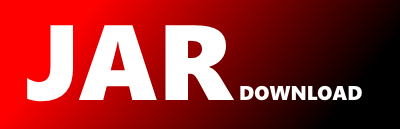
gu.simplemq.IProducerSingle Maven / Gradle / Ivy
The newest version!
package gu.simplemq;
import java.lang.reflect.Array;
import java.lang.reflect.Type;
import java.util.Collection;
import java.util.concurrent.BlockingDeque;
import com.google.common.reflect.TypeToken;
/**
* 生产者模型接口(单队列)
* @author guyadong
*
* @param
*/
public interface IProducerSingle {
public static abstract class AbstractHandler implements IProducerSingle {
/** 是否向队列末尾添加 */
protected boolean offerLast = true;
protected final Type type;
protected final Class> rawType;
public AbstractHandler(Type type) {
super();
this.type = type;
this.rawType = TypeToken.of(type).getRawType();
}
@Override
public int produce(@SuppressWarnings("unchecked") T...array){
int count = 0;
for(T t: array){
if(null != t){
produce(t);
++count;
}
}
return count;
}
@SuppressWarnings("unchecked")
@Override
public int produce(Collection c){
return null ==c?0:produce(c.toArray((T[])Array.newInstance(this.rawType, c.size())));
}
@Override
public IProducerSingle setOfferLast(boolean offerLast) {
this.offerLast = offerLast;
return this;
}
@Override
public int produce(boolean offerLast, @SuppressWarnings("unchecked") T... array) {
int count = 0;
for(T t: array){
if(null != t){
produce(t,offerLast);
++count;
}
}
return count;
}
@SuppressWarnings("unchecked")
@Override
public int produce(boolean offerLast, Collection c) {
return null ==c?0:produce(offerLast,c.toArray((T[])Array.newInstance(this.rawType, c.size())));
}
}
/**
* 向队列中压入数据
* @param t
* @param offerLast 为true向队列末尾添加
* 为false时队列头部添加,要求队列必须为双向队列{@link BlockingDeque}
*/
boolean produce(T t, boolean offerLast);
/**
* 向队列尾部添加数据
* @param t
*/
boolean produce(T t);
/**
* 向队列添加一组对象
* @param offerLast 为{@code true}向队列头部添加
* @param array
* @return 实际添加的对象数目
*/
int produce(boolean offerLast,@SuppressWarnings("unchecked") T... array);
/**
* 参见 {@link #produce(boolean, Object...)}
* @param offerLast
* @param c
*/
int produce(boolean offerLast,Collection c);
/**
* 向队列添加一组对象
* @param array
* @return 实际添加的对象数目
*/
int produce(@SuppressWarnings("unchecked") T... array);
/**
* 参见 {@link #produce(Object...)}
* @param c
*/
int produce(Collection c);
/**
* 设置是否向队列末尾添加
* @param offerLast
*/
IProducerSingle setOfferLast(boolean offerLast);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy