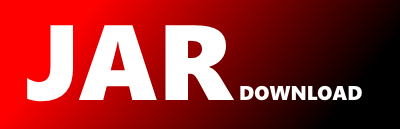
gu.simplemq.ConsumerSingle Maven / Gradle / Ivy
package gu.simplemq;
import java.util.concurrent.BlockingDeque;
import java.util.concurrent.BlockingQueue;
import java.util.concurrent.LinkedBlockingDeque;
import java.util.concurrent.TimeUnit;
import gu.simplemq.exceptions.SmqUnsubscribeException;
/**
* 基于阻塞队列 {@link BlockingQueue} 实现单个消费者模型
* 默认配置为队列模型,设置{@link #isFifo}为false,则为栈模型,为栈模型时 {@link #queue}必须为双向队列{@link BlockingDeque}
* 需要显式调用 {@link #open()}开启消费线程
* 对象可以复用(反复打开关闭)
* 应用程序结束时要调用 {@link #close()} 才能结束消费线程
* 当设置为{@link #daemon}为true时,无需{@link #close()}关闭
* @author guyadong
*
* @param 消费数据类型
*/
public class ConsumerSingle extends BaseConsumer implements IQueueComponent{
protected BlockingQueue queue = new LinkedBlockingDeque();
private final Runnable customRunnable = new Runnable(){
@Override
public void run() {
try {
T t;
if(isFifo)
t = queue.poll(timeoutMills, TimeUnit.MILLISECONDS);
else{
if(queue instanceof BlockingDeque){
t = ((BlockingDeque)queue).pollLast(timeoutMills, TimeUnit.MILLISECONDS);
}else{
throw new UnsupportedOperationException(" queue must be instance of BlockingDeque");
}
}
if(null != t){
try{
adapter.onSubscribe(t);
} catch (SmqUnsubscribeException e) {
logger.info("consumer thread finished because UnsubscribeException");
close();
} catch (Throwable e) {
e.printStackTrace();
}
}
} catch (Throwable e) {
e.printStackTrace();
}
}
};
public ConsumerSingle() {
}
public ConsumerSingle(BlockingQueue queue) {
super();
this.queue = queue;
}
/** 消息处理器 */
private IMessageAdapter adapter = new IMessageAdapter(){
@Override
public void onSubscribe(T t) throws SmqUnsubscribeException {
}};
public ConsumerSingle setAdapter(IMessageAdapter adapter) {
if(null != adapter){
this.adapter = adapter;
}
return this;
}
@Override
protected Runnable getCustomRunnable(){
return customRunnable;
}
@Override
public BlockingQueue getQueue() {
return queue;
}
public ConsumerSingle setQueue(BlockingQueue queue) {
if(null != queue){
this.queue = queue;
}
return this;
}
@Override
public String getQueueName() {
return "unknow";
}
/**
* 改为public访问,调用者必须显式调用此方法
*/
@Override
public void open() {
super.open();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy