.getTargetId() == deviceId;
break;
default:
throw new IllegalArgumentException(String.format("UNSUPPORTED target type %s", targetInfo.getTargetType()));
}
}
return permit ? targetInfo : null ;
}
boolean isValidTempPwd4Device(String pwd,int deviceId){
return null != getTargetInfo4PwdOnDevice(pwd,deviceId);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy