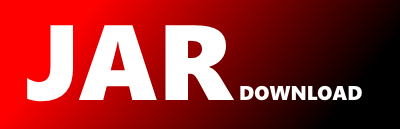
gu.sql2java.store.BaseURLStore Maven / Gradle / Ivy
package gu.sql2java.store;
import static gu.sql2java.store.BinaryUtils.*;
import static gu.sql2java.store.URLInfo.wrap;
import java.io.IOException;
import java.net.URL;
import java.net.URLStreamHandler;
import java.net.URLStreamHandlerFactory;
import java.util.Collections;
import java.util.HashMap;
import java.util.Hashtable;
import java.util.Map;
import ref.org.apache.commons.jnet.Installer;
/**
* 基于{@link URLStreamHandler}实现二进制存储接口{@link URLStore}的抽象类
* 实现{@link URLStreamHandlerFactory}接口,应用启动时应调用{@link #intall()}将当前实例安装到JVM
* @author guyadong
*
*/
public abstract class BaseURLStore implements URLStreamHandlerFactory, URLStore {
private volatile boolean installed = false;
private final Map> optionalParamTypes = new Hashtable<>();
protected final ThreadLocal
© 2015 - 2025 Weber Informatics LLC | Privacy Policy