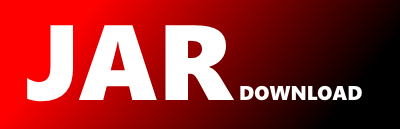
gu.simplemq.BaseMQContext Maven / Gradle / Ivy
The newest version!
package gu.simplemq;
import static com.google.common.base.Preconditions.checkNotNull;
public abstract class BaseMQContext implements IMQContext {
private final MessageQueueType mqType;
private final String clientImplType;
protected BaseMQContext(MessageQueueType mqType, String clientImplType) {
super();
this.mqType = checkNotNull(mqType,"checkNotNull is null");
this.clientImplType = checkNotNull(clientImplType,"clientImplType is null");
}
@Override
public final MessageQueueType getMessageQueueType() {
return mqType;
}
@Override
public final String getClientImplType(){
return clientImplType;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((clientImplType == null) ? 0 : clientImplType.hashCode());
result = prime * result + ((mqType == null) ? 0 : mqType.hashCode());
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (!(obj instanceof BaseMQContext))
return false;
BaseMQContext other = (BaseMQContext) obj;
if (clientImplType == null) {
if (other.clientImplType != null)
return false;
} else if (!clientImplType.equals(other.clientImplType))
return false;
if (mqType != other.mqType)
return false;
return true;
}
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
builder.append("BaseMQContext [mqType=");
builder.append(mqType);
builder.append(", clientImplType=");
builder.append(clientImplType);
builder.append("]");
return builder.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy