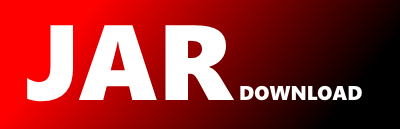
gu.simplemq.json.FastjsonEncoder Maven / Gradle / Ivy
package gu.simplemq.json;
import java.lang.reflect.Type;
import java.nio.ByteBuffer;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Map.Entry;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONObject;
import com.alibaba.fastjson.parser.ParserConfig;
import com.alibaba.fastjson.serializer.SerializeConfig;
import com.alibaba.fastjson.serializer.SerializerFeature;
import gu.simplemq.exceptions.SmqNotBeanException;
import gu.simplemq.utils.TypeUtils;
/**
*
* 基于fastjson实现{@link BaseJsonEncoder}
* @author guyadong
*
*/
public class FastjsonEncoder extends BaseJsonEncoder {
private static final FastjsonEncoder INSTANCE = new FastjsonEncoder();
public static final SerializerFeature[] DEFAULT_SERIALIZER_FEATURES = new SerializerFeature[]{SerializerFeature.WriteMapNullValue,SerializerFeature.WriteNonStringKeyAsString};
private static SerializerFeature[] serializerFeatures = DEFAULT_SERIALIZER_FEATURES;
static {
// 增加对 ByteBuffer 序列化支持
ParserConfig.global.putDeserializer(ByteBuffer.class, ByteBufferCodec.instance);
SerializeConfig.globalInstance.put(ByteBuffer.wrap(new byte[]{}).getClass(), ByteBufferCodec.instance);
}
public static FastjsonEncoder getInstance(){
return INSTANCE;
}
protected FastjsonEncoder() {}
protected JSONObject doToJSONObject(Object bean){
// 先序列化再解析成JSONObject对象
return (JSONObject) JSON.parse(this.toJsonString(bean));
}
@Override
public String toJsonString(Object obj) {
// java对象序列化(输出 null 字段)
return JSON.toJSONString(obj,serializerFeatures);
}
@Override
public Map toJsonMap(Object bean)throws SmqNotBeanException {
if(null ==bean ){
return null;
}
if(!TypeUtils.isJavaBean(bean.getClass())){
throw new SmqNotBeanException("invalid type,not a java bean object");
}
JSONObject jsonObject = doToJSONObject(bean);
Map fields = new LinkedHashMap();
for(Entry entry : jsonObject.entrySet()) {
Object value = entry.getValue();
fields.put(entry.getKey(), null == value ? null : this.toJsonString(value));
}
return fields;
}
@SuppressWarnings("unchecked")
@Override
public T fromJson(String json, Type type) {
if(type instanceof Class>){
return JSON.parseObject(json, (Class)type);
}else{
return JSON.parseObject(json, type);
}
}
@Override
public T fromJson(Map fieldHash, Type type)throws SmqNotBeanException {
if(!TypeUtils.isJavaBean(type)){
throw new SmqNotBeanException("invalid type,not a java bean");
}
if(null == fieldHash || fieldHash.isEmpty()){
return null;
}
Map fields = new LinkedHashMap();
for(Entry entry:fieldHash.entrySet()){
fields.put(entry.getKey(), JSON.parse(entry.getValue()));
}
return com.alibaba.fastjson.util.TypeUtils.cast(fields, type, null);
}
/**
* @return serializerFeature
*/
public static SerializerFeature[] getSerializerFeature() {
return serializerFeatures;
}
/**
* @param serializerFeatures 要设置的 serializerFeature
*/
public static void setSerializerFeature(SerializerFeature[] serializerFeatures) {
if(serializerFeatures != null){
FastjsonEncoder.serializerFeatures = serializerFeatures;
}else{
FastjsonEncoder.serializerFeatures = new SerializerFeature[0];
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy