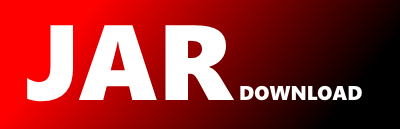
templates.velocity.java5g.pertable.bean.vm Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sql2java-generator Show documentation
Show all versions of sql2java-generator Show documentation
executable jar of sql2java generator
##included template: bean.include.vm
#parse( "header.include.vm" )
#parse( "table.include.vm" )
#parse( "macros.include.vm" )
#set ( $beanClass = $table.asBeanClass($isGeneral) )
#set ( $beanClassFullName = "${table.package}.${beanClass}" )
$codewriter.setCurrentJavaFilename(${table.package}, "${beanClass}.java")
package ${table.package};
import java.io.Serializable;
import java.util.Objects;
import gu.sql2java.BaseRow;
#swiftImport()
#swaggerImport()
/**
* $beanClass is a mapping of $table.getName() Table.
#if ( $table.getRemarks().length() > 0 )
*
Meta Data Information (in progress):
*
* - comments: $table.getRemarks()
*
#end
* @author guyadong
*/
#swiftThriftStruct()
#swaggerModel($table.getRemarks())
#set( $fieldIndex = 1 )
public $!{final} class ${beanClass} extends BaseRow
implements Serializable,Constant
#if ($implementsClasses)
#foreach( $implements in $implementsClasses )$implements #end
#end
{
private static final long serialVersionUID = ${table.getSerialVersionUID($beanClassFullName)}L;
#foreach ( $column in $columns )
#if ( $column.getRemarks().length() > 0 )
/** comments:$column.getRemarks() */
#end
#swaggerColumn($column)
private $column.getJavaType() $column.getVarName();
#end
/** columns modified flag */
#swaggerField("columns modified flag","$table.stateVarType()",true)
private $table.stateVarType() modified;
/** columns initialized flag */
#swaggerField("columns initialized flag","$table.stateVarType()",true)
private $table.stateVarType() initialized;
/** new record flag */
#swaggerField("new record flag","boolean",true)
private boolean isNew;
#if( $swiftBeanSupport && $table.stateVarTypeIsArray() )
private static final java.util.List<${codewriter.bitStateClassWrapName}> toList(${codewriter.bitStateClassName}[] array) {
java.util.ArrayList<${codewriter.bitStateClassWrapName}> result = new java.util.ArrayList<${codewriter.bitStateClassWrapName}>(array.length);
for (int i = 0; i < array.length; i++) {
result.add(new ${codewriter.bitStateClassWrapName}(array[i]));
}
return result;
}
private static final void toPrimitive(java.util.List<${codewriter.bitStateClassWrapName}> list,${codewriter.bitStateClassName}[]dst) {
if ( null == list || list.size() != dst.length) {
return;
}
Long element;
for (int i = 0; i < dst.length; i++) {
if(null != (element = list.get(i)))
dst[i] = element.${codewriter.bitStateClassName}Value();
}
}
#end
################## IMMUTABLE STATUS ###########
#swiftThriftField( $fieldIndex 'Requiredness.REQUIRED' '_new')
@Override
public boolean isNew()
{
return this.isNew;
}
/**
* Specifies to the object if it has been set as new.
*
* @param isNew the boolean value to be assigned to the isNew field
*/
#swiftThriftField( $null $null $null)
@Override
public void setNew(boolean isNew)
{
this.isNew = isNew;
}
/**
* @return the modified status of columns
*/
#set( $fieldIndex = $fieldIndex + 1 )
#swiftThriftField( $fieldIndex 'Requiredness.REQUIRED' $null)
@Override
#if( $swiftBeanSupport && $table.stateVarTypeIsArray() )
public java.util.List<${codewriter.bitStateClassWrapName}> getModified(){
return toList(modified);
}
#else
public $table.stateVarType() getModified(){
return modified;
}
#end
/**
* @param modified the modified status bit to be assigned to {@link #modified}
*/
#swiftThriftField( $null $null $null)
@Override
#if( $swiftBeanSupport && $table.stateVarTypeIsArray() )
public void setModified(List<${codewriter.bitStateClassWrapName}> modified){
toPrimitive(modified,this.modified);
}
#else
public void setModified($table.stateVarType() modified){
$table.stateVarAssignStatement("modified","this.modified");
}
#end
/**
* @return the initialized status of columns
*/
#set( $fieldIndex = $fieldIndex + 1 )
#swiftThriftField( $fieldIndex 'Requiredness.REQUIRED' $null)
@Override
#if( $swiftBeanSupport && $table.stateVarTypeIsArray() )
public java.util.List<${codewriter.bitStateClassWrapName}> getModified(){
return toList(initialized);
}
#else
public $table.stateVarType() getInitialized(){
return initialized;
}
#end
/**
* @param initialized the initialized status bit to be assigned to {@link #initialized}
*/
#swiftThriftField( $null $null $null)
@Override
#if( $swiftBeanSupport && $table.stateVarTypeIsArray() )
public void setInitialized($table.stateVarType() initialized){
toPrimitive(initialized,this.initialized);
}
#else
public void setInitialized($table.stateVarType() initialized){
$table.stateVarAssignStatement("initialized","this.initialized");
}
#end
public ${beanClass}(){
reset();
}
#if ( $table.hasPrimaryKey() )
/**
* construct a new instance filled with primary keys
* #join($primaryKeys '@param $e.getVarName() PK# $velocityCount ' '
')
*/
public ${beanClass}(#join($primaryKeys '$e.getJavaType() $e.getVarName()' ',')){
#join($primaryKeys '$e.getSetMethod()($e.getVarName());' '
')
}
#end
#foreach ( $column in $columns )
#set( $fieldIndex = $fieldIndex + 1 )
/**
* Getter method for {@link #$column.getVarName()}.
#if ( $column.isPrimaryKey() )
* PRIMARY KEY.
#end
* Meta Data Information (in progress):
*
* - full name: $column.getFullName()
#foreach ( $fKey in $column.getForeignKeys() )
* - foreign key: ${fKey.getTableName()}.${fKey.getName()}
#end
#foreach ( $iKey in $column.getImportedKeys() )
* - imported key: ${iKey.getTableName()}.${iKey.getName()}
#end
#if ( !$column.getRemarks().empty )
* - comments: $column.getRemarks()
#end
#if ( $column.getOriginalDefaultValue() )
* - default value: '$column.getOriginalDefaultValue()'
#end
#if ($column.isAutoincrement())
* - AUTO_INCREMENT
#end
#if ($column.isNotNull())
* - NOT NULL
#end
* - column size: $column.getSize()
* - JDBC type returned by the driver: $column.getJavaTypeAsTypeName()
*
*
* @return the value of $column.getVarName()
*/
#if(!$column.isDate() && !$column.isFloat())#swiftThriftField( $fieldIndex $null)#end
public $column.getJavaType() ${column.getGetMethod()}(){
return $column.getVarName();
}
#if($swiftBeanSupport && $column.isDate())
/**
* use Long to represent date type for thrift:swift support
* @see #${column.getGetMethod()}()
*/
@ThriftField(name = "$column.getVarName()",value = $fieldIndex)
public Long ${column.getReadMethod()}(){
return null == $column.getVarName() ? null:${column.getVarName()}.getTime();
}
#end
#if($swiftBeanSupport && $column.isFloat())
/**
* use Double to represent Float type for thrift:swift support
* @see #${column.getGetMethod()}()
*/
@ThriftField(name = "$column.getVarName()",value = $fieldIndex)
public Double ${column.getReadMethod()}(){
return null == $column.getVarName() ? null:${column.getVarName()}.doubleValue();
}
#end
/**
* Setter method for {@link #$column.getVarName()}.
#if ( $column.useEqualsInSetter() )
* The new value is set only if equals() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
#elseif ($column.hasCompareTo())
* The new value is set only if compareTo() says it is different,
* or if one of either the new value or the current value is null.
* In case the new value is different, it is set and the field is marked as 'modified'.
#else
* Attention, there will be no comparison with current value which
* means calling this method will mark the field as 'modified' in all cases.
#end
*
* @param newVal the new value#if( !$column.isAutoincrement() && $column.isNotNull())( NOT NULL)#end to be assigned to $column.getVarName()
*/
public void $column.getSetMethod()($column.getJavaType() newVal)
{
$column.bitORAssignExpression("modified");
$column.bitORAssignExpression("initialized");
#if ($column.useEqualsInSetter())
if (Objects.equals(newVal, $column.getVarName())) {
return;
}
#elseif ($column.hasCompareTo())
if (equals(newVal, $column.getVarName())) {
return;
}
#end
#if ( $column.hasPrimaryType() && $codewriter.getProperty('bean.compatible_axis2') )
#if($column.getJavaType()=='Integer')
//compatible for axis2
$column.getVarName() = null==newVal||newVal==Integer.MIN_VALUE?null:newVal;
#elseif($column.getJavaType()=='Long')
//compatible for axis2
$column.getVarName() = null==newVal||newVal==Long.MIN_VALUE?null:newVal;
#elseif($column.getJavaType()=='Byte')
//compatible for axis2
$column.getVarName() = null==newVal||newVal==Byte.MIN_VALUE?null:newVal;
#elseif($column.getJavaType()=='Double')
//compatible for axis2
$column.getVarName() = null==newVal||newVal==Double.MIN_VALUE?null:newVal;
#elseif($column.getJavaType()=='Float')
//compatible for axis2
$column.getVarName() = null==newVal||newVal==Float.MIN_VALUE?null:newVal;
#elseif($column.getJavaType()=='Character')
//compatible for axis2
$column.getVarName() = null==newVal||newVal==Character.MIN_VALUE?null:newVal;
#elseif($column.getJavaType()=='Short')
//compatible for axis2
$column.getVarName() = null==newVal||newVal==Short.MIN_VALUE?null:newVal;
#elseif($column.getJavaType()=='Boolean')
//compatible for axis2
$column.getVarName() = null==newVal||newVal==Boolean.FALSE?null:newVal;
#else
$column.getVarName() = newVal;
#end
#else
$column.getVarName() = newVal;
#end###if ( $column.hasPrimaryType() && $codewriter.getProperty('bean.compatible_axis2') )
}
################ SETTER METHOD FOR SWIFT ###############
#if($swiftBeanSupport)
/**
* setter for thrift:swift support
* without modification for {@link ${esc.hash}modified} and {@link ${esc.hash}initialized}
* NOTE:DO NOT use the method in your code
*/
#if($column.isDate())
@ThriftField(name = "$column.getVarName()")
public void ${column.getWriteMethod()}(Long newVal){
$column.getVarName() = null == newVal?null:new ${column.getJavaType()}(newVal);
}
#elseif($column.isFloat())
@ThriftField(name = "$column.getVarName()")
public void ${column.getWriteMethod()}(Double newVal){
$column.getVarName() = null == newVal?null:newVal.floatValue();
}
#else
@ThriftField(name = "$column.getVarName()")
public void $column.getWriteMethod()($column.getJavaType() newVal){
$column.getVarName() = newVal;
}
#end
#end###if($swiftBeanSupport)
#if ( $column.hasPrimaryType() )
#if($primitiveSetterSupport)
/**
* Setter method for {@link #$column.getVarName()}.
* Convenient for those who do not want to deal with Objects for primary types.
*
* @param newVal the new value to be assigned to $column.getVarName()
*/
public void $column.getSetMethod()($column.getJavaPrimaryType() newVal)
{
$column.getSetMethod()(new $column.getJavaType()(newVal));
}
#end
#if($column.isDate() && $dateLongSetterSupport)
/**
* Setter method for {@link ${esc.hash}$column.getVarName()}.
* @param newVal the number of milliseconds since January 1, 1970, 00:00:00 GMT represented by this Date object.
*/
public void $column.getSetMethod()(Long newVal)
{
$column.getSetMethod()(null == newVal ? null : new $column.getJavaType()(newVal));
}
#end
#end
/**
* Determines if the $column.getVarName() has been modified.
*
* @return true if the field has been modified, false if the field has not been modified
*/
public boolean ${column.getModifiedMethod()}()
{
return 0 != ${column.bitAndExpression("modified")};
}
/**
* Determines if the $column.getVarName() has been initialized.
*
* It is useful to determine if a field is null on purpose or just because it has not been initialized.
*
* @return true if the field has been initialized, false otherwise
*/
public boolean ${column.getInitializedMethod()}()
{
return 0 != ${column.bitAndExpression("initialized")};
}
#end
@Override
public boolean isModified()
{
#if( $table.stateVarTypeIsArray() )
for( ${codewriter.bitStateClassName} m: modified )if( 0 != m )return true;
return false;
#else
return 0 != modified;
#end
}
@Override
public boolean isModified(int columnID){
#if($table.countColumns()>32)
return columnID>=0 && columnID < metaData.columnCount && 0 != (modified[columnID/32] & (1 << (columnID%32)));
#else
return columnID>=0 && columnID < metaData.columnCount && 0 != (modified & (1 << columnID));
#end##($table.countColumns()>32)
}
@Override
public boolean isInitialized(int columnID){
#if($table.countColumns()>32)
return columnID>=0 && columnID < metaData.columnCount && 0 != (initialized[columnID/32] & (1 << (columnID%32)));
#else
return columnID>=0 && columnID < metaData.columnCount && 0 != (initialized & (1 << columnID));
#end##($table.countColumns()>32)
}
@Override
public void resetIsModified()
{
#if( $table.stateVarTypeIsArray() )
java.util.Arrays.fill(modified,0$!{codewriter.bitStateConstSuffix});
#else
modified = 0$!{codewriter.bitStateConstSuffix};
#end
}
@Override
public void resetPrimaryKeysModified()
{
$table.bitResetAssignExpression($primaryKeys,"modified",' ');
}
/**
* Resets columns modification status except primary keys to 'not modified'.
*/
public void resetModifiedExceptPrimaryKeys()
{
$table.bitResetAssignExpression($table.getColumnsExceptPrimary(),"modified",' ');
}
/** reset all fields to initial value, equal to a new bean */
public void reset(){
#foreach($column in $columns)
#if($column.originalDefaultValue)
### Default value
$!{column.commentOfDefaultValue()}
#end
this.$column.getVarName()${column.getDefaultValueAssignment(true)};
#end
this.isNew = true;
this.modified = $table.maskInitializeWithZero();
this.initialized = $table.maskInitializeWithDefaultValue();
}
@Override
public $beanClass clone(){
return ($beanClass) super.clone();
}
################## NULL BEAN ###########
#if($table.stateVarTypeIsArray())
private static final boolean isAllZero(${codewriter.bitStateClassName}[] array){
for(${codewriter.bitStateClassName} e : array)if(0$!{codewriter.bitStateConstSuffix} != e)return false;
return true;
}
#end
############################################
public static final Builder builder(){
return new Builder().reset();
}
/**
* a builder for $beanClass,the template instance is thread local variable
* a instance of Builder can be reused.
*/
public static final class Builder{
/** $beanClass instance used for template to create new $beanClass instance. */
static final ThreadLocal<$beanClass> TEMPLATE = new ThreadLocal<$beanClass>(){
@Override
protected $beanClass initialValue() {
return new ${beanClass}();
}};
private Builder() {}
/**
* reset the bean as template
* @see ${beanClass}${esc.hash}reset()
*/
public Builder reset(){
TEMPLATE.get().reset();
return this;
}
/** set a bean as template,must not be {@code null} */
public Builder template($beanClass bean){
if(null == bean){
throw new NullPointerException();
}
TEMPLATE.set(bean);
return this;
}
/** return a clone instance of {@link ${esc.hash}TEMPLATE}*/
public $beanClass build(){
return TEMPLATE.get().clone();
}
#foreach($column in $columns)
/**
* fill the field : $column.fullName
* @param $column.varName $!{column.remarks}
* @see $beanClass${esc.hash}${column.getGetMethod()}()
* @see $beanClass${esc.hash}${column.getSetMethod()}($column.javaType)
*/
public Builder ${column.varName}($column.javaType $column.varName){
TEMPLATE.get().${column.getSetMethod()}($column.varName);
return this;
}
#end
}
####### FOR EXTENSION ##############
#parse( $codewriter.getProperty("template.extension.bean"))
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy