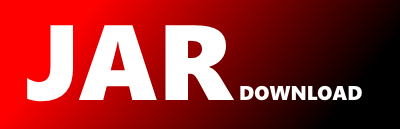
cn.cliveyuan.robin.base.BaseServiceImpl Maven / Gradle / Ivy
/*
* Copyright (c) 2020 Clive Yuan ([email protected]).
*/
package cn.cliveyuan.robin.base;
import cn.cliveyuan.robin.base.common.Pagination;
import cn.cliveyuan.robin.base.condition.Criteria;
import cn.cliveyuan.robin.base.condition.Example;
import cn.cliveyuan.robin.base.condition.GeneratedCriteria;
import cn.cliveyuan.robin.base.condition.LambdaCriteria;
import cn.cliveyuan.robin.base.condition.PageQueryExample;
import cn.cliveyuan.robin.base.condition.Query;
import cn.cliveyuan.robin.base.util.ReflectUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.util.Assert;
import java.util.List;
import java.util.Map;
import java.util.Objects;
/**
* 基础 Service 实现类
*
* @author Clive Yuan
* @date 2020/10/29
*/
@SuppressWarnings({"serial", "unchecked"})
public class BaseServiceImpl implements BaseService {
@Autowired
private BaseMapper baseMapper;
@Override
public int insert(T entity) {
this.checkEntity(entity);
return baseMapper.insert(entity);
}
@Override
public int insertAll(T entity) {
this.checkEntity(entity);
return baseMapper.insertAll(entity);
}
@Override
public int insertIgnore(T entity) {
this.checkEntity(entity);
return baseMapper.insertIgnore(entity);
}
@Override
public int save(T entity) {
this.checkEntity(entity);
return ReflectUtils.isIdNull(entity) ? this.insert(entity) : this.update(entity);
}
@Override
public int saveAll(T entity) {
this.checkEntity(entity);
return ReflectUtils.isIdNull(entity) ? this.insertAll(entity) : this.updateAll(entity);
}
@Override
public int batchInsert(List list) {
this.checkEntityList(list);
return baseMapper.batchInsert(list);
}
@Override
public int batchInsertIgnore(List list) {
this.checkEntityList(list);
return baseMapper.batchInsertIgnore(list);
}
@Override
public int delete(Long id) {
this.checkId(id);
return baseMapper.delete(id);
}
@Override
public int batchDelete(List ids) {
this.checkIdList(ids);
return baseMapper.batchDelete(ids);
}
@Override
public int deleteByExample(Query query) {
this.checkQuery(query);
return baseMapper.deleteByExample(this.convertQueryToExample(query));
}
@Override
public int update(T entity) {
this.checkEntity(entity);
return baseMapper.update(entity);
}
@Override
public int updateAll(T entity) {
this.checkEntity(entity);
return baseMapper.updateAll(entity);
}
@Override
public int updateByExample(T entity, Query query) {
this.checkEntity(entity);
return baseMapper.updateByExample(entity, this.convertQueryToExample(query));
}
@Override
public int updateByExampleAll(T entity, Query query) {
this.checkEntity(entity);
return baseMapper.updateByExampleAll(entity, this.convertQueryToExample(query));
}
@Override
public T get(Long id) {
this.checkId(id);
return baseMapper.get(id);
}
@Override
public List batchGet(List ids) {
this.checkIdList(ids);
return baseMapper.batchGet(ids);
}
@Override
public T getByExample(Query query) {
this.checkQuery(query);
return baseMapper.getByExample(this.convertQueryToExample(query));
}
@Override
public int count(Query query) {
this.checkQuery(query);
return baseMapper.count(this.convertQueryToExample(query));
}
@Override
public List list(Query query) {
this.checkQuery(query);
return baseMapper.list(this.convertQueryToExample(query));
}
@Override
public Pagination page(PageQueryExample query) {
this.checkQuery(query);
Example example = this.convertQueryToExample(query);
int page = this.getPage(query.getPageNo());
int rowsPerPage = this.getRowsPerPage(query.getPageSize());
example.setLimitStart(this.getOffset(page, rowsPerPage));
example.setLimitEnd(rowsPerPage);
int totalCount = baseMapper.count(example);
if (totalCount == 0) {
return Pagination.buildEmpty();
}
return new Pagination<>(totalCount, baseMapper.list(example), page, rowsPerPage);
}
private Class> getEntityClass() {
return ReflectUtils.getSuperClassGenericType(getClass(), 0);
}
private Example convertQueryToExample(Query query) {
T entity = query.getEntity();
if (Objects.nonNull(entity)) {
Map fieldAndValue = ReflectUtils.resolveEntityFieldAndValue(entity);
GeneratedCriteria criteria = query.getExistCriteria();
if (criteria instanceof LambdaCriteria) {
LambdaCriteria lambdaCriteria = (LambdaCriteria) criteria;
fieldAndValue.forEach(lambdaCriteria::eq);
} else if (criteria instanceof Criteria) {
fieldAndValue.forEach(criteria::eq);
}
}
return (Example) query;
}
private void checkId(Long id) {
Assert.notNull(id, "id can't be null");
}
private void checkEntity(T entity) {
Assert.notNull(entity, "entity can't be null");
}
private void checkQuery(Query query) {
Assert.notNull(query, "query can't be null");
}
private void checkIdList(List idList) {
Assert.isTrue(Objects.nonNull(idList) && idList.size() > 0, "ids can't be empty");
}
private void checkEntityList(List list) {
Assert.isTrue(Objects.nonNull(list) && list.size() > 0, "list can't be empty");
}
private int getPage(Integer page) {
if (Objects.isNull(page) || page <= 0) {
page = PageQueryExample.DEFAULT_PAGE_NO;
}
return page;
}
private int getRowsPerPage(Integer rowsPerPage) {
if (Objects.isNull(rowsPerPage) || rowsPerPage <= 0) {
rowsPerPage = PageQueryExample.DEFAULT_PAGE_SIZE;
}
return rowsPerPage;
}
private int getOffset(int page, int rowsPerPage) {
return (page - 1) * rowsPerPage;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy