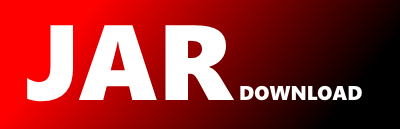
templates.velocity.java5g.perschema.bean.converter.utils.vm Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sql2java-generator Show documentation
Show all versions of sql2java-generator Show documentation
executable jar of sql2java generator
#parse( "schema.include.vm" )
#parse( "header.include.vm" )
#parse( "macros.include.vm" )
#set ( $javaClassName = 'BeanConverterUtils' )
#set( $ignorefields= $codewriter.getProperty('general.beanconverter.tonative.ignore') )
$codewriter.setCurrentJavaFilename($pkg, "${javaClassName}.java")
package ${pkg};
import java.io.PrintWriter;
import java.io.StringWriter;
import java.lang.reflect.Method;
import java.nio.ByteBuffer;
import java.util.Map;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Hashtable;
import java.util.List;
import gu.sql2java.IBeanConverter;
#foreach ( $table in $tables )
import ${pkg}.${table.asBeanClassNSP()};
#end
/**
* generic type converter classes of {@link IBeanConverter} implementation for #join($tables "$e.name" ',')
* @author guyadong
*
*/
public class ${javaClassName} implements Constant {
public static final String GET_INITIALIZED = "getInitialized";
public static final String SET_INITIALIZED = "setInitialized";
public static final String GET_MODIFIED = "getModified";
public static final String SET_MODIFIED = "setModified";
public static final String SET_NEW = "setNew";
public static final String IS_NEW = "isNew";
private static class NullCastPrimitiveException extends ClassCastException {
private static final long serialVersionUID = 1L;
NullCastPrimitiveException(String message) {
super(message);
}
}
private ${javaClassName}(){}
/**
* 返回buffer中所有字节(position~limit),不改变buffer状态
* @param buffer
* @return
*/
private static final byte[] getBytesInBuffer(ByteBuffer buffer){
int pos = buffer.position();
try{
byte[] bytes = new byte[buffer.remaining()];
buffer.get(bytes);
return bytes;
}finally{
buffer.position(pos);
}
}
private static final List toList(long[] array) {
ArrayList result = new ArrayList(array.length);
for (int i = 0; i < array.length; i++) {
result.add(new Long(array[i]));
}
return result;
}
private static final long[] toPrimitive(List list) {
long[] dst = new long[list.size()];
Long element;
for (int i = 0; i < dst.length; i++) {
if(null == (element = list.get(i))){
throw new IllegalArgumentException("can't cast List to long[] because of null element");
}
dst[i] = element.longValue();
}
return dst;
}
/**
* {@code source}转为{@code type}指定的类型
* @param type destination type
* @param source source object
* @return
*/
@SuppressWarnings({ "unchecked" })
private static final T cast(Class type,Object source){
try{
if(null ==source && type.isPrimitive()){
throw new NullCastPrimitiveException(String.format("can't convert null to primitive type %s",type.getSimpleName()));
}
return (T) source;
}catch(ClassCastException cce){
// long[] -> List
if(List.class.isAssignableFrom(type) && null != source && source instanceof long[]){
return (T) toList((long[]) source);
}
// List -> long[]
if(long[].class == type && null != source && source instanceof List){
return (T) toPrimitive( (List) source);
}
// Long -> Date
if(java.util.Date.class.isAssignableFrom(type) && null != source && source instanceof Long){
try {
// call constructor,such as java.util.Date#Date(long), java.sql.Time.Time(long)
return type.getConstructor(long.class).newInstance(source);
} catch (Exception e) {
StringWriter writer = new StringWriter();
e.printStackTrace(new PrintWriter(writer));
throw new ClassCastException(writer.toString());
}
}
// Date -> Long,long
if( long.class == type || Long.class == type){
if(null != source && source instanceof java.util.Date){
Long time = ((java.util.Date)source).getTime();
return (T)time;
}
}
// byte[] -> ByteBuffer
if(ByteBuffer.class == type && null != source && source instanceof byte[]){
return (T) ByteBuffer.wrap((byte[]) source);
}
// ByteBuffer -> byte[]
if(byte[].class == type && null != source && source instanceof ByteBuffer){
return (T) getBytesInBuffer((ByteBuffer) source);
}
throw cce;
}
}
private static final boolean bitCheck(int index,${codewriter.bitStateClassName}...bits){
return 0 != (bits[index>>STATE_BIT_SHIFT]&(1$!{codewriter.bitStateConstSuffix}<<(index&${codewriter.bitStateMaskHex})));
}
private static final ${codewriter.bitStateClassName}[] bitOR(int index,${codewriter.bitStateClassName}... bits){
bits[index>>STATE_BIT_SHIFT] |= (1$!{codewriter.bitStateConstSuffix}<<(index&${codewriter.bitStateMaskHex}));
return bits;
}
#foreach ( $table in $tables )
#set ( $leftClass = "${table.asBeanClassNSP()}" )
#set ( $rightClass = "R_${table.asCoreClassNSP().toUpperCase()}" )
/**
* implementation of {@link IBeanConverter} by reflect
* generic type converter between {@link $leftClass} and $rightClass
* @author guyadong
* @param <$rightClass> right type
*
*/
public static class #converterClass(${leftClass})<$rightClass> extends IBeanConverter.AbstractHandle<${leftClass},${rightClass}>{
static enum Column{
/** column method info */
#join($table.columns '${e.varName}("$e.getGetMethod()","$e.getSetMethod()")' ',
');
final String getter;
final String setter;
Column(String getter,String setter){
this.getter = setter;
this.setter = setter;
}
}
private final Map methods = new Hashtable();
private final Map rightIndexs = new Hashtable();
private final Map> setterParams = new Hashtable>();
private boolean bitCheck(String name,${codewriter.bitStateClassName}...bits){
Integer id = rightIndexs.get(name);
return (null == id)?false:BeanConverterUtils.bitCheck(id.intValue(),bits);
}
private ${codewriter.bitStateClassName}[] bitOR(String name,${codewriter.bitStateClassName}... bits){
return BeanConverterUtils.bitOR(rightIndexs.get(name),bits);
}
private void getGetter(String name){
try{
methods.put(name,rightType.getMethod(name));
}catch(NoSuchMethodException e){}
}
private void getSetter(String name, Class>...types) throws NoSuchMethodException{
for(Class>paramType:types){
try{
methods.put(name,rightType.getMethod(name,paramType));
setterParams.put(name, paramType);
return;
}catch(NoSuchMethodException e){
continue;
}
}
throw new NoSuchMethodException();
}
private void getSetterNoThrow(String name, Class>...types){
try{
getSetter(name,types);
}catch(NoSuchMethodException e){}
}
/**
* usage: new #converterClass(${leftClass})<Model>(javaFields){};
* @param javaFields a comma splice string,including all field name of $rightClass,
* if null or empty, use default string:{@link Constant#$table.name.toUpperCase()_JAVA_FIELDS}
*/
public #converterClass(${leftClass})(String javaFields){
super();
init(javaFields);
}
/** @see ${esc.hash}#converterClass(${leftClass})(String) */
public #converterClass(${leftClass})(){
this(null);
}
/**
* constructor
* @param leftClass
* @param rightClass
* @param javaFields see also {@link ${esc.hash}#converterClass(${leftClass})(String)}
*/
public #converterClass(${leftClass}) (Class<${leftClass}> leftClass, Class<$rightClass> rightClass,String javaFields){
super(leftClass,rightClass);
init(javaFields);
}
/** @see ${esc.hash}#converterClass(${leftClass})(Class,Class,String) */
public #converterClass(${leftClass}) (Class<${leftClass}> leftClass, Class<$rightClass> rightClass){
this(leftClass,rightClass,null);
}
private void init(String javaFields){
if(null == javaFields || javaFields.isEmpty()){
javaFields = $table.name.toUpperCase()_JAVA_FIELDS;
}
String []rightFields = javaFields.split(",");
for(int i = 0 ; i < rightFields.length; ++i){
String field = rightFields[i].trim();
if(!field.matches("\\w+")){
throw new IllegalArgumentException("invalid 'javaFields':" + javaFields);
}
rightIndexs.put(field,i);
}
try{
methods.put(IS_NEW,rightType.getMethod(IS_NEW));
methods.put(GET_INITIALIZED,rightType.getMethod(GET_INITIALIZED));
getSetter(SET_NEW,boolean.class);
if(rightIndexs.size() > STATE_BIT_NUM){
getSetter(SET_INITIALIZED,${codewriter.bitStateClassName}[].class,List.class);
}else{
getSetter(SET_INITIALIZED,${codewriter.bitStateClassName}.class);
}
getGetter(GET_MODIFIED);
if(rightIndexs.size() > STATE_BIT_NUM){
getSetter(SET_MODIFIED,${codewriter.bitStateClassName}[].class,List.class);
}else{
getSetter(SET_MODIFIED,${codewriter.bitStateClassName}.class);
}
}catch(NoSuchMethodException e){
throw new RuntimeException(e);
}
#foreach ( $column in $table.columns )
#set ( $setterName = "Column.${column.varName}.setter")
#set ( $javaType = ${column.getJavaType()})
#set ( $javaPrimaryType = ${column.getJavaPrimaryType()})
getGetter(Column.${column.varName}.getter);
#if ( $column.hasPrimaryType())
#if($column.isDate())
getSetterNoThrow($setterName,${javaType}.class,Long.class,long.class);
#else
getSetterNoThrow($setterName,${javaType}.class,${javaPrimaryType}.class);
#end
#elseif($column.isBinary())
getSetterNoThrow($setterName,java.nio.ByteBuffer.class,byte[].class);
#else
getSetterNoThrow($setterName,${javaType}.class);
#end
#end###foreach ( $column in $table.columns )
}
@Override
protected void doFromRight(${leftClass} left, ${rightClass} right) {
try{
Method getterMethod;
left.resetIsModified();
$table.stateVarType() selfModified = $table.maskInitializeWithZero();
${codewriter.bitStateClassName}[] initialized;
${codewriter.bitStateClassName}[] modified;
if(rightIndexs.size() > STATE_BIT_NUM){
initialized = (${codewriter.bitStateClassName}[])methods.get(GET_INITIALIZED).invoke(right);
modified = (${codewriter.bitStateClassName}[])methods.get(GET_MODIFIED).invoke(right);
}else{
initialized = new ${codewriter.bitStateClassName}[]{(${codewriter.bitStateClassWrapName})methods.get(GET_INITIALIZED).invoke(right)};
modified = new ${codewriter.bitStateClassName}[]{(${codewriter.bitStateClassWrapName})methods.get(GET_MODIFIED).invoke(right)};
}
#foreach ( $column in $table.columns )
#set ($name = "Column.${column.varName}.name()")
if( bitCheck($name,initialized) && (null != (getterMethod = methods.get(Column.${column.varName}.getter)))){
left.$column.getSetMethod()(cast(${column.getJavaType()}.class,getterMethod.invoke(right)));
if(bitCheck($name,modified)){
$column.bitORAssignExpression("selfModified");
}
}
#end###foreach ( $column in $table.columns )
left.setNew((Boolean)methods.get(IS_NEW).invoke(right));
left.setModified(selfModified);
}catch(RuntimeException e){
throw e;
}catch(Exception e){
throw new RuntimeException(e);
}
}
@Override
protected void doToRight(${leftClass} left, ${rightClass} right) {
try{
Method setterMethod;
${codewriter.bitStateClassName}[] initialized = new ${codewriter.bitStateClassName}[(rightIndexs.size() + STATE_BIT_NUM - 1)>>STATE_BIT_SHIFT];
${codewriter.bitStateClassName}[] modified = new ${codewriter.bitStateClassName}[(rightIndexs.size() + STATE_BIT_NUM - 1)>>STATE_BIT_SHIFT];
Arrays.fill(initialized, 0$!{codewriter.bitStateConstSuffix});
Arrays.fill(modified, 0$!{codewriter.bitStateConstSuffix});
#foreach ( $column in $table.columns )
#set ($name = "Column.${column.varName}.name()")
#set ( $setterName = "Column.${column.varName}.setter")
#set($isIgnore = $ignorefields.contains($column.name))
#if( $isIgnore )// IGNORE field $column.fullName , controlled by 'general.beanconverter.tonative.ignore' in properties file
/*
#end
if(null != (setterMethod = methods.get(Column.${column.varName}.setter)) && left.${column.getInitializedMethod()}()){
try{
setterMethod.invoke(right,cast(setterParams.get($setterName),left.${column.getGetMethod()}()));
bitOR($name,initialized);
if(left.${column.getModifiedMethod()}()){
bitOR($name,modified);
}
}catch(NullCastPrimitiveException e){}
}
#if( $isIgnore )*/
#end
#end###foreach ( $column in $table.columns )
if(null != (setterMethod = methods.get(SET_MODIFIED))){
if( initialized.length > 1){
setterMethod.invoke(right,cast(setterParams.get(SET_MODIFIED),initialized));
}else{
setterMethod.invoke(right,initialized[0]);
}
}
methods.get(SET_NEW).invoke(right,left.isNew());
if( initialized.length > 1){
methods.get(SET_INITIALIZED).invoke(right,cast(setterParams.get(SET_INITIALIZED),initialized));
methods.get(SET_MODIFIED).invoke(right,cast(setterParams.get(SET_MODIFIED),modified));
}else{
methods.get(SET_INITIALIZED).invoke(right,initialized[0]);
methods.get(SET_MODIFIED).invoke(right,modified[0]);
}
}catch(RuntimeException e){
throw e;
}catch(Exception e){
throw new RuntimeException(e);
}
}
};
#end###foreach ( $table in $tables )
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy