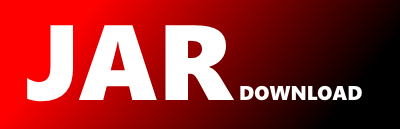
gu.sql2java.RowMapView Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sql2java-base Show documentation
Show all versions of sql2java-base Show documentation
sql2java common class package
package gu.sql2java;
import java.util.AbstractMap;
import java.util.Collections;
import java.util.Set;
import com.google.common.collect.Sets;
import static com.google.common.base.Preconditions.*;
class RowMapView extends AbstractMap {
private final BaseRow row;
private final Set> entrySet;
public RowMapView(BaseRow bean) {
this.row = checkNotNull(bean,"bean is null");
Set> s = Sets.newLinkedHashSet();
for(int i =0; i < bean.metaData.columnCount; ++i){
s.add(new RowEntry(i));
}
entrySet = Collections.unmodifiableSet(s);
}
@Override
public Set> entrySet() {
return entrySet;
}
@Override
public Object put(String key, Object value) {
int columnId = row.metaData.columnIDOf(key);
checkArgument(columnId >=0, "INVALID column name %s",key);
Object old = row.getValue(columnId);
row.setValue(columnId, value);
return old;
}
private class RowEntry implements Entry{
private final int columnId;
public RowEntry(int columnId) {
super();
this.columnId = columnId;
}
@Override
public String getKey() {
return row.metaData.columnNames.get(columnId);
}
@Override
public Object getValue() {
return row.getValue(columnId);
}
@Override
public Object setValue(Object value) {
Object old = row.getValue(columnId);
row.setValue(columnId, value);
return old;
}}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy