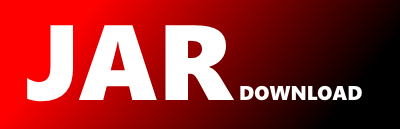
org.apache.commons.beanutils.MapPropertyDescriptor Maven / Gradle / Ivy
package org.apache.commons.beanutils;
import java.beans.IntrospectionException;
import java.beans.PropertyDescriptor;
import java.lang.reflect.Method;
import java.util.Map;
import com.google.common.base.Throwables;
import static com.google.common.base.Preconditions.checkNotNull;
import static com.google.common.base.MoreObjects.firstNonNull;
/**
* 实现对{@link Map}实例的属性封装
* @author guyadong
*
*/
public class MapPropertyDescriptor extends PropertyDescriptor implements MorePropertyDescriptor {
private final Method readMethod;
private final Method writeMethod;
private final Class> propertyType;
public MapPropertyDescriptor(Class> propertyType, String name)
throws IntrospectionException {
/** 将属性名中的.替换为_,以避免对nested name解析出错 */
super(checkNotNull(name,"field is null").replace(".", "_"), null, null);
setDisplayName(name);
this.propertyType = firstNonNull(propertyType, Object.class);
try {
readMethod = MapPropertyDescriptor.class.getMethod("readMethod", Object.class,String.class);
writeMethod = MapPropertyDescriptor.class.getMethod("writeMethod", Object.class,String.class,Object.class);
} catch (Exception e) {
Throwables.throwIfUnchecked(e);
throw new RuntimeException(e);
}
}
/**
* 字段读取方法实现
* @param bean 读取目标对象
* @param name 字段名
* @return 字段值
*/
@SuppressWarnings("rawtypes")
public static Object readMethod(Object bean,String name){
if(bean instanceof Map){
return ((Map)bean).get(name);
}
return null;
}
/**
* 字段写入方法实现
* @param bean 写入目标对象
* @param name 字段名
* @param value 要写入的值
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
public static void writeMethod(Object bean,String name,Object value){
if(bean instanceof Map){
((Map)bean).put(name, value);
}
}
@Override
public Method getReadMethod() {
return readMethod;
}
@Override
public Method getWriteMethod() {
return writeMethod;
}
@Override
public Class> getPropertyType() {
return propertyType;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy