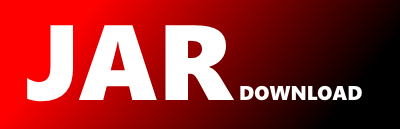
gu.sql2java.ArraySupport Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sql2java-base Show documentation
Show all versions of sql2java-base Show documentation
sql2java common class package
package gu.sql2java;
import static com.google.common.base.Preconditions.checkArgument;
import com.google.common.io.BaseEncoding;
public class ArraySupport {
private static final String HEX_REGEX = "^([a-fA-F0-9]{2})+$";
/**
* 字符串验证器,根据正则表达式判断字符串是否为十六进制(HEX)字符串
* 输入为null或空或正则表达式不匹配则返回false
*/
private static final boolean validHEX(String input){
return input != null && (input.length()&1) == 0 && input.matches(HEX_REGEX);
}
/**
* cast byte array to HEX string
*
* @param input
* @return hex string or null if not byte[]
*/
public static final String toHex(byte[] input) {
if (input instanceof byte[]){
return BaseEncoding.base16().encode(input);
}
return null;
}
/**
* cast integral Number to HEX string
* @param input
* @return hex string or null if not Number
* @since 4.2.0
*/
public static final String toHex(Number input){
if(input instanceof Integer) {
return String.format("%08X",input.intValue());
}else if(input instanceof Long) {
return String.format("%016X",input.longValue());
}else if(input instanceof Short) {
return String.format("%04X",input.shortValue());
}else if(input instanceof Byte) {
return String.format("%02X",input.byteValue());
}else if(null != input) {
return String.format("%08X",input.intValue());
}
return null;
}
/**
* cast byte array or number to HEX string
* @param input
* @return hex string or null if not byte[] or Number.
* @since 4.2.0
*/
public static final String toHex(Object input) {
if (input instanceof byte[]){
return toHex((byte[])input);
}else if(input instanceof Number) {
return toHex((Number)input);
}else if(input instanceof String) {
String s = (String)input;
if(validHEX(s)) {
return s;
}
}
return null;
}
/**
* cast HEX string to byte array
* @param input
* @return {@code null} if {@code input} is null
*/
public static final byte[] fromHex(String input){
if (null == input){
return null;
}
return BaseEncoding.base16().decode(input);
}
/**
* cast HEX string to byte array,copy to this destination,same length required with {@code dest}
* @param hex
* @param dest
*/
public static void copyToByteArray(String hex, byte[] dest){
checkArgument(null != hex && null != dest,"hex or dest is null");
byte[] src = fromHex(hex);
checkArgument(src.length == dest.length,"INVALID HEX String length, %s required",dest.length);
System.arraycopy(src, 0, dest, 0, src.length);
}
/**
* return {@code true} if bit specialized by {@code index} in byte array {@code bits} is 1,otherwise {@code false}
* @param bits
* @param index
* @since 4.0.0
*/
public static final boolean bitCheck(byte[]bits,int index){
return 0 != (bits[bits.length - 1 - (index>>3)]&(byte)(1<<(index&0x07)));
}
/**
* set bit specialized by {@code index} in byte array {@code bits} to 1
* @param bits
* @param index
* @return always {@code bits}
* @since 4.0.0
*/
public static final byte[] bitSet(byte[] bits,int index){
bits[bits.length - 1 - (index>>3)] |= (byte)(1<<(index&0x07));
return bits;
}
/**
* set bit specialized by {@code index} in byte array {@code bits} to 0
* @param bits
* @param index
* @return always {@code bits}
* @since 4.0.0
*/
public static final byte[] bitReset(byte[] bits,int index){
bits[bits.length - 1 - (index>>3)] &= (byte)(~(1<<(index&0x07)));
return bits;
}
/**
* set bit specialized by {@code index} in byte array {@code bits} to 0 if {@code set1} is {@code true},otherwise set to 0
* @param bits
* @param index
* @param set1
* @return always {@code bits}
* @since 4.0.0
*/
public static final byte[] bitSet(byte[] bits,int index,boolean set1){
return set1 ? bitSet(bits, index) : bitReset(bits, index);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy