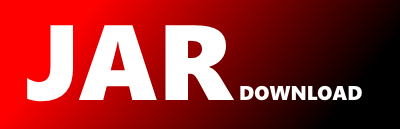
gu.sql2java.TableManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sql2java-base Show documentation
Show all versions of sql2java-base Show documentation
sql2java common class package
package gu.sql2java;
import java.util.List;
import gu.sql2java.exception.ObjectRetrievalException;
import gu.sql2java.exception.RuntimeDaoException;
import java.util.Collection;
/**
* Interface to handle database calls (save, load, count, etc...) for table.
* @author guyadong
*/
public interface TableManager extends SqlRunner{
public interface Action{
/**
* do action for {@code bean}
* @param bean input bean
*/
void call(B bean);
}
//_____________________________________________________________________
//
// COUNT
//_____________________________________________________________________
//24
/**
* Retrieves the number of rows of the table.
*
* @return the number of rows returned
* @throws RuntimeDaoException
*/
public int countAll()throws RuntimeDaoException;
//27
/**
* count the number of elements of a specific bean
*
* @param bean the bean to look for ant count
* @return the number of rows returned
* @throws RuntimeDaoException
*/
public int countUsingTemplate( B bean)throws RuntimeDaoException;
//20
/**
* count the number of elements of a specific bean given the search type
*
* @param bean the template to look for
* @param searchType exact ? like ? starting like ? ending link ?
* {@value Constant#SEARCH_EXACT} {@link Constant#SEARCH_EXACT}
* {@value Constant#SEARCH_LIKE} {@link Constant#SEARCH_LIKE}
* {@value Constant#SEARCH_STARTING_LIKE} {@link Constant#SEARCH_STARTING_LIKE}
* {@value Constant#SEARCH_ENDING_LIKE} {@link Constant#SEARCH_ENDING_LIKE}
* @return the number of rows returned
* @throws RuntimeDaoException
*/
public int countUsingTemplate(B bean, int searchType)throws RuntimeDaoException;
//25
/**
* Retrieves the number of rows of the table with a 'where' clause.
* It is up to you to pass the 'WHERE' in your where clauses.
*
* @param where the restriction clause
* @return the number of rows returned
* @throws RuntimeDaoException
*/
public int countWhere(String where)throws RuntimeDaoException;
//10
/**
* Deletes all rows from table.
* @return the number of deleted rows.
* @throws RuntimeDaoException
*/
public int deleteAll()throws RuntimeDaoException;
//11
/**
* Deletes rows from the table using a 'where' clause.
* It is up to you to pass the 'WHERE' in your where clauses.
*
Attention, if 'WHERE' is omitted it will delete all records.
*
* @param where the sql 'where' clause
* @return the number of deleted rows
* @throws RuntimeDaoException
*/
public int deleteByWhere(String where)throws RuntimeDaoException;
//21
/**
* Deletes rows using a template.
*
* @param bean the template object(s) to be deleted
* @return the number of deleted objects
* @throws RuntimeDaoException
*/
public int deleteUsingTemplate(B bean)throws RuntimeDaoException;
//2.1
/**
* Delete row according to its primary keys.
*
* @param keys primary keys value
* for fd_face table
* PK# 1 fd_face.id type Integer
* for fd_feature table
* PK# 1 fd_feature.md5 type String
* for fd_image table
* PK# 1 fd_image.md5 type String
* for fd_store table
* PK# 1 fd_store.md5 type String
* @return the number of deleted rows
* @throws RuntimeDaoException
*/
public int deleteByPrimaryKey(Object ...keys)throws RuntimeDaoException;
//2.2
/**
* Delete row according to primary keys of bean.
*
* @param bean will be deleted ,all keys must not be null
* @return the number of deleted rows,0 returned if bean is null
* @throws RuntimeDaoException
*/
public int delete(B bean)throws RuntimeDaoException;
//2.4
/**
* Delete beans.
*
* @param beans B array will be deleted
* @return the number of deleted rows
* @throws RuntimeDaoException
*/
@SuppressWarnings("unchecked")
public int delete(B... beans)throws RuntimeDaoException;
//2.5
/**
* Delete beans.
*
* @param beans B collection will be deleted
* @return the number of deleted rows
* @throws RuntimeDaoException
*/
public int delete(Collection beans)throws RuntimeDaoException;
//////////////////////////////////////
// LOAD ALL
//////////////////////////////////////
//5
/**
* Loads all the rows from table.
*
* @return an array of B bean
* @throws RuntimeDaoException
*/
public B[] loadAll()throws RuntimeDaoException;
//5-1
/**
* Loads each row from table and dealt with action.
* @param action Action object for do something(not null)
* @return the count dealt by action
* @throws RuntimeDaoException
*/
public int loadAll(Action action)throws RuntimeDaoException;
//6
/**
* Loads the given number of rows from table, given the start row.
*
* @param startRow the start row to be used (first row = 1, last row = -1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @return an array of B bean
* @throws RuntimeDaoException
*/
public B[] loadAll(int startRow, int numRows)throws RuntimeDaoException;
//6-1
/**
* Loads the given number of rows from table, given the start row and dealt with action.
* @param startRow the start row to be used (first row = 1, last row = -1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @param action Action object for do something(not null)
* @return the count dealt by action
* @throws RuntimeDaoException
*/
public int loadAll(int startRow, int numRows,Action action)throws RuntimeDaoException;
//5-2
/**
* Loads all the rows from table.
*
* @return a list of B bean
* @throws RuntimeDaoException
*/
public List loadAllAsList()throws RuntimeDaoException;
//6-2
/**
* Loads the given number of rows from table, given the start row.
*
* @param startRow the start row to be used (first row = 1, last row = -1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @return a list of B bean
* @throws RuntimeDaoException
*/
public List loadAllAsList(int startRow, int numRows)throws RuntimeDaoException;
//1.2
/**
* Loads a B bean from the table using primary key fields of {@code bean}.
* @param bean the B bean with primary key fields
* @return a unique B or {@code null} if not found or bean is null
* @throws RuntimeDaoException
*/
public B loadByPrimaryKey(B bean)throws RuntimeDaoException;
//1.2.2
/**
* see also {@link #loadByPrimaryKey(BaseBean)}
* @param bean
* @return a unique B ,otherwise throw exception
* @throws ObjectRetrievalException not found
* @throws RuntimeDaoException
*/
public B loadByPrimaryKeyChecked(B bean)throws RuntimeDaoException,ObjectRetrievalException;
//1.3
/**
* Loads a B bean from the table using primary key fields.
* when you don't know which is primary key of table,you can use the method.
* @param keys primary keys value:
* for fd_face table
* PK# 1 fd_face.id type Integer
* for fd_feature table
* PK# 1 fd_feature.md5 type String
* for fd_image table
* PK# 1 fd_image.md5 type String
* for fd_store table
* PK# 1 fd_store.md5 type String
* @return a unique B or {@code null} if not found
* @throws RuntimeDaoException
*/
public B loadByPrimaryKey(Object ...keys)throws RuntimeDaoException;
//1.3.2
/**
* see also {@link #loadByPrimaryKey(Object...)}
* @param keys
* @return a unique B,otherwise throw exception
* @throws ObjectRetrievalException not found
* @throws RuntimeDaoException
*/
public B loadByPrimaryKeyChecked(Object ...keys)throws RuntimeDaoException,ObjectRetrievalException;
//1.5
/**
* Returns true if this table contains row with primary key fields.
* @param keys primary keys value
* @see #loadByPrimaryKey(Object...)
* @return
* @throws RuntimeDaoException
*/
public boolean existsPrimaryKey(Object ...keys)throws RuntimeDaoException;
//1.6
/**
* Returns true if this table contains row specified by primary key fields of B.
* when you don't know which is primary key of table,you can use the method.
* @param bean the B bean with primary key fields
* @return
* @see #loadByPrimaryKey(BaseBean)
* @throws RuntimeDaoException
*/
public boolean existsByPrimaryKey(B bean)throws RuntimeDaoException;
//1.7
/**
* Check duplicated row by primary keys,if row exists throw exception
* @param bean the B bean with primary key fields
* @return always bean
* @see #existsByPrimaryKey(BaseBean)
* @throws ObjectRetrievalException has duplicated record
* @throws RuntimeDaoException
*/
public B checkDuplicate(B bean)throws RuntimeDaoException,ObjectRetrievalException;
//////////////////////////////////////
// SQL 'WHERE' METHOD
//////////////////////////////////////
//7
/**
* Retrieves an array of B given a sql 'where' clause.
*
* @param where the sql 'where' clause
* @return
* @throws RuntimeDaoException
*/
public B[] loadByWhere(String where)throws RuntimeDaoException;
//7-1
/**
* Retrieves each row of B bean given a sql 'where' clause and dealt with action.
* @param where the sql 'where' clause
* @param action Action object for do something(not null)
* @return the count dealt by action
* @throws RuntimeDaoException
*/
public int loadByWhere(String where,Action action)throws RuntimeDaoException;
//8
/**
* Retrieves an array of B bean given a sql where clause, and a list of fields.
* It is up to you to pass the 'WHERE' in your where clauses.
*
* @param where the sql 'WHERE' clause
* @param fieldList array of field's ID
* @return
* @throws RuntimeDaoException
*/
public B[] loadByWhere(String where, int[] fieldList)throws RuntimeDaoException;
//8-1
/**
* Retrieves each row of B bean given a sql where clause, and a list of fields,
* and dealt with action.
* It is up to you to pass the 'WHERE' in your where clauses.
* @param where the sql 'WHERE' clause
* @param fieldList array of field's ID
* @param action Action object for do something(not null)
* @return the count dealt by action
* @throws RuntimeDaoException
*/
public int loadByWhere(String where, int[] fieldList,Action action)throws RuntimeDaoException;
//9
/**
* Retrieves an array of B bean given a sql where clause and a list of fields, and startRow and numRows.
* It is up to you to pass the 'WHERE' in your where clauses.
*
* @param where the sql 'where' clause
* @param fieldList table of the field's associated constants
* @param startRow the start row to be used (first row = 1, last row = -1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @return
* @throws RuntimeDaoException
*/
public B[] loadByWhere(String where, int[] fieldList, int startRow, int numRows)throws RuntimeDaoException;
//9-1
/**
* Retrieves each row of B bean given a sql where clause and a list of fields, and startRow and numRows,
* and dealt with action.
* It is up to you to pass the 'WHERE' in your where clauses.
*
* @param where the sql 'where' clause
* @param fieldList table of the field's associated constants
* @param startRow the start row to be used (first row = 1, last row = -1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @param action Action object for do something(not null)
* @return the count dealt by action
* @throws RuntimeDaoException
*/
public int loadByWhere(String where, int[] fieldList, int startRow, int numRows,Action action)throws RuntimeDaoException;
//7
/**
* Retrieves a list of B bean given a sql 'where' clause.
*
* @param where the sql 'where' clause
* @return
* @throws RuntimeDaoException
*/
public List loadByWhereAsList(String where)throws RuntimeDaoException;
//8
/**
* Retrieves a list of B bean given a sql where clause, and a list of fields.
* It is up to you to pass the 'WHERE' in your where clauses.
*
* @param where the sql 'WHERE' clause
* @param fieldList array of field's ID
* @return
* @throws RuntimeDaoException
*/
public List loadByWhereAsList(String where, int[] fieldList)throws RuntimeDaoException;
//9-2
/**
* Retrieves a list of B bean given a sql where clause and a list of fields, and startRow and numRows.
* It is up to you to pass the 'WHERE' in your where clauses.
*
* @param where the sql 'where' clause
* @param fieldList table of the field's associated constants
* @param startRow the start row to be used (first row = 1, last row = -1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @return
* @throws RuntimeDaoException
*/
public List loadByWhereAsList(String where, int[] fieldList, int startRow, int numRows)throws RuntimeDaoException;
//9-3
/**
* Retrieves each row of B bean given a sql where clause and a list of fields, and startRow and numRows,
* and dealt wity action
* It is up to you to pass the 'WHERE' in your where clauses.
*
* @param where the sql 'where' clause
* @param fieldList table of the field's associated constants
* @param startRow the start row to be used (first row = 1, last row = -1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @param action Action object for do something(not null)
* @return the count dealt by action
* @throws RuntimeDaoException
*/
public int loadByWhereForAction(String where, int[] fieldList, int startRow, int numRows,Action action)throws RuntimeDaoException;
//_____________________________________________________________________
//
// USING TEMPLATE
//_____________________________________________________________________
//18
/**
* Loads a unique B bean from a template one giving a c
*
* @param bean the B bean to look for
* @return the bean matching the template,or {@code null} if not found or null input argument
* @throws ObjectRetrievalException more than one row
* @throws RuntimeDaoException
*/
public B loadUniqueUsingTemplate(B bean)throws RuntimeDaoException;
//18-1
/**
* Loads a unique B bean from a template one giving a c
*
* @param bean the B bean to look for
* @return the bean matching the template
* @throws ObjectRetrievalException not found or more than one row
* @throws RuntimeDaoException
*/
public B loadUniqueUsingTemplateChecked(B bean)throws RuntimeDaoException,ObjectRetrievalException;
//19
/**
* Loads an array of B from a template one.
*
* @param bean the B bean template to look for
* @return all the B beans matching the template
* @throws RuntimeDaoException
*/
public B[] loadUsingTemplate(B bean)throws RuntimeDaoException;
//19-1
/**
* Loads each row from a template one and dealt with action.
*
* @param bean the B bean template to look for
* @param action Action object for do something(not null)
* @return the count dealt by action
* @throws RuntimeDaoException
*/
public int loadUsingTemplate(B bean,Action action)throws RuntimeDaoException;
//20
/**
* Loads an array of B bean from a template one, given the start row and number of rows.
*
* @param bean the B bean template to look for
* @param startRow the start row to be used (first row = 1, last row=-1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @return all the B matching the template
* @throws RuntimeDaoException
*/
public B[] loadUsingTemplate(B bean, int startRow, int numRows)throws RuntimeDaoException;
//20-1
/**
* Loads each row from a template one, given the start row and number of rows and dealt with action.
*
* @param bean the B bean template to look for
* @param startRow the start row to be used (first row = 1, last row=-1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @param action Action object for do something(not null)
* @return the count dealt by action
* @throws RuntimeDaoException
*/
public int loadUsingTemplate(B bean, int startRow, int numRows,Action action)throws RuntimeDaoException;
//20-5
/**
* Loads each row from a template one, given the start row and number of rows and dealt with action.
*
* @param bean the B template to look for
* @param fieldList table of the field's associated constants
* @param startRow the start row to be used (first row = 1, last row=-1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @param searchType exact ? like ? starting like ? ending link ?
* {@value Constant#SEARCH_EXACT} {@link Constant#SEARCH_EXACT}
* {@value Constant#SEARCH_LIKE} {@link Constant#SEARCH_LIKE}
* {@value Constant#SEARCH_STARTING_LIKE} {@link Constant#SEARCH_STARTING_LIKE}
* {@value Constant#SEARCH_ENDING_LIKE} {@link Constant#SEARCH_ENDING_LIKE}
* @param action Action object for do something(not null)
* @return the count dealt by action
* @throws RuntimeDaoException
*/
public int loadUsingTemplate(B bean, int[] fieldList, int startRow, int numRows,int searchType, Action action)throws RuntimeDaoException;
//20-4
/**
* Loads a list of B bean from a template one, given the start row and number of rows.
*
* @param bean the B bean template to look for
* @param startRow the start row to be used (first row = 1, last row=-1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @param searchType exact ? like ? starting like ? ending link ?
* {@value Constant#SEARCH_EXACT} {@link Constant#SEARCH_EXACT}
* {@value Constant#SEARCH_LIKE} {@link Constant#SEARCH_LIKE}
* {@value Constant#SEARCH_STARTING_LIKE} {@link Constant#SEARCH_STARTING_LIKE}
* {@value Constant#SEARCH_ENDING_LIKE} {@link Constant#SEARCH_ENDING_LIKE}
* @return all the B bean matching the template
* @throws RuntimeDaoException
*/
public B[] loadUsingTemplate(B bean, int startRow, int numRows, int searchType)throws RuntimeDaoException;
//19-2
/**
* Loads a list of B bean from a template one.
*
* @param bean the B bean template to look for
* @return all the B beans matching the template
* @throws RuntimeDaoException
*/
public List loadUsingTemplateAsList(B bean)throws RuntimeDaoException;
//20-2
/**
* Loads a list of B bean from a template one, given the start row and number of rows.
*
* @param bean the B bean template to look for
* @param startRow the start row to be used (first row = 1, last row=-1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @return all the B bean matching the template
* @throws RuntimeDaoException
*/
public List loadUsingTemplateAsList(B bean, int startRow, int numRows)throws RuntimeDaoException;
//20-3
/**
* Loads an array of B bean from a template one, given the start row and number of rows.
*
* @param bean the B bean template to look for
* @param startRow the start row to be used (first row = 1, last row=-1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @param searchType exact ? like ? starting like ? ending link?
* {@value Constant#SEARCH_EXACT} {@link Constant#SEARCH_EXACT}
* {@value Constant#SEARCH_LIKE} {@link Constant#SEARCH_LIKE}
* {@value Constant#SEARCH_STARTING_LIKE} {@link Constant#SEARCH_STARTING_LIKE}
* {@value Constant#SEARCH_ENDING_LIKE} {@link Constant#SEARCH_ENDING_LIKE}
* @return all the B beans matching the template
* @throws RuntimeDaoException
*/
public List loadUsingTemplateAsList(B bean, int startRow, int numRows, int searchType)throws RuntimeDaoException;
//_____________________________________________________________________
//
// LISTENER
//_____________________________________________________________________
//35
/**
* Registers a unique {@link TableListener} listener.
* do nothing if {@code TableListener} instance exists
* @param listener
*/
public void registerListener(TableListener listener);
//36
/**
* remove listener.
* @param listener
*/
public void unregisterListener(TableListener listener);
//_____________________________________________________________________
//
// SAVE
//_____________________________________________________________________
//12
/**
* Saves the B bean into the database.
*
* @param bean the B bean to be saved
* @return the inserted or updated bean,or null if bean is null
* @throws RuntimeDaoException
*/
public B save(B bean)throws RuntimeDaoException;
//15
/**
* Saves an array of B bean into the database.
*
* @param beans the array of B bean to be saved
* @return always beans saved
* @throws RuntimeDaoException
*/
public B[] save(B[] beans)throws RuntimeDaoException;
//15-2
/**
* Saves a collection of B bean into the database.
*
* @param beans the B bean table to be saved
* @return alwarys beans saved
* @throws RuntimeDaoException
*/
public > C saveAsTransaction(C beans)throws RuntimeDaoException;
//15-3
/**
* Saves an array of B bean into the database as transaction.
*
* @param beans the B bean table to be saved
* @return alwarys beans saved
* @see #save(BaseBean[])
* @throws RuntimeDaoException
*/
public B[] saveAsTransaction(B[] beans)throws RuntimeDaoException;
//15-4
/**
* Saves a collection of B bean into the database as transaction.
*
* @param beans the B bean table to be saved
* @return alwarys beans saved
* @throws RuntimeDaoException
*/
public > C save(C beans)throws RuntimeDaoException;
/**
* Load column from table.
* @param column column name or java file name of B
* @param distinct select distinct values
* @param where the sql 'where' clause
* @param startRow the start row to be used (first row = 1, last row = -1)
* @param numRows the number of rows to be retrieved (all rows = a negative number)
* @return an list of column
* @throws RuntimeDaoException
*/
public List loadColumnAsList(String column,boolean distinct,String where,int startRow,int numRows)throws RuntimeDaoException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy