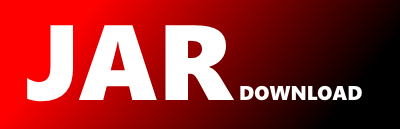
gu.sql2java.manager.cache.CacheManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sql2java-manager Show documentation
Show all versions of sql2java-manager Show documentation
sql2java manager class package for accessing database
The newest version!
package gu.sql2java.manager.cache;
import java.sql.ResultSet;
import java.util.Map;
import java.util.concurrent.TimeUnit;
import gu.sql2java.BaseBean;
import gu.sql2java.Constant;
import gu.sql2java.ListenerContainer;
import gu.sql2java.TableListener;
import gu.sql2java.TableManager;
import gu.sql2java.exception.DaoException;
import gu.sql2java.exception.ObjectRetrievalException;
import gu.sql2java.exception.RuntimeDaoException;
import gu.sql2java.manager.BaseTableManager;
import gu.sql2java.manager.TableManagerDecorator;
/**
* cache implementation for BaseTableManager
* @author guyadong
*/
public class CacheManager extends BaseTableManager
{
/** instance of {@link TableCache} */
private final TableCache cache;
CacheManager(String tablename,UpdateStrategy updateStrategy,Long maximumSize, Long duration, TimeUnit unit) {
super(tablename);
cache = new TableCache<>(metaData,updateStrategy,maximumSize,duration,unit);
cache.registerListener();
}
CacheManager(String tablename){
this(tablename, null, null, null, null);
}
/**
* create a instance of DeviceCacheManager
* @param tablename
* @param updateStrategy cache update strategy,{@link Constant#DEFAULT_STRATEGY} be used if {@code null}
* @param maximumSize maximum capacity of cache ,{@link Constant#DEFAULT_CACHE_MAXIMUMSIZE } be used if {@code null} or <=0,see also {@link com.google.common.cache.CacheBuilder#maximumSize(long)}
* @param duration cache data expired time,{@link Constant#DEFAULT_DURATION} be used if {@code null} or <=0,see also {@link com.google.common.cache.CacheBuilder#expireAfterAccess(long, TimeUnit)}
* @param unit time unit for {@code duration},{@link Constant#DEFAULT_TIME_UNIT} be used if {@code null},see also {@link com.google.common.cache.CacheBuilder#expireAfterAccess(long, TimeUnit)}
*/
public static synchronized final TableManager> makeCacheInstance(
String tablename,
UpdateStrategy updateStrategy,
long maximumSize,
long duration,
TimeUnit unit){
CacheManager manager = new CacheManager(tablename,updateStrategy,maximumSize,duration,unit);
return TableManagerDecorator.makeInterfaceInstance(manager);
}
@Override
protected BaseBean doLoadByPrimaryKeyChecked(Object ...keys)throws RuntimeDaoException,ObjectRetrievalException{
return cache.getBean(keys);
}
@Override
protected boolean doExistsPrimaryKey(Object ...keys)throws RuntimeDaoException{
return null != cache.getBeanUnchecked(keys);
}
@Override
protected int actionOnResultSet(ResultSet rs, int[] fieldList, int startRow, int numRows, Action action) throws DaoException{
if(null == fieldList || fieldList.length == 0){
action = cache.wrap(action);
}
return super.actionOnResultSet(rs, fieldList, startRow, numRows, action);
}
@Override
protected BaseBean doLoadUniqueByIndex(String indexName,Object ...indexValues)throws RuntimeDaoException{
return cache.getBeanByIndexUnchecked(indexName, indexValues);
}
@Override
protected BaseBean doLoadUniqueByIndexChecked(String indexName,Object ...indexValues)throws ObjectRetrievalException{
return cache.getBeanByIndex(indexName, indexValues);
}
@Override
public Map> getForeignKeyDeleteListeners(){
return cache.getManager().getForeignKeyDeleteListeners();
}
@Override
public ListenerContainer getListenerContainer() {
return cache.getManager().getListenerContainer();
}
/**
* 删除cache中主键(keys)指定的记录
* @param primaryKeys 主键值
* @since 3.30.0
*/
public void removeCached(Object... primaryKeys) {
cache.removeCached(primaryKeys);
}
/**
* 根据索引名删除缓存中索引值(keys)指定的记录
* @param indexName 索引名
* @param indexKeys 索引对象的字段值
* @since 3.30.0
*/
public void removeCachedByIndex(String indexName,Object... indexKeys) {
cache.removeCachedByIndex(indexName, indexKeys);
}
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
builder.append("CacheManager [cache=");
builder.append(cache);
builder.append("]");
return builder.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy