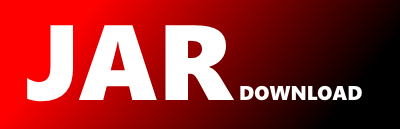
gu.sql2java.redis.cache.BaseBeanSupport Maven / Gradle / Ivy
package gu.sql2java.redis.cache;
import static com.google.common.base.Preconditions.checkNotNull;
import static com.gitee.l0km.com4j.basex.CaseSupport.toCamelcase;
import static com.google.common.base.Preconditions.checkArgument;
import java.lang.reflect.Field;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
import com.google.common.base.Throwables;
import gu.sql2java.BaseBean;
import gu.sql2java.RowMetaData;
public class BaseBeanSupport {
protected BaseBeanSupport() {
}
/**
* 返回{@code jsonFieldID}指定字段的JSON对象
* @param bean
* @param jsonFieldID
* @return
*/
private static JSON readJsonValue(B bean,int jsonFieldID){
RowMetaData metaData = RowMetaData.getMetaData(bean.tableName());
try {
String columnName = checkNotNull(metaData.columnNameOf(jsonFieldID),"INVALID jsonFieldID %s",jsonFieldID);
/** 驼峰命名法的成员名 */
String fieldName=toCamelcase(columnName);
Field field=bean.getClass().getDeclaredField(fieldName);
if(String.class.equals(field.getType())){
return JSON.parseObject((String)bean.getValue(jsonFieldID), JSON.class);
}else if (JSON.class.isAssignableFrom(field.getType())) {
/** 生成驼峰命名格式的read方法名 */
String readMethodName = toCamelcase("read_" +columnName);
/** 调用read方法返回JSON对象 */
return (JSON) bean.getClass().getMethod(readMethodName).invoke(bean);
}else{
throw new RuntimeException(String.format("%s is not a JSON field",columnName));
}
} catch (Exception e) {
Throwables.throwIfUnchecked(e);
throw new RuntimeException(e);
}
}
/**
* 将JSON对象写入{@code jsonFieldID}指定的JSON字段
* @param bean
* @param jsonFieldID
* @param value
*/
private static void writeJsonValue(B bean,int jsonFieldID,JSON value){
RowMetaData metaData = RowMetaData.getMetaData(bean.tableName());
try {
String columnName = checkNotNull(metaData.columnNameOf(jsonFieldID),"INVALID jsonFieldID %s",jsonFieldID);
/** 驼峰命名法的成员名 */
String fieldName=toCamelcase(columnName);
Field field=bean.getClass().getDeclaredField(fieldName);
if(String.class.equals(field.getType())){
bean.setValue(jsonFieldID,JSON.toJSONString(value));
}else if (JSON.class.isAssignableFrom(field.getType())) {
/** 生成驼峰命名格式的write方法名 */
String writeMethodName = toCamelcase("write_" +columnName);
/** 调用write方法返回JSON对象 */
bean.getClass().getMethod(writeMethodName,field.getType()).invoke(bean,value);
}else{
throw new RuntimeException(String.format("%s is not a JSON field",columnName));
}
} catch (Exception e) {
Throwables.throwIfUnchecked(e);
throw new RuntimeException(e);
}
}
/**
* 更新bean的 {@code jsonFieldID}指定字段(JSON)
* @param bean
* @param jsonFieldID 字段ID
* @param input
* @return bean always
*/
public static B updateJsonField(B bean,int jsonFieldID,JSON input){
if( null != bean && input != null){
JSON dst;
if((dst = readJsonValue(bean,jsonFieldID)) != null){
if(dst instanceof JSONObject && input instanceof JSONObject){
((JSONObject)dst).fluentPutAll((JSONObject)input);
}else if(dst instanceof JSONArray && input instanceof JSONArray){
((JSONArray)dst).addAll((JSONArray)input);
}else {
throw new UnsupportedOperationException(String.format("type of input must be same with column %s",jsonFieldID));
}
}else{
dst = input;
}
writeJsonValue(bean,jsonFieldID,dst);
}
return bean;
}
/**
* 更新bean的 {@code jsonFieldID}指定字段(JSON)
* @param bean
* @param jsonFieldID 字段ID
* @param jsonProps 包含多个字段的JSON字符串
* @return bean always
*/
public static B updateJsonField(B bean,int jsonFieldID,String jsonProps){
if( null != bean && jsonProps != null){
JSON input = JSON.parseObject(jsonProps, JSON.class);
updateJsonField(bean, jsonFieldID, input);
}
return bean;
}
/**
* 更新bean的 {@code jsonFieldID}指定字段(JSON)
* @param bean
* @param jsonFieldID 字段ID
* @param key JSON字段名
* @param value JSON字段值
* @return bean always
*/
public static B updateJsonField(B bean,int jsonFieldID,String key,Object value){
if( null !=bean && key != null){
JSONObject dst = (JSONObject) readJsonValue(bean, jsonFieldID);
if(dst != null){
checkArgument(dst instanceof JSONObject,"type of column %s is not JSONObject",jsonFieldID);
if(null == value ){
dst.fluentRemove(key);
}else{
dst.fluentPut(key, value);
}
writeJsonValue(bean, jsonFieldID, dst);
}else if(null != value){
writeJsonValue(bean, jsonFieldID, new JSONObject().fluentPut(key,value));
}
}
return bean;
}
/**
* 返回JSON中指定字段的值,不存在则返回{@code null}
* @param bean
* @param jsonFieldID 保存JSON数据的字段ID
* @param key
*/
public static Object getFieldOfJson(B bean,int jsonFieldID,String key){
if(bean != null && key != null){
JSONObject json = (JSONObject) readJsonValue(bean, jsonFieldID);
return null != json ? json.get(key) : null;
}
return null;
}
/**
* 返回{@link BaseBean}对象中字段ID({@code jsonFieldID})指定的字段解析的JSON对象,
* 如果输入参数为{@code null}或字段为空或{@code null}返回空的{@link JSONObject}对象,
* JSON解析失败则抛出异常
* @param bean
* @param jsonFieldID 字段ID
*/
public static JSON asJson(B bean,int jsonFieldID){
if(null != bean){
return readJsonValue(bean, jsonFieldID);
}
return new JSONObject();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy