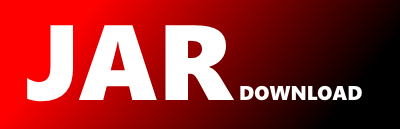
defaults.tpl.service.impl.executor.tpl Maven / Gradle / Ivy
The newest version!
package #{java.vars.current.packages};
${item}
/**
* #{table.comment}业务处理类
*
* @author zhh
* @version
*/
${item}
@Transactional(propagation = Propagation.REQUIRED, rollbackFor = Throwable.class)
public class #{java.vars.current.capitalizeName} implements #{java.vars.service.api.executor.capitalizeName} {
/** 日志对象 **/
private static final Logger log = LoggerFactory.getLogger(#{table.capitalizeName}Executor.class);
@Autowired
private #{java.vars.service.basic.capitalizeName} #{java.vars.service.basic.normativeName};
@Override
public void create(#{java.vars.model.bean.capitalizeName} model, IAccount me) throws ServiceException {
String msg = "Failed to create #{table.capitalizeName}. ";
if (#{VerifyTools.capitalizeName}.isBlank(me)) {
log.error(msg + "params is null: me");
throw new ServiceException(ResultCode.PARAMETER_IS_REQUIRED);
}
if (#{VerifyTools.capitalizeName}.isBlank(model)) {
log.error(msg + "params is null: model");
throw new ServiceException(ResultCode.PARAMETER_IS_REQUIRED);
}
// TODO: 参数验证
// if (#{VerifyTools.capitalizeName}.isBlank(model.getXxx())) {
// log.error(msg + "params is null: xxx");
// throw new ServiceException(ResultCode.PARAMETER_IS_REQUIRED);
// }
// 业务逻辑
model.set#{table.primaryKey.capitalizeName}(null); // 主键由Basic生成, 不允许外部传入
// TODO: 补充其他业务逻辑
// 设置创建人信息
// model.setCreatorId(me.getUserId()); // 创建人ID
// model.setCreatorName(me.getDisplayName()); // 创建人姓名
// 向#{table.originalName}表插入记录
#{java.vars.service.basic.normativeName}.create(model);
throw new UnsupportedOperationException("该方法的业务逻辑暂未实现"); // TODO 完成业务逻辑的实现之后删除此行
}
@Override
public void update(#{java.vars.model.update.capitalizeName} model, IAccount me) throws ServiceException {
String msg = "Failed to update #{table.capitalizeName}. ";
if (#{VerifyTools.capitalizeName}.isBlank(me)) {
log.error(msg + "params is null: me");
throw new ServiceException(ResultCode.PARAMETER_IS_REQUIRED);
}
if (#{VerifyTools.capitalizeName}.isBlank(model)) {
log.error(msg + "params is null: model");
throw new ServiceException(ResultCode.PARAMETER_IS_REQUIRED);
}
// TODO: 参数验证
// if (#{VerifyTools.capitalizeName}.isBlank(model.getXxx())) {
// log.error(msg + "params is null: Xxx");
// throw new ServiceException(ResultCode.PARAMETER_IS_REQUIRED);
// }
#{table.primaryKey.dataType.typedName} #{table.primaryKey.normativeName} = model.get#{table.primaryKey.capitalizeName}();
if (#{VerifyTools.capitalizeName}.isBlank(#{table.primaryKey.normativeName}) && model.getWhere() != null) {
#{table.primaryKey.normativeName} = model.getWhere().get#{table.primaryKey.capitalizeName}();
}
if (#{VerifyTools.capitalizeName}.isBlank(#{table.primaryKey.normativeName})) {
log.error(msg + "params is null: #{table.primaryKey.normativeName}");
throw new ServiceException(ResultCode.PARAMETER_IS_REQUIRED);
}
// 原始记录检查
#{java.vars.model.bean.capitalizeName} older = #{java.vars.service.basic.normativeName}.findBy#{table.primaryKey.shortName}(#{table.primaryKey.normativeName});
if (older == null) {
log.error(msg + "record is not found. #{table.primaryKey.normativeName}=" + #{table.primaryKey.normativeName});
throw new ServiceException(ResultCode.RECORD_NOT_EXIST);
}
#{table.primaryKey.dataType.typedName} #{table.primaryKey.normativeName} = model.get#{table.primaryKey.capitalizeName}();
if (#{VerifyTools.capitalizeName}.isBlank(#{table.primaryKey.normativeName}) && model.getWhere() != null) {
#{table.primaryKey.normativeName} = model.getWhere().get#{table.primaryKey.capitalizeName}();
}
#{table.businessKey.dataType.typedName} #{table.businessKey.normativeName} = model.get#{table.businessKey.capitalizeName}();
if (#{VerifyTools.capitalizeName}.isBlank(#{table.businessKey.normativeName}) && model.getWhere() != null) {
#{table.businessKey.normativeName} = model.getWhere().get#{table.businessKey.capitalizeName}();
}
if (#{VerifyTools.capitalizeName}.isAllBlank(#{table.primaryKey.normativeName}, #{table.businessKey.normativeName})) {
log.error(msg + "#{table.primaryKey.normativeName} or #{table.businessKey.normativeName} is required.");
throw new ServiceException(ResultCode.PARAMETER_IS_REQUIRED);
}
// 原始记录检查
#{java.vars.model.bean.capitalizeName} older;
if (#{VerifyTools.capitalizeName}.isNotBlank(#{table.primaryKey.normativeName})) {
older = #{java.vars.service.basic.normativeName}.findBy#{table.primaryKey.shortName}(#{table.primaryKey.normativeName});
if (older == null) {
log.error(msg + "record is not found. #{table.primaryKey.normativeName}=" + #{table.primaryKey.normativeName});
throw new ServiceException(ResultCode.RECORD_NOT_EXIST);
}
} else {
older = #{java.vars.service.basic.normativeName}.findBy#{table.businessKey.shortName}(#{table.businessKey.normativeName});
if (older == null) {
log.error(msg + "record is not found. #{table.businessKey.normativeName}=" + #{table.businessKey.normativeName});
throw new ServiceException(ResultCode.RECORD_NOT_EXIST);
}
}
// TODO: 业务逻辑
// 判断哪些状态的记录不允许修改...
// if (#{VerifyTools.capitalizeName}.isExists(older.getState(), State.DISABLED, State.Xxx)) {
// throw new ServiceException(ResultCode.RECORD_STATE_ERROR);
// }
#{java.vars.model.update.capitalizeName} newer = createChangedModel(model, older);
if (newer != null) {
更新时间不能在SQL中直接修改,例如用户购买商品后修改商品的销量,更新时间应保持不变
newer.set${field.capitalizeName}(new Date()); // ${field.comment}
// 更新#{table.originalName}表的记录
#{java.vars.service.basic.normativeName}.update(newer, false);
}
throw new UnsupportedOperationException("该方法的业务逻辑暂未实现"); // TODO 完成业务逻辑的实现之后删除此行
}
@Override
public void updateState(#{table.primaryKey.dataType.typedName} #{table.primaryKey.normativeName}, #{table.stateField.dataType.typedName} #{table.stateField.normativeName}, IAccount me) throws ServiceException {
String msg = "Failed to update state for #{table.capitalizeName}. ";
if (#{VerifyTools.capitalizeName}.isBlank(me)) {
log.error(msg + "params is null: me");
throw new ServiceException(ResultCode.PARAMETER_IS_REQUIRED);
}
if (#{VerifyTools.capitalizeName}.isBlank(#{table.primaryKey.normativeName})) {
log.error(msg + "params is null: #{table.primaryKey.normativeName}");
throw new ServiceException(ResultCode.PARAMETER_IS_REQUIRED);
}
if (#{VerifyTools.capitalizeName}.isBlank(#{table.stateField.normativeName})) {
log.error(msg + "params is null: #{table.stateField.normativeName}");
throw new ServiceException(ResultCode.PARAMETER_IS_REQUIRED);
}
// TODO: 参数验证
// if (#{VerifyTools.capitalizeName}.isBlank(model.getXxx())) {
// log.error(msg + "params is null: Xxx");
// throw new ServiceException(ResultCode.PARAMETER_IS_REQUIRED);
// }
// 原始记录检查
#{java.vars.model.bean.capitalizeName} older = #{java.vars.service.basic.normativeName}.findBy#{table.primaryKey.shortName}(#{table.primaryKey.normativeName});
if (older == null) {
log.error(msg + "record is not found. #{table.primaryKey.normativeName}=" + #{table.primaryKey.normativeName});
throw new ServiceException(ResultCode.RECORD_NOT_EXIST);
}
// TODO: 业务逻辑
// 判断哪些状态的记录不允许修改...
// if (#{VerifyTools.capitalizeName}.isExists(older.getState(), State.DISABLED, State.Xxx)) {
// throw new ServiceException(ResultCode.RECORD_STATE_ERROR);
// }
#{java.vars.model.update.capitalizeName} newer = new #{java.vars.model.update.capitalizeName}();
newer.set#{table.stateField.capitalizeName}(#{table.stateField.normativeName});
更新时间不能在SQL中直接修改,例如用户购买商品后修改商品的销量,更新时间应保持不变
newer.set${field.capitalizeName}(new Date()); // ${field.comment}
#{java.vars.model.where.capitalizeName} where = new #{java.vars.model.where.capitalizeName}();
where.set#{table.primaryKey.capitalizeName}(#{table.primaryKey.normativeName});
where.set#{table.stateField.capitalizeName}(older.get#{table.stateField.capitalizeName}());
newer.setWhere(where);
// 更新#{table.originalName}表的记录
#{java.vars.service.basic.normativeName}.update(newer, false);
throw new UnsupportedOperationException("该方法的业务逻辑暂未实现"); // TODO 完成业务逻辑的实现之后删除此行
}
@Override
public void delete(#{table.primaryKey.dataType.typedName} #{table.primaryKey.normativeName}, IAccount me) throws ServiceException {
String msg = "Failed to delete #{table.capitalizeName}. ";
if (#{VerifyTools.capitalizeName}.isBlank(me)) {
log.error(msg + "params is null: me");
throw new ServiceException(ResultCode.PARAMETER_IS_REQUIRED);
}
if (#{VerifyTools.capitalizeName}.isBlank(#{table.primaryKey.normativeName})) {
log.error(msg + "params is null: #{table.primaryKey.normativeName}");
throw new ServiceException(ResultCode.PARAMETER_IS_REQUIRED);
}
// 从#{table.originalName}表查询原记录
#{java.vars.model.bean.capitalizeName} older = #{java.vars.service.basic.normativeName}.findBy#{table.primaryKey.shortName}(#{table.primaryKey.normativeName});
if (older == null) {
log.error(msg + "record is not found.\n\t#{table.primaryKey.normativeName} is " + #{table.primaryKey.normativeName});
throw new ServiceException(ResultCode.RECORD_NOT_EXIST);
}
// TODO: 业务逻辑
// 判断哪些状态的记录不允许删除...
// if (#{VerifyTools.capitalizeName}.isExists(older.getState(), State.DISABLED, State.Xxx)) {
// throw new ServiceException(ResultCode.RECORD_STATE_ERROR);
// }
// 删除#{table.originalName}表的记录
#{java.vars.service.basic.normativeName}.deleteBy#{table.primaryKey.listData.shortName}(Arrays.asList(#{table.primaryKey.normativeName}), false);
throw new UnsupportedOperationException("该方法的业务逻辑暂未实现"); // TODO 完成业务逻辑的实现之后删除此行
}
@Override
public void delete(List<#{table.primaryKey.dataType.typedName}> #{table.primaryKey.listData.normativeName}, IAccount me) throws ServiceException {
String msg = "Failed to delete #{table.capitalizeName}. ";
if (#{VerifyTools.capitalizeName}.isBlank(me)) {
log.error(msg + "params is null: me");
throw new ServiceException(ResultCode.PARAMETER_IS_REQUIRED);
}
if (#{VerifyTools.capitalizeName}.isBlank(#{table.primaryKey.listData.normativeName})) {
log.error(msg + "params is null: #{table.primaryKey.listData.normativeName}");
throw new ServiceException(ResultCode.PARAMETER_IS_REQUIRED);
}
// 删除#{table.originalName}表的记录
#{java.vars.service.basic.normativeName}.deleteBy#{table.primaryKey.listData.shortName}(#{table.primaryKey.listData.normativeName}, false);
throw new UnsupportedOperationException("该方法的业务逻辑暂未实现"); // TODO 完成业务逻辑的实现之后删除此行
}
/**
* 创建一个新的对象, 只包含改动过的字段
*
* @author zhh
* @param model 目标对象(一般是参数传入的)
* @param older 源对象(一般是从数据库查询得到的)
* @return 只包含有更新的字段对象
*/
private #{java.vars.model.update.capitalizeName} createChangedModel(#{java.vars.model.update.capitalizeName} model, #{java.vars.model.bean.capitalizeName} older) {
// #{java.vars.model.update.capitalizeName} newer = new #{java.vars.model.update.capitalizeName}();
// newer.setWhere(model.getWhere());
//
// boolean changed = false;
// newer.set#{table.primaryKey.capitalizeName}(model.get#{table.primaryKey.capitalizeName}()); // #{table.primaryKey.comment}
// newer.set#{table.businessKey.capitalizeName}(model.get#{table.businessKey.capitalizeName}()); // #{table.businessKey.comment}
//
// if (#{VerifyTools.capitalizeName}.isChanged(model.get${field.capitalizeName}(), older.get${field.capitalizeName}())) {
// changed = true;
// newer.set${field.capitalizeName}(model.get${field.capitalizeName}()); // ${field.comment}
// }
// if (Boolean.TRUE.equals(model.is${field.capitalizeName}ToNull())) {
// changed = true;
// newer.set${field.capitalizeName}ToNull(true); // ${field.comment}更新为空值
// }
// if (Boolean.TRUE.equals(model.is${field.capitalizeName}ToCurrent())) {
// changed = true;
// newer.set${field.capitalizeName}ToCurrent(true); // ${field.comment}更新为数据库当前时间
// }
// if (#{VerifyTools.capitalizeName}.isNotBlank(model.get${field.capitalizeName}Add()) && model.get${field.capitalizeName}Add() != 0) {
// changed = true;
// newer.set${field.capitalizeName}Add(model.get${field.capitalizeName}Add()); // ${field.comment}的增加值
// }
// if (#{VerifyTools.capitalizeName}.isNotBlank(model.get${field.capitalizeName}Add()) && model.get${field.capitalizeName}Add() != 0) {
// changed = true;
// newer.set${field.capitalizeName}Add(model.get${field.capitalizeName}Add()); // ${field.comment}的增加值
// }
// return changed ? newer : null;
throw new UnsupportedOperationException("请确认#{java.vars.model.update.capitalizeName}哪些字段是允许修改的"); // TODO 完成业务逻辑的实现之后删除此行
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy