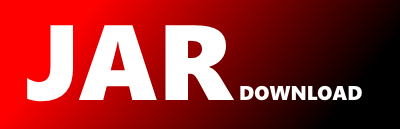
gu.sql2java.BaseColumnCodec Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sql2java-base Show documentation
Show all versions of sql2java-base Show documentation
sql2java common class package
The newest version!
package gu.sql2java;
import static com.google.common.base.Preconditions.checkNotNull;
import java.lang.reflect.Type;
import com.google.common.reflect.TypeToken;
import gu.sql2java.exception.ResultSetCodecException;
import gu.sql2java.exception.UnsupportTypeException;
/**
* @author guyadong
* @since 3.21.0
*/
public abstract class BaseColumnCodec implements ColumnCodec {
protected BaseColumnCodec() {
}
protected abstract T doDeserialize(Object columnValue, Class targetType) throws ResultSetCodecException;
protected abstract T doDeserialize(Object columnValue, Type targetType) throws ResultSetCodecException;
protected abstract T doSerialize(Object obj, Class targetType) throws ResultSetCodecException;
@SuppressWarnings("unchecked")
@Override
public T deserialize(Object columnValue, Class targetType) throws ResultSetCodecException {
if(null == columnValue) {
if(null != targetType && targetType.isPrimitive()) {
throw new UnsupportTypeException(
"targetType must not be primitive " + targetType.getName()+ " if columnValue is null");
}
return null;
}else if(checkNotNull(targetType,"targetType is null").isInstance(columnValue)) {
return (T)columnValue;
}
return doDeserialize(columnValue,targetType);
}
@SuppressWarnings("unchecked")
public T deserialize(Object columnValue, Type targetType) throws ResultSetCodecException {
if(null == columnValue) {
if(null != targetType && TypeToken.of(targetType).getRawType().isPrimitive()) {
throw new UnsupportTypeException(
"targetType must not be primitive " + targetType.toString()+ " if columnValue is null");
}
return null;
}else if(TypeToken.of(checkNotNull(targetType,"targetType is null")).getRawType().isInstance(columnValue)) {
return (T)columnValue;
}
return doDeserialize(columnValue,targetType);
}
@SuppressWarnings("unchecked")
@Override
public T serialize(Object obj, Class targetType) throws ResultSetCodecException {
if(null == obj) {
if(null != targetType && targetType.isPrimitive()) {
throw new UnsupportTypeException(
"targetType must not be primitive " + targetType.getName() + " if obj is null");
}
return null;
}else if(checkNotNull(targetType,"targetType is null").isInstance(obj)) {
return (T) obj;
}
return doSerialize(obj, targetType);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy